Module selz
API
Definitions
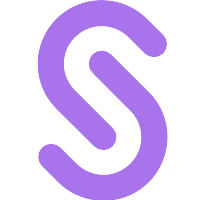
ballerinax/selz Ballerina library
Overview
This is a generated connector for selz API v1.0 OpenAPI specification. The Selz API is organized around REST. Our API has predictable resource-oriented URLs, accepts form-encoded request bodies, returns JSON-encoded responses, and uses standard HTTP response codes, authentication, and verbs.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create an selz account
- Obtain tokens by following this guide
Quickstart
To use the atSpoke connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/selz
module into the Ballerina project.
import ballerinax/selz;
Step 2: Create a new connector instance
Create a selz:ClientConfig
with the access token
obtained, and initialize the connector with it.
selz:ClientConfig clientConfig = { auth: { token: <ACCESS_TOKEN> } }; selz:Client baseClient = check new Client(clientConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to list all customers using the connector.
List all customers
public function main() returns error? { selz:SelzApiPublicModelsCustomersCustomerresponse response = check baseClient->getCustomers(); log:printInfo(response.toString()); }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
selz: Client
This is a generated connector for selz API v1.0 OpenAPI specification. The Selz API is organized around REST. Our API has predictable resource-oriented URLs, accepts form-encoded request bodies, returns JSON-encoded responses, and uses standard HTTP response codes, authentication, and verbs.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a selz account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string - URL of the target service
getBlogposts
function getBlogposts(int? 'limit, string? startingAfter, string? endingBefore, string? createdDateMin, string? createdDateMax, string? updatedDateMin, string? updatedDateMax) returns SelzApiPublicModelsSlicedlistresponse1SelzApiPublicModelsBlogpostsBlogpostresponseSelzApiPublicModelsVersion1000CultureNeutralPublickeytokenNull|error
List all blog posts
Parameters
- 'limit int? (default ()) - A limit on the number of objects to be returned. Limit can range between 1 and 100 items. (default is 10)
- startingAfter string? (default ()) - A cursor for use in pagination. starting_after is an object ID that defines your place in the list. For instance, if you make a list request and receive 100 objects, ending with obj_foo, your subsequent call can include starting_after=obj_foo in order to fetch the next page of the list.
- endingBefore string? (default ()) - A cursor for use in pagination. ending_before is an object ID that defines your place in the list. For instance, if you make a list request and receive 100 objects, starting with obj_bar, your subsequent call can include ending_before=obj_bar in order to fetch the previous page of the list.
- createdDateMin string? (default ()) - Filter by minimum created date
- createdDateMax string? (default ()) - Filter by maximum created date
- updatedDateMin string? (default ()) - Filter by minimum updated date.
- updatedDateMax string? (default ()) - Filter by maximum updated date.
Return Type
createBlogposts
function createBlogposts(SelzApiPublicModelsBlogpostsBlogpostrequest payload) returns SelzApiPublicModelsBlogpostsBlogpostresponse|error
Create a blog post
Parameters
- payload SelzApiPublicModelsBlogpostsBlogpostrequest - Create blogposts request
Return Type
retrieveBlogposts
function retrieveBlogposts(string id) returns SelzApiPublicModelsBlogpostsBlogpostresponse|error
Retrieve a blog post
Parameters
- id string - The identifier of the blog post to be retrieved.
Return Type
updateBlogposts
function updateBlogposts(string id, SelzApiPublicModelsBlogpostsBlogpostputrequest payload) returns SelzApiPublicModelsBlogpostsBlogpostresponse|error
Update a blog post
Parameters
- id string - The identifier of the blog post to be updated.
- payload SelzApiPublicModelsBlogpostsBlogpostputrequest - The fields to update
Return Type
deleteBlogposts
Delete a blog post
Parameters
- id string - The identifier of the blog post to be deleted.
addimageBlogposts
function addimageBlogposts(string id, SelzApiPublicModelsBlogpostsAddimagerequest payload) returns SelzApiPublicModelsBlogpostsAddimageresponse|error
Add blog post hero image
Parameters
- id string - The identifier of the blog post.
- payload SelzApiPublicModelsBlogpostsAddimagerequest - Add blog post hero image request
Return Type
removeimageBlogposts
Remove blog post hero image
Parameters
- id string - The identifier of the blog post.
getCategories
function getCategories(string? productId, int? 'limit, string? startingAfter, string? endingBefore, string? createdDateMin, string? createdDateMax, string? updatedDateMin, string? updatedDateMax) returns SelzCoreApiModelsSlicedlistresponse1SelzApiPublicModelsCategoriesCategoryresponseSelzApiPublicModelsVersion1000CultureNeutralPublickeytokenNull|error
List all categories
Parameters
- productId string? (default ()) - Only return orders that contain a product with the given product id.
- 'limit int? (default ()) - A limit on the number of objects to be returned. Limit can range between 1 and 100 items. (default is 10)
- startingAfter string? (default ()) - A cursor for use in pagination. starting_after is an object ID that defines your place in the list. For instance, if you make a list request and receive 100 objects, ending with obj_foo, your subsequent call can include starting_after=obj_foo in order to fetch the next page of the list.
- endingBefore string? (default ()) - A cursor for use in pagination. ending_before is an object ID that defines your place in the list. For instance, if you make a list request and receive 100 objects, starting with obj_bar, your subsequent call can include ending_before=obj_bar in order to fetch the previous page of the list.
- createdDateMin string? (default ()) - Filter by minimum created date
- createdDateMax string? (default ()) - Filter by maximum created date
- updatedDateMin string? (default ()) - Filter by minimum updated date.
- updatedDateMax string? (default ()) - Filter by maximum updated date.
Return Type
createCategories
function createCategories(SelzApiPublicModelsCategoriesCategoryrequest payload) returns SelzApiPublicModelsCategoriesCategoryresponse|error
Create a category
Parameters
- payload SelzApiPublicModelsCategoriesCategoryrequest - Create a category request
Return Type
retrieveCategories
function retrieveCategories(string id) returns SelzApiPublicModelsCategoriesCategoryresponse|error
Retrieve a category
Parameters
- id string - The identifier of the category to be retrieved.
Return Type
updateCategories
function updateCategories(string id, SelzApiPublicModelsCategoriesCategoryputrequest payload) returns SelzApiPublicModelsCategoriesCategoryresponse|error
Update a category
Parameters
- id string - The identifier of the category to be updated.
- payload SelzApiPublicModelsCategoriesCategoryputrequest - The fields to update
Return Type
deleteCategories
Delete a category
Parameters
- id string - The identifier of the category to be deleted.
getCustomers
function getCustomers(string? email, int? 'limit, string? startingAfter, string? endingBefore, string? createdDateMin, string? createdDateMax, string? updatedDateMin, string? updatedDateMax) returns SelzApiPublicModelsCustomersCustomerresponse|error
List all customers
Parameters
- email string? (default ()) - Filter by customer email
- 'limit int? (default ()) - A limit on the number of objects to be returned. Limit can range between 1 and 100 items. (default is 10)
- startingAfter string? (default ()) - A cursor for use in pagination. starting_after is an object ID that defines your place in the list. For instance, if you make a list request and receive 100 objects, ending with obj_foo, your subsequent call can include starting_after=obj_foo in order to fetch the next page of the list.
- endingBefore string? (default ()) - A cursor for use in pagination. ending_before is an object ID that defines your place in the list. For instance, if you make a list request and receive 100 objects, starting with obj_bar, your subsequent call can include ending_before=obj_bar in order to fetch the previous page of the list.
- createdDateMin string? (default ()) - Filter by minimum created date
- createdDateMax string? (default ()) - Filter by maximum created date
- updatedDateMin string? (default ()) - Filter by minimum updated date.
