salesforce.pardot
Module salesforce.pardot
API
Definitions
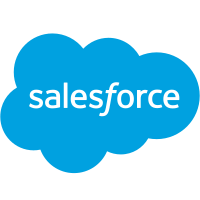
ballerinax/salesforce.pardot Ballerina library
Overview
This is a generated connector for Salesforce Pardot API v5 OpenAPI specification.
The version 5 of the Pardot API to read and update a variety of assets and objects, like File and Layout Template objects.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Salesforce Account.
- Obtain tokens by following this guide.
Quickstart
To use the Salesfore Pardot connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/salesforce.pardot
module into the Ballerina project.
import ballerinax/salesforce.pardot;
Step 2 - Create a new connector instance
You can now make the connection configuration using the access token.
pardot:ClientConfig clientConfig = { auth : { token: token } }; pardot:Client baseClient = check new Client(clientConfig);
Step 3 - Invoke connector operation
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example to get data of a campaign using the connector.
public function main() { string fieldsToRead = "id,name,isDeleted"; string campaignId = "7015g000000LioNAAS"; pardot:Record|error data = baseClient->readCampaign(fieldsToRead, campaignId); if (data is pardot:Record) { log:printInfo("Campaign " + data.toString()); } else { log:printInfo("Error Occured"); } }
- Use
bal run
command to compile and run the Ballerina program.
Clients
salesforce.pardot: Client
This is a generated connector for Salesforce Pardot API v5 OpenAPI specification. The version 5 of the Pardot API to read and update a variety of assets and objects, like File and Layout Template objects.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Salesforce account and obtain tokens following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://pi.demo.pardot.com/api/v5" - URL of the target service
readCampaign
Retrieves a single campaign.
queryCampaigns
function queryCampaigns(string fields, string? orderBy, int? id, int? idGreaterThan, int? idGreaterThanOrEqualTo, int? idLessThan, int? idLessThanOrEqualTo, string? name, string? createdAt, string? createdAtAfter, string? createdAtAfterOrEqualTo, string? createdAtBefore, string? createdAtBeforeOrEqualTo, string? updatedAt, string? updatedAtAfter, string? updatedAtAfterOrEqualTo, string? updatedAtBefore, string? updatedAtBeforeOrEqualTo) returns Record|error
Retrieves a collection of campaigns.
Parameters
- fields string - Record fields to return
- orderBy string? (default ()) - When executing a query, the following fields can be specified in the orderBy parameter.
- id int? (default ()) - Returns any records where ID is equal to the given integer value.
- idGreaterThan int? (default ()) - Returns any records where ID is greater than the specified value, non-inclusive.
- idGreaterThanOrEqualTo int? (default ()) - Returns any records where ID is greater than or equal to the specified value.
- idLessThan int? (default ()) - Returns any records where ID is less than the specified value, non-inclusive.
- idLessThanOrEqualTo int? (default ()) - Returns any records where ID is less than or equal to the specified value.
- name string? (default ()) - Returns any records where Name is equal to the given string value.
- createdAt string? (default ()) - Returns any records where CreatedAt is equal to the given datetime value.
- createdAtAfter string? (default ()) - Returns any records where CreatedAt is after the given datetime value, non-inclusive.
- createdAtAfterOrEqualTo string? (default ()) - Returns any records where CreatedAt is after or equal to the given datetime value.
- createdAtBefore string? (default ()) - Returns any records where CreatedAt is before the given datetime value, non-inclusive.
- createdAtBeforeOrEqualTo string? (default ()) - Returns any records where CreatedAt is before or equal to the given datetime value.
- updatedAt string? (default ()) - Returns any records where UpdatedAt is equal to the given datetime value.e.
- updatedAtAfter string? (default ()) - Returns any records where UpdatedAt is after the given datetime value, non-inclusive.
- updatedAtAfterOrEqualTo string? (default ()) - Returns any records where UpdatedAt is after or equal to the given datetime value.
- updatedAtBefore string? (default ()) - Returns any records where UpdatedAt is before the given datetime value, non-inclusive.
- updatedAtBeforeOrEqualTo string? (default ()) - Returns any records where UpdatedAt is before or equal to the given datetime value.
readCustomField
Retrieves a single custom field.
deleteCustomField
Deletes a custom field.
Parameters
- id string - (required) CustomField record id
updateCustomField
function updateCustomField(string fields, string id, CustomFieldUpdateRequest payload) returns Record|error
Updates a custom field.
Parameters
- fields string - Record fields to return
- id string - Record id
- payload CustomFieldUpdateRequest - Custom Field update request
queryCustomFields
function queryCustomFields(string fields, string? orderBy, int? id, int? idGreaterThan, int? idGreaterThanOrEqualTo, int? idLessThan, int? idLessThanOrEqualTo, string? createdAt, string? createdAtAfter, string? createdAtAfterOrEqualTo, string? createdAtBefore, string? createdAtBeforeOrEqualTo, string? updatedAt, string? updatedAtAfter, string? updatedAtAfterOrEqualTo, string? updatedAtBefore, string? updatedAtBeforeOrEqualTo) returns Record|error
Retrieves a collection of custom fields.
Parameters
- fields string - Record fields to return
- orderBy string? (default ()) - When executing a query, the following fields can be specified in the orderBy parameter.
