sendgrid
Module sendgrid
API
Definitions
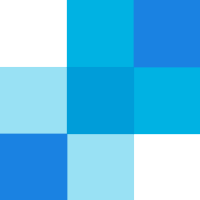
ballerinax/sendgrid Ballerina library
Overview
This is a generated connector for Sendgrid API v3 OpenAPI Specification.
The Beta endpoints for the new Email Activity APIs - functionality is subject to change without notice. You may not have access to this Beta endpoint.
Email Activity offers filtering and search by event type for two days worth of data. There is an optional add-on to store 60 days worth of data. This add-on also gives you access to the ability to download a CSV of the 60 days worth of email event data.
Configuring connector
Prerequisites
- Vist Sendgrid and create an account.
- Obtain token
- Obtain API Key visiting Settings -> APIKeys and provide obtained API Key as the token at HTTP client initialization.
- Configure required permissions when generating the API Key.
Quickstart
To use the Sendgrid connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/sendgrid module into the Ballerina project.
import ballerinax/sendgrid;
Step 2: Create a new connector instance
Configure the connection using the API-Key obtained.
sendgrid:ClientConfig configuration = { auth: { token: "<API-Key>" } }; sendgrid:Client sendgridClient = check new (configuration);
Step 3: Invoke connector operation
- send a marketing email to a customer.
public function main() returns error? { sendgrid:SendMailRequest emailPayload = { personalizations : [{ to: [{ email: "<Receiver-Email>", name: "<Receiver-Name>" }] }], 'from: { email: "<Sender-Email>", name: "<Sender-Name>" }, content : [{ 'type: "text/plain", value: "<Email-Content>" }], subject: "<Email-Subject>" }; _ = check sendgridClient->sendMail(emailPayload); }
- Use
bal run
command to compile and run the Ballerina program.
Clients
sendgrid: Client
This is a generated connector for Sendgrid API v3 OpenAPI Specification. The Beta endpoints for the new Email Activity APIs - functionality is subject to change without notice. You may not have access to this Beta endpoint. Email Activity offers filtering and search by event type for two days worth of data. There is an optional add-on to store 60 days worth of data. This add-on also gives you access to the ability to download a CSV of the 60 days worth of email event data.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Sendgrid account and obtain an API Key visiting Settings -> APIKeys. Use the obtained API Key as the token at connector initialization. Configure required permissions when generating the API Key.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.sendgrid.com/v3" - URL of the target service
sendMail
function sendMail(SendEmailRequest payload) returns Response|error
Send Mail
Parameters
- payload SendEmailRequest - Mail content
getAlerts
function getAlerts(string? onBehalfOf) returns AlertResponse[]|error
Retrieve all alerts
Parameters
- onBehalfOf string? (default ()) - The subuser's username. This header generates the API call as if the subuser account was making the call.
Return Type
- AlertResponse[]|error - Details related to alerts
postAlerts
function postAlerts(PostAlertsRequest payload, string? onBehalfOf) returns PostAlertsResponse|error
Create a new Alert
Parameters
- payload PostAlertsRequest - Alert Content
- onBehalfOf string? (default ()) - The subuser's username. This header generates the API call as if the subuser account was making the call.
Return Type
- PostAlertsResponse|error - Created alert details
deleteAlertById
Delete an alert
Parameters
- alertId int - The ID of the alert you would like to retrieve.
- onBehalfOf string? (default ()) - The subuser's username. This header generates the API call as if the subuser account was making the call.
updateAlertbyId
function updateAlertbyId(int alertId, UpdateAlertbyIdRequest payload, string? onBehalfOf) returns UpdateAlertbyIdResponse|error
Update an alert
Parameters
- alertId int - The ID of the alert you would like to retrieve.
- payload UpdateAlertbyIdRequest - Alert content to update
- onBehalfOf string? (default ()) - The subuser's username. This header generates the API call as if the subuser account was making the call.
Return Type
- UpdateAlertbyIdResponse|error - Updated alert details
getSubusers
List all Subusers
Parameters
- username string? (default ()) - The username of this subuser.
- 'limit int? (default ()) - The number of results you would like to get in each request.