- updatedDateMax string? (default ()) - Filter by maximum updated date.
Return Type
createCustomers
function createCustomers(SelzApiPublicModelsCustomersCustomerrequest payload) returns SelzApiPublicModelsCustomersCustomerresponse|error
Create a customer
Parameters
- payload SelzApiPublicModelsCustomersCustomerrequest - Customer to be created
Return Type
retrieveCustomers
function retrieveCustomers(string id) returns SelzApiPublicModelsCustomersCustomerresponse|error
Retrieve a customer
Parameters
- id string - The identifier of the customer to be retrieved.
Return Type
updateCustomers
function updateCustomers(string id, SelzApiPublicModelsCustomersCustomerputrequest payload) returns SelzApiPublicModelsCustomersCustomerresponse|error
Update a customer
Parameters
- id string - The identifier of the customer to be updated.
- payload SelzApiPublicModelsCustomersCustomerputrequest - The fields to update
Return Type
deleteCustomers
Delete a customer
Parameters
- id string - The identifier of the customer to be deleted.
getCustomersByemail
function getCustomersByemail(string email) returns SelzApiPublicModelsCustomersCustomerresponse|error
Retrieve a customer by email
Parameters
- email string - email of the customer
Return Type
getDiscounts
function getDiscounts(string? discountCode, int? 'limit, string? startingAfter, string? endingBefore, string? createdDateMin, string? createdDateMax, string? updatedDateMin, string? updatedDateMax) returns SelzApiPublicModelsSlicedlistresponse1SelzApiPublicModelsDiscountsDiscountresponseSelzApiPublicModelsVersion1000CultureNeutralPublickeytokenNull|error
List all discounts
Parameters
- discountCode string? (default ()) - Filter by the case-insensitive discount code.
- 'limit int? (default ()) - A limit on the number of objects to be returned. Limit can range between 1 and 100 items. (default is 10)
- startingAfter string? (default ()) - A cursor for use in pagination. starting_after is an object ID that defines your place in the list. For instance, if you make a list request and receive 100 objects, ending with obj_foo, your subsequent call can include starting_after=obj_foo in order to fetch the next page of the list.
- endingBefore string? (default ()) - A cursor for use in pagination. ending_before is an object ID that defines your place in the list. For instance, if you make a list request and receive 100 objects, starting with obj_bar, your subsequent call can include ending_before=obj_bar in order to fetch the previous page of the list.
- createdDateMin string? (default ()) - Filter by minimum created date
- createdDateMax string? (default ()) - Filter by maximum created date
- updatedDateMin string? (default ()) - Filter by minimum updated date.
- updatedDateMax string? (default ()) - Filter by maximum updated date.
Return Type
createDiscounts
function createDiscounts(SelzApiPublicModelsDiscountsDiscountrequest payload) returns SelzApiPublicModelsDiscountsDiscountresponse|error
Create a discount
Parameters
- payload SelzApiPublicModelsDiscountsDiscountrequest - Create a discount request
Return Type
retrieveDiscounts
function retrieveDiscounts(string id) returns SelzApiPublicModelsDiscountsDiscountresponse|error
Retrieve a discount
Parameters
- id string - The identifier of the discount to be retrieved.
Return Type
updateDiscounts
function updateDiscounts(string id, SelzApiPublicModelsDiscountsDiscountputrequest payload) returns SelzApiPublicModelsDiscountsDiscountresponse|error
Update a discount
Parameters
- id string - The identifier of the discount to be updated.
- payload SelzApiPublicModelsDiscountsDiscountputrequest - The fields to update
Return Type
deleteDiscounts
Delete a discount
Parameters
- id string - The identifier of the discount to be deleted.
getDisputes
function getDisputes(string? status, int? 'limit, string? startingAfter, string? endingBefore, string? createdDateMin, string? createdDateMax, string? updatedDateMin, string? updatedDateMax) returns SelzApiPublicModelsSlicedlistresponse1SelzApiPublicModelsDisputesDisputeresponseSelzApiPublicModelsVersion1000CultureNeutralPublickeytokenNull|error
List Disputes
Parameters
- status string? (default ()) - Filter by the case-insensitive dispute status. Value Can be one of NeedsResponse, UnderReview, CannotRespond, Closed, Refunded, Won, Lost
- 'limit int? (default ()) - A limit on the number of objects to be returned. Limit can range between 1 and 100 items. (default is 10)
- startingAfter string? (default ()) - A cursor for use in pagination. starting_after is an object ID that defines your place in the list. For instance, if you make a list request and receive 100 objects, ending with obj_foo, your subsequent call can include starting_after=obj_foo in order to fetch the next page of the list.
- endingBefore string? (default ()) - A cursor for use in pagination. ending_before is an object ID that defines your place in the list. For instance, if you make a list request and receive 100 objects, starting with obj_bar, your subsequent call can include ending_before=obj_bar in order to fetch the previous page of the list.
- createdDateMin string? (default ()) - Filter by minimum created date
- createdDateMax string? (default ()) - Filter by maximum created date
- updatedDateMin string? (default ()) - Filter by minimum updated date.
- updatedDateMax string? (default ()) - Filter by maximum updated date.
Return Type
retrieveDisputes
function retrieveDisputes(string id) returns SelzApiPublicModelsDisputesDisputeresponse|error
Retrieve a dispute
Parameters
- id string - The identifier of the dispute to be retrieved.
Return Type
getOrders
function getOrders(string? customerEmail, string? orderStatus, int? 'limit, string? startingAfter, string? endingBefore, string? createdDateMin, string? createdDateMax, string? updatedDateMin, string? updatedDateMax) returns SelzApiPublicModelsSlicedlistresponse1SelzApiPublicModelsOrdersOrderresponseSelzApiPublicModelsVersion1000CultureNeutralPublickeytokenNull|error
List all Orders
Parameters
- customerEmail string? (default ()) - A filter on the list based on the customer’s email field. The value must be a string.
- orderStatus string? (default ()) - Only return orders that have the given status.
- 'limit int? (default ()) - A limit on the number of objects to be returned. Limit can range between 1 and 100 items. (default is 10)
- startingAfter string? (default ()) - A cursor for use in pagination. starting_after is an object ID that defines your place in the list. For instance, if you make a list request and receive 100 objects, ending with obj_foo, your subsequent call can include starting_after=obj_foo in order to fetch the next page of the list.
- endingBefore string? (default ()) - A cursor for use in pagination. ending_before is an object ID that defines your place in the list. For instance, if you make a list request and receive 100 objects, starting with obj_bar, your subsequent call can include ending_before=obj_bar in order to fetch the previous page of the list.
- createdDateMin string? (default ()) - Filter by minimum created date
- createdDateMax string? (default ()) - Filter by maximum created date
- updatedDateMin string? (default ()) - Filter by minimum updated date.
- updatedDateMax string? (default ()) - Filter by maximum updated date.
Return Type
retrieveOrders
function retrieveOrders(string id) returns SelzApiPublicModelsOrdersOrderresponse|error
Retrieve an Order
Parameters
- id string - The identifier of the Order to be retrieved.
Return Type
- SelzApiPublicModelsOrdersOrderresponse|error - Success
completeOrders
function completeOrders(string id) returns SelzApiPublicModelsOrdersOrderresponse|error
Update an order to complete status
Parameters
- id string - The identifier of the order to be updated.
Return Type
- SelzApiPublicModelsOrdersOrderresponse|error - Success
getPayouts
function getPayouts() returns SelzApiPublicModelsSlicedlistresponse1SelzApiPublicModelsPayoutsPayoutresponseSelzApiPublicModelsVersion1000CultureNeutralPublickeytokenNull|error
List Payouts
Return Type
retrievePayouts
function retrievePayouts(string id) returns SelzApiPublicModelsPayoutsPayoutresponse|error
Retrieve a payout
Parameters
- id string - The identifier of the payout to be retrieved.
