redis
Module redis
API
Declarations
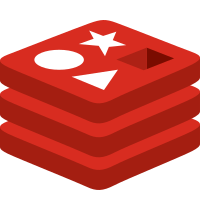
ballerinax/redis Ballerina library
Overview
Redis is an open-source, in-memory data structure store that can be used as a database, cache, and message broker. It supports various data structures such as strings, hashes, lists, sets, and more.
The ballerinax/redis
package offers APIs to connect to Redis servers and to manipulate key-value data, providing
functionality to utilize Redis both as a database and cache. The current connector is compatible Redis server
versions up to 7.2.x.
Setup guide
To use the Redis connector, you need to have a Redis server running and accessible. For that, you can either install Redis locally or use a cloud-based Redis service.
Setting up a Redis server locally
Step 1: Install Redis server
-
Download and install Redis from the official website.
-
Follow the installation instructions based on your operating system.
Step 2: Start Redis server
To start the Redis server, open a terminal and execute the following command.
redis-server
Step 3: Verify Redis connectivity
After starting the Redis server, you can verify the connectivity using the Redis CLI. Open a new terminal and execute the following command.
redis-cli ping
If the server is running, you will receive a response of "PONG"
.
Setting up a cloud-based Redis service
Several cloud providers offer managed Redis services. Some of the popular ones are:
- Redis Labs: Redis Labs offers Redis Enterprise Cloud, a fully-managed Redis service in the cloud.
- Amazon ElastiCache: Amazon Web Services provides ElastiCache, a managed Redis service for deploying, operating, and scaling Redis in the AWS Cloud.
- Google Cloud Memorystore: Google Cloud offers Memorystore for Redis, a fully-managed Redis service on Google Cloud Platform.
- Microsoft Azure Cache for Redis: Azure Cache for Redis is a fully-managed Redis service provided by Microsoft Azure.
Choose the hosting solution that best fits your requirements and proceed with its setup instructions.
Quickstart
To use the redis
connector in your Ballerina application, follow the steps below:
Step 1: Import the module
Import the redis
module.
import ballerinax/redis;
Step 2: Instantiate a new connector
Create a redis:ConnectionConfig
instance by giving the Redis server configuration and
initialize the Ballerina Redis client using it.
redis:Client redis = check new ( connection = { host: "localhost", port: 6379 } );
Step 3: Invoke the connector operation
Now, you can use the available connector operations to interact with Redis.
Set a key-value pair
check redis->set("key", "value");
Get value by key
string value = check redis->get("key");
Step 4: Run the Ballerina application
Save the changes and run the Ballerina application using the following command.
bal run
Examples
The Redis connector provides practical examples illustrating usage in various scenarios. Explore these examples covering common Redis operations.
-
Cache management - Implement caching using Redis to improve application performance.
-
Session management - Use Redis to manage user sessions efficiently.
-
Rate limiting - Implement rate limiting for API endpoints using Redis.
Clients
redis: Client
Ballerina Redis connector provides the capability to access Redis cache. This connector lets you to perform operations to access and manipulate key-value data stored in a Redis database.
Constructor
Initialize the Redis client.
init (*ConnectionConfig config)
- config *ConnectionConfig - configuration for the connector
append
Append a value to a key.
bitCount
Count set bits in a string.
Parameters
- key string - Key referring to a value
bitOpAnd
Perform bitwise AND between strings.
Parameters
- destination string - Result key of the operation
- keys string[] - Input keys to perform AND between
Return Type
bitOpOr
Perform bitwise OR between strings.
Parameters
- destination string - Result key of the operation
- keys string[] - Input keys to perform OR between
Return Type
bitOpNot
Perform bitwise NOT on a string.
Return Type
bitOpXor
Perform bitwise XOR between strings.
Parameters
- destination string - Result key of the operation
- keys string[] - Input keys to perform XOR between
Return Type
decr
Decrement integer value of a key by one.
Parameters
- key string - Key referring to a value
decrBy
Decrement integer value of a key by the given number.
getBit
Returns bit value at offset in the string value stored at key.
getRange
Get substring of string stored at a key.
Parameters
- key string - Key referring to a value
- startPos int - Starting point of substring
- end int - End point of substring
getSet
Set string value of key and return its existing value.
Return Type
get
Get value of key.
Parameters
- key string - Key referring to a value
Return Type
incr
Increment integer value of a key by one.
