shipstation
Module shipstation
API
Definitions
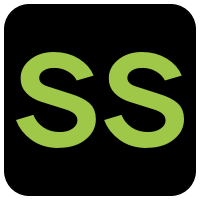
ballerinax/shipstation Ballerina library
Overview
This is a generated connector for ShipStation API v1.0 OpenAPI specification.
ShipStation strives to streamline shipping for online sellers, no matter where they sell their products online.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a ShipStation account
- Obtain tokens by following this guide
Quickstart
To use the ShipStation connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/shipstation
module into the Ballerina project.
import ballerinax/shipstation;
Step 2: Create a new connector instance
Create a shipstation:ClientConfig
with the API key
and API Secret
obtained, and initialize the connector with it.
shipstation:ClientConfig clientConfig = { auth: { username: <API_KEY>, password: <API_SECRET> } }; shipstation:Client baseClient = check new Client(clientConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to list customers using the connector.
public function main() returns error? { shipstation:ListCustomersResponse response = check baseClient->listCustomers(); log:printInfo(response.toString()); }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
shipstation: Client
This is a generated connector for ShipStation API v1.0 OpenAPI specification. ShipStation strives to streamline shipping for online sellers, no matter where they sell their products online.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a ShipStation account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://ssapi.shipstation.com/" - URL of the target service
registerAccount
function registerAccount(string contentType, RegisterAccountrequest payload) returns RegisterAccountresponse|error
Creates a new ShipStation account and generates an apiKey and apiSecret to be used by the newly created account.
listTags
function listTags() returns ListTagsresponse[]|error
Lists all tags defined for this account.
listCarriers
function listCarriers() returns ListCarriersresponse[]|error
Lists all shipping providers connected to this account.
getCarrier
function getCarrier(string carrierCode) returns GetCarrierresponse|error
Retrieves the shipping carrier account details for the specified carrierCode. Use this method to determine a carrier's account balance.
Parameters
- carrierCode string - The code for the carrier account to retrieve.
addFunds
function addFunds(AddFundsrequest payload) returns AddFundsresponse|error
Adds funds to a carrier account using the payment information on file.
Parameters
- payload AddFundsrequest -
listPackages
function listPackages(string carrierCode) returns ListPackagesresponse[]|error
Retrieves a list of packages for the specified carrier
Parameters
- carrierCode string - The carrier's code
listServices
function listServices(string carrierCode) returns ListServicesresponse[]|error
Retrieves the list of available shipping services provided by the specified carrier
Parameters
- carrierCode string - The carrier's code
getCustomer
function getCustomer(int customerId) returns GetCustomerresponse|error
Get Customer
Parameters
- customerId int - The system generated identifier for the Customer.
listCustomers
function listCustomers(string? stateCode, string? countryCode, int? marketplaceId, int? tagId, SortBy? sortBy, SortDir? sortDir, int? page, int? pageSize) returns ListCustomersResponse|error
Obtains a list of customers that match the specified criteria.
Parameters
- stateCode string? (default ()) - Returns customers that reside in the specified stateCode.
- countryCode string? (default ()) - Returns customers that reside in the specified countryCode.
- marketplaceId int? (default ()) - Returns customers that purchased items from the specified marketplaceId.
- tagId int? (default ()) - Returns customers that have been tagged with the specified tagId.
- sortBy SortBy? (default ()) - Sorts the order of the response based off the specified value.
- sortDir SortDir? (default ()) - Sets the direction of the sort order.
- page int? (default ()) - Page number.
- pageSize int? (default ()) - Requested page size. Max value is 500.
listFulfillments
function listFulfillments(int? fulfillmentId, int? orderId, string? orderNumber, string? trackingNumber, string? recipientName, string? createDateStart, string? createDateEnd, string? shipDateStart, string? shipDateEnd, SortBy1? sortBy, SortDir? sortDir, int? page, int? pageSize) returns ListFulfillmentsResponse|error
Obtains a list of fulfillments that match the specified criteria. Please note the following:
- Orders that have been marked as shipped either through the UI or the API will appear in the response as they are considered fulfillments. All of the available filters are optional. They do not need to be included in the URL. If you do include them, here's what the URL may look like: Url format with filters:
fulfillments?fulfillmentId={fulfillmentId} &orderId={orderId} &orderNumber={orderNumber} &trackingNumber={trackingNumber} &recipientName={recipientName} &createDateStart={createDateStart} &createDateEnd={createDateEnd} &shipDateStart={shipDateStart} &shipDateEnd={shipDateEnd} &sortBy={sortBy} &sortDir={sortDir} &page={page} &pageSize={pageSize}
Parameters
- fulfillmentId int? (default ()) - Returns the fulfillment with the specified fulfillment ID.
- orderId int? (default ()) - Returns fulfillments whose orders have the specified order ID.
- orderNumber string? (default ()) - Returns fulfillments whose orders have the specified order number.
- trackingNumber string? (default ()) - Returns fulfillments with the specified tracking number.
- recipientName string? (default ()) - Returns fulfillments shipped to the specified recipient name.
- createDateStart string? (default ()) - Returns fulfillments created on or after the specified
createDate
- createDateEnd string? (default ()) - Returns fulfillments created on or before the specified
createDate
- shipDateStart string? (default ()) - Returns fulfillments with the
shipDate
on or after the specified date
- shipDateEnd string? (default ()) - Returns fulfillments with the
shipDate
on or before the specified date
- sortBy SortBy1? (default ()) - Sort the responses by a set value. The response will be sorted based off the ascending dates (oldest to most current.) If left empty, the response will be sorted by ascending
createDate
.
- sortDir SortDir? (default ()) - Sets the direction of the sort order.
- page int? (default ()) - page number.
- pageSize int? (default ()) - page size.
getOrder
function getOrder(int orderId) returns GetOrderresponse|error
Retrieves a single order from the database.
