sap.s4hana.api_sales_quotation_srv
Module sap.s4hana.api_sales_quotation_srv
API
Definitions
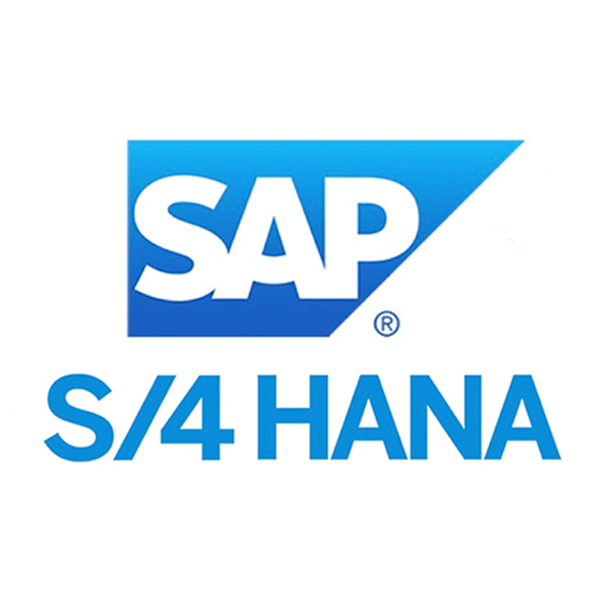
ballerinax/sap.s4hana.api_sales_quotation_srv Ballerina library
Overview
S/4HANA is a robust enterprise resource planning (ERP) solution, designed for large-scale enterprises by SAP SE.
The ballerinax/sap.s4hana.api_sales_quotation_srv
package provides APIs that enable seamless integration with the Sales Quotation (A2X) API v1.0.0. The service allows to create, read, update, and delete sales quotation.
Setup guide
-
Sign in to your S/4HANA dashboard.
-
Under the
Communication Management
section, click on theDisplay Communications Scenario
title. -
In the search bar, type
Sales Quotation Integration
and select the corresponding scenario from the results. -
In the top right corner of the screen, click on
Create Communication Arrangement
. -
Enter a unique name for the arrangement.
-
Choose an existing
Communication System
from the dropdown menu and save your arrangement. -
The hostname (
<unique id>-api.s4hana.cloud.sap
) will be displayed in the top right corner of the screen.
Quickstart
To use the sap.s4hana.api_sales_quotation_srv
connector in your Ballerina application, modify the .bal
file as follows:
Step 1: Import the module
Import the sap.s4hana.api_sales_quotation_srv
module.
import ballerinax/sap.s4hana.api_sales_quotation_srv as quotation;
Step 2: Instantiate a new connector
Use the hostname and credentials to initiate a client
configurable string hostname = ?; configurable string username = ?; configurable string password = ?; quotation:Client quotationClient = check new ( { auth: { username, password } }, hostname );
Step 3: Invoke the connector operation
Now, utilize the available connector operations.
quotation:CollectionOfA_SalesQuotationWrapper listASalesOrder = check quotationClient->listA_SalesQuotations();
Step 4: Run the Ballerina application
bal run
Examples
The S/4 HANA Sales and Distribution Ballerina connectors provide practical examples illustrating usage in various scenarios. Explore these examples, covering use cases like accessing S/4HANA Sales Order (A2X) API.
-
Salesforce to S/4HANA Integration - Demonstrates leveraging the
sap.s4hana.api_sales_order_srv:Client
in Ballerina for S/4HANA API interactions. It specifically showcases how to respond to a Salesforce Opportunity Close Event by automatically generating a Sales Order in the S/4HANA SD module. -
Shopify to S/4HANA Integration - Details the integration process between Shopify, a leading e-commerce platform, and SAP S/4HANA, a comprehensive ERP system. The objective is to automate SAP sales order creation for new orders placed on Shopify, enhancing efficiency and accuracy in order management.
Clients
sap.s4hana.api_sales_quotation_srv: Client
In every API call, you can make use of the following operations:
- You can read entire sales quotations or only parts of the data, using the provided filters.
- You can create sales quotations. You can use “deep insert” requests to create a header together with one or more related entities (for example, header partners or items).
- For existing sales quotations, you can create new items. You can use “deep insert” requests to create an item together with one or more related entities (for example, item partners).
- For existing sales quotations, you can update the header, header partner, header pricing element, header text, item, item partner, item pricing element, and item text.
- For existing sales quotations, you can delete the header, header partner, header pricing element, header text, header related object, item, item partner, item pricing element, item text, and item related object.
- You can accept or deny approval requests for sales quotations that cannot be processed without the consent of an approver.
Constructor
Gets invoked to initialize the connector
.
init (ConnectionConfig config, string hostname, int port)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- hostname string -
- port int 443 -
createA_SalesQuotation
function createA_SalesQuotation(CreateA_SalesQuotation payload, map<string|string[]> headers) returns A_SalesQuotationWrapper|error
Creates a sales quotation.
Parameters
- payload CreateA_SalesQuotation - New entity
Return Type
- A_SalesQuotationWrapper|error - Created entity
createA_SalesQuotationItem
function createA_SalesQuotationItem(CreateA_SalesQuotationItem payload, map<string|string[]> headers) returns A_SalesQuotationItemWrapper|error
Creates a sales quotation item.
Parameters
- payload CreateA_SalesQuotationItem - New entity
Return Type
- A_SalesQuotationItemWrapper|error - Created entity
createA_SalesQuotationRelatedObject
function createA_SalesQuotationRelatedObject(CreateA_SalesQuotationRelatedObject payload, map<string|string[]> headers) returns A_SalesQuotationRelatedObjectWrapper|error
Creates a related object for a sales quotation.
Parameters
- payload CreateA_SalesQuotationRelatedObject - New entity
Return Type
- A_SalesQuotationRelatedObjectWrapper|error - Created entity
createA_SlsQtanItemRelatedObject
function createA_SlsQtanItemRelatedObject(CreateA_SlsQtanItemRelatedObject payload, map<string|string[]> headers) returns A_SlsQtanItemRelatedObjectWrapper|error
Creates a related object for a sales quotation item.
Parameters
- payload CreateA_SlsQtanItemRelatedObject - New entity
Return Type
- A_SlsQtanItemRelatedObjectWrapper|error - Created entity
createItemOfA_SalesQuotation
function createItemOfA_SalesQuotation(string SalesQuotation, CreateA_SalesQuotationItem payload, map<string|string[]> headers) returns A_SalesQuotationItemWrapper|error
Creates a sales quotation item for a specific sales quotation.
Return Type
- A_SalesQuotationItemWrapper|error - Created entity
createRelatedObjectOfA_SalesQuotation
function createRelatedObjectOfA_SalesQuotation(string SalesQuotation, CreateA_SalesQuotationRelatedObject payload, map<string|string[]> headers) returns A_SalesQuotationRelatedObjectWrapper|error
Creates related objects for a sales quotation.
Parameters
- SalesQuotation string - Sales Quotation
- payload CreateA_SalesQuotationRelatedObject - New entity
Return Type
- A_SalesQuotationRelatedObjectWrapper|error - Created entity
createRelatedObjectOfA_SalesQuotationItem
function createRelatedObjectOfA_SalesQuotationItem(string SalesQuotation, string SalesQuotationItem, CreateA_SlsQtanItemRelatedObject payload, map<string|string[]> headers) returns A_SlsQtanItemRelatedObjectWrapper|error
Creates related objects for a sales quotation item.
Parameters
- SalesQuotation string - Sales Quotation
- SalesQuotationItem string - Sales Quotation Item
- payload CreateA_SlsQtanItemRelatedObject - New entity
Return Type
- A_SlsQtanItemRelatedObjectWrapper|error - Created entity
deleteA_SalesQuotation
function deleteA_SalesQuotation(string SalesQuotation, map<string|string[]> headers) returns Response|error
Deletes a specific sales quotation.
Parameters
- SalesQuotation string - Sales Quotation
deleteA_SalesQuotationItem
function deleteA_SalesQuotationItem(string SalesQuotation, string SalesQuotationItem, map<string|string[]> headers) returns Response|error
Deletes a specific sales quotation item.
deleteA_SalesQuotationItemPartner
function deleteA_SalesQuotationItemPartner(string SalesQuotation, string SalesQuotationItem, string PartnerFunction, map<string|string[]> headers) returns Response|error
Deletes the item partners of a specific item and with a specific partner function in a specific sales quotation.
Parameters
- SalesQuotation string - Sales and Distribution Document Number
- SalesQuotationItem string - Item number of the SD document
- PartnerFunction string - Partner Function
deleteA_SalesQuotationItemPrcgElmnt
function deleteA_SalesQuotationItemPrcgElmnt(string SalesQuotation, string SalesQuotationItem, string PricingProcedureStep, string PricingProcedureCounter, map<string|string[]> headers) returns Response|error
Deletes an item pricing element.
Parameters
- SalesQuotation string - Sales Document
- SalesQuotationItem string - Condition item number
- PricingProcedureStep string - Step Number
- PricingProcedureCounter string - Condition Counter
deleteA_SalesQuotationItemText
function deleteA_SalesQuotationItemText(string SalesQuotation, string SalesQuotationItem, string Language, string LongTextID, map<string|string[]> headers) returns Response|error
Deletes a specific item text.
Parameters
- SalesQuotation string - Sales Quotation
- SalesQuotationItem string - Sales Quotation Item
- Language string - Language Key
- LongTextID string - Text ID
deleteA_SalesQuotationPartner
function deleteA_SalesQuotationPartner(string SalesQuotation, string PartnerFunction, map<string|string[]> headers) returns Response|error
Deletes the header partners of a specific sales quotation and with a specific partner function.
Parameters
- SalesQuotation string - Sales and Distribution Document Number
- PartnerFunction string - Partner Function
deleteA_SalesQuotationPrcgElmnt
function deleteA_SalesQuotationPrcgElmnt(string SalesQuotation, string PricingProcedureStep, string PricingProcedureCounter, map<string|string[]> headers) returns Response|error
Deletes a header pricing element for a specific sales quotation.
Parameters
- SalesQuotation string - Sales Document
- PricingProcedureStep string - Step Number
- PricingProcedureCounter string - Condition Counter
deleteA_SalesQuotationRelatedObject
function deleteA_SalesQuotationRelatedObject(string SalesQuotation, string SDDocRelatedObjectSequenceNmbr, map<string|string[]> headers) returns Response|error
Deletes a related object from a sales quotation.
Parameters
- SalesQuotation string - Sales Quotation
- SDDocRelatedObjectSequenceNmbr string - Sequence Number of the Related Object of an SD Document
deleteA_SalesQuotationText
function deleteA_SalesQuotationText(string SalesQuotation, string Language, string LongTextID, map<string|string[]> headers) returns Response|error
Deletes a specific header text for a specific sales quotation.
Parameters
- SalesQuotation string - Sales Quotation
- Language string - Language Key
- LongTextID string - Text ID
deleteA_SlsQtanItemRelatedObject
function deleteA_SlsQtanItemRelatedObject(string SalesQuotation, string SalesQuotationItem, string SDDocRelatedObjectSequenceNmbr, map<string|string[]> headers) returns Response|error
Deletes a related object from a sales quotation item.
Parameters
- SalesQuotation string - Sales Quotation
- SalesQuotationItem string - Sales Quotation Item
- SDDocRelatedObjectSequenceNmbr string - Sequence Number of the Related Object of an SD Document
getA_SalesQuotation
function getA_SalesQuotation(string SalesQuotation, map<string|string[]> headers, *GetA_SalesQuotationQueries queries) returns A_SalesQuotationWrapper|error
Reads the header of a specific sales quotation.
Parameters
- SalesQuotation string - Sales Quotation
- queries *GetA_SalesQuotationQueries - Queries to be sent with the request
Return Type
- A_SalesQuotationWrapper|error - Retrieved entity
getA_SalesQuotationItem
function getA_SalesQuotationItem(string SalesQuotation, string SalesQuotationItem, map<string|string[]> headers, *GetA_SalesQuotationItemQueries queries) returns A_SalesQuotationItemWrapper|error
Reads a specific sales quotation item.
Parameters
- SalesQuotation string - Sales Quotation
- SalesQuotationItem string - Sales Quotation Item
- queries *GetA_SalesQuotationItemQueries - Queries to be sent with the request
Return Type
- A_SalesQuotationItemWrapper|error - Retrieved entity
getA_SalesQuotationItemPartner
function getA_SalesQuotationItemPartner(string SalesQuotation, string SalesQuotationItem, string PartnerFunction, map<string|string[]> headers, *GetA_SalesQuotationItemPartnerQueries queries) returns A_SalesQuotationItemPartnerWrapper|error
Reads the item partners of a specific item and with a specific partner function in a specific sales quotation.
Parameters
- SalesQuotation string - Sales and Distribution Document Number
- SalesQuotationItem string - Item number of the SD document
- PartnerFunction string - Partner Function
- queries *GetA_SalesQuotationItemPartnerQueries - Queries to be sent with the request
Return Type
- A_SalesQuotationItemPartnerWrapper|error - Retrieved entity
getA_SalesQuotationItemPrcgElmnt
function getA_SalesQuotationItemPrcgElmnt(string SalesQuotation, string SalesQuotationItem, string PricingProcedureStep, string PricingProcedureCounter, map<string|string[]> headers, *GetA_SalesQuotationItemPrcgElmntQueries queries) returns A_SalesQuotationItemPrcgElmntWrapper|error
Reads the pricing element of a specific sales quotation item.
Parameters
- SalesQuotation string - Sales Document
- SalesQuotationItem string - Condition item number
- PricingProcedureStep string - Step Number
- PricingProcedureCounter string - Condition Counter
- queries *GetA_SalesQuotationItemPrcgElmntQueries - Queries to be sent with the request
Return Type
- A_SalesQuotationItemPrcgElmntWrapper|error - Retrieved entity
getA_SalesQuotationItemText
function getA_SalesQuotationItemText(string SalesQuotation, string SalesQuotationItem, string Language, string LongTextID, map<string|string[]> headers, *GetA_SalesQuotationItemTextQueries queries) returns A_SalesQuotationItemTextWrapper|error
Reads a specific item text.
Parameters
- SalesQuotation string - Sales Quotation
- SalesQuotationItem string - Sales Quotation Item
- Language string - Language Key
- LongTextID string - Text ID
- queries *GetA_SalesQuotationItemTextQueries - Queries to be sent with the request
Return Type
- A_SalesQuotationItemTextWrapper|error - Retrieved entity
getA_SalesQuotationPartner
function getA_SalesQuotationPartner(string SalesQuotation, string PartnerFunction, map<string|string[]> headers, *GetA_SalesQuotationPartnerQueries queries) returns A_SalesQuotationPartnerWrapper|error
Reads the header partners of a specific sales quotation and with a specific partner function.
Parameters
- SalesQuotation string - Sales and Distribution Document Number
- PartnerFunction string - Partner Function
- queries *GetA_SalesQuotationPartnerQueries - Queries to be sent with the request
Return Type
- A_SalesQuotationPartnerWrapper|error - Retrieved entity
getA_SalesQuotationPrcgElmnt
function getA_SalesQuotationPrcgElmnt(string SalesQuotation, string PricingProcedureStep, string PricingProcedureCounter, map<string|string[]> headers, *GetA_SalesQuotationPrcgElmntQueries queries) returns A_SalesQuotationPrcgElmntWrapper|error
Reads the header pricing element for a specific sales quotation.
Parameters
- SalesQuotation string - Sales Document
- PricingProcedureStep string - Step Number
- PricingProcedureCounter string - Condition Counter
- queries *GetA_SalesQuotationPrcgElmntQueries - Queries to be sent with the request
Return Type
- A_SalesQuotationPrcgElmntWrapper|error - Retrieved entity
getA_SalesQuotationRelatedObject
function getA_SalesQuotationRelatedObject(string SalesQuotation, string SDDocRelatedObjectSequenceNmbr, map<string|string[]> headers, *GetA_SalesQuotationRelatedObjectQueries queries) returns A_SalesQuotationRelatedObjectWrapper|error
Reads a related object of a sales quotation.
Parameters
- SalesQuotation string - Sales Quotation
- SDDocRelatedObjectSequenceNmbr string - Sequence Number of the Related Object of an SD Document
- queries *GetA_SalesQuotationRelatedObjectQueries - Queries to be sent with the request
Return Type
- A_SalesQuotationRelatedObjectWrapper|error - Retrieved entity
getA_SalesQuotationText
function getA_SalesQuotationText(string SalesQuotation, string Language, string LongTextID, map<string|string[]> headers, *GetA_SalesQuotationTextQueries queries) returns A_SalesQuotationTextWrapper|error
Reads the header texts of a specific sales quotation.
Parameters
- SalesQuotation string - Sales Quotation
- Language string - Language Key
- LongTextID string - Text ID
- queries *GetA_SalesQuotationTextQueries - Queries to be sent with the request
Return Type
- A_SalesQuotationTextWrapper|error - Retrieved entity
getA_SlsQtanItemRelatedObject
function getA_SlsQtanItemRelatedObject(string SalesQuotation, string SalesQuotationItem, string SDDocRelatedObjectSequenceNmbr, map<string|string[]> headers, *GetA_SlsQtanItemRelatedObjectQueries queries) returns A_SlsQtanItemRelatedObjectWrapper|error
Reads a related object from a sales quotation item.
Parameters
- SalesQuotation string - Sales Quotation
- SalesQuotationItem string - Sales Quotation Item
- SDDocRelatedObjectSequenceNmbr string - Sequence Number of the Related Object of an SD Document
- queries *GetA_SlsQtanItemRelatedObjectQueries - Queries to be sent with the request
Return Type
- A_SlsQtanItemRelatedObjectWrapper|error - Retrieved entity
getA_SlsQtanItmPrecdgProcFlow
function getA_SlsQtanItmPrecdgProcFlow(string SalesQuotation, string SalesQuotationItem, string DocRelationshipUUID, map<string|string[]> headers, *GetA_SlsQtanItmPrecdgProcFlowQueries queries) returns A_SlsQtanItmPrecdgProcFlowWrapper|error
Reads a preceding item of a sales quotation item.
Parameters
- SalesQuotation string - Subsequent Sales and Distribution Document
- SalesQuotationItem string - Subsequent Item of an SD Document
- DocRelationshipUUID string - SD Unique Document Relationship Identification
- queries *GetA_SlsQtanItmPrecdgProcFlowQueries - Queries to be sent with the request
Return Type
- A_SlsQtanItmPrecdgProcFlowWrapper|error - Retrieved entity
getA_SlsQtanItmSubsqntProcFlow
function getA_SlsQtanItmSubsqntProcFlow(string SalesQuotation, string SalesQuotationItem, string DocRelationshipUUID, map<string|string[]> headers, *GetA_SlsQtanItmSubsqntProcFlowQueries queries) returns A_SlsQtanItmSubsqntProcFlowWrapper|error
Reads a subsequent item of a sales quotation item.