Parameters
- key string - Key referring to a value
incrBy
Increment integer value of key by the given amount.
incrByFloat
Increment integer value of key by the given float.
mGet
Get values of all given keys.
Parameters
- keys string[] - Keys of which values need to be retrieved
mSet
Set multiple keys to multiple values.
Parameters
- keyValueMap map<any> - Map of key-value pairs to be set
mSetNx
Set multiple keys to multiple values, only if none of the keys exist.
Parameters
- keyValueMap map<any> - Map of key-value pairs to be set
pSetEx
Set value and expiration in milliseconds of a key.
Parameters
- key string - Key referring to a value
- value string - Value to be set
- expirationTime int - Expiration time in milli seconds
set
Set the value of a key.
setBit
Sets or clears the bit at offset in the string value stored at key.
Parameters
- key string - Key referring to a value
- value int - Value to be set
- offset int - Offset at which the value should be set
setEx
Set the value and expiration of a key.
Parameters
- key string - Key referring to a value
- value string - Value to be set
- expirationTime int - Expiration time to be set, in seconds
setNx
Set value of a key, only if key does not exist.
setRange
Overwrite part of string at key starting at the specified offset.
Parameters
- key string - Key referring to a value
- offset int - Offset at which the value should be set
- value string - Value to be set
strLen
Get length of value stored in a key.
Parameters
- key string - Key referring to a value
Return Type
lPush
Prepend one or multiple values to list.
lPop
Remove and get the first element in a list.
Parameters
- key string - Key referring to a value
Return Type
lPushX
Prepend one or multiple values to a list, only if the list exists.
bLPop
Remove and get the first element in a list, or block until one is available.
Return Type
bRPop
Remove and get the last element in a list, or block until one is available.
Return Type
lIndex
Get an element from list by its index.
Parameters
- key string - Key referring to a value
- index int - Index from which the element should be retrieved
lInsert
Insert an element before or after another element in a list.
Parameters
- key string - Key referring to a value
- before boolean - Boolean value representing Whether element should be inserted before or after the pivot
- pivot string - Pivot position
- value string - Value to insert
Return Type
lLen
Get length of a list.
Parameters
- key string - Key referring to a value
lRange
Get a range of elements from a list.
Parameters
- key string - Key referring to a value
- startPos int - Begining index of the range
- stopPos int - Last index of the range
Return Type
lRem
Remove elements from list.
Parameters
- key string - Key referring to a value
- count int - Number of elements to be removed
- value string - Value which the elements to be removed should be equal to
lSet
Set the value of an element in a list by its index.
Parameters
- key string - Key of the list
- index int - Index of the element of which the value needs to be set
- value string - Value to be set
Return Type
lTrim
Trim list to the specified range.
Parameters
- key string - Key of the list
- startPos int - Starting index of the range
- stopPos int - End index of the range
rPop
Remove and get the last element in a list.
Parameters
- key string - Key of the list
Return Type
rPopLPush
Remove the last element in a list, append it to another list and return it.
rPush
Append one or multiple values to a list.
Return Type
rPushX
Append one or multiple values to a list, only if the list exists.
Return Type
sAdd
Add one or more members to a set.
Return Type
sCard
Get the number of members in a set
Parameters
- key string - Key of the set
Return Type
sDiff
Return set resulting from the difference between the first set and all the successive sets
Parameters
- keys string[] - The keys of the sets
Return Type
sDiffStore
Obtain the set resulting from the difference between the first set and all the successive. sets and store at the provided destination.
Parameters
- destination string - Destination key of the resulting set
- keys string[] - Keys of the sets to find the difference of
sInter
Return the intersection of the provided sets.
Parameters
- keys string[] - Keys of the sets to be intersected
sInterStore
Obtain the intersection of the provided sets and store at the provided destination.
Parameters
- destination string - Destination key of the resulting set
- keys string[] - Keys of the sets to be intersected
sIsMember
Determine if a given value is a member of a set.
Return Type
sMembers
Get all members in a set.
Parameters
- key string - Key of the set
sMove
Move a member from one set to another.
Parameters
- src string - Source key
- destination string - Destination key
- member string - Member to be moved
Return Type
sPop
Remove and return a random member from a set.
Return Type
sRandMember
Get one or multiple random members from a set.
Return Type
sRem
Remove one or more members from a set.
Return Type
sUnion
Return the union of multiple sets.
Parameters
- keys string[] - Array of keys of sets
sUnionStore
Return the union of multiple sets.