- id int? (default ()) - Returns any records where ID is equal to the given integer value.
- idGreaterThan int? (default ()) - Returns any records where ID is greater than the specified value, non-inclusive.
- idGreaterThanOrEqualTo int? (default ()) - Returns any records where ID is greater than or equal to the specified value.
- idLessThan int? (default ()) - Returns any records where ID is less than the specified value, non-inclusive.
- idLessThanOrEqualTo int? (default ()) - Returns any records where ID is less than or equal to the specified value.
- createdAt string? (default ()) - Returns any records where CreatedAt is equal to the given datetime value.
- createdAtAfter string? (default ()) - Returns any records where CreatedAt is after the given datetime value, non-inclusive.
- createdAtAfterOrEqualTo string? (default ()) - Returns any records where CreatedAt is after or equal to the given datetime value.
- createdAtBefore string? (default ()) - Returns any records where CreatedAt is before the given datetime value, non-inclusive.
- createdAtBeforeOrEqualTo string? (default ()) - Returns any records where CreatedAt is before or equal to the given datetime value.
- updatedAt string? (default ()) - Returns any records where UpdatedAt is equal to the given datetime value.e.
- updatedAtAfter string? (default ()) - Returns any records where UpdatedAt is after the given datetime value, non-inclusive.
- updatedAtAfterOrEqualTo string? (default ()) - Returns any records where UpdatedAt is after or equal to the given datetime value.
- updatedAtBefore string? (default ()) - Returns any records where UpdatedAt is before the given datetime value, non-inclusive.
- updatedAtBeforeOrEqualTo string? (default ()) - Returns any records where UpdatedAt is before or equal to the given datetime value.
createCustomField
function createCustomField(string fields, CustomFieldCreateRequest payload) returns Record|error
Creates a custom field.
Parameters
- fields string - Record fields to return
- payload CustomFieldCreateRequest - Custom Field create request
readCustomRedirect
Retrieves a single custom redirect.
deleteCustomRedirect
Deletes a custom redirect.
Parameters
- id string - Record id
updateCustomRedirect
function updateCustomRedirect(string fields, string id, CustomRedirectsUpdateRequest payload) returns Record|error
Updates a custom redirect.
Parameters
- fields string - Record fields to return
- id string - Record id
- payload CustomRedirectsUpdateRequest - Custom Redirects update request
queryCustomRedirects
function queryCustomRedirects(string fields, string? orderBy, int? id, int? idGreaterThan, int? idGreaterThanOrEqualTo, int? idLessThan, int? idLessThanOrEqualTo) returns Record|error
Retrieves a collection of custom redirects.
Parameters
- fields string - Record fields to return
- orderBy string? (default ()) - When executing a query, the following fields can be specified in the orderBy parameter.
- id int? (default ()) - Returns any records where ID is equal to the given integer value.
- idGreaterThan int? (default ()) - Returns any records where ID is greater than the specified value, non-inclusive.
- idGreaterThanOrEqualTo int? (default ()) - Returns any records where ID is greater than or equal to the specified value.
- idLessThan int? (default ()) - Returns any records where ID is less than the specified value, non-inclusive.
- idLessThanOrEqualTo int? (default ()) - Returns any records where ID is less than or equal to the specified value.
createCustomRedirect
function createCustomRedirect(string fields, CustomRedirectsCreateRequest payload) returns Record|error
Creates a custom redirect.
Parameters
- fields string - (required) CustomRedirect fields to return
- payload CustomRedirectsCreateRequest - Custom Redirects create request
createExternalActivity
function createExternalActivity(ExternalActivityCreateRequest payload) returns Record|error
Submits external activity to Pardot.
Parameters
- payload ExternalActivityCreateRequest - External Activity create request
readFile
Retrieves a single file.
deleteFile
Deletes a file.
Parameters
- id string - (required) record id
updateFile
function updateFile(string fields, string id, FileUpdateRequest payload) returns Record|error
Updates a file.
Parameters
- fields string - Record fields to return
- id string - Record id
- payload FileUpdateRequest - File update request
queryFiles
Retrieves a collection of files.
Parameters
- fields string - Record fields to return
- orderBy string? (default ()) - When executing a query, the following fields can be specified in the orderBy parameter.
createFile
function createFile(string fields, FileCreateRequestObject payload) returns Record|error
Creates a file.
Parameters
- fields string - Record fields to return
- payload FileCreateRequestObject - File create request payload
readFolder
Retrieve a single folder.
queryFolders
function queryFolders(string fields, string? orderBy, int? id, int? idGreaterThan, int? idGreaterThanOrEqualTo, int? idLessThan, int? idLessThanOrEqualTo, int? parentFolderId, int? parentFolderIdGreaterThan, int? parentFolderIdGreaterThanOrEqualTo, int? parentFolderIdLessThan, int? parentFolderIdLessThanOrEqualTo, boolean? parentFolderIdIsNull, boolean? parentFolderIdIsNotNull) returns Record|error
Retrieves a collection of folders.
Parameters
- fields string - Record fields to return
- orderBy string? (default ()) - When executing a query, the following fields can be specified in the orderBy parameter.
- id int? (default ()) - Returns any records where ID is equal to the given integer value.