Return Type
findProducts
function findProducts(string? productType, boolean? isPublished, int? 'limit, string? startingAfter, string? endingBefore, string? createdDateMin, string? createdDateMax, string? updatedDateMin, string? updatedDateMax) returns SelzCoreApiModelsSlicedlistresponse1SelzApiPublicModelsProductsProductresponseSelzApiPublicModelsVersion1000CultureNeutralPublickeytokenNull|error
List all products
Parameters
- productType string? (default ()) - Filter by Product Type
- isPublished boolean? (default ()) - Filter by IsPublished
- 'limit int? (default ()) - A limit on the number of objects to be returned. Limit can range between 1 and 100 items. (default is 10)
- startingAfter string? (default ()) - A cursor for use in pagination. starting_after is an object ID that defines your place in the list. For instance, if you make a list request and receive 100 objects, ending with obj_foo, your subsequent call can include starting_after=obj_foo in order to fetch the next page of the list.
- endingBefore string? (default ()) - A cursor for use in pagination. ending_before is an object ID that defines your place in the list. For instance, if you make a list request and receive 100 objects, starting with obj_bar, your subsequent call can include ending_before=obj_bar in order to fetch the previous page of the list.
- createdDateMin string? (default ()) - Filter by minimum created date
- createdDateMax string? (default ()) - Filter by maximum created date
- updatedDateMin string? (default ()) - Filter by minimum updated date.
- updatedDateMax string? (default ()) - Filter by maximum updated date.
Return Type
createProducts
function createProducts(SelzApiPublicModelsProductsProductrequest payload) returns SelzApiPublicModelsProductsProductresponse|error
Create a product
Parameters
- payload SelzApiPublicModelsProductsProductrequest - Create a product request
Return Type
retrieveProducts
function retrieveProducts(string id) returns SelzApiPublicModelsProductsProductresponse|error
Retrieve a product
Parameters
- id string - The identifier of the product to be retrieved.
Return Type
productsUpdate
function productsUpdate(string id, SelzApiPublicModelsProductsProductputrequest payload) returns SelzApiPublicModelsProductsProductresponse|error
Update a product
Parameters
- id string - The identifier of the product to be updated.
- payload SelzApiPublicModelsProductsProductputrequest - The fields to update
Return Type
deleteProducts
Delete a product
Parameters
- id string - The identifier of the product to be deleted.
getProductsCategories
function getProductsCategories(string id, string? productId, int? 'limit, string? startingAfter, string? endingBefore, string? createdDateMin, string? createdDateMax, string? updatedDateMin, string? updatedDateMax) returns SelzApiPublicModelsSlicedlistresponse1SelzApiPublicModelsCategoriesCategoryresponseSelzApiPublicModelsVersion1000CultureNeutralPublickeytokenNull|error
List categories
Parameters
- id string - ID
- productId string? (default ()) - Only return categories that contain this product id
- 'limit int? (default ()) - A limit on the number of objects to be returned. Limit can range between 1 and 100 items. (default is 10)
- startingAfter string? (default ()) - A cursor for use in pagination. starting_after is an object ID that defines your place in the list. For instance, if you make a list request and receive 100 objects, ending with obj_foo, your subsequent call can include starting_after=obj_foo in order to fetch the next page of the list.
- endingBefore string? (default ()) - A cursor for use in pagination. ending_before is an object ID that defines your place in the list. For instance, if you make a list request and receive 100 objects, starting with obj_bar, your subsequent call can include ending_before=obj_bar in order to fetch the previous page of the list.
- createdDateMin string? (default ()) - Filter by minimum created date
- createdDateMax string? (default ()) - Filter by maximum created date
- updatedDateMin string? (default ()) - Filter by minimum updated date.
- updatedDateMax string? (default ()) - Filter by maximum updated date.
Return Type
addProductToCategory
function addProductToCategory(string id, SelzApiPublicModelsProductsAddproducttocategoryrequest payload) returns SelzApiPublicModelsProductsAddproducttocategoryresponse|error
Assign to category
Parameters
- id string - the product id
- payload SelzApiPublicModelsProductsAddproducttocategoryrequest - Assign to category request
Return Type
removeProductFromCategory
Remove from category
verifyProductsLicense
function verifyProductsLicense(string id, SelzApiPublicModelsProductsLicenseverifyrequest payload) returns SelzApiPublicModelsProductsLicenseverifyresponse|error
Verify a license key
Parameters
- id string - the product id
- payload SelzApiPublicModelsProductsLicenseverifyrequest - Verify a license key request
Return Type
addProductsImage
function addProductsImage(string id, SelzApiPublicModelsProductsAddimagerequest payload) returns SelzApiPublicModelsProductsAddimageresponse|error
Add product image
Parameters
- id string - The identifier of the product.
- payload SelzApiPublicModelsProductsAddimagerequest - Add product image request
Return Type
removeAllProductsImages
Remove product images
Parameters
- id string - The identifier of the product.
addProductVariant
function addProductVariant(string id, SelzApiPublicModelsProductsProductvariantrequest payload) returns SelzApiPublicModelsProductsProductvariantresponse|error
Adds new variant to a product
Parameters
- id string - the product id
- payload SelzApiPublicModelsProductsProductvariantrequest - Adds new variant to a product request
Return Type
updateProductVariant
function updateProductVariant(string id, string variantId, SelzApiPublicModelsProductsProductvariantputrequest payload) returns SelzApiPublicModelsProductsProductvariantresponse|error
Update product variant
Parameters
- id string - The identifier of the product to be updated.
- variantId string - The identifier of the variant to be updated.
- payload SelzApiPublicModelsProductsProductvariantputrequest - The fields of the variant to update
Return Type
deleteProductVariant
Deletes existing variant from a product
findRefunds
function findRefunds(string? status, int? 'limit, string? startingAfter, string? endingBefore, string? createdDateMin, string? createdDateMax, string? updatedDateMin, string? updatedDateMax) returns SelzApiPublicModelsSlicedlistresponse1SelzOrdersApiModelsRefundresponseSelzOrdersApiModelsVersion1000CultureNeutralPublickeytokenNull|error
List all refunds
Parameters
- status string? (default ()) - Filter by refund status
- 'limit int? (default ()) - A limit on the number of objects to be returned. Limit can range between 1 and 100 items. (default is 10)
- startingAfter string? (default ()) - A cursor for use in pagination. starting_after is an object ID that defines your place in the list. For instance, if you make a list request and receive 100 objects, ending with obj_foo, your subsequent call can include starting_after=obj_foo in order to fetch the next page of the list.
- endingBefore string? (default ()) - A cursor for use in pagination. ending_before is an object ID that defines your place in the list. For instance, if you make a list request and receive 100 objects, starting with obj_bar, your subsequent call can include ending_before=obj_bar in order to fetch the previous page of the list.
- createdDateMin string? (default ()) - Filter by minimum created date
- createdDateMax string? (default ()) - Filter by maximum created date
- updatedDateMin string? (default ()) - Filter by minimum updated date.
- updatedDateMax string? (default ()) - Filter by maximum updated date.