- offset int? (default ()) - The number of subusers to skip.
postSubusers
function postSubusers(PostSubusersRequest payload) returns SubuserPost|error
Create Subuser
Parameters
- payload PostSubusersRequest - New subuser details
Return Type
- SubuserPost|error - Created subuser's details
deleteSubuserByName
Delete a subuser
Parameters
- subuserName string - Subuser name
getSuppressionBlocks
function getSuppressionBlocks(int? startTime, int? endTime, int? 'limit, int? offset, string? onBehalfOf) returns SuppressionBlocks[]|error
Retrieve all blocks
Parameters
- startTime int? (default ()) - Refers start of the time range in unix timestamp when a blocked email was created (inclusive).
- endTime int? (default ()) - Refers end of the time range in unix timestamp when a blocked email was created (inclusive).
- 'limit int? (default ()) - Limit the number of results to be displayed per page.
- offset int? (default ()) - The point in the list to begin displaying results.
- onBehalfOf string? (default ()) - The subuser's username. This header generates the API call as if the subuser account was making the call.
Return Type
- SuppressionBlocks[]|error - List of all blocks
getSuppressionSpamReports
function getSuppressionSpamReports(int? startTime, int? endTime, int? 'limit, int? offset, string? onBehalfOf) returns SpamReportDetails[]|error
Retrieve all spam reports
Parameters
- startTime int? (default ()) - Refers start of the time range in unix timestamp when a spam report was created (inclusive).
- endTime int? (default ()) - Refers end of the time range in unix timestamp when a spam report was created (inclusive).
- 'limit int? (default ()) - Limit the number of results to be displayed per page.
- offset int? (default ()) - Paging offset. The point in the list to begin displaying results.
- onBehalfOf string? (default ()) - The subuser's username. This header generates the API call as if the subuser account was making the call.
Return Type
- SpamReportDetails[]|error - Received spam reports
Records
sendgrid: AlertResponse
Alert details
Fields
- created_at int - A Unix timestamp indicating when the alert was created.
- email_to string - The email address that the alert will be sent to.
- frequency string? - If the alert is of type stats_notification, this indicates how frequently the stats notifications will be sent. For example, "daily", "weekly", or "monthly".
- id int - The ID of the alert.
- percentage int? - If the alert is of type usage_limit, this indicates the percentage of email usage that must be reached before the alert will be sent.
- 'type string - The type of alert.
- updated_at int? - A Unix timestamp indicating when the alert was last modified.
sendgrid: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
sendgrid: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
sendgrid: EmailObject
Email details
Fields
- email string - Email Address
- name string? - The name of the person to whom you are sending an email.
sendgrid: ErrorDetails
Error details
Fields
- 'field string? - The field that generated the error.
- message string - The error message.
sendgrid: Errors
List of errors
Fields
- errors ErrorsErrors[]? - Error message
sendgrid: ErrorsErrors
Fields
- 'field string? - The field that has the error.
- message string? - The message the API caller will receive.
sendgrid: GlobalErrorresponse
Global error response
Fields
- errors ErrorDetails[]? - Error details
sendgrid: InlineResponse400
Fields
- 'field string? -
- message string? -
sendgrid: PostAlertsRequest
Alert Object
Fields
- email_to string - The email address the alert will be sent to. Example: test@example.com
- frequency string? - Required for stats_notification. How frequently the alert will be sent. Example: daily
- percentage int? - Required for usage_alert. When this usage threshold is reached, the alert will be sent. Example: 90
- 'type string - The type of alert you want to create. Can be either usage_limit or stats_notification. Example: usage_limit
sendgrid: PostAlertsResponse
Fields
- created_at int - A Unix timestamp indicating when the alert was created.
- email_to string - The email address that the alert will be sent to.
- frequency string? - If the alert is of type stats_notification, this indicates how frequently the stats notifications will be sent. For example, "daily", "weekly", or "monthly".
- id int - The ID of the alert.
- percentage int? - If the alert is of type usage_limit, this indicates the percentage of email usage that must be reached before the alert will be sent.
- 'type string - The type of alert.
- updated_at int - A Unix timestamp indicating when the alert was last modified.
sendgrid: PostSubusersRequest
Fields
- email string - The email address of the subuser.
- ips string[] - The IP addresses that should be assigned to this subuser.
- password string - The password this subuser will use when logging into SendGrid.