- idGreaterThan int? (default ()) - Returns any records where ID is greater than the specified value, non-inclusive.
- idGreaterThanOrEqualTo int? (default ()) - Returns any records where ID is greater than or equal to the specified value.
- idLessThan int? (default ()) - Returns any records where ID is less than the specified value, non-inclusive.
- idLessThanOrEqualTo int? (default ()) - Returns any records where ID is less than or equal to the specified value.
- parentFolderId int? (default ()) - Returns any records where the parentFolderId is equal to the given integer value.
- parentFolderIdGreaterThan int? (default ()) - Returns any records where the parentFolderId is greater than the specified value, non-inclusive.
- parentFolderIdGreaterThanOrEqualTo int? (default ()) - Returns any records where the parentFolderId is greater than or equal to the specified value.
- parentFolderIdLessThan int? (default ()) - Returns any records where the parentFolderId is less than the specified value, non-inclusive.
- parentFolderIdLessThanOrEqualTo int? (default ()) - Returns any records where the parentFolderId is less than or equal to the specified value.
- parentFolderIdIsNull boolean? (default ()) - Returns any records where the parentFolderId is null when true is specified.
- parentFolderIdIsNotNull boolean? (default ()) - Returns any records where the parentFolderId isn’t null when true is specified.
readForm
Retrieve a single form.
queryForms
function queryForms(string fields, string? orderBy, int? id, int? idGreaterThan, int? idGreaterThanOrEqualTo, int? idLessThan, int? idLessThanOrEqualTo) returns Record|error
Retrieve a collection of forms.
Parameters
- fields string - Record fields to return
- orderBy string? (default ()) - When executing a query, the following fields can be specified in the orderBy parameter.
- id int? (default ()) - Returns any records where ID is equal to the given integer value.
- idGreaterThan int? (default ()) - Returns any records where ID is greater than the specified value, non-inclusive.
- idGreaterThanOrEqualTo int? (default ()) - Returns any records where ID is greater than or equal to the specified value.
- idLessThan int? (default ()) - Returns any records where ID is less than the specified value, non-inclusive.
- idLessThanOrEqualTo int? (default ()) - Returns any records where ID is less than or equal to the specified value.
readLayoutTemplates
Retrieve a single layout template.
deleteLayoutTemplate
Delete a layout template.
Parameters
- id string - (required) record id
updateLayoutTemplate
function updateLayoutTemplate(string fields, string id, LayoutTemplateUpdateRequest payload) returns Record|error
Update a layout template.
Parameters
- fields string - Record fields to return
- id string - Record id
- payload LayoutTemplateUpdateRequest - Layout Template update request
queryLayoutTemplates
function queryLayoutTemplates(string fields, string? orderBy, int? id, int? idGreaterThan, int? idGreaterThanOrEqualTo, int? idLessThan, int? idLessThanOrEqualTo) returns Record|error
Retrieve a collection of layout templates.
Parameters
- fields string - Record fields to return
- orderBy string? (default ()) - When executing a query, the following fields can be specified in the orderBy parameter.
- id int? (default ()) - Returns any records where ID is equal to the given integer value.
- idGreaterThan int? (default ()) - Returns any records where ID is greater than the specified value, non-inclusive.
- idGreaterThanOrEqualTo int? (default ()) - Returns any records where ID is greater than or equal to the specified value.
- idLessThan int? (default ()) - Returns any records where ID is less than the specified value, non-inclusive.
- idLessThanOrEqualTo int? (default ()) - Returns any records where ID is less than or equal to the specified value.
createLayoutTemplate
function createLayoutTemplate(string fields, LayoutTemplateCreateRequest payload) returns Record|error
Create a layout template.
Parameters
- fields string - Record fields to return
- payload LayoutTemplateCreateRequest - Layout Template create request
readList
Retrieve a single list.
deleteList
Delete a list.
Parameters
- id string - Record id
updateList
function updateList(string fields, string id, ListUpdateRequest payload) returns Record|error
Update a list.
Parameters
- fields string - Record fields to return
- id string - Record id
- payload ListUpdateRequest - List update request
queryLists
function queryLists(string fields, string? orderBy, int? id, int? idGreaterThan, int? idGreaterThanOrEqualTo, int? idLessThan, int? idLessThanOrEqualTo, string? name, string? createdAt, string? createdAtAfter, string? createdAtAfterOrEqualTo, string? createdAtBefore, string? createdAtBeforeOrEqualTo, string? updatedAt, string? updatedAtAfter, string? updatedAtAfterOrEqualTo, string? updatedAtBefore, string? updatedAtBeforeOrEqualTo) returns Record|error
Retrieve a collection of lists.
Parameters
- fields string - Record fields to return
- orderBy string? (default ()) - When executing a query, the following fields can be specified in the orderBy parameter.
- id int? (default ()) - Returns any records where ID is equal to the given integer value.
- idGreaterThan int? (default ()) - Returns any records where ID is greater than the specified value, non-inclusive.
- idGreaterThanOrEqualTo int? (default ()) - Returns any records where ID is greater than or equal to the specified value.
- idLessThan int? (default ()) - Returns any records where ID is less than the specified value, non-inclusive.
- idLessThanOrEqualTo int? (default ()) - Returns any records where ID is less than or equal to the specified value.