Parameters
- orderId int - The system generated identifier for the order.
deleteOrder
function deleteOrder(int orderId) returns DeleteOrderresponse|error
Removes order from ShipStation's UI. Note this is a "soft" delete action so the order will still exist in the database, but will be set to inactive
Parameters
- orderId int - The system generated identifier for the order.
addTagToOrder
function addTagToOrder(string contentType, AddTagtoOrderrequest payload) returns AddTagtoOrderresponse|error
Adds a tag to an order
assignUserToOrder
function assignUserToOrder(string contentType, AssignUsertoOrderrequest payload) returns AssignUsertoOrderresponse|error
Assigns a user to an order
createLabelForOrder
function createLabelForOrder(string contentType, CreateLabelforOrderrequest payload) returns CreateLabelforOrderresponse|error
Creates a shipping label for a given order.
createUpdateorder
function createUpdateorder(string contentType, CreateOrUpdateOrderrequest payload) returns CreateOrUpdateOrderresponse|error
Create a new order or update an existing order. If the orderKey is specified, ShipStation will attempt to locate the order with the specified orderKey. If found, the existing order with that key will be updated. If the orderKey is not found, a new order will be created with that orderKey.
createUpdatemultipleorders
function createUpdatemultipleorders(string contentType, CreateOrUpdateMultipleOrdersrequest[] payload) returns CreateOrUpdateMultipleOrdersresponse|error
Create or update multiple orders in one request. If the orderKey is specified, ShipStation will attempt to locate the order with the specified orderKey. If found, the existing order with that key will be updated. If the orderKey is not found, a new order will be created with that orderKey.
holdOrderUntil
function holdOrderUntil(string contentType, HoldOrderUntilrequest payload) returns HoldOrderUntilresponse|error
Change the status of the given order to On Hold until the date specified, when the status will automatically change to Awaiting Shipment.
listOrders
function listOrders(string? customerName, string? itemKeyword, string? createDateStart, string? createDateEnd, string? modifyDateStart, string? modifyDateEnd, string? orderDateStart, string? orderDateEnd, string? orderNumber, OrderStatus? orderStatus, string? paymentDateStart, string? paymentDateEnd, int? storeId, SortBy2? sortBy, SortDir? sortDir, string? page, string? pageSize) returns ListOrdersResponse|error
List orders.
Parameters
- customerName string? (default ()) - Returns orders that match the specified name.
- itemKeyword string? (default ()) - Returns orders that contain items that match the specified keyword. Fields searched are Sku, Description, and Options
- createDateStart string? (default ()) - Returns orders that were created in ShipStation after the specified date
- createDateEnd string? (default ()) - Returns orders that were created in ShipStation before the specified date
- modifyDateStart string? (default ()) - Returns orders that were modified after the specified date
- modifyDateEnd string? (default ()) - Returns orders that were modified before the specified date
- orderDateStart string? (default ()) - Returns orders greater than the specified date
- orderDateEnd string? (default ()) - Returns orders less than or equal to the specified date
- orderNumber string? (default ()) - Filter by order number, performs a "starts with" search.
- orderStatus OrderStatus? (default ()) - Filter by order status. If left empty, orders of all statuses are returned.
- paymentDateStart string? (default ()) - Returns orders that were paid after the specified date
- paymentDateEnd string? (default ()) - Returns orders that were paid before the specified date
- storeId int? (default ()) - Filters orders to a single store. Call List Stores to obtain a list of store Ids.
- sortBy SortBy2? (default ()) - Sort the responses by a set value. The response will be sorted based off the ascending dates (oldest to most current.) If left empty, the response will be sorted by ascending
orderId
.
- sortDir SortDir? (default ()) - Sets the direction of the sort order.
- page string? (default ()) - Page number
- pageSize string? (default ()) - Requested page size. Max value is 500.
listOrdersByTag
function listOrdersByTag(OrderStatus? orderStatus, int? tagId, string? page, string? pageSize) returns ListOrdersbyTagresponse|error
Lists all orders that match the specified status and tag ID
Parameters
- orderStatus OrderStatus? (default ()) - The order's status.
- tagId int? (default ()) - ID of the tag. Call Accounts/ListTags to obtain a list of tags for this account.
- page string? (default ()) - Page number
- pageSize string? (default ()) - Requested page size. Max value is 500.
markAnOrderAsShipped
function markAnOrderAsShipped(string contentType, MarkanOrderasShippedrequest payload) returns MarkanOrderasShippedresponse|error
Marks an order as shipped without creating a label in ShipStation.
removeTagFromOrder
function removeTagFromOrder(string contentType, RemoveTagfromOrderrequest payload) returns RemoveTagfromOrderresponse|error
Removes a tag from the specified order.
restoreOrderFromOnHold
function restoreOrderFromOnHold(string contentType, RestoreOrderfromOnHoldrequest payload) returns RestoreOrderfromOnHoldresponse|error
Change the status of the given order from On Hold to Awaiting Shipment
unassignUserFromOrder
function unassignUserFromOrder(string contentType, UnassignUserfromOrderrequest payload) returns UnassignUserfromOrderresponse|error
Unassigns a user from an order.
getProduct
function getProduct(int productId) returns GetProductresponse|error
Get product
Parameters
- productId int - The system generated identifier for the Product.
updateProduct
function updateProduct(int productId, string contentType, UpdateProductrequest payload) returns UpdateProductresponse|error
Updates an existing product.
Parameters
- productId int - The system generated identifier for the Product.
- contentType string -
- payload UpdateProductrequest -
listProducts
function listProducts(string? sku, string? name, string? productCategoryId, string? productTypeId, string? tagId, string? startDate, string? endDate, SortBy3? sortBy, SortDir? sortDir, string? page, string? pageSize, string? showInactive) returns ListProductsResponse|error
List products.
Parameters
- sku string? (default ()) - Returns products that match the specified SKU.
- name string? (default ()) - Returns products that match the specified product name.
- productCategoryId string? (default ()) - Returns products that match the specified productCategoryId.
- productTypeId string? (default ()) - Returns products that match the specified productTypeId.
- tagId string? (default ()) - Returns products that match the specified tagId.
- startDate string? (default ()) - Returns products that were created after the specified date.
