recurly
Module recurly
API
Definitions
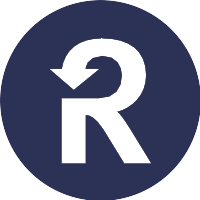
ballerinax/recurly Ballerina library
Overview
This is a generated connector for Recurly API V3 OpenAPI specification. Recurly is a subscription management platform delivering a competitive advantage for leading brands worldwide. Trusted by Sling TV, BarkBox, Asana, and thousands more to drive recurring revenue. Onboard and manage customers through Recurly API.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create an Recurly account
- Obtain tokens by following this guide
Quickstart
To use the atSpoke connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/recurly
module into the Ballerina project.
import ballerinax/recurly;
Step 2: Create a new connector instance
Create a recurly:ClientConfig
with the access token
obtained, and initialize the connector with it.
recurly:ClientConfig clientConfig = { auth: { token: <ACCESS_TOKEN> } }; recurly:Client baseClient = check new Client(clientConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to list a site's accounts using the connector.
List a site's accounts
public function main() returns error? { recurly:AccountList response = check baseClient->listAccounts(); log:printInfo(response.toString()); }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
recurly: Client
This is a generated connector for Recurly API V3 OpenAPI specification. Recurly is a subscription management platform delivering a competitive advantage for leading brands worldwide. Trusted by Sling TV, BarkBox, Asana, and thousands more to drive recurring revenue. Onboard and manage customers through Recurly API.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Recurly account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://v3.recurly.com" - URL of the target service
listSites
function listSites(string[]? ids, int 'limit, string 'order, string sort, string? state) returns SiteList|error
List sites
Parameters
- ids string[]? (default ()) - Filter results by their IDs. Up to 200 IDs can be passed at once using commas as separators, e.g.
ids=h1at4d57xlmy,gyqgg0d3v9n1,jrsm5b4yefg6
. Important notes: * Theids
parameter cannot be used with any other ordering or filtering parameters (limit
,order
,sort
,begin_time
,end_time
, etc) * Invalid or unknown IDs will be ignored, so you should check that the results correspond to your request. * Records are returned in an arbitrary order. Since results are all returned at once you can sort the records yourself.
- 'limit int (default 20) - Limit number of records 1-200.
- 'order string (default "desc") - Sort order.
- sort string (default "created_at") - Sort field. You really only want to sort by
updated_at
in ascending order. In descending order updated records will move behind the cursor and could prevent some records from being returned.
- state string? (default ()) - Filter by state.
getSite
Fetch a site
Parameters
- siteId string - Site ID or subdomain. For ID no prefix is used e.g.
e28zov4fw0v2
. For subdomain use prefixsubdomain-
, e.g.subdomain-recurly
.
listAccounts
function listAccounts(string[]? ids, int 'limit, string 'order, string sort, string? beginTime, string? endTime, string? email, boolean? subscriber, string? pastDue) returns AccountList|error
List a site's accounts
Parameters
- ids string[]? (default ()) - Filter results by their IDs. Up to 200 IDs can be passed at once using commas as separators, e.g.
ids=h1at4d57xlmy,gyqgg0d3v9n1,jrsm5b4yefg6
. Important notes: * Theids
parameter cannot be used with any other ordering or filtering parameters (limit
,order
,sort
,begin_time
,end_time
, etc) * Invalid or unknown IDs will be ignored, so you should check that the results correspond to your request. * Records are returned in an arbitrary order. Since results are all returned at once you can sort the records yourself.
- 'limit int (default 20) - Limit number of records 1-200.
- 'order string (default "desc") - Sort order.
- sort string (default "created_at") - Sort field. You really only want to sort by
updated_at
in ascending order. In descending order updated records will move behind the cursor and could prevent some records from being returned.
- beginTime string? (default ()) - Inclusively filter by begin_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- endTime string? (default ()) - Inclusively filter by end_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- email string? (default ()) - Filter for accounts with this exact email address. A blank value will return accounts with both
null
and""
email addresses. Note that multiple accounts can share one email address.
- subscriber boolean? (default ()) - Filter for accounts with or without a subscription in the
active
,canceled
, orfuture
state.
- pastDue string? (default ()) - Filter for accounts with an invoice in the
past_due
state.
Return Type
- AccountList|error - A list of the site's accounts.
createAccount
function createAccount(AccountCreate payload) returns Account|error
Create an account
Parameters
- payload AccountCreate -
getAccount
Fetch an account
Parameters
- accountId string - Account ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-bob
.
updateAccount
function updateAccount(string accountId, AccountUpdate payload) returns Account|error
Update an account
Parameters
- accountId string - Account ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-bob
.
- payload AccountUpdate -
deactivateAccount
Deactivate an account
Parameters
- accountId string - Account ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-bob
.
getAccountAcquisition
function getAccountAcquisition(string accountId) returns AccountAcquisition|error
Fetch an account's acquisition data
Parameters
- accountId string - Account ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-bob
.
Return Type
- AccountAcquisition|error - An account's acquisition data.
updateAccountAcquisition
function updateAccountAcquisition(string accountId, AccountAcquisitionUpdate payload) returns AccountAcquisition|error
Update an account's acquisition data
Parameters
- accountId string - Account ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-bob
.
- payload AccountAcquisitionUpdate -
Return Type
- AccountAcquisition|error - An account's updated acquisition data.
removeAccountAcquisition
Remove an account's acquisition data
Parameters
- accountId string - Account ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-bob
.
reactivateAccount
Reactivate an inactive account
Parameters
- accountId string - Account ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-bob
.
getAccountBalance
function getAccountBalance(string accountId) returns AccountBalance|error
Fetch an account's balance and past due status
Parameters
- accountId string - Account ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-bob
.
Return Type
- AccountBalance|error - An account's balance.
getBillingInfo
function getBillingInfo(string accountId) returns BillingInfo|error
Fetch an account's billing information
Parameters
- accountId string - Account ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-bob
.
Return Type
- BillingInfo|error - An account's billing information.
updateBillingInfo
function updateBillingInfo(string accountId, BillingInfoCreate payload) returns BillingInfo|error
Set an account's billing information
Parameters
- accountId string - Account ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-bob
.
- payload BillingInfoCreate -
Return Type
- BillingInfo|error - Updated billing information.
removeBillingInfo
Remove an account's billing information
Parameters
- accountId string - Account ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-bob
.
verifyBillingInfo
function verifyBillingInfo(string accountId, BillingInfoVerify payload) returns Transaction|error
Verify an account's credit card billing information
Parameters
- accountId string - Account ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-bob
.
- payload BillingInfoVerify -
Return Type
- Transaction|error - Transaction information from verify.
listBillingInfos
function listBillingInfos(string accountId, string[]? ids, string sort, string? beginTime, string? endTime) returns BillingInfoList|error
Get the list of billing information associated with an account
Parameters
- accountId string - Account ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-bob
.
- ids string[]? (default ()) - Filter results by their IDs. Up to 200 IDs can be passed at once using commas as separators, e.g.
ids=h1at4d57xlmy,gyqgg0d3v9n1,jrsm5b4yefg6
. Important notes: * Theids
parameter cannot be used with any other ordering or filtering parameters (limit
,order
,sort
,begin_time
,end_time
, etc) * Invalid or unknown IDs will be ignored, so you should check that the results correspond to your request. * Records are returned in an arbitrary order. Since results are all returned at once you can sort the records yourself.
- sort string (default "created_at") - Sort field. You really only want to sort by
updated_at
in ascending order. In descending order updated records will move behind the cursor and could prevent some records from being returned.
- beginTime string? (default ()) - Inclusively filter by begin_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- endTime string? (default ()) - Inclusively filter by end_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
Return Type
- BillingInfoList|error - A list of the the billing information for an account's
createBillingInfo
function createBillingInfo(string accountId, BillingInfoCreate payload) returns BillingInfo|error
Add new billing information on an account
Parameters
- accountId string - Account ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-bob
.
- payload BillingInfoCreate -
Return Type
- BillingInfo|error - Updated billing information.
getABillingInfo
function getABillingInfo(string accountId, string billingInfoId) returns BillingInfo|error
Fetch a billing info
Parameters
- accountId string - Account ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-bob
.
- billingInfoId string - Billing Info ID.
Return Type
- BillingInfo|error - A billing info.
updateABillingInfo
function updateABillingInfo(string accountId, string billingInfoId, BillingInfoCreate payload) returns BillingInfo|error
Update an account's billing information
Parameters
- accountId string - Account ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-bob
.
- billingInfoId string - Billing Info ID.
- payload BillingInfoCreate -
Return Type
- BillingInfo|error - Updated billing information.
removeABillingInfo
Remove an account's billing information
Parameters
- accountId string - Account ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-bob
.
- billingInfoId string - Billing Info ID.
listAccountCouponRedemptions
function listAccountCouponRedemptions(string accountId, string[]? ids, string sort, string? beginTime, string? endTime, string? state) returns CouponRedemptionList|error
Show the coupon redemptions for an account
Parameters
- accountId string - Account ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-bob
.
- ids string[]? (default ()) - Filter results by their IDs. Up to 200 IDs can be passed at once using commas as separators, e.g.
ids=h1at4d57xlmy,gyqgg0d3v9n1,jrsm5b4yefg6
. Important notes: * Theids
parameter cannot be used with any other ordering or filtering parameters (limit
,order
,sort
,begin_time
,end_time
, etc) * Invalid or unknown IDs will be ignored, so you should check that the results correspond to your request. * Records are returned in an arbitrary order. Since results are all returned at once you can sort the records yourself.
- sort string (default "created_at") - Sort field. You really only want to sort by
updated_at
in ascending order. In descending order updated records will move behind the cursor and could prevent some records from being returned.
- beginTime string? (default ()) - Inclusively filter by begin_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- endTime string? (default ()) - Inclusively filter by end_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- state string? (default ()) - Filter by state.
Return Type
- CouponRedemptionList|error - A list of the the coupon redemptions on an account.
listActiveCouponRedemptions
function listActiveCouponRedemptions(string accountId) returns CouponRedemptionList|error
Show the coupon redemptions that are active on an account
Parameters
- accountId string - Account ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-bob
.
Return Type
- CouponRedemptionList|error - Active coupon redemptions on an account.
createCouponRedemption
function createCouponRedemption(string accountId, CouponRedemptionCreate payload) returns CouponRedemption|error
Generate an active coupon redemption on an account or subscription
Parameters
- accountId string - Account ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-bob
.
- payload CouponRedemptionCreate -
Return Type
- CouponRedemption|error - Returns the new coupon redemption.
removeCouponRedemption
function removeCouponRedemption(string accountId) returns CouponRedemption|error
Delete the active coupon redemption from an account
Parameters
- accountId string - Account ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-bob
.
Return Type
- CouponRedemption|error - Coupon redemption deleted.
listAccountCreditPayments
function listAccountCreditPayments(string accountId, int 'limit, string 'order, string sort, string? beginTime, string? endTime) returns CreditPaymentList|error
List an account's credit payments
Parameters
- accountId string - Account ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-bob
.
- 'limit int (default 20) - Limit number of records 1-200.
- 'order string (default "desc") - Sort order.
- sort string (default "created_at") - Sort field. You really only want to sort by
updated_at
in ascending order. In descending order updated records will move behind the cursor and could prevent some records from being returned.
- beginTime string? (default ()) - Inclusively filter by begin_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- endTime string? (default ()) - Inclusively filter by end_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
Return Type
- CreditPaymentList|error - A list of the account's credit payments.
listAccountInvoices
function listAccountInvoices(string accountId, string[]? ids, int 'limit, string 'order, string sort, string? beginTime, string? endTime, string? 'type) returns InvoiceList|error
List an account's invoices
Parameters
- accountId string - Account ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-bob
.
- ids string[]? (default ()) - Filter results by their IDs. Up to 200 IDs can be passed at once using commas as separators, e.g.
ids=h1at4d57xlmy,gyqgg0d3v9n1,jrsm5b4yefg6
. Important notes: * Theids
parameter cannot be used with any other ordering or filtering parameters (limit
,order
,sort
,begin_time
,end_time
, etc) * Invalid or unknown IDs will be ignored, so you should check that the results correspond to your request. * Records are returned in an arbitrary order. Since results are all returned at once you can sort the records yourself.
- 'limit int (default 20) - Limit number of records 1-200.
- 'order string (default "desc") - Sort order.
- sort string (default "created_at") - Sort field. You really only want to sort by
updated_at
in ascending order. In descending order updated records will move behind the cursor and could prevent some records from being returned.
- beginTime string? (default ()) - Inclusively filter by begin_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- endTime string? (default ()) - Inclusively filter by end_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- 'type string? (default ()) - Filter by type when: -
type=charge
, only charge invoices will be returned. -type=credit
, only credit invoices will be returned. -type=non-legacy
, only charge and credit invoices will be returned. -type=legacy
, only legacy invoices will be returned.
Return Type
- InvoiceList|error - A list of the account's invoices.
createInvoice
function createInvoice(string accountId, InvoiceCreate payload) returns InvoiceCollection|error
Create an invoice for pending line items
Parameters
- accountId string - Account ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-bob
.
- payload InvoiceCreate -
Return Type
- InvoiceCollection|error - Returns the new invoices.
previewInvoice
function previewInvoice(string accountId, InvoiceCreate payload) returns InvoiceCollection|error
Preview new invoice for pending line items
Parameters
- accountId string - Account ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-bob
.
- payload InvoiceCreate -
Return Type
- InvoiceCollection|error - Returns the invoice previews.
listAccountLineItems
function listAccountLineItems(string accountId, string[]? ids, int 'limit, string 'order, string sort, string? beginTime, string? endTime, string? original, string? state, string? 'type) returns LineItemList|error
List an account's line items
Parameters
- accountId string - Account ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-bob
.
- ids string[]? (default ()) - Filter results by their IDs. Up to 200 IDs can be passed at once using commas as separators, e.g.
ids=h1at4d57xlmy,gyqgg0d3v9n1,jrsm5b4yefg6
. Important notes: * Theids
parameter cannot be used with any other ordering or filtering parameters (limit
,order
,sort
,begin_time
,end_time
, etc) * Invalid or unknown IDs will be ignored, so you should check that the results correspond to your request. * Records are returned in an arbitrary order. Since results are all returned at once you can sort the records yourself.
- 'limit int (default 20) - Limit number of records 1-200.
- 'order string (default "desc") - Sort order.
- sort string (default "created_at") - Sort field. You really only want to sort by
updated_at
in ascending order. In descending order updated records will move behind the cursor and could prevent some records from being returned.
- beginTime string? (default ()) - Inclusively filter by begin_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- endTime string? (default ()) - Inclusively filter by end_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- original string? (default ()) - Filter by original field.
- state string? (default ()) - Filter by state field.
- 'type string? (default ()) - Filter by type field.
Return Type
- LineItemList|error - A list of the account's line items.
createLineItem
function createLineItem(string accountId, LineItemCreate payload) returns LineItem|error
Create a new line item for the account
Parameters
- accountId string - Account ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-bob
.
- payload LineItemCreate -
listAccountNotes
function listAccountNotes(string accountId, string[]? ids) returns AccountNoteList|error
Fetch a list of an account's notes
Parameters
- accountId string - Account ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-bob
.
- ids string[]? (default ()) - Filter results by their IDs. Up to 200 IDs can be passed at once using commas as separators, e.g.
ids=h1at4d57xlmy,gyqgg0d3v9n1,jrsm5b4yefg6
. Important notes: * Theids
parameter cannot be used with any other ordering or filtering parameters (limit
,order
,sort
,begin_time
,end_time
, etc) * Invalid or unknown IDs will be ignored, so you should check that the results correspond to your request. * Records are returned in an arbitrary order. Since results are all returned at once you can sort the records yourself.
Return Type
- AccountNoteList|error - A list of an account's notes.
getAccountNote
function getAccountNote(string accountId, string accountNoteId) returns AccountNote|error
Fetch an account note
Parameters
- accountId string - Account ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-bob
.
- accountNoteId string - Account Note ID.
Return Type
- AccountNote|error - An account note.
listShippingAddresses
function listShippingAddresses(string accountId, string[]? ids, int 'limit, string 'order, string sort, string? beginTime, string? endTime) returns ShippingAddressList|error
Fetch a list of an account's shipping addresses
Parameters
- accountId string - Account ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-bob
.
- ids string[]? (default ()) - Filter results by their IDs. Up to 200 IDs can be passed at once using commas as separators, e.g.
ids=h1at4d57xlmy,gyqgg0d3v9n1,jrsm5b4yefg6
. Important notes: * Theids
parameter cannot be used with any other ordering or filtering parameters (limit
,order
,sort
,begin_time
,end_time
, etc) * Invalid or unknown IDs will be ignored, so you should check that the results correspond to your request. * Records are returned in an arbitrary order. Since results are all returned at once you can sort the records yourself.
- 'limit int (default 20) - Limit number of records 1-200.
- 'order string (default "desc") - Sort order.
- sort string (default "created_at") - Sort field. You really only want to sort by
updated_at
in ascending order. In descending order updated records will move behind the cursor and could prevent some records from being returned.
- beginTime string? (default ()) - Inclusively filter by begin_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- endTime string? (default ()) - Inclusively filter by end_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
Return Type
- ShippingAddressList|error - A list of an account's shipping addresses.
createShippingAddress
function createShippingAddress(string accountId, ShippingAddressCreate payload) returns ShippingAddress|error
Create a new shipping address for the account
Parameters
- accountId string - Account ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-bob
.
- payload ShippingAddressCreate -
Return Type
- ShippingAddress|error - Returns the new shipping address.
getShippingAddress
function getShippingAddress(string accountId, string shippingAddressId) returns ShippingAddress|error
Fetch an account's shipping address
Parameters
- accountId string - Account ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-bob
.
- shippingAddressId string - Shipping Address ID.
Return Type
- ShippingAddress|error - A shipping address.
updateShippingAddress
function updateShippingAddress(string accountId, string shippingAddressId, ShippingAddressUpdate payload) returns ShippingAddress|error
Update an account's shipping address
Parameters
- accountId string - Account ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-bob
.
- shippingAddressId string - Shipping Address ID.
- payload ShippingAddressUpdate -
Return Type
- ShippingAddress|error - The updated shipping address.
removeShippingAddress
Remove an account's shipping address
Parameters
- accountId string - Account ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-bob
.
- shippingAddressId string - Shipping Address ID.
listAccountSubscriptions
function listAccountSubscriptions(string accountId, string[]? ids, int 'limit, string 'order, string sort, string? beginTime, string? endTime, string? state) returns SubscriptionList|error
List an account's subscriptions
Parameters
- accountId string - Account ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-bob
.
- ids string[]? (default ()) - Filter results by their IDs. Up to 200 IDs can be passed at once using commas as separators, e.g.
ids=h1at4d57xlmy,gyqgg0d3v9n1,jrsm5b4yefg6
. Important notes: * Theids
parameter cannot be used with any other ordering or filtering parameters (limit
,order
,sort
,begin_time
,end_time
, etc) * Invalid or unknown IDs will be ignored, so you should check that the results correspond to your request. * Records are returned in an arbitrary order. Since results are all returned at once you can sort the records yourself.
- 'limit int (default 20) - Limit number of records 1-200.
- 'order string (default "desc") - Sort order.
- sort string (default "created_at") - Sort field. You really only want to sort by
updated_at
in ascending order. In descending order updated records will move behind the cursor and could prevent some records from being returned.
- beginTime string? (default ()) - Inclusively filter by begin_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- endTime string? (default ()) - Inclusively filter by end_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- state string? (default ()) - Filter by state. - When
state=active
,state=canceled
,state=expired
, orstate=future
, subscriptions with states that match the query and only those subscriptions will be returned. - Whenstate=in_trial
, only subscriptions that have a trial_started_at date earlier than now and a trial_ends_at date later than now will be returned. - Whenstate=live
, only subscriptions that are in an active, canceled, or future state or are in trial will be returned.
Return Type
- SubscriptionList|error - A list of the account's subscriptions.
listAccountTransactions
function listAccountTransactions(string accountId, string[]? ids, int 'limit, string 'order, string sort, string? beginTime, string? endTime, string? 'type, string? success) returns TransactionList|error
List an account's transactions
Parameters
- accountId string - Account ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-bob
.
- ids string[]? (default ()) - Filter results by their IDs. Up to 200 IDs can be passed at once using commas as separators, e.g.
ids=h1at4d57xlmy,gyqgg0d3v9n1,jrsm5b4yefg6
. Important notes: * Theids
parameter cannot be used with any other ordering or filtering parameters (limit
,order
,sort
,begin_time
,end_time
, etc) * Invalid or unknown IDs will be ignored, so you should check that the results correspond to your request. * Records are returned in an arbitrary order. Since results are all returned at once you can sort the records yourself.
- 'limit int (default 20) - Limit number of records 1-200.
- 'order string (default "desc") - Sort order.
- sort string (default "created_at") - Sort field. You really only want to sort by
updated_at
in ascending order. In descending order updated records will move behind the cursor and could prevent some records from being returned.
- beginTime string? (default ()) - Inclusively filter by begin_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- endTime string? (default ()) - Inclusively filter by end_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- 'type string? (default ()) - Filter by type field. The value
payment
will return bothpurchase
andcapture
transactions.
- success string? (default ()) - Filter by success field.
Return Type
- TransactionList|error - A list of the account's transactions.
listChildAccounts
function listChildAccounts(string accountId, string[]? ids, int 'limit, string 'order, string sort, string? beginTime, string? endTime, string? email, boolean? subscriber, string? pastDue) returns AccountList|error
List an account's child accounts
Parameters
- accountId string - Account ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-bob
.
