servicenow
Module servicenow
API
Definitions
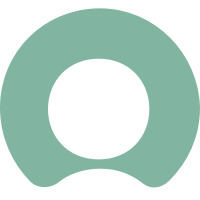
ballerinax/servicenow Ballerina library
Overview
This is a generated connector for ServiceNow REST API Quebec version OpenAPI Specification.
ServiceNow provides extensive access to instances through a set of RESTful APIs.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
-
Create a ServiceNow instance (If you already have one, you can use that).
-
Obtain tokens - Follow this guide.
Quickstart
To use the ServiceNow
connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
Import the ballerinax/servicenow module into the Ballerina project.
import ballerinax/servicenow;
Step 2: Create a new connector instance.
You can now make the connection configuration using either one of the following, and initialize the connector with it.
- The OAuth2 refresh token grant config.
ClientConfig clientConfig = { auth : { refreshUrl : "<YOUR INSTANCE URL>/oauth_token", refreshToken : "<REFRESH TOKEN>", clientId : "<CLIENT ID>", clientSecret : "<CLIENT SECRET>" } }; ervicenow:Client servicenowClient = check new (configuration, serviceUrl = <YOUR INSTANCE URL>);
- The bearer token config.
ClientConfig clientConfig = { auth : { token : "<YOUR ACCESS TOKEN>" } }; ervicenow:Client servicenowClient = check new (configuration, serviceUrl = <YOUR INSTANCE URL>);
- The credentials config (with username and password).
ClientConfig clientConfig = { auth : { username: "<USERNAME>", password: "<PASSWORD>" } }; ervicenow:Client servicenowClient = check new (configuration, serviceUrl = <YOUR INSTANCE URL>);
Step 3: Invoke connector operation
- Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to insert a record to a table using
ballerinax/servicenow
connector.
public function main() returns error? { string tableName = "incident json payload = { "short_description":"Unable to connect to office wifi", "assignment_group":"287ebd7da9fe198100f92cc8d1d2154e", "urgency":"2", "impact":"2"}; json response = check servicenowClient->createRecord(tableName, payload); }
- Use
bal run
command to compile and run the Ballerina program.
Clients
servicenow: Client
This is a generated connector for [ServiceNow REST API Quebec version] (https://developer.servicenow.com/dev.do#!/reference/api/quebec/rest) OpenAPI Specification. ServiceNow provides extensive access to instances through a set of RESTful APIs.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a ServiceNow instance and obtain tokens by following this guide.
init (ClientConfig clientConfig, string serviceUrl)
- clientConfig ClientConfig - The configurations to be used when initializing the
connector
- serviceUrl string - URL of the target service
getRecordList
function getRecordList(string tableName, string? nameValuePairs, string? sysparmDisplayValue, boolean? sysparmExcludeReferenceLink, string? sysparmFields, decimal? sysparmLimit, boolean? sysparmNoCount, decimal? sysparmOffset, string? sysparmQuery, string? sysparmQueryCategory, boolean? sysparmQueryNoDomain, boolean? sysparmSuppressPaginationHeader, string? sysparmView, string? accept) returns json|error
Retrieves multiple records for the specified table.
Parameters
- tableName string - Name of the table from which to retrieve the record. <i>Example: Incident
- nameValuePairs string? (default ()) - Name-value pairs to use to filter the result set. This parameter is mutually exclusive with sysparm_query.
- sysparmDisplayValue string? (default ()) - Data retrieval operation for reference and choice fields.
- sysparmExcludeReferenceLink boolean? (default ()) - Flag that indicates whether to exclude Table API links for reference fields
- sysparmFields string? (default ()) - Comma-separated list of fields to return in the response.
- sysparmLimit decimal? (default ()) - Maximum number of records to return. For requests that exceed this number of records, use the sysparm_offset parameter to paginate record retrieval. Note - Unusually large sysparm_limit values can impact system performance.
- sysparmNoCount boolean? (default ()) - Flag that indicates whether to execute a select count(*) query on the table to return the number of rows in the associated table.
- sysparmOffset decimal? (default ()) - Starting record index for which to begin retrieving records. Use this value to paginate record retrieval. This functionality enables the retrieval of all records, regardless of the number of records, in small manageable chunks.
- sysparmQuery string? (default ()) - Encoded query used to filter the result set. Syntax - sysparm_query=<col_name><operator><value>.
- sysparmQueryCategory string? (default ()) - Name of the category to use for queries.