Return Type
retrieveRefunds
function retrieveRefunds(string id) returns SelzApiPublicModelsRefundsRefundresponse|error
Retrieve a refund
Parameters
- id string - the identifier of the refund to be retrieved
Return Type
retrieveStore
function retrieveStore() returns SelzApiPublicModelsStoreStoreresponse|error
Retrieve store details
Return Type
- SelzApiPublicModelsStoreStoreresponse|error - Success
getWebhooks
function getWebhooks(string? eventType, int? 'limit, string? startingAfter, string? endingBefore, string? createdDateMin, string? createdDateMax, string? updatedDateMin, string? updatedDateMax) returns SelzApiPublicModelsSlicedlistresponse1SelzApiPublicModelsWebhooksWebhookresponseSelzApiPublicModelsVersion1000CultureNeutralPublickeytokenNull|error
List all webhooks
Parameters
- eventType string? (default ()) - A filter on the list based on the webhook event type
- 'limit int? (default ()) - A limit on the number of objects to be returned. Limit can range between 1 and 100 items. (default is 10)
- startingAfter string? (default ()) - A cursor for use in pagination. starting_after is an object ID that defines your place in the list. For instance, if you make a list request and receive 100 objects, ending with obj_foo, your subsequent call can include starting_after=obj_foo in order to fetch the next page of the list.
- endingBefore string? (default ()) - A cursor for use in pagination. ending_before is an object ID that defines your place in the list. For instance, if you make a list request and receive 100 objects, starting with obj_bar, your subsequent call can include ending_before=obj_bar in order to fetch the previous page of the list.
- createdDateMin string? (default ()) - Filter by minimum created date
- createdDateMax string? (default ()) - Filter by maximum created date
- updatedDateMin string? (default ()) - Filter by minimum updated date.
- updatedDateMax string? (default ()) - Filter by maximum updated date.
Return Type
createWebhooks
function createWebhooks(SelzApiPublicModelsWebhooksWebhookrequest payload) returns SelzApiPublicModelsWebhooksWebhookresponse|error
Create a webhook
Parameters
- payload SelzApiPublicModelsWebhooksWebhookrequest - Create a webhook request
Return Type
retrieveWebhooks
function retrieveWebhooks(string id) returns SelzApiPublicModelsWebhooksWebhookresponse|error
Retrieve a webhook
Parameters
- id string - The identifier of the webhook to be retrieved.
Return Type
putWebhooks
function putWebhooks(string id, SelzApiPublicModelsWebhooksWebhookputrequest payload) returns Response|error
Update a webhook
Parameters
- id string - The identifier of the webhook to be updated.
- payload SelzApiPublicModelsWebhooksWebhookputrequest - The fields to update
deleteWebhooks
Delete a webhook
Parameters
- id string - The identifier of the webhook to be deleted.
Records
selz: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
selz: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
selz: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
selz: SelzApiPublicModelsBlogpostsAddimagerequest
Add image to blog post by base64 encoded string or url to download
Fields
- attachment string? - A base64 encoded string representing the file
- filename string? - The name of the file, required if using base64 encoded string
- url string? - The source URL of the file that will be downloaded by Selz
selz: SelzApiPublicModelsBlogpostsAddimageresponse
Added image for the blog post
Fields
- url string? - Url of the added image
selz: SelzApiPublicModelsBlogpostsBlogpostputrequest
Patch a blog post
Fields
- title string? - The title of the blog.
- slug string? - A human-friendly unique string for the blogPost automatically generated from its title
- excerpt string? - An excerpt of the blog post, complete with HTML markup
- content string? - The content of the blog post
- meta_title string? - The HTML meta title that specifies the title of the blog web page
- meta_description string? - The HTML meta description that specifies the description of the blog web page
- is_published boolean? - States whether the blog post is visible. Valid values are "true" for visible and "false" for hidden. By default false
- disable_comments boolean? - States whether comments are allowed on the blog post
- image SelzApiPublicModelsBlogpostsShopimagerequest? - Image
- tags string? - Comma separated tags Id associated with this blog post
selz: SelzApiPublicModelsBlogpostsBlogpostrequest
Create a blog post
Fields
- title string? - The title of the blog.
- slug string? - A human-friendly unique string for the blogPost automatically generated from its title
- excerpt string? - An excerpt of the blog post, complete with HTML markup
- content string? - The content of the blog post
- meta_title string? - The HTML meta title that specifies the title of the blog web page
- meta_description string? - The HTML meta description that specifies the description of the blog web page
- is_published boolean? - States whether the blog post is visible. Valid values are "true" for visible and "false" for hidden. By default false
- disable_comments boolean? - States whether comments are allowed on the blog post
- image SelzApiPublicModelsBlogpostsShopimagerequest? - Image
- tags string? - Comma separated tags Id associated with this blog post
selz: SelzApiPublicModelsBlogpostsBlogpostresponse
A Blog post
Fields
- id string? - Unique identifier of the customer
- title string? - The title of the blogPost.
- meta_title string? - The HTML meta title that specifies the title of the categoty web page
- excerpt string? - The description of the blogPost, complete with HTML markup
- meta_description string? - The HTML meta description that specifies the description of the categoty web page
- content string? - The content of the blog post
- image SelzApiPublicModelsBlogpostsShopimageresponse? - Represent an image
- slug string? - A human-friendly unique string for the blogPost automatically generated from its title
- is_published boolean? - States whether the blogPost is visible. Valid values are "true" for visible and "false" for hidden. By default false
- created_time string? - The date and time when the blogPost was created. The API returns this value in ISO 8601 format.
- updated_time string? - The date and time when the blogPost was last updated. The API returns this value in ISO 8601 format.
- tags string? - Tags associated with this blog post
selz: SelzApiPublicModelsBlogpostsShopimagerequest
Image
Fields
- url string? - Url of the blog post image
selz: SelzApiPublicModelsBlogpostsShopimageresponse
Represent an image
Fields
- original string? - Original size
- is_featured boolean? - Is featured image
- is_default boolean? - Is default image
- pico string? - Resized 24 * 24 size image
- icon string? - Resized 48 * 48 size image
- thumb string? - Resized 64 * 64 size image
- small string? - Resized 96 * 96 size image
- compact string? - Resized 192 * 192 size image
- medium string? - Resized 256 * 256 size image
- large string? - Resized 640 * 640 size image
- grande string? - Resized 1280 * 1280 size image
- mucho_grande string? - Resized 1600 * 1600 size image
- huge string? - Resized 2048 * 2048 size image
selz: SelzApiPublicModelsCategoriesCategoryputrequest
Represents fields of Category object to be updated
Fields
- parent_id string? - An optional unique identifier of the parent category
- title string? - The title of the category.
- meta_title string? - The HTML meta title that specifies the title of the category web page
- slug string? - A human-friendly unique string for the category automatically generated from its title
- description string? - The description of the category, complete with HTML markup
- meta_description string? - The HTML meta description that specifies the description of the categoty web page
- is_published boolean? - States whether the category is visible. Valid values are "true" for visible and "false" for hidden. By default false
- products string[]? - list of product id's that belong to the category
- sort_type string? - The order in which products in the category appear
selz: SelzApiPublicModelsCategoriesCategoryrequest
Represent an Category object to be created
Fields
- parent_id string? - An optional unique identifier of the parent category
- title string? - The title of the category.
- meta_title string? - The HTML meta title that specifies the title of the category web page
- slug string? - A human-friendly unique string for the category automatically generated from its title
- description string? - The description of the category, complete with HTML markup
- meta_description string? - The HTML meta description that specifies the description of the categoty web page
- is_published boolean? - States whether the category is visible. Valid values are "true" for visible and "false" for hidden. By default false
- products string[]? - List of product Ids which belong to the category
- sort_type string? - Sort type of category: "manual", "alpha_asc", "alpha_desc", "created_asc", "created_desc", "price_asc", "price_desc"
- image SelzApiPublicModelsProductsImagerequest? - ImageRequest
selz: SelzApiPublicModelsCategoriesCategoryresponse
Represent Category detail
Fields
- id string? - Unique identifier of the category
- parent_id string? - Unique identifier of the parent category
- title string? - The title of the category.
