ronin
Module ronin
API
Definitions
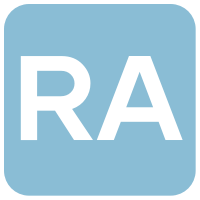
ballerinax/ronin Ballerina library
Overview
This is a generated connector for Ronin API v2 OpenAPI specification. Ronin is a full featured time tracking and invoicing application. It allows you to easily manage almost all aspects of your online projects, though it has a focus on the billing side.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Ronin account
- Obtain tokens by following this guide
Quickstart
To use the Ronin connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/ronin
module into the Ballerina project.
import ballerinax/ronin;
Step 2: Create a new connector instance
Create a ronin:ClientConfig
with the api_token
obtained, and initialize the connector with it.
ronin:ClientConfig clientConfig = { auth: { username: <api_token>, password: <api_token> } }; ronin:Client baseClient = check new Client(clientConfig, serviceUrl = "https://{yourdomain}.roninapp.com");
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to get all invoices using the connector.
Get all invoices
public function main() returns error? { ronin:Invoices response = check baseClient->listInvoices(); log:printInfo(response.toJsonString()); }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
ronin: Client
This is a generated connector for Ronin API v1 OpenAPI specification. Ronin is a full featured time tracking and invoicing application. It allows you to easily manage almost all aspects of your online projects, though it has a focus on the billing side.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Ronin account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string - URL of the target service
listClients
Get all clients
Parameters
- page int? (default ()) - Specifies the page of clients to retrieve.
- updatedSince string? (default ()) - Filter by update time for clients.
- sort string? (default ()) - You can get the most recently created clients by date, rather than the default sort which is based on client name.
createClient
function createClient(CreateClientRequest payload) returns ClientObject|error
Creates a new client. Clients represent companies, groups, organizations or other contact containers. All contacts must belong to a client. Clients are also the main containers for Projects and Invoices.
Parameters
- payload CreateClientRequest - The data required to create a client.
Return Type
- ClientObject|error - Created client
getClient
function getClient(string id) returns ClientObject|error
Get a client.
Parameters
- id string - The client ID
Return Type
- ClientObject|error - A client object
listContactsByClient
Get all contacts specific to a client
Parameters
- clientId string - The client ID
- page int? (default ()) - Specifies the page of contacts to retrieve.
- pageSize string? (default ()) - You may also use a different page_size parameter. The maximum allowed page_size is 100.
Return Type
createContact
function createContact(string clientId, CreateContactRequest payload) returns Contact|error
Creates a new contact. Contacts represent individuals belonging to Clients. In many cases, if a Contact is a Client, the Contact is where the email and other important information for the client whereas the Client is just a shell container.
Parameters
- clientId string - The client ID
- payload CreateContactRequest - The data required to create a contact.
listContacts
Get all contacts
Parameters
- page int? (default ()) - Specifies the page of contacts to retrieve.
- pageSize string? (default ()) - You may also use a different page_size parameter. The maximum allowed page_size is 100.
Return Type
getContact
Get a contact.
Parameters
- id string - The contact ID
listEstimates
Get all estimates
Parameters
- page int? (default ()) - Specifies the page of clients to retrieve.
Return Type
createEstimate
function createEstimate(CreateEstimateRequest payload) returns Estimate|error
Creates a new estimate. Estimates serve as proposals or quotes to be sent to Clients.
Parameters
- payload CreateEstimateRequest - The data required to create an estimate.
getEstimate
Get an estimate.
Parameters
- id string - The estimate ID
listInvoices
Get all invoices
Parameters
- page int? (default ()) - Specifies the page of invoices to retrieve.
- updatedSince string? (default ()) - Filter by update time for invoices.
Return Type
createInvoice
function createInvoice(CreateInvoiceRequest payload) returns Invoice|error
Creates a new invoice. Ronin provides programmatic access to invoices and invoice items.
Parameters
- payload CreateInvoiceRequest - The data required to create an invoice.
getInvoice
Get a invoice.
Parameters
- id string - The invoice ID
listProjects
Get all projects
Parameters
- page int? (default ()) - Specifies the page of projects to retrieve.
- filter string? (default ()) - By default, this end point will only return active and billable projects. To also retrieve closed projects, use the filter parameter with value all.
Return Type
createProject
function createProject(CreateProjectRequest payload) returns Project|error
Creates a new project. Projects represent hubs of work, tasks, logged hours, expenses, and invoices related to a Client.
Parameters
- payload CreateProjectRequest - The data required to create a project.
getProject
Get a project.