- sysparmQueryNoDomain boolean? (default ()) - Flag that indicates whether to restrict the record search to only the domains for which the logged in user is configured.
- sysparmSuppressPaginationHeader boolean? (default ()) - Flag that indicates whether to remove the Link header from the response. The Link header provides various URLs to relative pages in the record set which you can use to paginate the returned record set.
- sysparmView string? (default ()) - UI view for which to render the data. Determines the fields returned in the response.
- accept string? (default ()) - Data format of the response body.
Return Type
- json|error - If successful, returns a JSON object that includes all records of the table. Otherwise returns the relevant error.
createRecord
function createRecord(string tableName, json payload, string? sysparmDisplayValue, boolean? sysparmExcludeReferenceLink, string? sysparmFields, boolean? sysparmInputDisplayValue, string? sysparmView, string? accept, string? contentType, string? xNoResponseBody) returns json|error
Inserts one record in the specified table. Multiple record insertion is not supported by this method.
Parameters
- tableName string - Name of the table in which to save the record.
- payload json - Field name and the associated value for each parameter to define in the specified record in JSON format.
- sysparmDisplayValue string? (default ()) - Data retrieval operation for reference and choice fields.
- sysparmExcludeReferenceLink boolean? (default ()) - Flag that indicates whether to exclude Table API links for reference fields
- sysparmFields string? (default ()) - Comma-separated list of fields to return in the response.
- sysparmInputDisplayValue boolean? (default ()) - Flag that indicates whether to set field values using the display value or the actual value.
- sysparmView string? (default ()) - UI view for which to render the data. Determines the fields returned in the response.
- accept string? (default ()) - Data format of the response body.
- contentType string? (default ()) - Data format of the request body. Supported types - application/json - application/xml.
- xNoResponseBody string? (default ()) - By default, responses include body content detailing the new record. Set this header to true in the request to suppress the response body.
Return Type
- json|error - If successful, returns a JSON object that includes created record of the table. Otherwise returns the relevant error.
getRecordById
function getRecordById(string tableName, string sysId, string? sysparmDisplayValue, boolean? sysparmExcludeReferenceLink, string? sysparmFields, boolean? sysparmQueryNoDomain, string? sysparmView, string? accept) returns json|error
Retrieves the record identified by the specified sys_id from the specified table.
Parameters
- tableName string - Name of the table from which to retrieve the record. <i>Example: Incident
- sysId string - Sys_id of the record to retrieve <i>Example: b7a00a202f1030105b5b2b5df699b618
- sysparmDisplayValue string? (default ()) - Data retrieval operation for reference and choice fields.
- sysparmExcludeReferenceLink boolean? (default ()) - Flag that indicates whether to exclude Table API links for reference fields
- sysparmFields string? (default ()) - Comma-separated list of fields to return in the response. In the use case of query incidents those fields are number,sys_id,sys_created_on,cmdb_ci,correlation_id,state,assignment_group,short_description,description,close_code,close_notes <i>Example: number,sys_id,sys_created_on,cmdb_ci,correlation_id,state,assignment_group,short_description,description,close_code,close_notes
- sysparmQueryNoDomain boolean? (default ()) - Flag that indicates whether to restrict the record search to only the domains for which the logged in user is configured.
- sysparmView string? (default ()) - UI view for which to render the data. Determines the fields returned in the response.
- accept string? (default ()) - Data format of the response body.
Return Type
- json|error - If successful, returns a JSON object that includes specified record of the table. Otherwise returns the relevant error.
updateRecord
function updateRecord(string tableName, string sysId, json payload, string? sysparmDisplayValue, boolean? sysparmExcludeReferenceLink, string? sysparmFields, boolean? sysparmInputDisplayValue, boolean? sysparmQueryNoDomain, string? sysparmView, string? accept, string? contentType, string? xNoResponseBody) returns json|error
Updates the specified record with the request body.
Parameters
- tableName string - Name of the table in which the record is located.
- sysId string - Unique identifier of the record to update.
- payload json - Name-value pairs for the field(s) to update in the associated table in JSON format.
- sysparmDisplayValue string? (default ()) - Data retrieval operation for reference and choice fields.
- sysparmExcludeReferenceLink boolean? (default ()) - Flag that indicates whether to exclude Table API links for reference fields
- sysparmFields string? (default ()) - Comma-separated list of fields to return in the response.
- sysparmInputDisplayValue boolean? (default ()) - Flag that indicates whether to set field values using the display value or the actual value.