Parameters
- SalesQuotation string - Preceding sales and distribution document
- SalesQuotationItem string - Preceding Item of an SD Document
- DocRelationshipUUID string - SD Unique Document Relationship Identification
- queries *GetA_SlsQtanItmSubsqntProcFlowQueries - Queries to be sent with the request
Return Type
- A_SlsQtanItmSubsqntProcFlowWrapper|error - Retrieved entity
getA_SlsQtanPrecdgProcFlow
function getA_SlsQtanPrecdgProcFlow(string SalesQuotation, string DocRelationshipUUID, map<string|string[]> headers, *GetA_SlsQtanPrecdgProcFlowQueries queries) returns A_SlsQtanPrecdgProcFlowWrapper|error
Reads a preceding document of a sales quotation.
Parameters
- SalesQuotation string - Subsequent Sales and Distribution Document
- DocRelationshipUUID string - SD Unique Document Relationship Identification
- queries *GetA_SlsQtanPrecdgProcFlowQueries - Queries to be sent with the request
Return Type
- A_SlsQtanPrecdgProcFlowWrapper|error - Retrieved entity
getA_SlsQtanSubsqntProcFlow
function getA_SlsQtanSubsqntProcFlow(string SalesQuotation, string DocRelationshipUUID, map<string|string[]> headers, *GetA_SlsQtanSubsqntProcFlowQueries queries) returns A_SlsQtanSubsqntProcFlowWrapper|error
Reads a subsequent document of a sales quotation.
Parameters
- SalesQuotation string - Preceding sales and distribution document
- DocRelationshipUUID string - SD Unique Document Relationship Identification
- queries *GetA_SlsQtanSubsqntProcFlowQueries - Queries to be sent with the request
Return Type
- A_SlsQtanSubsqntProcFlowWrapper|error - Retrieved entity
getSalesQuotationItemOfA_SalesQuotationItemPartner
function getSalesQuotationItemOfA_SalesQuotationItemPartner(string SalesQuotation, string SalesQuotationItem, string PartnerFunction, map<string|string[]> headers, *GetSalesQuotationItemOfA_SalesQuotationItemPartnerQueries queries) returns A_SalesQuotationItemWrapper|error
Reads the sales quotation item for a specific partner function of a sales quotation item.
Parameters
- SalesQuotation string - Sales and Distribution Document Number
- SalesQuotationItem string - Item number of the SD document
- PartnerFunction string - Partner Function
- queries *GetSalesQuotationItemOfA_SalesQuotationItemPartnerQueries - Queries to be sent with the request
Return Type
- A_SalesQuotationItemWrapper|error - Retrieved entity
getSalesQuotationItemOfA_SalesQuotationItemPrcgElmnt
function getSalesQuotationItemOfA_SalesQuotationItemPrcgElmnt(string SalesQuotation, string SalesQuotationItem, string PricingProcedureStep, string PricingProcedureCounter, map<string|string[]> headers, *GetSalesQuotationItemOfA_SalesQuotationItemPrcgElmntQueries queries) returns A_SalesQuotationItemWrapper|error
Reads the sales quotation item for a specific pricing element.
Parameters
- SalesQuotation string - Sales Document
- SalesQuotationItem string - Condition item number
- PricingProcedureStep string - Step Number
- PricingProcedureCounter string - Condition Counter
- queries *GetSalesQuotationItemOfA_SalesQuotationItemPrcgElmntQueries - Queries to be sent with the request
Return Type
- A_SalesQuotationItemWrapper|error - Retrieved entity
getSalesQuotationItemOfA_SalesQuotationItemText
function getSalesQuotationItemOfA_SalesQuotationItemText(string SalesQuotation, string SalesQuotationItem, string Language, string LongTextID, map<string|string[]> headers, *GetSalesQuotationItemOfA_SalesQuotationItemTextQueries queries) returns A_SalesQuotationItemWrapper|error
Reads the sales quotation item for a specific item text.
Parameters
- SalesQuotation string - Sales Quotation
- SalesQuotationItem string - Sales Quotation Item
- Language string - Language Key
- LongTextID string - Text ID
- queries *GetSalesQuotationItemOfA_SalesQuotationItemTextQueries - Queries to be sent with the request
Return Type
- A_SalesQuotationItemWrapper|error - Retrieved entity
getSalesQuotationItemOfA_SlsQtanItemRelatedObject
function getSalesQuotationItemOfA_SlsQtanItemRelatedObject(string SalesQuotation, string SalesQuotationItem, string SDDocRelatedObjectSequenceNmbr, map<string|string[]> headers, *GetSalesQuotationItemOfA_SlsQtanItemRelatedObjectQueries queries) returns A_SalesQuotationItemWrapper|error
Reads the sales quotation item for a related object of a sales quotation item.
Parameters
- SalesQuotation string - Sales Quotation
- SalesQuotationItem string - Sales Quotation Item
- SDDocRelatedObjectSequenceNmbr string - Sequence Number of the Related Object of an SD Document
- queries *GetSalesQuotationItemOfA_SlsQtanItemRelatedObjectQueries - Queries to be sent with the request
Return Type
- A_SalesQuotationItemWrapper|error - Retrieved entity
getSalesQuotationItemOfA_SlsQtanItmPrecdgProcFlow
function getSalesQuotationItemOfA_SlsQtanItmPrecdgProcFlow(string SalesQuotation, string SalesQuotationItem, string DocRelationshipUUID, map<string|string[]> headers, *GetSalesQuotationItemOfA_SlsQtanItmPrecdgProcFlowQueries queries) returns A_SalesQuotationItemWrapper|error
Reads the sales quotation item for a preceding item of a sales quotation item.
Parameters
- SalesQuotation string - Subsequent Sales and Distribution Document
- SalesQuotationItem string - Subsequent Item of an SD Document
- DocRelationshipUUID string - SD Unique Document Relationship Identification
- queries *GetSalesQuotationItemOfA_SlsQtanItmPrecdgProcFlowQueries - Queries to be sent with the request
Return Type
- A_SalesQuotationItemWrapper|error - Retrieved entity
getSalesQuotationItemOfA_SlsQtanItmSubsqntProcFlow
function getSalesQuotationItemOfA_SlsQtanItmSubsqntProcFlow(string SalesQuotation, string SalesQuotationItem, string DocRelationshipUUID, map<string|string[]> headers, *GetSalesQuotationItemOfA_SlsQtanItmSubsqntProcFlowQueries queries) returns A_SalesQuotationItemWrapper|error
Reads the sales quotation item for a subsequent item of a sales quotation item.
Parameters
- SalesQuotation string - Preceding sales and distribution document
- SalesQuotationItem string - Preceding Item of an SD Document
- DocRelationshipUUID string - SD Unique Document Relationship Identification
- queries *GetSalesQuotationItemOfA_SlsQtanItmSubsqntProcFlowQueries - Queries to be sent with the request
Return Type
- A_SalesQuotationItemWrapper|error - Retrieved entity
getSalesQuotationOfA_SalesQuotationItem
function getSalesQuotationOfA_SalesQuotationItem(string SalesQuotation, string SalesQuotationItem, map<string|string[]> headers, *GetSalesQuotationOfA_SalesQuotationItemQueries queries) returns A_SalesQuotationWrapper|error
Reads the sales quotation header for a specific item.
Parameters
- SalesQuotation string - Sales Quotation
- SalesQuotationItem string - Sales Quotation Item
- queries *GetSalesQuotationOfA_SalesQuotationItemQueries - Queries to be sent with the request
Return Type
- A_SalesQuotationWrapper|error - Retrieved entity
getSalesQuotationOfA_SalesQuotationItemPartner
function getSalesQuotationOfA_SalesQuotationItemPartner(string SalesQuotation, string SalesQuotationItem, string PartnerFunction, map<string|string[]> headers, *GetSalesQuotationOfA_SalesQuotationItemPartnerQueries queries) returns A_SalesQuotationWrapper|error
Reads the sales quotation header for a specific partner function of a sales quotation item.
Parameters
- SalesQuotation string - Sales and Distribution Document Number
- SalesQuotationItem string - Item number of the SD document
- PartnerFunction string - Partner Function
- queries *GetSalesQuotationOfA_SalesQuotationItemPartnerQueries - Queries to be sent with the request
Return Type
- A_SalesQuotationWrapper|error - Retrieved entity
getSalesQuotationOfA_SalesQuotationItemPrcgElmnt
function getSalesQuotationOfA_SalesQuotationItemPrcgElmnt(string SalesQuotation, string SalesQuotationItem, string PricingProcedureStep, string PricingProcedureCounter, map<string|string[]> headers, *GetSalesQuotationOfA_SalesQuotationItemPrcgElmntQueries queries) returns A_SalesQuotationWrapper|error
Reads the sales quotation header for a specific pricing element.
Parameters
- SalesQuotation string - Sales Document
- SalesQuotationItem string - Condition item number
- PricingProcedureStep string - Step Number
- PricingProcedureCounter string - Condition Counter
- queries *GetSalesQuotationOfA_SalesQuotationItemPrcgElmntQueries - Queries to be sent with the request
Return Type
- A_SalesQuotationWrapper|error - Retrieved entity
getSalesQuotationOfA_SalesQuotationItemText
function getSalesQuotationOfA_SalesQuotationItemText(string SalesQuotation, string SalesQuotationItem, string Language, string LongTextID, map<string|string[]> headers, *GetSalesQuotationOfA_SalesQuotationItemTextQueries queries) returns A_SalesQuotationWrapper|error
Reads the sales quotation header for a specific text of a sales quotation item.
Parameters
- SalesQuotation string - Sales Quotation
- SalesQuotationItem string - Sales Quotation Item
- Language string - Language Key
- LongTextID string - Text ID
- queries *GetSalesQuotationOfA_SalesQuotationItemTextQueries - Queries to be sent with the request
Return Type
- A_SalesQuotationWrapper|error - Retrieved entity
getSalesQuotationOfA_SalesQuotationPartner
function getSalesQuotationOfA_SalesQuotationPartner(string SalesQuotation, string PartnerFunction, map<string|string[]> headers, *GetSalesQuotationOfA_SalesQuotationPartnerQueries queries) returns A_SalesQuotationWrapper|error
Reads the sales quotation header for a specific header partner.
Parameters
- SalesQuotation string - Sales and Distribution Document Number
- PartnerFunction string - Partner Function
- queries *GetSalesQuotationOfA_SalesQuotationPartnerQueries - Queries to be sent with the request
Return Type
- A_SalesQuotationWrapper|error - Retrieved entity
getSalesQuotationOfA_SalesQuotationPrcgElmnt
function getSalesQuotationOfA_SalesQuotationPrcgElmnt(string SalesQuotation, string PricingProcedureStep, string PricingProcedureCounter, map<string|string[]> headers, *GetSalesQuotationOfA_SalesQuotationPrcgElmntQueries queries) returns A_SalesQuotationWrapper|error
Reads the sales quotation header for a specific pricing element.
Parameters
- SalesQuotation string - Sales Document
- PricingProcedureStep string - Step Number
- PricingProcedureCounter string - Condition Counter
- queries *GetSalesQuotationOfA_SalesQuotationPrcgElmntQueries - Queries to be sent with the request
Return Type
- A_SalesQuotationWrapper|error - Retrieved entity
getSalesQuotationOfA_SalesQuotationRelatedObject
function getSalesQuotationOfA_SalesQuotationRelatedObject(string SalesQuotation, string SDDocRelatedObjectSequenceNmbr, map<string|string[]> headers, *GetSalesQuotationOfA_SalesQuotationRelatedObjectQueries queries) returns A_SalesQuotationWrapper|error
Reads the sales quotation header for a related object of a sales quotation.
Parameters
- SalesQuotation string - Sales Quotation
- SDDocRelatedObjectSequenceNmbr string - Sequence Number of the Related Object of an SD Document
- queries *GetSalesQuotationOfA_SalesQuotationRelatedObjectQueries - Queries to be sent with the request
Return Type
- A_SalesQuotationWrapper|error - Retrieved entity
getSalesQuotationOfA_SalesQuotationText
function getSalesQuotationOfA_SalesQuotationText(string SalesQuotation, string Language, string LongTextID, map<string|string[]> headers, *GetSalesQuotationOfA_SalesQuotationTextQueries queries) returns A_SalesQuotationWrapper|error
Reads the sales quotation header for a specific header text.
Parameters
- SalesQuotation string - Sales Quotation
- Language string - Language Key
- LongTextID string - Text ID
- queries *GetSalesQuotationOfA_SalesQuotationTextQueries - Queries to be sent with the request
Return Type
- A_SalesQuotationWrapper|error - Retrieved entity
getSalesQuotationOfA_SlsQtanItemRelatedObject
function getSalesQuotationOfA_SlsQtanItemRelatedObject(string SalesQuotation, string SalesQuotationItem, string SDDocRelatedObjectSequenceNmbr, map<string|string[]> headers, *GetSalesQuotationOfA_SlsQtanItemRelatedObjectQueries queries) returns A_SalesQuotationWrapper|error
Reads the sales quotation header for a related object of a sales quotation item.
Parameters
- SalesQuotation string - Sales Quotation
- SalesQuotationItem string - Sales Quotation Item
- SDDocRelatedObjectSequenceNmbr string - Sequence Number of the Related Object of an SD Document
- queries *GetSalesQuotationOfA_SlsQtanItemRelatedObjectQueries - Queries to be sent with the request
Return Type
- A_SalesQuotationWrapper|error - Retrieved entity
getSalesQuotationOfA_SlsQtanItmPrecdgProcFlow
function getSalesQuotationOfA_SlsQtanItmPrecdgProcFlow(string SalesQuotation, string SalesQuotationItem, string DocRelationshipUUID, map<string|string[]> headers, *GetSalesQuotationOfA_SlsQtanItmPrecdgProcFlowQueries queries) returns A_SalesQuotationWrapper|error
Reads the sales quotation header for a preceding item of a sales quotation item.
Parameters
- SalesQuotation string - Subsequent Sales and Distribution Document
- SalesQuotationItem string - Subsequent Item of an SD Document
- DocRelationshipUUID string - SD Unique Document Relationship Identification
- queries *GetSalesQuotationOfA_SlsQtanItmPrecdgProcFlowQueries - Queries to be sent with the request
Return Type
- A_SalesQuotationWrapper|error - Retrieved entity
getSalesQuotationOfA_SlsQtanItmSubsqntProcFlow
function getSalesQuotationOfA_SlsQtanItmSubsqntProcFlow(string SalesQuotation, string SalesQuotationItem, string DocRelationshipUUID, map<string|string[]> headers, *GetSalesQuotationOfA_SlsQtanItmSubsqntProcFlowQueries queries) returns A_SalesQuotationWrapper|error
Reads the sales quotation header for a subsequent item of a sales quotation item.
Parameters
- SalesQuotation string - Preceding sales and distribution document
- SalesQuotationItem string - Preceding Item of an SD Document
- DocRelationshipUUID string - SD Unique Document Relationship Identification
- queries *GetSalesQuotationOfA_SlsQtanItmSubsqntProcFlowQueries - Queries to be sent with the request
Return Type
- A_SalesQuotationWrapper|error - Retrieved entity
getSalesQuotationOfA_SlsQtanPrecdgProcFlow
function getSalesQuotationOfA_SlsQtanPrecdgProcFlow(string SalesQuotation, string DocRelationshipUUID, map<string|string[]> headers, *GetSalesQuotationOfA_SlsQtanPrecdgProcFlowQueries queries) returns A_SalesQuotationWrapper|error
Reads the sales quotation header for a preceding document of a sales quotation.
Parameters
- SalesQuotation string - Subsequent Sales and Distribution Document
- DocRelationshipUUID string - SD Unique Document Relationship Identification
- queries *GetSalesQuotationOfA_SlsQtanPrecdgProcFlowQueries - Queries to be sent with the request
Return Type
- A_SalesQuotationWrapper|error - Retrieved entity
getSalesQuotationOfA_SlsQtanSubsqntProcFlow
function getSalesQuotationOfA_SlsQtanSubsqntProcFlow(string SalesQuotation, string DocRelationshipUUID, map<string|string[]> headers, *GetSalesQuotationOfA_SlsQtanSubsqntProcFlowQueries queries) returns A_SalesQuotationWrapper|error
Reads the sales quotation header for a subsequent document of a sales quotation.
Parameters
- SalesQuotation string - Preceding sales and distribution document
- DocRelationshipUUID string - SD Unique Document Relationship Identification
- queries *GetSalesQuotationOfA_SlsQtanSubsqntProcFlowQueries - Queries to be sent with the request
Return Type
- A_SalesQuotationWrapper|error - Retrieved entity
listA_SalesQuotationItemPartners
function listA_SalesQuotationItemPartners(map<string|string[]> headers, *ListA_SalesQuotationItemPartnersQueries queries) returns CollectionOfA_SalesQuotationItemPartnerWrapper|error
Reads the item partners for all sales quotations.
Parameters
- queries *ListA_SalesQuotationItemPartnersQueries - Queries to be sent with the request
Return Type
- CollectionOfA_SalesQuotationItemPartnerWrapper|error - Retrieved entities
listA_SalesQuotationItemPrcgElmnts
function listA_SalesQuotationItemPrcgElmnts(map<string|string[]> headers, *ListA_SalesQuotationItemPrcgElmntsQueries queries) returns CollectionOfA_SalesQuotationItemPrcgElmntWrapper|error
Reads the item pricing elements of all sales quotations.
Parameters
- queries *ListA_SalesQuotationItemPrcgElmntsQueries - Queries to be sent with the request
Return Type
- CollectionOfA_SalesQuotationItemPrcgElmntWrapper|error - Retrieved entities
listA_SalesQuotationItemTexts
function listA_SalesQuotationItemTexts(map<string|string[]> headers, *ListA_SalesQuotationItemTextsQueries queries) returns CollectionOfA_SalesQuotationItemTextWrapper|error
Reads item texts of all sales quotations.
Parameters
- queries *ListA_SalesQuotationItemTextsQueries - Queries to be sent with the request
Return Type
- CollectionOfA_SalesQuotationItemTextWrapper|error - Retrieved entities
listA_SalesQuotationItems
function listA_SalesQuotationItems(map<string|string[]> headers, *ListA_SalesQuotationItemsQueries queries) returns CollectionOfA_SalesQuotationItemWrapper|error
Reads all sales quotation items.
Parameters
- queries *ListA_SalesQuotationItemsQueries - Queries to be sent with the request
Return Type
- CollectionOfA_SalesQuotationItemWrapper|error - Retrieved entities
listA_SalesQuotationPartners
function listA_SalesQuotationPartners(map<string|string[]> headers, *ListA_SalesQuotationPartnersQueries queries) returns CollectionOfA_SalesQuotationPartnerWrapper|error
Reads the header partners of all sales quotations.