- username string - The username for this subuser.
sendgrid: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
sendgrid: SendEmailRequest
Fields
- asm SendemailrequestAsm? - An object allowing you to specify how to handle unsubscribes.
- attachments SendemailrequestAttachments[]? - An array of objects in which you can specify any attachments you want to include.
- batch_id string? - This ID represents a batch of emails to be sent at the same time. Including a batch_id in your request allows you include this email in that batch, and also enables you to cancel or pause the delivery of that batch. For more information, see https://sendgrid.com/docs/API_Reference/Web_API_v3/cancel_schedule_send.html
- categories SendemailrequestCategoriesItemsString[]? - An array of category names for this message. Each category name may not exceed 255 characters.
- content SendemailrequestContent[] - An array in which you may specify the content of your email. You can include multiple mime types of content, but you must specify at least one mime type. To include more than one mime type, simply add another object to the array containing the
type
andvalue
parameters.
- custom_args record {}? - Values that are specific to the entire send that will be carried along with the email and its activity data. Substitutions will not be made on custom arguments, so any string that is entered into this parameter will be assumed to be the custom argument that you would like to be used. This parameter is overridden by personalizations[x].custom_args if that parameter has been defined. Total custom args size may not exceed 10,000 bytes.
- 'from EmailObject - Email details
- headers record {}? - An object containing key/value pairs of header names and the value to substitute for them. You must ensure these are properly encoded if they contain unicode characters. Must not be one of the reserved headers.
- ip_pool_name string? - The IP Pool that you would like to send this email from.
- mail_settings SendemailrequestMailSettings? - A collection of different mail settings that you can use to specify how you would like this email to be handled.
- personalizations SendemailrequestPersonalizations[] - An array of messages and their metadata. Each object within personalizations can be thought of as an envelope - it defines who should receive an individual message and how that message should be handled.
- reply_to EmailObject? - Email details
- sections record {}? - An object of key/value pairs that define block sections of code to be used as substitutions.
- send_at int? - A unix timestamp allowing you to specify when you want your email to be delivered. This may be overridden by the personalizations[x].send_at parameter. Scheduling more ta 72 hours in advance is forbidden.
- subject string - The global, or “message level”, subject of your email. This may be overridden by personalizations[x].subject.
- template_id string? - The id of a template that you would like to use. If you use a template that contains a subject and content (either text or html), you do not need to specify those at the personalizations nor message level.
- tracking_settings SendemailrequestTrackingSettings? - Settings to determine how you would like to track the metrics of how your recipients interact with your email.
sendgrid: SendemailrequestAsm
An object allowing you to specify how to handle unsubscribes.
Fields
- group_id int - The unsubscribe group to associate with this email.
- groups_to_display int[]? - An array containing the unsubscribe groups that you would like to be displayed on the unsubscribe preferences page.
sendgrid: SendemailrequestAttachments
Fields
- content string - The Base64 encoded content of the attachment.
- content_id string? - The content id for the attachment. This is used when the disposition is set to “inline” and the attachment is an image, allowing the file to be displayed within the body of your email.
- disposition string(default "attachment") - The content-disposition of the attachment specifying how you would like the attachment to be displayed. For example, “inline” results in the attached file being displayed automatically within the message while “attachment” results in the attached file requiring some action to be taken before it is displayed (e.g. opening or downloading the file).
- filename string - The filename of the attachment.
- 'type string? - The mime type of the content you are attaching. For example, “text/plain” or “text/html”.
sendgrid: SendemailrequestContent
Fields
- 'type string - The mime type of the content you are including in your email. For example, “text/plain” or “text/html”.
- value string - The actual content of the specified mime type that you are including in your email.
sendgrid: SendemailrequestMailSettings
A collection of different mail settings that you can use to specify how you would like this email to be handled.
Fields
- bcc SendemailrequestMailSettingsBcc? - This allows you to have a blind carbon copy automatically sent to the specified email address for every email that is sent.
- bypass_list_management SendemailrequestMailSettingsBypassListManagement? - Allows you to bypass all unsubscribe groups and suppressions to ensure that the email is delivered to every single recipient. This should only be used in emergencies when it is absolutely necessary that every recipient receives your email.