- sysparmQueryNoDomain boolean? (default ()) - Flag that indicates whether to restrict the record search to only the domains for which the logged in user is configured.
- sysparmView string? (default ()) - UI view for which to render the data. Determines the fields returned in the response.
- accept string? (default ()) - Data format of the response body.
- contentType string? (default ()) - Data format of the request body. Supported types - application/json - application/xml.
- xNoResponseBody string? (default ()) - By default, responses include body content detailing the new record. Set this header to true in the request to suppress the response body.
Return Type
- json|error - If successful, returns a JSON object that includes updated record of the table. Otherwise returns the relevant error.
deleteRecord
function deleteRecord(string tableName, string sysId, boolean? sysparmQueryNoDomain, string? accept) returns Response|error
Deletes the specified record from the specified table.
Parameters
- tableName string - Name of the table from which to delete the specified record, such as "incident" or "asset".
- sysId string - Sys_id of the record to delete.
- sysparmQueryNoDomain boolean? (default ()) - Flag that indicates whether to restrict the record search to only the domains for which the logged in user is configured.
- accept string? (default ()) - Data format of the response body.
patchRecordById
function patchRecordById(string tableName, string sysId, json payload, string? sysparmDisplayValue, string? sysparmFields, boolean? sysparmInputDisplayValue, boolean? sysparmQueryNoDomain, string? sysparmView, string? accept, string? contentType, string? xNoResponseBody) returns json|error
Updates the specified record with the name-value pairs included in the request body.
Parameters
- tableName string - Name of the table in which to the specified record is located.
- sysId string - Sys_id of the record to update.
- payload json - Field name and the associated value for each parameter to define in the specified record in JSON format.
- sysparmDisplayValue string? (default ()) - Data retrieval operation for reference and choice fields.
- sysparmFields string? (default ()) - Comma-separated list of fields to return in the response.
- sysparmInputDisplayValue boolean? (default ()) - Flag that indicates whether to set field values using the display value or the actual value.
- sysparmQueryNoDomain boolean? (default ()) - Flag that indicates whether to restrict the record search to only the domains for which the logged in user is configured.
- sysparmView string? (default ()) - UI view for which to render the data. Determines the fields returned in the response.
- accept string? (default ()) - Data format of the response body.
- contentType string? (default ()) - Data format of the request body. Supported types - application/json - application/xml.
- xNoResponseBody string? (default ()) - By default, responses include body content detailing the new record. Set this header to true in the request to suppress the response body.
Return Type
- json|error - If successful, returns a JSON object that includes updated record of the table. Otherwise returns the relevant error.
aggregate
function aggregate(string tableName, string? nameValuePairs, string? sysparmAvgFields, string? sysparmMinFields, string? sysparmMaxFields, string? sysparmSumFields, string? sysparmCount, string? sysparmDisplayValue, string? sysparmGroupBy, string? sysparmHaving, string? sysparmOrderby, string? sysparmQuery, string? accept) returns json|error
Retrieves records for the specified table and performs aggregate functions on the returned values.
Parameters
- tableName string - Name of the table for which to retrieve records.
- nameValuePairs string? (default ()) - An alternative to using the sysparm_query parameter. You can filter a query using key-value pairs where the key is the name of a field.
- sysparmAvgFields string? (default ()) - List of fields on which to perform avg aggregate operation. You can specify multiple fields by separating each with a comma.
- sysparmMinFields string? (default ()) - List of fields on which to perform min aggregate operation. You can specify multiple fields by separating each with a comma.
- sysparmMaxFields string? (default ()) - List of fields on which to perform max aggregate operation. You can specify multiple fields by separating each with a comma.
- sysparmSumFields string? (default ()) - List of fields on which to perform sum aggregate operation. You can specify multiple fields by separating each with a comma.
- sysparmCount string? (default ()) - Flag that determines whether to return the number of records returned by the query.
- sysparmDisplayValue string? (default ()) - Data retrieval operation when grouping by reference or choice fields. Based on this value, the query returns either the display value, the actual value in the database, or both.
- sysparmGroupBy string? (default ()) - Fields by which to group the returned data. You can specify multiple fields by separating each field with a comma, such as sysparm_group_by=priority,state.
- sysparmHaving string? (default ()) - Additional query that enables you to filter the data based on an aggregate operation. The value for this parameter must follow the syntax aggregate^field^operator^value, such as count^priority^>^3 to obtain the number of records within the query results with a priority greater than 3. You can specify multiple queries by separating each with a comma, such ascount^state^=^1,avg^priority^>^3.