- endDate string? (default ()) - Returns products that were created before the specified date.
- sortBy SortBy3? (default ()) - Sorts the order of the response based off the specified value.
- sortDir SortDir? (default ()) - Sets the direction of the sort order.
- page string? (default ()) - Page number.
- pageSize string? (default ()) - Requested page size. Max value is 500.
- showInactive string? (default ()) - Specifies whether the list should include inactive products.
listShipments
function listShipments(string? recipientName, string? recipientCountryCode, string? orderNumber, int? orderId, string? carrierCode, string? serviceCode, string? trackingNumber, string? createDateStart, string? createDateEnd, string? shipDateStart, string? shipDateEnd, string? voidDateStart, string? voidDateEnd, int? storeId, boolean? includeShipmentItems, SortBy1? sortBy, SortDir? sortDir, int? page, int? pageSize) returns ListShipmentsResponse|error
List shipments.
Parameters
- recipientName string? (default ()) - Returns shipments shipped to the specified recipient name.
- recipientCountryCode string? (default ()) - Returns shipments shipped to the specified country code.
- orderNumber string? (default ()) - Returns shipments whose orders have the specified order number.
- orderId int? (default ()) - Returns shipments whose orders have the specified order ID.
- carrierCode string? (default ()) - Returns shipments shipped with the specified carrier.
- serviceCode string? (default ()) - Returns shipments shipped with the specified shipping service.
- trackingNumber string? (default ()) - Returns shipments with the specified tracking number.
- createDateStart string? (default ()) - Returns shipments created on or after the specified
createDate
- createDateEnd string? (default ()) - Returns shipments created on or before the specified
createDate
- shipDateStart string? (default ()) - Returns shipments with the
shipDate
on or after the specified date
- shipDateEnd string? (default ()) - Returns shipments with the
shipDate
on or before the specified date
- voidDateStart string? (default ()) - Returns shipments voided on or after the specified date
- voidDateEnd string? (default ()) - Returns shipments voided on or before the specified date
- storeId int? (default ()) - Returns shipments whose orders belong to the specified store ID.
- includeShipmentItems boolean? (default ()) - Specifies whether to include shipment items with results Default value: false.
- sortBy SortBy1? (default ()) - Sort the responses by a set value. The response will be sorted based off the ascending dates (oldest to most current.) If left empty, the response will be sorted by ascending
createDate
.
- sortDir SortDir? (default ()) - Sets the direction of the sort order.
- page int? (default ()) - page number.
- pageSize int? (default ()) - page size.
createShipmentLabel
function createShipmentLabel(string contentType, CreateShipmentLabelrequest payload) returns CreateShipmentLabelresponse|error
Create a shipment label
getRates
function getRates(string contentType, GetRatesrequest payload) returns GetRatesresponse[]|error
Retrieves shipping rates for the specified shipping details.
voidLabel
function voidLabel(string contentType, VoidLabelrequest payload) returns VoidLabelresponse|error
Voids the specified label by shipmentId.
getStore
function getStore(int storeId) returns GetStoreresponse|error
Get store
Parameters
- storeId int - A unique ID generated by ShipStation and assigned to each store.
updateStore
function updateStore(int storeId, string contentType, UpdateStorerequest payload) returns UpdateStoreresponse|error
Update store
Parameters
- storeId int - A unique ID generated by ShipStation and assigned to each store.
- contentType string -
- payload UpdateStorerequest -
getStoreRefreshStatus
function getStoreRefreshStatus(int? storeId) returns GetStoreRefreshStatusresponse|error
Retrieves the refresh status of a given store.
Parameters
- storeId int? (default ()) - Specifies the store whose status will be retrieved.
refreshStore
function refreshStore(string contentType, RefreshStorerequest payload, int? storeId, string? refreshDate) returns RefreshStoreresponse|error
Refresh store
Parameters
- contentType string -
- payload RefreshStorerequest -
- storeId int? (default ()) - Specifies the store which will get refreshed. If the storeId is not specified, a store refresh will be initiated for all refreshable stores on that account.
- refreshDate string? (default ()) - Specifies the starting date for new order imports. If the refreshDate is not specified, ShipStation will use the last recorded refreshDate for that store.
listStores
function listStores(boolean? showInactive, int? marketplaceId) returns ListStoresresponse[]|error
Retrieve the list of installed stores on the account.
Parameters
- showInactive boolean? (default ()) - Determines whether inactive stores will be returned in the list of stores.
- marketplaceId int? (default ()) - Returns stores of this marketplace type.
listMarketplaces
function listMarketplaces() returns ListMarketplacesresponse[]|error
Lists the marketplaces that can be integrated with ShipStation.
deactivateStore
function deactivateStore(string contentType, DeactivateStorerequest payload) returns DeactivateStoreresponse|error
Deactivate store
reactivateStore
function reactivateStore(string contentType, ReactivateStorerequest payload) returns ReactivateStoreresponse|error
Reactivate store
listUsers
function listUsers(boolean? showInactive) returns ListUsersresponse|error
List users
Parameters
- showInactive boolean? (default ()) - Determines whether inactive users will be returned in the response.
getWarehouse
function getWarehouse(int warehouseId) returns GetWarehouseresponse|error
Returns a list of active Ship From Locations (formerly known as warehouses) on the ShipStation account. Warehouses are now called "Ship From Locations" in the UI.
Parameters
- warehouseId int - A unique ID generated by ShipStation and assigned to each Ship From Location (formerly known as warehouse).
updateWarehouse
function updateWarehouse(string contentType, int warehouseId, UpdateWarehouserequest payload) returns UpdateWarehouseresponse|error
Updates an existing Ship From Location (formerly known as warehouse).
Parameters
- contentType string -
- warehouseId int - A unique ID generated by ShipStation and assigned to each Ship From Location (formerly known as warehouse).