Parameters
- destination string - Destination key of the resulting set
- keys string[] - Array of keys of sets
zAdd
Add one or more members to a sorted set, or update its score if it already exist.
Parameters
- key string - Key of the sorted set
- memberScoreMap map<any> - Map of members and corresponding scores
Return Type
zCard
Get the number of members in a sorted set.
Parameters
- key string - Key of the sorted set
Return Type
zCount
Count the members in a sorted set with scores within the given range.
Parameters
- key string - Key of the sorted set
- min float - Minimum score of the range
- max float - Maximum score of the range
Return Type
zIncrBy
Increment the score of a member in a sorted set.
Parameters
- key string - Key of the sorted set
- amount float - Amount to increment
- member string - Member whose score to be incremented
zInterStore
Intersect multiple sorted sets and store the resulting sorted set in a new key.
Parameters
- destination string - Destination key of the resulting sorted set
- keys string[] - Keys of the sorted sets to be intersected
Return Type
zLexCount
Count the members in a sorted set within the given lexicographical range.
Parameters
- key string - Key of the sorted set
- min string - Minimum lexicographical value of the range
- max string - Maximum lexicographical value of the range
Return Type
zRange
Return a range of members in a sorted set, by index.
Parameters
- key string - Key of the sorted set
- min int - Minimum index of the range
- max int - Maximum index of the range
Return Type
zRangeByLex
Return a range of members in a sorted set, by lexicographical range from lowest to highest.
Parameters
- key string - Key of the sorted set
- min string - Minimum lexicographical value of the range
- max string - Maximum lexicographical value of the range
Return Type
zRevRangeByLex
Return a range of members in a sorted set, by lexicographical range ordered from highest to lowest.
Parameters
- key string - Key of the sorted set
- min string - Lexicographical value of the range
- max string - Maximum lexicographical value of the range
Return Type
zRangeByScore
Return a range of members in a sorted set, by score from lowest to highest.
Parameters
- key string - Key of sorted set
- min float - Minimum score of range
- max float - Maximum score of range
Return Type
zRank
Determine index of a member in a sorted set.
Parameters
- key string - Key of the sorted set
- member string - Member of which the index needs to be obtained
zRem
Remove one or more members from a sorted set
Return Type
zRemRangeByLex
Remove all members in a sorted set between the given lexicographical range.
Parameters
- key string - Key of the sorted set
- min string - Minimum lexicographical value of the range
- max string - Maximum lexicographical value of the range
Return Type
zRemRangeByRank
Remove all members in a sorted set within the given indices.
Parameters
- key string - Key of the sorted set
- min int - Minimum index of the range
- max int - Maximum index of the range
Return Type
zRemRangeByScore
Remove all members in a sorted set within the given scores.
Parameters
- key string - Key of the sorted set
- min float - Minimum score of the range
- max float - Maximum score of the range
Return Type
zRevRange
Return a range of members in a sorted set, by index, ordered highest to lowest.
Parameters
- key string - Key of the sorted set
- min int - Minimum index of the range
- max int - Maximum index of the range
Return Type
zRevRangeByScore
Return a range of members in a sorted set, by score from highest to lowest.
Parameters
- key string - Key of the sorted set
- min float - Minimum score of the range
- max float - Maximum score of the range
Return Type
zRevRank
Determine the index of a member in a sorted set
Parameters
- key string - Key of the sorted set
- member string - Member of which the index needs to be obtained
zScore
Determine the score of a member in a sorted set
Parameters
- key string - Key of the sorted set
- member string - Member of which the score needs to be obtained
zUnionStore
Return the union of multiple sorted sets
Parameters
- destination string - Destination key of the resulting set
- keys string[] - Array of keys of sorted sets
Return Type
hDel
Delete one or more hash fields.
Return Type
hExists
Determine if a hash field exists.
Return Type
hGet
Get the value of a hash field.
hGetAll
Get the all values of a hash.
Parameters
- key string - Key of the hash
hIncrBy
Increment the integer value of a hash field by the given number.
hIncrByFloat
Increment the float value of a hash field by the given number.
hKeys
Get all the fields in a hash.
Parameters
- key string - Key of the hash
hLen
Get the number of fields in a hash.
Parameters
- key string - Key of the hash
hMGet
Get the values of all the given hash fields.
hMSet
Set multiple hash fields to multiple values.
Return Type
hSet
Set the string value of a hash field.
Return Type
hSetNx
Set the string value of a hash field, only if the field does not exist.
Return Type
hStrLen
Get the string length of the field value in a hash.