- ids string[]? (default ()) - Filter results by their IDs. Up to 200 IDs can be passed at once using commas as separators, e.g.
ids=h1at4d57xlmy,gyqgg0d3v9n1,jrsm5b4yefg6
. Important notes: * Theids
parameter cannot be used with any other ordering or filtering parameters (limit
,order
,sort
,begin_time
,end_time
, etc) * Invalid or unknown IDs will be ignored, so you should check that the results correspond to your request. * Records are returned in an arbitrary order. Since results are all returned at once you can sort the records yourself.
- 'limit int (default 20) - Limit number of records 1-200.
- 'order string (default "desc") - Sort order.
- sort string (default "created_at") - Sort field. You really only want to sort by
updated_at
in ascending order. In descending order updated records will move behind the cursor and could prevent some records from being returned.
- beginTime string? (default ()) - Inclusively filter by begin_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- endTime string? (default ()) - Inclusively filter by end_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- email string? (default ()) - Filter for accounts with this exact email address. A blank value will return accounts with both
null
and""
email addresses. Note that multiple accounts can share one email address.
- subscriber boolean? (default ()) - Filter for accounts with or without a subscription in the
active
,canceled
, orfuture
state.
- pastDue string? (default ()) - Filter for accounts with an invoice in the
past_due
state.
Return Type
- AccountList|error - A list of an account's child accounts.
listAccountAcquisition
function listAccountAcquisition(string[]? ids, int 'limit, string 'order, string sort, string? beginTime, string? endTime) returns AccountAcquisitionList|error
List a site's account acquisition data
Parameters
- ids string[]? (default ()) - Filter results by their IDs. Up to 200 IDs can be passed at once using commas as separators, e.g.
ids=h1at4d57xlmy,gyqgg0d3v9n1,jrsm5b4yefg6
. Important notes: * Theids
parameter cannot be used with any other ordering or filtering parameters (limit
,order
,sort
,begin_time
,end_time
, etc) * Invalid or unknown IDs will be ignored, so you should check that the results correspond to your request. * Records are returned in an arbitrary order. Since results are all returned at once you can sort the records yourself.
- 'limit int (default 20) - Limit number of records 1-200.
- 'order string (default "desc") - Sort order.
- sort string (default "created_at") - Sort field. You really only want to sort by
updated_at
in ascending order. In descending order updated records will move behind the cursor and could prevent some records from being returned.
- beginTime string? (default ()) - Inclusively filter by begin_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- endTime string? (default ()) - Inclusively filter by end_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
Return Type
- AccountAcquisitionList|error - A list of the site's account acquisition data.
listCoupons
function listCoupons(string[]? ids, int 'limit, string 'order, string sort, string? beginTime, string? endTime) returns CouponList|error
List a site's coupons
Parameters
- ids string[]? (default ()) - Filter results by their IDs. Up to 200 IDs can be passed at once using commas as separators, e.g.
ids=h1at4d57xlmy,gyqgg0d3v9n1,jrsm5b4yefg6
. Important notes: * Theids
parameter cannot be used with any other ordering or filtering parameters (limit
,order
,sort
,begin_time
,end_time
, etc) * Invalid or unknown IDs will be ignored, so you should check that the results correspond to your request. * Records are returned in an arbitrary order. Since results are all returned at once you can sort the records yourself.
- 'limit int (default 20) - Limit number of records 1-200.
- 'order string (default "desc") - Sort order.
- sort string (default "created_at") - Sort field. You really only want to sort by
updated_at
in ascending order. In descending order updated records will move behind the cursor and could prevent some records from being returned.
- beginTime string? (default ()) - Inclusively filter by begin_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- endTime string? (default ()) - Inclusively filter by end_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
Return Type
- CouponList|error - A list of the site's coupons.
createCoupon
function createCoupon(CouponCreate payload) returns Coupon|error
Create a new coupon
Parameters
- payload CouponCreate -
getCoupon
Fetch a coupon
Parameters
- couponId string - Coupon ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-10off
.
updateCoupon
function updateCoupon(string couponId, CouponUpdate payload) returns Coupon|error
Update an active coupon
Parameters
- couponId string - Coupon ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-10off
.
- payload CouponUpdate -
deactivateCoupon
Expire a coupon
Parameters
- couponId string - Coupon ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-10off
.
generateUniqueCouponCodes
function generateUniqueCouponCodes(string couponId, CouponBulkCreate payload) returns UniqueCouponCodeParams|error
Generate unique coupon codes
Parameters
- couponId string - Coupon ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-10off
.
- payload CouponBulkCreate -
Return Type
- UniqueCouponCodeParams|error - A set of parameters that can be passed to the
list_unique_coupon_codes
endpoint to obtain only the newly generatedUniqueCouponCodes
.
restoreCoupon
function restoreCoupon(string couponId, CouponUpdate payload) returns Coupon|error
Restore an inactive coupon
Parameters
- couponId string - Coupon ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-10off
.
- payload CouponUpdate -
listUniqueCouponCodes
function listUniqueCouponCodes(string couponId, string[]? ids, int 'limit, string 'order, string sort, string? beginTime, string? endTime) returns UniqueCouponCodeList|error
List unique coupon codes associated with a bulk coupon
Parameters
- couponId string - Coupon ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-10off
.
- ids string[]? (default ()) - Filter results by their IDs. Up to 200 IDs can be passed at once using commas as separators, e.g.
ids=h1at4d57xlmy,gyqgg0d3v9n1,jrsm5b4yefg6
. Important notes: * Theids
parameter cannot be used with any other ordering or filtering parameters (limit
,order
,sort
,begin_time
,end_time
, etc) * Invalid or unknown IDs will be ignored, so you should check that the results correspond to your request. * Records are returned in an arbitrary order. Since results are all returned at once you can sort the records yourself.
- 'limit int (default 20) - Limit number of records 1-200.
- 'order string (default "desc") - Sort order.
- sort string (default "created_at") - Sort field. You really only want to sort by
updated_at
in ascending order. In descending order updated records will move behind the cursor and could prevent some records from being returned.
- beginTime string? (default ()) - Inclusively filter by begin_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- endTime string? (default ()) - Inclusively filter by end_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
Return Type
- UniqueCouponCodeList|error - A list of unique coupon codes that were generated
listCreditPayments
function listCreditPayments(int 'limit, string 'order, string sort, string? beginTime, string? endTime) returns CreditPaymentList|error
List a site's credit payments
Parameters
- 'limit int (default 20) - Limit number of records 1-200.
- 'order string (default "desc") - Sort order.
- sort string (default "created_at") - Sort field. You really only want to sort by
updated_at
in ascending order. In descending order updated records will move behind the cursor and could prevent some records from being returned.
- beginTime string? (default ()) - Inclusively filter by begin_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- endTime string? (default ()) - Inclusively filter by end_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
Return Type
- CreditPaymentList|error - A list of the site's credit payments.
getCreditPayment
function getCreditPayment(string creditPaymentId) returns CreditPayment|error
Fetch a credit payment
Parameters
- creditPaymentId string - Credit Payment ID or UUID. For ID no prefix is used e.g.
e28zov4fw0v2
. For UUID use prefixuuid-
, e.g.uuid-123457890
.
Return Type
- CreditPayment|error - A credit payment.
listCustomFieldDefinitions
function listCustomFieldDefinitions(string[]? ids, int 'limit, string 'order, string sort, string? beginTime, string? endTime, string? relatedType) returns CustomFieldDefinitionList|error
List a site's custom field definitions
Parameters
- ids string[]? (default ()) - Filter results by their IDs. Up to 200 IDs can be passed at once using commas as separators, e.g.
ids=h1at4d57xlmy,gyqgg0d3v9n1,jrsm5b4yefg6
. Important notes: * Theids
parameter cannot be used with any other ordering or filtering parameters (limit
,order
,sort
,begin_time
,end_time
, etc) * Invalid or unknown IDs will be ignored, so you should check that the results correspond to your request. * Records are returned in an arbitrary order. Since results are all returned at once you can sort the records yourself.
- 'limit int (default 20) - Limit number of records 1-200.
- 'order string (default "desc") - Sort order.
- sort string (default "created_at") - Sort field. You really only want to sort by
updated_at
in ascending order. In descending order updated records will move behind the cursor and could prevent some records from being returned.
- beginTime string? (default ()) - Inclusively filter by begin_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- endTime string? (default ()) - Inclusively filter by end_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- relatedType string? (default ()) - Filter by related type.
Return Type
- CustomFieldDefinitionList|error - A list of the site's custom field definitions.
getCustomFieldDefinition
function getCustomFieldDefinition(string customFieldDefinitionId) returns CustomFieldDefinition|error
Fetch an custom field definition
Parameters
- customFieldDefinitionId string - Custom Field Definition ID
Return Type
- CustomFieldDefinition|error - An custom field definition.
listItems
function listItems(string[]? ids, int 'limit, string 'order, string sort, string? beginTime, string? endTime, string? state) returns ItemList|error
List a site's items
Parameters
- ids string[]? (default ()) - Filter results by their IDs. Up to 200 IDs can be passed at once using commas as separators, e.g.
ids=h1at4d57xlmy,gyqgg0d3v9n1,jrsm5b4yefg6
. Important notes: * Theids
parameter cannot be used with any other ordering or filtering parameters (limit
,order
,sort
,begin_time
,end_time
, etc) * Invalid or unknown IDs will be ignored, so you should check that the results correspond to your request. * Records are returned in an arbitrary order. Since results are all returned at once you can sort the records yourself.
- 'limit int (default 20) - Limit number of records 1-200.
- 'order string (default "desc") - Sort order.
- sort string (default "created_at") - Sort field. You really only want to sort by
updated_at
in ascending order. In descending order updated records will move behind the cursor and could prevent some records from being returned.
- beginTime string? (default ()) - Inclusively filter by begin_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- endTime string? (default ()) - Inclusively filter by end_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- state string? (default ()) - Filter by state.
createItem
function createItem(ItemCreate payload) returns Item|error
Create a new item
Parameters
- payload ItemCreate -
getItem
Fetch an item
Parameters
- itemId string - Item ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-red
.
updateItem
function updateItem(string itemId, ItemUpdate payload) returns Item|error
Update an active item
Parameters
- itemId string - Item ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-red
.
- payload ItemUpdate -
deactivateItem
Deactivate an item
Parameters
- itemId string - Item ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-red
.
reactivateItem
Reactivate an inactive item
Parameters
- itemId string - Item ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-red
.
listMeasuredUnit
function listMeasuredUnit(string[]? ids, int 'limit, string 'order, string sort, string? beginTime, string? endTime, string? state) returns MeasuredUnitList|error
List a site's measured units
Parameters
- ids string[]? (default ()) - Filter results by their IDs. Up to 200 IDs can be passed at once using commas as separators, e.g.
ids=h1at4d57xlmy,gyqgg0d3v9n1,jrsm5b4yefg6
. Important notes: * Theids
parameter cannot be used with any other ordering or filtering parameters (limit
,order
,sort
,begin_time
,end_time
, etc) * Invalid or unknown IDs will be ignored, so you should check that the results correspond to your request. * Records are returned in an arbitrary order. Since results are all returned at once you can sort the records yourself.
- 'limit int (default 20) - Limit number of records 1-200.
- 'order string (default "desc") - Sort order.
- sort string (default "created_at") - Sort field. You really only want to sort by
updated_at
in ascending order. In descending order updated records will move behind the cursor and could prevent some records from being returned.
- beginTime string? (default ()) - Inclusively filter by begin_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- endTime string? (default ()) - Inclusively filter by end_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- state string? (default ()) - Filter by state.
Return Type
- MeasuredUnitList|error - A list of the site's measured units.
createMeasuredUnit
function createMeasuredUnit(MeasuredUnitCreate payload) returns MeasuredUnit|error
Create a new measured unit
Parameters
- payload MeasuredUnitCreate -
Return Type
- MeasuredUnit|error - A new measured unit.
getMeasuredUnit
function getMeasuredUnit(string measuredUnitId) returns MeasuredUnit|error
Fetch a measured unit
Parameters
- measuredUnitId string - Measured unit ID or name. For ID no prefix is used e.g.
e28zov4fw0v2
. For name use prefixname-
, e.g.name-Storage
.
Return Type
- MeasuredUnit|error - An item.
updateMeasuredUnit
function updateMeasuredUnit(string measuredUnitId, MeasuredUnitUpdate payload) returns MeasuredUnit|error
Update a measured unit
Parameters
- measuredUnitId string - Measured unit ID or name. For ID no prefix is used e.g.
e28zov4fw0v2
. For name use prefixname-
, e.g.name-Storage
.
- payload MeasuredUnitUpdate -
Return Type
- MeasuredUnit|error - The updated measured_unit.
removeMeasuredUnit
function removeMeasuredUnit(string measuredUnitId) returns MeasuredUnit|error
Remove a measured unit
Parameters
- measuredUnitId string - Measured unit ID or name. For ID no prefix is used e.g.
e28zov4fw0v2
. For name use prefixname-
, e.g.name-Storage
.
Return Type
- MeasuredUnit|error - A measured unit.
listInvoices
function listInvoices(string[]? ids, int 'limit, string 'order, string sort, string? beginTime, string? endTime, string? 'type) returns InvoiceList|error
List a site's invoices
Parameters
- ids string[]? (default ()) - Filter results by their IDs. Up to 200 IDs can be passed at once using commas as separators, e.g.
ids=h1at4d57xlmy,gyqgg0d3v9n1,jrsm5b4yefg6
. Important notes: * Theids
parameter cannot be used with any other ordering or filtering parameters (limit
,order
,sort
,begin_time
,end_time
, etc) * Invalid or unknown IDs will be ignored, so you should check that the results correspond to your request. * Records are returned in an arbitrary order. Since results are all returned at once you can sort the records yourself.
- 'limit int (default 20) - Limit number of records 1-200.
- 'order string (default "desc") - Sort order.
- sort string (default "created_at") - Sort field. You really only want to sort by
updated_at
in ascending order. In descending order updated records will move behind the cursor and could prevent some records from being returned.
- beginTime string? (default ()) - Inclusively filter by begin_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- endTime string? (default ()) - Inclusively filter by end_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- 'type string? (default ()) - Filter by type when: -
type=charge
, only charge invoices will be returned. -type=credit
, only credit invoices will be returned. -type=non-legacy
, only charge and credit invoices will be returned. -type=legacy
, only legacy invoices will be returned.
Return Type
- InvoiceList|error - A list of the site's invoices.
getInvoice
Fetch an invoice
Parameters
- invoiceId string - Invoice ID or number. For ID no prefix is used e.g.
e28zov4fw0v2
. For number use prefixnumber-
, e.g.number-1000
.
updateInvoice
function updateInvoice(string invoiceId, InvoiceUpdate payload) returns Invoice|error
Update an invoice
Parameters
- invoiceId string - Invoice ID or number. For ID no prefix is used e.g.
e28zov4fw0v2
. For number use prefixnumber-
, e.g.number-1000
.
- payload InvoiceUpdate -
getInvoicePdf
function getInvoicePdf(string invoiceId) returns BinaryFile|error
Fetch an invoice as a PDF
Parameters
- invoiceId string - Invoice ID or number. For ID no prefix is used e.g.
e28zov4fw0v2
. For number use prefixnumber-
, e.g.number-1000
.
Return Type
- BinaryFile|error - An invoice as a PDF.
collectInvoice
function collectInvoice(string invoiceId, InvoiceCollect payload) returns Invoice|error
Collect a pending or past due, automatic invoice
Parameters
- invoiceId string - Invoice ID or number. For ID no prefix is used e.g.
e28zov4fw0v2
. For number use prefixnumber-
, e.g.number-1000
.
- payload InvoiceCollect -
markInvoiceFailed
Mark an open invoice as failed
Parameters
- invoiceId string - Invoice ID or number. For ID no prefix is used e.g.
e28zov4fw0v2
. For number use prefixnumber-
, e.g.number-1000
.
markInvoiceSuccessful
Mark an open invoice as successful
Parameters
- invoiceId string - Invoice ID or number. For ID no prefix is used e.g.
e28zov4fw0v2
. For number use prefixnumber-
, e.g.number-1000
.
reopenInvoice
Reopen a closed, manual invoice
Parameters
- invoiceId string - Invoice ID or number. For ID no prefix is used e.g.
e28zov4fw0v2
. For number use prefixnumber-
, e.g.number-1000
.
voidInvoice
Void a credit invoice.
Parameters
- invoiceId string - Invoice ID or number. For ID no prefix is used e.g.
e28zov4fw0v2
. For number use prefixnumber-
, e.g.number-1000
.
recordExternalTransaction
function recordExternalTransaction(string invoiceId, ExternalTransaction payload) returns Transaction|error
Record an external payment for a manual invoices.
Parameters
- invoiceId string - Invoice ID or number. For ID no prefix is used e.g.
e28zov4fw0v2
. For number use prefixnumber-
, e.g.number-1000
.
- payload ExternalTransaction -
Return Type
- Transaction|error - The recorded transaction.
listInvoiceLineItems
function listInvoiceLineItems(string invoiceId, string[]? ids, int 'limit, string 'order, string sort, string? beginTime, string? endTime, string? original, string? state, string? 'type) returns LineItemList|error
List an invoice's line items
Parameters
- invoiceId string - Invoice ID or number. For ID no prefix is used e.g.
e28zov4fw0v2
. For number use prefixnumber-
, e.g.number-1000
.
- ids string[]? (default ()) - Filter results by their IDs. Up to 200 IDs can be passed at once using commas as separators, e.g.
ids=h1at4d57xlmy,gyqgg0d3v9n1,jrsm5b4yefg6
. Important notes: * Theids
parameter cannot be used with any other ordering or filtering parameters (limit
,order
,sort
,begin_time
,end_time
, etc) * Invalid or unknown IDs will be ignored, so you should check that the results correspond to your request. * Records are returned in an arbitrary order. Since results are all returned at once you can sort the records yourself.
- 'limit int (default 20) - Limit number of records 1-200.
- 'order string (default "desc") - Sort order.
- sort string (default "created_at") - Sort field. You really only want to sort by
updated_at
in ascending order. In descending order updated records will move behind the cursor and could prevent some records from being returned.
- beginTime string? (default ()) - Inclusively filter by begin_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- endTime string? (default ()) - Inclusively filter by end_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- original string? (default ()) - Filter by original field.
- state string? (default ()) - Filter by state field.
- 'type string? (default ()) - Filter by type field.
Return Type
- LineItemList|error - A list of the invoice's line items.
listInvoiceCouponRedemptions
function listInvoiceCouponRedemptions(string invoiceId, string[]? ids, string sort, string? beginTime, string? endTime) returns CouponRedemptionList|error
Show the coupon redemptions applied to an invoice
Parameters
- invoiceId string - Invoice ID or number. For ID no prefix is used e.g.
e28zov4fw0v2
. For number use prefixnumber-
, e.g.number-1000
.
- ids string[]? (default ()) - Filter results by their IDs. Up to 200 IDs can be passed at once using commas as separators, e.g.
ids=h1at4d57xlmy,gyqgg0d3v9n1,jrsm5b4yefg6
. Important notes: * Theids
parameter cannot be used with any other ordering or filtering parameters (limit
,order
,sort
,begin_time
,end_time
, etc) * Invalid or unknown IDs will be ignored, so you should check that the results correspond to your request. * Records are returned in an arbitrary order. Since results are all returned at once you can sort the records yourself.
- sort string (default "created_at") - Sort field. You really only want to sort by
updated_at
in ascending order. In descending order updated records will move behind the cursor and could prevent some records from being returned.
- beginTime string? (default ()) - Inclusively filter by begin_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- endTime string? (default ()) - Inclusively filter by end_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
Return Type
- CouponRedemptionList|error - A list of the the coupon redemptions associated with the invoice.
listRelatedInvoices
function listRelatedInvoices(string invoiceId) returns InvoiceList|error
List an invoice's related credit or charge invoices
Parameters
- invoiceId string - Invoice ID or number. For ID no prefix is used e.g.
e28zov4fw0v2
. For number use prefixnumber-
, e.g.number-1000
.
Return Type
- InvoiceList|error - A list of the credit or charge invoices associated with the invoice.
refundInvoice
function refundInvoice(string invoiceId, InvoiceRefund payload) returns Invoice|error
Refund an invoice
Parameters
- invoiceId string - Invoice ID or number. For ID no prefix is used e.g.
e28zov4fw0v2
. For number use prefixnumber-
, e.g.number-1000
.
- payload InvoiceRefund -
listLineItems
function listLineItems(string[]? ids, int 'limit, string 'order, string sort, string? beginTime, string? endTime, string? original, string? state, string? 'type) returns LineItemList|error
List a site's line items
Parameters
- ids string[]? (default ()) - Filter results by their IDs. Up to 200 IDs can be passed at once using commas as separators, e.g.
ids=h1at4d57xlmy,gyqgg0d3v9n1,jrsm5b4yefg6
. Important notes: * Theids
parameter cannot be used with any other ordering or filtering parameters (limit
,order
,sort
,begin_time
,end_time
, etc) * Invalid or unknown IDs will be ignored, so you should check that the results correspond to your request. * Records are returned in an arbitrary order. Since results are all returned at once you can sort the records yourself.
- 'limit int (default 20) - Limit number of records 1-200.
- 'order string (default "desc") - Sort order.
- sort string (default "created_at") - Sort field. You really only want to sort by
updated_at
in ascending order. In descending order updated records will move behind the cursor and could prevent some records from being returned.
- beginTime string? (default ()) - Inclusively filter by begin_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- endTime string? (default ()) - Inclusively filter by end_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- original string? (default ()) - Filter by original field.
- state string? (default ()) - Filter by state field.
- 'type string? (default ()) - Filter by type field.