- name string? (default ()) - Returns any records where Name is equal to the given string value.
- createdAt string? (default ()) - Returns any records where CreatedAt is equal to the given datetime value.
- createdAtAfter string? (default ()) - Returns any records where CreatedAt is after the given datetime value, non-inclusive.
- createdAtAfterOrEqualTo string? (default ()) - Returns any records where CreatedAt is after or equal to the given datetime value.
- createdAtBefore string? (default ()) - Returns any records where CreatedAt is before the given datetime value, non-inclusive.
- createdAtBeforeOrEqualTo string? (default ()) - Returns any records where CreatedAt is before or equal to the given datetime value.
- updatedAt string? (default ()) - Returns any records where UpdatedAt is equal to the given datetime value.e.
- updatedAtAfter string? (default ()) - Returns any records where UpdatedAt is after the given datetime value, non-inclusive.
- updatedAtAfterOrEqualTo string? (default ()) - Returns any records where UpdatedAt is after or equal to the given datetime value.
- updatedAtBefore string? (default ()) - Returns any records where UpdatedAt is before the given datetime value, non-inclusive.
- updatedAtBeforeOrEqualTo string? (default ()) - Returns any records where UpdatedAt is before or equal to the given datetime value.
createList
function createList(string fields, ListCreateRequest payload) returns Record|error
Create a list.
readProspect
Retrieve a single prospect.
queryProspects
function queryProspects(string fields, string? orderBy, int? id, int? idGreaterThan, int? idGreaterThanOrEqualTo, int? idLessThan, int? idLessThanOrEqualTo, string? createdAt, string? createdAtAfter, string? createdAtAfterOrEqualTo, string? createdAtBefore, string? createdAtBeforeOrEqualTo, string? updatedAt, string? updatedAtAfter, string? updatedAtAfterOrEqualTo, string? updatedAtBefore, string? updatedAtBeforeOrEqualTo, string? lastActivityAt, string? lastActivityAtAfter, string? lastActivityAtAfterOrEqualTo, string? lastActivityAtBefore, string? lastActivityAtBeforeOrEqualTo, int? userId, int? userIdGreaterThan, int? userIdGreaterThanOrEqualTo, int? userIdLessThan, int? userIdLessThanOrEqualTo, int? assignedToId, int? assignedToIdGreaterThan, int? assignedToIdGreaterThanOrEqualTo, int? assignedToIdLessThan, int? assignedToIdLessThanOrEqualTo) returns Record|error
Retrieve a collection of prospects.
Parameters
- fields string - Record fields to return
- orderBy string? (default ()) - When executing a query, the following fields can be specified in the orderBy parameter.
- id int? (default ()) - Returns any records where ID is equal to the given integer value.
- idGreaterThan int? (default ()) - Returns any records where ID is greater than the specified value, non-inclusive.
- idGreaterThanOrEqualTo int? (default ()) - Returns any records where ID is greater than or equal to the specified value.
- idLessThan int? (default ()) - Returns any records where ID is less than the specified value, non-inclusive.
- idLessThanOrEqualTo int? (default ()) - Returns any records where ID is less than or equal to the specified value.
- createdAt string? (default ()) - Returns any records where CreatedAt is equal to the given datetime value.
- createdAtAfter string? (default ()) - Returns any records where CreatedAt is after the given datetime value, non-inclusive.
- createdAtAfterOrEqualTo string? (default ()) - Returns any records where CreatedAt is after or equal to the given datetime value.
- createdAtBefore string? (default ()) - Returns any records where CreatedAt is before the given datetime value, non-inclusive.
- createdAtBeforeOrEqualTo string? (default ()) - Returns any records where CreatedAt is before or equal to the given datetime value.
- updatedAt string? (default ()) - Returns any records where UpdatedAt is equal to the given datetime value.e.
- updatedAtAfter string? (default ()) - Returns any records where UpdatedAt is after the given datetime value, non-inclusive.
- updatedAtAfterOrEqualTo string? (default ()) - Returns any records where UpdatedAt is after or equal to the given datetime value.
- updatedAtBefore string? (default ()) - Returns any records where UpdatedAt is before the given datetime value, non-inclusive.
- updatedAtBeforeOrEqualTo string? (default ()) - Returns any records where UpdatedAt is before or equal to the given datetime value.
- lastActivityAt string? (default ()) - Returns any records where LastActivityAt is equal to the given datetime value.
- lastActivityAtAfter string? (default ()) - Returns any records where LastActivityAt is after the given datetime value, non-inclusive.
- lastActivityAtAfterOrEqualTo string? (default ()) - Returns any records where LastActivityAt is after or equal to the given datetime value.
- lastActivityAtBefore string? (default ()) - Returns any records where LastActivityAt is before the given datetime value, non-inclusive.
- lastActivityAtBeforeOrEqualTo string? (default ()) - Returns any records where LastActivityAt is before or equal to the given datetime value.
- userId int? (default ()) - Returns any records where UserId is equal to the given integer value.
- userIdGreaterThan int? (default ()) - Returns any records where UserId is greater than the specified value, non-inclusive.
- userIdGreaterThanOrEqualTo int? (default ()) - Returns any records where UserId is greater than or equal to the specified value.