- payload UpdateWarehouserequest -
deleteWarehouse
function deleteWarehouse(int warehouseId) returns DeleteWarehouseresponse|error
Delete warehouse
Parameters
- warehouseId int - A unique ID generated by ShipStation and assigned to each Ship From Location (formerly known as warehouse).
createWarehouse
function createWarehouse(string contentType, CreateWarehouserequest payload) returns CreateWarehouseresponse|error
Create warehouse
listWarehouses
function listWarehouses() returns ListWarehousesresponse[]|error
Retrieves a list of your Ship From Locations (formerly known as warehouses).
listWebhooks
function listWebhooks() returns ListWebhooksresponse|error
Retrieves a list of registered webhooks for the account
subscribeToWebhook
function subscribeToWebhook(string contentType, SubscribetoWebhookrequest payload) returns SubscribetoWebhookresponse|error
Subscribe to webhook
unsubscribeToWebhook
Unsubscribe to Webhook
Records
shipstation: AddFundsrequest
Fields
- carrierCode string -
- amount decimal -
shipstation: AddFundsresponse
Fields
- name string -
- code string -
- accountNumber string -
- requiresFundedAccount boolean -
- balance decimal -
shipstation: AddTagtoOrderrequest
Fields
- orderId int -
- tagId int -
shipstation: AddTagtoOrderresponse
Fields
- success boolean -
- message string -
shipstation: AdvancedOptions
Fields
- warehouseId int -
- nonMachinable boolean -
- saturdayDelivery boolean -
- containsAlcohol boolean -
- mergedOrSplit boolean -
- mergedIds string[] -
- parentId string? -
- storeId int -
- customField1 string -
- customField2 string -
- customField3 string -
- 'source string -
- billToParty string? -
- billToAccount string? -
- billToPostalCode string? -
- billToCountryCode string? -
shipstation: AdvancedOptions4
Fields
- warehouseId int -
- nonMachinable boolean -
- saturdayDelivery boolean -
- containsAlcohol boolean -
- mergedOrSplit boolean -
- mergedIds string[] -
- parentId string? -
- storeId int -
- customField1 string -
- customField2 string -
- customField3 string? -
- 'source string? -
- billToParty string? -
- billToAccount string? -
- billToPostalCode string? -
- billToCountryCode string? -
shipstation: AssignUsertoOrderrequest
Fields
- orderIds int[] -
- userId string -
shipstation: AssignUsertoOrderresponse
Fields
- success boolean -
- message string -
shipstation: BillTo
Fields
- name string -
- company string? -
- street1 string? -
- street2 string? -
- street3 string? -
- city string? -
- state string? -
- postalCode string? -
- country string? -
- phone string? -
- residential string? -
- addressVerified string? -
shipstation: BillTo1
Fields
- name string -
- company string? -
- street1 string? -
- street2 string? -
- street3 string? -
- city string? -
- state string? -
- postalCode string? -
- country string? -
- phone string? -
- residential string? -
shipstation: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
shipstation: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth CredentialsConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
shipstation: CreateLabelforOrderrequest
Fields
- orderId int -
- carrierCode string -
- serviceCode string -
- packageCode string -
- confirmation string? -
- shipDate string -
- weight Weight -
- dimensions string? -
- insuranceOptions string? -
- internationalOptions string? -
- advancedOptions string? -
- testLabel boolean -
shipstation: CreateLabelforOrderresponse
Fields
- shipmentId int -
- shipmentCost decimal -
- insuranceCost int -
- trackingNumber string -
- labelData string -
- formData string? -
shipstation: CreateOrUpdateMultipleOrdersrequest
Fields
- orderNumber string -
- orderKey string -
- orderDate string -
- paymentDate string -
- shipByDate string -
- orderStatus string -
- customerId int -
- customerUsername string -
- customerEmail string -
- billTo BillTo1 -
- shipTo ShipTo3 -
- items Item1[] -
- amountPaid decimal -
- taxAmount int -
- shippingAmount int -
- customerNotes string -
- internalNotes string -
- gift boolean -
- giftMessage string -
- paymentMethod string -
- requestedShippingService string -
- carrierCode string -
- serviceCode string -
- packageCode string -
- confirmation string -
- shipDate string -
- weight Weight -
- dimensions Dimensions -
- insuranceOptions InsuranceOptions -
- internationalOptions InternationalOptions1 -
- advancedOptions AdvancedOptions -
- tagIds int[] -
shipstation: CreateOrUpdateMultipleOrdersresponse
Fields
- hasErrors boolean -
- results Result[] -
shipstation: CreateOrUpdateOrderrequest
Fields
- orderNumber string -
- orderKey string -
- orderDate string -
- paymentDate string -
- shipByDate string -
- orderStatus string -
- customerId int -
- customerUsername string -
- customerEmail string -
- billTo BillTo1 -
- shipTo ShipTo3 -
- items Item1[] -
- amountPaid decimal -
- taxAmount int -
- shippingAmount int -
- customerNotes string -
- internalNotes string -
- gift boolean -
- giftMessage string -
- paymentMethod string -
- requestedShippingService string -
- carrierCode string -
- serviceCode string -
- packageCode string -
- confirmation string -
- shipDate string -
- weight Weight -
- dimensions Dimensions -
- insuranceOptions InsuranceOptions -
- internationalOptions InternationalOptions1 -
- advancedOptions AdvancedOptions -
- tagIds int[] -
shipstation: CreateOrUpdateOrderresponse
Fields
- orderId int -
- orderNumber string -
- orderKey string -
- orderDate string -
- createDate string -
- modifyDate string -
- paymentDate string -
- shipByDate string -
- orderStatus string -
- customerId string? -
- customerUsername string -
- customerEmail string -
- billTo BillTo -
- shipTo ShipTo2 -
- items Item2[] -
- orderTotal decimal -
- amountPaid decimal -
- taxAmount int -
- shippingAmount int -
- customerNotes string -
- internalNotes string -
- gift boolean -
- giftMessage string -
- paymentMethod string -
- requestedShippingService string -
- carrierCode string -