- sysparmOrderby string? (default ()) - Data retrieval operation when grouping by reference or choice fields. Based on this value, the query returns either the display value, the actual value in the database, or both.
- sysparmQuery string? (default ()) - An encoded query. For example- (sysparm_query=active=true)(sysparm_query=caller_id=javascript:gs.getUserID()^active=true)
- accept string? (default ()) - Data format of the response body.
Return Type
- json|error - If successful, returns a JSON object depends on specified table and specified request parameters. Otherwise returns the relevant error.
getImportSet
Retrieves the specified import staging record and resulting transformation result.
Parameters
- stagingTableName string - Name of the table from which to obtain the import data.
- sysId string - Sys_id of the record that contains the data.
- accept string? (default ()) - Data format of the response body.
Return Type
- json|error - If successful, returns a JSON object. Otherwise returns the relevant error.
createImportSet
function createImportSet(string stagingTableName, json payload, string? accept, string? contentType) returns json|error
Inserts incoming data into a specified staging table and triggers transformation based on predefined transform maps in the import set table.
Parameters
- stagingTableName string - Name of the table from which to import the data.
- payload json - Name-value pairs to insert in the import fields in JSON format.
- accept string? (default ()) - Data format of the response body.
- contentType string? (default ()) - Data format of the request body.
Return Type
- json|error - If successful, returns a JSON object. Otherwise returns the relevant error.
createMultipleImportSet
function createMultipleImportSet(string stagingTableName, json payload, string? multiImportSetId, string? runAfter, string? accept, string? contentType) returns json|error
Inserts multiple records into a specified staging table and triggers transformation based on predefined transform maps or Robust Transform Engine (RTE) configurations in a single request.
Parameters
- stagingTableName string - Name of the table from which to import the data.
- payload json - Name-value pairs to insert in the import fields in JSON format.
- multiImportSetId string? (default ()) - Sys_id of an entry in the Multi Import Sets [sys_multi_import_set] table. If specified, adds the current import to this multiple import set instead of adding to a new multiple import set.
- runAfter string? (default ()) - Sys_id of an entry in the Import Sets [sys_import_set] table. Enables running the current import set after the specified import set is complete. You can use this parameter to enforce the sequential order of imports. This parameter is only valid in asynchronous transformations.
- accept string? (default ()) - Data format of the response body.
- contentType string? (default ()) - Data format of the request body.
Return Type
- json|error - If successful, returns a JSON object. Otherwise returns the relevant error.
getCaseById
function getCaseById(string id, string? sysparmActivityType, decimal? sysparmLimit, decimal? sysparmOffset, string? accept) returns json|error
Retrieves the activity stream for a specified Customer Service Management (CSM) case.
Parameters
- id string - Sys_id or case number of the case to retrieve. Located in the Case [sn_customerservice_case] table. Results will be unpredictable if a specified case number is shared by two or more cases.
- sysparmActivityType string? (default ()) - Filters the type of activities to return from the activity stream. The entries.element parameter contains the activity type for each activity.
- sysparmLimit decimal? (default ()) - Maximum number of records to return. Unusually large sysparm_limit values can impact system performance. For requests that exceed this number of records, use the sysparm_offset parameter to paginate record retrieval.
- sysparmOffset decimal? (default ()) - Starting record index for which to begin retrieving records. Use this value to paginate record retrieval. This functionality enables the retrieval of all records, regardless of the number of records, in small manageable chunks.
- accept string? (default ()) - Data format of the response body.
Return Type
- json|error - If successful, returns a JSON object. Otherwise returns the relevant error.
getValuesByFieldNameInCase
function getValuesByFieldNameInCase(string fieldName, string? sysparmDependentValue, decimal? sysparmLimit, decimal? sysparmOffset, string? sysparmReferenceFieldColumns, string? sysparmQuery, string? sysparmRefQualInput, string? accept) returns json|error
Retrieves the list of possible field values for a choice or reference field in the Case [sn_customerservice_case] table.
Parameters
- fieldName string - Name of a choice or reference field in the Case [sn_customerservice_case] table.
- sysparmDependentValue string? (default ()) - Value to select in the Case [sn_customerservice_case] table choice field that the requested field is dependent on. Use only when requesting a choice field that is dependent on another field.