- userIdLessThan int? (default ()) - Returns any records where UserId is less than the specified value, non-inclusive.
- userIdLessThanOrEqualTo int? (default ()) - Returns any records where UserId is less than or equal to the specified value.
- assignedToId int? (default ()) - Returns any records where AssignedToId is equal to the given integer value.
- assignedToIdGreaterThan int? (default ()) - Returns any records where AssignedToId is greater than the specified value, non-inclusive.
- assignedToIdGreaterThanOrEqualTo int? (default ()) - Returns any records where AssignedToId is greater than or equal to the specified value.
- assignedToIdLessThan int? (default ()) - Returns any records where AssignedToId is less than the specified value, non-inclusive.
- assignedToIdLessThanOrEqualTo int? (default ()) - Returns any records where AssignedToId is less than or equal to the specified value.
readProspectAccount
Retrieve a single prospect account.
queryProspectAccounts
function queryProspectAccounts(string fields, string? orderBy, int? id, int? idGreaterThan, int? idGreaterThanOrEqualTo, int? idLessThan, int? idLessThanOrEqualTo) returns Record|error
Retrieve a collection of prospect accounts.
Parameters
- fields string - Record fields to return
- orderBy string? (default ()) - When executing a query, the following fields can be specified in the orderBy parameter.
- id int? (default ()) - Returns any records where ID is equal to the given integer value.
- idGreaterThan int? (default ()) - Returns any records where ID is greater than the specified value, non-inclusive.
- idGreaterThanOrEqualTo int? (default ()) - Returns any records where ID is greater than or equal to the specified value.
- idLessThan int? (default ()) - Returns any records where ID is less than the specified value, non-inclusive.
- idLessThanOrEqualTo int? (default ()) - Returns any records where ID is less than or equal to the specified value.
readTrackerDomain
Retrieve a single tracker domain.
queryTrackerDomains
function queryTrackerDomains(string fields, string? orderBy, int? id, int? idGreaterThan, int? idGreaterThanOrEqualTo, int? idLessThan, int? idLessThanOrEqualTo) returns Record|error
Retrieve a collection of tracker domains.
Parameters
- fields string - Record fields to return
- orderBy string? (default ()) - When executing a query, the following fields can be specified in the orderBy parameter.
- id int? (default ()) - Returns any records where ID is equal to the given integer value.
- idGreaterThan int? (default ()) - Returns any records where ID is greater than the specified value, non-inclusive.
- idGreaterThanOrEqualTo int? (default ()) - Returns any records where ID is greater than or equal to the specified value.
- idLessThan int? (default ()) - Returns any records where ID is less than the specified value, non-inclusive.
- idLessThanOrEqualTo int? (default ()) - Returns any records where ID is less than or equal to the specified value.
readUser
Retrieve a single user.
queryUsers
function queryUsers(string fields, string? orderBy, int? id, int? idGreaterThan, int? idGreaterThanOrEqualTo, int? idLessThan, int? idLessThanOrEqualTo, string? email, string? createdAt, string? createdAtAfter, string? createdAtAfterOrEqualTo, string? createdAtBefore, string? createdAtBeforeOrEqualTo, string? updatedAt, string? updatedAtAfter, string? updatedAtAfterOrEqualTo, string? updatedAtBefore, string? updatedAtBeforeOrEqualTo) returns Record|error
Retrieve a collection of users.
Parameters
- fields string - Record fields to return
- orderBy string? (default ()) - When executing a query, the following fields can be specified in the orderBy parameter.
- id int? (default ()) - Returns any records where ID is equal to the given integer value.
- idGreaterThan int? (default ()) - Returns any records where ID is greater than the specified value, non-inclusive.
- idGreaterThanOrEqualTo int? (default ()) - Returns any records where ID is greater than or equal to the specified value.
- idLessThan int? (default ()) - Returns any records where ID is less than the specified value, non-inclusive.
- idLessThanOrEqualTo int? (default ()) - Returns any records where ID is less than or equal to the specified value.
- email string? (default ()) - Returns any records where Email is equal to the given string value.
- createdAt string? (default ()) - Returns any records where CreatedAt is equal to the given datetime value.
- createdAtAfter string? (default ()) - Returns any records where CreatedAt is after the given datetime value, non-inclusive.
- createdAtAfterOrEqualTo string? (default ()) - Returns any records where CreatedAt is after or equal to the given datetime value.
- createdAtBefore string? (default ()) - Returns any records where CreatedAt is before the given datetime value, non-inclusive.
- createdAtBeforeOrEqualTo string? (default ()) - Returns any records where CreatedAt is before or equal to the given datetime value.
- updatedAt string? (default ()) - Returns any records where UpdatedAt is equal to the given datetime value.e.
- updatedAtAfter string? (default ()) - Returns any records where UpdatedAt is after the given datetime value, non-inclusive.
- updatedAtAfterOrEqualTo string? (default ()) - Returns any records where UpdatedAt is after or equal to the given datetime value.
- updatedAtBefore string? (default ()) - Returns any records where UpdatedAt is before the given datetime value, non-inclusive.