- serviceCode string -
- packageCode string -
- confirmation string -
- shipDate string -
- holdUntilDate string? -
- weight Weight -
- dimensions Dimensions -
- insuranceOptions InsuranceOptions -
- internationalOptions InternationalOptions -
- advancedOptions AdvancedOptions -
- tagIds string? -
- userId string? -
- externallyFulfilled boolean -
- externallyFulfilledBy string? -
shipstation: CreateShipmentLabelrequest
Fields
- carrierCode string -
- serviceCode string -
- packageCode string -
- confirmation string? -
- shipDate string -
- weight Weight -
- dimensions Dimensions? -
- shipFrom ShipFrom -
- shipTo ShipTo11 -
- insuranceOptions string? -
- internationalOptions string? -
- advancedOptions string? -
- testLabel boolean -
shipstation: CreateShipmentLabelresponse
Fields
- shipmentId int -
- orderId string? -
- userId string? -
- customerEmail string? -
- orderNumber string? -
- createDate string -
- shipDate string -
- shipmentCost decimal -
- insuranceCost int -
- trackingNumber string -
- isReturnLabel boolean -
- batchNumber string? -
- carrierCode string -
- serviceCode string -
- packageCode string -
- confirmation string -
- warehouseId string? -
- voided boolean -
- voidDate string? -
- marketplaceNotified boolean -
- notifyErrorMessage string? -
- shipTo string? -
- weight string? -
- dimensions string? -
- insuranceOptions string? -
- advancedOptions string? -
- shipmentItems string? -
- labelData string -
- formData string? -
shipstation: CreateWarehouserequest
Fields
- warehouseName string -
- originAddress OriginAddress3 -
- returnAddress string? -
- isDefault boolean -
shipstation: CreateWarehouseresponse
Fields
- warehouseId int -
- warehouseName string -
- originAddress OriginAddress4 -
- returnAddress ReturnAddress3 -
- createDate string -
- isDefault boolean -
shipstation: Customer
Fields
- customerId int -
- createDate string -
- modifyDate string? -
- name string -
- company string -
- street1 string -
- street2 string -
- city string -
- state string -
- postalCode string -
- countryCode string -
- phone string -
- email string -
- addressVerified string -
- marketplaceUsernames MarketplaceUsername[] -
- tags Tag[] -
shipstation: CustomsItem
Fields
- customsItemId int -
- description string -
- quantity int -
- value int -
- harmonizedTariffCode string? -
- countryOfOrigin string -
shipstation: DeactivateStorerequest
Fields
- storeId string -
shipstation: DeactivateStoreresponse
Fields
- success string -
- message string -
shipstation: DeleteOrderresponse
Fields
- success boolean -
- message string -
shipstation: DeleteWarehouseresponse
Fields
- success boolean -
- message string -
shipstation: Dimensions
Fields
- units string -
- length int -
- width int -
- height int -
shipstation: Fulfillment
Fields
- fulfillmentId int -
- orderId int -
- orderNumber string -
- userId string -
- customerEmail string -
- trackingNumber string -
- createDate string -
- shipDate string -
- voidDate string? -
- deliveryDate string? -
- carrierCode string -
- fulfillmentProviderCode string? -
- fulfillmentServiceCode string? -
- fulfillmentFee int -
- voidRequested boolean -
- voided boolean -
- marketplaceNotified boolean -
- notifyErrorMessage string? -
- shipTo ShipTo -
shipstation: GetCarrierresponse
Fields
- name string -
- code string -
- accountNumber string -
- requiresFundedAccount boolean -
- balance decimal -
- nickname string? -
- shippingProviderId int -
- primary boolean -
shipstation: GetCustomerresponse
Fields
- customerId int -
- createDate string -
- modifyDate string -
- name string -
- company string -
- street1 string -
- street2 string -
- city string -
- state string -
- postalCode string -
- countryCode string -
- phone string -
- email string -
- addressVerified string -
- marketplaceUsernames MarketplaceUsername[] -
- tags Tag[] -
shipstation: GetOrderresponse
Fields
- orderId int -
- orderNumber string -
- orderKey string -
- orderDate string -
- createDate string -
- modifyDate string -
- paymentDate string -
- shipByDate string -
- orderStatus string -
- customerId int -
- customerUsername string -
- customerEmail string -
- billTo BillTo -
- shipTo ShipTo2 -
- items Item[] -
- orderTotal decimal -
- amountPaid decimal -
- taxAmount int -
- shippingAmount int -
- customerNotes string -
- internalNotes string -
- gift boolean -
- giftMessage string -
- paymentMethod string -
- requestedShippingService string -
- carrierCode string -
- serviceCode string -
- packageCode string -
- confirmation string -
- shipDate string -
- holdUntilDate string? -
- weight Weight -
- dimensions Dimensions -
- insuranceOptions InsuranceOptions -
- internationalOptions InternationalOptions -
- advancedOptions AdvancedOptions -
- tagIds string? -
- userId string? -
- externallyFulfilled boolean -
- externallyFulfilledBy string? -
shipstation: GetProductresponse
Fields
- aliases string? -
- productId int -
- sku string -
- name string -
- price int -
- defaultCost int -
- length int -
- width int -
- height int -
- weightOz int -
- internalNotes string? -
- fulfillmentSku string -
- createDate string -
- modifyDate string -
- active boolean -
- productCategory ProductCategory -
- productType string? -
- warehouseLocation string -
- defaultCarrierCode string -
- defaultServiceCode string -
- defaultPackageCode string -
- defaultIntlCarrierCode string -
- defaultIntlServiceCode string -
- defaultIntlPackageCode string -
- defaultConfirmation string -
- defaultIntlConfirmation string -
- customsDescription string? -
- customsValue string? -
- customsTariffNo string? -
- customsCountryCode string? -
- noCustoms string? -
- tags Tag[] -
shipstation: GetRatesrequest
Fields
- carrierCode string -
- serviceCode string? -
- packageCode string? -
- fromPostalCode string -
- toState string -
- toCountry string -
- toPostalCode string -
- toCity string -
- weight Weight -
- dimensions Dimensions -
- confirmation string -
- residential boolean -