- footer SendemailrequestMailSettingsFooter? - The default footer that you would like included on every email.
- sandbox_mode SendemailrequestMailSettingsSandboxMode? - This allows you to send a test email to ensure that your request body is valid and formatted correctly.
- spam_check SendemailrequestMailSettingsSpamCheck? - This allows you to test the content of your email for spam.
sendgrid: SendemailrequestMailSettingsBcc
This allows you to have a blind carbon copy automatically sent to the specified email address for every email that is sent.
Fields
- email string? - The email address that you would like to receive the BCC.
- enable boolean? - Indicates if this setting is enabled.
sendgrid: SendemailrequestMailSettingsBypassListManagement
Allows you to bypass all unsubscribe groups and suppressions to ensure that the email is delivered to every single recipient. This should only be used in emergencies when it is absolutely necessary that every recipient receives your email.
Fields
- enable boolean? - Indicates if this setting is enabled.
sendgrid: SendemailrequestMailSettingsFooter
The default footer that you would like included on every email.
Fields
- enable boolean? - Indicates if this setting is enabled.
- html string? - The HTML content of your footer.
- text string? - The plain text content of your footer.
sendgrid: SendemailrequestMailSettingsSandboxMode
This allows you to send a test email to ensure that your request body is valid and formatted correctly.
Fields
- enable boolean? - Indicates if this setting is enabled.
sendgrid: SendemailrequestMailSettingsSpamCheck
This allows you to test the content of your email for spam.
Fields
- enable boolean? - Indicates if this setting is enabled.
- post_to_url string? - An Inbound Parse URL that you would like a copy of your email along with the spam report to be sent to.
- threshold int? - The threshold used to determine if your content qualifies as spam on a scale from 1 to 10, with 10 being most strict, or most likely to be considered as spam.
sendgrid: SendemailrequestPersonalizations
Fields
- bcc EmailObject[]? - An array of recipients who will receive a blind carbon copy of your email. Each object within this array may contain the name, but must always contain the email, of a recipient.
- cc EmailObject[]? - An array of recipients who will receive a copy of your email. Each object within this array may contain the name, but must always contain the email, of a recipient.
- custom_args record {}? - Values that are specific to this personalization that will be carried along with the email and its activity data. Substitutions will not be made on custom arguments, so any string that is entered into this parameter will be assumed to be the custom argument that you would like to be used. May not exceed 10,000 bytes.
- headers record {}? - A collection of JSON key/value pairs allowing you to specify specific handling instructions for your email. You may not overwrite the following headers: x-sg-id, x-sg-eid, received, dkim-signature, Content-Type, Content-Transfer-Encoding, To, From, Subject, Reply-To, CC, BCC
- send_at int? - A unix timestamp allowing you to specify when you want your email to be delivered. Scheduling more than 72 hours in advance is forbidden.
- subject string? - The subject of your email. Char length requirements, according to the RFC - http://stackoverflow.com/questions/1592291/what-is-the-email-subject-length-limit#answer-1592310
- substitutions record {}? - A collection of key/value pairs following the pattern "substitution_tag":"value to substitute". All are assumed to be strings. These substitutions will apply to the text and html content of the body of your email, in addition to the
subject
andreply-to
parameters.
- to EmailObject[] - An array of recipients. Each object within this array may contain the name, but must always contain the email, of a recipient.
sendgrid: SendemailrequestTrackingSettings
Settings to determine how you would like to track the metrics of how your recipients interact with your email.
Fields
- click_tracking SendemailrequestTrackingSettingsClickTracking? - Allows you to track whether a recipient clicked a link in your email.
- ganalytics SendemailrequestTrackingSettingsGanalytics? - Allows you to enable tracking provided by Google Analytics.
- open_tracking SendemailrequestTrackingSettingsOpenTracking? - Allows you to track whether the email was opened or not, but including a single pixel image in the body of the content. When the pixel is loaded, we can log that the email was opened.
- subscription_tracking SendemailrequestTrackingSettingsSubscriptionTracking? - Allows you to insert a subscription management link at the bottom of the text and html bodies of your email. If you would like to specify the location of the link within your email, you may use the substitution_tag.
sendgrid: SendemailrequestTrackingSettingsClickTracking
Allows you to track whether a recipient clicked a link in your email.