- updatedAtBeforeOrEqualTo string? (default ()) - Returns any records where UpdatedAt is before or equal to the given datetime value.
readVisit
Retrieve a single visit.
queryVisits
function queryVisits(string fields, string? orderBy, int? id, int? idGreaterThan, int? idGreaterThanOrEqualTo, int? idLessThan, int? idLessThanOrEqualTo, string? createdAt, string? createdAtAfter, string? createdAtAfterOrEqualTo, string? createdAtBefore, string? createdAtBeforeOrEqualTo, int? visitorId, int? visitorIdGreaterThan, int? visitorIdGreaterThanOrEqualTo, int? visitorIdLessThan, int? visitorIdLessThanOrEqualTo, int? prospectId, int? prospectIdGreaterThan, int? prospectIdGreaterThanOrEqualTo, int? prospectIdLessThan, int? prospectIdLessThanOrEqualTo, string? updatedAt, string? updatedAtAfter, string? updatedAtAfterOrEqualTo, string? updatedAtBefore, string? updatedAtBeforeOrEqualTo) returns Record|error
Retrieve a collection of visits.
Parameters
- fields string - Record fields to return
- orderBy string? (default ()) - When executing a query, the following fields can be specified in the orderBy parameter.
- id int? (default ()) - Returns any records where ID is equal to the given integer value.
- idGreaterThan int? (default ()) - Returns any records where ID is greater than the specified value, non-inclusive.
- idGreaterThanOrEqualTo int? (default ()) - Returns any records where ID is greater than or equal to the specified value.
- idLessThan int? (default ()) - Returns any records where ID is less than the specified value, non-inclusive.
- idLessThanOrEqualTo int? (default ()) - Returns any records where ID is less than or equal to the specified value.
- createdAt string? (default ()) - Returns any records where CreatedAt is equal to the given datetime value.
- createdAtAfter string? (default ()) - Returns any records where CreatedAt is after the given datetime value, non-inclusive.
- createdAtAfterOrEqualTo string? (default ()) - Returns any records where CreatedAt is after or equal to the given datetime value.
- createdAtBefore string? (default ()) - Returns any records where CreatedAt is before the given datetime value, non-inclusive.
- createdAtBeforeOrEqualTo string? (default ()) - Returns any records where CreatedAt is before or equal to the given datetime value.
- visitorId int? (default ()) - Returns any records where VisitorId is equal to the given integer value.
- visitorIdGreaterThan int? (default ()) - Returns any records where VisitorId is greater than the specified value, non-inclusive.
- visitorIdGreaterThanOrEqualTo int? (default ()) - Returns any records where VisitorId is greater than or equal to the specified value.
- visitorIdLessThan int? (default ()) - Returns any records where VisitorId is less than the specified value, non-inclusive.
- visitorIdLessThanOrEqualTo int? (default ()) - Returns any records where CreatedAt is before the given datetime value, non-inclusive.
- prospectId int? (default ()) - Returns any records where ProspectId is equal to the given integer value.
- prospectIdGreaterThan int? (default ()) - Returns any records where ProspectId is greater than the specified value, non-inclusive.
- prospectIdGreaterThanOrEqualTo int? (default ()) - Returns any records where ProspectId is greater than or equal to the specified value.
- prospectIdLessThan int? (default ()) - Returns any records where ProspectId is less than the specified value, non-inclusive.
- prospectIdLessThanOrEqualTo int? (default ()) - Returns any records where ProspectId is less than or equal to the specified value.
- updatedAt string? (default ()) - Returns any records where UpdatedAt is equal to the given datetime value.e.
- updatedAtAfter string? (default ()) - Returns any records where UpdatedAt is after the given datetime value, non-inclusive.
- updatedAtAfterOrEqualTo string? (default ()) - Returns any records where UpdatedAt is after or equal to the given datetime value.
- updatedAtBefore string? (default ()) - Returns any records where UpdatedAt is before the given datetime value, non-inclusive.
- updatedAtBeforeOrEqualTo string? (default ()) - Returns any records where UpdatedAt is before or equal to the given datetime value.
getVisitorPageViews
Retrieve a single visitor page view.
queryVisitorPageViews
function queryVisitorPageViews(string fields, string? orderBy, int? id, int? idGreaterThan, int? idGreaterThanOrEqualTo, int? idLessThan, int? idLessThanOrEqualTo, int? visitorId, int? visitorIdGreaterThan, int? visitorIdGreaterThanOrEqualTo, int? visitorIdLessThan, int? visitorIdLessThanOrEqualTo, int? visitId, int? visitIdGreaterThan, int? visitIdGreaterThanOrEqualTo, int? visitIdLessThan, int? visitIdLessThanOrEqualTo, string? createdAt, string? createdAtAfter, string? createdAtAfterOrEqualTo, string? createdAtBefore, string? createdAtBeforeOrEqualTo, string? updatedAt, string? updatedAtAfter, string? updatedAtAfterOrEqualTo, string? updatedAtBefore, string? updatedAtBeforeOrEqualTo) returns Record|error
Retrieve a collection of visitor page views.
Parameters
- fields string - Record fields to return
- orderBy string? (default ()) - When executing a query, the following fields can be specified in the orderBy parameter.
- id int? (default ()) - Returns any records where ID is equal to the given integer value.
- idGreaterThan int? (default ()) - Returns any records where ID is greater than the specified value, non-inclusive.
- idGreaterThanOrEqualTo int? (default ()) - Returns any records where ID is greater than or equal to the specified value.
