shippit
Module shippit
API
Definitions
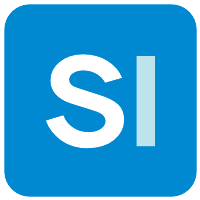
ballerinax/shippit Ballerina library
Overview
This is a generated connector for Shippit API v3.0.20201008 OpenAPI specification. Shippit powers simpler delivery for e-commerce retailers of all shapes and sizes. It fulfil orders and track shipments anywhere you are, anytime you like. It’s cloud-based multi-carrier shipping software for retailers that takes the guesswork out of shipping decisions.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Shippit account
- Obtain tokens by following this guide
Quickstart
To use the Shippit connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/shippit
module into the Ballerina project.
import ballerinax/shippit;
Step 2: Create a new connector instance
Create a shippit:ClientConfig
with the API key
obtained, and initialize the connector with it.
shippit:ClientConfig clientConfig = { auth: { token: <API_KEY> } }; shippit:Client baseClient = check new Client(clientConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to track an order using the connector.
Track order
public function main() { shippit:TrackingResponse|error response = baseClient->getBudgets(); if (response is shippit:TrackingResponse) { log:printInfo(response.toString()); } else { log:printError(response.message()); } }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
shippit: Client
This is a generated connector for Shippit API v3.0.20201008 OpenAPI specification. Shippit powers simpler delivery for e-commerce retailers of all shapes and sizes. It fulfil orders and track shipments anywhere you are, anytime you like. It’s cloud-based multi-carrier shipping software for retailers that takes the guesswork out of shipping decisions.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Shippit account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://app.staging.shippit.com/api/3" - URL of the target service
createOrder
function createOrder(OrderRequest payload) returns OrderResponse|error
Create an Order
Parameters
- payload OrderRequest - Passes an Order object under the
order
field.
Return Type
- OrderResponse|error - Successful order response
cancelOrder
function cancelOrder(string trackingNumber) returns OrderDeleteResponse|error
Cancel an Order
Parameters
- trackingNumber string - The tracking number of the Order.
Return Type
- OrderDeleteResponse|error - Returns the Order with state =
cancelled
getOrderLabel
function getOrderLabel(string trackingNumber) returns LabelResponse|error
Get Label information for an Order
Parameters
- trackingNumber string - The tracking number of the Order.
Return Type
- LabelResponse|error - Returns an Order and related label information.
trackOrder
function trackOrder(string trackingNumber) returns TrackingResponse|error
Track Order
Parameters
- trackingNumber string - The tracking number of the Order
Return Type
- TrackingResponse|error - Returns tracking info related to the Order
getQuote
function getQuote(QuoteRequest payload) returns QuoteResponse|error
Retrieve Quote
Parameters
- payload QuoteRequest - Quote request
Return Type
- QuoteResponse|error - Successful quote response
getMerchant
function getMerchant() returns MerchantResponse|error
Get Merchant settings
Return Type
- MerchantResponse|error - Returns an object representing your merchant account settings.
updateMerchant
function updateMerchant(MerchantRequest payload) returns MerchantResponse|error
Update Merchant settings
Parameters
- payload MerchantRequest - Merchant request
Return Type
- MerchantResponse|error - The updated merchant account.
trackOrderHook
function trackOrderHook(WebhookRequest payload) returns Response|error
Webhook
Parameters
- payload WebhookRequest - Updated status info about the Order sent by the webhook.
Return Type
bookOrder
function bookOrder(BookRequest payload) returns BookResponse|error
Book Order
Parameters
- payload BookRequest - An array of Order tracking numbers to book
Return Type
- BookResponse|error - Returns the status of the bookings at each courier. This API will validate that all of the requested orders are accurate and ready to be booked, if all of the orders sent in cannot be booked, the response will detail which orders cannot be found. If this response is received, none of the order numbers sent in will have been booked. If a manifest fails to be generated, the response will include an array of orders on each manifest which were not booked.
