reckon.one
Module reckon.one
API
Definitions
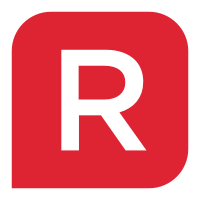
ballerinax/reckon.one Ballerina library
Overview
This is a generated connector for Reckon One API v2 OpenAPI specification. Reckon One is a new generation, modular cloud accounting solution that scales with your business – perfect for startups. This is version 2 of the Reckon One API.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Reckon account
- Obtain tokens by following this guide
Quickstart
To use the Reckon One connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/reckon.one
module into the Ballerina project.
import ballerinax/reckon.one;
Step 2: Create a new connector instance
Create a one:ClientConfig
with the Bearer_Token
obtained, and initialize the connector with it.
one:ClientConfig clientConfig = { auth: { token: <Bearer_Token> } }; one:Client baseClient = check new Client(clientConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to list books that the current user has access to, using the connector.
Gets a list of books that the current user has access to.
public function main() returns error? { one:Books response = check baseClient->getBooks(); log:printInfo(response.toString()); }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
reckon.one: Client
This is a generated connector for Reckon One API v2 OpenAPI specification. Reckon One is a new generation, modular cloud accounting solution that scales with your business – perfect for startups. This is version 2 of the Reckon One API.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create an Reckon account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://api-v2.reckonone.com/" - URL of the target service
getBooks
Gets a list of books that the current user has access to.
getBook
Gets details of the specified book.
Parameters
- bookId string - The book's id.
getCreditNotes
function getCreditNotes(string bookId, int page, int perPage) returns CreditNotes|error
Gets a list of credit notes.
Parameters
- bookId string - The book's id.
- page int (default 1) - The page to retrieve.
- perPage int (default 0) - The number of credit notes per page.
Return Type
- CreditNotes|error - A paged list of credit notes for the specified book.
getCreditNote
function getCreditNote(string bookId, string creditNoteId, string? format) returns CreditNote|error
Gets a credit note.
Parameters
- bookId string - The book's id.
- creditNoteId string - The credit note's id.
- format string? (default ()) - The format to use, e.g. pdf.
Return Type
- CreditNote|error - The requested credit note.
updateCreditNote
function updateCreditNote(string bookId, string creditNoteId, CreditNoteInput payload) returns Response|error
Updates an existing credit note.
Parameters
- bookId string - The book's id.
- creditNoteId string - The credit note's id.
- payload CreditNoteInput - Details of the updated credit note.
deleteCreditNote
Deletes an existing credit note.
patchCreditNote
function patchCreditNote(string bookId, string creditNoteId, CreditNotePatch payload) returns Response|error
Updates selected fields of an existing credit note.
Parameters
- bookId string - The book's id.
- creditNoteId string - The credit note's id.
- payload CreditNotePatch - Details to update.
createCreditNote
function createCreditNote(string bookId, CreditNoteInput payload) returns IdResponse|error
Creates a new credit note.
Return Type
- IdResponse|error - The id of the credit note that has been created.
getCreditNoteLineItem
function getCreditNoteLineItem(string bookId, string creditNoteId, string lineItemId) returns CreditNoteLineItem|error
Gets a line item from a credit note.
Parameters
- bookId string - The book's id.
- creditNoteId string - The credit note's id.
- lineItemId string - The line item's id.
Return Type
- CreditNoteLineItem|error - The requested credit note line item.
updateCreditNoteLineItem
function updateCreditNoteLineItem(string bookId, string creditNoteId, string lineItemId, CreditNoteLineItemInput payload) returns Response|error
Updates an existing credit note's line item.
Parameters
- bookId string - The book's id.
- creditNoteId string - The credit note's id.
- lineItemId string - The credit note line item's id.
- payload CreditNoteLineItemInput - Details of the updated credit note's line item.
deleteLineItem
function deleteLineItem(string bookId, string creditNoteId, string lineItemId) returns Response|error
Deletes an existing credit note line item.
Parameters
- bookId string - The book's id.
- creditNoteId string - The credit note's id.
- lineItemId string - The id of the line item to delete.
patchCreditNoteLineItem
function patchCreditNoteLineItem(string bookId, string creditNoteId, string lineItemId, CreditNoteLineItemPatch payload) returns Response|error
Updates selected fields of an existing credit note's line item.
Parameters
- bookId string - The book's id.
- creditNoteId string - The credit note's id.
- lineItemId string - The credit note line item's id.
- payload CreditNoteLineItemPatch - Details to update.
addLineItemToCreditNote
function addLineItemToCreditNote(string bookId, string creditNoteId, CreditNoteLineItemInput payload) returns IdResponse|error
Creates a new line item within an existing credit note.
Parameters
- bookId string - The book's id.
- creditNoteId string - The credit note's id.
- payload CreditNoteLineItemInput - Details of the new line item.
Return Type
- IdResponse|error - The id of the line item that has been created.
createCreditNoteTransactionLink
function createCreditNoteTransactionLink(string bookId, string creditNoteId, CreditNoteTransactionLinkInput payload) returns StringIdResponse|error
Creates a new transaction link.
Parameters
- bookId string - The book's id.
- creditNoteId string - The credit note's id.
- payload CreditNoteTransactionLinkInput - Details of the transaction to link.
Return Type
- StringIdResponse|error - The linked transaction's id.
unlinkTransaction
function unlinkTransaction(string bookId, string creditNoteId, string linkId) returns Response|error
Unlinks an existing transaction from a credit note.