Return Type
- LineItemList|error - A list of the site's line items.
getLineItem
Fetch a line item
Parameters
- lineItemId string - Line Item ID.
removeLineItem
Delete an uninvoiced line item
Parameters
- lineItemId string - Line Item ID.
listPlans
function listPlans(string[]? ids, int 'limit, string 'order, string sort, string? beginTime, string? endTime, string? state) returns PlanList|error
List a site's plans
Parameters
- ids string[]? (default ()) - Filter results by their IDs. Up to 200 IDs can be passed at once using commas as separators, e.g.
ids=h1at4d57xlmy,gyqgg0d3v9n1,jrsm5b4yefg6
. Important notes: * Theids
parameter cannot be used with any other ordering or filtering parameters (limit
,order
,sort
,begin_time
,end_time
, etc) * Invalid or unknown IDs will be ignored, so you should check that the results correspond to your request. * Records are returned in an arbitrary order. Since results are all returned at once you can sort the records yourself.
- 'limit int (default 20) - Limit number of records 1-200.
- 'order string (default "desc") - Sort order.
- sort string (default "created_at") - Sort field. You really only want to sort by
updated_at
in ascending order. In descending order updated records will move behind the cursor and could prevent some records from being returned.
- beginTime string? (default ()) - Inclusively filter by begin_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- endTime string? (default ()) - Inclusively filter by end_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- state string? (default ()) - Filter by state.
createPlan
function createPlan(PlanCreate payload) returns Plan|error
Create a plan
Parameters
- payload PlanCreate -
getPlan
Fetch a plan
Parameters
- planId string - Plan ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-gold
.
updatePlan
function updatePlan(string planId, PlanUpdate payload) returns Plan|error
Update a plan
Parameters
- planId string - Plan ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-gold
.
- payload PlanUpdate -
removePlan
Remove a plan
Parameters
- planId string - Plan ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-gold
.
listPlanAddOns
function listPlanAddOns(string planId, string[]? ids, int 'limit, string 'order, string sort, string? beginTime, string? endTime, string? state) returns AddOnList|error
List a plan's add-ons
Parameters
- planId string - Plan ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-gold
.
- ids string[]? (default ()) - Filter results by their IDs. Up to 200 IDs can be passed at once using commas as separators, e.g.
ids=h1at4d57xlmy,gyqgg0d3v9n1,jrsm5b4yefg6
. Important notes: * Theids
parameter cannot be used with any other ordering or filtering parameters (limit
,order
,sort
,begin_time
,end_time
, etc) * Invalid or unknown IDs will be ignored, so you should check that the results correspond to your request. * Records are returned in an arbitrary order. Since results are all returned at once you can sort the records yourself.
- 'limit int (default 20) - Limit number of records 1-200.
- 'order string (default "desc") - Sort order.
- sort string (default "created_at") - Sort field. You really only want to sort by
updated_at
in ascending order. In descending order updated records will move behind the cursor and could prevent some records from being returned.
- beginTime string? (default ()) - Inclusively filter by begin_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- endTime string? (default ()) - Inclusively filter by end_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- state string? (default ()) - Filter by state.
createPlanAddOn
function createPlanAddOn(string planId, AddOnCreate payload) returns AddOn|error
Create an add-on
Parameters
- planId string - Plan ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-gold
.
- payload AddOnCreate -
getPlanAddOn
Fetch a plan's add-on
Parameters
- planId string - Plan ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-gold
.
- addOnId string - Add-on ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-gold
.
updatePlanAddOn
function updatePlanAddOn(string planId, string addOnId, AddOnUpdate payload) returns AddOn|error
Update an add-on
Parameters
- planId string - Plan ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-gold
.
- addOnId string - Add-on ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-gold
.
- payload AddOnUpdate -
removePlanAddOn
Remove an add-on
Parameters
- planId string - Plan ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-gold
.
- addOnId string - Add-on ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-gold
.
listAddOns
function listAddOns(string[]? ids, int 'limit, string 'order, string sort, string? beginTime, string? endTime, string? state) returns AddOnList|error
List a site's add-ons
Parameters
- ids string[]? (default ()) - Filter results by their IDs. Up to 200 IDs can be passed at once using commas as separators, e.g.
ids=h1at4d57xlmy,gyqgg0d3v9n1,jrsm5b4yefg6
. Important notes: * Theids
parameter cannot be used with any other ordering or filtering parameters (limit
,order
,sort
,begin_time
,end_time
, etc) * Invalid or unknown IDs will be ignored, so you should check that the results correspond to your request. * Records are returned in an arbitrary order. Since results are all returned at once you can sort the records yourself.
- 'limit int (default 20) - Limit number of records 1-200.
- 'order string (default "desc") - Sort order.
- sort string (default "created_at") - Sort field. You really only want to sort by
updated_at
in ascending order. In descending order updated records will move behind the cursor and could prevent some records from being returned.
- beginTime string? (default ()) - Inclusively filter by begin_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- endTime string? (default ()) - Inclusively filter by end_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- state string? (default ()) - Filter by state.
getAddOn
Fetch an add-on
Parameters
- addOnId string - Add-on ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-gold
.
listShippingMethods
function listShippingMethods(string[]? ids, int 'limit, string 'order, string sort, string? beginTime, string? endTime) returns ShippingMethodList|error
List a site's shipping methods
Parameters
- ids string[]? (default ()) - Filter results by their IDs. Up to 200 IDs can be passed at once using commas as separators, e.g.
ids=h1at4d57xlmy,gyqgg0d3v9n1,jrsm5b4yefg6
. Important notes: * Theids
parameter cannot be used with any other ordering or filtering parameters (limit
,order
,sort
,begin_time
,end_time
, etc) * Invalid or unknown IDs will be ignored, so you should check that the results correspond to your request. * Records are returned in an arbitrary order. Since results are all returned at once you can sort the records yourself.
- 'limit int (default 20) - Limit number of records 1-200.
- 'order string (default "desc") - Sort order.
- sort string (default "created_at") - Sort field. You really only want to sort by
updated_at
in ascending order. In descending order updated records will move behind the cursor and could prevent some records from being returned.
- beginTime string? (default ()) - Inclusively filter by begin_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- endTime string? (default ()) - Inclusively filter by end_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
Return Type
- ShippingMethodList|error - A list of the site's shipping methods.
createShippingMethod
function createShippingMethod(ShippingMethodCreate payload) returns ShippingMethod|error
Create a new shipping method
Parameters
- payload ShippingMethodCreate -
Return Type
- ShippingMethod|error - A new shipping method.
getShippingMethod
function getShippingMethod(string shippingMethodId) returns ShippingMethod|error
Fetch a shipping method
Parameters
- shippingMethodId string - Shipping Method ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-usps_2-day
.
Return Type
- ShippingMethod|error - A shipping method.
updateShippingMethod
function updateShippingMethod(string shippingMethodId, ShippingMethodUpdate payload) returns ShippingMethod|error
Update an active Shipping Method
Parameters
- shippingMethodId string - Shipping Method ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-usps_2-day
.
- payload ShippingMethodUpdate -
Return Type
- ShippingMethod|error - The updated shipping method.
deactivateShippingMethod
function deactivateShippingMethod(string shippingMethodId) returns ShippingMethod|error
Deactivate a shipping method
Parameters
- shippingMethodId string - Shipping Method ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-usps_2-day
.
Return Type
- ShippingMethod|error - A shipping method.
listSubscriptions
function listSubscriptions(string[]? ids, int 'limit, string 'order, string sort, string? beginTime, string? endTime, string? state) returns SubscriptionList|error
List a site's subscriptions
Parameters
- ids string[]? (default ()) - Filter results by their IDs. Up to 200 IDs can be passed at once using commas as separators, e.g.
ids=h1at4d57xlmy,gyqgg0d3v9n1,jrsm5b4yefg6
. Important notes: * Theids
parameter cannot be used with any other ordering or filtering parameters (limit
,order
,sort
,begin_time
,end_time
, etc) * Invalid or unknown IDs will be ignored, so you should check that the results correspond to your request. * Records are returned in an arbitrary order. Since results are all returned at once you can sort the records yourself.
- 'limit int (default 20) - Limit number of records 1-200.
- 'order string (default "desc") - Sort order.
- sort string (default "created_at") - Sort field. You really only want to sort by
updated_at
in ascending order. In descending order updated records will move behind the cursor and could prevent some records from being returned.
- beginTime string? (default ()) - Inclusively filter by begin_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- endTime string? (default ()) - Inclusively filter by end_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- state string? (default ()) - Filter by state. - When
state=active
,state=canceled
,state=expired
, orstate=future
, subscriptions with states that match the query and only those subscriptions will be returned. - Whenstate=in_trial
, only subscriptions that have a trial_started_at date earlier than now and a trial_ends_at date later than now will be returned. - Whenstate=live
, only subscriptions that are in an active, canceled, or future state or are in trial will be returned.
Return Type
- SubscriptionList|error - A list of the site's subscriptions.
createSubscription
function createSubscription(SubscriptionCreate payload) returns Subscription|error
Create a new subscription
Parameters
- payload SubscriptionCreate -
Return Type
- Subscription|error - A subscription.
getSubscription
function getSubscription(string subscriptionId) returns Subscription|error
Fetch a subscription
Parameters
- subscriptionId string - Subscription ID or UUID. For ID no prefix is used e.g.
e28zov4fw0v2
. For UUID use prefixuuid-
, e.g.uuid-123457890
.
Return Type
- Subscription|error - A subscription.
updateSubscription
function updateSubscription(string subscriptionId, SubscriptionUpdate payload) returns Subscription|error
Update a subscription
Parameters
- subscriptionId string - Subscription ID or UUID. For ID no prefix is used e.g.
e28zov4fw0v2
. For UUID use prefixuuid-
, e.g.uuid-123457890
.
- payload SubscriptionUpdate -
Return Type
- Subscription|error - A subscription.
terminateSubscription
function terminateSubscription(string subscriptionId, string refund, boolean charge) returns Subscription|error
Terminate a subscription
Parameters
- subscriptionId string - Subscription ID or UUID. For ID no prefix is used e.g.
e28zov4fw0v2
. For UUID use prefixuuid-
, e.g.uuid-123457890
.
- refund string (default "none") - The type of refund to perform: *
full
- Performs a full refund of the last invoice for the current subscription term. *partial
- Prorates a refund based on the amount of time remaining in the current bill cycle. *none
- Terminates the subscription without a refund. In the event that the most recent invoice is a $0 invoice paid entirely by credit, Recurly will apply the credit back to the customer’s account. You may also terminate a subscription with no refund and then manually refund specific invoices.
- charge boolean (default true) - Applicable only if the subscription has usage based add-ons and unbilled usage logged for the current billing cycle. If true, current billing cycle unbilled usage is billed on the final invoice. If false, Recurly will create a negative usage record for current billing cycle usage that will zero out the final invoice line items.
Return Type
- Subscription|error - An expired subscription.
cancelSubscription
function cancelSubscription(string subscriptionId, SubscriptionCancel payload) returns Subscription|error
Cancel a subscription
Parameters
- subscriptionId string - Subscription ID or UUID. For ID no prefix is used e.g.
e28zov4fw0v2
. For UUID use prefixuuid-
, e.g.uuid-123457890
.
- payload SubscriptionCancel -
Return Type
- Subscription|error - A canceled or failed subscription.
reactivateSubscription
function reactivateSubscription(string subscriptionId) returns Subscription|error
Reactivate a canceled subscription
Parameters
- subscriptionId string - Subscription ID or UUID. For ID no prefix is used e.g.
e28zov4fw0v2
. For UUID use prefixuuid-
, e.g.uuid-123457890
.
Return Type
- Subscription|error - An active subscription.
pauseSubscription
function pauseSubscription(string subscriptionId, SubscriptionPause payload) returns Subscription|error
Pause subscription
Parameters
- subscriptionId string - Subscription ID or UUID. For ID no prefix is used e.g.
e28zov4fw0v2
. For UUID use prefixuuid-
, e.g.uuid-123457890
.
- payload SubscriptionPause -
Return Type
- Subscription|error - A subscription.
resumeSubscription
function resumeSubscription(string subscriptionId) returns Subscription|error
Resume subscription
Parameters
- subscriptionId string - Subscription ID or UUID. For ID no prefix is used e.g.
e28zov4fw0v2
. For UUID use prefixuuid-
, e.g.uuid-123457890
.
Return Type
- Subscription|error - A subscription.
convertTrial
function convertTrial(string subscriptionId) returns Subscription|error
Convert trial subscription
Parameters
- subscriptionId string - Subscription ID or UUID. For ID no prefix is used e.g.
e28zov4fw0v2
. For UUID use prefixuuid-
, e.g.uuid-123457890
.
Return Type
- Subscription|error - A subscription.
getPreviewRenewal
function getPreviewRenewal(string subscriptionId) returns InvoiceCollection|error
Fetch a preview of a subscription's renewal invoice(s)
Parameters
- subscriptionId string - Subscription ID or UUID. For ID no prefix is used e.g.
e28zov4fw0v2
. For UUID use prefixuuid-
, e.g.uuid-123457890
.
Return Type
- InvoiceCollection|error - A preview of the subscription's renewal invoice(s).
getSubscriptionChange
function getSubscriptionChange(string subscriptionId) returns SubscriptionChange|error
Fetch a subscription's pending change
Parameters
- subscriptionId string - Subscription ID or UUID. For ID no prefix is used e.g.
e28zov4fw0v2
. For UUID use prefixuuid-
, e.g.uuid-123457890
.
Return Type
- SubscriptionChange|error - A subscription's pending change.
createSubscriptionChange
function createSubscriptionChange(string subscriptionId, SubscriptionChangeCreate payload) returns SubscriptionChange|error
Create a new subscription change
Parameters
- subscriptionId string - Subscription ID or UUID. For ID no prefix is used e.g.
e28zov4fw0v2
. For UUID use prefixuuid-
, e.g.uuid-123457890
.
- payload SubscriptionChangeCreate -
Return Type
- SubscriptionChange|error - A subscription change.
removeSubscriptionChange
Delete the pending subscription change
Parameters
- subscriptionId string - Subscription ID or UUID. For ID no prefix is used e.g.
e28zov4fw0v2
. For UUID use prefixuuid-
, e.g.uuid-123457890
.
previewSubscriptionChange
function previewSubscriptionChange(string subscriptionId, SubscriptionChangeCreate payload) returns SubscriptionChange|error
Preview a new subscription change
Parameters
- subscriptionId string - Subscription ID or UUID. For ID no prefix is used e.g.
e28zov4fw0v2
. For UUID use prefixuuid-
, e.g.uuid-123457890
.
- payload SubscriptionChangeCreate -
Return Type
- SubscriptionChange|error - A subscription change.
listSubscriptionInvoices
function listSubscriptionInvoices(string subscriptionId, string[]? ids, int 'limit, string 'order, string sort, string? beginTime, string? endTime, string? 'type) returns InvoiceList|error
List a subscription's invoices
Parameters
- subscriptionId string - Subscription ID or UUID. For ID no prefix is used e.g.
e28zov4fw0v2
. For UUID use prefixuuid-
, e.g.uuid-123457890
.
- ids string[]? (default ()) - Filter results by their IDs. Up to 200 IDs can be passed at once using commas as separators, e.g.
ids=h1at4d57xlmy,gyqgg0d3v9n1,jrsm5b4yefg6
. Important notes: * Theids
parameter cannot be used with any other ordering or filtering parameters (limit
,order
,sort
,begin_time
,end_time
, etc) * Invalid or unknown IDs will be ignored, so you should check that the results correspond to your request. * Records are returned in an arbitrary order. Since results are all returned at once you can sort the records yourself.
- 'limit int (default 20) - Limit number of records 1-200.
- 'order string (default "desc") - Sort order.
- sort string (default "created_at") - Sort field. You really only want to sort by
updated_at
in ascending order. In descending order updated records will move behind the cursor and could prevent some records from being returned.
- beginTime string? (default ()) - Inclusively filter by begin_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- endTime string? (default ()) - Inclusively filter by end_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- 'type string? (default ()) - Filter by type when: -
type=charge
, only charge invoices will be returned. -type=credit
, only credit invoices will be returned. -type=non-legacy
, only charge and credit invoices will be returned. -type=legacy
, only legacy invoices will be returned.
Return Type
- InvoiceList|error - A list of the subscription's invoices.
listSubscriptionLineItems
function listSubscriptionLineItems(string subscriptionId, string[]? ids, int 'limit, string 'order, string sort, string? beginTime, string? endTime, string? original, string? state, string? 'type) returns LineItemList|error
List a subscription's line items
Parameters
- subscriptionId string - Subscription ID or UUID. For ID no prefix is used e.g.
e28zov4fw0v2
. For UUID use prefixuuid-
, e.g.uuid-123457890
.
- ids string[]? (default ()) - Filter results by their IDs. Up to 200 IDs can be passed at once using commas as separators, e.g.
ids=h1at4d57xlmy,gyqgg0d3v9n1,jrsm5b4yefg6
. Important notes: * Theids
parameter cannot be used with any other ordering or filtering parameters (limit
,order
,sort
,begin_time
,end_time
, etc) * Invalid or unknown IDs will be ignored, so you should check that the results correspond to your request. * Records are returned in an arbitrary order. Since results are all returned at once you can sort the records yourself.
- 'limit int (default 20) - Limit number of records 1-200.
- 'order string (default "desc") - Sort order.
- sort string (default "created_at") - Sort field. You really only want to sort by
updated_at
in ascending order. In descending order updated records will move behind the cursor and could prevent some records from being returned.
- beginTime string? (default ()) - Inclusively filter by begin_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- endTime string? (default ()) - Inclusively filter by end_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- original string? (default ()) - Filter by original field.
- state string? (default ()) - Filter by state field.
- 'type string? (default ()) - Filter by type field.
Return Type
- LineItemList|error - A list of the subscription's line items.
listSubscriptionCouponRedemptions
function listSubscriptionCouponRedemptions(string subscriptionId, string[]? ids, string sort, string? beginTime, string? endTime) returns CouponRedemptionList|error
Show the coupon redemptions for a subscription
Parameters
- subscriptionId string - Subscription ID or UUID. For ID no prefix is used e.g.
e28zov4fw0v2
. For UUID use prefixuuid-
, e.g.uuid-123457890
.
- ids string[]? (default ()) - Filter results by their IDs. Up to 200 IDs can be passed at once using commas as separators, e.g.
ids=h1at4d57xlmy,gyqgg0d3v9n1,jrsm5b4yefg6
. Important notes: * Theids
parameter cannot be used with any other ordering or filtering parameters (limit
,order
,sort
,begin_time
,end_time
, etc) * Invalid or unknown IDs will be ignored, so you should check that the results correspond to your request. * Records are returned in an arbitrary order. Since results are all returned at once you can sort the records yourself.
- sort string (default "created_at") - Sort field. You really only want to sort by
updated_at
in ascending order. In descending order updated records will move behind the cursor and could prevent some records from being returned.
- beginTime string? (default ()) - Inclusively filter by begin_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- endTime string? (default ()) - Inclusively filter by end_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
Return Type
- CouponRedemptionList|error - A list of the the coupon redemptions on a subscription.
listUsage
function listUsage(string subscriptionId, string addOnId, string[]? ids, int 'limit, string 'order, string sort, string? beginTime, string? endTime, string billingStatus) returns UsageList|error
List a subscription add-on's usage records
Parameters
- subscriptionId string - Subscription ID or UUID. For ID no prefix is used e.g.
e28zov4fw0v2
. For UUID use prefixuuid-
, e.g.uuid-123457890
.
- addOnId string - Add-on ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-gold
.
- ids string[]? (default ()) - Filter results by their IDs. Up to 200 IDs can be passed at once using commas as separators, e.g.
ids=h1at4d57xlmy,gyqgg0d3v9n1,jrsm5b4yefg6
. Important notes: * Theids
parameter cannot be used with any other ordering or filtering parameters (limit
,order
,sort
,begin_time
,end_time
, etc) * Invalid or unknown IDs will be ignored, so you should check that the results correspond to your request. * Records are returned in an arbitrary order. Since results are all returned at once you can sort the records yourself.
- 'limit int (default 20) - Limit number of records 1-200.
- 'order string (default "desc") - Sort order.
- sort string (default "usage_timestamp") - Sort field. You really only want to sort by
usage_timestamp
in ascending order. In descending order updated records will move behind the cursor and could prevent some records from being returned.
- beginTime string? (default ()) - Inclusively filter by begin_time when
sort=usage_timestamp
orsort=recorded_timestamp
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- endTime string? (default ()) - Inclusively filter by end_time when
sort=usage_timestamp
orsort=recorded_timestamp
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- billingStatus string (default "unbilled") - Filter by usage record's billing status
createUsage
function createUsage(string subscriptionId, string addOnId, UsageCreate payload) returns Usage|error
Log a usage record on this subscription add-on
Parameters
- subscriptionId string - Subscription ID or UUID. For ID no prefix is used e.g.
e28zov4fw0v2
. For UUID use prefixuuid-
, e.g.uuid-123457890
.
- addOnId string - Add-on ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-gold
.
- payload UsageCreate -
getUsage
Get a usage record
Parameters
- usageId string - Usage Record ID.
updateUsage
function updateUsage(string usageId, UsageCreate payload) returns Usage|error
Update a usage record
removeUsage
Delete a usage record.
Parameters
- usageId string - Usage Record ID.
listTransactions
function listTransactions(string[]? ids, int 'limit, string 'order, string sort, string? beginTime, string? endTime, string? 'type, string? success) returns TransactionList|error
List a site's transactions
Parameters
- ids string[]? (default ()) - Filter results by their IDs. Up to 200 IDs can be passed at once using commas as separators, e.g.
ids=h1at4d57xlmy,gyqgg0d3v9n1,jrsm5b4yefg6
. Important notes: * Theids
parameter cannot be used with any other ordering or filtering parameters (limit
,order
,sort
,begin_time
,end_time
, etc) * Invalid or unknown IDs will be ignored, so you should check that the results correspond to your request. * Records are returned in an arbitrary order. Since results are all returned at once you can sort the records yourself.