Parameters
- queries *ListA_SalesQuotationPartnersQueries - Queries to be sent with the request
Return Type
- CollectionOfA_SalesQuotationPartnerWrapper|error - Retrieved entities
listA_SalesQuotationPrcgElmnts
function listA_SalesQuotationPrcgElmnts(map<string|string[]> headers, *ListA_SalesQuotationPrcgElmntsQueries queries) returns CollectionOfA_SalesQuotationPrcgElmntWrapper|error
Reads the header pricing elements of all sales quotations.
Parameters
- queries *ListA_SalesQuotationPrcgElmntsQueries - Queries to be sent with the request
Return Type
- CollectionOfA_SalesQuotationPrcgElmntWrapper|error - Retrieved entities
listA_SalesQuotationRelatedObjects
function listA_SalesQuotationRelatedObjects(map<string|string[]> headers, *ListA_SalesQuotationRelatedObjectsQueries queries) returns CollectionOfA_SalesQuotationRelatedObjectWrapper|error
Reads the related objects of all sales quotations.
Parameters
- queries *ListA_SalesQuotationRelatedObjectsQueries - Queries to be sent with the request
Return Type
- CollectionOfA_SalesQuotationRelatedObjectWrapper|error - Retrieved entities
listA_SalesQuotationTexts
function listA_SalesQuotationTexts(map<string|string[]> headers, *ListA_SalesQuotationTextsQueries queries) returns CollectionOfA_SalesQuotationTextWrapper|error
Reads the header texts of all sales quotations.
Parameters
- queries *ListA_SalesQuotationTextsQueries - Queries to be sent with the request
Return Type
- CollectionOfA_SalesQuotationTextWrapper|error - Retrieved entities
listA_SalesQuotations
function listA_SalesQuotations(map<string|string[]> headers, *ListA_SalesQuotationsQueries queries) returns CollectionOfA_SalesQuotationWrapper|error
Reads all sales quotation headers.
Parameters
- queries *ListA_SalesQuotationsQueries - Queries to be sent with the request
Return Type
- CollectionOfA_SalesQuotationWrapper|error - Retrieved entities
listA_SlsQtanItemRelatedObjects
function listA_SlsQtanItemRelatedObjects(map<string|string[]> headers, *ListA_SlsQtanItemRelatedObjectsQueries queries) returns CollectionOfA_SlsQtanItemRelatedObjectWrapper|error
Reads related objects from all sales quotation items.
Parameters
- queries *ListA_SlsQtanItemRelatedObjectsQueries - Queries to be sent with the request
Return Type
- CollectionOfA_SlsQtanItemRelatedObjectWrapper|error - Retrieved entities
listA_SlsQtanItmPrecdgProcFlows
function listA_SlsQtanItmPrecdgProcFlows(map<string|string[]> headers, *ListA_SlsQtanItmPrecdgProcFlowsQueries queries) returns CollectionOfA_SlsQtanItmPrecdgProcFlowWrapper|error
Reads the preceding items of all sales quotation items.
Parameters
- queries *ListA_SlsQtanItmPrecdgProcFlowsQueries - Queries to be sent with the request
Return Type
- CollectionOfA_SlsQtanItmPrecdgProcFlowWrapper|error - Retrieved entities
listA_SlsQtanItmSubsqntProcFlows
function listA_SlsQtanItmSubsqntProcFlows(map<string|string[]> headers, *ListA_SlsQtanItmSubsqntProcFlowsQueries queries) returns CollectionOfA_SlsQtanItmSubsqntProcFlowWrapper|error
Reads the subsequent items of all sales quotation items.
Parameters
- queries *ListA_SlsQtanItmSubsqntProcFlowsQueries - Queries to be sent with the request
Return Type
- CollectionOfA_SlsQtanItmSubsqntProcFlowWrapper|error - Retrieved entities
listA_SlsQtanPrecdgProcFlows
function listA_SlsQtanPrecdgProcFlows(map<string|string[]> headers, *ListA_SlsQtanPrecdgProcFlowsQueries queries) returns CollectionOfA_SlsQtanPrecdgProcFlowWrapper|error
Reads the preceding documents of all sales quotations.
Parameters
- queries *ListA_SlsQtanPrecdgProcFlowsQueries - Queries to be sent with the request
Return Type
- CollectionOfA_SlsQtanPrecdgProcFlowWrapper|error - Retrieved entities
listA_SlsQtanSubsqntProcFlows
function listA_SlsQtanSubsqntProcFlows(map<string|string[]> headers, *ListA_SlsQtanSubsqntProcFlowsQueries queries) returns CollectionOfA_SlsQtanSubsqntProcFlowWrapper|error
Reads the subsequent documents of all sales quotations.
Parameters
- queries *ListA_SlsQtanSubsqntProcFlowsQueries - Queries to be sent with the request
Return Type
- CollectionOfA_SlsQtanSubsqntProcFlowWrapper|error - Retrieved entities
listItemsOfA_SalesQuotation
function listItemsOfA_SalesQuotation(string SalesQuotation, map<string|string[]> headers, *ListItemsOfA_SalesQuotationQueries queries) returns CollectionOfA_SalesQuotationItemWrapper|error
Reads all items of a specific sales quotation.
Parameters
- SalesQuotation string - Sales Quotation
- queries *ListItemsOfA_SalesQuotationQueries - Queries to be sent with the request
Return Type
- CollectionOfA_SalesQuotationItemWrapper|error - Retrieved entities
listPartnersOfA_SalesQuotation
function listPartnersOfA_SalesQuotation(string SalesQuotation, map<string|string[]> headers, *ListPartnersOfA_SalesQuotationQueries queries) returns CollectionOfA_SalesQuotationPartnerWrapper|error
Reads the header partners of a specific sales quotation.
Parameters
- SalesQuotation string - Sales Quotation
- queries *ListPartnersOfA_SalesQuotationQueries - Queries to be sent with the request
Return Type
- CollectionOfA_SalesQuotationPartnerWrapper|error - Retrieved entities
listPartnersOfA_SalesQuotationItem
function listPartnersOfA_SalesQuotationItem(string SalesQuotation, string SalesQuotationItem, map<string|string[]> headers, *ListPartnersOfA_SalesQuotationItemQueries queries) returns CollectionOfA_SalesQuotationItemPartnerWrapper|error
Reads the item partners of a specific sales quotation item.
Parameters
- SalesQuotation string - Sales Quotation
- SalesQuotationItem string - Sales Quotation Item
- queries *ListPartnersOfA_SalesQuotationItemQueries - Queries to be sent with the request
Return Type
- CollectionOfA_SalesQuotationItemPartnerWrapper|error - Retrieved entities
listPrecedingProcFlowDocItemsOfA_SalesQuotationItem
function listPrecedingProcFlowDocItemsOfA_SalesQuotationItem(string SalesQuotation, string SalesQuotationItem, map<string|string[]> headers, *ListPrecedingProcFlowDocItemsOfA_SalesQuotationItemQueries queries) returns CollectionOfA_SlsQtanItmPrecdgProcFlowWrapper|error
Reads the preceding items of a sales quotation item.
Parameters
- SalesQuotation string - Sales Quotation
- SalesQuotationItem string - Sales Quotation Item
- queries *ListPrecedingProcFlowDocItemsOfA_SalesQuotationItemQueries - Queries to be sent with the request
Return Type
- CollectionOfA_SlsQtanItmPrecdgProcFlowWrapper|error - Retrieved entities
listPrecedingProcFlowDocsOfA_SalesQuotation
function listPrecedingProcFlowDocsOfA_SalesQuotation(string SalesQuotation, map<string|string[]> headers, *ListPrecedingProcFlowDocsOfA_SalesQuotationQueries queries) returns CollectionOfA_SlsQtanPrecdgProcFlowWrapper|error
Reads the preceding documents of a sales quotation.
Parameters
- SalesQuotation string - Sales Quotation
- queries *ListPrecedingProcFlowDocsOfA_SalesQuotationQueries - Queries to be sent with the request
Return Type
- CollectionOfA_SlsQtanPrecdgProcFlowWrapper|error - Retrieved entities
listPricingElementsOfA_SalesQuotation
function listPricingElementsOfA_SalesQuotation(string SalesQuotation, map<string|string[]> headers, *ListPricingElementsOfA_SalesQuotationQueries queries) returns CollectionOfA_SalesQuotationPrcgElmntWrapper|error
Reads the header pricing element of a specific sales quotation.
Parameters
- SalesQuotation string - Sales Quotation
- queries *ListPricingElementsOfA_SalesQuotationQueries - Queries to be sent with the request
Return Type
- CollectionOfA_SalesQuotationPrcgElmntWrapper|error - Retrieved entities
listPricingElementsOfA_SalesQuotationItem
function listPricingElementsOfA_SalesQuotationItem(string SalesQuotation, string SalesQuotationItem, map<string|string[]> headers, *ListPricingElementsOfA_SalesQuotationItemQueries queries) returns CollectionOfA_SalesQuotationItemPrcgElmntWrapper|error
Reads the pricing element of a specific sales quotation item.
Parameters
- SalesQuotation string - Sales Quotation
- SalesQuotationItem string - Sales Quotation Item
- queries *ListPricingElementsOfA_SalesQuotationItemQueries - Queries to be sent with the request
Return Type
- CollectionOfA_SalesQuotationItemPrcgElmntWrapper|error - Retrieved entities
listRelatedObjectsOfA_SalesQuotation
function listRelatedObjectsOfA_SalesQuotation(string SalesQuotation, map<string|string[]> headers, *ListRelatedObjectsOfA_SalesQuotationQueries queries) returns CollectionOfA_SalesQuotationRelatedObjectWrapper|error
Reads the related objects of a sales quotation.
Parameters
- SalesQuotation string - Sales Quotation
- queries *ListRelatedObjectsOfA_SalesQuotationQueries - Queries to be sent with the request
Return Type
- CollectionOfA_SalesQuotationRelatedObjectWrapper|error - Retrieved entities
listRelatedObjectsOfA_SalesQuotationItem
function listRelatedObjectsOfA_SalesQuotationItem(string SalesQuotation, string SalesQuotationItem, map<string|string[]> headers, *ListRelatedObjectsOfA_SalesQuotationItemQueries queries) returns CollectionOfA_SlsQtanItemRelatedObjectWrapper|error
Reads the related objects of a sales quotation item.
Parameters
- SalesQuotation string - Sales Quotation
- SalesQuotationItem string - Sales Quotation Item
- queries *ListRelatedObjectsOfA_SalesQuotationItemQueries - Queries to be sent with the request
Return Type
- CollectionOfA_SlsQtanItemRelatedObjectWrapper|error - Retrieved entities
listSubsequentProcFlowDocItemsOfA_SalesQuotationItem
function listSubsequentProcFlowDocItemsOfA_SalesQuotationItem(string SalesQuotation, string SalesQuotationItem, map<string|string[]> headers, *ListSubsequentProcFlowDocItemsOfA_SalesQuotationItemQueries queries) returns CollectionOfA_SlsQtanItmSubsqntProcFlowWrapper|error
Reads the subsequent items of a sales quotation item.
Parameters
- SalesQuotation string - Sales Quotation
- SalesQuotationItem string - Sales Quotation Item
- queries *ListSubsequentProcFlowDocItemsOfA_SalesQuotationItemQueries - Queries to be sent with the request
Return Type
- CollectionOfA_SlsQtanItmSubsqntProcFlowWrapper|error - Retrieved entities
listSubsequentProcFlowDocsOfA_SalesQuotation
function listSubsequentProcFlowDocsOfA_SalesQuotation(string SalesQuotation, map<string|string[]> headers, *ListSubsequentProcFlowDocsOfA_SalesQuotationQueries queries) returns CollectionOfA_SlsQtanSubsqntProcFlowWrapper|error
Reads the subsequent documents of a sales quotation.
Parameters
- SalesQuotation string - Sales Quotation
- queries *ListSubsequentProcFlowDocsOfA_SalesQuotationQueries - Queries to be sent with the request
Return Type
- CollectionOfA_SlsQtanSubsqntProcFlowWrapper|error - Retrieved entities
listTextsOfA_SalesQuotation
function listTextsOfA_SalesQuotation(string SalesQuotation, map<string|string[]> headers, *ListTextsOfA_SalesQuotationQueries queries) returns CollectionOfA_SalesQuotationTextWrapper|error
Reads the header texts of a specific sales quotation.
Parameters
- SalesQuotation string - Sales Quotation
- queries *ListTextsOfA_SalesQuotationQueries - Queries to be sent with the request
Return Type
- CollectionOfA_SalesQuotationTextWrapper|error - Retrieved entities
listTextsOfA_SalesQuotationItem
function listTextsOfA_SalesQuotationItem(string SalesQuotation, string SalesQuotationItem, map<string|string[]> headers, *ListTextsOfA_SalesQuotationItemQueries queries) returns CollectionOfA_SalesQuotationItemTextWrapper|error
Reads the text of a specific sales quotation item.
Parameters
- SalesQuotation string - Sales Quotation
- SalesQuotationItem string - Sales Quotation Item
- queries *ListTextsOfA_SalesQuotationItemQueries - Queries to be sent with the request
Return Type
- CollectionOfA_SalesQuotationItemTextWrapper|error - Retrieved entities
patchA_SalesQuotation
function patchA_SalesQuotation(string SalesQuotation, Modified\ A_SalesQuotationType payload, map<string|string[]> headers) returns Response|error
Updates a specific sales quotation.
Parameters
- SalesQuotation string - Sales Quotation
- payload Modified\ A_SalesQuotationType - New property values
patchA_SalesQuotationItem
function patchA_SalesQuotationItem(string SalesQuotation, string SalesQuotationItem, Modified\ A_SalesQuotationItemType payload, map<string|string[]> headers) returns Response|error
Updates a specific sales quotation item.
Parameters
- SalesQuotation string - Sales Quotation
- SalesQuotationItem string - Sales Quotation Item
- payload Modified\ A_SalesQuotationItemType - New property values
patchA_SalesQuotationItemPartner
function patchA_SalesQuotationItemPartner(string SalesQuotation, string SalesQuotationItem, string PartnerFunction, Modified\ A_SalesQuotationItemPartnerType payload, map<string|string[]> headers) returns Response|error
Updates the item partners of a specific item and with a specific partner function in a specific sales quotation.
Parameters
- SalesQuotation string - Sales and Distribution Document Number
- SalesQuotationItem string - Item number of the SD document
- PartnerFunction string - Partner Function
- payload Modified\ A_SalesQuotationItemPartnerType - New property values
patchA_SalesQuotationItemPrcgElmnt
function patchA_SalesQuotationItemPrcgElmnt(string SalesQuotation, string SalesQuotationItem, string PricingProcedureStep, string PricingProcedureCounter, Modified\ A_SalesQuotationItemPrcgElmntType payload, map<string|string[]> headers) returns Response|error
Updates an item pricing element.
Parameters
- SalesQuotation string - Sales Document
- SalesQuotationItem string - Condition item number
- PricingProcedureStep string - Step Number
- PricingProcedureCounter string - Condition Counter
- payload Modified\ A_SalesQuotationItemPrcgElmntType - New property values
patchA_SalesQuotationItemText
function patchA_SalesQuotationItemText(string SalesQuotation, string SalesQuotationItem, string Language, string LongTextID, Modified\ A_SalesQuotationItemTextType payload, map<string|string[]> headers) returns Response|error
Updates a specific item text.
Parameters
- SalesQuotation string - Sales Quotation
- SalesQuotationItem string - Sales Quotation Item
- Language string - Language Key
- LongTextID string - Text ID
- payload Modified\ A_SalesQuotationItemTextType - New property values
patchA_SalesQuotationPartner
function patchA_SalesQuotationPartner(string SalesQuotation, string PartnerFunction, Modified\ A_SalesQuotationPartnerType payload, map<string|string[]> headers) returns Response|error
Updates the header partners of a specific sales quotation and with a specific partner function.
Parameters
- SalesQuotation string - Sales and Distribution Document Number
- PartnerFunction string - Partner Function
- payload Modified\ A_SalesQuotationPartnerType - New property values
patchA_SalesQuotationPrcgElmnt
function patchA_SalesQuotationPrcgElmnt(string SalesQuotation, string PricingProcedureStep, string PricingProcedureCounter, Modified\ A_SalesQuotationPrcgElmntType payload, map<string|string[]> headers) returns Response|error
Updates a header pricing element for a specific sales quotation.
Parameters
- SalesQuotation string - Sales Document
- PricingProcedureStep string - Step Number
- PricingProcedureCounter string - Condition Counter
- payload Modified\ A_SalesQuotationPrcgElmntType - New property values
patchA_SalesQuotationText
function patchA_SalesQuotationText(string SalesQuotation, string Language, string LongTextID, Modified\ A_SalesQuotationTextType payload, map<string|string[]> headers) returns Response|error
Updates a specific header text for a specific sales quotation.