Return Type
hVals
Get all the values in a hash.
Parameters
- key string - Key of the hash
Return Type
del
Delete one or more keys.
Parameters
- keys string[] - Key to be deleted
exists
Determine how many keys exist.
Parameters
- keys string[] - Keys of which existence to be found out
expire
Set a key's time to live in seconds.
Return Type
keys
Find all keys matching the given pattern.
Parameters
- pattern string - Pattern to match
Return Type
move
Move a key to another database.
Return Type
persist
Remove the expiration from a key.
Parameters
- key string - Key of which expiry time should be removed
Return Type
pExpire
Set a key's time to live in milliseconds.
Parameters
- key string - Key of which expiry time should be removed
- expirationTime int - Expiry time in milli seconds
Return Type
pTtl
Get the time to live for a key in milliseconds.
Parameters
- key string - Key of which time-to-live should be obtained
randomKey
Return a random key from the keyspace.
Return Type
rename
Rename a key.
Return Type
renameNx
Rename a key, only if the new key does not exist.
Return Type
sort
Sort elements in a list, set or sorted set.
Parameters
- key string - Key of the data type to be sorted
Return Type
ttl
Get the time to live for a key.
Parameters
- key string - Key of which the time to live needs to be obtained
Return Type
redisType
Determine the type stored at key.
Parameters
- key string - Key of which the type needs to be obtained
clusterInfo
Retrieve information and statistics about the cluster observed by the current node.
This command is exclusively available in cluster mode. If the connection is in a non-clustered mode,
the API will return a redis:Error
. Other errors will also be appropriately handled.
Return Type
ping
Ping the server.
auth
Authenticate to the server.
Parameters
- password string - Password to authenticate
Return Type
echo
Echo the given string.
Parameters
- message string - Message to be echo-ed
Return Type
close
function close() returns Error?
Close the connection.
Return Type
- Error? -
nil
if the operation was successful or anredis:Error
if an error occurs
Enums
redis: SslVerifyMode
Represents the SSL/TLS verification mode.
Members
Records
redis: CertKey
Represents a combination of certificate, private key, and private key password if encrypted.
Fields
- certFile string - File containing the certificate
- keyFile string - File containing the private key in PKCS8 format
- keyPassword? string - Password of the private key if it is encrypted
redis: ConnectionConfig
The client endpoint configuration for Redis.
Fields
- connection ConnectionUri|ConnectionParams(default "redis://localhost:6379") - Connection configurations of the Redis server. This can be either a single URI or a set of parameters
- connectionPooling boolean(default false) - Flag to indicate whether connection pooling is enabled
- isClusterConnection boolean(default false) - Flag to indicate whether the connection is a cluster connection
- secureSocket? SecureSocket - Configurations related to SSL/TLS encryption
redis: ConnectionParams
The connection parameters based configurations.
Fields
- host string(default "localhost") - Host address of the Redis database
- port int(default 6379) - Port of the Redis database
- username? string - The username for the Redis database
- password? string - The password for the Redis database
- options Options(default {}) - Other connection options of the connection configuration
redis: Options
Connection options for Redis client endpoint.
Fields
- clientName? string - Name of the client
- database int(default 0) - Database index which the client should interact with. Not applicable for cluster connections
- connectionTimeout int(default 60) - Connection timeout in seconds
redis: SecureSocket
Configurations for secure communication with the Redis server.
Fields
- cert? TrustStore|string - Configurations associated with
crypto:TrustStore
or single certificate file that the client trusts
- protocols? string[] - List of protocols used for the connection established to Redis Server, such as TLSv1.2, TLSv1.1, TLSv1.
- ciphers? string[] - List of ciphers to be used for SSL connections
- verifyMode SslVerifyMode(default FULL) - The SSL/TLS verification mode. This can be either NONE, CA, or FULL.
- startTls boolean(default false) - Whether StartTLS is enabled
Errors
redis: Error
Represents a redis generic error
String types
redis: ConnectionUri
ConnectionUri
The redis Connection URI based configurations. This can become useful when working with managed Redis databases, where the cloud provider usually provides a connection URI.
Import
import ballerinax/redis;
Metadata
Released date: 4 months ago
Version: 3.1.0
License: Apache-2.0
Compatibility
Platform: java21
Ballerina version: 2201.11.0
GraalVM compatible: Yes
Pull count
Total: 35637
Current verison: 4090
Weekly downloads
Keywords
IT Operations/Databases
Cost/Freemium
Contributors