- 'limit int (default 20) - Limit number of records 1-200.
- 'order string (default "desc") - Sort order.
- sort string (default "created_at") - Sort field. You really only want to sort by
updated_at
in ascending order. In descending order updated records will move behind the cursor and could prevent some records from being returned.
- beginTime string? (default ()) - Inclusively filter by begin_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- endTime string? (default ()) - Inclusively filter by end_time when
sort=created_at
orsort=updated_at
. Note: this value is an ISO8601 timestamp. A partial timestamp that does not include a time zone will default to UTC.
- 'type string? (default ()) - Filter by type field. The value
payment
will return bothpurchase
andcapture
transactions.
- success string? (default ()) - Filter by success field.
Return Type
- TransactionList|error - A list of the site's transactions.
getTransaction
function getTransaction(string transactionId) returns Transaction|error
Fetch a transaction
Parameters
- transactionId string - Transaction ID or UUID. For ID no prefix is used e.g.
e28zov4fw0v2
. For UUID use prefixuuid-
, e.g.uuid-123457890
.
Return Type
- Transaction|error - A transaction.
getUniqueCouponCode
function getUniqueCouponCode(string uniqueCouponCodeId) returns UniqueCouponCode|error
Fetch a unique coupon code
Parameters
- uniqueCouponCodeId string - Unique Coupon Code ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-abc-8dh2-def
.
Return Type
- UniqueCouponCode|error - A unique coupon code.
deactivateUniqueCouponCode
function deactivateUniqueCouponCode(string uniqueCouponCodeId) returns UniqueCouponCode|error
Deactivate a unique coupon code
Parameters
- uniqueCouponCodeId string - Unique Coupon Code ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-abc-8dh2-def
.
Return Type
- UniqueCouponCode|error - A unique coupon code.
reactivateUniqueCouponCode
function reactivateUniqueCouponCode(string uniqueCouponCodeId) returns UniqueCouponCode|error
Restore a unique coupon code
Parameters
- uniqueCouponCodeId string - Unique Coupon Code ID or code. For ID no prefix is used e.g.
e28zov4fw0v2
. For code use prefixcode-
, e.g.code-abc-8dh2-def
.
Return Type
- UniqueCouponCode|error - A unique coupon code.
createPurchase
function createPurchase(PurchaseCreate payload) returns InvoiceCollection|error
Create a new purchase
Parameters
- payload PurchaseCreate -
Return Type
- InvoiceCollection|error - Returns the new invoices
previewPurchase
function previewPurchase(PurchaseCreate payload) returns InvoiceCollection|error
Preview a new purchase
Parameters
- payload PurchaseCreate -
Return Type
- InvoiceCollection|error - Returns preview of the new invoices
getExportDates
function getExportDates() returns ExportDates|error
List the dates that have an available export to download.
Return Type
- ExportDates|error - Returns a list of dates.
getExportFiles
function getExportFiles(string exportDate) returns ExportFiles|error
List of the export files that are available to download.
Parameters
- exportDate string - Date for which to get a list of available automated export files. Date must be in YYYY-MM-DD format.
Return Type
- ExportFiles|error - Returns a list of export files to download.
Records
recurly: Account
Fields
- Fields Included from *AccountReadOnly
- id string
- object string
- state string
- hosted_login_token string
- shipping_addresses ShippingAddress[]
- has_live_subscription boolean
- has_active_subscription boolean
- has_future_subscription boolean
- has_canceled_subscription boolean
- has_paused_subscription boolean
- has_past_due_invoice boolean
- created_at string
- updated_at string
- deleted_at string
- anydata...
- Fields Included from *AccountResponse
- code string
- username string
- email string
- preferred_locale string
- cc_emails string
- first_name string
- last_name string
- company string
- vat_number string
- tax_exempt boolean
- exemption_certificate string
- parent_account_id string
- bill_to string
- address Address
- billing_info BillingInfo
- custom_fields CustomFields
- anydata...
recurly: AccountAcquisition
Fields
- Fields Included from *AccountAcquisitionUpdate
- Fields Included from *AccountAcquisitionReadOnly
- id string
- object string
- account AccountMini
- created_at string
- updated_at string
- anydata...
recurly: AccountAcquisitionList
Fields
- 'object string? - Will always be List.
- has_more boolean? - Indicates there are more results on subsequent pages.
- next string? - Path to subsequent page of results.
- data AccountAcquisition[]? -
recurly: AccountAcquisitionReadOnly
Fields
- id string? -
- 'object string? -
- account AccountMini? -
- created_at string? - When the account acquisition data was created.
- updated_at string? - When the account acquisition data was last changed.
recurly: AccountAcquisitionUpdate
Fields
- channel string? - The channel through which the account was acquired.
- subchannel string? - An arbitrary subchannel string representing a distinction/subcategory within a broader channel.
- campaign string? - An arbitrary identifier for the marketing campaign that led to the acquisition of this account.
recurly: AccountBalance
Fields
- 'object string? -
- account AccountMini? -
- past_due boolean? -
- balances AccountBalanceAmount[]? -
recurly: AccountBalanceAmount
Fields
- currency string? - 3-letter ISO 4217 currency code.
- amount float? - Total amount the account is past due.
recurly: AccountCreate
Fields
- code string - The unique identifier of the account. This cannot be changed once the account is created.
- acquisition AccountAcquisitionUpdate? -
- shipping_addresses ShippingAddressCreate[]? -
- Fields Included from *AccountUpdate
- username string
- email string
- preferred_locale string
- cc_emails string
- first_name string
- last_name string
- company string
- vat_number string
- tax_exempt boolean
- exemption_certificate string
- parent_account_code string
- parent_account_id string
- bill_to string
- transaction_type string
- address Address
- billing_info BillingInfoCreate
- custom_fields CustomFields
- anydata...
recurly: AccountList
Fields
- 'object string? - Will always be List.
- has_more boolean? - Indicates there are more results on subsequent pages.
- next string? - Path to subsequent page of results.
- data Account[]? -
recurly: AccountMini
Fields
- id string? -
- 'object string? -
- code string? - The unique identifier of the account.
- email string? - The email address used for communicating with this customer.
- first_name string? -
- last_name string? -
- company string? -
- parent_account_id string? -
- bill_to string? -
recurly: AccountNote
Fields
- id string? -
- 'object string? -
- account_id string? -
- user User? -
- message string -
- created_at string? -
recurly: AccountNoteList
Fields
- 'object string? - Will always be List.
- has_more boolean? - Indicates there are more results on subsequent pages.
- next string? - Path to subsequent page of results.
- data AccountNote[]? -
recurly: AccountPurchase
Fields
- id string? - Optional, but if present allows an existing account to be used and updated as part of the purchase.
- code string - The unique identifier of the account. This cannot be changed once the account is created.
- acquisition AccountAcquisitionUpdate? -
- Fields Included from *AccountUpdate
- username string
- email string
- preferred_locale string
- cc_emails string
- first_name string
- last_name string
- company string
- vat_number string
- tax_exempt boolean
- exemption_certificate string
- parent_account_code string
- parent_account_id string
- bill_to string
- transaction_type string
- address Address
- billing_info BillingInfoCreate
- custom_fields CustomFields
- anydata...
recurly: AccountReadOnly
Fields
- id string? -
- 'object string? -
- state string? - Accounts can be either active or inactive.
- hosted_login_token string? - The unique token for automatically logging the account in to the hosted management pages. You may automatically log the user into their hosted management pages by directing the user to:
https://{subdomain}.recurly.com/account/{hosted_login_token}
.
- shipping_addresses ShippingAddress[]? - The shipping addresses on the account.
- has_live_subscription boolean? - Indicates if the account has a subscription that is either active, canceled, future, or paused.
- has_active_subscription boolean? - Indicates if the account has an active subscription.
- has_future_subscription boolean? - Indicates if the account has a future subscription.
- has_canceled_subscription boolean? - Indicates if the account has a canceled subscription.
- has_paused_subscription boolean? - Indicates if the account has a paused subscription.
- has_past_due_invoice boolean? - Indicates if the account has a past due invoice.
- created_at string? - When the account was created.
- updated_at string? - When the account was last changed.
- deleted_at string? - If present, when the account was last marked inactive.
recurly: AccountResponse
Fields
- code string? - The unique identifier of the account. This cannot be changed once the account is created.
- username string? - A secondary value for the account.
- email string? - The email address used for communicating with this customer. The customer will also use this email address to log into your hosted account management pages. This value does not need to be unique.
- preferred_locale string? - Used to determine the language and locale of emails sent on behalf of the merchant to the customer.
- cc_emails string? - Additional email address that should receive account correspondence. These should be separated only by commas. These CC emails will receive all emails that the
email
field also receives.
- first_name string? -
- last_name string? -
- company string? -
- vat_number string? - The VAT number of the account (to avoid having the VAT applied). This is only used for manually collected invoices.
- tax_exempt boolean? - The tax status of the account.
true
exempts tax on the account,false
applies tax on the account.
- exemption_certificate string? - The tax exemption certificate number for the account. If the merchant has an integration for the Vertex tax provider, this optional value will be sent in any tax calculation requests for the account.
- parent_account_id string? - The UUID of the parent account associated with this account.
- bill_to string? - An enumerable describing the billing behavior of the account, specifically whether the account is self-paying or will rely on the parent account to pay.
- address Address? -
- billing_info BillingInfo? -
- custom_fields CustomFields? - The custom fields will only be altered when they are included in a request. Sending an empty array will not remove any existing values. To remove a field send the name with a null or empty value.
recurly: AccountUpdate
Fields
- username string? - A secondary value for the account.
- email string? - The email address used for communicating with this customer. The customer will also use this email address to log into your hosted account management pages. This value does not need to be unique.
- preferred_locale string? - Used to determine the language and locale of emails sent on behalf of the merchant to the customer. The list of locales is restricted to those the merchant has enabled on the site.
- cc_emails string? - Additional email address that should receive account correspondence. These should be separated only by commas. These CC emails will receive all emails that the
email
field also receives.
- first_name string? -
- last_name string? -
- company string? -
- vat_number string? - The VAT number of the account (to avoid having the VAT applied). This is only used for manually collected invoices.
- tax_exempt boolean? - The tax status of the account.
true
exempts tax on the account,false
applies tax on the account.
- exemption_certificate string? - The tax exemption certificate number for the account. If the merchant has an integration for the Vertex tax provider, this optional value will be sent in any tax calculation requests for the account.
- parent_account_code string? - The account code of the parent account to be associated with this account. Passing an empty value removes any existing parent association from this account. If both
parent_account_code
andparent_account_id
are passed, the non-blank value inparent_account_id
will be used. Only one level of parent child relationship is allowed. You cannot assign a parent account that itself has a parent account.
- parent_account_id string? - The UUID of the parent account to be associated with this account. Passing an empty value removes any existing parent association from this account. If both
parent_account_code
andparent_account_id
are passed, the non-blank value inparent_account_id
will be used. Only one level of parent child relationship is allowed. You cannot assign a parent account that itself has a parent account.
- bill_to string? - An enumerable describing the billing behavior of the account, specifically whether the account is self-paying or will rely on the parent account to pay.
- transaction_type string? - An optional type designation for the payment gateway transaction created by this request. Supports 'moto' value, which is the acronym for mail order and telephone transactions.
- address Address? -
- billing_info BillingInfoCreate? -
- custom_fields CustomFields? - The custom fields will only be altered when they are included in a request. Sending an empty array will not remove any existing values. To remove a field send the name with a null or empty value.
recurly: AddOn
Full add-on details.
Fields
- id string? -
- 'object string? -
- plan_id string? -
- code string - The unique identifier for the add-on within its plan.
- state string? - Add-ons can be either active or inactive.
- name string - Describes your add-on and will appear in subscribers' invoices.
- add_on_type string? - Whether the add-on type is fixed, or usage-based.
- usage_type string? - Type of usage, returns usage type if
add_on_type
isusage
.
- usage_percentage float? - The percentage taken of the monetary amount of usage tracked. This can be up to 4 decimal places. A value between 0.0 and 100.0.
- measured_unit_id string? - System-generated unique identifier for an measured unit associated with the add-on.
- accounting_code string? - Accounting code for invoice line items for this add-on. If no value is provided, it defaults to add-on's code.
- revenue_schedule_type string? - When this add-on is invoiced, the line item will use this revenue schedule. If
item_code
/item_id
is part of the request thenrevenue_schedule_type
must be absent in the request as the value will be set from the item.
- avalara_transaction_type int? - Used by Avalara for Communications taxes. The transaction type in combination with the service type describe how the add-on is taxed. Refer to the documentation for more available t/s types.
- avalara_service_type int? - Used by Avalara for Communications taxes. The transaction type in combination with the service type describe how the add-on is taxed. Refer to the documentation for more available t/s types.
- tax_code string? - Used by Avalara, Vertex, and Recurly’s EU VAT tax feature. The tax code values are specific to each tax system. If you are using Recurly’s EU VAT feature you can use
unknown
,physical
, ordigital
.
- display_quantity boolean(default false) - Determines if the quantity field is displayed on the hosted pages for the add-on.
- default_quantity int(default 1) - Default quantity for the hosted pages.
- optional boolean? - Whether the add-on is optional for the customer to include in their purchase on the hosted payment page. If false, the add-on will be included when a subscription is created through the Recurly UI. However, the add-on will not be included when a subscription is created through the API.
- currencies AddOnPricing[]? -
- item ItemMini? - Just the important parts.
- tier_type string(default "flat") - The pricing model for the add-on. For more information, click here. See our Guide for an overview of how to configure quantity-based pricing models.
- tiers Tier[]? -
- external_sku string? - Optional, stock keeping unit to link the item to other inventory systems.
- created_at string? -
- updated_at string? -
- deleted_at string? -
recurly: AddOnCreate
Full add-on details.
Fields
- item_code string? - Unique code to identify an item. Available when the
Credit Invoices
andSubscription Billing Terms
features are enabled. Ifitem_id
anditem_code
are both present,item_id
will be used.
- item_id string? - System-generated unique identifier for an item. Available when the
Credit Invoices
andSubscription Billing Terms
features are enabled. Ifitem_id
anditem_code
are both present,item_id
will be used.
- code string - The unique identifier for the add-on within its plan. If
item_code
/item_id
is part of the request thencode
must be absent. Ifitem_code
/item_id
is not presentcode
is required.
- name string - Describes your add-on and will appear in subscribers' invoices. If
item_code
/item_id
is part of the request thenname
must be absent. Ifitem_code
/item_id
is not presentname
is required.
- add_on_type string(default "fixed") - Whether the add-on type is fixed, or usage-based.
- usage_percentage float? - The percentage taken of the monetary amount of usage tracked. This can be up to 4 decimal places. A value between 0.0 and 100.0. Required if
add_on_type
is usage,tier_type
isflat
andusage_type
is percentage. Must be omitted otherwise.
- measured_unit_id string? - System-generated unique identifier for a measured unit to be associated with the add-on. Either
measured_unit_id
ormeasured_unit_name
are required whenadd_on_type
isusage
. Ifmeasured_unit_id
andmeasured_unit_name
are both present,measured_unit_id
will be used.
- measured_unit_name string? - Name of a measured unit to be associated with the add-on. Either
measured_unit_id
ormeasured_unit_name
are required whenadd_on_type
isusage
. Ifmeasured_unit_id
andmeasured_unit_name
are both present,measured_unit_id
will be used.
- plan_id string? -
- accounting_code string? - Accounting code for invoice line items for this add-on. If no value is provided, it defaults to add-on's code. If
item_code
/item_id
is part of the request thenaccounting_code
must be absent.
- revenue_schedule_type string? - When this add-on is invoiced, the line item will use this revenue schedule. If
item_code
/item_id
is part of the request thenrevenue_schedule_type
must be absent in the request as the value will be set from the item.
- display_quantity boolean(default false) - Determines if the quantity field is displayed on the hosted pages for the add-on.
- default_quantity int(default 1) - Default quantity for the hosted pages.
- optional boolean? - Whether the add-on is optional for the customer to include in their purchase on the hosted payment page. If false, the add-on will be included when a subscription is created through the Recurly UI. However, the add-on will not be included when a subscription is created through the API.
- avalara_transaction_type int? - Used by Avalara for Communications taxes. The transaction type in combination with the service type describe how the add-on is taxed. Refer to the documentation for more available t/s types. If an
Item
is associated to theAddOn
, then theavalara_transaction_type
must be absent.
- avalara_service_type int? - Used by Avalara for Communications taxes. The transaction type in combination with the service type describe how the add-on is taxed. Refer to the documentation for more available t/s types. If an
Item
is associated to theAddOn
, then theavalara_service_type
must be absent.
- tax_code string? - Optional field used by Avalara, Vertex, and Recurly's EU VAT tax feature to determine taxation rules. If you have your own AvaTax or Vertex account configured, use their tax codes to assign specific tax rules. If you are using Recurly's EU VAT feature, you can use values of
unknown
,physical
, ordigital
. Ifitem_code
/item_id
is part of the request thentax_code
must be absent.
- currencies AddOnPricing[]? -
- If
item_code
/item_id
is part of the request and the item has a default currency thencurrencies
is optional. If the item does not have a default currency, thencurrencies
is required. Ifitem_code
/item_id
is not presentcurrencies
is required. - If the add-on's
tier_type
istiered
,volume
, orstairstep
, thencurrencies
must be absent. - Must be absent if
add_on_type
isusage
andusage_type
ispercentage
.
- If
- tier_type string(default "flat") - The pricing model for the add-on. For more information, click here. See our Guide for an overview of how to configure quantity-based pricing models.
- tiers Tier[]? - If the tier_type is
flat
, thentiers
must be absent. Thetiers
object must include one to many tiers withending_quantity
andunit_amount
for the desiredcurrencies
, or alternatively,usage_percentage
for usage percentage type usage add ons. There must be one tier with anending_quantity
of 999999999 which is the default if not provided.
recurly: AddOnList
Fields
- 'object string? - Will always be List.
- has_more boolean? - Indicates there are more results on subsequent pages.
- next string? - Path to subsequent page of results.
- data AddOn[]? -
recurly: AddOnMini
Just the important parts.
Fields
- id string? -
- 'object string? -
- code string? - The unique identifier for the add-on within its plan.
- name string? - Describes your add-on and will appear in subscribers' invoices.
- add_on_type string? - Whether the add-on type is fixed, or usage-based.
- usage_type string? - Type of usage, returns usage type if
add_on_type
isusage
.
- usage_percentage float? - The percentage taken of the monetary amount of usage tracked. This can be up to 4 decimal places. A value between 0.0 and 100.0.
- measured_unit_id string? - System-generated unique identifier for an measured unit associated with the add-on.
- item_id string? -
- external_sku string? - Optional, stock keeping unit to link the item to other inventory systems.
- accounting_code string? - Accounting code for invoice line items for this add-on. If no value is provided, it defaults to add-on's code.
recurly: AddOnPricing
Fields
- currency string - 3-letter ISO 4217 currency code.
- unit_amount float? - Allows up to 2 decimal places. Required unless
unit_amount_decimal
is provided.
- unit_amount_decimal string? - Allows up to 9 decimal places. Only supported when
add_on_type
=usage
. Ifunit_amount_decimal
is provided,unit_amount
cannot be provided.
recurly: AddOnUpdate
Full add-on details.
Fields
- id string? -
- code string? - The unique identifier for the add-on within its plan. If an
Item
is associated to theAddOn
thencode
must be absent.
- name string? - Describes your add-on and will appear in subscribers' invoices. If an
Item
is associated to theAddOn
thenname
must be absent.
- usage_percentage float? - The percentage taken of the monetary amount of usage tracked. This can be up to 4 decimal places. A value between 0.0 and 100.0. Required if
add_on_type
is usage,tier_type
isflat
andusage_type
is percentage. Must be omitted otherwise.
- measured_unit_id string? - System-generated unique identifier for a measured unit to be associated with the add-on. Either
measured_unit_id
ormeasured_unit_name
are required whenadd_on_type
isusage
. Ifmeasured_unit_id
andmeasured_unit_name
are both present,measured_unit_id
will be used.
- measured_unit_name string? - Name of a measured unit to be associated with the add-on. Either
measured_unit_id
ormeasured_unit_name
are required whenadd_on_type
isusage
. Ifmeasured_unit_id
andmeasured_unit_name
are both present,measured_unit_id
will be used.
- accounting_code string? - Accounting code for invoice line items for this add-on. If no value is provided, it defaults to add-on's code. If an
Item
is associated to theAddOn
thenaccounting code
must be absent.
- revenue_schedule_type string? - When this add-on is invoiced, the line item will use this revenue schedule. If
item_code
/item_id
is part of the request thenrevenue_schedule_type
must be absent in the request as the value will be set from the item.
- avalara_transaction_type int? - Used by Avalara for Communications taxes. The transaction type in combination with the service type describe how the add-on is taxed. Refer to the documentation for more available t/s types. If an
Item
is associated to theAddOn
, then theavalara_transaction_type
must be absent.
- avalara_service_type int? - Used by Avalara for Communications taxes. The transaction type in combination with the service type describe how the add-on is taxed. Refer to the documentation for more available t/s types. If an
Item
is associated to theAddOn
, then theavalara_service_type
must be absent.
- tax_code string? - Optional field used by Avalara, Vertex, and Recurly's EU VAT tax feature to determine taxation rules. If you have your own AvaTax or Vertex account configured, use their tax codes to assign specific tax rules. If you are using Recurly's EU VAT feature, you can use values of
unknown
,physical
, ordigital
. If anItem
is associated to theAddOn
thentax code
must be absent.
- display_quantity boolean? - Determines if the quantity field is displayed on the hosted pages for the add-on.
- default_quantity int? - Default quantity for the hosted pages.