Parameters
- SalesQuotation string - Sales Quotation
- Language string - Language Key
- LongTextID string - Text ID
- payload Modified\ A_SalesQuotationTextType - New property values
performBatchOperation
function performBatchOperation(Request request, map<string|string[]> headers) returns Response|error
Send a group of requests
Parameters
- request Request - Batch request
rejectApprovalRequest
function rejectApprovalRequest(map<string|string[]> headers, *RejectApprovalRequestQueries queries) returns FunctionResult_2|error
Invoke action rejectApprovalRequest
Parameters
- queries *RejectApprovalRequestQueries - Queries to be sent with the request
Return Type
- FunctionResult_2|error - Success
releaseApprovalRequest
function releaseApprovalRequest(map<string|string[]> headers, *ReleaseApprovalRequestQueries queries) returns FunctionResult_1|error
Invoke action releaseApprovalRequest
Parameters
- queries *ReleaseApprovalRequestQueries - Queries to be sent with the request
Return Type
- FunctionResult_1|error - Success
Records
sap.s4hana.api_sales_quotation_srv: A_SalesQuotation
Fields
- SalesQuotation string? -
- SalesQuotationType string? -
- SalesOrganization string? -
- DistributionChannel string? -
- OrganizationDivision string? -
- SalesGroup string? -
- SalesOffice string? -
- SalesDistrict string? -
- SoldToParty string? -
- CreationDate string? - Record Creation Date
- CreatedByUser string? - Name of Person Responsible for Creating the Object
- LastChangeDate string? - Last Changed On
- LastChangeDateTime string? - UTC Time Stamp in Long Form (YYYYMMDDhhmmssmmmuuun)
- PurchaseOrderByCustomer string? -
- CustomerPurchaseOrderType string? - Customer Purchase Order Type
- CustomerPurchaseOrderDate string? -
- SalesQuotationDate string? - Document Date (Date Received/Sent)
- TotalNetAmount string? - Net Value of the Sales Document in Document Currency
- TransactionCurrency string? - SD Document Currency
- SDDocumentReason string? - Order Reason (Reason for the Business Transaction)
- PricingDate string? - Date for Pricing and Exchange Rate
- RequestedDeliveryDate string? -
- ShippingCondition string? -
- CompleteDeliveryIsDefined boolean? - Complete Delivery Defined for Each Sales Order
- ShippingType string? -
- HeaderBillingBlockReason string? - Billing Block in SD Document
- DeliveryBlockReason string? - Delivery Block (Document Header)
- BindingPeriodValidityStartDate string? - Quotation/Inquiry is Valid From
- BindingPeriodValidityEndDate string? - Date Until Which Bid/Quotation is Binding (Valid-To Date)
- HdrOrderProbabilityInPercent string? - Sales probability
- ExpectedOrderNetAmount string? - Expected Order Net Amount
- IncotermsClassification string? - Incoterms (Part 1)
- IncotermsTransferLocation string? -
- IncotermsLocation1 string? -
- IncotermsLocation2 string? -
- IncotermsVersion string? -
- CustomerPaymentTerms string? - Key for Terms of Payment
- CustomerPriceGroup string? -
- PriceListType string? -
- PaymentMethod string? -
- CustomerTaxClassification1 string? - Alternative Tax Classification
- CustomerTaxClassification2 string? - Tax Classification 2 for Customer
- CustomerTaxClassification3 string? - Tax Classification 3 for Customer
- CustomerTaxClassification4 string? - Tax Classification 4 for Customer
- CustomerTaxClassification5 string? - Tax Classification 5 for Customer
- CustomerTaxClassification6 string? - Tax Classification 6 for Customer
- CustomerTaxClassification7 string? - Tax Classification 7 for Customer
- CustomerTaxClassification8 string? - Tax Classification 8 for Customer
- CustomerTaxClassification9 string? - Tax Classification 9 for Customer
- ReferenceSDDocument string? - Document Number of Reference Document
- ReferenceSDDocumentCategory string? - Document Category of Preceding SD Document
- SalesQuotationApprovalReason string? - Approval Request Reason ID
- SalesDocApprovalStatus string? - Document Approval Status
- OverallSDProcessStatus string? - Overall Processing Status (Header/All Items)
- TotalCreditCheckStatus string? - Overall Status of Credit Checks
- OverallSDDocumentRejectionSts string? - Rejection Status (All Items)
- to_Item A_SalesQuotation_to_Item? -
- to_Partner A_SalesQuotation_to_Partner? -
- to_PrecedingProcFlowDoc A_SalesQuotation_to_PrecedingProcFlowDoc? -
- to_PricingElement A_SalesQuotation_to_PricingElement? -
- to_RelatedObject A_SalesQuotation_to_RelatedObject? -
- to_SubsequentProcFlowDoc A_SalesQuotation_to_SubsequentProcFlowDoc? -
- to_Text A_SalesQuotation_to_Text? -
sap.s4hana.api_sales_quotation_srv: A_SalesQuotation_to_Item
Fields
- results A_SalesQuotationItem[]? -
sap.s4hana.api_sales_quotation_srv: A_SalesQuotation_to_Partner
Fields
- results A_SalesQuotationPartner[]? -
sap.s4hana.api_sales_quotation_srv: A_SalesQuotation_to_PrecedingProcFlowDoc
Fields
- results A_SlsQtanPrecdgProcFlow[]? -
sap.s4hana.api_sales_quotation_srv: A_SalesQuotation_to_PricingElement
Fields
- results A_SalesQuotationPrcgElmnt[]? -
sap.s4hana.api_sales_quotation_srv: A_SalesQuotation_to_RelatedObject
Fields
- results A_SalesQuotationRelatedObject[]? -
sap.s4hana.api_sales_quotation_srv: A_SalesQuotation_to_SubsequentProcFlowDoc
Fields
- results A_SlsQtanSubsqntProcFlow[]? -
sap.s4hana.api_sales_quotation_srv: A_SalesQuotation_to_Text
Fields
- results A_SalesQuotationText[]? -
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationItem
Fields
- SalesQuotation string? -
- SalesQuotationItem string? -
- HigherLevelItem string? - Higher-Level Item in Bill of Material Structures
- SalesQuotationItemCategory string? - Sales Document Item Category
- SalesQuotationItemText string? - Short Text for Sales Order Item
- PurchaseOrderByCustomer string? -
- Material string? - Material Number
- MaterialByCustomer string? - Material Number Used by Customer
- PricingReferenceMaterial string? - Pricing Reference Material
- RequestedQuantity string? -
- RequestedQuantityUnit string? - Unit of the Requested Quantity
- RequestedQuantitySAPUnit string? - SAP Unit Code for Requested Quantity
- RequestedQuantityISOUnit string? - ISO Unit Code for Requested Quantity
- ItemOrderProbabilityInPercent string? - Order Probability of the Item
- AlternativeToItem string? - Item for Which this Item is an Alternative
- ItemGrossWeight string? - Gross Weight of the Item
- ItemNetWeight string? - Net Weight of the Item
- ItemWeightUnit string? -
- ItemWeightSAPUnit string? - SAP Unit Code for Item Weight
- ItemWeightISOUnit string? - ISO Unit Code for Item Weight
- ItemVolume string? - Volume of the item
- ItemVolumeUnit string? -
- ItemVolumeSAPUnit string? - SAP Unit Code for Item Volume
- ItemVolumeISOUnit string? - ISO Unit Code for Item Volume
- TransactionCurrency string? - SD Document Currency
- NetAmount string? - Net Value of the Document Item in Document Currency
- MaterialGroup string? -
- MaterialPricingGroup string? -
- Batch string? - Batch Number
- Plant string? - Plant (Own or External)
- IncotermsClassification string? - Incoterms (Part 1)
- IncotermsTransferLocation string? -
- IncotermsLocation1 string? -
- IncotermsLocation2 string? -
- CustomerPaymentTerms string? - Key for Terms of Payment
- ProductTaxClassification1 string? - Tax Classification for Material
- ProductTaxClassification2 string? - Tax Classification for Material
- ProductTaxClassification3 string? - Tax Classification for Material
- ProductTaxClassification4 string? - Tax Classification for Material
- ProductTaxClassification5 string? - Tax Classification for Material
- ProductTaxClassification6 string? - Tax Classification for Material
- ProductTaxClassification7 string? - Tax Classification for Material
- ProductTaxClassification8 string? - Tax Classification for Material
- ProductTaxClassification9 string? - Tax Classification for Material
- SalesDocumentRjcnReason string? - Reason for Rejection of Sales Documents
- WBSElement string? - Work Breakdown Structure Element (WBS Element)
- ProfitCenter string? -
- ReferenceSDDocument string? - Document Number of Reference Document
- ReferenceSDDocumentItem string? - Item Number of the Reference Item
- SDProcessStatus string? - Overall Processing Status (Item)
- Subtotal1Amount string? - Subtotal 1 from Pricing Procedure for Price Element
- Subtotal2Amount string? - Subtotal 2 from Pricing Procedure for Price Element
- Subtotal3Amount string? - Subtotal 3 from Pricing Procedure for Price Element
- Subtotal4Amount string? - Subtotal 4 from Pricing Procedure for Price Element
- Subtotal5Amount string? - Subtotal 5 from Pricing Procedure for Price Element
- Subtotal6Amount string? - Subtotal 6 from Pricing Procedure for Price Element
- to_Partner A_SalesQuotationItem_to_Partner? -
- to_PrecedingProcFlowDocItem A_SalesQuotationItem_to_PrecedingProcFlowDocItem? -
- to_PricingElement A_SalesQuotationItem_to_PricingElement? -
- to_RelatedObject A_SalesQuotationItem_to_RelatedObject? -
- to_SalesQuotation A_SalesQuotation? -
- to_SubsequentProcFlowDocItem A_SalesQuotationItem_to_SubsequentProcFlowDocItem? -
- to_Text A_SalesQuotationItem_to_Text? -
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationItem_to_Partner
Fields
- results A_SalesQuotationItemPartner[]? -
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationItem_to_PrecedingProcFlowDocItem
Fields
- results A_SlsQtanItmPrecdgProcFlow[]? -
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationItem_to_PricingElement
Fields
- results A_SalesQuotationItemPrcgElmnt[]? -
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationItem_to_RelatedObject
Fields
- results A_SlsQtanItemRelatedObject[]? -
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationItem_to_SubsequentProcFlowDocItem
Fields
- results A_SlsQtanItmSubsqntProcFlow[]? -
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationItem_to_Text
Fields
- results A_SalesQuotationItemText[]? -
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationItemPartner
Fields
- SalesQuotation string? - Sales and Distribution Document Number
- SalesQuotationItem string? - Item number of the SD document
- PartnerFunction string? -
- PartnerFunctionInternalCode string? -
- Customer string? - Customer Number
- Supplier string? - Account Number of Supplier
- Personnel string? -
- ContactPerson string? - Number of Contact Person
- ReferenceBusinessPartner string? - Business Partner Number
- to_SalesQuotation A_SalesQuotation? -
- to_SalesQuotationItem A_SalesQuotationItem? -
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationItemPartnerWrapper
Fields
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationItemPrcgElmnt
Fields
- SalesQuotation string? -
- SalesQuotationItem string? - Condition item number
- PricingProcedureStep string? -
- PricingProcedureCounter string? - Condition Counter
- ConditionType string? -
- PricingDateTime string? - Timestamp for Pricing
- PriceConditionDeterminationDte string? - Condition Pricing Date
- ConditionCalculationType string? - Calculation Type for Condition
- ConditionBaseValue string? -
- ConditionRateValue string? - Condition Amount or Percentage
- ConditionCurrency string? - Currency Key
- ConditionQuantity string? - Condition Pricing Unit
- ConditionQuantityUnit string? - Condition Unit in the Document
- ConditionQuantitySAPUnit string? - SAP Unit Code for Condition Quantity
- ConditionQuantityISOUnit string? - ISO Unit Code for Condition Quantity
- ConditionCategory string? - Condition Category (Examples: Tax, Freight, Price, Cost)
- ConditionIsForStatistics boolean? - Condition is used for statistics
- PricingScaleType string? -
- IsRelevantForAccrual boolean? - Condition is Relevant for Accrual (e.g. Freight)
- CndnIsRelevantForInvoiceList string? - Condition for Invoice List
- ConditionOrigin string? - Origin of the Condition
- IsGroupCondition string? -
- ConditionRecord string? - Number of Condition Record
- ConditionSequentialNumber string? - Sequential Number of the Condition
- TaxCode string? - Tax on Sales/Purchases Code
- WithholdingTaxCode string? - Withholding Tax Code
- CndnRoundingOffDiffAmount string? - Rounding-Off Difference of the Condition
- ConditionAmount string? -
- TransactionCurrency string? - SD Document Currency
- ConditionControl string? -
- ConditionInactiveReason string? - Condition is Inactive
- ConditionClass string? -
- PrcgProcedureCounterForHeader string? - Condition Counter (Header)
- StructureCondition string? -
- PricingScaleBasis string? - Scale Basis Indicator
- ConditionScaleBasisValue string? - Scale Base Value
- ConditionScaleBasisUnit string? - Condition Scale Unit of Measure
- ConditionScaleBasisCurrency string? -
- CndnIsRelevantForIntcoBilling boolean? - Condition for Intercompany Billing
- ConditionIsManuallyChanged boolean? - Condition Changed Manually
- ConditionIsForConfiguration boolean? - Condition Used for Variant Configuration
- VariantCondition string? - Variant Condition Key
- to_SalesQuotation A_SalesQuotation? -
- to_SalesQuotationItem A_SalesQuotationItem? -
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationItemPrcgElmntWrapper
Fields
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationItemText
Fields
- SalesQuotation string? -
- SalesQuotationItem string? -
- Language string? -
- LongTextID string? -
- LongText string? -
- to_SalesQuotation A_SalesQuotation? -
- to_SalesQuotationItem A_SalesQuotationItem? -
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationItemTextWrapper
Fields
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationItemWrapper
Fields
- d A_SalesQuotationItem? -
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationPartner
Fields
- SalesQuotation string? - Sales and Distribution Document Number
- PartnerFunction string? -
- PartnerFunctionInternalCode string? -
- Customer string? - Customer Number
- Supplier string? - Account Number of Supplier
- Personnel string? -
- ContactPerson string? - Number of Contact Person
- ReferenceBusinessPartner string? - Business Partner Number
- to_SalesQuotation A_SalesQuotation? -
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationPartnerWrapper
Fields
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationPrcgElmnt
Fields
- SalesQuotation string? -
- PricingProcedureStep string? -
- PricingProcedureCounter string? - Condition Counter
- ConditionType string? -
- PricingDateTime string? - Timestamp for Pricing
- PriceConditionDeterminationDte string? - Condition Pricing Date
- ConditionCalculationType string? - Calculation Type for Condition
- ConditionBaseValue string? -
- ConditionRateValue string? - Condition Amount or Percentage
- ConditionCurrency string? - Currency Key
- ConditionQuantity string? - Condition Pricing Unit
- ConditionQuantityUnit string? - Condition Unit in the Document
- ConditionQuantitySAPUnit string? - SAP Unit Code for Condition Quantity
- ConditionQuantityISOUnit string? - ISO Unit Code for Condition Quantity
- ConditionCategory string? - Condition Category (Examples: Tax, Freight, Price, Cost)
- ConditionIsForStatistics boolean? - Condition is used for statistics
- PricingScaleType string? -
- ConditionOrigin string? - Origin of the Condition
- IsGroupCondition string? -
- ConditionRecord string? - Number of Condition Record
- ConditionSequentialNumber string? - Sequential Number of the Condition
- TaxCode string? - Tax on Sales/Purchases Code
- WithholdingTaxCode string? - Withholding Tax Code
- CndnRoundingOffDiffAmount string? - Rounding-Off Difference of the Condition
- ConditionAmount string? -
- TransactionCurrency string? - SD Document Currency
- ConditionControl string? -
- ConditionInactiveReason string? - Condition is Inactive
- ConditionClass string? -
- PrcgProcedureCounterForHeader string? - Condition Counter (Header)
- StructureCondition string? -
- PricingScaleBasis string? - Scale Basis Indicator
- ConditionScaleBasisValue string? - Scale Base Value
- ConditionScaleBasisUnit string? - Condition Scale Unit of Measure
- ConditionScaleBasisCurrency string? -
- CndnIsRelevantForIntcoBilling boolean? - Condition for Intercompany Billing
- ConditionIsManuallyChanged boolean? - Condition Changed Manually
- ConditionIsForConfiguration boolean? - Condition Used for Variant Configuration
- VariantCondition string? - Variant Condition Key
- to_SalesQuotation A_SalesQuotation? -
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationPrcgElmntWrapper
Fields
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationRelatedObject
Fields
- SalesQuotation string? -
- SDDocRelatedObjectSequenceNmbr string? - Sequence Number of the Related Object of an SD Document
- SDDocumentRelatedObjectType string? - Type of the Related Object of an SD Document
- SDDocRelatedObjectSystem string? - System of the Related Object of an SD Document
- SDDocRelatedObjectReference1 string? - Reference of the Related Object of an SD Document
- SDDocRelatedObjectReference2 string? - Reference of the Related Object of an SD Document
- to_SalesQuotation A_SalesQuotation? -
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationRelatedObjectWrapper
Fields
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationText
Fields
- SalesQuotation string? -
- Language string? -
- LongTextID string? -
- LongText string? -
- to_SalesQuotation A_SalesQuotation? -
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationTextWrapper
Fields
- d A_SalesQuotationText? -
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationWrapper
Fields
- d A_SalesQuotation? -
sap.s4hana.api_sales_quotation_srv: A_SlsQtanItemRelatedObject
Fields
- SalesQuotation string? -
- SalesQuotationItem string? -
- SDDocRelatedObjectSequenceNmbr string? - Sequence Number of the Related Object of an SD Document
- SDDocumentRelatedObjectType string? - Type of the Related Object of an SD Document
- SDDocRelatedObjectSystem string? - System of the Related Object of an SD Document