Records
shippit: Book
The book API accepts an array of orders to book, this will initiate a booking with the respective carriers of the orders sent in.
shippit: BookingStatus
Describes the status of booking an order at a courier
Fields
- courier string? - The courier where the booking was made
- manifest string? - The Manifest ID of the booking
- manifest_pdf string? - A URL to a printable copy of the shipping manifest. This is a pre-signed URL, generated by the Shippit platform to provide access to a secured document. The pre-signed URL remains valid for 7 days. If you need to access this document after expiration, please make another request for a new URL to be issued.
- order_count int? - How many orders were included in this manifest.
- success boolean? - Whether or not the booking succeeded
- orders string[]? - An array of order tracking numbers included in this status. Used to indicate which orders failed to manifest in the case where
success
=false
.
- 'error string? - Error code that applies to the individual bookings / orders referenced.
- error_description string? - Human-readable error message applied to the individual bookings / orders referenced.
shippit: BookRequest
The book API accepts an array of orders to book, this will initiate a booking with the respective carriers of the orders sent in.
Fields
- orders string[] - an array of Shippit tracking numbers, corresponding to the orders which you would like to book.
shippit: BookResponse
Represents a response to the Book API, which returns the status of booking the order at each courier.
Fields
- response BookingStatus[]? - Array of booking statuses at each courier
- 'error string? - Error code returned by Shippit For the book response, there can be errors at the top-level request or for individual orders booked. If the error occurs at the top-level request (order format, authorization), then this error code applies. Otherwise, the response will be successful and there will be individual errors applied in the body of each booked item.
- error_description string? - Human-readable description of the top-level error encountered.
- count int? - Number of items in the response
shippit: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
shippit: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
shippit: DataResponse
Response message containing data.
shippit: ErrorResponse
Response message containing an error.
Fields
- 'error anydata - Error code returned by Shippit
- error_description string? - Human-readable response
shippit: GenericErrorResponse
Response message containing an error.
Fields
- 'error string - Error code returned by Shippit
- error_description string? - Human-readable description of error encountered.
- Fields Included from *ErrorResponse
- error anydata
- error_description string
- anydata...
shippit: Label
Represents an Order and related labelling information
Fields
- data record {}? - Data
- id int? - Shippit internal ID of the generated label.
- 'order OrderResponseOrder - Represents an Order that was created in Shippit
- qualified_url string - URL to a printable label for the Order. This is a pre-signed URL, generated by the Shippit platform to provide access to a secured label. The pre-signed URL remains valid for 7 days. If you need to access this label after expiration, please make another request for a new URL to be issued.
shippit: LabelDocument
A Label or document in the Labels API, representing a printable document available on a public URL.
Fields
- url string? - URL to a printable PDF document. This is a pre-signed URL, generated by the Shippit platform to provide access to a secured document. The pre-signed URL remains valid for 7 days. If you need to access this document after expiration, please make another request for a new URL to be issued.
- page_size string? - Page size
- file_type string? - File type of the label
- encoded_label string? - If you have ZPL for your account, printable ZPL data for the document.
shippit: LabelResponse
Represents a response to the Label API, which provides an Order and related label information
Fields
- Fields Included from *DataResponse
- anydata...
shippit: Merchant
Represents a merchant account
Fields
- store_name string? - Store name
- company_name string? - Company name
- contact_name string? - Contact name
- contact_phone string? - Contact phone
- shipping_cart_method_name string? - Shipping cart method name
- preparation_time int? - Preparation time
- website_url string? - Website URL
- address_1 string? - Address
- suburb string? - Suburb
- state string? - State
- postcode string? - Postcode
shippit: MerchantRequest
Represents a request to update a merchant
Fields
- merchant Merchant - Represents a merchant account
shippit: MerchantResponse
Represents a response to the Merchant request API, which shows either the generated Merchant object, or an error code with a description of the error.
Fields
- Fields Included from *DataResponse
- anydata...
shippit: Order
Represents an Order to be delivered by Shippit.
Fields
- delivery_address string - delivery address for the order
- delivery_postcode string - delivery postcode for the order
- delivery_state string - delivery state for the order
Australian States valid options are
NSW
,QLD
,ACT
,WA
,NT
,SA
,VIC
,TAS
- delivery_suburb string - delivery suburb for the order
- delivery_instructions string? - special delivery instructions for the order, limited to 55 characters
- receiver_contact_number string? -
conditional
-- number of the person receiving the order, may be different than the user who purchased the order. Mandatory for International orders.
