sakari
Module sakari
API
Definitions
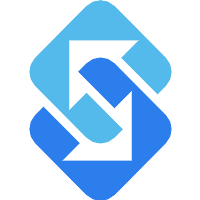
ballerinax/sakari Ballerina library
Overview
This is a generated connector from Sakari OpenAPI Specification.
Sakari provides an advanced platform to drive large scale customized SMS communication. To find out more about our product offering, please visit https://sakari.io.
This module supports Sakari REST API v1.0.1.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create Sakari developer account
- Obtain tokens - Login into https://hub.sakari.io and obtain tokens following this guide
Quickstart
To use the Sakari connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/sakari module into the Ballerina project.
import ballerinax/sakari;
Step 2: Create a new connector instance
You can now make the connection configuration using the obtained credentials.
sakari:ClientConfig configuration = { auth: { clientId: <>, clientSecret: <>, tokenUrl: "https://api.sakari.io/oauth2/token" } }; sakari:Client sakariClient = check new Client(configuration);
Step 3: Invoke the operation
- Now you can use the operations available within the connector. Following code demonstrates how to fetch all Sakari contacts.
public function main() returns error? { string accountId = <ACCOUNT_ID>; sakari:ContactsResponse contactsResponse = check sakariClient->fetchAllContacts(accountId); }
- Use
bal run
command to compile and run the Ballerina program.
Clients
sakari: Client
This is a generated connector from Sakari OpenAPI Specification. Sakari provides an advanced platform to drive large scale customized SMS communication To find out more about our product offering, please visit https://sakari.io.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Please create a Sakari account and obtain tokens following this guide
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.sakari.io/v1" - URL of the target service
authToken
function authToken(TokenRequest payload) returns TokenResponse|error
Get token for accessing APIs
Parameters
- payload TokenRequest - Request payload for obtaining token
Return Type
- TokenResponse|error - successful operation
fetchAllContacts
function fetchAllContacts(string accountId, int? offset, int? 'limit, string? firstName, string? lastName, string? mobile, string? email, string? tags) returns ContactsResponse|error
Fetch contacts
Parameters
- accountId string - Account to apply operations to
- offset int? (default ()) - Results to skip when paginating through a result set
- 'limit int? (default ()) - Maximum number of results to return
- firstName string? (default ()) - Filter by first name or part of
- lastName string? (default ()) - Filter by last name or part of
- mobile string? (default ()) - Filter by mobile or part of
- email string? (default ()) - Filter by email or part of
- tags string? (default ()) - Filter by tag(s)
Return Type
- ContactsResponse|error - successful operation
createContact
function createContact(string accountId, ContactRequest payload, string? mergeStrategy) returns InlineResponse201|error
Create contact
Parameters
- accountId string - Account to apply operations to
- payload ContactRequest - Create contact request payload
- mergeStrategy string? (default ()) - Determines how existing contacts with matching mobile numbers are treated
Return Type
- InlineResponse201|error - successful operation
fetchContact
function fetchContact(string accountId, string contactId) returns ContactResponse|error
Fetch contact by ID
Parameters
- accountId string - Account to apply operations to
- contactId string - ID of contact to return
Return Type
- ContactResponse|error - successful operation
contactsUpdate
function contactsUpdate(string accountId, string contactId) returns ContactResponse|error
Updates a contact
Return Type
- ContactResponse|error - successful operation
removeContact
function removeContact(string accountId, string contactId) returns InlineResponse200|error
Deletes a contact
Return Type
- InlineResponse200|error - successful operation
fetchAllMessages
function fetchAllMessages(string accountId, int? offset, int? 'limit, string? contactId, string? conversationId) returns MessagesResponse|error
Fetch messages
Parameters
- accountId string - Account to apply operations to
- offset int? (default ()) - Results to skip when paginating through a result set
- 'limit int? (default ()) - Maximum number of results to return
- contactId string? (default ()) - ID of contact
- conversationId string? (default ()) - ID of conversation
Return Type
- MessagesResponse|error - successful operation
sendMessage
function sendMessage(string accountId, SendMessagesRequest payload) returns SendMessagesResponse|error
Send Messages
Parameters
- accountId string - Account to apply operations to
- payload SendMessagesRequest - Send message request payload
Return Type
- SendMessagesResponse|error - successful operation
fetchMessages
function fetchMessages(string accountId, string messageId) returns MessageResponse|error
Fetch message by id
Parameters
- accountId string - Account to apply operations to
- messageId string - ID of message to return
Return Type
- MessageResponse|error - successful operation
fetchAllTemplates
function fetchAllTemplates(string accountId, int? offset, int? 'limit, string? name) returns TemplatesResponse|error
Fetch templates
Parameters
- accountId string - Account to apply operations to
- offset int? (default ()) - Results to skip when paginating through a result set
- 'limit int? (default ()) - Maximum number of results to return
- name string? (default ()) - Filter by name or part of
Return Type
- TemplatesResponse|error - successful operation
createTemplate
function createTemplate(string accountId, TemplateRequest payload) returns TemplatesResponse|error
Create template
Parameters
- accountId string - Account to apply operations to
- payload TemplateRequest - Create template request payload.