- SDDocRelatedObjectReference1 string? - Reference of the Related Object of an SD Document
- SDDocRelatedObjectReference2 string? - Reference of the Related Object of an SD Document
- to_SalesQuotation A_SalesQuotation? -
- to_SalesQuotationItem A_SalesQuotationItem? -
sap.s4hana.api_sales_quotation_srv: A_SlsQtanItemRelatedObjectWrapper
Fields
sap.s4hana.api_sales_quotation_srv: A_SlsQtanItmPrecdgProcFlow
Fields
- SalesQuotation string? - Subsequent Sales and Distribution Document
- SalesQuotationItem string? - Subsequent Item of an SD Document
- DocRelationshipUUID string? - SD Unique Document Relationship Identification
- PrecedingDocument string? - Preceding sales and distribution document
- PrecedingDocumentItem string? - Preceding Item of an SD Document
- PrecedingDocumentCategory string? - Document Category of Preceding SD Document
- ProcessFlowLevel string? - Level of the document flow record
- StatusCode string? - General SD status
- SDDocumentStatusDesc string? - Status Description
- CreationDate string? - Record Creation Date
- CreationTime string? - Entry time
- LastChangeDate string? - Last Changed On
- to_SalesQuotation A_SalesQuotation? -
- to_SalesQuotationItem A_SalesQuotationItem? -
sap.s4hana.api_sales_quotation_srv: A_SlsQtanItmPrecdgProcFlowWrapper
Fields
sap.s4hana.api_sales_quotation_srv: A_SlsQtanItmSubsqntProcFlow
Fields
- SalesQuotation string? - Preceding sales and distribution document
- SalesQuotationItem string? - Preceding Item of an SD Document
- DocRelationshipUUID string? - SD Unique Document Relationship Identification
- SubsequentDocument string? - Subsequent Sales and Distribution Document
- SubsequentDocumentItem string? - Subsequent Item of an SD Document
- SubsequentDocumentCategory string? - Document Category of Subsequent Document
- ProcessFlowLevel string? - Level of the document flow record
- StatusCode string? - General SD status
- SDDocumentStatusDesc string? - Status Description
- CreationDate string? - Record Creation Date
- CreationTime string? - Entry time
- LastChangeDate string? - Last Changed On
- to_SalesQuotation A_SalesQuotation? -
- to_SalesQuotationItem A_SalesQuotationItem? -
sap.s4hana.api_sales_quotation_srv: A_SlsQtanItmSubsqntProcFlowWrapper
Fields
sap.s4hana.api_sales_quotation_srv: A_SlsQtanPrecdgProcFlow
Fields
- SalesQuotation string? - Subsequent Sales and Distribution Document
- DocRelationshipUUID string? - SD Unique Document Relationship Identification
- PrecedingDocument string? - Preceding sales and distribution document
- PrecedingDocumentCategory string? - Document Category of Preceding SD Document
- ProcessFlowLevel string? - Level of the document flow record
- StatusCode string? - General SD status
- SDDocumentStatusDesc string? - Status Description
- CreationDate string? - Record Creation Date
- CreationTime string? - Entry time
- LastChangeDate string? - Last Changed On
- to_SalesQuotation A_SalesQuotation? -
sap.s4hana.api_sales_quotation_srv: A_SlsQtanPrecdgProcFlowWrapper
Fields
sap.s4hana.api_sales_quotation_srv: A_SlsQtanSubsqntProcFlow
Fields
- SalesQuotation string? - Preceding sales and distribution document
- DocRelationshipUUID string? - SD Unique Document Relationship Identification
- SubsequentDocument string? - Subsequent Sales and Distribution Document
- SubsequentDocumentCategory string? - Document Category of Subsequent Document
- ProcessFlowLevel string? - Level of the document flow record
- StatusCode string? - General SD status
- SDDocumentStatusDesc string? - Status Description
- CreationDate string? - Record Creation Date
- CreationTime string? - Entry time
- LastChangeDate string? - Last Changed On
- to_SalesQuotation A_SalesQuotation? -
sap.s4hana.api_sales_quotation_srv: A_SlsQtanSubsqntProcFlowWrapper
Fields
sap.s4hana.api_sales_quotation_srv: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
sap.s4hana.api_sales_quotation_srv: CollectionOfA_SalesQuotation
Fields
- __count count? -
- results A_SalesQuotation[]? -
sap.s4hana.api_sales_quotation_srv: CollectionOfA_SalesQuotationItem
Fields
- __count count? -
- results A_SalesQuotationItem[]? -
sap.s4hana.api_sales_quotation_srv: CollectionOfA_SalesQuotationItemPartner
Fields
- __count count? -
- results A_SalesQuotationItemPartner[]? -
sap.s4hana.api_sales_quotation_srv: CollectionOfA_SalesQuotationItemPartnerWrapper
Fields
sap.s4hana.api_sales_quotation_srv: CollectionOfA_SalesQuotationItemPrcgElmnt
Fields
- __count count? -
- results A_SalesQuotationItemPrcgElmnt[]? -
sap.s4hana.api_sales_quotation_srv: CollectionOfA_SalesQuotationItemPrcgElmntWrapper
Fields
sap.s4hana.api_sales_quotation_srv: CollectionOfA_SalesQuotationItemText
Fields
- __count count? -
- results A_SalesQuotationItemText[]? -
sap.s4hana.api_sales_quotation_srv: CollectionOfA_SalesQuotationItemTextWrapper
Fields
sap.s4hana.api_sales_quotation_srv: CollectionOfA_SalesQuotationItemWrapper
Fields
sap.s4hana.api_sales_quotation_srv: CollectionOfA_SalesQuotationPartner
Fields
- __count count? -
- results A_SalesQuotationPartner[]? -
sap.s4hana.api_sales_quotation_srv: CollectionOfA_SalesQuotationPartnerWrapper
Fields
sap.s4hana.api_sales_quotation_srv: CollectionOfA_SalesQuotationPrcgElmnt
Fields
- __count count? -
- results A_SalesQuotationPrcgElmnt[]? -
sap.s4hana.api_sales_quotation_srv: CollectionOfA_SalesQuotationPrcgElmntWrapper
Fields
sap.s4hana.api_sales_quotation_srv: CollectionOfA_SalesQuotationRelatedObject
Fields
- __count count? -
- results A_SalesQuotationRelatedObject[]? -
sap.s4hana.api_sales_quotation_srv: CollectionOfA_SalesQuotationRelatedObjectWrapper
Fields
sap.s4hana.api_sales_quotation_srv: CollectionOfA_SalesQuotationText
Fields
- __count count? -
- results A_SalesQuotationText[]? -
sap.s4hana.api_sales_quotation_srv: CollectionOfA_SalesQuotationTextWrapper
Fields
sap.s4hana.api_sales_quotation_srv: CollectionOfA_SalesQuotationWrapper
Fields
sap.s4hana.api_sales_quotation_srv: CollectionOfA_SlsQtanItemRelatedObject
Fields
- __count count? -
- results A_SlsQtanItemRelatedObject[]? -
sap.s4hana.api_sales_quotation_srv: CollectionOfA_SlsQtanItemRelatedObjectWrapper
Fields
sap.s4hana.api_sales_quotation_srv: CollectionOfA_SlsQtanItmPrecdgProcFlow
Fields
- __count count? -
- results A_SlsQtanItmPrecdgProcFlow[]? -
sap.s4hana.api_sales_quotation_srv: CollectionOfA_SlsQtanItmPrecdgProcFlowWrapper
Fields
sap.s4hana.api_sales_quotation_srv: CollectionOfA_SlsQtanItmSubsqntProcFlow
Fields
- __count count? -
- results A_SlsQtanItmSubsqntProcFlow[]? -
sap.s4hana.api_sales_quotation_srv: CollectionOfA_SlsQtanItmSubsqntProcFlowWrapper
Fields
sap.s4hana.api_sales_quotation_srv: CollectionOfA_SlsQtanPrecdgProcFlow
Fields
- __count count? -
- results A_SlsQtanPrecdgProcFlow[]? -
sap.s4hana.api_sales_quotation_srv: CollectionOfA_SlsQtanPrecdgProcFlowWrapper
Fields
sap.s4hana.api_sales_quotation_srv: CollectionOfA_SlsQtanSubsqntProcFlow
Fields
- __count count? -
- results A_SlsQtanSubsqntProcFlow[]? -
sap.s4hana.api_sales_quotation_srv: CollectionOfA_SlsQtanSubsqntProcFlowWrapper
Fields
sap.s4hana.api_sales_quotation_srv: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig|CredentialsConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
sap.s4hana.api_sales_quotation_srv: CreateA_SalesQuotation
Fields
- SalesQuotation string -
- SalesQuotationType string? -
- SalesOrganization string? -
- DistributionChannel string? -
- OrganizationDivision string? -
- SalesGroup string? -
- SalesOffice string? -
- SalesDistrict string? -
- SoldToParty string? -
- PurchaseOrderByCustomer string? -
- CustomerPurchaseOrderType string? - Customer Purchase Order Type
- CustomerPurchaseOrderDate string? -
- SalesQuotationDate string? - Document Date (Date Received/Sent)
- TransactionCurrency string? - SD Document Currency
- SDDocumentReason string? - Order Reason (Reason for the Business Transaction)
- PricingDate string? - Date for Pricing and Exchange Rate
- RequestedDeliveryDate string? -
- ShippingCondition string? -
- CompleteDeliveryIsDefined boolean? - Complete Delivery Defined for Each Sales Order
- ShippingType string? -
- HeaderBillingBlockReason string? - Billing Block in SD Document
- DeliveryBlockReason string? - Delivery Block (Document Header)
- BindingPeriodValidityStartDate string? - Quotation/Inquiry is Valid From
- BindingPeriodValidityEndDate string? - Date Until Which Bid/Quotation is Binding (Valid-To Date)
- IncotermsClassification string? - Incoterms (Part 1)
- IncotermsTransferLocation string? -
- IncotermsLocation1 string? -
- IncotermsLocation2 string? -
- IncotermsVersion string? -
- CustomerPaymentTerms string? - Key for Terms of Payment
- CustomerPriceGroup string? -
- PriceListType string? -
- PaymentMethod string? -
- CustomerTaxClassification1 string? - Alternative Tax Classification
- CustomerTaxClassification2 string? - Tax Classification 2 for Customer
- CustomerTaxClassification3 string? - Tax Classification 3 for Customer
- CustomerTaxClassification4 string? - Tax Classification 4 for Customer
- CustomerTaxClassification5 string? - Tax Classification 5 for Customer
- CustomerTaxClassification6 string? - Tax Classification 6 for Customer
- CustomerTaxClassification7 string? - Tax Classification 7 for Customer
- CustomerTaxClassification8 string? - Tax Classification 8 for Customer
- CustomerTaxClassification9 string? - Tax Classification 9 for Customer
- ReferenceSDDocument string? - Document Number of Reference Document
- to_Item CreateA_SalesQuotation_to_Item? -
- to_Partner CreateA_SalesQuotation_to_Partner? -
- to_PrecedingProcFlowDoc CreateA_SalesQuotation_to_PrecedingProcFlowDoc? -
- to_PricingElement CreateA_SalesQuotation_to_PricingElement? -
- to_RelatedObject CreateA_SalesQuotation_to_RelatedObject? -
- to_SubsequentProcFlowDoc CreateA_SalesQuotation_to_SubsequentProcFlowDoc? -
- to_Text CreateA_SalesQuotation_to_Text? -
sap.s4hana.api_sales_quotation_srv: CreateA_SalesQuotation_to_Item
Fields
- results CreateA_SalesQuotationItem[]? -
sap.s4hana.api_sales_quotation_srv: CreateA_SalesQuotation_to_Partner
Fields
- results CreateA_SalesQuotationPartner[]? -
sap.s4hana.api_sales_quotation_srv: CreateA_SalesQuotation_to_PrecedingProcFlowDoc
Fields
- results CreateA_SlsQtanPrecdgProcFlow[]? -
sap.s4hana.api_sales_quotation_srv: CreateA_SalesQuotation_to_PricingElement
Fields
- results CreateA_SalesQuotationPrcgElmnt[]? -
sap.s4hana.api_sales_quotation_srv: CreateA_SalesQuotation_to_RelatedObject
Fields
- results CreateA_SalesQuotationRelatedObject[]? -
sap.s4hana.api_sales_quotation_srv: CreateA_SalesQuotation_to_SubsequentProcFlowDoc
Fields
- results CreateA_SlsQtanSubsqntProcFlow[]? -
sap.s4hana.api_sales_quotation_srv: CreateA_SalesQuotation_to_Text
Fields
- results CreateA_SalesQuotationText[]? -
sap.s4hana.api_sales_quotation_srv: CreateA_SalesQuotationItem
Fields
- SalesQuotationItem string -
- HigherLevelItem string? - Higher-Level Item in Bill of Material Structures
- SalesQuotationItemCategory string? - Sales Document Item Category
- SalesQuotationItemText string? - Short Text for Sales Order Item
- PurchaseOrderByCustomer string? -
- Material string? - Material Number
- MaterialByCustomer string? - Material Number Used by Customer
- PricingReferenceMaterial string? - Pricing Reference Material
- RequestedQuantity string? -
- RequestedQuantityUnit string? - Unit of the Requested Quantity
- RequestedQuantitySAPUnit string? - SAP Unit Code for Requested Quantity
- RequestedQuantityISOUnit string? - ISO Unit Code for Requested Quantity
- ItemOrderProbabilityInPercent string? - Order Probability of the Item
- AlternativeToItem string? - Item for Which this Item is an Alternative
- ItemWeightSAPUnit string? - SAP Unit Code for Item Weight
- ItemWeightISOUnit string? - ISO Unit Code for Item Weight
- ItemVolumeSAPUnit string? - SAP Unit Code for Item Volume
- ItemVolumeISOUnit string? - ISO Unit Code for Item Volume
- MaterialGroup string? -
- MaterialPricingGroup string? -
- Plant string? - Plant (Own or External)
- IncotermsClassification string? - Incoterms (Part 1)
- IncotermsTransferLocation string? -
- IncotermsLocation1 string? -
- IncotermsLocation2 string? -
- CustomerPaymentTerms string? - Key for Terms of Payment
- ProductTaxClassification1 string? - Tax Classification for Material
- ProductTaxClassification2 string? - Tax Classification for Material
- ProductTaxClassification3 string? - Tax Classification for Material
- ProductTaxClassification4 string? - Tax Classification for Material
- ProductTaxClassification5 string? - Tax Classification for Material
- ProductTaxClassification6 string? - Tax Classification for Material
- ProductTaxClassification7 string? - Tax Classification for Material
- ProductTaxClassification8 string? - Tax Classification for Material
- ProductTaxClassification9 string? - Tax Classification for Material
- SalesDocumentRjcnReason string? - Reason for Rejection of Sales Documents
- WBSElement string? - Work Breakdown Structure Element (WBS Element)
- ProfitCenter string? -
- ReferenceSDDocument string? - Document Number of Reference Document
- ReferenceSDDocumentItem string? - Item Number of the Reference Item
- to_Partner CreateA_SalesQuotationItem_to_Partner? -
- to_PrecedingProcFlowDocItem CreateA_SalesQuotationItem_to_PrecedingProcFlowDocItem? -
- to_PricingElement CreateA_SalesQuotationItem_to_PricingElement? -
- to_RelatedObject CreateA_SalesQuotationItem_to_RelatedObject? -
- to_SalesQuotation CreateA_SalesQuotation? -
- to_SubsequentProcFlowDocItem CreateA_SalesQuotationItem_to_SubsequentProcFlowDocItem? -
- to_Text CreateA_SalesQuotationItem_to_Text? -
sap.s4hana.api_sales_quotation_srv: CreateA_SalesQuotationItem_to_Partner
Fields
- results CreateA_SalesQuotationItemPartner[]? -
sap.s4hana.api_sales_quotation_srv: CreateA_SalesQuotationItem_to_PrecedingProcFlowDocItem
Fields
- results CreateA_SlsQtanItmPrecdgProcFlow[]? -
sap.s4hana.api_sales_quotation_srv: CreateA_SalesQuotationItem_to_PricingElement
Fields
- results CreateA_SalesQuotationItemPrcgElmnt[]? -
sap.s4hana.api_sales_quotation_srv: CreateA_SalesQuotationItem_to_RelatedObject
Fields
- results CreateA_SlsQtanItemRelatedObject[]? -
sap.s4hana.api_sales_quotation_srv: CreateA_SalesQuotationItem_to_SubsequentProcFlowDocItem
Fields
- results CreateA_SlsQtanItmSubsqntProcFlow[]? -
sap.s4hana.api_sales_quotation_srv: CreateA_SalesQuotationItem_to_Text
Fields
- results CreateA_SalesQuotationItemText[]? -
sap.s4hana.api_sales_quotation_srv: CreateA_SalesQuotationItemPartner
Fields
- PartnerFunction string -
- Customer string? - Customer Number
- Supplier string? - Account Number of Supplier
- Personnel string? -
- ContactPerson string? - Number of Contact Person
- to_SalesQuotation CreateA_SalesQuotation? -
- to_SalesQuotationItem CreateA_SalesQuotationItem? -
sap.s4hana.api_sales_quotation_srv: CreateA_SalesQuotationItemPrcgElmnt
Fields
- PricingProcedureStep string -
- PricingProcedureCounter string - Condition Counter
- ConditionType string? -
- ConditionRateValue string? - Condition Amount or Percentage
- ConditionCurrency string? - Currency Key
- ConditionQuantity string? - Condition Pricing Unit
- ConditionQuantityUnit string? - Condition Unit in the Document
- ConditionQuantitySAPUnit string? - SAP Unit Code for Condition Quantity
- ConditionQuantityISOUnit string? - ISO Unit Code for Condition Quantity
- TransactionCurrency string? - SD Document Currency
- to_SalesQuotation CreateA_SalesQuotation? -
- to_SalesQuotationItem CreateA_SalesQuotationItem? -
sap.s4hana.api_sales_quotation_srv: CreateA_SalesQuotationItemText
Fields
- Language string -
- LongTextID string -
- LongText string? -
- to_SalesQuotation CreateA_SalesQuotation? -
- to_SalesQuotationItem CreateA_SalesQuotationItem? -
sap.s4hana.api_sales_quotation_srv: CreateA_SalesQuotationPartner
Fields
- PartnerFunction string -
- Customer string? - Customer Number
- Supplier string? - Account Number of Supplier
- Personnel string? -
- ContactPerson string? - Number of Contact Person
- to_SalesQuotation CreateA_SalesQuotation? -
sap.s4hana.api_sales_quotation_srv: CreateA_SalesQuotationPrcgElmnt
Fields
- PricingProcedureStep string -
- PricingProcedureCounter string - Condition Counter
- ConditionType string? -
- ConditionRateValue string? - Condition Amount or Percentage
- ConditionCurrency string? - Currency Key
- ConditionQuantity string? - Condition Pricing Unit
- ConditionQuantityUnit string? - Condition Unit in the Document
- ConditionQuantitySAPUnit string? - SAP Unit Code for Condition Quantity
- ConditionQuantityISOUnit string? - ISO Unit Code for Condition Quantity
- TransactionCurrency string? - SD Document Currency
- to_SalesQuotation CreateA_SalesQuotation? -
sap.s4hana.api_sales_quotation_srv: CreateA_SalesQuotationRelatedObject
Fields
- SDDocumentRelatedObjectType string? - Type of the Related Object of an SD Document
- SDDocRelatedObjectSystem string? - System of the Related Object of an SD Document
- SDDocRelatedObjectReference1 string? - Reference of the Related Object of an SD Document
- SDDocRelatedObjectReference2 string? - Reference of the Related Object of an SD Document
- to_SalesQuotation CreateA_SalesQuotation? -
sap.s4hana.api_sales_quotation_srv: CreateA_SalesQuotationText
Fields
- Language string -
- LongTextID string -
- LongText string? -
- to_SalesQuotation CreateA_SalesQuotation? -
sap.s4hana.api_sales_quotation_srv: CreateA_SlsQtanItemRelatedObject
Fields
- SDDocumentRelatedObjectType string? - Type of the Related Object of an SD Document
- SDDocRelatedObjectSystem string? - System of the Related Object of an SD Document
- SDDocRelatedObjectReference1 string? - Reference of the Related Object of an SD Document
- SDDocRelatedObjectReference2 string? - Reference of the Related Object of an SD Document