- receiver_name string? - name of the person receiving the order if different than the user who purchased the order
- retailer_invoice string? - Merchant invoice number - the customer-facing sales order reference of the order. This would be what the recipient sees on the shipping label, packslip, customs declaration, receipt, etc.
- receiver_language_code string? - Two-letter ISO 639-1 language code of the recipient. Used to determine the language of tracking notifications and other communications. Defaults to
EN
- delivery_date string? - The date of the order for priority orders.
The recommended format is in ISO-8601
YYYY-MM-DD
, although the Shippit is flexible enough to parseYYYY/MM/DD
.conditional
-- specifies the date of the order for priority ordersdelivery_date
must be in the future.
- delivery_window string? - expected format:
00:00-00:00
conditional
-- specifies the time window of the order, to be used when sending a priority orderdelivery_window
must be in the future.
- courier_job_id string? - Used in tracking_order's, the ID assigned by some carriers to the shipment / consignment.
- delivery_country_code string? - The destination country code for the order in
ISO 3166 Alpha-2
format. If unassigned, defaults to the country code of the merchant's primary location, or otherwise 'AU'.
- delivery_district_city string? - Used in some countries to add additional district city information in addition to the
delivery_suburb
anddelivery_state
. Most of this time this is determined using thedelivery_suburb
anddelivery_postcode
.
shippit: OrderDeleteErrorResponse
Fields
- order_state string - The current state of the order should a DELETE request fail
- Fields Included from *GenericErrorResponse
shippit: OrderRequest
Represents a request to submit an order to shippit.
Fields
- 'order OrderRequestOrder - Represents parameters that can be used to create an order in Shippit
shippit: OrderResponseOrderDocumentsHash
A keyword hash of documents generated for an order. Usually populated during the LABEL call.
Fields
- archive_awb LabelDocument? - A Label or document in the Labels API, representing a printable document available on a public URL.
- customs_invoice LabelDocument? - A Label or document in the Labels API, representing a printable document available on a public URL.
- dangerous_goods_declaration LabelDocument? - A Label or document in the Labels API, representing a printable document available on a public URL.
- packing_slip LabelDocument? - A Label or document in the Labels API, representing a printable document available on a public URL.
- shipping_label LabelDocument? - A Label or document in the Labels API, representing a printable document available on a public URL.
shippit: OrderResponseOrderParcel
Represents a parcel attached to an order.
Fields
- Fields Included from *Parcel
shippit: OrderResponseTrackingHistory
For tracking-only
orders, an object representing the tracking events provided in order creation.
The object returned is in the following format:
{ "status_name": "ISO-8601-TIMESTAMP" }
.
shippit: OrderValidationErrorResponse
Response message for order create requests with validate
flag set to true.
Fields
- delivery_postcode string[]? - List of error messages related to the postcode.
- delivery_suburb string[]? - List of error messages related to the suburb.
- suggested_address OrdervalidationerrorresponseSuggestedAddress? - Suggested address, if one is found.
- delivery_country_code string[]? - List of error messages related to the country code.
- delivery_state string[]? - List of error messages related to the state.
- products string[]? - List of error messages related to the provided product information. Typically appears in international orders.
- Fields Included from *GenericErrorResponse
shippit: OrdervalidationerrorresponseSuggestedAddress
Suggested address, if one is found.
Fields
- suburb string? - Suburb
- postcode string? - Postcode
- state string? - State
- country_code string? - Country code
shippit: Parcel
Represents a parcel attached to an order.
parcels vs products
How parcels relate to products is controlled by whether or not a product_attributes
key is also defined. If a product_attributes
is not defined, then the number of cartons is determined by the Settings -> Pick & Pack -> Allocate each item in an order to a separate carton
option in Shippit, and the qty
field will now specify either the number of parcels or products.
If a product_attributes
key is specified in the request, then the products can be specified separately from the parcels. In which case, the qty
field should not be present: each parcel attributes object represents a separate parcel, and the number of products is specified in the product_attributes
key. There is a maximum of 1000 parcels per request.
Fields
- weight decimal? - Weight of the parcel in kilos.