Return Type
- TemplatesResponse|error - successful operation
fetchTemplate
function fetchTemplate(string accountId, string templateId) returns TemplateResponse|error
Fetch template by ID
Parameters
- accountId string - Account to apply operations to
- templateId string - ID of template to return
Return Type
- TemplateResponse|error - successful operation
updateTemplate
function updateTemplate(string accountId, string templateId) returns TemplateResponse|error
Updates a template
Return Type
- TemplateResponse|error - successful operation
removeTemplate
function removeTemplate(string accountId, string templateId) returns InlineResponse200|error
Deletes a template
Parameters
- accountId string - Account to apply operations to
- templateId string - Template id to delete
Return Type
- InlineResponse200|error - successful operation
fetchAllCampaigns
function fetchAllCampaigns(string accountId, int? offset, int? 'limit, string? name) returns CampaignsResponse|error
Fetch campaigns
Parameters
- accountId string - Account to apply operations to
- offset int? (default ()) - Results to skip when paginating through a result set
- 'limit int? (default ()) - Maximum number of results to return
- name string? (default ()) - Filter by name or part of
Return Type
- CampaignsResponse|error - successful operation
createCampaigns
function createCampaigns(string accountId, CampaignRequest payload) returns CampaignResponse|error
Create campaign
Parameters
- accountId string - Account to apply operations to
- payload CampaignRequest - Create campaign request payload
Return Type
- CampaignResponse|error - successful operation
fetchCampaign
function fetchCampaign(string accountId, string campaignId) returns CampaignResponse|error
Fetch campaign by ID
Parameters
- accountId string - Account to apply operations to
- campaignId string - ID of campaign to return
Return Type
- CampaignResponse|error - successful operation
updateCampaign
function updateCampaign(string accountId, string campaignId) returns CampaignResponse|error
Updates a campaign
Return Type
- CampaignResponse|error - successful operation
removeCampaign
function removeCampaign(string accountId, string campaignId) returns InlineResponse200|error
Deletes a campaign
Parameters
- accountId string - Account to apply operations to
- campaignId string - Campaign id to delete
Return Type
- InlineResponse200|error - successful operation
fetchAllConversations
function fetchAllConversations(string accountId, int? offset, int? 'limit) returns ConversationsResponse|error
Fetch conversations
Parameters
- accountId string - Account to apply operations to
- offset int? (default ()) - Results to skip when paginating through a result set
- 'limit int? (default ()) - Maximum number of results to return
Return Type
- ConversationsResponse|error - successful operation
fetchConversation
function fetchConversation(string accountId, string conversationId) returns ConversationResponse|error
Fetch conversation by ID
Parameters
- accountId string - Account to apply operations to
- conversationId string - ID of template to return
Return Type
- ConversationResponse|error - successful operation
closeConversation
function closeConversation(string accountId, string conversationId) returns ConversationResponse|error
Closes a conversation
Parameters
- accountId string - Account to apply operations to
- conversationId string - ID of conversation
Return Type
- ConversationResponse|error - successful operation
shareFile
function shareFile(byte[] payload) returns ShareFileResponse|error
Share file - use to host a file and generate a short link to be used directly in a message or as a link to media for a MMS
Parameters
- payload byte[] - Binary form of the file
Return Type
- ShareFileResponse|error - successful operation
fetchAllWebhooks
function fetchAllWebhooks(string accountId) returns WebhooksResponse|error
Fetch active webhooks
Parameters
- accountId string - Account to apply operations to
Return Type
- WebhooksResponse|error - successful operation
subscribeWebhooks
function subscribeWebhooks(string accountId, AccountidWebhooksBody payload) returns WebhookResponse|error
Subscribe to message events
Parameters
- accountId string - Account to apply operations to
- payload AccountidWebhooksBody - Message event subscribe request payload
Return Type
- WebhookResponse|error - successful operation
unsubscribeWebhooks
Unsubscribe to message events
Records
sakari: AccountEvent
Fields
- 'type EventType? - Type of the event
- accountId AccountId? - Id of the account to apply operations to
sakari: AccountEventPayload
Fields
- balance decimal? -
sakari: AccountidWebhooksBody
Fields
- url string? -
- eventTypes string[]? -
sakari: AttributeFilter