- to_SalesQuotation CreateA_SalesQuotation? -
- to_SalesQuotationItem CreateA_SalesQuotationItem? -
sap.s4hana.api_sales_quotation_srv: CreateA_SlsQtanItmPrecdgProcFlow
Fields
- SalesQuotation string - Subsequent Sales and Distribution Document
- SalesQuotationItem string - Subsequent Item of an SD Document
- DocRelationshipUUID string - SD Unique Document Relationship Identification
- PrecedingDocument string? - Preceding sales and distribution document
- PrecedingDocumentItem string? - Preceding Item of an SD Document
- PrecedingDocumentCategory string? - Document Category of Preceding SD Document
- ProcessFlowLevel string? - Level of the document flow record
- StatusCode string? - General SD status
- SDDocumentStatusDesc string? - Status Description
- CreationDate string? - Record Creation Date
- CreationTime string? - Entry time
- LastChangeDate string? - Last Changed On
- to_SalesQuotation CreateA_SalesQuotation? -
- to_SalesQuotationItem CreateA_SalesQuotationItem? -
sap.s4hana.api_sales_quotation_srv: CreateA_SlsQtanItmSubsqntProcFlow
Fields
- SalesQuotation string - Preceding sales and distribution document
- SalesQuotationItem string - Preceding Item of an SD Document
- DocRelationshipUUID string - SD Unique Document Relationship Identification
- SubsequentDocument string? - Subsequent Sales and Distribution Document
- SubsequentDocumentItem string? - Subsequent Item of an SD Document
- SubsequentDocumentCategory string? - Document Category of Subsequent Document
- ProcessFlowLevel string? - Level of the document flow record
- StatusCode string? - General SD status
- SDDocumentStatusDesc string? - Status Description
- CreationDate string? - Record Creation Date
- CreationTime string? - Entry time
- LastChangeDate string? - Last Changed On
- to_SalesQuotation CreateA_SalesQuotation? -
- to_SalesQuotationItem CreateA_SalesQuotationItem? -
sap.s4hana.api_sales_quotation_srv: CreateA_SlsQtanPrecdgProcFlow
Fields
- SalesQuotation string - Subsequent Sales and Distribution Document
- DocRelationshipUUID string - SD Unique Document Relationship Identification
- PrecedingDocument string? - Preceding sales and distribution document
- PrecedingDocumentCategory string? - Document Category of Preceding SD Document
- ProcessFlowLevel string? - Level of the document flow record
- StatusCode string? - General SD status
- SDDocumentStatusDesc string? - Status Description
- CreationDate string? - Record Creation Date
- CreationTime string? - Entry time
- LastChangeDate string? - Last Changed On
- to_SalesQuotation CreateA_SalesQuotation? -
sap.s4hana.api_sales_quotation_srv: CreateA_SlsQtanSubsqntProcFlow
Fields
- SalesQuotation string - Preceding sales and distribution document
- DocRelationshipUUID string - SD Unique Document Relationship Identification
- SubsequentDocument string? - Subsequent Sales and Distribution Document
- SubsequentDocumentCategory string? - Document Category of Subsequent Document
- ProcessFlowLevel string? - Level of the document flow record
- StatusCode string? - General SD status
- SDDocumentStatusDesc string? - Status Description
- CreationDate string? - Record Creation Date
- CreationTime string? - Entry time
- LastChangeDate string? - Last Changed On
- to_SalesQuotation CreateA_SalesQuotation? -
sap.s4hana.api_sales_quotation_srv: FunctionResult
Fields
- Boolean boolean? -
sap.s4hana.api_sales_quotation_srv: FunctionResult_1
Fields
- d FunctionResult_1_d? -
sap.s4hana.api_sales_quotation_srv: FunctionResult_1_d
Fields
- releaseApprovalRequest FunctionResult? -
sap.s4hana.api_sales_quotation_srv: FunctionResult_2
Fields
- d FunctionResult_2_d? -
sap.s4hana.api_sales_quotation_srv: FunctionResult_2_d
Fields
- rejectApprovalRequest FunctionResult? -
sap.s4hana.api_sales_quotation_srv: GetA_SalesQuotationItemPartnerQueries
Represents the Queries record for the operation: getA_SalesQuotationItemPartner
Fields
- \$expand A_SalesQuotationItemPartnerExpandOptions? - Expand related entities, see Expand
- \$select A_SalesQuotationItemPartnerSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: GetA_SalesQuotationItemPrcgElmntQueries
Represents the Queries record for the operation: getA_SalesQuotationItemPrcgElmnt
Fields
- \$expand A_SalesQuotationItemPrcgElmntExpandOptions? - Expand related entities, see Expand
- \$select A_SalesQuotationItemPrcgElmntSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: GetA_SalesQuotationItemQueries
Represents the Queries record for the operation: getA_SalesQuotationItem
Fields
- \$expand A_SalesQuotationItemExpandOptions? - Expand related entities, see Expand
- \$select A_SalesQuotationItemSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: GetA_SalesQuotationItemTextQueries
Represents the Queries record for the operation: getA_SalesQuotationItemText
Fields
- \$expand A_SalesQuotationItemTextExpandOptions? - Expand related entities, see Expand
- \$select A_SalesQuotationItemTextSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: GetA_SalesQuotationPartnerQueries
Represents the Queries record for the operation: getA_SalesQuotationPartner
Fields
- \$expand A_SalesQuotationPartnerExpandOptions? - Expand related entities, see Expand
- \$select A_SalesQuotationPartnerSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: GetA_SalesQuotationPrcgElmntQueries
Represents the Queries record for the operation: getA_SalesQuotationPrcgElmnt
Fields
- \$expand A_SalesQuotationPrcgElmntExpandOptions? - Expand related entities, see Expand
- \$select A_SalesQuotationPrcgElmntSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: GetA_SalesQuotationQueries
Represents the Queries record for the operation: getA_SalesQuotation
Fields
- \$expand A_SalesQuotationExpandOptions? - Expand related entities, see Expand
- \$select A_SalesQuotationSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: GetA_SalesQuotationRelatedObjectQueries
Represents the Queries record for the operation: getA_SalesQuotationRelatedObject
Fields
- \$expand A_SalesQuotationRelatedObjectExpandOptions? - Expand related entities, see Expand
- \$select A_SalesQuotationRelatedObjectSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: GetA_SalesQuotationTextQueries
Represents the Queries record for the operation: getA_SalesQuotationText
Fields
- \$expand A_SalesQuotationTextExpandOptions? - Expand related entities, see Expand
- \$select A_SalesQuotationTextSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: GetA_SlsQtanItemRelatedObjectQueries
Represents the Queries record for the operation: getA_SlsQtanItemRelatedObject
Fields
- \$expand A_SlsQtanItemRelatedObjectExpandOptions? - Expand related entities, see Expand
- \$select A_SlsQtanItemRelatedObjectSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: GetA_SlsQtanItmPrecdgProcFlowQueries
Represents the Queries record for the operation: getA_SlsQtanItmPrecdgProcFlow
Fields
- \$expand A_SlsQtanItmPrecdgProcFlowExpandOptions? - Expand related entities, see Expand
- \$select A_SlsQtanItmPrecdgProcFlowSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: GetA_SlsQtanItmSubsqntProcFlowQueries
Represents the Queries record for the operation: getA_SlsQtanItmSubsqntProcFlow
Fields
- \$expand A_SlsQtanItmSubsqntProcFlowExpandOptions? - Expand related entities, see Expand
- \$select A_SlsQtanItmSubsqntProcFlowSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: GetA_SlsQtanPrecdgProcFlowQueries
Represents the Queries record for the operation: getA_SlsQtanPrecdgProcFlow
Fields
- \$expand A_SlsQtanPrecdgProcFlowExpandOptions? - Expand related entities, see Expand
- \$select A_SlsQtanPrecdgProcFlowSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: GetA_SlsQtanSubsqntProcFlowQueries
Represents the Queries record for the operation: getA_SlsQtanSubsqntProcFlow
Fields
- \$expand A_SlsQtanSubsqntProcFlowExpandOptions? - Expand related entities, see Expand
- \$select A_SlsQtanSubsqntProcFlowSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: GetSalesQuotationItemOfA_SalesQuotationItemPartnerQueries
Represents the Queries record for the operation: getSalesQuotationItemOfA_SalesQuotationItemPartner
Fields
- \$expand SalesQuotationItemOfA_SalesQuotationItemPartnerExpandOptions? - Expand related entities, see Expand
- \$select SalesQuotationItemOfA_SalesQuotationItemPartnerSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: GetSalesQuotationItemOfA_SalesQuotationItemPrcgElmntQueries
Represents the Queries record for the operation: getSalesQuotationItemOfA_SalesQuotationItemPrcgElmnt
Fields
- \$expand SalesQuotationItemOfA_SalesQuotationItemPrcgElmntExpandOptions? - Expand related entities, see Expand
- \$select SalesQuotationItemOfA_SalesQuotationItemPrcgElmntSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: GetSalesQuotationItemOfA_SalesQuotationItemTextQueries
Represents the Queries record for the operation: getSalesQuotationItemOfA_SalesQuotationItemText
Fields
- \$expand SalesQuotationItemOfA_SalesQuotationItemTextExpandOptions? - Expand related entities, see Expand
- \$select SalesQuotationItemOfA_SalesQuotationItemTextSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: GetSalesQuotationItemOfA_SlsQtanItemRelatedObjectQueries
Represents the Queries record for the operation: getSalesQuotationItemOfA_SlsQtanItemRelatedObject
Fields
- \$expand SalesQuotationItemOfA_SlsQtanItemRelatedObjectExpandOptions? - Expand related entities, see Expand
- \$select SalesQuotationItemOfA_SlsQtanItemRelatedObjectSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: GetSalesQuotationItemOfA_SlsQtanItmPrecdgProcFlowQueries
Represents the Queries record for the operation: getSalesQuotationItemOfA_SlsQtanItmPrecdgProcFlow
Fields
- \$expand SalesQuotationItemOfA_SlsQtanItmPrecdgProcFlowExpandOptions? - Expand related entities, see Expand
- \$select SalesQuotationItemOfA_SlsQtanItmPrecdgProcFlowSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: GetSalesQuotationItemOfA_SlsQtanItmSubsqntProcFlowQueries
Represents the Queries record for the operation: getSalesQuotationItemOfA_SlsQtanItmSubsqntProcFlow
Fields
- \$expand SalesQuotationItemOfA_SlsQtanItmSubsqntProcFlowExpandOptions? - Expand related entities, see Expand
- \$select SalesQuotationItemOfA_SlsQtanItmSubsqntProcFlowSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: GetSalesQuotationOfA_SalesQuotationItemPartnerQueries
Represents the Queries record for the operation: getSalesQuotationOfA_SalesQuotationItemPartner
Fields
- \$expand SalesQuotationOfA_SalesQuotationItemPartnerExpandOptions? - Expand related entities, see Expand
- \$select SalesQuotationOfA_SalesQuotationItemPartnerSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: GetSalesQuotationOfA_SalesQuotationItemPrcgElmntQueries
Represents the Queries record for the operation: getSalesQuotationOfA_SalesQuotationItemPrcgElmnt
Fields
- \$expand SalesQuotationOfA_SalesQuotationItemPrcgElmntExpandOptions? - Expand related entities, see Expand
- \$select SalesQuotationOfA_SalesQuotationItemPrcgElmntSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: GetSalesQuotationOfA_SalesQuotationItemQueries
Represents the Queries record for the operation: getSalesQuotationOfA_SalesQuotationItem
Fields
- \$expand SalesQuotationOfA_SalesQuotationItemExpandOptions? - Expand related entities, see Expand
- \$select SalesQuotationOfA_SalesQuotationItemSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: GetSalesQuotationOfA_SalesQuotationItemTextQueries
Represents the Queries record for the operation: getSalesQuotationOfA_SalesQuotationItemText
Fields
- \$expand SalesQuotationOfA_SalesQuotationItemTextExpandOptions? - Expand related entities, see Expand
- \$select SalesQuotationOfA_SalesQuotationItemTextSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: GetSalesQuotationOfA_SalesQuotationPartnerQueries
Represents the Queries record for the operation: getSalesQuotationOfA_SalesQuotationPartner
Fields
- \$expand SalesQuotationOfA_SalesQuotationPartnerExpandOptions? - Expand related entities, see Expand
- \$select SalesQuotationOfA_SalesQuotationPartnerSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: GetSalesQuotationOfA_SalesQuotationPrcgElmntQueries
Represents the Queries record for the operation: getSalesQuotationOfA_SalesQuotationPrcgElmnt
Fields
- \$expand SalesQuotationOfA_SalesQuotationPrcgElmntExpandOptions? - Expand related entities, see Expand
- \$select SalesQuotationOfA_SalesQuotationPrcgElmntSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: GetSalesQuotationOfA_SalesQuotationRelatedObjectQueries
Represents the Queries record for the operation: getSalesQuotationOfA_SalesQuotationRelatedObject
Fields
- \$expand SalesQuotationOfA_SalesQuotationRelatedObjectExpandOptions? - Expand related entities, see Expand
- \$select SalesQuotationOfA_SalesQuotationRelatedObjectSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: GetSalesQuotationOfA_SalesQuotationTextQueries
Represents the Queries record for the operation: getSalesQuotationOfA_SalesQuotationText
Fields
- \$expand SalesQuotationOfA_SalesQuotationTextExpandOptions? - Expand related entities, see Expand
- \$select SalesQuotationOfA_SalesQuotationTextSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: GetSalesQuotationOfA_SlsQtanItemRelatedObjectQueries
Represents the Queries record for the operation: getSalesQuotationOfA_SlsQtanItemRelatedObject
Fields
- \$expand SalesQuotationOfA_SlsQtanItemRelatedObjectExpandOptions? - Expand related entities, see Expand
- \$select SalesQuotationOfA_SlsQtanItemRelatedObjectSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: GetSalesQuotationOfA_SlsQtanItmPrecdgProcFlowQueries
Represents the Queries record for the operation: getSalesQuotationOfA_SlsQtanItmPrecdgProcFlow
Fields
- \$expand SalesQuotationOfA_SlsQtanItmPrecdgProcFlowExpandOptions? - Expand related entities, see Expand
- \$select SalesQuotationOfA_SlsQtanItmPrecdgProcFlowSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: GetSalesQuotationOfA_SlsQtanItmSubsqntProcFlowQueries
Represents the Queries record for the operation: getSalesQuotationOfA_SlsQtanItmSubsqntProcFlow
Fields
- \$expand SalesQuotationOfA_SlsQtanItmSubsqntProcFlowExpandOptions? - Expand related entities, see Expand
- \$select SalesQuotationOfA_SlsQtanItmSubsqntProcFlowSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: GetSalesQuotationOfA_SlsQtanPrecdgProcFlowQueries
Represents the Queries record for the operation: getSalesQuotationOfA_SlsQtanPrecdgProcFlow
Fields
- \$expand SalesQuotationOfA_SlsQtanPrecdgProcFlowExpandOptions? - Expand related entities, see Expand
- \$select SalesQuotationOfA_SlsQtanPrecdgProcFlowSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: GetSalesQuotationOfA_SlsQtanSubsqntProcFlowQueries
Represents the Queries record for the operation: getSalesQuotationOfA_SlsQtanSubsqntProcFlow
Fields
- \$expand SalesQuotationOfA_SlsQtanSubsqntProcFlowExpandOptions? - Expand related entities, see Expand
- \$select SalesQuotationOfA_SlsQtanSubsqntProcFlowSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: ListA_SalesQuotationItemPartnersQueries
Represents the Queries record for the operation: listA_SalesQuotationItemPartners
Fields
- \$skip int? - Skip the first n items, see Paging - Skip
- \$top int? - Show only the first n items, see Paging - Top
- \$orderby A_SalesQuotationItemPartnerOrderByOptions? - Order items by property values, see Sorting
- \$expand A_SalesQuotationItemPartnerExpandOptions? - Expand related entities, see Expand
- \$inlinecount "allpages"|"none" ? - Include count of items, see Inlinecount
- \$select A_SalesQuotationItemPartnerSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: ListA_SalesQuotationItemPrcgElmntsQueries
Represents the Queries record for the operation: listA_SalesQuotationItemPrcgElmnts
Fields
- \$skip int? - Skip the first n items, see Paging - Skip
- \$top int? - Show only the first n items, see Paging - Top
- \$orderby A_SalesQuotationItemPrcgElmntOrderByOptions? - Order items by property values, see Sorting
- \$expand A_SalesQuotationItemPrcgElmntExpandOptions? - Expand related entities, see Expand
- \$inlinecount "allpages"|"none" ? - Include count of items, see Inlinecount
- \$select A_SalesQuotationItemPrcgElmntSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: ListA_SalesQuotationItemsQueries
Represents the Queries record for the operation: listA_SalesQuotationItems
Fields
- \$skip int? - Skip the first n items, see Paging - Skip
- \$top int? - Show only the first n items, see Paging - Top
- \$orderby A_SalesQuotationItemOrderByOptions? - Order items by property values, see Sorting
- \$expand A_SalesQuotationItemExpandOptions? - Expand related entities, see Expand
- \$inlinecount "allpages"|"none" ? - Include count of items, see Inlinecount
- \$select A_SalesQuotationItemSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: ListA_SalesQuotationItemTextsQueries
Represents the Queries record for the operation: listA_SalesQuotationItemTexts
Fields
- \$skip int? - Skip the first n items, see Paging - Skip
- \$top int? - Show only the first n items, see Paging - Top
- \$orderby A_SalesQuotationItemTextOrderByOptions? - Order items by property values, see Sorting
- \$expand A_SalesQuotationItemTextExpandOptions? - Expand related entities, see Expand
- \$inlinecount "allpages"|"none" ? - Include count of items, see Inlinecount
- \$select A_SalesQuotationItemTextSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: ListA_SalesQuotationPartnersQueries
Represents the Queries record for the operation: listA_SalesQuotationPartners
Fields
- \$skip int? - Skip the first n items, see Paging - Skip
- \$top int? - Show only the first n items, see Paging - Top
- \$orderby A_SalesQuotationPartnerOrderByOptions? - Order items by property values, see Sorting
- \$expand A_SalesQuotationPartnerExpandOptions? - Expand related entities, see Expand