- meta_title string? - The HTML meta title that specifies the title of the categoty web page
- description string? - The description of the category, complete with HTML markup
- meta_description string? - The HTML meta description that specifies the description of the category web page
- image SelzApiPublicModelsProductsImageresponse? - ImageResponse
- slug string? - A human-friendly unique string for the category automatically generated from its title
- sort_type string? - The order in which products in the category appear
- is_published boolean? - States whether the category is visible. Valid values are "true" for visible and "false" for hidden. By default false
- created_time string? - The date and time when the category was created. The API returns this value in ISO 8601 format.
- updated_time string? - The date and time when the category was last updated. The API returns this value in ISO 8601 format.
selz: SelzApiPublicModelsCustomersAddressputrequest
Represents fields of customer's Address object to be updated
Fields
- line1 string? - Street address
- line2 string? - An optional additional field for the street address
- city string? - City/Suburb/Town/Village
- post_code string? - Zip/Postal Code
- state_code string? - Code for the state or region
- country_code string? - Two-letter ISO code for the country
selz: SelzApiPublicModelsCustomersAddressrequest
Address Request
Fields
- line1 string? - Street address
- line2 string? - An optional additional field for the street address
- city string? - City/Suburb/Town/Village
- post_code string? - Zip/Postal Code
- state_code string? - Code for the state or region
- country_code string? - Two-letter ISO code for the country
selz: SelzApiPublicModelsCustomersAddressresponse
Address Response
Fields
- line1 string? - Street address
- line2 string? - An optional additional field for the street address
- city string? - City/Suburb/Town/Village
- post_code string? - Zip/Postal Code
- state_code string? - Code for the state or region
- country_code string? - Two-letter ISO code for the country
selz: SelzApiPublicModelsCustomersCustomerputrequest
Represents fields of customer object to be updated
Fields
- first_name string? - Customer first name
- last_name string? - Customer last name
- email string? - Customer email address
- accepts_marketing boolean? - Customer accepts marketing
- company string? - Company
- delivery_address SelzApiPublicModelsCustomersAddressputrequest? - Represents fields of customer's Address object to be updated
- billing_address SelzApiPublicModelsCustomersAddressputrequest? - Represents fields of customer's Address object to be updated
selz: SelzApiPublicModelsCustomersCustomerrequest
Represents a customer object to be create
Fields
- first_name string? - Customer first name
- last_name string? - Customer last name
- email string? - Customer email address
- accepts_marketing boolean? - Accepts marketing
- company string? - Company
- business_number string? - Business number
- delivery_address SelzApiPublicModelsCustomersAddressrequest? - Address Request
- billing_address SelzApiPublicModelsCustomersAddressrequest? - Address Request
selz: SelzApiPublicModelsCustomersCustomerresponse
A customer
Fields
- id string? - Unique identifier of the customer
- first_name string? - Customer first name
- last_name string? - Customer last name
- email string? - Customer email address
- accepts_marketing boolean? - Customer accepts marketing
- company string? - Company
- delivery_address SelzApiPublicModelsCustomersAddressresponse? - Address Response
- billing_address SelzApiPublicModelsCustomersAddressresponse? - Address Response
- created_time string? - The date and time when the customer was created. The API returns this value in ISO 8601 format.
- updated_time string? - The date and time when the customer was last updated. The API returns this value in ISO 8601 format.
selz: SelzApiPublicModelsDiscountsDiscountputrequest
Represents an object that is required to update existing discount.
Fields
- name string? - A friendly name to remember the discount
- minimum_value string? - The minimum order value for the discount to be valid. This value excludes tax and shipping and applies to the order discounts only.
- free_shipping boolean? - Discount includes free shipping.
- value_off string? - Value that will be taken off.
- percent_off int? - Percent that will be taken off.
- currency_code string? - Three-letter ISO currency code representing the currency of the category or order type discount value. Product discounts are in the product currency.
- quantity int? - Maximum number of times the discount can be applied.
- valid_from string? - The date the discount becomes valid for use during checkout in ISO 8601 format.
- valid_to string? - The date when the discount code becomes disabled in ISO 8601 format.
- is_published boolean? - States whether the discount is visible. Valid values are "true" for visible and "false" for hidden. By default false
selz: SelzApiPublicModelsDiscountsDiscountrequest
Represents an object that is required to create a discount.
Fields
- name string? - A friendly name to remember the discount
- code string? - The case-insensitive discount code that customers use at checkout.
- 'type string? - Specify to what the discount's value is applied to a product, category or an order.
- target_id string? - The unique identifier of the product or the category to which discount applies to.
- minimum_value string? - The minimum order value for the discount to be valid. This value excludes tax and shipping and applies to the order discounts only.
- value_off string? - Value that will be taken off.
- free_shipping boolean? - Discount includes free shipping.
- percent_off int? - Percent that will be taken off.
- currency_code string? - Three-letter ISO currency code representing the currency of the category or order type discount value. Product discounts are in the product currency.
- quantity int? - Maximum number of times the discount can be applied.
- valid_from string? - The date the discount becomes valid for use during checkout in ISO 8601 format.
- valid_to string? - The date when the discount code becomes disabled in ISO 8601 format.
- is_published boolean? - States whether the discount is visible. Valid values are "true" for visible and "false" for hidden. By default false
selz: SelzApiPublicModelsDiscountsDiscountresponse
Object representing discount
Fields
- id string? - The unique identifier for the discount.
- name string? - A friendly name to remember the discount
- code string? - The case-insensitive discount code that customers use at checkout.
- short_url string? - The shorten url associated with the discount
- 'type string? - Specify to what the discount's value is applied to a product, category or an order.
- target_id string? - The unique identifier of the product or the category to which discount applies to.
- minimum_value string? - The minimum order value for the discount to be valid. This value excludes tax and shipping and applies to the order discounts only.
- free_shipping boolean? - Discount includes free shipping.
- value_off string? - Value that will be taken off.
- percent_off int? - Percent that will be taken off.
- currency_code string? - Three-letter ISO currency code representing the currency of the category or order type discount value. Product discounts are in the product currency.
- quantity int? - Maximum number of times the discount can be applied.
- quantity_sold int? - Number of times the discount was applied.
- valid_from string? - The date the discount becomes valid for use during checkout in ISO 8601 format.
- valid_to string? - The date when the discount code becomes disabled in ISO 8601 format.
- is_published boolean? - States whether the discount is visible. Valid values are "true" for visible and "false" for hidden. By default false
- created_time string? - The date and time when the discount was created. The API returns this value in ISO 8601 format.
- updated_time string? - The date and time when the discount was last updated. The API returns this value in ISO 8601 format.
selz: SelzApiPublicModelsDisputesDisputeresponse
Object representing Dispute
Fields
- id string? - The unique identifier for dispute.
- order_id string? - Order Id associated with this dispute.
- status string? - Status of the dispute.
- total_amount string? - Total amount
- currency string? - Three-letter ISO currency code representing the currency of the dispute
- reason string? - Reason of the Dispute
- created_time string? - The date and time when the dispute was created. The API returns this value in ISO 8601 format.
- updated_time string? - The date and time when the dispute was created. The API returns this value in ISO 8601 format.
selz: SelzApiPublicModelsErrorresponse
Error response model
Fields
- 'type string? - The type of error returned. Can be: api_connection_error, api_error, authentication_error, invalid_request_error, or rate_limit_error.
- message string? - A human-readable message providing more details about the error.