- optional boolean? - Whether the add-on is optional for the customer to include in their purchase on the hosted payment page. If false, the add-on will be included when a subscription is created through the Recurly UI. However, the add-on will not be included when a subscription is created through the API.
- currencies AddOnPricing[]? - If the add-on's
tier_type
istiered
,volume
, orstairstep
, then currencies must be absent. Must also be absent ifadd_on_type
isusage
andusage_type
ispercentage
.
- tiers Tier[]? - If the tier_type is
flat
, thentiers
must be absent. Thetiers
object must include one to many tiers withending_quantity
andunit_amount
for the desiredcurrencies
, or alternatively,usage_percentage
for usage percentage type usage add ons. There must be one tier with anending_quantity
of 999999999 which is the default if not provided.
recurly: Address
Fields
- phone string? -
- street1 string? -
- street2 string? -
- city string? -
- region string? - State or province.
- postal_code string? - Zip or postal code.
- country string? - Country, 2-letter ISO 3166-1 alpha-2 code.
recurly: BillingInfo
Fields
- id string? -
- 'object string? -
- account_id string? -
- first_name string? -
- last_name string? -
- company string? -
- address Address? -
- vat_number string? - Customer's VAT number (to avoid having the VAT applied). This is only used for automatically collected invoices.
- valid boolean? -
- payment_method PaymentMethod? -
- fraud FraudInformation? - Most recent fraud result.
- primary_payment_method boolean? - The
primary_payment_method
field is used to indicate the primary billing info on the account. The first billing info created on an account will always become primary. This payment method will be used
- backup_payment_method boolean? - The
backup_payment_method
field is used to indicate a billing info as a backup on the account that will be tried if the initial billing info used for an invoice is declined.
- created_at string? - When the billing information was created.
- updated_at string? - When the billing information was last changed.
- updated_by BillinginfoUpdatedBy? -
recurly: BillingInfoCreate
Fields
- token_id string? - A token generated by Recurly.js.
- first_name string? -
- last_name string? -
- company string? -
- address Address? -
- number string? - Credit card number, spaces and dashes are accepted.
- month string? -
- year string? -
- cvv string? - STRONGLY RECOMMENDED
- vat_number string? -
- ip_address string? - STRONGLY RECOMMENDED Customer's IP address when updating their billing information.
- gateway_token string? -
- gateway_code string? -
- amazon_billing_agreement_id string? -
- paypal_billing_agreement_id string? -
- fraud_session_id string? -
- transaction_type string? - An optional type designation for the payment gateway transaction created by this request. Supports 'moto' value, which is the acronym for mail order and telephone transactions.
- three_d_secure_action_result_token_id string? - A token generated by Recurly.js after completing a 3-D Secure device fingerprinting or authentication challenge.
- iban string? - The International Bank Account Number, up to 34 alphanumeric characters comprising a country code; two check digits; and a number that includes the domestic bank account number, branch identifier, and potential routing information
- tax_identifier string? - Tax identifier is required if adding a billing info that is a consumer card in Brazil or in Argentina. This would be the customer's CPF (Brazil) and CUIT (Argentina). CPF and CUIT are tax identifiers for all residents who pay taxes in Brazil and Argentina respectively.
- tax_identifier_type string? - This field and a value of
cpf
orcuit
are required if adding a billing info that is an elo or hipercard type in Brazil or in Argentina.
- primary_payment_method boolean? - The
primary_payment_method
field is used to designate the primary billing info on the account. The first billing info created on an account will always become primary. Adding additional billing infos provides the flexibility to mark another billing info as primary, or adding additional non-primary billing infos. This can be accomplished by passing theprimary_payment_method
with a value oftrue
. When adding billing infos via the billing_info and /accounts endpoints, this value is not permitted, and will return an error if provided.
- backup_payment_method boolean? - The
backup_payment_method
field is used to designate a billing info as a backup on the account that will be tried if the initial billing info used for an invoice is declined. All payment methods, including the billing info markedprimary_payment_method
can be set as a backup. An account can have a maximum of 1 backup, if a user sets a different payment method as a backup, the existing backup will no longer be marked as such.
recurly: BillingInfoList
Fields
- 'object string? - Will always be List.
- has_more boolean? - Indicates there are more results on subsequent pages.
- next string? - Path to subsequent page of results.
- data BillingInfo[]? -
recurly: BillinginfoUpdatedBy
Fields
- ip string? - Customer's IP address when updating their billing information.
- country string? - Country, 2-letter ISO 3166-1 alpha-2 code matching the origin IP address, if known by Recurly.
recurly: BillingInfoVerify
Fields
- gateway_code string? - An identifier for a specific payment gateway.
recurly: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
recurly: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth CredentialsConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
recurly: Coupon
Fields
- id string? -
- 'object string? -
- code string? - The code the customer enters to redeem the coupon.
- name string? - The internal name for the coupon.
- state string? - Indicates if the coupon is redeemable, and if it is not, why.
- max_redemptions int? - A maximum number of redemptions for the coupon. The coupon will expire when it hits its maximum redemptions.
- max_redemptions_per_account int? - Redemptions per account is the number of times a specific account can redeem the coupon. Set redemptions per account to
1
if you want to keep customers from gaming the system and getting more than one discount from the coupon campaign.
- unique_coupon_codes_count int? - When this number reaches
max_redemptions
the coupon will no longer be redeemable.
- unique_code_template string? - On a bulk coupon, the template from which unique coupon codes are generated.
- unique_coupon_code UniqueCouponCode? - Will be populated when the Coupon being returned is a
UniqueCouponCode
.
- duration string? -
- "single_use" coupons applies to the first invoice only.
- "temporal" coupons will apply to invoices for the duration determined by the
temporal_unit
andtemporal_amount
attributes.
- temporal_amount int? - If
duration
is "temporal" thantemporal_amount
is an integer which is multiplied bytemporal_unit
to define the duration that the coupon will be applied to invoices for.
- temporal_unit string? - If
duration
is "temporal" thantemporal_unit
is multiplied bytemporal_amount
to define the duration that the coupon will be applied to invoices for.
- free_trial_unit string? - Description of the unit of time the coupon is for. Used with
free_trial_amount
to determine the duration of time the coupon is for.
- free_trial_amount int? - Sets the duration of time the
free_trial_unit
is for.
- applies_to_all_plans boolean? - The coupon is valid for all plans if true. If false then
plans
will list the applicable plans.
- applies_to_all_items boolean? - The coupon is valid for all items if true. If false then
items
will list the applicable items.
- applies_to_non_plan_charges boolean? - The coupon is valid for one-time, non-plan charges if true.
- plans PlanMini[]? - A list of plans for which this coupon applies. This will be
null
ifapplies_to_all_plans=true
.
- items ItemMini[]? - A list of items for which this coupon applies. This will be
null
ifapplies_to_all_items=true
.
- redemption_resource string? - Whether the discount is for all eligible charges on the account, or only a specific subscription.
- discount CouponDiscount? - Details of the discount a coupon applies. Will contain a
type
property and one of the following properties:percent
,fixed
,trial
.
- coupon_type string? - Whether the coupon is "single_code" or "bulk". Bulk coupons will require a
unique_code_template
and will generate unique codes through the/generate
endpoint.
- hosted_page_description string? - This description will show up when a customer redeems a coupon on your Hosted Payment Pages, or if you choose to show the description on your own checkout page.
- invoice_description string? - Description of the coupon on the invoice.
- redeem_by string? - The date and time the coupon will expire and can no longer be redeemed. Time is always 11:59:59, the end-of-day Pacific time.
- created_at string? -
- updated_at string? -
- expired_at string? - The date and time the coupon was expired early or reached its
max_redemptions
.
recurly: CouponBulkCreate
Fields
- number_of_unique_codes int? - The quantity of unique coupon codes to generate
recurly: CouponCreate
Fields
- Fields Included from *CouponUpdate
- code string - The code the customer enters to redeem the coupon.
- discount_type string - The type of discount provided by the coupon (how the amount discounted is calculated)
- discount_percent int? - The percent of the price discounted by the coupon. Required if
discount_type
ispercent
.
- free_trial_unit string? - Description of the unit of time the coupon is for. Used with
free_trial_amount
to determine the duration of time the coupon is for. Required ifdiscount_type
isfree_trial
.
- free_trial_amount int? - Sets the duration of time the
free_trial_unit
is for. Required ifdiscount_type
isfree_trial
.
- currencies CouponPricing[]? - Fixed discount currencies by currency. Required if the coupon type is
fixed
. This parameter should contain the coupon discount values
- applies_to_non_plan_charges boolean(default false) - The coupon is valid for one-time, non-plan charges if true.
- applies_to_all_plans boolean(default true) - The coupon is valid for all plans if true. If false then
plans
will list the applicable plans.
- applies_to_all_items boolean(default false) - To apply coupon to Items in your Catalog, include a list
of
item_codes
in the request that the coupon will apply to. Or set value to true to apply to all Items in your Catalog. The following values are not permitted whenapplies_to_all_items
is included:free_trial_amount
andfree_trial_unit
.
- plan_codes string[]? - List of plan codes to which this coupon applies. Required
if
applies_to_all_plans
is false. Overridesapplies_to_all_plans
whenapplies_to_all_plans
is true.
- item_codes string[]? - List of item codes to which this coupon applies. Sending
item_codes
is only permitted whenapplies_to_all_items
is set to false. The following values are not permitted whenitem_codes
is included:free_trial_amount
andfree_trial_unit
.
- duration string(default "forever") - This field does not apply when the discount_type is
free_trial
.- "single_use" coupons applies to the first invoice only.
- "temporal" coupons will apply to invoices for the duration determined by the
temporal_unit
andtemporal_amount
attributes. - "forever" coupons will apply to invoices forever.
- temporal_amount int? - If
duration
is "temporal" thantemporal_amount
is an integer which is multiplied bytemporal_unit
to define the duration that the coupon will be applied to invoices for.
- temporal_unit string? - If
duration
is "temporal" thantemporal_unit
is multiplied bytemporal_amount
to define the duration that the coupon will be applied to invoices for.
- coupon_type string? - Whether the coupon is "single_code" or "bulk". Bulk coupons will require a
unique_code_template
and will generate unique codes through the/generate
endpoint.
- unique_code_template string? - On a bulk coupon, the template from which unique coupon codes are generated.
- You must start the template with your coupon_code wrapped in single quotes.
- Outside of single quotes, use a 9 for a character that you want to be a random number.
- Outside of single quotes, use an "x" for a character that you want to be a random letter.
- Outside of single quotes, use an * for a character that you want to be a random number or letter.
- Use single quotes ' ' for characters that you want to remain static. These strings can be alphanumeric and may contain a - _ or +. For example: "'abc-'****'-def'"
- redemption_resource string(default "account") - Whether the discount is for all eligible charges on the account, or only a specific subscription.
recurly: CouponDiscount
Details of the discount a coupon applies. Will contain a type
property and one of the following properties: percent
, fixed
, trial
.
Fields
- 'type string? -
- percent int? - This is only present when
type=percent
.
- currencies CouponDiscountPricing[]? - This is only present when
type=fixed
.
- trial CoupondiscountTrial? - This is only present when
type=free_trial
.
recurly: CouponDiscountPricing
Fields
- currency string? - 3-letter ISO 4217 currency code.
- amount float? - Value of the fixed discount that this coupon applies.
recurly: CoupondiscountTrial
This is only present when type=free_trial
.
Fields
- unit string? - Temporal unit of the free trial
- length int? - Trial length measured in the units specified by the sibling
unit
property
recurly: CouponList
Fields
- 'object string? - Will always be List.
- has_more boolean? - Indicates there are more results on subsequent pages.
- next string? - Path to subsequent page of results.
- data Coupon[]? -
recurly: CouponMini
Fields
- id string? -
- 'object string? -
- code string? - The code the customer enters to redeem the coupon.
- name string? - The internal name for the coupon.
- state string? - Indicates if the coupon is redeemable, and if it is not, why.
- discount CouponDiscount? - Details of the discount a coupon applies. Will contain a
type
property and one of the following properties:percent
,fixed
,trial
.
- coupon_type string? - Whether the coupon is "single_code" or "bulk". Bulk coupons will require a
unique_code_template
and will generate unique codes through the/generate
endpoint.
- expired_at string? - The date and time the coupon was expired early or reached its
max_redemptions
.
recurly: CouponPricing
Fields
- currency string? - 3-letter ISO 4217 currency code.
- discount float? - The fixed discount (in dollars) for the corresponding currency.
recurly: CouponRedemption
Fields
- id string? -
- 'object string? - Will always be
coupon
.
- account AccountMini? -
- subscription_id string? -
- coupon Coupon? -
- state string? -
- currency string? - 3-letter ISO 4217 currency code.
- discounted float? - The amount that was discounted upon the application of the coupon, formatted with the currency.
- created_at string? -
- updated_at string? -
- removed_at string? - The date and time the redemption was removed from the account (un-redeemed).
recurly: CouponRedemptionCreate
Fields
- coupon_id string -
- currency string? - 3-letter ISO 4217 currency code.
- subscription_id string? -
recurly: CouponRedemptionList
Fields
- 'object string? - Will always be List.
- has_more boolean? - Indicates there are more results on subsequent pages.
- next string? - Path to subsequent page of results.
- data CouponRedemption[]? -
recurly: CouponRedemptionMini
Fields
- id string? -
- 'object string? - Will always be
coupon
.
- coupon CouponMini? -
- state string? -
- discounted float? - The amount that was discounted upon the application of the coupon, formatted with the currency.
- created_at string? -
recurly: CouponUpdate
Fields
- name string? - The internal name for the coupon.
- max_redemptions int? - A maximum number of redemptions for the coupon. The coupon will expire when it hits its maximum redemptions.
- max_redemptions_per_account int? - Redemptions per account is the number of times a specific account can redeem the coupon. Set redemptions per account to
1
if you want to keep customers from gaming the system and getting more than one discount from the coupon campaign.
- hosted_description string? - This description will show up when a customer redeems a coupon on your Hosted Payment Pages, or if you choose to show the description on your own checkout page.
- invoice_description string? - Description of the coupon on the invoice.
- redeem_by_date string? - The date and time the coupon will expire and can no longer be redeemed. Time is always 11:59:59, the end-of-day Pacific time.
recurly: CreditPayment
Fields
- id string? -
- 'object string? -
- uuid string? - The UUID is useful for matching data with the CSV exports and building URLs into Recurly's UI.
- action string? - The action for which the credit was created.
- account AccountMini? -
- applied_to_invoice InvoiceMini? -
- original_invoice InvoiceMini? -
- currency string? - 3-letter ISO 4217 currency code.
- amount float? - Total credit payment amount applied to the charge invoice.
- original_credit_payment_id string? - For credit payments with action
refund
, this is the credit payment that was refunded.
- refund_transaction Transaction? -
- created_at string? -
- updated_at string? -
- voided_at string? -
recurly: CreditPaymentList
Fields
- 'object string? - Will always be List.
- has_more boolean? - Indicates there are more results on subsequent pages.
- next string? - Path to subsequent page of results.
- data CreditPayment[]? -
recurly: CustomField
Fields
- name string - Fields must be created in the UI before values can be assigned to them.
- value string - Any values that resemble a credit card number or security code (CVV/CVC) will be rejected.
recurly: CustomFieldDefinition
Fields
- id string? -
- 'object string? -
- related_type string? -
- name string? - Used by the API to identify the field or reading and writing. The name can only be used once per Recurly object type.
- user_access string? - The access control applied inside Recurly's admin UI:
api_only
- No one will be able to view or edit this field's data via the admin UI.read_only
- Users with the Customers role will be able to view this field's data via the admin UI, but editing will only be available via the API.write
- Users with the Customers role will be able to view and edit this field's data via the admin UI.
- display_name string? - Used to label the field when viewing and editing the field in Recurly's admin UI.
- tooltip string? - Displayed as a tooltip when editing the field in the Recurly admin UI.
- created_at string? -
- updated_at string? -
- deleted_at string? - Definitions are initially soft deleted, and once all the values are removed from the accouts or subscriptions, will be hard deleted an no longer visible.
recurly: CustomFieldDefinitionList
Fields
- 'object string? - Will always be List.
- has_more boolean? - Indicates there are more results on subsequent pages.
- next string? - Path to subsequent page of results.
- data CustomFieldDefinition[]? -
recurly: Empty
recurly: Error
Fields
- 'type string? -
- message string? -
- params ErrorParams[]? -
recurly: ErrorMayHaveTransaction
Fields
- Fields Included from *Error
- type string
- message string
- params ErrorParams[]
- anydata...
- transaction_error TransactionErrorDetails? - This is only included on errors with
type=transaction
.
recurly: ErrorParams
Fields
- param string? -
recurly: ExportDates
Fields
- 'object string? -
- dates string[]? -
recurly: ExportFile
Fields
- name string? - Name of the export file.
- md5sum string? - MD5 hash of the export file.
- href string? - A presigned link to download the export file.
recurly: ExportFiles
Fields
- 'object string? -
- files ExportFile[]? -
recurly: ExternalTransaction
Fields
- payment_method string? - Payment method used for external transaction.
- description string? - Used as the transaction's description.
- amount float? - The total amount of the transcaction. Cannot excceed the invoice total.
- collected_at string? - Datetime that the external payment was collected. Defaults to current datetime.
recurly: FraudInformation
Most recent fraud result.
Fields
- score int? -
- decision string? -
- risk_rules_triggered record {}? -
recurly: Invoice
Fields
- id string? -
- 'object string? -
- 'type string? - Invoices are either charge, credit, or legacy invoices.
- origin string? - The event that created the invoice.
- state string? -
- account AccountMini? -
- billing_info_id string? - The
billing_info_id
is the value that represents a specific billing info for an end customer. Whenbilling_info_id
is used to assign billing info to the subscription, all future billing events for the subscription will bill to the specified billing info.
- subscription_ids InvoiceSubscriptionidsItemsString[]? - If the invoice is charging or refunding for one or more subscriptions, these are their IDs.
- previous_invoice_id string? - On refund invoices, this value will exist and show the invoice ID of the purchase invoice the refund was created from.
- number string? - If VAT taxation and the Country Invoice Sequencing feature are enabled, invoices will have country-specific invoice numbers for invoices billed to EU countries (ex: FR1001). Non-EU invoices will continue to use the site-level invoice number sequence.
- collection_method string? - An automatic invoice means a corresponding transaction is run using the account's billing information at the same time the invoice is created. Manual invoices are created without a corresponding transaction. The merchant must enter a manual payment transaction or have the customer pay the invoice with an automatic method, like credit card, PayPal, Amazon, or ACH bank payment.
- po_number string? - For manual invoicing, this identifies the PO number associated with the subscription.
- net_terms int? - Integer representing the number of days after an invoice's creation that the invoice will become past due. If an invoice's net terms are set to '0', it is due 'On Receipt' and will become past due 24 hours after it’s created. If an invoice is due net 30, it will become past due at 31 days exactly.
- address InvoiceAddress? -
- shipping_address ShippingAddress? -
- currency string? - 3-letter ISO 4217 currency code.
- discount float? - Total discounts applied to this invoice.
- subtotal float? - The summation of charges and credits, before discounts and taxes.
- tax float? - The total tax on this invoice.
- total float? - The final total on this invoice. The summation of invoice charges, discounts, credits, and tax.
- refundable_amount float? - The refundable amount on a charge invoice. It will be null for all other invoices.
- paid float? - The total amount of successful payments transaction on this invoice.
- balance float? - The outstanding balance remaining on this invoice.
- tax_info TaxInfo? -
- vat_number string? - VAT registration number for the customer on this invoice. This will come from the VAT Number field in the Billing Info or the Account Info depending on your tax settings and the invoice collection method.
- vat_reverse_charge_notes string? - VAT Reverse Charge Notes only appear if you have EU VAT enabled or are using your own Avalara AvaTax account and the customer is in the EU, has a VAT number, and is in a different country than your own. This will default to the VAT Reverse Charge Notes text specified on the Tax Settings page in your Recurly admin, unless custom notes were created with the original subscription.
- terms_and_conditions string? - This will default to the Terms and Conditions text specified on the Invoice Settings page in your Recurly admin. Specify custom notes to add or override Terms and Conditions.
- customer_notes string? - This will default to the Customer Notes text specified on the Invoice Settings. Specify custom notes to add or override Customer Notes.
- line_items LineItem[]? -
- has_more_line_items boolean? - Identifies if the invoice has more line items than are returned in
line_items
. Ifhas_more_line_items
istrue
, then a request needs to be made to thelist_invoice_line_items
endpoint.
- transactions Transaction[]? -
- credit_payments CreditPayment[]? -
- created_at string? -
- updated_at string? -
- due_at string? - Date invoice is due. This is the date the net terms are reached.
- closed_at string? - Date invoice was marked paid or failed.
recurly: InvoiceCollect
Fields
- three_d_secure_action_result_token_id string? - A token generated by Recurly.js after completing a 3-D Secure device fingerprinting or authentication challenge.
- transaction_type string? - An optional type designation for the payment gateway transaction created by this request. Supports 'moto' value, which is the acronym for mail order and telephone transactions.
- billing_info_id string? - The
billing_info_id
is the value that represents a specific billing info for an end customer. Whenbilling_info_id
is used to assign billing info to the subscription, all future billing events for the subscription will bill to the specified billing info.
recurly: InvoiceCollection
Fields
- 'object string? -
- charge_invoice Invoice? -
- credit_invoices Invoice[]? -
recurly: InvoiceCreate
Fields
- currency string - 3-letter ISO 4217 currency code.
- collection_method string(default "automatic") - An automatic invoice means a corresponding transaction is run using the account's billing information at the same time the invoice is created. Manual invoices are created without a corresponding transaction. The merchant must enter a manual payment transaction or have the customer pay the invoice with an automatic method, like credit card, PayPal, Amazon, or ACH bank payment.