- \$inlinecount "allpages"|"none" ? - Include count of items, see Inlinecount
- \$select A_SalesQuotationPartnerSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: ListA_SalesQuotationPrcgElmntsQueries
Represents the Queries record for the operation: listA_SalesQuotationPrcgElmnts
Fields
- \$skip int? - Skip the first n items, see Paging - Skip
- \$top int? - Show only the first n items, see Paging - Top
- \$orderby A_SalesQuotationPrcgElmntOrderByOptions? - Order items by property values, see Sorting
- \$expand A_SalesQuotationPrcgElmntExpandOptions? - Expand related entities, see Expand
- \$inlinecount "allpages"|"none" ? - Include count of items, see Inlinecount
- \$select A_SalesQuotationPrcgElmntSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: ListA_SalesQuotationRelatedObjectsQueries
Represents the Queries record for the operation: listA_SalesQuotationRelatedObjects
Fields
- \$skip int? - Skip the first n items, see Paging - Skip
- \$top int? - Show only the first n items, see Paging - Top
- \$orderby A_SalesQuotationRelatedObjectOrderByOptions? - Order items by property values, see Sorting
- \$expand A_SalesQuotationRelatedObjectExpandOptions? - Expand related entities, see Expand
- \$inlinecount "allpages"|"none" ? - Include count of items, see Inlinecount
- \$select A_SalesQuotationRelatedObjectSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: ListA_SalesQuotationsQueries
Represents the Queries record for the operation: listA_SalesQuotations
Fields
- \$skip int? - Skip the first n items, see Paging - Skip
- \$top int? - Show only the first n items, see Paging - Top
- \$orderby A_SalesQuotationOrderByOptions? - Order items by property values, see Sorting
- \$expand A_SalesQuotationExpandOptions? - Expand related entities, see Expand
- \$inlinecount "allpages"|"none" ? - Include count of items, see Inlinecount
- \$select A_SalesQuotationSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: ListA_SalesQuotationTextsQueries
Represents the Queries record for the operation: listA_SalesQuotationTexts
Fields
- \$skip int? - Skip the first n items, see Paging - Skip
- \$top int? - Show only the first n items, see Paging - Top
- \$orderby A_SalesQuotationTextOrderByOptions? - Order items by property values, see Sorting
- \$expand A_SalesQuotationTextExpandOptions? - Expand related entities, see Expand
- \$inlinecount "allpages"|"none" ? - Include count of items, see Inlinecount
- \$select A_SalesQuotationTextSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: ListA_SlsQtanItemRelatedObjectsQueries
Represents the Queries record for the operation: listA_SlsQtanItemRelatedObjects
Fields
- \$skip int? - Skip the first n items, see Paging - Skip
- \$top int? - Show only the first n items, see Paging - Top
- \$orderby A_SlsQtanItemRelatedObjectOrderByOptions? - Order items by property values, see Sorting
- \$expand A_SlsQtanItemRelatedObjectExpandOptions? - Expand related entities, see Expand
- \$inlinecount "allpages"|"none" ? - Include count of items, see Inlinecount
- \$select A_SlsQtanItemRelatedObjectSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: ListA_SlsQtanItmPrecdgProcFlowsQueries
Represents the Queries record for the operation: listA_SlsQtanItmPrecdgProcFlows
Fields
- \$skip int? - Skip the first n items, see Paging - Skip
- \$top int? - Show only the first n items, see Paging - Top
- \$orderby A_SlsQtanItmPrecdgProcFlowOrderByOptions? - Order items by property values, see Sorting
- \$expand A_SlsQtanItmPrecdgProcFlowExpandOptions? - Expand related entities, see Expand
- \$inlinecount "allpages"|"none" ? - Include count of items, see Inlinecount
- \$select A_SlsQtanItmPrecdgProcFlowSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: ListA_SlsQtanItmSubsqntProcFlowsQueries
Represents the Queries record for the operation: listA_SlsQtanItmSubsqntProcFlows
Fields
- \$skip int? - Skip the first n items, see Paging - Skip
- \$top int? - Show only the first n items, see Paging - Top
- \$orderby A_SlsQtanItmSubsqntProcFlowOrderByOptions? - Order items by property values, see Sorting
- \$expand A_SlsQtanItmSubsqntProcFlowExpandOptions? - Expand related entities, see Expand
- \$inlinecount "allpages"|"none" ? - Include count of items, see Inlinecount
- \$select A_SlsQtanItmSubsqntProcFlowSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: ListA_SlsQtanPrecdgProcFlowsQueries
Represents the Queries record for the operation: listA_SlsQtanPrecdgProcFlows
Fields
- \$skip int? - Skip the first n items, see Paging - Skip
- \$top int? - Show only the first n items, see Paging - Top
- \$orderby A_SlsQtanPrecdgProcFlowOrderByOptions? - Order items by property values, see Sorting
- \$expand A_SlsQtanPrecdgProcFlowExpandOptions? - Expand related entities, see Expand
- \$inlinecount "allpages"|"none" ? - Include count of items, see Inlinecount
- \$select A_SlsQtanPrecdgProcFlowSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: ListA_SlsQtanSubsqntProcFlowsQueries
Represents the Queries record for the operation: listA_SlsQtanSubsqntProcFlows
Fields
- \$skip int? - Skip the first n items, see Paging - Skip
- \$top int? - Show only the first n items, see Paging - Top
- \$orderby A_SlsQtanSubsqntProcFlowOrderByOptions? - Order items by property values, see Sorting
- \$expand A_SlsQtanSubsqntProcFlowExpandOptions? - Expand related entities, see Expand
- \$inlinecount "allpages"|"none" ? - Include count of items, see Inlinecount
- \$select A_SlsQtanSubsqntProcFlowSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: ListItemsOfA_SalesQuotationQueries
Represents the Queries record for the operation: listItemsOfA_SalesQuotation
Fields
- \$skip int? - Skip the first n items, see Paging - Skip
- \$top int? - Show only the first n items, see Paging - Top
- \$orderby A_SalesQuotationItemOrderByOptions? - Order items by property values, see Sorting
- \$expand A_SalesQuotationItemExpandOptions? - Expand related entities, see Expand
- \$inlinecount "allpages"|"none" ? - Include count of items, see Inlinecount
- \$select A_SalesQuotationItemSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: ListPartnersOfA_SalesQuotationItemQueries
Represents the Queries record for the operation: listPartnersOfA_SalesQuotationItem
Fields
- \$skip int? - Skip the first n items, see Paging - Skip
- \$top int? - Show only the first n items, see Paging - Top
- \$orderby A_SalesQuotationItemPartnerOrderByOptions? - Order items by property values, see Sorting
- \$expand A_SalesQuotationItemPartnerExpandOptions? - Expand related entities, see Expand
- \$inlinecount "allpages"|"none" ? - Include count of items, see Inlinecount
- \$select A_SalesQuotationItemPartnerSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: ListPartnersOfA_SalesQuotationQueries
Represents the Queries record for the operation: listPartnersOfA_SalesQuotation
Fields
- \$skip int? - Skip the first n items, see Paging - Skip
- \$top int? - Show only the first n items, see Paging - Top
- \$orderby A_SalesQuotationPartnerOrderByOptions? - Order items by property values, see Sorting
- \$expand A_SalesQuotationPartnerExpandOptions? - Expand related entities, see Expand
- \$inlinecount "allpages"|"none" ? - Include count of items, see Inlinecount
- \$select A_SalesQuotationPartnerSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: ListPrecedingProcFlowDocItemsOfA_SalesQuotationItemQueries
Represents the Queries record for the operation: listPrecedingProcFlowDocItemsOfA_SalesQuotationItem
Fields
- \$skip int? - Skip the first n items, see Paging - Skip
- \$top int? - Show only the first n items, see Paging - Top
- \$orderby PrecedingProcFlowDocItemOfA_SalesQuotationItemOrderByOptions? - Order items by property values, see Sorting
- \$expand PrecedingProcFlowDocItemOfA_SalesQuotationItemExpandOptions? - Expand related entities, see Expand
- \$inlinecount "allpages"|"none" ? - Include count of items, see Inlinecount
- \$select PrecedingProcFlowDocItemOfA_SalesQuotationItemSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: ListPrecedingProcFlowDocsOfA_SalesQuotationQueries
Represents the Queries record for the operation: listPrecedingProcFlowDocsOfA_SalesQuotation
Fields
- \$skip int? - Skip the first n items, see Paging - Skip
- \$top int? - Show only the first n items, see Paging - Top
- \$orderby PrecedingProcFlowDocOfA_SalesQuotationOrderByOptions? - Order items by property values, see Sorting
- \$expand PrecedingProcFlowDocOfA_SalesQuotationExpandOptions? - Expand related entities, see Expand
- \$inlinecount "allpages"|"none" ? - Include count of items, see Inlinecount
- \$select PrecedingProcFlowDocOfA_SalesQuotationSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: ListPricingElementsOfA_SalesQuotationItemQueries
Represents the Queries record for the operation: listPricingElementsOfA_SalesQuotationItem
Fields
- \$skip int? - Skip the first n items, see Paging - Skip
- \$top int? - Show only the first n items, see Paging - Top
- \$orderby PricingElementOfA_SalesQuotationItemOrderByOptions? - Order items by property values, see Sorting
- \$expand PricingElementOfA_SalesQuotationItemExpandOptions? - Expand related entities, see Expand
- \$inlinecount "allpages"|"none" ? - Include count of items, see Inlinecount
- \$select PricingElementOfA_SalesQuotationItemSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: ListPricingElementsOfA_SalesQuotationQueries
Represents the Queries record for the operation: listPricingElementsOfA_SalesQuotation
Fields
- \$skip int? - Skip the first n items, see Paging - Skip
- \$top int? - Show only the first n items, see Paging - Top
- \$orderby PricingElementOfA_SalesQuotationOrderByOptions? - Order items by property values, see Sorting
- \$expand PricingElementOfA_SalesQuotationExpandOptions? - Expand related entities, see Expand
- \$inlinecount "allpages"|"none" ? - Include count of items, see Inlinecount
- \$select PricingElementOfA_SalesQuotationSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: ListRelatedObjectsOfA_SalesQuotationItemQueries
Represents the Queries record for the operation: listRelatedObjectsOfA_SalesQuotationItem
Fields
- \$skip int? - Skip the first n items, see Paging - Skip
- \$top int? - Show only the first n items, see Paging - Top
- \$orderby RelatedObjectOfA_SalesQuotationItemOrderByOptions? - Order items by property values, see Sorting
- \$expand RelatedObjectOfA_SalesQuotationItemExpandOptions? - Expand related entities, see Expand
- \$inlinecount "allpages"|"none" ? - Include count of items, see Inlinecount
- \$select RelatedObjectOfA_SalesQuotationItemSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: ListRelatedObjectsOfA_SalesQuotationQueries
Represents the Queries record for the operation: listRelatedObjectsOfA_SalesQuotation
Fields
- \$skip int? - Skip the first n items, see Paging - Skip
- \$top int? - Show only the first n items, see Paging - Top
- \$orderby A_SalesQuotationRelatedObjectOrderByOptions? - Order items by property values, see Sorting
- \$expand A_SalesQuotationRelatedObjectExpandOptions? - Expand related entities, see Expand
- \$inlinecount "allpages"|"none" ? - Include count of items, see Inlinecount
- \$select A_SalesQuotationRelatedObjectSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: ListSubsequentProcFlowDocItemsOfA_SalesQuotationItemQueries
Represents the Queries record for the operation: listSubsequentProcFlowDocItemsOfA_SalesQuotationItem
Fields
- \$skip int? - Skip the first n items, see Paging - Skip
- \$top int? - Show only the first n items, see Paging - Top
- \$orderby SubsequentProcFlowDocItemOfA_SalesQuotationItemOrderByOptions? - Order items by property values, see Sorting
- \$expand SubsequentProcFlowDocItemOfA_SalesQuotationItemExpandOptions? - Expand related entities, see Expand
- \$inlinecount "allpages"|"none" ? - Include count of items, see Inlinecount
- \$select SubsequentProcFlowDocItemOfA_SalesQuotationItemSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: ListSubsequentProcFlowDocsOfA_SalesQuotationQueries
Represents the Queries record for the operation: listSubsequentProcFlowDocsOfA_SalesQuotation
Fields
- \$skip int? - Skip the first n items, see Paging - Skip
- \$top int? - Show only the first n items, see Paging - Top
- \$orderby SubsequentProcFlowDocOfA_SalesQuotationOrderByOptions? - Order items by property values, see Sorting
- \$expand SubsequentProcFlowDocOfA_SalesQuotationExpandOptions? - Expand related entities, see Expand
- \$inlinecount "allpages"|"none" ? - Include count of items, see Inlinecount
- \$select SubsequentProcFlowDocOfA_SalesQuotationSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: ListTextsOfA_SalesQuotationItemQueries
Represents the Queries record for the operation: listTextsOfA_SalesQuotationItem
Fields
- \$skip int? - Skip the first n items, see Paging - Skip
- \$top int? - Show only the first n items, see Paging - Top
- \$orderby A_SalesQuotationItemTextOrderByOptions? - Order items by property values, see Sorting
- \$expand A_SalesQuotationItemTextExpandOptions? - Expand related entities, see Expand
- \$inlinecount "allpages"|"none" ? - Include count of items, see Inlinecount
- \$select A_SalesQuotationItemTextSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: ListTextsOfA_SalesQuotationQueries
Represents the Queries record for the operation: listTextsOfA_SalesQuotation
Fields
- \$skip int? - Skip the first n items, see Paging - Skip
- \$top int? - Show only the first n items, see Paging - Top
- \$orderby A_SalesQuotationTextOrderByOptions? - Order items by property values, see Sorting
- \$expand A_SalesQuotationTextExpandOptions? - Expand related entities, see Expand
- \$inlinecount "allpages"|"none" ? - Include count of items, see Inlinecount
- \$select A_SalesQuotationTextSelectOptions? - Select properties to be returned, see Select
sap.s4hana.api_sales_quotation_srv: Modified\ A_SalesQuotationItemPartnerType
Fields
sap.s4hana.api_sales_quotation_srv: Modified\ A_SalesQuotationItemPrcgElmntType
Fields
sap.s4hana.api_sales_quotation_srv: Modified\ A_SalesQuotationItemTextType
Fields
sap.s4hana.api_sales_quotation_srv: Modified\ A_SalesQuotationItemType
Fields
sap.s4hana.api_sales_quotation_srv: Modified\ A_SalesQuotationPartnerType
Fields
sap.s4hana.api_sales_quotation_srv: Modified\ A_SalesQuotationPrcgElmntType
Fields
sap.s4hana.api_sales_quotation_srv: Modified\ A_SalesQuotationTextType
Fields
sap.s4hana.api_sales_quotation_srv: Modified\ A_SalesQuotationType
Fields
sap.s4hana.api_sales_quotation_srv: OAuth2RefreshTokenGrantConfig
OAuth2 Refresh Token Grant Configs
Fields
- Fields Included from *OAuth2RefreshTokenGrantConfig
- refreshUrl string(default "https://{host}:{port}") - Refresh URL
sap.s4hana.api_sales_quotation_srv: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
sap.s4hana.api_sales_quotation_srv: RejectApprovalRequestQueries
Represents the Queries record for the operation: rejectApprovalRequest
Fields
- SalesQuotation string - Value needs to be enclosed in single quotes
sap.s4hana.api_sales_quotation_srv: ReleaseApprovalRequestQueries
Represents the Queries record for the operation: releaseApprovalRequest
Fields
- SalesQuotation string - Value needs to be enclosed in single quotes
sap.s4hana.api_sales_quotation_srv: UpdateA_SalesQuotation
Fields
- SalesQuotationType string? -
- SalesOrganization string? -
- DistributionChannel string? -
- OrganizationDivision string? -
- SalesGroup string? -
- SalesOffice string? -
- SalesDistrict string? -
- SoldToParty string? -
- PurchaseOrderByCustomer string? -
- CustomerPurchaseOrderType string? - Customer Purchase Order Type
- CustomerPurchaseOrderDate string? -
- SalesQuotationDate string? - Document Date (Date Received/Sent)
- TransactionCurrency string? - SD Document Currency
- SDDocumentReason string? - Order Reason (Reason for the Business Transaction)
- PricingDate string? - Date for Pricing and Exchange Rate
- RequestedDeliveryDate string? -
- ShippingCondition string? -
- CompleteDeliveryIsDefined boolean? - Complete Delivery Defined for Each Sales Order
- ShippingType string? -
- HeaderBillingBlockReason string? - Billing Block in SD Document
- DeliveryBlockReason string? - Delivery Block (Document Header)
- BindingPeriodValidityStartDate string? - Quotation/Inquiry is Valid From
- BindingPeriodValidityEndDate string? - Date Until Which Bid/Quotation is Binding (Valid-To Date)
- IncotermsClassification string? - Incoterms (Part 1)
- IncotermsTransferLocation string? -
- IncotermsLocation1 string? -
- IncotermsLocation2 string? -
- IncotermsVersion string? -
- CustomerPaymentTerms string? - Key for Terms of Payment
- CustomerPriceGroup string? -
- PriceListType string? -
- PaymentMethod string? -
- CustomerTaxClassification1 string? - Alternative Tax Classification
- CustomerTaxClassification2 string? - Tax Classification 2 for Customer
- CustomerTaxClassification3 string? - Tax Classification 3 for Customer
- CustomerTaxClassification4 string? - Tax Classification 4 for Customer
- CustomerTaxClassification5 string? - Tax Classification 5 for Customer
- CustomerTaxClassification6 string? - Tax Classification 6 for Customer
- CustomerTaxClassification7 string? - Tax Classification 7 for Customer
- CustomerTaxClassification8 string? - Tax Classification 8 for Customer
- CustomerTaxClassification9 string? - Tax Classification 9 for Customer
- ReferenceSDDocument string? - Document Number of Reference Document
sap.s4hana.api_sales_quotation_srv: UpdateA_SalesQuotationItem
Fields
- HigherLevelItem string? - Higher-Level Item in Bill of Material Structures
- SalesQuotationItemCategory string? - Sales Document Item Category
- SalesQuotationItemText string? - Short Text for Sales Order Item
- PurchaseOrderByCustomer string? -
- Material string? - Material Number