- errors record {}? - The parameter the error relates to if the error is parameter-specific.
selz: SelzApiPublicModelsOrdersAddressresponse
Address
Fields
- line1 string? - Street address
- line2 string? - An optional additional field for the street address
- city string? - City/Suburb/Town/Village
- post_code string? - Zip/Postal Code
- state_code string? - Code for the state or region
- country_code string? - Two-letter ISO code for the country
selz: SelzApiPublicModelsOrdersBuyerresponse
BuyerResponse
Fields
- first_name string? - Customer first name
- last_name string? - Customer last name
- email string? - Customer email address
- accepts_marketing boolean? - Customer accepts marketing
- company string? - Company
- delivery_address SelzApiPublicModelsOrdersAddressresponse? - Address
- billing_address SelzApiPublicModelsOrdersAddressresponse? - Address
selz: SelzApiPublicModelsOrdersCardpaymentdetailsresponse
CardPaymentDetailsResponse
Fields
- reference string? - Reference id from the payment gateway response
- brand string? - Brand of the credit card
- name string? - Name on the credit card
- country string? - Country the credit card was issued in
- last_four string? - Last four digits of the credit card
selz: SelzApiPublicModelsOrdersOrderitemresponse
Order item line
Fields
- product_id string? - Unique identifier of the product.
- product_title string? - The name of the product.
- product_sku string? - Stock keeping unit of the product.
- product_variant_id string? - Unique identifier of the product variant.
- product_variant_title string? - The name of the product variant.
- product_variant_sku string? - Stock keeping unit of the product variant.
- discount_code string? - Discount code applied to the line item
- quantity int? - The number of products ordered
- unit_price string? - Unit price of the line item
- currency string? - Currency of the product ordered
- tax_rate decimal? - Tax rate of the line item
- tax_name string? - Tax name of the line item
- total_tax decimal? - Tax amount of the line item
- total_discount decimal? - Discount amount of the line item
selz: SelzApiPublicModelsOrdersOrderresponse
Order
Fields
- id string? - Unique identifier of the order
- reference_id string? - Order reference id used by the seller and customer
- customer SelzApiPublicModelsOrdersBuyerresponse? - BuyerResponse
- items SelzApiPublicModelsOrdersOrderitemresponse[]? - Line items of the order
- currency string? - Three-letter ISO currency code representing the currency of the product price
- total_price string? - Total price of the order
- total_shipping string? - Total price of the shipping method
- shipping_tax string? - Total tax charged on shipping
- shipping_tax_rate decimal? - Shipping tax rate
- shipping_tax_name string? - Shipping tax name
- total_tax string? - Total amount of tax charged for the whole order (including shipping)
- price_includes_tax boolean? - Tax is including in the price (true/false)
- total_fees string? - Total amount of fees charged
- status string? - Status of the order (processed, failed, completed, refunded, disputed, etc)
- created_time string? - The date and time when the order was created. The API returns this value in ISO 8601 format.
- updated_time string? - The date and time when the order was updated. The API returns this value in ISO 8601 format.
- tracking_id string? - Custom tracking id
- custom_fields record {}? - Custom fields
- payment_details SelzApiPublicModelsOrdersPaymentdetailsresponse? - PaymentDetailsResponse
- payment_processor_type string? - Type of payment processor
selz: SelzApiPublicModelsOrdersPaymentdetailsresponse
PaymentDetailsResponse
Fields
- 'type string? - Type of the payment, e.g. "card" or "paypal"
- card SelzApiPublicModelsOrdersCardpaymentdetailsresponse? - CardPaymentDetailsResponse
- paypal SelzApiPublicModelsOrdersPaypalpaymentdetailsresponse? - PaypalPaymentDetailsResponse
selz: SelzApiPublicModelsOrdersPaypalpaymentdetailsresponse
PaypalPaymentDetailsResponse
Fields
- reference string? - Reference id returned from Paypal for the transaction
- email string? - Email address of the Paypal account used for the transaction
selz: SelzApiPublicModelsPayoutsPayoutdestinationbankresponsemodel
Bank detail of a transfer
Fields
- name string? - Name
- country string? - Country
- routing string? - Routing
- last_four string? - Last four digit of the card
selz: SelzApiPublicModelsPayoutsPayoutdestinationresponsemodel
Bank detail of the payout
Fields
- 'type string? - Type
- bank SelzApiPublicModelsPayoutsPayoutdestinationbankresponsemodel? - Bank detail of a transfer
selz: SelzApiPublicModelsPayoutsPayoutresponse
Object representing Payout
Fields
- id string? - The unique identifier for payout.
- status string? - Status of the payout
- total_amount string? - Total amount associated with the payout
- currency string? - Three-letter ISO currency code representing the currency of the payout
- destination SelzApiPublicModelsPayoutsPayoutdestinationresponsemodel? - Bank detail of the payout
- created_time string? - The date and time when the Payout was created. The API returns this value in ISO 8601 format.
- updated_time string? - The date and time when the Payout was last updated. The API returns this value in ISO 8601 format.
- scheduled_time string? - The date and time when the Payout is scheduled. The API returns this value in ISO 8601 format.
selz: SelzApiPublicModelsProductsAddimagerequest
Add image to product by base64 encoded string or url to download
Fields
- attachment string? - A base64 encoded string representing the file
- filename string? - The name of the file, required if using base64 encoded string
- url string? - The source URL of the file that will be downloaded by Selz
selz: SelzApiPublicModelsProductsAddimageresponse
AddImageResponse
Fields
- url string? - The Url of the image
selz: SelzApiPublicModelsProductsAddproducttocategoryrequest
AddProductToCategoryRequest
Fields
- category_id string? - The category Id
selz: SelzApiPublicModelsProductsAddproducttocategoryresponse
AddProductToCategoryResponse
Fields
- category_id string? - The category Id
selz: SelzApiPublicModelsProductsCatalogaddressresponse
CatalogAddressResponse
Fields
- line1 string? - Street address
- line2 string? - An optional additional field for the street address
- city string? - City/Suburb/Town/Village
- post_code string? - Zip/Postal Code
- state_code string? - Code for the state or region
- country_code string? - Two-letter ISO code for the country
selz: SelzApiPublicModelsProductsCatalogbuyerresponse
CatalogBuyerResponse
Fields
- first_name string? - Customer first name
- last_name string? - Customer last name
- email string? - Customer email address
- accepts_marketing boolean? - Customer accepts marketing
- company string? - Company
- delivery_address SelzApiPublicModelsProductsCatalogaddressresponse? - CatalogAddressResponse
- billing_address SelzApiPublicModelsProductsCatalogaddressresponse? - CatalogAddressResponse
selz: SelzApiPublicModelsProductsCatalogorderitemresponse
CatalogOrderItemResponse
Fields
- product_id string? - Unique identifier of the product.
- product_title string? - The name of the product.
- product_sku string? - Stock keeping unit of the product.
- product_variant_id string? - Unique identifier of the product variant.
- product_variant_title string? - The name of the product variant.
- product_variant_sku string? - Stock keeping unit of the product variant.
- discount_code string? - Discount code applied to the line item
- quantity int? - The number of products ordered
- unit_price string? - Unit price of the line item
- currency string? - Currency of the product ordered
selz: SelzApiPublicModelsProductsCatalogorderresponse
CatalogOrderResponse
Fields
- id string? - Unique identifier of the order
- reference_id string? - Order reference id used by the seller and customer
- customer SelzApiPublicModelsProductsCatalogbuyerresponse? - CatalogBuyerResponse
- items SelzApiPublicModelsProductsCatalogorderitemresponse[]? - Line items of the order
- currency string? - Three-letter ISO currency code representing the currency of the product price
- total_price string? - Total price of the order
- total_shipping string? - Total price of the shipping method
- total_tax string? - Total amount of tax charged
- price_includes_tax boolean? - Tax is including in the price (true/false)
- status string? - Status of the order (processed, failed, completed, refunded, disputed, etc)
- created_time string? - The date and time when the order was created. The API returns this value in ISO 8601 format.