- sysparmLimit decimal? (default ()) - Maximum number of records to return. Unusually large sysparm_limit values can impact system performance. For requests that exceed this number of records, use the sysparm_offset parameter to paginate record retrieval.
- sysparmOffset decimal? (default ()) - Starting record index for which to begin retrieving records. Use this value to paginate record retrieval. This functionality enables the retrieval of all records, regardless of the number of records, in small manageable chunks.
- sysparmReferenceFieldColumns string? (default ()) - Comma-separated list of column names, from the table of the specified reference field, to return in the response.
- sysparmQuery string? (default ()) - Encoded query used to filter the result set. Queries defined using this parameter are appended to any encoded queries produced by reference qualifiers.
- sysparmRefQualInput string? (default ()) - Encoded set of field values representing a current object to pass to reference qualifiers that use JavaScript functions. Use only when requesting a reference field.
- accept string? (default ()) - Data format of the response body.
Return Type
- json|error - If successful, returns a JSON object. Otherwise returns the relevant error.
getValuesByCaseIdAndFieldName
function getValuesByCaseIdAndFieldName(string fieldName, string id, string? sysparmDependentValue, decimal? sysparmLimit, decimal? sysparmOffset, string? sysparmReferenceFieldColumns, string? sysparmQuery, string? sysparmRefQualInput, string? accept) returns json|error
Retrieves the list of possible field values for a choice or reference field for a specified record in the Case [sn_customerservice_case] table.
Parameters
- fieldName string - Name of a choice or reference field in the Case [sn_customerservice_case] table.
- id string - Sys_id of a record. Located in the Case [sn_customerservice_case] table to use when evaluating reference qualifiers.
- sysparmDependentValue string? (default ()) - Value to select in the Case [sn_customerservice_case] table choice field that the requested field is dependent on. Use only when requesting a choice field that is dependent on another field.
- sysparmLimit decimal? (default ()) - Maximum number of records to return. Unusually large sysparm_limit values can impact system performance. For requests that exceed this number of records, use the sysparm_offset parameter to paginate record retrieval.
- sysparmOffset decimal? (default ()) - Starting record index for which to begin retrieving records. Use this value to paginate record retrieval. This functionality enables the retrieval of all records, regardless of the number of records, in small manageable chunks.
- sysparmReferenceFieldColumns string? (default ()) - Comma-separated list of column names, from the table of the specified reference field, to return in the response.
- sysparmQuery string? (default ()) - Encoded query used to filter the result set. Queries defined using this parameter are appended to any encoded queries produced by reference qualifiers.
- sysparmRefQualInput string? (default ()) - Encoded set of field values representing a current object to pass to reference qualifiers that use JavaScript functions. Use only when requesting a reference field.
- accept string? (default ()) - Data format of the response body.
Return Type
- json|error - If successful, returns a JSON object. Otherwise returns the relevant error.
getCSMCaseById
Retrieves the specified Customer Service Management (CSM) case.
Parameters
- id string - Sys_id or case number for the case to retrieve. Located in the Case [sn_customerservice_case] table. Results will be unpredictable if a specified case number is shared by two or more cases.
- sysparmDisplayValue string? (default ()) - Data retrieval operation for reference and choice fields. Based on this value, retrieves the display value and/or the actual value from the database.
- accept string? (default ()) - Data format of the response body.
Return Type
- json|error - If successful, returns a JSON object. Otherwise returns the relevant error.
updateCSMCase
function updateCSMCase(string id, json payload, string? sysparmDisplayValue, string? sysparmFields, string? sysparmGetCaseDetails, string? accept, string? contentType) returns json|error
Updates the specified existing Customer Service Management (CSM) case with the passed-in parameters.
Parameters
- id string - Sys_id of the case to update. Located in the Case [sn_customerservice_case] table.
- payload json - Field name and the associated value for each parameter to define in the specified record in JSON format.
- sysparmDisplayValue string? (default ()) - Data retrieval operation for reference and choice fields. Based on this value, retrieves the display value and/or the actual value from the database.
- sysparmFields string? (default ()) - Comma-separated list of fields to return in the response.
- sysparmGetCaseDetails string? (default ()) - Flag that indicates whether to return the updated case record.
- accept string? (default ()) - Data format of the response body.
- contentType string? (default ()) - Data format of the request body.