Parameters
- id string - The project ID
listTasks
Get all tasks
createTask
function createTask(CreateTaskRequest payload) returns Task|error
Creates a new task. Tasks are TODO items that can be added to projects and assigned to staff members.
Parameters
- payload CreateTaskRequest - The data required to create a task.
getTask
Get a task.
Parameters
- id string - The task ID
updateTask
function updateTask(string id, UpdateTaskRequest payload) returns Response|error
Updates a new task. Tasks are TODO items that can be added to projects and assigned to staff members.
Records
ronin: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
ronin: ClientObject
Clients represent companies, groups, organizations or other contact containers. All contacts must belong to a client. Clients are also the main containers for Projects and Invoices.
Fields
- number string? - The unique number for the client
- name string? - The name of the client
- city string? - The city of the client
- address string? - The address of the client
- zip string? - The zip code of the client
- country string? - The country of the client
- id int? - Client ID
- website string? - The website of the client
- address_2 string? - The address2 of the client
- description string? - The description about the client
- state string? - The state of the client
ronin: ClientRequest
Clients represent companies, groups, organizations or other contact containers. All contacts must belong to a client. Clients are also the main containers for Projects and Invoices.
Fields
- number string? - The unique number for the client
- name string - The name of the client
- city string? - The city of the client
- address string? - The address of the client
- zip string? - The zip code of the client
- country string? - The country of the client
- website string? - The website of the client
- address_2 string? - The address2 of the client
- description string? - The description about the client
- state string? - The state of the client
ronin: Clients
An object with a property clients which is an array of Client objects.
Fields
- page_count int? - The page count
- page_size int? - The page size
- total_count int? - The total count
- clients ClientObject[]? - An array of Client objects.
ronin: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth CredentialsConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
ronin: Contact
Contacts represent individuals belonging to Clients. In many cases, if a Contact is a Client, the Contact is where the email and other important information for the client whereas the Client is just a shell container.
Fields
- name string - The name of the contact
- ext string? - The ext number of the contact
- title string? - The title of the contact
- id int? - Contact ID
- client_id int? - The ID of the client which the contact belongs
- mobile string? - The mobile number of the contact
- phone string? - The phone number of the contact
- avatar string? - The avatar
- email string - The e-mail address of the contact
ronin: ContactRequest
Contacts represent individuals belonging to Clients. In many cases, if a Contact is a Client, the Contact is where the email and other important information for the client whereas the Client is just a shell container.
Fields
- name string - The name of the contact
- ext string? - The ext number of the contact
- title string? - The title of the contact
- client_id int? - The ID of the client which the contact belongs
- mobile string? - The mobile number of the contact
- phone string? - The phone number of the contact
- avatar string? - The avatar
- email string - The e-mail address of the contact
ronin: Contacts
An object with a property contacts which is an array of Contact objects.
Fields
- page_count int? - The page count
- page_size int? - The page size
- total_count int? - The total count
- contacts Contact[]? - An array of Contact objects.
ronin: CreateClientRequest
The data required to create a client.
Fields
- 'client ClientRequest? - Clients represent companies, groups, organizations or other contact containers. All contacts must belong to a client. Clients are also the main containers for Projects and Invoices.
ronin: CreateContactRequest
The data required to create a contact.
Fields
- client_user ContactRequest? - Contacts represent individuals belonging to Clients. In many cases, if a Contact is a Client, the Contact is where the email and other important information for the client whereas the Client is just a shell container.
ronin: CreateEstimateRequest
The data required to create an estimate.
Fields
- estimate EstimateRequest? - Estimates serve as proposals or quotes to be sent to Clients.
ronin: CreateInvoiceRequest
The data required to create an invoice.
Fields
- invoice InvoiceRequest? - Ronin provides programmatic access to invoices and invoice items.
ronin: CreateProjectRequest
The data required to create a project.
Fields
- project ProjectRequest? - Projects represent hubs of work, tasks, logged hours, expenses, and invoices related to a Client. budget_type can be 0 (total budgeted hours) or 1 (total budgeted amount). project_type can be 0 (hourly rate project) or 2 (fixed amount project). Rate the total cost of the project in the latter case.
ronin: CreateTaskRequest
The data required to create a task.
Fields
- task TaskRequest? - Tasks are TODO items that can be added to projects and assigned to staff members.
ronin: Estimate
Estimates serve as proposals or quotes to be sent to Clients.