- tracking_id string? - Custom tracking id
- custom_fields record {}? - List of custom fields
selz: SelzApiPublicModelsProductsImagerequest
ImageRequest
Fields
- url string? - The image url
selz: SelzApiPublicModelsProductsImageresponse
ImageResponse
Fields
- original string? - The url if the original image
- is_featured boolean? - Is featured
- is_default boolean? - Is default
- pico string? - 24 x 24 pixels image
- icon string? - 48 x 48 pixels image
- thumb string? - 64 x 64 pixels image
- small string? - 96 x 96 pixels image
- compact string? - 192 x 192 pixels image
- medium string? - 256 x 256 pixels image
- large string? - 640 x 640 pixels image
- grande string? - 1280 x 1280 pixels
- mucho_grande string? - 1600 x 1600 pixels
- huge string? - 2048 x 2048 pixels
selz: SelzApiPublicModelsProductsLicenseverifyrequest
LicenseVerifyRequest
Fields
- 'key string? - The license key itself
- increment_usage_count boolean? - Should the license usage count be incremented
selz: SelzApiPublicModelsProductsLicenseverifyresponse
LicenseVerifyResponse
Fields
- is_active boolean? - True if the license is active, false otherwise
- usage_count int? - Number of time the licenses has been used
- 'order SelzApiPublicModelsProductsCatalogorderresponse? - CatalogOrderResponse
selz: SelzApiPublicModelsProductsProductputrequest
ProductPutRequest
Fields
- product_type string? - The type of Product, can be physical, digital or service
- sku string? - Stock keeping unit of the product
- title string? - The name of the product
- description string? - The description of the product, complete with HTML formatting
- page_title string? - Page title used for SEO
- meta_description string? - Meta description used for SEO
- slug string? - The slug of the product
- quantity int? - The number of items in stock for this product
- quantity_available int? - The number of items in stock which is available for this product
- is_unlimited_quantity boolean? - Flag indicating that number of items in stock is unlimited
- currency_code string? - Three-letter ISO currency code representing the currency of the product price
- price string? - The price of the product
- regular_price string? - The original price of the product
- is_price_flexible boolean? - Is the price flexible
- is_published boolean? - The published state of the product
- is_free_shipping boolean? - Flag indicating if product has free shipping
- weight string? - The weight of the product in the unit system specified with weight_unit.
- weight_unit string? - The unit system that the product's weight is measure in. The weight_unit can be either "g", "kg, "oz", or "lb".
- variants SelzApiPublicModelsProductsProductvariantrequest[]? - A list of variant objects, each one representing a slightly different version of the product
- image SelzApiPublicModelsProductsImagerequest? - ImageRequest
selz: SelzApiPublicModelsProductsProductrequest
ProductRequest
Fields
- product_type string? - Type of product (e.g. physical, digital, etc)
- sku string? - Stock keeping unit of the product
- title string? - The name of the product
- description string? - The description of the product, complete with HTML formatting
- page_title string? - Page title used for SEO
- meta_description string? - Meta description used for SEO
- slug string? - The slug of the product
- quantity int? - The number of items in stock for this product
- currency_code string? - Three-letter ISO currency code representing the currency of the product price
- price string? - The price of the product
- regular_price string? - The original price of the product
- is_price_flexible boolean? - Is the price flexible
- is_published boolean? - The published state of the product
- is_free_shipping boolean? - Flag indicating if product has free shipping
- weight string? - The weight of the product in the unit system specified with weight_unit.
- weight_unit string? - The unit system that the product's weight is measure in. The weight_unit can be either "g", "kg, "oz", or "lb". Default is "g".
- variants SelzApiPublicModelsProductsProductvariantrequest[]? - A list of variant objects, each one representing a slightly different version of the product
- images SelzApiPublicModelsProductsImagerequest[]? - Product image
selz: SelzApiPublicModelsProductsProductresponse
ProductResponse
Fields
- id string? - Unique identifier of the product
- sku string? - Stock keeping unit of the product
- title string? - The name of the product
- slug string? - The slug of the product
- description string? - The description of the product, complete with HTML formatting
- short_url string? - The Product short URL
- product_type string? - The type of Product, can be physical, digital or service
- currency_code string? - Three-letter ISO currency code representing the currency of the product price
- price decimal? - The price of the product
- regular_price decimal? - The original price of the product
- is_price_flexible boolean? - TBC
- quantity int? - The number of items in stock for this product
- quantity_available int? - The number of items in stock which is available for this product
- variants SelzApiPublicModelsProductsProductvariantresponse[]? - A list of variant objects, each one representing a slightly different version of the product
- variant_attributes SelzApiPublicModelsProductsVariantattributeresponse[]? - A list of variants attributes
- images SelzApiPublicModelsProductsImageresponse[]? - A list of image objects, each one representing an image associated with the product
- featured_image SelzApiPublicModelsProductsImageresponse? - ImageResponse
- media_type string? - The type of media (e.g. Video, Audio, YouTube or Vimeo)
- media_url string? - The URL for the media. In the case of YouTube and Vimeo, the video ID
- media_cover SelzApiPublicModelsProductsImageresponse? - ImageResponse
- is_published boolean? - The published state of the product
- is_free_shipping boolean? - If the product includes free shipping
- created_time string? - The date and time when the product was created. The API returns this value in ISO 8601 format
- updated_time string? - The date and time when the product was last updated.
selz: SelzApiPublicModelsProductsProductvariantputrequest
ProductVariantRequest
Fields
- sku string? - Stock keeping unit of the product variant
- title string? - The title of the product variant.
- price string? - The price of the product variant
- quantity int? - The number of items in stock for this product variant
- quantity_available int? - The number of items in stock which is available for this product
- is_unlimited_quantity boolean? - Flag indicating that number of items in stock for this product variant is unlimited
- options record {}? - A list of object Id's as strings representing variant options
selz: SelzApiPublicModelsProductsProductvariantrequest
ProductVariantRequest
Fields
- id string? - Id of the product variant
- sku string? - Stock keeping unit of the product variant
- title string? - The title of the product variant.
- price string? - The price of the product variant
- quantity int? - The number of items in stock for this product variant
- is_unlimited_quantity boolean? - Flag indicating that number of items in stock for this product variant is unlimited
- options record {}? - A key value pair representing variant options eg:{"Color","Blue"}
selz: SelzApiPublicModelsProductsProductvariantresponse
ProductVariantResponse
Fields
- id string? - Unique identifier of the product variant
- sku string? - Stock keeping unit of the product variant
- title string? - The title of the product variant.
- price_regular decimal? - The original price of the product variant
- price decimal? - The price of the product variant
- quantity int? - The number of items in stock for this product variant
- quantity_available int? - The number of items in stock which is available for this product variant
- options string[]? - A list of options, each one representing a slightly different version of the product variant
selz: SelzApiPublicModelsProductsVariantattributeresponse
VariantAttributeResponse
Fields
- id string? - Unique identifier of the variant option
- name string? - Attribute name (e.g: "Size", "Color", etc)
- options SelzApiPublicModelsProductsVariantoptionresponse[]? - Attribute option (e.g: "Red", "Green", "Yellow", etc)
selz: SelzApiPublicModelsProductsVariantoptionresponse
VariantOptionResponse
Fields
- id string? - Unique identifier of the variant option
- value string? - Variant option value (e.g: "Red", "Green", "Yellow", etc)
selz: SelzApiPublicModelsRefundsRefundresponse
RefundResponse
Fields
- id string? - The refund's identifier.
- order_id string? - The identifier of the order the refund is associated with.
- status string? - The current status of refund.
- total_amount string? - The total amount refunded
- currency string? - Three-letter ISO currency code representing the currency of the refund
- created_time string? - The date and time when the refund was created. The API returns this value in ISO 8601 format.