- charge_customer_notes string? - This will default to the Customer Notes text specified on the Invoice Settings for charge invoices. Specify custom notes to add or override Customer Notes on charge invoices.
- credit_customer_notes string? - This will default to the Customer Notes text specified on the Invoice Settings for credit invoices. Specify customer notes to add or override Customer Notes on credit invoices.
- net_terms int(default 0) - Integer representing the number of days after an invoice's creation that the invoice will become past due. If an invoice's net terms are set to '0', it is due 'On Receipt' and will become past due 24 hours after it’s created. If an invoice is due net 30, it will become past due at 31 days exactly.
- po_number string? - For manual invoicing, this identifies the PO number associated with the subscription.
- terms_and_conditions string? - This will default to the Terms and Conditions text specified on the Invoice Settings page in your Recurly admin. Specify custom notes to add or override Terms and Conditions.
- vat_reverse_charge_notes string? - VAT Reverse Charge Notes only appear if you have EU VAT enabled or are using your own Avalara AvaTax account and the customer is in the EU, has a VAT number, and is in a different country than your own. This will default to the VAT Reverse Charge Notes text specified on the Tax Settings page in your Recurly admin, unless custom notes were created with the original subscription.
recurly: InvoiceList
Fields
- 'object string? - Will always be List.
- has_more boolean? - Indicates there are more results on subsequent pages.
- next string? - Path to subsequent page of results.
- data Invoice[]? -
recurly: InvoiceMini
Fields
- id string? -
- 'object string? -
- number string? -
- 'type string? -
- state string? -
recurly: InvoiceRefund
Fields
- 'type string - The type of refund. Amount and line items cannot both be specified in the request.
- amount float? - The amount to be refunded. The amount will be split between the line items. If no amount is specified, it will default to refunding the total refundable amount on the invoice.
- line_items LineItemRefund[]? - The line items to be refunded. This is required when
type=line_items
.
- refund_method string(default "credit_first") - Indicates how the invoice should be refunded when both a credit and transaction are present on the invoice:
transaction_first
– Refunds the transaction first, then any amount is issued as credit back to the account. Default value when Credit Invoices feature is enabled.credit_first
– Issues credit back to the account first, then refunds any remaining amount back to the transaction. Default value when Credit Invoices feature is not enabled.all_credit
– Issues credit to the account for the entire amount of the refund. Only available when the Credit Invoices feature is enabled.all_transaction
– Refunds the entire amount back to transactions, using transactions from previous invoices if necessary. Only available when the Credit Invoices feature is enabled.
- credit_customer_notes string? - Used as the Customer Notes on the credit invoice. This field can only be include when the Credit Invoices feature is enabled.
- external_refund InvoicerefundExternalRefund? - Indicates that the refund was settled outside of Recurly, and a manual transaction should be created to track it in Recurly.
Required when:
- refunding a manually collected charge invoice, and
refund_method
is notall_credit
- refunding a credit invoice that refunded manually collecting invoices
- refunding a credit invoice for a partial amount
- refunding a manually collected charge invoice, and
recurly: InvoicerefundExternalRefund
Indicates that the refund was settled outside of Recurly, and a manual transaction should be created to track it in Recurly.
Required when:
- refunding a manually collected charge invoice, and
refund_method
is notall_credit
- refunding a credit invoice that refunded manually collecting invoices
- refunding a credit invoice for a partial amount
This field can only be included when the Credit Invoices feature is enabled.
Fields
- payment_method string - Payment method used for external refund transaction.
- description string? - Used as the refund transactions' description.
- refunded_at string? - Date the external refund payment was made. Defaults to the current date-time.
recurly: InvoiceUpdate
Fields
- po_number string? - This identifies the PO number associated with the invoice. Not editable for credit invoices.
- vat_reverse_charge_notes string? - VAT Reverse Charge Notes are editable only if there was a VAT reverse charge applied to the invoice.
- terms_and_conditions string? - Terms and conditions are an optional note field. Not editable for credit invoices.
- customer_notes string? - Customer notes are an optional note field.
- net_terms int? - Integer representing the number of days after an invoice's creation that the invoice will become past due. Changing Net terms changes due_on, and the invoice could move between past due and pending.
- address InvoiceAddress? -
recurly: Item
Full item details.
Fields
- id string? -
- 'object string? -
- code string? - Unique code to identify the item.
- state string? - The current state of the item.
- name string? - This name describes your item and will appear on the invoice when it's purchased on a one time basis.
- description string? - Optional, description.
- external_sku string? - Optional, stock keeping unit to link the item to other inventory systems.
- accounting_code string? - Accounting code for invoice line items.
- revenue_schedule_type string? -
- avalara_transaction_type int? - Used by Avalara for Communications taxes. The transaction type in combination with the service type describe how the item is taxed. Refer to the documentation for more available t/s types.
- avalara_service_type int? - Used by Avalara for Communications taxes. The transaction type in combination with the service type describe how the item is taxed. Refer to the documentation for more available t/s types.
- tax_code string? - Used by Avalara, Vertex, and Recurly’s EU VAT tax feature. The tax code values are specific to each tax system. If you are using Recurly’s EU VAT feature you can use
unknown
,physical
, ordigital
.
- tax_exempt boolean? -
true
exempts tax on the item,false
applies tax on the item.
- custom_fields CustomFields? - The custom fields will only be altered when they are included in a request. Sending an empty array will not remove any existing values. To remove a field send the name with a null or empty value.
- currencies Pricing[]? -
- created_at string? -
- updated_at string? -
- deleted_at string? -
recurly: ItemCreate
Fields
- code string - Unique code to identify the item.
- name string - This name describes your item and will appear on the invoice when it's purchased on a one time basis.
- description string? - Optional, description.
- external_sku string? - Optional, stock keeping unit to link the item to other inventory systems.
- accounting_code string? - Accounting code for invoice line items.
- revenue_schedule_type string? -
- avalara_transaction_type int? - Used by Avalara for Communications taxes. The transaction type in combination with the service type describe how the item is taxed. Refer to the documentation for more available t/s types.
- avalara_service_type int? - Used by Avalara for Communications taxes. The transaction type in combination with the service type describe how the item is taxed. Refer to the documentation for more available t/s types.
- tax_code string? - Used by Avalara, Vertex, and Recurly’s EU VAT tax feature. The tax code values are specific to each tax system. If you are using Recurly’s EU VAT feature you can use
unknown
,physical
, ordigital
.
- tax_exempt boolean? -
true
exempts tax on the item,false
applies tax on the item.
- custom_fields CustomFields? - The custom fields will only be altered when they are included in a request. Sending an empty array will not remove any existing values. To remove a field send the name with a null or empty value.
- currencies Pricing[]? -
recurly: ItemList
Fields
- 'object string? - Will always be List.
- has_more boolean? - Indicates there are more results on subsequent pages.
- next string? - Path to subsequent page of results.
- data Item[]? -
recurly: ItemMini
Just the important parts.
Fields
- id string? -
- 'object string? -
- code string? - Unique code to identify the item.
- state string? - The current state of the item.
- name string? - This name describes your item and will appear on the invoice when it's purchased on a one time basis.
- description string? - Optional, description.
recurly: ItemUpdate
Fields
- code string? - Unique code to identify the item.
- name string? - This name describes your item and will appear on the invoice when it's purchased on a one time basis.
- description string? - Optional, description.
- external_sku string? - Optional, stock keeping unit to link the item to other inventory systems.
- accounting_code string? - Accounting code for invoice line items.
- revenue_schedule_type string? -
- avalara_transaction_type int? - Used by Avalara for Communications taxes. The transaction type in combination with the service type describe how the item is taxed. Refer to the documentation for more available t/s types.
- avalara_service_type int? - Used by Avalara for Communications taxes. The transaction type in combination with the service type describe how the item is taxed. Refer to the documentation for more available t/s types.
- tax_code string? - Used by Avalara, Vertex, and Recurly’s EU VAT tax feature. The tax code values are specific to each tax system. If you are using Recurly’s EU VAT feature you can use
unknown
,physical
, ordigital
.
- tax_exempt boolean? -
true
exempts tax on the item,false
applies tax on the item.
- custom_fields CustomFields? - The custom fields will only be altered when they are included in a request. Sending an empty array will not remove any existing values. To remove a field send the name with a null or empty value.
- currencies Pricing[]? -
recurly: LineItem
Fields
- id string? -
- 'object string? -
- uuid string? - The UUID is useful for matching data with the CSV exports and building URLs into Recurly's UI.
- 'type string? - Charges are positive line items that debit the account. Credits are negative line items that credit the account.
- item_code string? - Unique code to identify an item. Available when the Credit Invoices and Subscription Billing Terms features are enabled.
- item_id string? - System-generated unique identifier for an item. Available when the Credit Invoices and Subscription Billing Terms features are enabled.
- external_sku string? - Optional Stock Keeping Unit assigned to an item. Available when the Credit Invoices and Subscription Billing Terms features are enabled.
- revenue_schedule_type string? -
- state string? - Pending line items are charges or credits on an account that have not been applied to an invoice yet. Invoiced line items will always have an
invoice_id
value.
- legacy_category string? - Category to describe the role of a line item on a legacy invoice:
- "charges" refers to charges being billed for on this invoice.
- "credits" refers to refund or proration credits. This portion of the invoice can be considered a credit memo.
- "applied_credits" refers to previous credits applied to this invoice. See their original_line_item_id to determine where the credit first originated.
- "carryforwards" can be ignored. They exist to consume any remaining credit balance. A new credit with the same amount will be created and placed back on the account.
- account AccountMini? -
- subscription_id string? - If the line item is a charge or credit for a subscription, this is its ID.
- plan_id string? - If the line item is a charge or credit for a plan or add-on, this is the plan's ID.
- plan_code string? - If the line item is a charge or credit for a plan or add-on, this is the plan's code.
- add_on_id string? - If the line item is a charge or credit for an add-on this is its ID.
- add_on_code string? - If the line item is a charge or credit for an add-on, this is its code.
- invoice_id string? - Once the line item has been invoiced this will be the invoice's ID.
- invoice_number string? - Once the line item has been invoiced this will be the invoice's number. If VAT taxation and the Country Invoice Sequencing feature are enabled, invoices will have country-specific invoice numbers for invoices billed to EU countries (ex: FR1001). Non-EU invoices will continue to use the site-level invoice number sequence.
- previous_line_item_id string? - Will only have a value if the line item is a credit created from a previous credit, or if the credit was created from a charge refund.
- original_line_item_invoice_id string? - The invoice where the credit originated. Will only have a value if the line item is a credit created from a previous credit, or if the credit was created from a charge refund.
- origin string? - A credit created from an original charge will have the value of the charge's origin.
- accounting_code string? - Internal accounting code to help you reconcile your revenue to the correct ledger. Line items created as part of a subscription invoice will use the plan or add-on's accounting code, otherwise the value will only be present if you define an accounting code when creating the line item.
- product_code string? - For plan-related line items this will be the plan's code, for add-on related line items it will be the add-on's code. For item-related line items it will be the item's
external_sku
.
- credit_reason_code string? - The reason the credit was given when line item is
type=credit
.
- currency string? - 3-letter ISO 4217 currency code.
- amount float? -
(quantity * unit_amount) - (discount + tax)
- description string? - Description that appears on the invoice. For subscription related items this will be filled in automatically.
- quantity int? - This number will be multiplied by the unit amount to compute the subtotal before any discounts or taxes.
- unit_amount float? - Positive amount for a charge, negative amount for a credit.
- unit_amount_decimal string? - Positive amount for a charge, negative amount for a credit.
- subtotal float? -
quantity * unit_amount
- discount float? - The discount applied to the line item.
- tax float? - The tax amount for the line item.
- taxable boolean? -
true
if the line item is taxable,false
if it is not.
- tax_exempt boolean? -
true
exempts tax on charges,false
applies tax on charges. If not defined, then defaults to the Plan and Site settings. This attribute does not work for credits (negative line items). Credits are always applied post-tax. Pre-tax discounts should use the Coupons feature.
- avalara_transaction_type int? - Used by Avalara for Communications taxes. The transaction type in combination with the service type describe how the line item is taxed. Refer to the documentation for more available t/s types.
- avalara_service_type int? - Used by Avalara for Communications taxes. The transaction type in combination with the service type describe how the line item is taxed. Refer to the documentation for more available t/s types.
- tax_code string? - Used by Avalara, Vertex, and Recurly’s EU VAT tax feature. The tax code values are specific to each tax system. If you are using Recurly’s EU VAT feature you can use
unknown
,physical
, ordigital
.
- tax_info TaxInfo? -
- proration_rate float? - When a line item has been prorated, this is the rate of the proration. Proration rates were made available for line items created after March 30, 2017. For line items created prior to that date, the proration rate will be
null
, even if the line item was prorated.
- refund boolean? -
- refunded_quantity int? - For refund charges, the quantity being refunded. For non-refund charges, the total quantity refunded (possibly over multiple refunds).
- credit_applied float? - The amount of credit from this line item that was applied to the invoice.
- shipping_address ShippingAddress? -
- start_date string? - If an end date is present, this is value indicates the beginning of a billing time range. If no end date is present it indicates billing for a specific date.
- end_date string? - If this date is provided, it indicates the end of a time range.
- created_at string? - When the line item was created.
- updated_at string? - When the line item was last changed.
recurly: LineItemCreate
Fields
- currency string - 3-letter ISO 4217 currency code. If
item_code
/item_id
is part of the request thencurrency
is optional, if the site has a single default currency.currency
is required ifitem_code
/item_id
is present, and there are multiple currencies defined on the site. Ifitem_code
/item_id
is not presentcurrency
is required.
- unit_amount float - A positive or negative amount with
type=charge
will result in a positiveunit_amount
. A positive or negative amount withtype=credit
will result in a negativeunit_amount
. Ifitem_code
/item_id
is present,unit_amount
can be passed in, to override theItem
'sunit_amount
. Ifitem_code
/item_id
is not present thenunit_amount
is required.
- quantity int(default 1) - This number will be multiplied by the unit amount to compute the subtotal before any discounts or taxes.
- description string? - Description that appears on the invoice. If
item_code
/item_id
is part of the request thendescription
must be absent.
- item_code string? - Unique code to identify an item. Available when the Credit Invoices and Subscription Billing Terms features are enabled.
- item_id string? - System-generated unique identifier for an item. Available when the Credit Invoices and Subscription Billing Terms features are enabled.
- revenue_schedule_type string? -
- 'type string - Line item type. If
item_code
/item_id
is present thentype
should not be present. Ifitem_code
/item_id
is not present thentype
is required.
- credit_reason_code string(default "general") - The reason the credit was given when line item is
type=credit
. When the Credit Invoices feature is enabled, the value can be set and will default togeneral
. When the Credit Invoices feature is not enabled, the value will always benull
.
- accounting_code string? - Accounting Code for the
LineItem
. Ifitem_code
/item_id
is part of the request thenaccounting_code
must be absent.
- tax_exempt boolean? -
true
exempts tax on charges,false
applies tax on charges. If not defined, then defaults to the Plan and Site settings. This attribute does not work for credits (negative line items). Credits are always applied post-tax. Pre-tax discounts should use the Coupons feature.
- avalara_transaction_type int? - Used by Avalara for Communications taxes. The transaction type in combination with the service type describe how the line item is taxed. Refer to the documentation for more available t/s types. If an
Item
is associated to theLineItem
, then theavalara_transaction_type
must be absent.
- avalara_service_type int? - Used by Avalara for Communications taxes. The transaction type in combination with the service type describe how the line item is taxed. Refer to the documentation for more available t/s types. If an
Item
is associated to theLineItem
, then theavalara_service_type
must be absent.
- tax_code string? - Optional field used by Avalara, Vertex, and Recurly's EU VAT tax feature to determine taxation rules. If you have your own AvaTax or Vertex account configured, use their tax codes to assign specific tax rules. If you are using Recurly's EU VAT feature, you can use values of
unknown
,physical
, ordigital
.
- product_code string? - Optional field to track a product code or SKU for the line item. This can be used to later reporting on product purchases. For Vertex tax calculations, this field will be used as the Vertex
product
field. Ifitem_code
/item_id
is part of the request thenproduct_code
must be absent.
- origin string? - Origin
external_gift_card
is allowed if the Gift Cards feature is enabled on your site andtype
iscredit
. Set this value in order to track gift card credits from external gift cards (like InComm). It also skips billing information requirements. Originprepayment
is only allowed iftype
ischarge
andtax_exempt
is left blank or set to true. This origin creates a charge and opposite credit on the account to be used for future invoices.
- start_date string? - If an end date is present, this is value indicates the beginning of a billing time range. If no end date is present it indicates billing for a specific date. Defaults to the current date-time.
- end_date string? - If this date is provided, it indicates the end of a time range.
recurly: LineItemList
Fields
- 'object string? - Will always be List.
- has_more boolean? - Indicates there are more results on subsequent pages.
- next string? - Path to subsequent page of results.
- data LineItem[]? -
recurly: LineItemRefund
Fields
- id string? -
- quantity int? - Line item quantity to be refunded.
- prorate boolean? - Set to
true
if the line item should be prorated; set tofalse
if not. This can only be used on line items that have a start and end date.
recurly: MeasuredUnit
Fields
- id string? -
- 'object string? -
- name string? - Unique internal name of the measured unit on your site.
- display_name string? - Display name for the measured unit. Can only contain spaces, underscores and must be alphanumeric.
- state string? - The current state of the measured unit.
- description string? - Optional internal description.
- created_at string? -
- updated_at string? -
- deleted_at string? -
recurly: MeasuredUnitCreate
Fields
- name string - Unique internal name of the measured unit on your site.
- display_name string - Display name for the measured unit.
- description string? - Optional internal description.
recurly: MeasuredUnitList
Fields
- 'object string? - Will always be List.
- has_more boolean? - Indicates there are more results on subsequent pages.
- next string? - Path to subsequent page of results.
- data MeasuredUnit[]? -
recurly: MeasuredUnitUpdate
Fields
- name string? - Unique internal name of the measured unit on your site.
- display_name string? - Display name for the measured unit.
- description string? - Optional internal description.
recurly: PaymentMethod
Fields
- 'object string? -
- card_type string? - Visa, MasterCard, American Express, Discover, JCB, etc.
- first_six string? - Credit card number's first six digits.
- last_four string? - Credit card number's last four digits. Will refer to bank account if payment method is ACH.
- last_two string? - The IBAN bank account's last two digits.
- exp_month int? - Expiration month.
- exp_year int? - Expiration year.
- gateway_token string? - A token used in place of a credit card in order to perform transactions.
- cc_bin_country string? - The 2-letter ISO 3166-1 alpha-2 country code associated with the credit card BIN, if known by Recurly. Available on the BillingInfo object only. Available when the BIN country lookup feature is enabled.
- gateway_code string? - An identifier for a specific payment gateway.
- billing_agreement_id string? - Billing Agreement identifier. Only present for Amazon or Paypal payment methods.
- name_on_account string? - The name associated with the bank account.
- account_type string? - The bank account type. Only present for ACH payment methods.
- routing_number string? - The bank account's routing number. Only present for ACH payment methods.
- routing_number_bank string? - The bank name of this routing number.
recurly: Plan
Full plan details.
Fields
- id string? -
- 'object string? -
- code string - Unique code to identify the plan. This is used in Hosted Payment Page URLs and in the invoice exports.
- state string? - The current state of the plan.
- name string - This name describes your plan and will appear on the Hosted Payment Page and the subscriber's invoice.
- description string? - Optional description, not displayed.
- interval_unit string(default "months") - Unit for the plan's billing interval.
- interval_length int(default 1) - Length of the plan's billing interval in
interval_unit
.
- trial_unit string(default "months") - Units for the plan's trial period.
- trial_length int(default 0) - Length of plan's trial period in
trial_units
.0
meansno trial
.
- trial_requires_billing_info boolean(default true) - Allow free trial subscriptions to be created without billing info. Should not be used if billing info is needed for initial invoice due to existing uninvoiced charges or setup fee.
- total_billing_cycles int? - Automatically terminate subscriptions after a defined number of billing cycles. Number of billing cycles before the plan automatically stops renewing, defaults to
null
for continuous, automatic renewal.
- auto_renew boolean(default true) - Subscriptions will automatically inherit this value once they are active. If
auto_renew
istrue
, then a subscription will automatically renew its term at renewal. Ifauto_renew
isfalse
, then a subscription will expire at the end of its term.auto_renew
can be overridden on the subscription record itself.
- revenue_schedule_type string? -
- setup_fee_revenue_schedule_type string? -
- accounting_code string? - Accounting code for invoice line items for the plan. If no value is provided, it defaults to plan's code.
- setup_fee_accounting_code string? - Accounting code for invoice line items for the plan's setup fee. If no value is provided, it defaults to plan's accounting code.
- avalara_transaction_type int? - Used by Avalara for Communications taxes. The transaction type in combination with the service type describe how the plan is taxed. Refer to the documentation for more available t/s types.
- avalara_service_type int? - Used by Avalara for Communications taxes. The transaction type in combination with the service type describe how the plan is taxed. Refer to the documentation for more available t/s types.
- tax_code string? - Used by Avalara, Vertex, and Recurly’s EU VAT tax feature. The tax code values are specific to each tax system. If you are using Recurly’s EU VAT feature you can use
unknown
,physical
, ordigital
.
- tax_exempt boolean? -
true
exempts tax on the plan,false
applies tax on the plan.
- currencies PlanPricing[]? -
- hosted_pages PlanHostedPages? -
- allow_any_item_on_subscriptions boolean? - Used to determine whether items can be assigned as add-ons to individual subscriptions.
If
true
, items can be assigned as add-ons to individual subscription add-ons. Iffalse
, only plan add-ons can be used.
- created_at string? -
- updated_at string? -
- deleted_at string? -
recurly: PlanCreate
Fields
- code string - Unique code to identify the plan. This is used in Hosted Payment Page URLs and in the invoice exports.