- MaterialByCustomer string? - Material Number Used by Customer
- PricingReferenceMaterial string? - Pricing Reference Material
- RequestedQuantity string? -
- RequestedQuantityUnit string? - Unit of the Requested Quantity
- RequestedQuantitySAPUnit string? - SAP Unit Code for Requested Quantity
- RequestedQuantityISOUnit string? - ISO Unit Code for Requested Quantity
- ItemOrderProbabilityInPercent string? - Order Probability of the Item
- AlternativeToItem string? - Item for Which this Item is an Alternative
- ItemWeightSAPUnit string? - SAP Unit Code for Item Weight
- ItemWeightISOUnit string? - ISO Unit Code for Item Weight
- ItemVolumeSAPUnit string? - SAP Unit Code for Item Volume
- ItemVolumeISOUnit string? - ISO Unit Code for Item Volume
- MaterialGroup string? -
- MaterialPricingGroup string? -
- Plant string? - Plant (Own or External)
- IncotermsClassification string? - Incoterms (Part 1)
- IncotermsTransferLocation string? -
- IncotermsLocation1 string? -
- IncotermsLocation2 string? -
- CustomerPaymentTerms string? - Key for Terms of Payment
- ProductTaxClassification1 string? - Tax Classification for Material
- ProductTaxClassification2 string? - Tax Classification for Material
- ProductTaxClassification3 string? - Tax Classification for Material
- ProductTaxClassification4 string? - Tax Classification for Material
- ProductTaxClassification5 string? - Tax Classification for Material
- ProductTaxClassification6 string? - Tax Classification for Material
- ProductTaxClassification7 string? - Tax Classification for Material
- ProductTaxClassification8 string? - Tax Classification for Material
- ProductTaxClassification9 string? - Tax Classification for Material
- SalesDocumentRjcnReason string? - Reason for Rejection of Sales Documents
- WBSElement string? - Work Breakdown Structure Element (WBS Element)
- ProfitCenter string? -
- ReferenceSDDocument string? - Document Number of Reference Document
- ReferenceSDDocumentItem string? - Item Number of the Reference Item
sap.s4hana.api_sales_quotation_srv: UpdateA_SalesQuotationItemPartner
Fields
- Customer string? - Customer Number
- Supplier string? - Account Number of Supplier
- Personnel string? -
- ContactPerson string? - Number of Contact Person
sap.s4hana.api_sales_quotation_srv: UpdateA_SalesQuotationItemPrcgElmnt
Fields
- ConditionType string? -
- ConditionRateValue string? - Condition Amount or Percentage
- ConditionCurrency string? - Currency Key
- ConditionQuantity string? - Condition Pricing Unit
- ConditionQuantityUnit string? - Condition Unit in the Document
- ConditionQuantitySAPUnit string? - SAP Unit Code for Condition Quantity
- ConditionQuantityISOUnit string? - ISO Unit Code for Condition Quantity
- TransactionCurrency string? - SD Document Currency
sap.s4hana.api_sales_quotation_srv: UpdateA_SalesQuotationItemText
Fields
- LongText string? -
sap.s4hana.api_sales_quotation_srv: UpdateA_SalesQuotationPartner
Fields
- Customer string? - Customer Number
- Supplier string? - Account Number of Supplier
- Personnel string? -
- ContactPerson string? - Number of Contact Person
sap.s4hana.api_sales_quotation_srv: UpdateA_SalesQuotationPrcgElmnt
Fields
- ConditionType string? -
- ConditionRateValue string? - Condition Amount or Percentage
- ConditionCurrency string? - Currency Key
- ConditionQuantity string? - Condition Pricing Unit
- ConditionQuantityUnit string? - Condition Unit in the Document
- ConditionQuantitySAPUnit string? - SAP Unit Code for Condition Quantity
- ConditionQuantityISOUnit string? - ISO Unit Code for Condition Quantity
- TransactionCurrency string? - SD Document Currency
sap.s4hana.api_sales_quotation_srv: UpdateA_SalesQuotationText
Fields
- LongText string? -
Array types
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationItemExpandOptions
A_SalesQuotationItemExpandOptions
sap.s4hana.api_sales_quotation_srv: SalesQuotationOfA_SalesQuotationItemSelectOptions
SalesQuotationOfA_SalesQuotationItemSelectOptions
sap.s4hana.api_sales_quotation_srv: SalesQuotationOfA_SlsQtanSubsqntProcFlowSelectOptions
SalesQuotationOfA_SlsQtanSubsqntProcFlowSelectOptions
sap.s4hana.api_sales_quotation_srv: PricingElementOfA_SalesQuotationItemSelectOptions
PricingElementOfA_SalesQuotationItemSelectOptions
sap.s4hana.api_sales_quotation_srv: SalesQuotationItemOfA_SlsQtanItmSubsqntProcFlowExpandOptions
SalesQuotationItemOfA_SlsQtanItmSubsqntProcFlowExpandOptions
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationTextExpandOptions
A_SalesQuotationTextExpandOptions
sap.s4hana.api_sales_quotation_srv: SalesQuotationOfA_SalesQuotationItemPrcgElmntExpandOptions
SalesQuotationOfA_SalesQuotationItemPrcgElmntExpandOptions
sap.s4hana.api_sales_quotation_srv: PrecedingProcFlowDocItemOfA_SalesQuotationItemSelectOptions
PrecedingProcFlowDocItemOfA_SalesQuotationItemSelectOptions
sap.s4hana.api_sales_quotation_srv: SalesQuotationOfA_SalesQuotationPrcgElmntExpandOptions
SalesQuotationOfA_SalesQuotationPrcgElmntExpandOptions
sap.s4hana.api_sales_quotation_srv: SalesQuotationOfA_SlsQtanItmSubsqntProcFlowExpandOptions
SalesQuotationOfA_SlsQtanItmSubsqntProcFlowExpandOptions
sap.s4hana.api_sales_quotation_srv: SalesQuotationOfA_SlsQtanItmSubsqntProcFlowSelectOptions
SalesQuotationOfA_SlsQtanItmSubsqntProcFlowSelectOptions
sap.s4hana.api_sales_quotation_srv: SubsequentProcFlowDocOfA_SalesQuotationSelectOptions
SubsequentProcFlowDocOfA_SalesQuotationSelectOptions
sap.s4hana.api_sales_quotation_srv: SalesQuotationOfA_SalesQuotationTextSelectOptions
SalesQuotationOfA_SalesQuotationTextSelectOptions
sap.s4hana.api_sales_quotation_srv: A_SlsQtanSubsqntProcFlowSelectOptions
A_SlsQtanSubsqntProcFlowSelectOptions
sap.s4hana.api_sales_quotation_srv: SalesQuotationOfA_SalesQuotationRelatedObjectSelectOptions
SalesQuotationOfA_SalesQuotationRelatedObjectSelectOptions
sap.s4hana.api_sales_quotation_srv: SubsequentProcFlowDocOfA_SalesQuotationExpandOptions
SubsequentProcFlowDocOfA_SalesQuotationExpandOptions
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationItemPrcgElmntOrderByOptions
A_SalesQuotationItemPrcgElmntOrderByOptions
sap.s4hana.api_sales_quotation_srv: A_SlsQtanItmPrecdgProcFlowSelectOptions
A_SlsQtanItmPrecdgProcFlowSelectOptions
sap.s4hana.api_sales_quotation_srv: PrecedingProcFlowDocItemOfA_SalesQuotationItemOrderByOptions
PrecedingProcFlowDocItemOfA_SalesQuotationItemOrderByOptions
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationTextSelectOptions
A_SalesQuotationTextSelectOptions
sap.s4hana.api_sales_quotation_srv: PricingElementOfA_SalesQuotationExpandOptions
PricingElementOfA_SalesQuotationExpandOptions
sap.s4hana.api_sales_quotation_srv: SalesQuotationOfA_SalesQuotationPrcgElmntSelectOptions
SalesQuotationOfA_SalesQuotationPrcgElmntSelectOptions
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationOrderByOptions
A_SalesQuotationOrderByOptions
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationItemTextExpandOptions
A_SalesQuotationItemTextExpandOptions
sap.s4hana.api_sales_quotation_srv: A_SlsQtanPrecdgProcFlowSelectOptions
A_SlsQtanPrecdgProcFlowSelectOptions
sap.s4hana.api_sales_quotation_srv: SalesQuotationOfA_SalesQuotationItemPrcgElmntSelectOptions
SalesQuotationOfA_SalesQuotationItemPrcgElmntSelectOptions
sap.s4hana.api_sales_quotation_srv: SalesQuotationOfA_SalesQuotationPartnerExpandOptions
SalesQuotationOfA_SalesQuotationPartnerExpandOptions
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationPrcgElmntSelectOptions
A_SalesQuotationPrcgElmntSelectOptions
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationItemSelectOptions
A_SalesQuotationItemSelectOptions
sap.s4hana.api_sales_quotation_srv: PricingElementOfA_SalesQuotationItemExpandOptions
PricingElementOfA_SalesQuotationItemExpandOptions
sap.s4hana.api_sales_quotation_srv: PrecedingProcFlowDocOfA_SalesQuotationExpandOptions
PrecedingProcFlowDocOfA_SalesQuotationExpandOptions
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationItemTextOrderByOptions
A_SalesQuotationItemTextOrderByOptions
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationPrcgElmntExpandOptions
A_SalesQuotationPrcgElmntExpandOptions
sap.s4hana.api_sales_quotation_srv: A_SlsQtanItmSubsqntProcFlowOrderByOptions
A_SlsQtanItmSubsqntProcFlowOrderByOptions
sap.s4hana.api_sales_quotation_srv: A_SlsQtanItemRelatedObjectOrderByOptions
A_SlsQtanItemRelatedObjectOrderByOptions
sap.s4hana.api_sales_quotation_srv: SalesQuotationItemOfA_SalesQuotationItemPartnerExpandOptions
SalesQuotationItemOfA_SalesQuotationItemPartnerExpandOptions
sap.s4hana.api_sales_quotation_srv: A_SlsQtanPrecdgProcFlowOrderByOptions
A_SlsQtanPrecdgProcFlowOrderByOptions
sap.s4hana.api_sales_quotation_srv: PricingElementOfA_SalesQuotationSelectOptions
PricingElementOfA_SalesQuotationSelectOptions
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationRelatedObjectOrderByOptions
A_SalesQuotationRelatedObjectOrderByOptions
sap.s4hana.api_sales_quotation_srv: PrecedingProcFlowDocItemOfA_SalesQuotationItemExpandOptions
PrecedingProcFlowDocItemOfA_SalesQuotationItemExpandOptions
sap.s4hana.api_sales_quotation_srv: A_SlsQtanSubsqntProcFlowExpandOptions
A_SlsQtanSubsqntProcFlowExpandOptions
sap.s4hana.api_sales_quotation_srv: SalesQuotationItemOfA_SalesQuotationItemTextSelectOptions
SalesQuotationItemOfA_SalesQuotationItemTextSelectOptions
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationItemPartnerExpandOptions
A_SalesQuotationItemPartnerExpandOptions
sap.s4hana.api_sales_quotation_srv: SalesQuotationOfA_SalesQuotationItemTextSelectOptions
SalesQuotationOfA_SalesQuotationItemTextSelectOptions
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationPartnerOrderByOptions
A_SalesQuotationPartnerOrderByOptions
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationSelectOptions
A_SalesQuotationSelectOptions
sap.s4hana.api_sales_quotation_srv: SalesQuotationItemOfA_SlsQtanItmPrecdgProcFlowSelectOptions
SalesQuotationItemOfA_SlsQtanItmPrecdgProcFlowSelectOptions
sap.s4hana.api_sales_quotation_srv: RelatedObjectOfA_SalesQuotationItemSelectOptions
RelatedObjectOfA_SalesQuotationItemSelectOptions
sap.s4hana.api_sales_quotation_srv: SubsequentProcFlowDocItemOfA_SalesQuotationItemExpandOptions
SubsequentProcFlowDocItemOfA_SalesQuotationItemExpandOptions
sap.s4hana.api_sales_quotation_srv: SalesQuotationItemOfA_SlsQtanItemRelatedObjectSelectOptions
SalesQuotationItemOfA_SlsQtanItemRelatedObjectSelectOptions
sap.s4hana.api_sales_quotation_srv: SalesQuotationOfA_SlsQtanPrecdgProcFlowExpandOptions
SalesQuotationOfA_SlsQtanPrecdgProcFlowExpandOptions
sap.s4hana.api_sales_quotation_srv: SalesQuotationItemOfA_SalesQuotationItemPrcgElmntSelectOptions
SalesQuotationItemOfA_SalesQuotationItemPrcgElmntSelectOptions
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationPartnerExpandOptions
A_SalesQuotationPartnerExpandOptions
sap.s4hana.api_sales_quotation_srv: SalesQuotationOfA_SlsQtanItmPrecdgProcFlowExpandOptions
SalesQuotationOfA_SlsQtanItmPrecdgProcFlowExpandOptions
sap.s4hana.api_sales_quotation_srv: SalesQuotationOfA_SlsQtanItemRelatedObjectExpandOptions
SalesQuotationOfA_SlsQtanItemRelatedObjectExpandOptions
sap.s4hana.api_sales_quotation_srv: SubsequentProcFlowDocItemOfA_SalesQuotationItemOrderByOptions
SubsequentProcFlowDocItemOfA_SalesQuotationItemOrderByOptions
sap.s4hana.api_sales_quotation_srv: SalesQuotationOfA_SalesQuotationItemPartnerSelectOptions
SalesQuotationOfA_SalesQuotationItemPartnerSelectOptions
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationItemPrcgElmntExpandOptions
A_SalesQuotationItemPrcgElmntExpandOptions
sap.s4hana.api_sales_quotation_srv: SalesQuotationItemOfA_SalesQuotationItemTextExpandOptions
SalesQuotationItemOfA_SalesQuotationItemTextExpandOptions
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationItemPartnerSelectOptions
A_SalesQuotationItemPartnerSelectOptions
sap.s4hana.api_sales_quotation_srv: A_SlsQtanItmPrecdgProcFlowOrderByOptions
A_SlsQtanItmPrecdgProcFlowOrderByOptions
sap.s4hana.api_sales_quotation_srv: SubsequentProcFlowDocOfA_SalesQuotationOrderByOptions
SubsequentProcFlowDocOfA_SalesQuotationOrderByOptions
sap.s4hana.api_sales_quotation_srv: A_SlsQtanItemRelatedObjectSelectOptions
A_SlsQtanItemRelatedObjectSelectOptions
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationItemOrderByOptions
A_SalesQuotationItemOrderByOptions
sap.s4hana.api_sales_quotation_srv: PricingElementOfA_SalesQuotationOrderByOptions
PricingElementOfA_SalesQuotationOrderByOptions
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationRelatedObjectSelectOptions
A_SalesQuotationRelatedObjectSelectOptions
sap.s4hana.api_sales_quotation_srv: SalesQuotationOfA_SalesQuotationPartnerSelectOptions
SalesQuotationOfA_SalesQuotationPartnerSelectOptions
sap.s4hana.api_sales_quotation_srv: A_SlsQtanPrecdgProcFlowExpandOptions
A_SlsQtanPrecdgProcFlowExpandOptions
sap.s4hana.api_sales_quotation_srv: PricingElementOfA_SalesQuotationItemOrderByOptions
PricingElementOfA_SalesQuotationItemOrderByOptions
sap.s4hana.api_sales_quotation_srv: PrecedingProcFlowDocOfA_SalesQuotationOrderByOptions
PrecedingProcFlowDocOfA_SalesQuotationOrderByOptions
sap.s4hana.api_sales_quotation_srv: A_SlsQtanItmSubsqntProcFlowSelectOptions
A_SlsQtanItmSubsqntProcFlowSelectOptions
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationItemTextSelectOptions
A_SalesQuotationItemTextSelectOptions
sap.s4hana.api_sales_quotation_srv: PrecedingProcFlowDocOfA_SalesQuotationSelectOptions
PrecedingProcFlowDocOfA_SalesQuotationSelectOptions
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationTextOrderByOptions
A_SalesQuotationTextOrderByOptions
sap.s4hana.api_sales_quotation_srv: A_SlsQtanItmSubsqntProcFlowExpandOptions
A_SlsQtanItmSubsqntProcFlowExpandOptions
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationRelatedObjectExpandOptions
A_SalesQuotationRelatedObjectExpandOptions
sap.s4hana.api_sales_quotation_srv: SalesQuotationItemOfA_SlsQtanItemRelatedObjectExpandOptions
SalesQuotationItemOfA_SlsQtanItemRelatedObjectExpandOptions
sap.s4hana.api_sales_quotation_srv: SalesQuotationItemOfA_SlsQtanItmPrecdgProcFlowExpandOptions
SalesQuotationItemOfA_SlsQtanItmPrecdgProcFlowExpandOptions
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationItemPartnerOrderByOptions
A_SalesQuotationItemPartnerOrderByOptions
sap.s4hana.api_sales_quotation_srv: RelatedObjectOfA_SalesQuotationItemExpandOptions
RelatedObjectOfA_SalesQuotationItemExpandOptions
sap.s4hana.api_sales_quotation_srv: SalesQuotationOfA_SalesQuotationItemTextExpandOptions
SalesQuotationOfA_SalesQuotationItemTextExpandOptions
sap.s4hana.api_sales_quotation_srv: SalesQuotationOfA_SalesQuotationTextExpandOptions
SalesQuotationOfA_SalesQuotationTextExpandOptions
sap.s4hana.api_sales_quotation_srv: SalesQuotationOfA_SalesQuotationRelatedObjectExpandOptions
SalesQuotationOfA_SalesQuotationRelatedObjectExpandOptions
sap.s4hana.api_sales_quotation_srv: A_SlsQtanItemRelatedObjectExpandOptions
A_SlsQtanItemRelatedObjectExpandOptions
sap.s4hana.api_sales_quotation_srv: A_SlsQtanItmPrecdgProcFlowExpandOptions
A_SlsQtanItmPrecdgProcFlowExpandOptions
sap.s4hana.api_sales_quotation_srv: SalesQuotationItemOfA_SlsQtanItmSubsqntProcFlowSelectOptions
SalesQuotationItemOfA_SlsQtanItmSubsqntProcFlowSelectOptions
sap.s4hana.api_sales_quotation_srv: RelatedObjectOfA_SalesQuotationItemOrderByOptions
RelatedObjectOfA_SalesQuotationItemOrderByOptions
sap.s4hana.api_sales_quotation_srv: A_SlsQtanSubsqntProcFlowOrderByOptions
A_SlsQtanSubsqntProcFlowOrderByOptions
sap.s4hana.api_sales_quotation_srv: SalesQuotationOfA_SlsQtanItemRelatedObjectSelectOptions
SalesQuotationOfA_SlsQtanItemRelatedObjectSelectOptions
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationItemPrcgElmntSelectOptions
A_SalesQuotationItemPrcgElmntSelectOptions
sap.s4hana.api_sales_quotation_srv: SalesQuotationOfA_SalesQuotationItemExpandOptions
SalesQuotationOfA_SalesQuotationItemExpandOptions
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationPartnerSelectOptions
A_SalesQuotationPartnerSelectOptions
sap.s4hana.api_sales_quotation_srv: SubsequentProcFlowDocItemOfA_SalesQuotationItemSelectOptions
SubsequentProcFlowDocItemOfA_SalesQuotationItemSelectOptions
sap.s4hana.api_sales_quotation_srv: SalesQuotationOfA_SalesQuotationItemPartnerExpandOptions
SalesQuotationOfA_SalesQuotationItemPartnerExpandOptions
sap.s4hana.api_sales_quotation_srv: SalesQuotationOfA_SlsQtanSubsqntProcFlowExpandOptions
SalesQuotationOfA_SlsQtanSubsqntProcFlowExpandOptions
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationPrcgElmntOrderByOptions
A_SalesQuotationPrcgElmntOrderByOptions
sap.s4hana.api_sales_quotation_srv: SalesQuotationItemOfA_SalesQuotationItemPrcgElmntExpandOptions
SalesQuotationItemOfA_SalesQuotationItemPrcgElmntExpandOptions
sap.s4hana.api_sales_quotation_srv: A_SalesQuotationExpandOptions
A_SalesQuotationExpandOptions
sap.s4hana.api_sales_quotation_srv: SalesQuotationItemOfA_SalesQuotationItemPartnerSelectOptions
SalesQuotationItemOfA_SalesQuotationItemPartnerSelectOptions
sap.s4hana.api_sales_quotation_srv: SalesQuotationOfA_SlsQtanItmPrecdgProcFlowSelectOptions
SalesQuotationOfA_SlsQtanItmPrecdgProcFlowSelectOptions
sap.s4hana.api_sales_quotation_srv: SalesQuotationOfA_SlsQtanPrecdgProcFlowSelectOptions
SalesQuotationOfA_SlsQtanPrecdgProcFlowSelectOptions
String types
sap.s4hana.api_sales_quotation_srv: count
count
The number of entities in the collection. Available when using the $inlinecount query option.
Import
import ballerinax/sap.s4hana.api_sales_quotation_srv;
Metadata
Released date: 7 months ago
Version: 1.0.0
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.9.0
GraalVM compatible: Yes
Pull count
Total: 1
Current verison: 1
Weekly downloads
Keywords
Business Management/ERP
Cost/Paid
Vendor/SAP
Sales and Distribution
SD
Contributors
Other versions
1.0.0