Fields
- name string? -
- comparator string? -
- value string? -
sakari: Campaign
Fields
- id string? -
sakari: CampaignRequest
Fields
- trigger CampaignrequestTrigger? -
- filters CampaignrequestFilters? -
- template string? -
sakari: CampaignrequestFilters
Fields
- contacts string[]? -
- tags string[]? -
- attributes string[]? -
sakari: CampaignrequestTrigger
Fields
- code string? - Campaign type specifies how it sources contacts and what event triggers its execution Sort order
M
- ManualS
- ScheduledFU
- File Upload
sakari: CampaignResponse
Fields
- success boolean? -
- data Campaign? -
sakari: CampaignsResponse
Fields
- Fields Included from *PaginatedResponse
- success boolean
- pagination PaginatedresponsePagination
- error PaginatedresponseError
- anydata...
- data Campaign[]? -
sakari: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
sakari: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth OAuth2ClientCredentialsGrantConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
sakari: Contact
Fields
- Fields Included from *ContactRequest
- valid boolean? - Validity
- 'error Error? - Represent API error
- created Updated? - Represent resource update details
- updated Updated? - Represent resource update details
sakari: ContactIdentifiers
Contact information
Fields
- id string? - Contact information Id
- email string? - Email address
- firstName string? - First name
- lastName string? - Last name
- mobile ContactidentifiersMobile? - Mobile number
sakari: ContactidentifiersMobile
Mobile number
Fields
- country string? - Country code
- number string? - Mobile number
sakari: ContactRequest
Fields
- Fields Included from *ContactIdentifiers
- id string
- email string
- firstName string
- lastName string
- mobile ContactidentifiersMobile
- anydata...
- tags Tag[]? -
- attributes record {}? -
sakari: ContactResponse
Contact response
Fields
- success boolean? - Whether the request is success or not
- data Contact? -
sakari: ContactsResponse
Fields
- Fields Included from *PaginatedResponse
- success boolean
- pagination PaginatedresponsePagination
- error PaginatedresponseError
- anydata...
- data Contact[]? -
sakari: ContactUploadResponse
Contact upload response
Fields
- success boolean? - Whether the request is success
- data ContactuploadresponseData? -
sakari: ContactuploadresponseData
Fields
- contacts Contact[]? - Uploaded contacts
- errors Contact[]? - Contacts that failed validation
- submitted int? -
- inserted int? -
- updated int? -
- success int? -
sakari: Conversation
Fields
- id string? -
- contact ContactIdentifiers? - Contact information
- closed boolean? -
- lastMessage Message? -
- phoneNumber PhoneNumber? - Phone number resource
- unread string[]? -
- created Updated? - Represent resource update details
- updated Updated? - Represent resource update details
sakari: ConversationResponse
Fields
- success boolean? -
- data Conversation? -
sakari: ConversationsResponse
Fields
- Fields Included from *PaginatedResponse
- success boolean
- pagination PaginatedresponsePagination
- error PaginatedresponseError
- anydata...
- data Conversation[]? -
sakari: Error
Represent API error
Fields
- code string? - Error code
- description string? - Error message
sakari: ErrorResponse
Represent response error
Fields
- success boolean? - Whether the request is success
- 'error ErrorresponseError? - Represent error
sakari: ErrorresponseError
Represent error
Fields
- code string? - Error code
- message string? - Error message
sakari: Event
Fields
- eventId string? -
- 'type EventType? - Type of the event
- accountId AccountId? - Id of the account to apply operations to
sakari: InlineResponse200
Fields
- success boolean? -
sakari: Message
Fields
- id string? -
- contact ContactIdentifiers? - Contact information
- conversation MessageConversation? -
- message string? -
- template string? -
- outgoing boolean? -
- phoneNumber string? -
- price decimal? -
- segments decimal? -
- read boolean? -
- status string? -
- media MessageMedia[]? - List of media objects attached to message
- 'error Error? - Represent API error
- created Updated? - Represent resource update details
- updated Updated? - Represent resource update details
sakari: MessageConversation
Fields
- id string? -
sakari: MessageEventPayload
Fields
- contact ContactIdentifiers? - Contact information
- message string? -
- status string? -
- 'error string? -
sakari: MessageMedia
Fields
- url string? -
- 'type string? -
- filename string? -
sakari: MessageResponse
Fields
- success boolean? -
- data Message? -
sakari: MessagesResponse
Fields
- Fields Included from *PaginatedResponse
- success boolean
- pagination PaginatedresponsePagination
- error PaginatedresponseError
- anydata...