Fields
- tax decimal? - Tax
- number string? - Invoice number
- total_cost decimal? - Total cost
- subtotal decimal? - Subtotal
- title string? - Title
- tax_label string? - Tax label
- compound_tax boolean? - Compound tax
- id int? - Invoice ID
- date string - Date
- client_id int? - Client ID
- note string? - Note
- estimate_items EstimateItem[]? - Estimate items
- tax2_label string? - Secondary tax label
- summary string? - Summary
- tax2 decimal? - Secondary tax
- status decimal? - Status
- currency_code string - Currency code
- tax3_label string? - Tertiary tax label
- tax3 decimal? - Tertiary tax
- estimate_items_attributes EstimateItemAttribute[]? - Estimate items attributes. estimate_items_attributes.title, estimate_items_attributes.quantity, estimate_items_attributes.price must be provided if any items are added.
ronin: EstimateItem
Estimate item
Fields
- price decimal? - Price
- title string? - Title
- quantity int? - Quantity
- taxable boolean? - Taxable
- id int? - Estimate Item ID
ronin: EstimateItemAttribute
Estimate item attribute
Fields
- title string? - Title
- quantity int? - Quantity
- price decimal? - Price
ronin: EstimateRequest
Estimates serve as proposals or quotes to be sent to Clients.
Fields
- tax decimal? - Tax
- number string? - Invoice number
- total_cost decimal? - Total cost
- subtotal decimal? - Subtotal
- title string? - Title
- tax_label string? - Tax label
- compound_tax boolean? - Compound tax
- date string - Date
- client_id int? - Client ID
- note string? - Note
- estimate_items EstimateItem[]? - Estimate items
- tax2_label string? - Secondary tax label
- summary string? - Summary
- tax2 decimal? - Secondary tax
- status decimal? - Status
- currency_code string - Currency code
- tax3_label string? - Tertiary tax label
- tax3 decimal? - Tertiary tax
- estimate_items_attributes EstimateItemAttribute[]? - Estimate items attributes. estimate_items_attributes.title, estimate_items_attributes.quantity, estimate_items_attributes.price must be provided if any items are added.
ronin: Estimates
An object with a property estimates which is an array of Estimate objects.
Fields
- page_count int? - The page count
- page_size int? - The page size
- total_count int? - The total count
- Estimates Estimate[]? - An array of Estimate objects.
ronin: Invoice
Ronin provides programmatic access to invoices and invoice items.
Fields
- tax decimal? - Tax
- number string? - Invoice number
- total_cost decimal? - Total cost
- balance decimal? - Balance
- subtotal decimal? - Sub total
- title string? - Title
- tax_label string? - Tax label
- compound_tax boolean? - Compound tax
- id int? - Invoice ID
- date string - Date
- client_id int? - Client ID
- note string? - A note about payment
- due_date string - Due date
- invoice_items InvoiceItem[]? - Invoice items
- tax2_label string? - Secondary tax label
- summary string? - Summary
- total_payments decimal? - Total payments
- tax2 decimal? - Secondary tax
- status decimal? - Status
- po string? - PO
- currency_code string - Currency code
- payments Payment[]? - Payments
- tax3_label string? - Tertiary tax label
- tax3 decimal? - Tertiary tax
- invoice_items_attributes InvoiceItemAttribute[]? - Invoice items attributes. invoice_item_attributes.title, invoice_item_attributes.quantity, invoice_item_attributes.price must be provided if any items are added.
ronin: InvoiceItem
Invoice item
Fields
- title string? - Title
- quantity int? - Quantity
- taxable boolean? - Taxable
- id int? - Invoice Item ID
ronin: InvoiceItemAttribute
Invoice item attribute
Fields
- title string? - Title
- quantity int? - Quantity
- price decimal? - Price
ronin: InvoiceRequest
Ronin provides programmatic access to invoices and invoice items.
Fields
- tax decimal? - Tax
- number string? - Invoice number
- total_cost decimal? - Total cost
- balance decimal? - Balance
- subtotal decimal? - Sub total
- title string? - Title
- tax_label string? - Tax label
- compound_tax boolean? - Compound tax
- date string - Date
- client_id int? - Client ID
- note string? - A note about payment
- due_date string - Due date
- invoice_items InvoiceItem[]? - Invoice items
- tax2_label string? - Secondary tax label
- summary string? - Summary
- total_payments decimal? - Total payments
- tax2 decimal? - Secondary tax
- status decimal? - Status
- po string? - PO
- currency_code string - Currency code
- payments Payment[]? - Payments
- tax3_label string? - Tertiary tax label
- tax3 decimal? - Tertiary tax
- invoice_items_attributes InvoiceItemAttribute[]? - Invoice items attributes. invoice_item_attributes.title, invoice_item_attributes.quantity, invoice_item_attributes.price must be provided if any items are added.
ronin: Invoices
An object with a property invoices which is an array of Invoice objects.