shipstation: GetRatesresponse
Fields
- serviceName string -
- serviceCode string -
- shipmentCost decimal -
- otherCost decimal -
shipstation: GetStoreRefreshStatusresponse
Fields
- storeId int -
- refreshStatusId int -
- refreshStatus string -
- lastRefreshAttempt string -
- refreshDate string -
shipstation: GetStoreresponse
Fields
- storeId int -
- storeName string -
- marketplaceId int -
- marketplaceName string -
- accountName string? -
- email string? -
- integrationUrl string -
- active boolean -
- companyName string -
- phone string -
- publicEmail string -
- website string -
- refreshDate string -
- lastRefreshAttempt string -
- createDate string -
- modifyDate string -
- autoRefresh boolean -
- statusMappings StatusMapping[] -
shipstation: GetWarehouseresponse
Fields
- warehouseId int -
- warehouseName string -
- originAddress OriginAddress -
- returnAddress ReturnAddress -
- createDate string -
- isDefault boolean -
shipstation: HoldOrderUntilrequest
Fields
- orderId int -
- holdUntilDate string -
shipstation: HoldOrderUntilresponse
Fields
- success boolean -
- message string -
shipstation: InsuranceOptions
Fields
- provider string -
- insureShipment boolean -
- insuredValue int -
shipstation: InsuranceOptions4
Fields
- provider string? -
- insureShipment boolean -
- insuredValue int -
shipstation: InternationalOptions
Fields
- contents string? -
- customsItems string? -
- nonDelivery string? -
shipstation: InternationalOptions1
Fields
- contents string? -
- customsItems string? -
shipstation: InternationalOptions4
Fields
- contents string -
- customsItems CustomsItem[] -
- nonDelivery string -
shipstation: Item
Fields
- orderItemId int -
- lineItemKey string -
- sku string -
- name string -
- imageUrl string? -
- weight Weight -
- quantity int -
- unitPrice decimal -
- taxAmount string? -
- shippingAmount string? -
- warehouseLocation string -
- options Option[] -
- productId int -
- fulfillmentSku string? -
- adjustment boolean -
- upc string? -
- createDate string -
- modifyDate string -
shipstation: Item1
Fields
- lineItemKey string -
- sku string -
- name string -
- imageUrl string? -
- weight Weight -
- quantity int -
- unitPrice decimal -
- taxAmount decimal -
- shippingAmount decimal -
- warehouseLocation string -
- options Option[] -
- productId int -
- fulfillmentSku string? -
- adjustment boolean -
- upc string -
shipstation: Item2
Fields
- orderItemId int -
- lineItemKey string -
- sku string -
- name string -
- imageUrl string? -
- weight Weight -
- quantity int -
- unitPrice decimal -
- taxAmount decimal -
- shippingAmount int -
- warehouseLocation string -
- options Option[] -
- productId string? -
- fulfillmentSku string? -
- adjustment boolean -
- upc string -
- createDate string -
- modifyDate string -
shipstation: Item4
Fields
- orderItemId int -
- lineItemKey string? -
- sku string -
- name string -
- imageUrl string? -
- weight Weight -
- quantity int -
- unitPrice decimal -
- taxAmount string? -
- shippingAmount string? -
- warehouseLocation string -
- options Option[] -
- productId int -
- fulfillmentSku string -
- adjustment boolean -
- upc string? -
- createDate string -
- modifyDate string -
shipstation: Item6
Fields
- orderItemId int -
- lineItemKey string -
- sku string -
- name string -
- imageUrl string? -
- weight Weight -
- quantity int -
- unitPrice decimal -
- taxAmount decimal -
- shippingAmount int -
- warehouseLocation string -
- options Option[] -
- productId int -
- fulfillmentSku string? -
- adjustment boolean -
- upc string -
- createDate string -
- modifyDate string -
shipstation: ListCarriersresponse
Fields
- name string -
- code string -
- accountNumber string -
- requiresFundedAccount boolean -
- balance decimal -
- nickname string? -
- shippingProviderId int -
- primary boolean -
shipstation: ListCustomersResponse
Fields
- customers Customer[] -
- total int -
- page int -
- pages int -
shipstation: ListFulfillmentsResponse
Fields
- fulfillments Fulfillment[] -
- total int -
- page int -
- pages int -
shipstation: ListMarketplacesresponse
Fields
- name string -
- marketplaceId int -
- canRefresh boolean -
- supportsCustomMappings boolean -
- supportsCustomStatuses boolean -
- canConfirmShipments boolean -
shipstation: ListOrdersbyTagresponse
Fields
- orders Order2[] -
- total int -
- page int -
- pages int -
shipstation: ListOrdersResponse
Fields
- orders Order[] -
- total int -
- page int -
- pages int -
shipstation: ListPackagesresponse
Fields
- carrierCode string -
- code string -
- name string -
- domestic boolean -
- international boolean -
shipstation: ListProductsResponse
Fields
- products Product[] -
- total int -
- page int -
- pages int -
shipstation: ListServicesresponse
Fields
- carrierCode string -
- code string -
- name string -
- domestic boolean -
- international boolean -
shipstation: ListShipmentsResponse
Fields
- shipments Shipment[] -
- total int -
- page int -
- pages int -
shipstation: ListStoresresponse
Fields
- storeId int -
- storeName string -
- marketplaceId int -
- marketplaceName string -
- accountName string? -
- email string? -
- integrationUrl string? -
- active boolean -
- companyName string -
- phone string -
- publicEmail string -
- website string -
- refreshDate string -
- lastRefreshAttempt string -
- createDate string -
- modifyDate string -
- autoRefresh boolean -
shipstation: ListTagsresponse
Fields
- tagId int -
- name string -
- color string -
shipstation: ListUsersresponse
Fields
- userId string -
- userName string -
- name string -
shipstation: ListWarehousesresponse
Fields
- warehouseId int -
- warehouseName string -
- originAddress OriginAddress4 -
- returnAddress ReturnAddress3 -
- createDate string -
- isDefault boolean -
shipstation: ListWebhooksresponse
Fields
- webhooks Webhook[] -
shipstation: MarkanOrderasShippedrequest
Fields
- orderId int -
- carrierCode string -
- shipDate string -
- trackingNumber string -
- notifyCustomer boolean -