- name string - This name describes your plan and will appear on the Hosted Payment Page and the subscriber's invoice.
- description string? - Optional description, not displayed.
- accounting_code string? - Accounting code for invoice line items for the plan. If no value is provided, it defaults to plan's code.
- interval_unit string(default "months") - Unit for the plan's billing interval.
- interval_length int(default 1) - Length of the plan's billing interval in
interval_unit
.
- trial_unit string(default "months") - Units for the plan's trial period.
- trial_length int(default 0) - Length of plan's trial period in
trial_units
.0
meansno trial
.
- trial_requires_billing_info boolean(default true) - Allow free trial subscriptions to be created without billing info. Should not be used if billing info is needed for initial invoice due to existing uninvoiced charges or setup fee.
- total_billing_cycles int? - Automatically terminate plans after a defined number of billing cycles.
- auto_renew boolean(default true) - Subscriptions will automatically inherit this value once they are active. If
auto_renew
istrue
, then a subscription will automatically renew its term at renewal. Ifauto_renew
isfalse
, then a subscription will expire at the end of its term.auto_renew
can be overridden on the subscription record itself.
- revenue_schedule_type string? -
- setup_fee_revenue_schedule_type string? -
- setup_fee_accounting_code string? - Accounting code for invoice line items for the plan's setup fee. If no value is provided, it defaults to plan's accounting code.
- avalara_transaction_type int? - Used by Avalara for Communications taxes. The transaction type in combination with the service type describe how the plan is taxed. Refer to the documentation for more available t/s types.
- avalara_service_type int? - Used by Avalara for Communications taxes. The transaction type in combination with the service type describe how the plan is taxed. Refer to the documentation for more available t/s types.
- tax_code string? - Optional field used by Avalara, Vertex, and Recurly's EU VAT tax feature to determine taxation rules. If you have your own AvaTax or Vertex account configured, use their tax codes to assign specific tax rules. If you are using Recurly's EU VAT feature, you can use values of
unknown
,physical
, ordigital
.
- tax_exempt boolean? -
true
exempts tax on the plan,false
applies tax on the plan.
- currencies PlanPricing[] -
- hosted_pages PlanHostedPages? -
- add_ons AddOnCreate[]? -
- allow_any_item_on_subscriptions boolean(default false) - Used to determine whether items can be assigned as add-ons to individual subscriptions.
If
true
, items can be assigned as add-ons to individual subscription add-ons. Iffalse
, only plan add-ons can be used.
recurly: PlanHostedPages
Fields
- success_url string? - URL to redirect to after signup on the hosted payment pages.
- cancel_url string? - URL to redirect to on canceled signup on the hosted payment pages.
- bypass_confirmation boolean? - If
true
, the customer will be sent directly to yoursuccess_url
after a successful signup, bypassing Recurly's hosted confirmation page.
- display_quantity boolean? - Determines if the quantity field is displayed on the hosted pages for the plan.
recurly: PlanList
Fields
- 'object string? - Will always be List.
- has_more boolean? - Indicates there are more results on subsequent pages.
- next string? - Path to subsequent page of results.
- data Plan[]? -
recurly: PlanMini
Just the important parts.
Fields
- id string? -
- 'object string? -
- code string? - Unique code to identify the plan. This is used in Hosted Payment Page URLs and in the invoice exports.
- name string? - This name describes your plan and will appear on the Hosted Payment Page and the subscriber's invoice.
recurly: PlanPricing
Fields
- currency string? - 3-letter ISO 4217 currency code.
- setup_fee float? - Amount of one-time setup fee automatically charged at the beginning of a subscription billing cycle. For subscription plans with a trial, the setup fee will be charged at the time of signup. Setup fees do not increase with the quantity of a subscription plan.
- unit_amount float? -
recurly: PlanUpdate
Fields
- id string? -
- code string? - Unique code to identify the plan. This is used in Hosted Payment Page URLs and in the invoice exports.
- name string? - This name describes your plan and will appear on the Hosted Payment Page and the subscriber's invoice.
- description string? - Optional description, not displayed.
- accounting_code string? - Accounting code for invoice line items for the plan. If no value is provided, it defaults to plan's code.
- trial_unit string? - Units for the plan's trial period.
- trial_length int? - Length of plan's trial period in
trial_units
.0
meansno trial
.
- trial_requires_billing_info boolean? - Allow free trial subscriptions to be created without billing info. Should not be used if billing info is needed for initial invoice due to existing uninvoiced charges or setup fee.
- total_billing_cycles int? - Automatically terminate plans after a defined number of billing cycles.
- auto_renew boolean? - Subscriptions will automatically inherit this value once they are active. If
auto_renew
istrue
, then a subscription will automatically renew its term at renewal. Ifauto_renew
isfalse
, then a subscription will expire at the end of its term.auto_renew
can be overridden on the subscription record itself.
- revenue_schedule_type string? -
- setup_fee_revenue_schedule_type string? -
- setup_fee_accounting_code string? - Accounting code for invoice line items for the plan's setup fee. If no value is provided, it defaults to plan's accounting code.
- avalara_transaction_type int? - Used by Avalara for Communications taxes. The transaction type in combination with the service type describe how the plan is taxed. Refer to the documentation for more available t/s types.
- avalara_service_type int? - Used by Avalara for Communications taxes. The transaction type in combination with the service type describe how the plan is taxed. Refer to the documentation for more available t/s types.
- tax_code string? - Optional field used by Avalara, Vertex, and Recurly's EU VAT tax feature to determine taxation rules. If you have your own AvaTax or Vertex account configured, use their tax codes to assign specific tax rules. If you are using Recurly's EU VAT feature, you can use values of
unknown
,physical
, ordigital
.
- tax_exempt boolean? -
true
exempts tax on the plan,false
applies tax on the plan.
- currencies PlanPricing[]? -
- hosted_pages PlanHostedPages? -
- allow_any_item_on_subscriptions boolean? - Used to determine whether items can be assigned as add-ons to individual subscriptions.
If
true
, items can be assigned as add-ons to individual subscription add-ons. Iffalse
, only plan add-ons can be used.
recurly: Pricing
Fields
- currency string - 3-letter ISO 4217 currency code.
- unit_amount float -
recurly: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
recurly: PurchaseCreate
A purchase is only a request data type and is not persistent in Recurly, an InvoiceCollection will be the returned type.
Fields
- currency string - 3-letter ISO 4217 currency code.
- account AccountPurchase -
- billing_info_id string? - The
billing_info_id
is the value that represents a specific billing info for an end customer. Whenbilling_info_id
is used to assign billing info to the subscription, all future billing events for the subscription will bill to the specified billing info.
- collection_method string(default "automatic") - Must be set to manual in order to preview a purchase for an Account that does not have payment information associated with the Billing Info.
- po_number string? - For manual invoicing, this identifies the PO number associated with the subscription.
- net_terms int(default 0) - Integer representing the number of days after an invoice's creation that the invoice will become past due. If an invoice's net terms are set to '0', it is due 'On Receipt' and will become past due 24 hours after it’s created. If an invoice is due net 30, it will become past due at 31 days exactly.
- terms_and_conditions string? - Terms and conditions to be put on the purchase invoice.
- customer_notes string? -
- vat_reverse_charge_notes string? - VAT reverse charge notes for cross border European tax settlement.
- credit_customer_notes string? - Notes to be put on the credit invoice resulting from credits in the purchase, if any.
- gateway_code string? - The default payment gateway identifier to be used for the purchase transaction. This will also be applied as the default for any subscriptions included in the purchase request.
- shipping PurchasecreateShipping? -
- line_items LineItemCreate[]? - A list of one time charges or credits to be created with the purchase.
- subscriptions SubscriptionPurchase[]? - A list of subscriptions to be created with the purchase.
- coupon_codes string[]? - A list of coupon_codes to be redeemed on the subscription or account during the purchase.
- gift_card_redemption_code string? - A gift card redemption code to be redeemed on the purchase invoice.
- transaction_type string? - An optional type designation for the payment gateway transaction created by this request. Supports 'moto' value, which is the acronym for mail order and telephone transactions.
recurly: PurchasecreateShipping
Fields
- address_id string? - Assign a shipping address from the account's existing shipping addresses. If this and
address
are both present,address
will take precedence.
- address ShippingAddressCreate? -
- fees ShippingFeeCreate[]? - A list of shipping fees to be created as charges with the purchase.
recurly: Settings
Fields
- billing_address_requirement string? -
- full: Full Address (Street, City, State, Postal Code and Country)
- streetzip: Street and Postal Code only
- zip: Postal Code only
- none: No Address
- accepted_currencies string[]? -
- default_currency string? - The default 3-letter ISO 4217 currency code.
recurly: ShippingAddress
Fields
- id string? -
- 'object string? -
- account_id string? -
- nickname string? -
- first_name string? -
- last_name string? -
- company string? -
- email string? -
- vat_number string? -
- phone string? -
- street1 string? -
- street2 string? -
- city string? -
- region string? - State or province.
- postal_code string? - Zip or postal code.
- country string? - Country, 2-letter ISO 3166-1 alpha-2 code.
- created_at string? -
- updated_at string? -
recurly: ShippingAddressCreate
Fields
- nickname string? -
- first_name string -
- last_name string -
- company string? -
- email string? -
- vat_number string? -
- phone string? -
- street1 string -
- street2 string? -
- city string -
- region string? - State or province.
- postal_code string - Zip or postal code.
- country string - Country, 2-letter ISO 3166-1 alpha-2 code.
recurly: ShippingAddressList
Fields
- 'object string? - Will always be List.
- has_more boolean? - Indicates there are more results on subsequent pages.
- next string? - Path to subsequent page of results.
- data ShippingAddress[]? -
recurly: ShippingAddressUpdate
Fields
- id string? -
- nickname string? -
- first_name string? -
- last_name string? -
- company string? -
- email string? -
- vat_number string? -
- phone string? -
- street1 string? -
- street2 string? -
- city string? -
- region string? - State or province.
- postal_code string? - Zip or postal code.
- country string? - Country, 2-letter ISO 3166-1 alpha-2 code.
recurly: ShippingFeeCreate
Fields
- method_id string? - The id of the shipping method used to deliver the purchase. If
method_id
andmethod_code
are both present,method_id
will be used.
- method_code string? - The code of the shipping method used to deliver the purchase. If
method_id
andmethod_code
are both present,method_id
will be used.
- amount float? - This is priced in the purchase's currency.
recurly: ShippingMethod
Fields
- id string? -
- 'object string? -
- code string? - The internal name used identify the shipping method.
- name string? - The name of the shipping method displayed to customers.
- accounting_code string? - Accounting code for shipping method.
- tax_code string? - Used by Avalara, Vertex, and Recurly’s built-in tax feature. The tax
code values are specific to each tax system. If you are using Recurly’s
built-in taxes the values are:
FR
– Common Carrier FOB DestinationFR022000
– Common Carrier FOB OriginFR020400
– Non Common Carrier FOB DestinationFR020500
– Non Common Carrier FOB OriginFR010100
– Delivery by Company Vehicle Before Passage of TitleFR010200
– Delivery by Company Vehicle After Passage of TitleNT
– Non-Taxable
- created_at string? -
- updated_at string? -
- deleted_at string? -
recurly: ShippingMethodCreate
Fields
- code string - The internal name used identify the shipping method.
- name string - The name of the shipping method displayed to customers.
- accounting_code string? - Accounting code for shipping method.
- tax_code string? - Used by Avalara, Vertex, and Recurly’s built-in tax feature. The tax
code values are specific to each tax system. If you are using Recurly’s
built-in taxes the values are:
FR
– Common Carrier FOB DestinationFR022000
– Common Carrier FOB OriginFR020400
– Non Common Carrier FOB DestinationFR020500
– Non Common Carrier FOB OriginFR010100
– Delivery by Company Vehicle Before Passage of TitleFR010200
– Delivery by Company Vehicle After Passage of TitleNT
– Non-Taxable
recurly: ShippingMethodList
Fields
- 'object string? - Will always be List.
- has_more boolean? - Indicates there are more results on subsequent pages.
- next string? - Path to subsequent page of results.
- data ShippingMethod[]? -
recurly: ShippingMethodMini
Fields
- id string? -
- 'object string? -
- code string? - The internal name used identify the shipping method.
- name string? - The name of the shipping method displayed to customers.
recurly: ShippingMethodUpdate
Fields
- code string? - The internal name used identify the shipping method.
- name string? - The name of the shipping method displayed to customers.
- accounting_code string? - Accounting code for shipping method.
- tax_code string? - Used by Avalara, Vertex, and Recurly’s built-in tax feature. The tax
code values are specific to each tax system. If you are using Recurly’s
built-in taxes the values are:
FR
– Common Carrier FOB DestinationFR022000
– Common Carrier FOB OriginFR020400
– Non Common Carrier FOB DestinationFR020500
– Non Common Carrier FOB OriginFR010100
– Delivery by Company Vehicle Before Passage of TitleFR010200
– Delivery by Company Vehicle After Passage of TitleNT
– Non-Taxable
recurly: Site
Fields
- id string? -
- 'object string? -
- subdomain string? -
- public_api_key string? - This value is used to configure RecurlyJS to submit tokenized billing information.
- mode string? -
- address Address? -
- settings Settings? -
- features string[]? - A list of features enabled for the site.
- created_at string? -
- updated_at string? -
- deleted_at string? -
recurly: SiteList
Fields
- 'object string? - Will always be List.
- has_more boolean? - Indicates there are more results on subsequent pages.
- next string? - Path to subsequent page of results.
- data Site[]? -
recurly: Subscription
Fields
- id string? -
- 'object string? -
- uuid string? - The UUID is useful for matching data with the CSV exports and building URLs into Recurly's UI.
- account AccountMini? -
- plan PlanMini? - Just the important parts.
- state string? -
- shipping SubscriptionShipping? -
- coupon_redemptions CouponRedemptionMini[]? - Returns subscription level coupon redemptions that are tied to this subscription.
- pending_change SubscriptionChange? -
- current_period_started_at string? -
- current_period_ends_at string? -
- current_term_started_at string? - The start date of the term when the first billing period starts. The subscription term is the length of time that a customer will be committed to a subscription. A term can span multiple billing periods.
- current_term_ends_at string? - When the term ends. This is calculated by a plan's interval and
total_billing_cycles
in a term. Subscription changes with atimeframe=renewal
will be applied on this date.
- trial_started_at string? -
- trial_ends_at string? -
- remaining_billing_cycles int? - The remaining billing cycles in the current term.
- total_billing_cycles int? - The number of cycles/billing periods in a term. When
remaining_billing_cycles=0
, ifauto_renew=true
the subscription will renew and a new term will begin, otherwise the subscription will expire.
- renewal_billing_cycles int? - If
auto_renew=true
, when a term completes,total_billing_cycles
takes this value as the length of subsequent terms. Defaults to the plan'stotal_billing_cycles
.
- auto_renew boolean? - Whether the subscription renews at the end of its term.
- paused_at string? - Null unless subscription is paused or will pause at the end of the current billing period.
- remaining_pause_cycles int? - Null unless subscription is paused or will pause at the end of the current billing period.
- currency string? - 3-letter ISO 4217 currency code.
- revenue_schedule_type string? -
- unit_amount float? -
- quantity int? -
- add_ons SubscriptionAddOn[]? -
- add_ons_total float? -
- subtotal float? -
- collection_method string? -
- po_number string? - For manual invoicing, this identifies the PO number associated with the subscription.
- net_terms int? - Integer representing the number of days after an invoice's creation that the invoice will become past due. If an invoice's net terms are set to '0', it is due 'On Receipt' and will become past due 24 hours after it’s created. If an invoice is due net 30, it will become past due at 31 days exactly.
- terms_and_conditions string? -
- customer_notes string? -
- expiration_reason string? -
- custom_fields CustomFields? - The custom fields will only be altered when they are included in a request. Sending an empty array will not remove any existing values. To remove a field send the name with a null or empty value.
- created_at string? -
- updated_at string? -
- activated_at string? -
- canceled_at string? -
- expires_at string? -
- bank_account_authorized_at string? - Recurring subscriptions paid with ACH will have this attribute set. This timestamp is used for alerting customers to reauthorize in 3 years in accordance with NACHA rules. If a subscription becomes inactive or the billing info is no longer a bank account, this timestamp is cleared.
- billing_info_id string? - Billing Info ID.
recurly: SubscriptionAddOn
This links an Add-on to a specific Subscription.
Fields
- id string? -
- 'object string? -
- subscription_id string? -
- add_on AddOnMini? - Just the important parts.
- add_on_source string? - Used to determine where the associated add-on data is pulled from. If this value is set to
plan_add_on
or left blank, then add-on data will be pulled from the plan's add-ons. If the associatedplan
hasallow_any_item_on_subscriptions
set totrue
and this field is set toitem
, then the associated add-on data will be pulled from the site's item catalog.
- quantity int? -
- unit_amount float? - Supports up to 2 decimal places.
- unit_amount_decimal string? - Supports up to 9 decimal places.
- revenue_schedule_type string? -
- tier_type string? - The pricing model for the add-on. For more information, click here. See our Guide for an overview of how to configure quantity-based pricing models.
- tiers SubscriptionAddOnTier[]? - If tiers are provided in the request, all existing tiers on the Subscription Add-on will be removed and replaced by the tiers in the request.
- usage_percentage float? - The percentage taken of the monetary amount of usage tracked. This can be up to 4 decimal places. A value between 0.0 and 100.0. Required if add_on_type is usage and usage_type is percentage.
- created_at string? -
- updated_at string? -
- expired_at string? -
recurly: SubscriptionAddOnCreate
Fields
- code string - If
add_on_source
is set toplan_add_on
or left blank, then plan's add-oncode
should be used. Ifadd_on_source
is set toitem
, then thecode
from the associated item should be used.
- add_on_source string(default "plan_add_on") - Used to determine where the associated add-on data is pulled from. If this value is set to
plan_add_on
or left blank, then add-on data will be pulled from the plan's add-ons. If the associatedplan
hasallow_any_item_on_subscriptions
set totrue
and this field is set toitem
, then the associated add-on data will be pulled from the site's item catalog.
- quantity int(default 1) -
- unit_amount float? - Allows up to 2 decimal places. Optionally, override the add-on's default unit amount.
If the plan add-on's
tier_type
istiered
,volume
, orstairstep
, thenunit_amount
cannot be provided.
- unit_amount_decimal string? - Allows up to 9 decimal places. Optionally, override the add-on's default unit amount.
If the plan add-on's
tier_type
istiered
,volume
, orstairstep
, thenunit_amount_decimal
cannot be provided. Only supported when the plan add-on'sadd_on_type
=usage
. Ifunit_amount_decimal
is provided,unit_amount
cannot be provided.
- tiers SubscriptionAddOnTier[]? - If the plan add-on's
tier_type
isflat
, thentiers
must be absent. Thetiers
object must include one to many tiers withending_quantity
andunit_amount
. There must be one tier with anending_quantity
of 999999999 which is the default if not provided. See our Guide for an overview of how to configure quantity-based pricing models.
- usage_percentage float? - The percentage taken of the monetary amount of usage tracked. This can be up to 4 decimal places. A value between 0.0 and 100.0. Required if
add_on_type
is usage andusage_type
is percentage. Must be omitted otherwise.usage_percentage
does not support tiers. See our Guide for an overview of how to configure usage add-ons.
- revenue_schedule_type string? -
recurly: SubscriptionAddOnTier
Fields
- ending_quantity int? -
- unit_amount float? - Allows up to 2 decimal places. Optionally, override the tiers' default unit amount. If add-on's
add_on_type
isusage
andusage_type
ispercentage
, cannot be provided.
- unit_amount_decimal string? - Allows up to 9 decimal places. Optionally, override tiers' default unit amount.
If
unit_amount_decimal
is provided,unit_amount
cannot be provided. If add-on'sadd_on_type
isusage
andusage_type
ispercentage
, cannot be provided.
- usage_percentage string? - The percentage taken of the monetary amount of usage tracked. This can be up to 4 decimal places represented as a string. A value between 0.0 and 100.0. Optionally, override tiers' default usage percentage. Required if add-on's
add_on_type
isusage
andusage_type
ispercentage
. Must be omitted otherwise.
recurly: SubscriptionAddOnUpdate
Fields
- id string? - When an id is provided, the existing subscription add-on attributes will persist unless overridden in the request.
- code string? - If a code is provided without an id, the subscription add-on attributes
will be set to the current value for those attributes on the plan add-on
unless provided in the request. If
add_on_source
is set toplan_add_on
or left blank, then plan's add-oncode
should be used. Ifadd_on_source
is set toitem
, then thecode
from the associated item should be used.
- add_on_source string? - Used to determine where the associated add-on data is pulled from. If this value is set to
plan_add_on
or left blank, then add-on data will be pulled from the plan's add-ons. If the associatedplan
hasallow_any_item_on_subscriptions
set totrue
and this field is set toitem
, then the associated add-on data will be pulled from the site's item catalog.
- quantity int? -
- unit_amount float? - Allows up to 2 decimal places. Optionally, override the add-on's default unit amount.
If the plan add-on's
tier_type
istiered
,volume
, orstairstep
, thenunit_amount
cannot be provided.
- unit_amount_decimal string? - Allows up to 9 decimal places. Optionally, override the add-on's default unit amount.
If the plan add-on's
tier_type
istiered
,volume
, orstairstep
, thenunit_amount_decimal
cannot be provided. Only supported when the plan add-on'sadd_on_type
=usage
. Ifunit_amount_decimal
is provided,unit_amount
cannot be provided.
- tiers SubscriptionAddOnTier[]? - If the plan add-on's
tier_type
isflat
, thentiers
must be absent. Thetiers
object must include one to many tiers withending_quantity
andunit_amount
. There must be one tier with anending_quantity
of 999999999 which is the default if not provided.