Fields
- page_count int? - The page count
- page_size int? - The page size
- total_count int? - The total count
- invoices Invoice[]? - An array of Invoice objects.
ronin: Payment
Payment details
Fields
- received_on string? - Payment received on
- updated_at string? - Payment updated at
- invoice_id int? - Invoice ID
- amount decimal? - Payment amount
- id int? - Payment ID
- note string? - Payment note
ronin: Project
Projects represent hubs of work, tasks, logged hours, expenses, and invoices related to a Client. budget_type can be 0 (total budgeted hours) or 1 (total budgeted amount). project_type can be 0 (hourly rate project) or 2 (fixed amount project). Rate the total cost of the project in the latter case.
Fields
- number string? - The unique identifier for the project
- name string - The name for the project
- budget_type decimal? - The budget type of the project
- 'client ClientObject? - Clients represent companies, groups, organizations or other contact containers. All contacts must belong to a client. Clients are also the main containers for Projects and Invoices.
- project_type decimal? - The type of the project
- worked_hours decimal? - Worked hours
- rate decimal - The billing rate for the project
- unbilled_hours decimal? - Unbilled hours
- id int? - Project ID
- cost decimal? - Cost
- description string? - The description about the project
- status decimal? - The status of the project
- end_date string? - The end date of the project
- currency_code string? - The currency code of the project
- budget decimal? - Budget
ronin: ProjectRequest
Projects represent hubs of work, tasks, logged hours, expenses, and invoices related to a Client. budget_type can be 0 (total budgeted hours) or 1 (total budgeted amount). project_type can be 0 (hourly rate project) or 2 (fixed amount project). Rate the total cost of the project in the latter case.
Fields
- number string? - The unique identifier for the project
- name string - The name for the project
- budget_type decimal? - The budget type of the project
- 'client ClientObject? - Clients represent companies, groups, organizations or other contact containers. All contacts must belong to a client. Clients are also the main containers for Projects and Invoices.
- project_type decimal? - The type of the project
- worked_hours decimal? - Worked hours
- rate decimal - The billing rate for the project
- unbilled_hours decimal? - Unbilled hours
- cost decimal? - Cost
- description string? - The description about the project
- status decimal? - The status of the project
- end_date string? - The end date of the project
- currency_code string? - The currency code of the project
- budget decimal? - Budget
ronin: Projects
An object with a property projects which is an array of Project objects.
Fields
- page_count int? - The page count
- page_size int? - The page size
- total_count int? - The total count
- projects Project[]? - An array of Project objects.
ronin: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
ronin: Task
Tasks are TODO items that can be added to projects and assigned to staff members.
Fields
- completed_at string? - Completed at
- created_at string? - Created at
- title string - The title of the task
- assignee_id int? - The assignee ID of the task
- updated_at string? - Updated at
- project_id int? - The project ID of the task
- id int? - The task ID
- user_id int? - The user ID
- client_id int? - The client ID of the task
- due_date string? - The due date of the task
- complete boolean? - The boolean flag to indicate that, task is completed or not
- description string? - The description
ronin: TaskRequest
Tasks are TODO items that can be added to projects and assigned to staff members.
Fields
- completed_at string? - Completed at
- created_at string? - Created at
- title string - The title of the task
- assignee_id int? - The assignee ID of the task
- updated_at string? - Updated at
- project_id int? - The project ID of the task
- user_id int? - The user ID
- client_id int? - The client ID of the task
- due_date string? - The due date of the task
- complete boolean? - The boolean flag to indicate that, task is completed or not
- description string? - The description
ronin: Tasks
An object with a property tasks which is an array of Task objects.
Fields
- page_count int? - The page count
- page_size int? - The page size
- total_count int? - The total count
- tasks Task[]? - An array of Task objects.
ronin: UpdateTaskRequest
The data required to update a task.
Fields
- task TaskRequest? - Tasks are TODO items that can be added to projects and assigned to staff members.
Import
import ballerinax/ronin;
Metadata
Released date: 10 months ago
Version: 1.5.2
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
Pull count
Total: 1734
Current verison: 2
Weekly downloads
Keywords
Finance/Billing
Cost/Paid
Contributors
Dependencies