- notifySalesChannel boolean -
shipstation: MarkanOrderasShippedresponse
Fields
- orderId int -
- orderNumber string -
shipstation: MarketplaceUsername
Fields
- customerUserId int -
- customerId int -
- createDate string -
- modifyDate string -
- marketplaceId int -
- marketplace string -
- username string -
shipstation: Option
Fields
- name string? -
- value string? -
shipstation: Order
Fields
- orderId int -
- orderNumber string -
- orderKey string -
- orderDate string -
- createDate string -
- modifyDate string -
- paymentDate string -
- shipByDate string -
- orderStatus string -
- customerId int -
- customerUsername string -
- customerEmail string -
- billTo BillTo -
- shipTo ShipTo6 -
- items Item4[] -
- orderTotal decimal -
- amountPaid decimal -
- taxAmount decimal -
- shippingAmount int -
- customerNotes string -
- internalNotes string -
- gift boolean -
- giftMessage string? -
- paymentMethod string? -
- requestedShippingService string -
- carrierCode string -
- serviceCode string -
- packageCode string -
- confirmation string -
- shipDate string -
- holdUntilDate string? -
- weight Weight -
- dimensions Dimensions -
- insuranceOptions InsuranceOptions4 -
- internationalOptions InternationalOptions4 -
- advancedOptions AdvancedOptions4 -
- tagIds string? -
- userId string? -
- externallyFulfilled boolean -
- externallyFulfilledBy string? -
shipstation: Order2
Fields
- orderId int -
- orderNumber string -
- orderKey string -
- orderDate string -
- createDate string -
- modifyDate string -
- paymentDate string -
- shipByDate string -
- orderStatus string -
- customerId int -
- customerUsername string -
- customerEmail string -
- billTo BillTo -
- shipTo ShipTo2 -
- items Item6[] -
- orderTotal decimal -
- amountPaid decimal -
- taxAmount int -
- shippingAmount int -
- customerNotes string -
- internalNotes string -
- gift boolean -
- giftMessage string -
- paymentMethod string -
- requestedShippingService string -
- carrierCode string -
- serviceCode string -
- packageCode string -
- confirmation string -
- shipDate string -
- holdUntilDate string? -
- weight Weight -
- dimensions Dimensions -
- insuranceOptions InsuranceOptions -
- internationalOptions InternationalOptions -
- advancedOptions AdvancedOptions -
- tagIds int[] -
- userId string -
- externallyFulfilled boolean -
- externallyFulfilledBy string? -
shipstation: OriginAddress
Fields
- name string -
- company string -
- street1 string -
- street2 string? -
- street3 string? -
- city string -
- state string -
- postalCode string -
- country string -
- phone string -
- residential boolean -
- addressVerified string? -
shipstation: OriginAddress3
Fields
- name string -
- company string -
- street1 string -
- street2 string? -
- street3 string? -
- city string -
- state string -
- postalCode string -
- country string -
- phone string -
- residential boolean -
shipstation: OriginAddress4
Fields
- name string -
- company string -
- street1 string -
- street2 string -
- street3 string -
- city string -
- state string -
- postalCode string -
- country string -
- phone string -
- residential boolean -
shipstation: Product
Fields
- aliases string? -
- productId int -
- sku string -
- name string -
- price int -
- defaultCost int -
- length int -
- width int -
- height int -
- weightOz int -
- internalNotes string? -
- fulfillmentSku string -
- createDate string -
- modifyDate string -
- active boolean -
- productCategory ProductCategory -
- productType string? -
- warehouseLocation string -
- defaultCarrierCode string -
- defaultServiceCode string -
- defaultPackageCode string -
- defaultIntlCarrierCode string -
- defaultIntlServiceCode string -
- defaultIntlPackageCode string -
- defaultConfirmation string -
- defaultIntlConfirmation string -
- customsDescription string? -
- customsValue string? -
- customsTariffNo string? -
- customsCountryCode string? -
- noCustoms string? -
- tags Tag[] -
shipstation: ProductCategory
Fields
- categoryId int -
- name string -
shipstation: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
shipstation: ReactivateStorerequest
Fields
- storeId string -
shipstation: ReactivateStoreresponse
Fields
- success string -
- message string -
shipstation: RefreshStorerequest
Fields
- storeId int -
- refreshDate string -
shipstation: RefreshStoreresponse
Fields
- success boolean -
- message string -
shipstation: RegisterAccountrequest
Fields
- firstName string -
- lastName string -
- email string -
- password string -
- companyName string? -
- addr1 string? -
- addr2 string? -
- city string? -
- state string? -
- zip string? -
- countryCode string? -
- phone string? -
shipstation: RegisterAccountresponse
Fields
- message string -
- sellerId int -
- success boolean -
- apiKey string -
- apiSecret string -
shipstation: RemoveTagfromOrderrequest
Fields
- orderId int -
- tagId int -
shipstation: RemoveTagfromOrderresponse
Fields
- success boolean -
- message string -
shipstation: RestoreOrderfromOnHoldrequest
Fields
- orderId int -
shipstation: RestoreOrderfromOnHoldresponse
Fields
- success boolean -
- message string -
shipstation: Result
Fields
- orderId int -
- orderNumber string -
- orderKey string -
- success boolean -
- errorMessage string? -
shipstation: ReturnAddress
Fields
- name string -
- company string -
- street1 string -
- street2 string? -
- street3 string? -
- city string -
- state string -
- postalCode string -
- country string -
- phone string -
- residential string? -
- addressVerified string? -
shipstation: ReturnAddress3
Fields
- name string -
- company string -
- street1 string -
- street2 string -
- street3 string -
- city string -
- state string -
- postalCode string -
- country string -
- phone string -
- residential string? -
shipstation: ShipFrom
Fields
- name string -
- company string -
- street1 string -
- street2 string -
- street3 string? -
- city string -
- state string -
- postalCode string -
- country string -