- updated_time string? - The date and time when the refund was updated. The API returns this value in ISO 8601 format.
selz: SelzApiPublicModelsSlicedlistresponse1SelzApiPublicModelsBlogpostsBlogpostresponseSelzApiPublicModelsVersion1000CultureNeutralPublickeytokenNull
SlicedListResponse
Fields
- 'limit int? - Limit on the number of objects to be returned
- has_more boolean? - Flag indicating if list has more objects
- data SelzApiPublicModelsBlogpostsBlogpostresponse[]? - List of objects
selz: SelzApiPublicModelsSlicedlistresponse1SelzApiPublicModelsCategoriesCategoryresponseSelzApiPublicModelsVersion1000CultureNeutralPublickeytokenNull
SlicedListResponse
Fields
- 'limit int? - Limit on the number of objects to be returned
- has_more boolean? - Flag indicating if list has more objects
- data SelzApiPublicModelsCategoriesCategoryresponse[]? - List of objects
selz: SelzApiPublicModelsSlicedlistresponse1SelzApiPublicModelsDiscountsDiscountresponseSelzApiPublicModelsVersion1000CultureNeutralPublickeytokenNull
SlicedListResponse
Fields
- 'limit int? - Limit on the number of objects to be returned
- has_more boolean? - Flag indicating if list has more objects
- data SelzApiPublicModelsDiscountsDiscountresponse[]? - List of objects
selz: SelzApiPublicModelsSlicedlistresponse1SelzApiPublicModelsDisputesDisputeresponseSelzApiPublicModelsVersion1000CultureNeutralPublickeytokenNull
SlicedListResponse
Fields
- 'limit int? - Limit on the number of objects to be returned
- has_more boolean? - Flag indicating if list has more objects
- data SelzApiPublicModelsDisputesDisputeresponse[]? - List of objects
selz: SelzApiPublicModelsSlicedlistresponse1SelzApiPublicModelsOrdersOrderresponseSelzApiPublicModelsVersion1000CultureNeutralPublickeytokenNull
SlicedListResponse
Fields
- 'limit int? - Limit on the number of objects to be returned
- has_more boolean? - Flag indicating if list has more objects
- data SelzApiPublicModelsOrdersOrderresponse[]? - List of objects
selz: SelzApiPublicModelsSlicedlistresponse1SelzApiPublicModelsPayoutsPayoutresponseSelzApiPublicModelsVersion1000CultureNeutralPublickeytokenNull
SlicedListResponse
Fields
- 'limit int? - Limit on the number of objects to be returned
- has_more boolean? - Flag indicating if list has more objects
- data SelzApiPublicModelsPayoutsPayoutresponse[]? - List of objects
selz: SelzApiPublicModelsSlicedlistresponse1SelzApiPublicModelsWebhooksWebhookresponseSelzApiPublicModelsVersion1000CultureNeutralPublickeytokenNull
SlicedListResponse
Fields
- 'limit int? - Limit on the number of objects to be returned
- has_more boolean? - Flag indicating if list has more objects
- data SelzApiPublicModelsWebhooksWebhookresponse[]? - List of objects
selz: SelzApiPublicModelsSlicedlistresponse1SelzOrdersApiModelsRefundresponseSelzOrdersApiModelsVersion1000CultureNeutralPublickeytokenNull
SlicedListResponse
Fields
- 'limit int? - Limit on the number of objects to be returned
- has_more boolean? - Flag indicating if list has more objects
- data SelzOrdersApiModelsRefundresponse[]? - List of objects
selz: SelzApiPublicModelsStoreAddressresponse
Address
Fields
- line1 string? - Street address
- line2 string? - An optional additional field for the street address
- city string? - City/Suburb/Town/Village
- post_code string? - Zip/Postal Code
- state_code string? - Code for the state or region
- country_code string? - Two-letter ISO code for the country
selz: SelzApiPublicModelsStoreOwnerresponse
Owner
Fields
- full_name string? - Name of the shop
- email string? - Email of the shop
- biography string? - Biography of the shop
- avatar string? - Avatar image url of the shop
- address SelzApiPublicModelsStoreAddressresponse? - Address
selz: SelzApiPublicModelsStoreShopsubscriptionresponse
Subscription
Fields
- plan_id string? - Plan Id of the subscription (e.g. Free, Basic, Standard, Advanced)
- plan_name string? - Plan name of the subscription (e.g. Free, Basic - 2019 Standard - 2019, Advanced - 2019)
selz: SelzApiPublicModelsStoreStoreresponse
Store
Fields
- id string? - Unique identifier of the store
- name string? - Name of the store
- logo string? - Logo url of the store
- owner SelzApiPublicModelsStoreOwnerresponse? - Owner
- icon string? - Icon url of the store
- display_name string? - User friendly display for the store
- domain string? - Domain of the store
- culture_name string? - Culture of the shop, ISO two-letter (639-1) language code (e.g. en)
- time_zone string? - ISO 8601 time zone name e.g. Pacific Standard Time, https://en.wikipedia.org/wiki/List_of_time_zone_abbreviations for the full list of time zone names.
- currency string? - ISO 4217 Currency code (e.g USD of U.S dollars)
- subscription SelzApiPublicModelsStoreShopsubscriptionresponse? - Subscription
- created_time string? - Store creation time
selz: SelzApiPublicModelsWebhooksWebhookputrequest
WebHookPutRequest
Fields
- url string? - The URL where the webHook should send the POST request when the event occurs.
- event_type string? - Event type: e.g order_payment_succeeded, product_soldout
selz: SelzApiPublicModelsWebhooksWebhookrequest
Webhook
Fields
- url string? - The URL where the webhook should send the POST request when the event occurs.
- event_type string? - Event type: e.g order_payment_succeeded, product_soldout
selz: SelzApiPublicModelsWebhooksWebhookresponse
WebHookResponse
Fields
- id string? - Unique identifier of the webHook
- 'key string? - A secret that you can use to validate the integrity of these webHooks
- url string? - The URL where the webHook should send the POST request when the event occurs.
- event_type string? - Description of the event: e.g order_payment_succeeded, product_soldout
- created_time string? - The date and time when the webHook was created. The API returns this value in ISO 8601 format
selz: SelzCoreApiModelsErrorresponse
Fields
- code string? -
- message string? -
selz: SelzCoreApiModelsSlicedlistmetadata
Fields
- 'limit int? -
- previous_cursor string? -
- next_cursor string? -
- has_previous boolean? -
- has_next boolean? -
selz: SelzCoreApiModelsSlicedlistresponse1SelzApiPublicModelsCategoriesCategoryresponseSelzApiPublicModelsVersion1000CultureNeutralPublickeytokenNull
Fields
- pagination SelzCoreApiModelsSlicedlistmetadata? -
- errors SelzCoreApiModelsErrorresponse[]? -
selz: SelzCoreApiModelsSlicedlistresponse1SelzApiPublicModelsProductsProductresponseSelzApiPublicModelsVersion1000CultureNeutralPublickeytokenNull
Fields
- pagination SelzCoreApiModelsSlicedlistmetadata? -
- errors SelzCoreApiModelsErrorresponse[]? -
selz: SelzOrdersApiModelsRefundresponse
Fields
- id string? -
- shop_id int? -
- order_id string? -
- order_created_date string? -
- order_reference_id string? -
- total_amount decimal? -
- total_amount_in_base_currency decimal? -
- disbursed decimal? -
- balance decimal? -
- balance_in_base_currency decimal? -
- currency_code string? -
- currency_rate decimal? -
- exclude_from_seller_balance boolean? -
- payment_gateway string? -
- payment_processor_type string? -
- status string? -
- created_by string? -
- created_time string? -
- updated_by string? -
- updated_time string? -
- extra_data record {}? -
Import
import ballerinax/selz;
Metadata
Released date: over 1 year ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 0
Current verison: 0
Weekly downloads
Keywords
Commerce/eCommerce
Cost/Paid
Contributors
Dependencies