- idLessThan int? (default ()) - Returns any records where ID is less than the specified value, non-inclusive.
- idLessThanOrEqualTo int? (default ()) - Returns any records where ID is less than or equal to the specified value.
- visitorId int? (default ()) - Returns any records where VisitorId is equal to the given integer value.
- visitorIdGreaterThan int? (default ()) - Returns any records where VisitorId is greater than the specified value, non-inclusive.
- visitorIdGreaterThanOrEqualTo int? (default ()) - Returns any records where VisitorId is greater than or equal to the specified value.
- visitorIdLessThan int? (default ()) - Returns any records where VisitorId is less than the specified value, non-inclusive.
- visitorIdLessThanOrEqualTo int? (default ()) - Returns any records where CreatedAt is before the given datetime value, non-inclusive.
- visitId int? (default ()) - Returns any visitor page view where VisitId is equal to the given integer value.
- visitIdGreaterThan int? (default ()) - Returns any visitor page view where VisitId is greater than the specified value, non-inclusive.
- visitIdGreaterThanOrEqualTo int? (default ()) - Returns any visitor page view where VisitId is greater than or equal to the specified value.
- visitIdLessThan int? (default ()) - Returns any visitor page view where VisitId is less than the specified value, non-inclusive.
- visitIdLessThanOrEqualTo int? (default ()) - Returns any visitor page view where VisitId is less than or equal to the specified value.
- createdAt string? (default ()) - Returns any records where CreatedAt is equal to the given datetime value.
- createdAtAfter string? (default ()) - Returns any records where CreatedAt is after the given datetime value, non-inclusive.
- createdAtAfterOrEqualTo string? (default ()) - Returns any records where CreatedAt is after or equal to the given datetime value.
- createdAtBefore string? (default ()) - Returns any records where CreatedAt is before the given datetime value, non-inclusive.
- createdAtBeforeOrEqualTo string? (default ()) - Returns any records where CreatedAt is before or equal to the given datetime value.
- updatedAt string? (default ()) - Returns any records where UpdatedAt is equal to the given datetime value.e.
- updatedAtAfter string? (default ()) - Returns any records where UpdatedAt is after the given datetime value, non-inclusive.
- updatedAtAfterOrEqualTo string? (default ()) - Returns any records where UpdatedAt is after or equal to the given datetime value.
- updatedAtBefore string? (default ()) - Returns any records where UpdatedAt is before the given datetime value, non-inclusive.
- updatedAtBeforeOrEqualTo string? (default ()) - Returns any records where UpdatedAt is before or equal to the given datetime value.
Records
salesforce.pardot: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
salesforce.pardot: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
salesforce.pardot: CustomFieldCreateRequest
Fields
- name string - Name of the object for identification in Pardot.
- fieldId string - User-defined ID of the object.
- 'type string - Possible values- Text, "Radio Button", Checkbox, Dropdown, Textarea, Multi-Select, Hidden, Number, Date, "CRM User"
- isRecordMultipleResponses boolean? - True if it records multiple responses, false otherwise.
- salesforceId string? - Salesforce Id of the object.
- isUseValues boolean? - True if values are used false otherwise.
- valuesPrefill string - Write-Only field on create operation. Possible values- Countries, "Country Codes", "US States", "US State Codes", "Canadian Provinces", "Canadian Province Codes", "US States and Canadian Provinces", "US State and Canadian Province Codes", "Japanese Prefectures", "Japanese Prefecture Codes"
- isRequired boolean? - True if the custom field is required.
salesforce.pardot: CustomFieldUpdateRequest
Fields
- name string - Name of the object for identification in Pardot.
- fieldId string - User-defined ID of the object.
- 'type string - Possible values- Text, "Radio Button", Checkbox, Dropdown, Textarea, Multi-Select, Hidden, Number, Date, "CRM User"
- isRecordMultipleResponses boolean? - True if it records multiple responses, false otherwise.
- salesforceId string? - Salesforce Id of the object.
- isUseValues boolean? - True if values are used false otherwise.
- valuesPrefill string? - Write-Only field on create operation. Possible values- Countries, "Country Codes", "US States", "US State Codes", "Canadian Provinces", "Canadian Province Codes", "US States and Canadian Provinces", "US State and Canadian Province Codes", "Japanese Prefectures", "Japanese Prefecture Codes"
- isRequired boolean? - True if the custom field is required.
salesforce.pardot: CustomRedirectsCreateRequest
Fields
- folderId int? - ID of the folder containing this object. Uses the asset type's uncategorized folder if not specified on create.
- name string - Name of the object for identification in Pardot.
- destinationUrl string - A fully qualified URL. The visitor who clicks the custom redirect will be directed to this location. E.g. https://example.com/content.html
- campaignId int - Pardot Campaign related to this object.
- trackerDomainId int? - ID of the tracker domain to use in the URL for this object. Uses the primary tracker domain for the account if not specified on create.
- vanityUrlPath string? - Vanity URL path (excluding protocol and host). Must be unique for each tracker domain. E.g. /my-link. The value assumes empty (/) if not specified on create.