Fields
- enable boolean? - Indicates if this setting is enabled.
- enable_text boolean? - Indicates if this setting should be included in the text/plain portion of your email.
sendgrid: SendemailrequestTrackingSettingsGanalytics
Allows you to enable tracking provided by Google Analytics.
Fields
- enable boolean? - Indicates if this setting is enabled.
- utm_campaign string? - The name of the campaign.
- utm_content string? - Used to differentiate your campaign from advertisements.
- utm_medium string? - Name of the marketing medium. (e.g. Email)
- utm_source string? - Name of the referrer source. (e.g. Google, SomeDomain.com, or Marketing Email)
- utm_term string? - Used to identify any paid keywords.
sendgrid: SendemailrequestTrackingSettingsOpenTracking
Allows you to track whether the email was opened or not, but including a single pixel image in the body of the content. When the pixel is loaded, we can log that the email was opened.
Fields
- enable boolean? - Indicates if this setting is enabled.
- substitution_tag string? - Allows you to specify a substitution tag that you can insert in the body of your email at a location that you desire. This tag will be replaced by the open tracking pixel.
sendgrid: SendemailrequestTrackingSettingsSubscriptionTracking
Allows you to insert a subscription management link at the bottom of the text and html bodies of your email. If you would like to specify the location of the link within your email, you may use the substitution_tag.
Fields
- enable boolean? - Indicates if this setting is enabled.
- html string? - HTML to be appended to the email, with the subscription tracking link. You may control where the link is by using the tag <% %>
- substitution_tag string? - A tag that will be replaced with the unsubscribe URL. for example: [unsubscribe_url]. If this parameter is used, it will override both the
text
andhtml
parameters. The URL of the link will be placed at the substitution tag’s location, with no additional formatting.
- text string? - Text to be appended to the email, with the subscription tracking link. You may control where the link is by using the tag <% %>
sendgrid: SpamReportDetails
Spam report details
Fields
- created int - A Unix timestamp indicating when the spam report was made.
- email string - The email address of the recipient who marked your message as spam.
- ip string - The IP address that the message was sent on.
sendgrid: Subuser
Details of the subuser
Fields
- disabled boolean - Whether or not the user is enabled or disabled.
- email string - The email address to contact this subuser.
- id decimal - The ID of this subuser.
- username string - The name by which this subuser will be referred.
sendgrid: SubuserPost
Created subuser's details
Fields
- authorization_token string? - Authorization tokens
- credit_allocation SubuserPostCreditAllocation? - Credit allocations
- email string - The email address for this subuser.
- signup_session_token string? - Signup session token
- user_id decimal - The user ID for this subuser.
- username string - The username of the subuser.
sendgrid: SubuserPostCreditAllocation
Credit allocations
Fields
- 'type string? - Credit allocation type
sendgrid: SuppressionBlocks
Details of the blocks
Fields
- created int - A Unix timestamp indicating when the email address was added to the blocks list.
- email string - The email address that was added to the block list.
- reason string - An explanation for the reason of the block.
- status string - The status of the block.
sendgrid: UpdateAlertbyIdRequest
Fields
- email_to string? - The new email address you want your alert to be sent to. Example: test@example.com
- frequency string? - The new frequency at which to send the stats_notification alert. Example: monthly
- percentage int? - The new percentage threshold at which the usage_limit alert will be sent. Example: 90
sendgrid: UpdateAlertbyIdResponse
Fields
- created_at int - A Unix timestamp indicating when the alert was created.
- email_to string - The email address that the alert will be sent to.
- frequency string? - If the alert is of type stats_notification, this indicates how frequently the stats notifications will be sent. For example: "daily", "weekly", or "monthly".
- id int - The ID of the alert.
- percentage int? - If the alert is of type usage_limit, this indicates the percentage of email usage that must be reached before the alert will be sent.
- 'type string - The type of alert.
- updated_at int - A Unix timestamp indicating when the alert was last modified.
String types
sendgrid: SendemailrequestCategoriesItemsString
SendemailrequestCategoriesItemsString
Import
import ballerinax/sendgrid;
Metadata
Released date: about 1 year ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 163
Current verison: 113
Weekly downloads
Keywords
Marketing/Marketing Automation
Cost/Freemium
Contributors