- depth decimal? - Depth of the parcel in metres
- length decimal? - Length of the parcel in metres
- width decimal? - Width of the parcel in metres.
- label_number string? - Parcel number in the carrier system. This is used when the order is a tracking order, to match the parcel number in a carrier against the parcel recorded in shippit.
- qty decimal? -
conditional
-- The number of products specified by the entry. There is a maximum of 1000 parcels per quote request. Should not be present if aproduct_attributes
key is present in the request. IfAllocate each item in an order to a separate carton
is enabled in Shippit, a separate parcel is generated for each product listed.
shippit: Product
Represents a single product line item in an order.
Fields
- title string? -
conditional
-- Title or description of the product. Mandatory for international orders with specific couriers.
- packed int? - The number of products packed; used in partial orders, or otherwise when the number of products in the parcel is less than the total in the order. If not present, set to product
quantity
. If this is present, at least 1 item should have packed greater than0
. For international orders, all items should have packed greater than0
.
- sku string? -
conditional
-- Stock Keeping Unit (SKU) code of the product, for stock keeping purposes. Mandatory on international orders with specific couriers.
- product_line_id string? - Product line associated with product. Like SKU, this is also for stock keeping purposes, and can be used when SKU would not be enough (e.g. an order containing multiple lines with the same SKU)
- origin_country_code string? - Two-letter country code (ISO 3166-1 Alpha-2) for the product's origin country. This is used in customs tracking for International shipments. Defaults to the home country of the Merchant.
- location string? - Location of the product
- dangerous_goods_code string? - This is the DG code required when making dangerous goods declarations.
Before you can declare orders as having dangerous goods, you must first request for dangerous goods to enabled for your account. Contact Shippit Support or your account manager to have this enabled.
DG surcharges are often charged on the weight of the order, so it is recommended to split your order into multiple orders if your order contains either:
- both dangerous and non-dangerous goods, or
- more than one dangerous goods code
- dangerous_goods_text string? - Additional information related to dangerous goods being shipped
- tariff_code string? - Tariff code for International orders declarations
- quantity int? - The number of products ordered.
- price decimal? - Unit price of each product line item. Used in the packing slip and customs declarations.
shippit: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
shippit: Quote
A quote returned by a carrier.
Fields
- delivery_date string? - Estimated date of delivery for the order
- delivery_window string? - For priority orders, machine-readable estimated range of times when the delivery could take place.
- delivery_window_desc string? - For priority orders, human-readable estimated range of times when the delivery could take place.
- price decimal? - Amount quoted by the carrier.
- courier_type string? -
optional
If returned inside a quote response for a service level (e.g.standard
), the carrier returning the quote. May be omitted if a specific carrier was requested.
- estimated_transit_time string? - Estimated amount of time for the quote to arrive.
shippit: QuoteErrorResponse
Request-level error response for the Quote API.
Fields
- success boolean? - Returns
false
.
- Fields Included from *GenericErrorResponse
shippit: QuoteRequest
Represents a request for carrier Quotes from Shippit.
Fields
- quote QuoteRequestDetails - A specification of the queries to include in the quote.
shippit: QuoteRequestDetails
A specification of the queries to include in the quote.
Fields
- dropoff_postcode string - destination postcode for the quote
- dropoff_state string? - destination state for the quote. Required when
dropoff_postcode
is unassigned or set toAU
- dropoff_suburb string - The dropoff suburb
- parcel_attributes Parcel[] - An array of parcel specifications to be included in the quote.
Each item can be used to specify the qty, dimensions, and other information about the products to be shipped, and the parcel used to ship it.
At minimum, only the
qty
andweight
fields are required. There is a maximum of 1000 parcels per quote request.
- order_date string? - delivery date for the order, required for
priority
orders
- return_all_quotes boolean? - If set to
true
, the system returns all quotes instead of cheapest/fastest quote. Defaults to false.
- dutiable_amount decimal? -
conditional
Declared value for customs when shipping international. Mandatory for International orders.
- dropoff_country_code string? - The destination country code for the quote in
ISO 3166 Alpha-2
format. If unassigned, defaults to the country code of the merchant's primary location, or otherwise 'AU'
shippit: QuoteResponse
Represents a response to an QuoteRequest, which shows either the generated Quote object or an error code with a description of the error.