- data Message[]? -
sakari: OAuth2ClientCredentialsGrantConfig
OAuth2 Client Credentials Grant Configs
Fields
- Fields Included from *OAuth2ClientCredentialsGrantConfig
- tokenUrl string(default "https://api.sakari.io/oauth2/token") - Token URL
sakari: PaginatedResponse
Pagination response
Fields
- success boolean? - Success
- pagination PaginatedresponsePagination? -
- 'error PaginatedresponseError? - Represent an API pagination error
sakari: PaginatedresponseError
Represent an API pagination error
Fields
- code string? - Error code
- message string? - Error message
sakari: PaginatedresponsePagination
Fields
- totalCount int? - Total record count
- 'limit int? - Per page count
- offset int? - Page offset
sakari: PhoneNumber
Phone number resource
Fields
- country string? - Country code
- number string? - Phone number
- active boolean? - Whether phone number is active
sakari: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
sakari: SendMessagesRequest
Fields
- conversations string[]? - List of conversation ids to send messages to
- contacts ContactRequest[]? -
- filters SendmessagesrequestFilters? -
- template string? -
- 'type string? -
- media SendmessagesrequestMedia[]? - List of media objects to attach to message
- conversationStrategy string? -
sakari: SendmessagesrequestFilters
Fields
- tags string[]? -
- attributes record {}[]? -
sakari: SendmessagesrequestMedia
Fields
- url string? -
sakari: SendMessagesResponse
Fields
- data SendmessagesresponseData? -
sakari: SendmessagesresponseData
Fields
- messages Message[]? -
- jobId string? -
- queued int? -
- estimatedPrice decimal? -
sakari: ShareFileResponse
Fields
- success boolean? -
- data SharefileresponseData? -
sakari: SharefileresponseData
Fields
- link string? -
- expires string? -
sakari: Tag
Filter by tag(s)
Fields
- tag string? - Tag type
- visible boolean? - Whether visible
sakari: Template
Fields
- Fields Included from *TemplateRequest
- id string? -
sakari: TemplateRequest
Fields
- name string? -
- 'type string? -
- template string? -
sakari: TemplateResponse
Fields
- success boolean? -
- data Template? -
sakari: TemplatesResponse
Fields
- Fields Included from *PaginatedResponse
- success boolean
- pagination PaginatedresponsePagination
- error PaginatedresponseError
- anydata...
- data Template[]? -
sakari: TokenRequest
Get token for accessing the API
Fields
- grant_type string? - Grant type
- client_id string? - Client Id
- client_secret string? - Client secret
sakari: TokenResponse
Fields
- access_token string? - Access token
- token_type string? - Token type
sakari: ToolsSharefileBody
Fields
- media string? -
sakari: Updated
Represent resource update details
Fields
- at string? - Updated date time
- 'by UpdatedBy? - Updated by
sakari: UpdatedBy
Updated by
Fields
- id string? - Id of the update author
- firstName string? - First name of the update author
- lastName string? - Last name of the update author
sakari: Webhook
Fields
- url string? -
- eventTypes string[]? -
sakari: WebhookResponse
Fields
- success boolean? -
- data Webhook? -
sakari: WebhooksResponse
Fields
- Fields Included from *PaginatedResponse
- success boolean
- pagination PaginatedresponsePagination
- error PaginatedresponseError
- anydata...
- data Webhook[]? -
Union types
sakari: InlineResponse201
InlineResponse201
String types
Import
import ballerinax/sakari;
Metadata
Released date: over 1 year ago
Version: 1.5.0
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 0
Current verison: 0
Weekly downloads
Keywords
Communication/Call & SMS
Cost/Paid
Contributors
Dependencies