Return Type
- json|error - If successful, returns a JSON object. Otherwise returns the relevant error.
getCSMCaseList
function getCSMCaseList(string? sysparmDisplayValue, string? sysparmFields, decimal? sysparmLimit, decimal? sysparmOffset, string? sysparmQuery, boolean? sysparmReferenceFields, string? accept) returns json|error
Retrieves a specified set of Customer Service Management (CSM) cases.
Parameters
- sysparmDisplayValue string? (default ()) - Data retrieval operation for reference and choice fields. Based on this value, retrieves the display value and/or the actual value from the database.
- sysparmFields string? (default ()) - Comma-separated list of fields to return in the response.
- sysparmLimit decimal? (default ()) - Maximum number of records to return. Unusually large sysparm_limit values can impact system performance. For requests that exceed this number of records, use the sysparm_offset parameter to paginate record retrieval.
- sysparmOffset decimal? (default ()) - Starting record index for which to begin retrieving records. Use this value to paginate record retrieval. This functionality enables the retrieval of all records, regardless of the number of records, in small manageable chunks.
- sysparmQuery string? (default ()) - Encoded query used to filter the result set.
- sysparmReferenceFields boolean? (default ()) - Whether or not to retrieve fields from reference tables such as account, contact, consumer, and sn_app_cs_social_social_profile. By default, these fields are returned if the user has read access to them, but this adds performance overhead. When retrieving a large number of case records, this overhead can be eliminated by setting sysparm_reference_fields=false.
- accept string? (default ()) - Data format of the response body.
Return Type
- json|error - If successful, returns a JSON object. Otherwise returns the relevant error.
createCSMCase
Creates a new Customer Service Management (CSM) case.
Parameters
- payload json - Field name and the associated value for each parameter to define in the specified record in JSON format.
- accept string? (default ()) - Data format of the response body.
- contentType string? (default ()) - Data format of the request body.
Return Type
- json|error - If successful, returns a JSON object. Otherwise returns the relevant error.
getCSMContactList
function getCSMContactList(decimal? sysparmLimit, decimal? sysparmOffset, string? sysparmQuery, string? accept) returns json|error
Retrieves a specified set of Customer Service Management (CSM) contacts.
Parameters
- sysparmLimit decimal? (default ()) - Maximum number of records to return. Unusually large sysparm_limit values can impact system performance. For requests that exceed this number of records, use the sysparm_offset parameter to paginate record retrieval.
- sysparmOffset decimal? (default ()) - Starting record index for which to begin retrieving records. Use this value to paginate record retrieval. This functionality enables the retrieval of all records, regardless of the number of records, in small manageable chunks.
- sysparmQuery string? (default ()) - Encoded query used to filter the result set.
- accept string? (default ()) - Data format of the response body.
Return Type
- json|error - If successful, returns a JSON object. Otherwise returns the relevant error.
createCSMContact
Creates a new Customer Service Management (CSM) contact.
Parameters
- payload json - Field name and the associated value for each parameter to define in the specified record in JSON format.
- accept string? (default ()) - Data format of the response body.
- contentType string? (default ()) - Data format of the request body.
Return Type
- json|error - If successful, returns a JSON object that includes result. Otherwise returns the relevant error.
getCSMContactById
Retrieves the specified Customer Service Management (CSM) contact.
Parameters
- id string - Sys_id of the contact to retrieve. Located in the Contact [customer_contact] table.
- accept string? (default ()) - Data format of the response body.
Return Type
- json|error - If successful, returns a JSON object. Otherwise returns the relevant error.
Records
servicenow: ClientConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig|CredentialsConfig - Configurations related to client authentication
- httpVersion string(default "1.1") - The HTTP version understood by the client
- http1Settings ClientHttp1Settings(default {}) - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings(default {}) - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- followRedirects FollowRedirects?(default ()) - Configurations associated with Redirection
- poolConfig PoolConfiguration?(default ()) - Configurations associated with request pooling
- cache CacheConfig(default {}) - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig?(default ()) - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig?(default ()) - Configurations associated with retrying
- cookieConfig CookieConfig?(default ()) - Configurations associated with cookies
- responseLimits ResponseLimitConfigs(default {}) - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket?(default ()) - SSL/TLS-related options
Import
import ballerinax/servicenow;
Metadata
Released date: over 2 years ago
Version: 1.1.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: slbeta6
GraalVM compatible: Yes
Pull count
Total: 2377
Current verison: 5
Weekly downloads
Keywords
Support/Customer Support
Cost/Freemium
Contributors
Dependencies