Fields
- response QuoteResult[]? - The Quote returned by the carrier
- count decimal? - Number of quote results returned.
- Fields Included from *DataResponse
- anydata...
shippit: QuoteResult
Represents a Quote returned by a courier
Fields
- courier_type string? - The name of the courier or service level giving the quote.
- 'error string? - Error response, if any, received from the carrier(s) during quoting.
- quotes Quote[]? - List of quotes returned for the service level or carrier.
- service_level string? - The service level associated with this quote or group of quotes.
- success boolean? - Whether or not this service level was able to obtain a valid quote from the carrier(s).
shippit: TrackingErrorResponse
Error response returned in tracking requests
Fields
- 'error TrackingErrorResponseDetails - A Hash of error codes returned by tracking response. Each error code is returned as a key, with an array of strings for each instance of the error.
- success boolean? - Returns
false
- error_description string? - Human-readable description of error encountered.
- Fields Included from *ErrorResponse
- error anydata
- error_description string
- anydata...
shippit: TrackingErrorResponseDetails
A Hash of error codes returned by tracking response.
Each error code is returned as a key, with an array of strings for each instance of the error.
Fields
- order_id string[]? - Indicates an order that cannot be found.
shippit: TrackingHistory
An event that tracks a change in status
of a parcel's journey to fulfillment, with a timestamp
of when the event happened.
This can be either be provided by the merchant as part of a tracking order request, or returned by a track request.
Fields
- status string - The status of the order
- timestamp string - The date / time when the status change occurred.
shippit: TrackingResponse
Represents a response to the Tracking API, which provides tracking info for a specific order.
If successful, will return a response object with tracking information. Otherwise, will return an error code at the top-level.
Fields
- response TrackingResponseOrder? - Order details returned by a tracking request
- Fields Included from *DataResponse
- anydata...
shippit: TrackingResponseOrder
Order details returned by a tracking request
Fields
- tracking_number string? - Tracking number of the Order
- tracking_url string? - URL presenting tracking info for the Order. This can be shown to the recipient to give them status updates on the Order.
- success boolean? - Whether or not this tracking request was successful - should always be
true
as tracking errors will be returned at the top-level response.
- 'error string? - Errors associated with the tracking request - should always be
null
as tracking errors will be returned at the top-level response.
- track TrackingResponseTrackingHistory[]? - A list of historical tracking statuses for the Order. This will at minimum list the order status, along with the date and time when it changed, and who was responsible for the status change.
shippit: UserAttributes
Represents the customers details attached to the order.
Fields
- email string - the customer's email address
- first_name string - the customer's first name. If
last_name
is not provided, then it is used as the customer's full name.
- last_name string? - customer's last name
- mobile string? - Mobile number of the user / receiver.
shippit: WebhookRequest
Fields
- tracking_number string? -
- tracking_url string? -
- current_state string? - Possible enumerations are
await_collection
,awaiting_collection
,awaiting_drop_off
,cancelled
,completed
,parcel_completed
,partially_completed
,customs_awaiting_paym
,customs_failed
,customs_on_hold
,damaged
,delivery_attempted
,delivery_failed
,in_transit
,insufficient_address
,invalidated
,lost
,pickup_failed
,processing
,ready_for_pickup
,return_booked
,return_booking_failed
,return_requested
,returned_to_sender
,untrackable
,with_customs
,with_driver
- retailer_order_number string? -
- courier_name string? -
- courier_job_id string? -
- delivery_address string? -
- delivery_suburb string? -
- delivery_postcode string? -
- delivery_state string? -
- merchant_url string? -
- status_history WebhookrequestStatusHistory[]? -
- products WebhookrequestProducts[]? -
shippit: WebhookrequestProducts
Fields
- quantity int? -
- sku string? -
- title string? -
- product_line_id string? -
shippit: WebhookrequestStatusHistory
Fields
- status string? -
- time string? -
Import
import ballerinax/shippit;
Metadata
Released date: about 2 years ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 5
Current verison: 5
Weekly downloads
Keywords
Commerce/eCommerce
Cost/Paid
Contributors
Dependencies