- usage_percentage float? - The percentage taken of the monetary amount of usage tracked. This can be up to 4 decimal places. A value between 0.0 and 100.0. Required if add_on_type is usage and usage_type is percentage.
- revenue_schedule_type string? -
recurly: SubscriptionCancel
Fields
- timeframe string? - The timeframe parameter controls when the expiration takes place. The
bill_date
timeframe causes the subscription to expire when the subscription is scheduled to bill next. Theterm_end
timeframe causes the subscription to continue to bill until the end of the subscription term, then expire.
recurly: SubscriptionChange
Fields
- id string? - The ID of the Subscription Change.
- 'object string? -
- subscription_id string? - The ID of the subscription that is going to be changed.
- plan PlanMini? - Just the important parts.
- add_ons SubscriptionAddOn[]? - These add-ons will be used when the subscription renews.
- unit_amount float? -
- quantity int? -
- shipping SubscriptionShipping? -
- activate_at string? -
- activated boolean? - Returns
true
if the subscription change is activated.
- revenue_schedule_type string? -
- invoice_collection InvoiceCollection? -
- custom_fields CustomFields? - The custom fields will only be altered when they are included in a request. Sending an empty array will not remove any existing values. To remove a field send the name with a null or empty value.
- created_at string? -
- updated_at string? -
- deleted_at string? -
- billing_info SubscriptionChangeBillingInfo? - Accept nested attributes for three_d_secure_action_result_token_id
recurly: SubscriptionChangeBillingInfo
Accept nested attributes for three_d_secure_action_result_token_id
Fields
- three_d_secure_action_result_token_id string? - A token generated by Recurly.js after completing a 3-D Secure device fingerprinting or authentication challenge.
recurly: SubscriptionChangeCreate
Fields
- timeframe string? - The timeframe parameter controls when the upgrade or downgrade takes place. The subscription change can occur now, when the subscription is next billed, or when the subscription term ends. Generally, if you're performing an upgrade, you will want the change to occur immediately (now). If you're performing a downgrade, you should set the timeframe to
term_end
orbill_date
so the change takes effect at a scheduled billing date. Therenewal
timeframe option is accepted as an alias forterm_end
.
- plan_id string? - If you want to change to a new plan, you can provide the plan's code or id. If both are provided the
plan_id
will be used.
- plan_code string? - If you want to change to a new plan, you can provide the plan's code or id. If both are provided the
plan_id
will be used.
- unit_amount float? - Optionally, sets custom pricing for the subscription, overriding the plan's default unit amount. The subscription's current currency will be used.
- quantity int? - Optionally override the default quantity of 1.
- shipping SubscriptionChangeShippingCreate? - The shipping address can currently only be changed immediately, using SubscriptionUpdate.
- coupon_codes string[]? - A list of coupon_codes to be redeemed on the subscription during the change. Only allowed if timeframe is now and you change something about the subscription that creates an invoice.
- add_ons SubscriptionAddOnUpdate[]? - If you provide a value for this field it will replace any
existing add-ons. So, when adding or modifying an add-on, you need to
include the existing subscription add-ons. Unchanged add-ons can be included
just using the subscription add-on''s ID:
{"id": "abc123"}
. If this value is omitted your existing add-ons will be unaffected. To remove all existing add-ons, this value should be an empty array.' If a subscription add-on'scode
is supplied without theid
,{"code": "def456"}
, the subscription add-on attributes will be set to the current values of the plan add-on unless provided in the request.- If an
id
is passed, any attributes not passed in will pull from the existing subscription add-on - If a
code
is passed, any attributes not passed in will pull from the current values of the plan add-on - Attributes passed in as part of the request will override either of the above scenarios
- If an
- collection_method string? -
- revenue_schedule_type string? -
- custom_fields CustomFields? - The custom fields will only be altered when they are included in a request. Sending an empty array will not remove any existing values. To remove a field send the name with a null or empty value.
- po_number string? - For manual invoicing, this identifies the PO number associated with the subscription.
- net_terms int? - Integer representing the number of days after an invoice's creation that the invoice will become past due. If an invoice's net terms are set to '0', it is due 'On Receipt' and will become past due 24 hours after it’s created. If an invoice is due net 30, it will become past due at 31 days exactly.
- transaction_type string? - An optional type designation for the payment gateway transaction created by this request. Supports 'moto' value, which is the acronym for mail order and telephone transactions.
- billing_info SubscriptionChangeBillingInfoCreate? -
recurly: SubscriptionChangeShippingCreate
The shipping address can currently only be changed immediately, using SubscriptionUpdate.
Fields
- method_id string? - The id of the shipping method used to deliver the subscription. To remove shipping set this to
null
and theamount=0
. Ifmethod_id
andmethod_code
are both present,method_id
will be used.
- method_code string? - The code of the shipping method used to deliver the subscription. To remove shipping set this to
null
and theamount=0
. Ifmethod_id
andmethod_code
are both present,method_id
will be used.
- amount float? -
recurly: SubscriptionCreate
Fields
- plan_code string - You must provide either a
plan_code
orplan_id
. If both are provided theplan_id
will be used.
- plan_id string? - You must provide either a
plan_code
orplan_id
. If both are provided theplan_id
will be used.
- account AccountCreate -
- billing_info_id string? - The
billing_info_id
is the value that represents a specific billing info for an end customer. Whenbilling_info_id
is used to assign billing info to the subscription, all future billing events for the subscription will bill to the specified billing info.
- shipping SubscriptionShippingCreate? -
- collection_method string(default "automatic") -
- currency string - 3-letter ISO 4217 currency code.
- unit_amount float? - Override the unit amount of the subscription plan by setting this value. If not provided, the subscription will inherit the price from the subscription plan for the provided currency.
- quantity int(default 1) - Optionally override the default quantity of 1.
- add_ons SubscriptionAddOnCreate[]? -
- coupon_codes string[]? - A list of coupon_codes to be redeemed on the subscription or account during the purchase.
- custom_fields CustomFields? - The custom fields will only be altered when they are included in a request. Sending an empty array will not remove any existing values. To remove a field send the name with a null or empty value.
- trial_ends_at string? - If set, overrides the default trial behavior for the subscription. When the current date time or a past date time is provided the subscription will begin with no trial phase (overriding any plan default trial). When a future date time is provided the subscription will begin with a trial phase ending at the specified date time.
- starts_at string? - If set, the subscription will begin in the future on this date. The subscription will apply the setup fee and trial period, unless the plan has no trial.
- next_bill_date string? - If present, this sets the date the subscription's next billing period will start (
current_period_ends_at
). This can be used to align the subscription’s billing to a specific day of the month. The initial invoice will be prorated for the period between the subscription's activation date and the billing period end date. Subsequent periods will be based off the plan interval. For a subscription with a trial period, this will change when the trial expires.
- total_billing_cycles int? - The number of cycles/billing periods in a term. When
remaining_billing_cycles=0
, ifauto_renew=true
the subscription will renew and a new term will begin, otherwise the subscription will expire.
- renewal_billing_cycles int? - If
auto_renew=true
, when a term completes,total_billing_cycles
takes this value as the length of subsequent terms. Defaults to the plan'stotal_billing_cycles
.
- auto_renew boolean(default true) - Whether the subscription renews at the end of its term.
- revenue_schedule_type string? -
- terms_and_conditions string? - This will default to the Terms and Conditions text specified on the Invoice Settings page in your Recurly admin. Specify custom notes to add or override Terms and Conditions. Custom notes will stay with a subscription on all renewals.
- customer_notes string? - This will default to the Customer Notes text specified on the Invoice Settings. Specify custom notes to add or override Customer Notes. Custom notes will stay with a subscription on all renewals.
- credit_customer_notes string? - If there are pending credits on the account that will be invoiced during the subscription creation, these will be used as the Customer Notes on the credit invoice.
- po_number string? - For manual invoicing, this identifies the PO number associated with the subscription.
- net_terms int(default 0) - Integer representing the number of days after an invoice's creation that the invoice will become past due. If an invoice's net terms are set to '0', it is due 'On Receipt' and will become past due 24 hours after it’s created. If an invoice is due net 30, it will become past due at 31 days exactly.
- transaction_type string? - An optional type designation for the payment gateway transaction created by this request. Supports 'moto' value, which is the acronym for mail order and telephone transactions.
recurly: SubscriptionList
Fields
- 'object string? - Will always be List.
- has_more boolean? - Indicates there are more results on subsequent pages.
- next string? - Path to subsequent page of results.
- data Subscription[]? -
recurly: SubscriptionPause
Fields
- remaining_pause_cycles int - Number of billing cycles to pause the subscriptions. A value of 0 will cancel any pending pauses on the subscription.
recurly: SubscriptionPurchase
Fields
- plan_code string -
- plan_id string? -
- unit_amount float? - Override the unit amount of the subscription plan by setting this value. If not provided, the subscription will inherit the price from the subscription plan for the provided currency.
- quantity int(default 1) - Optionally override the default quantity of 1.
- add_ons SubscriptionAddOnCreate[]? -
- custom_fields CustomFields? - The custom fields will only be altered when they are included in a request. Sending an empty array will not remove any existing values. To remove a field send the name with a null or empty value.
- shipping SubscriptionShippingPurchase? -
- trial_ends_at string? - If set, overrides the default trial behavior for the subscription. When the current date time or a past date time is provided the subscription will begin with no trial phase (overriding any plan default trial). When a future date time is provided the subscription will begin with a trial phase ending at the specified date time.
- starts_at string? - If set, the subscription will begin in the future on this date. The subscription will apply the setup fee and trial period, unless the plan has no trial.
- next_bill_date string? - If present, this sets the date the subscription's next billing period will start (
current_period_ends_at
). This can be used to align the subscription’s billing to a specific day of the month. The initial invoice will be prorated for the period between the subscription's activation date and the billing period end date. Subsequent periods will be based off the plan interval. For a subscription with a trial period, this will change when the trial expires.
- total_billing_cycles int? - The number of cycles/billing periods in a term. When
remaining_billing_cycles=0
, ifauto_renew=true
the subscription will renew and a new term will begin, otherwise the subscription will expire.
- renewal_billing_cycles int? - If
auto_renew=true
, when a term completes,total_billing_cycles
takes this value as the length of subsequent terms. Defaults to the plan'stotal_billing_cycles
.
- auto_renew boolean(default true) - Whether the subscription renews at the end of its term.
- revenue_schedule_type string? -
recurly: SubscriptionShipping
Fields
- 'object string? -
- address ShippingAddress? -
- method ShippingMethodMini? -
- amount float? -
recurly: SubscriptionShippingCreate
Fields
- address ShippingAddressCreate? -
- address_id string? - Assign a shipping address from the account's existing shipping addresses. If
address_id
andaddress
are both present,address
will be used.
- method_id string? - The id of the shipping method used to deliver the subscription. If
method_id
andmethod_code
are both present,method_id
will be used.
- method_code string? - The code of the shipping method used to deliver the subscription. If
method_id
andmethod_code
are both present,method_id
will be used.
- amount float? -
recurly: SubscriptionShippingPurchase
Fields
- method_id string? - The id of the shipping method used to deliver the subscription. If
method_id
andmethod_code
are both present,method_id
will be used.
- method_code string? - The code of the shipping method used to deliver the subscription. If
method_id
andmethod_code
are both present,method_id
will be used.
- amount float? -
recurly: SubscriptionShippingUpdate
Fields
- 'object string? -
- address ShippingAddressCreate? -
- address_id string? - Assign a shipping address from the account's existing shipping addresses.
recurly: SubscriptionUpdate
Fields
- collection_method string? -
- custom_fields CustomFields? - The custom fields will only be altered when they are included in a request. Sending an empty array will not remove any existing values. To remove a field send the name with a null or empty value.
- remaining_billing_cycles int? - The remaining billing cycles in the current term.
- renewal_billing_cycles int? - If
auto_renew=true
, when a term completes,total_billing_cycles
takes this value as the length of subsequent terms. Defaults to the plan'stotal_billing_cycles
.
- auto_renew boolean? - Whether the subscription renews at the end of its term.
- next_bill_date string? - If present, this sets the date the subscription's next billing period will start (
current_period_ends_at
). This can be used to align the subscription’s billing to a specific day of the month. For a subscription in a trial period, this will change when the trial expires. This parameter is useful for postponement of a subscription to change its billing date without proration.
- revenue_schedule_type string? -
- terms_and_conditions string? - Specify custom notes to add or override Terms and Conditions. Custom notes will stay with a subscription on all renewals.
- customer_notes string? - Specify custom notes to add or override Customer Notes. Custom notes will stay with a subscription on all renewals.
- po_number string? - For manual invoicing, this identifies the PO number associated with the subscription.
- net_terms int? - Integer representing the number of days after an invoice's creation that the invoice will become past due. If an invoice's net terms are set to '0', it is due 'On Receipt' and will become past due 24 hours after it’s created. If an invoice is due net 30, it will become past due at 31 days exactly.
- shipping SubscriptionShippingUpdate? -
- billing_info_id string? - The
billing_info_id
is the value that represents a specific billing info for an end customer. Whenbilling_info_id
is used to assign billing info to the subscription, all future billing events for the subscription will bill to the specified billing info.
recurly: TaxDetail
Fields
- 'type string? - Provides the tax type for the region. For Canadian Sales Tax, this will be GST, HST, QST or PST.
- region string? - Provides the tax region applied on an invoice. For Canadian Sales Tax, this will be either the 2 letter province code or country code.
- rate float? - Provides the tax rate for the region.
- tax float? - The total tax applied for this tax type.
recurly: TaxInfo
Fields
- 'type string? - Provides the tax type as "vat" for EU VAT, "usst" for U.S. Sales Tax, or the 2 letter country code for country level tax types like Canada, Australia, New Zealand, Israel, and all non-EU European countries.
- region string? - Provides the tax region applied on an invoice. For U.S. Sales Tax, this will be the 2 letter state code. For EU VAT this will be the 2 letter country code. For all country level tax types, this will display the regional tax, like VAT, GST, or PST.
- rate float? -
- tax_details TaxDetail[]? - Provides additional tax details for Canadian Sales Tax when there is tax applied at both the country and province levels. This will only be populated for the Invoice response when fetching a single invoice and not for the InvoiceList or LineItem.
recurly: Tier
Fields
- ending_quantity int? - Ending quantity for the tier. This represents a unit amount for unit-priced add ons, but for percentage type usage add ons, represents the site default currency in its minimum divisible unit.
- usage_percentage string? - Decimal usage percentage.
- currencies TierPricing[]? -
recurly: TierPricing
Fields
- currency string - 3-letter ISO 4217 currency code.
- unit_amount float? - Allows up to 2 decimal places. Required unless
unit_amount_decimal
is provided.
- unit_amount_decimal string? - Allows up to 9 decimal places. Only supported when
add_on_type
=usage
. Ifunit_amount_decimal
is provided,unit_amount
cannot be provided.
recurly: Transaction
Fields
- id string? -
- 'object string? -
- uuid string? - The UUID is useful for matching data with the CSV exports and building URLs into Recurly's UI.
- original_transaction_id string? - If this transaction is a refund (
type=refund
), this will be the ID of the original transaction on the invoice being refunded.
- account AccountMini? -
- invoice InvoiceMini? -
- voided_by_invoice InvoiceMini? -
- subscription_ids TransactionSubscriptionidsItemsString[]? - If the transaction is charging or refunding for one or more subscriptions, these are their IDs.
- 'type string? -
authorization
– verifies billing information and places a hold on money in the customer's account.capture
– captures funds held by an authorization and completes a purchase.purchase
– combines the authorization and capture in one transaction.refund
– returns all or a portion of the money collected in a previous transaction to the customer.verify
– a $0 or $1 transaction used to verify billing information which is immediately voided.
- origin string? - Describes how the transaction was triggered.
- currency string? - 3-letter ISO 4217 currency code.
- amount float? - Total transaction amount sent to the payment gateway.
- status string? - The current transaction status. Note that the status may change, e.g. a
pending
transaction may becomedeclined
orsuccess
may later becomevoid
.
- success boolean? - Did this transaction complete successfully?
- backup_payment_method_used boolean? - Indicates if the transaction was completed using a backup payment
- refunded boolean? - Indicates if part or all of this transaction was refunded.
- billing_address AddressWithName? -
- collection_method string? - The method by which the payment was collected.
- payment_method PaymentMethod? -
- ip_address_v4 string? - IP address provided when the billing information was collected:
- When the customer enters billing information into the Recurly.js or Hosted Payment Pages, Recurly records the IP address.
- When the merchant enters billing information using the API, the merchant may provide an IP address.
- When the merchant enters billing information using the UI, no IP address is recorded.
- ip_address_country string? -
- status_code string? -
- status_message string? - For declined (
success=false
) transactions, the message displayed to the merchant.
- customer_message string? - For declined (
success=false
) transactions, the message displayed to the customer.
- customer_message_locale string? -
- payment_gateway TransactionPaymentGateway? -
- gateway_message string? - Transaction message from the payment gateway.
- gateway_reference string? - Transaction reference number from the payment gateway.
- gateway_approval_code string? -
- gateway_response_code string? -
- gateway_response_time float? - Time, in seconds, for gateway to process the transaction.
- gateway_response_values record {}? - The values in this field will vary from gateway to gateway.
- cvv_check string? - When processed, result from checking the CVV/CVC value on the transaction.
- avs_check string? - When processed, result from checking the overall AVS on the transaction.
- created_at string? -
- updated_at string? -
- voided_at string? -
- collected_at string? -
recurly: TransactionErrorDetails
This is only included on errors with type=transaction
.
Fields
- 'object string? -
- transaction_id string? -
- category string? -
- code string? -
- message string? -
- merchant_advice string? -
- three_d_secure_action_token_id string? - Returned when 3-D Secure authentication is required for a transaction. Pass this value to Recurly.js so it can continue the challenge flow.
recurly: TransactionList
Fields
- 'object string? - Will always be List.
- has_more boolean? - Indicates there are more results on subsequent pages.
- next string? - Path to subsequent page of results.
- data Transaction[]? -
recurly: TransactionPaymentGateway
Fields
- id string? -
- 'object string? -
- 'type string? -
- name string? -
recurly: UniqueCouponCode
A unique coupon code for a bulk coupon.
Fields
- id string? -
- 'object string? -
- code string? - The code the customer enters to redeem the coupon.
- state string? - Indicates if the unique coupon code is redeemable or why not.
- bulk_coupon_id string? - The Coupon ID of the parent Bulk Coupon
- bulk_coupon_code string? - The Coupon code of the parent Bulk Coupon
- created_at string? -
- updated_at string? -
- redeemed_at string? - The date and time the unique coupon code was redeemed.
- expired_at string? - The date and time the coupon was expired early or reached its
max_redemptions
.
recurly: UniqueCouponCodeList
Fields
- 'object string? - Will always be List.
- has_more boolean? - Indicates there are more results on subsequent pages.
- next string? - Path to subsequent page of results.
- data UniqueCouponCode[]? -
recurly: UniqueCouponCodeParams
Parameters to be passed to the list_unique_coupon_codes
endpoint to obtain the newly generated codes.
Fields
- 'limit int? -
- 'order string? -
- sort string? -
- begin_time string? - The date-time to be included when listing UniqueCouponCodes
recurly: Usage
Fields
- id string? -
- 'object string? -
- merchant_tag string? - Custom field for recording the id in your own system associated with the usage, so you can provide auditable usage displays to your customers using a GET on this endpoint.
- amount float? - The amount of usage. Can be positive, negative, or 0. No decimals allowed, we will strip them. If the usage-based add-on is billed with a percentage, your usage will be a monetary amount you will want to format in cents. (e.g., $5.00 is "500").
- usage_type string? - Type of usage, returns usage type if
add_on_type
isusage
.
- tier_type string? - The pricing model for the add-on. For more information, click here. See our Guide for an overview of how to configure quantity-based pricing models.
- tiers SubscriptionAddOnTier[]? - The tiers and prices of the subscription based on the usage_timestamp. If tier_type = flat, tiers = null
- measured_unit_id string? - The ID of the measured unit associated with the add-on the usage record is for.
- recording_timestamp string? - When the usage was recorded in your system.
- usage_timestamp string? - When the usage actually happened. This will define the line item dates this usage is billed under and is important for revenue recognition.
- usage_percentage float? - The percentage taken of the monetary amount of usage tracked. This can be up to 4 decimal places. A value between 0.0 and 100.0.
- unit_amount float? -
- unit_amount_decimal string? - Unit price that can optionally support a sub-cent value.
- billed_at string? - When the usage record was billed on an invoice.
- created_at string? - When the usage record was created in Recurly.
- updated_at string? - When the usage record was billed on an invoice.
recurly: UsageCreate
Fields
- merchant_tag string? - Custom field for recording the id in your own system associated with the usage, so you can provide auditable usage displays to your customers using a GET on this endpoint.
- amount float? - The amount of usage. Can be positive, negative, or 0. No decimals allowed, we will strip them. If the usage-based add-on is billed with a percentage, your usage will be a monetary amount you will want to format in cents. (e.g., $5.00 is "500").
- recording_timestamp string? - When the usage was recorded in your system.
- usage_timestamp string? - When the usage actually happened. This will define the line item dates this usage is billed under and is important for revenue recognition.
recurly: UsageList
Fields
- 'object string? - Will always be List.
- has_more boolean? - Indicates there are more results on subsequent pages.
- next string? - Path to subsequent page of results.
- data Usage[]? -
recurly: User
Fields
- id string? -
- 'object string? -
- email string? -
- first_name string? -
- last_name string? -
- time_zone string? -
- created_at string? -
- deleted_at string? -
String types
Import
import ballerinax/recurly;
Metadata
Released date: almost 2 years ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 5
Current verison: 4
Weekly downloads
Keywords
Finance/Billing
Cost/Paid
Contributors