- gaSource string? - Campaign source
- gaMedium string? - Campaign medium
- gaTerm string? - Campaign keyword
- gaContent string? - Campaign content
- gaCampaign string? - Campaign ID
salesforce.pardot: CustomRedirectsUpdateRequest
Fields
- folderId int? - ID of the folder containing this object. Uses the asset type's uncategorized folder if not specified on create.
- name string? - Name of the object for identification in Pardot.
- destinationUrl string? - A fully qualified URL. The visitor who clicks the custom redirect will be directed to this location. E.g. https://example.com/content.html
- campaignId int? - Pardot Campaign related to this object.
- trackerDomainId int? - ID of the tracker domain to use in the URL for this object. Uses the primary tracker domain for the account if not specified on create.
- vanityUrlPath string? - Vanity URL path (excluding protocol and host). Must be unique for each tracker domain. E.g. /my-link. The value assumes empty (/) if not specified on create.
- gaSource string? - Campaign source
- gaMedium string? - Campaign medium
- gaTerm string? - Campaign keyword
- gaContent string? - Campaign content
- gaCampaign string? - Campaign ID
salesforce.pardot: ExternalActivityCreateRequest
Fields
- extension string - Name of the extension for this activity.
- 'type string - The type of external activity for this record. It must be a value from one of the registered types in the account.
- email string - The email address of the prospect related to this external activity. If there are multiple prospects with the specified email address, the one with the most recent activity is selected.
- value string - Any string value related to this activity. This value isn’t checked and can be any value. The value can be 100 characters or less.
- activityDate string? - The date the external activity happened. It can be used by the user to backdate the activity. If not specified, then the current date is used. Must be in ISO8601 format with offset. Example- 2021-01-01T11:08:00+00:00
salesforce.pardot: FileCreateRequest
File create request
Fields
- name string - Name of the object for identification in Pardot. Uses the name of the file being uploaded if not specified on create.
- campaignId int - Pardot campaign related to this object. Uses null if not specified on create.
- trackerDomainId int - ID of the TrackerDomain to use in the URL for this object. Uses the primary tracker domain for the account if not specified on create.
- folderId int - ID of the folder containing this object. Uses the asset type's uncategorized folder if not specified on create.
- vanityUrlPath string - Vanity URL path (excluding protocol and host). Must be unique for each tracker domain. For example /my-link. The value assumes empty (/) if not specified on create.
salesforce.pardot: FileCreateRequestObject
Fields
- file string - File content
- input FileCreateRequest - File create request
salesforce.pardot: FileUpdateRequest
Fields
- name string? - Name of the object for identification in Pardot. Uses the name of the file being uploaded if not specified on create.
- campaignId int? - Pardot campaign related to this object. Uses null if not specified on create.
- trackerDomainId int? - ID of the TrackerDomain to use in the URL for this object. Uses the primary tracker domain for the account if not specified on create.
- folderId int? - ID of the folder containing this object. Uses the asset type's uncategorized folder if not specified on create.
- vanityUrlPath string? - Vanity URL path (excluding protocol and host). Must be unique for each tracker domain. For example /my-link. The value assumes empty (/) if not specified on create.
salesforce.pardot: LayoutTemplateCreateRequest
Fields
- name string - Name of the object for identification in Pardot.
- layoutContent string - HTML content of this layout template encoded as JSON string.
- formContent string? - HTML content encoded as JSON string that controls form display logic. Uses default values if not provided.
- siteSearchContent string? - HTML content encoded as JSON string that controls the site search content. Uses default values if not provided.
- isIncludeDefaultCss boolean? - True if not supplying custom CSS styling.
- folderId int? - ID of the folder containing this object. Uses the asset type's uncategorized folder if not specified on create.
salesforce.pardot: LayoutTemplateUpdateRequest
Fields
- name string? - Name of the object for identification in Pardot.
- layoutContent string? - HTML content of this layout template encoded as JSON string.
- formContent string? - HTML content encoded as JSON string that controls form display logic. Uses default values if not provided.
- siteSearchContent string? - HTML content encoded as JSON string that controls the site search content. Uses default values if not provided.
- isIncludeDefaultCss boolean? - True if not supplying custom CSS styling.
- folderId int? - ID of the folder containing this object. Uses the asset type's uncategorized folder if not specified on create.
salesforce.pardot: ListCreateRequest
Fields
- name string - Name of the object for identification in Pardot.
- description string? - Description of the list object.
- title string? - Description of the list object.
- isPublic boolean? - True if the list is public.
- campaignId int? - Pardot campaign related to this object. Uses null if not specified on create.
- folderId int? - ID of the folder containing this object. Uses the asset type's uncategorized folder if not specified on create.
salesforce.pardot: ListUpdateRequest
Fields
- name string? - Name of the object for identification in Pardot.
- description string? - Description of the list object.
- title string? - Description of the list object.
- isPublic boolean? - True if the list is public.
- campaignId int? - Pardot campaign related to this object. Uses null if not specified on create.
- folderId int? - ID of the folder containing this object. Uses the asset type's uncategorized folder if not specified on create.
salesforce.pardot: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
salesforce.pardot: Record
Import
import ballerinax/salesforce.pardot;
Metadata
Released date: over 1 year ago
Version: 1.3.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 2
Current verison: 1
Weekly downloads
Keywords
Marketing/Marketing Automation
Cost/Paid
Contributors
Dependencies