- phone string? -
- residential boolean -
shipstation: Shipment
Fields
- shipmentId int -
- orderId int -
- orderKey string? -
- userId string -
- orderNumber string -
- createDate string -
- shipDate string -
- shipmentCost decimal -
- insuranceCost int -
- trackingNumber string -
- isReturnLabel boolean -
- batchNumber string -
- carrierCode string -
- serviceCode string -
- packageCode string -
- confirmation string -
- warehouseId int -
- voided boolean -
- voidDate string? -
- marketplaceNotified boolean -
- notifyErrorMessage string? -
- shipTo ShipTo9 -
- weight Weight -
- dimensions string? -
- insuranceOptions InsuranceOptions4 -
- advancedOptions string? -
- shipmentItems ShipmentItem[] -
- labelData string? -
- formData string? -
shipstation: ShipmentItem
Fields
- orderItemId int -
- lineItemKey string? -
- sku string -
- name string -
- imageUrl string? -
- weight string? -
- quantity int -
- unitPrice int -
- warehouseLocation string? -
- options string? -
- productId int -
- fulfillmentSku string? -
shipstation: ShipTo
Fields
- name string -
- company string? -
- street1 string -
- street2 string? -
- street3 string? -
- city string -
- state string -
- postalCode string -
- country string -
- phone string -
- residential string? -
- addressVerified string? -
shipstation: ShipTo11
Fields
- name string -
- company string -
- street1 string -
- street2 string -
- street3 string? -
- city string -
- state string -
- postalCode string -
- country string -
- phone string? -
- residential boolean -
shipstation: ShipTo2
Fields
- name string -
- company string -
- street1 string -
- street2 string -
- street3 string? -
- city string -
- state string -
- postalCode string -
- country string -
- phone string -
- residential boolean -
- addressVerified string -
shipstation: ShipTo3
Fields
- name string -
- company string -
- street1 string -
- street2 string -
- street3 string? -
- city string -
- state string -
- postalCode string -
- country string -
- phone string -
- residential boolean -
shipstation: ShipTo6
Fields
- name string -
- company string -
- street1 string -
- street2 string -
- street3 string? -
- city string -
- state string -
- postalCode string -
- country string -
- phone string? -
- residential boolean -
- addressVerified string -
shipstation: ShipTo9
Fields
- name string -
- company string -
- street1 string -
- street2 string -
- street3 string? -
- city string -
- state string -
- postalCode string -
- country string -
- phone string -
- residential string? -
shipstation: StatusMapping
Fields
- orderStatus string -
- statusKey string -
shipstation: SubscribetoWebhookrequest
Fields
- target_url string -
- event string -
- store_id string? -
- friendly_name string -
shipstation: SubscribetoWebhookresponse
Fields
- id int -
shipstation: Tag
Fields
- tagId int -
- name string -
shipstation: UnassignUserfromOrderrequest
Fields
- orderIds int[] -
shipstation: UnassignUserfromOrderresponse
Fields
- success boolean -
- message string -
shipstation: UpdateProductrequest
Fields
- aliases string? -
- productId int -
- sku string -
- name string -
- price int -
- defaultCost string? -
- length string? -
- width string? -
- height string? -
- weightOz string? -
- internalNotes string? -
- fulfillmentSku string? -
- active boolean -
- productCategory string? -
- productType string? -
- warehouseLocation string? -
- defaultCarrierCode string? -
- defaultServiceCode string? -
- defaultPackageCode string? -
- defaultIntlCarrierCode string? -
- defaultIntlServiceCode string? -
- defaultIntlPackageCode string? -
- defaultConfirmation string? -
- defaultIntlConfirmation string? -
- customsDescription string? -
- customsValue string? -
- customsTariffNo string? -
- customsCountryCode string? -
- noCustoms string? -
- tags string? -
shipstation: UpdateProductresponse
Fields
- success boolean -
- message string -
shipstation: UpdateStorerequest
Fields
- storeId int -
- storeName string -
- marketplaceId int -
- marketplaceName string -
- accountName string? -
- email string? -
- integrationUrl string -
- active boolean -
- companyName string -
- phone string -
- publicEmail string -
- website string -
- refreshDate string -
- lastRefreshAttempt string -
- createDate string -
- modifyDate string -
- autoRefresh boolean -
- statusMappings StatusMapping[] -
shipstation: UpdateStoreresponse
Fields
- storeId int -
- storeName string -
- marketplaceId int -
- marketplaceName string -
- accountName string? -
- email string? -
- integrationUrl string -
- active boolean -
- companyName string -
- phone string -
- publicEmail string -
- website string -
- refreshDate string -
- lastRefreshAttempt string -
- createDate string -
- modifyDate string -
- autoRefresh boolean -
- statusMappings StatusMapping[] -
shipstation: UpdateWarehouserequest
Fields
- warehouseId int -
- warehouseName string -
- originAddress OriginAddress -
- returnAddress ReturnAddress -
- createDate string -
- isDefault boolean -
shipstation: UpdateWarehouseresponse
Fields
- warehouseId int -
- warehouseName string -
- originAddress OriginAddress -
- returnAddress ReturnAddress -
- createDate string -
- isDefault boolean -
shipstation: VoidLabelrequest
Fields
- shipmentId int -
shipstation: VoidLabelresponse
Fields
- approved boolean -
- message string -
shipstation: Webhook
Fields
- IsLabelAPIHook boolean -
- WebHookID int -
- SellerID int -
- StoreID int -
- HookType string -
- MessageFormat string -
- Url string -
- Name string -
- BulkCopyBatchID string? -
- BulkCopyRecordID string? -
- Active boolean -
- WebhookLogs string[] -
- Seller string? -
- Store string? -
shipstation: Weight
Fields
- value int -
- units string -
String types
Import
import ballerinax/shipstation;
Metadata
Released date: about 2 years ago
Version: 1.4.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 6
Current verison: 6
Weekly downloads
Keywords
Commerce/eCommerce
Cost/Paid
Contributors
Dependencies