Parameters
- bookId string - The book's id.
- creditNoteId string - The credit note's id.
- linkId string - The link's id.
patchCreditNoteTransactionLink
function patchCreditNoteTransactionLink(string bookId, string creditNoteId, string linkId, CreditNoteTransactionLinkPatch payload) returns Response|error
Updates selected fields of an existing credit note's linked transaction.
Parameters
- bookId string - The book's id.
- creditNoteId string - The credit note's id.
- linkId string - The linked transaction's id.
- payload CreditNoteTransactionLinkPatch - Details of the linked transaction to update.
getCustomers
Gets a list of customers.
Parameters
- bookId string - The book's id.
- page int (default 1) - The page to retrieve.
- perPage int (default 0) - The number of customers per page.
createCustomer
function createCustomer(string bookId, CustomerInput payload) returns IdNameResponse|error
Creates a new customer.
Return Type
- IdNameResponse|error - The id of the customer that has been created.
getCustomer
Gets a customer.
updateCustomer
function updateCustomer(string bookId, string customerId, CustomerInput payload) returns Response|error
Updates an existing customer.
Parameters
- bookId string - The book's id.
- customerId string - The customer's id.
- payload CustomerInput - Details of the updated customer.
deleteCustomer
Deletes an existing customer.
patchCustomer
function patchCustomer(string bookId, string customerId, CustomerPatch payload) returns Response|error
Updates selected fields of an existing customer.
Parameters
- bookId string - The book's id.
- customerId string - The customer's id.
- payload CustomerPatch - Details of the customer to change.
linkExistingContactToCustomer
function linkExistingContactToCustomer(string bookId, string customerId, string contactId) returns Response|error
Links an existing contact to a customer.
Parameters
- bookId string - The book's id.
- customerId string - The customer's id.
- contactId string - The contact's id to be linked to the customer.
unlinkCustomerLinkedContact
function unlinkCustomerLinkedContact(string bookId, string customerId, string contactId) returns Response|error
Unlinks a customer's linked contact.
Parameters
- bookId string - The book's id.
- customerId string - The customer's id.
- contactId string - The customer's linked contact's id.
addNewContactToCustomer
function addNewContactToCustomer(string bookId, string customerId, LinkedContactInput payload) returns IdResponse|error
Creates a new contact and links it to the customer.
Parameters
- bookId string - The book's id.
- customerId string - The customer's id.
- payload LinkedContactInput - Details of the new contact.
Return Type
- IdResponse|error - The id of the contact that has been created.
getCustomerLinkedContact
function getCustomerLinkedContact(string bookId, string customerId, string contactId) returns Contact|error
Gets a customer's linked Contact details.
Parameters
- bookId string - The book's id.
- customerId string - The customer's id.
- contactId string - The contact's id.
deleteContactFromCustomer
function deleteContactFromCustomer(string bookId, string customerId, string contactId) returns Response|error
Deletes a contact from a customer
Parameters
- bookId string - The book's id
- customerId string - The customer's id
- contactId string - The contact's id being deleted
getCustomerPhoneNumbers
function getCustomerPhoneNumbers(string bookId, string customerId) returns PhoneNumbers|error
Gets a customer's phone numbers.
Return Type
- PhoneNumbers|error - The requested customer's phone numbers.
createCustomerPhoneNumber
function createCustomerPhoneNumber(string bookId, string customerId, PhoneNumberInput payload) returns TypeResponse|error
Adds a new phone number to an existing customer.
Parameters
- bookId string - The book's id.
- customerId string - The customer's id.
- payload PhoneNumberInput - The details of the phone number to add.
Return Type
- TypeResponse|error - The id and name of the phone number type that has been created.
getCustomerPhoneNumber
function getCustomerPhoneNumber(string bookId, string customerId, string 'type) returns PhoneNumber|error
Get a customer's phone number.
Parameters
- bookId string - The book's id.
- customerId string - The customer's id.
- 'type string - The phone number's type by name or id.
Return Type
- PhoneNumber|error - The customer's specific phone number.
updateCustomerPhoneNumber
function updateCustomerPhoneNumber(string bookId, string customerId, string 'type, UpdatePhoneNumberInput payload) returns Response|error
Updates a customer's phone number.
Parameters
- bookId string - The book's id.
- customerId string - The customer's id.
- 'type string - The phone number type by name or id.
- payload UpdatePhoneNumberInput - The phone number.
deleteCustomerPhoneNumber
function deleteCustomerPhoneNumber(string bookId, string customerId, string 'type) returns Response|error
Deletes an existing customer phone number.
Parameters
- bookId string - The book's id.
- customerId string - The customer's id.
- 'type string - The phone number's type by name or id.
patchCustomerPhoneNumber
function patchCustomerPhoneNumber(string bookId, string customerId, string 'type, PhoneNumberPatch payload) returns Response|error
Updates selected fields of an existing customer phone number.
Parameters
- bookId string - The book's id.
- customerId string - The customer's id.
- 'type string - The phone number's type by name or id.
- payload PhoneNumberPatch - Details of the phone number to change.
getCustomerAddresses
Gets a customer's addresses.
createCustomerAddress
function createCustomerAddress(string bookId, string customerId, AddressInput payload) returns TypeResponse|error
Adds a new address to an existing customer.
Parameters
- bookId string - The book's id.
- customerId string - The customer's id.
- payload AddressInput - Details of the new address.
Return Type
- TypeResponse|error - The id of the address that has been created.
getCustomerAddress
Gets a customer's address.
Parameters
- bookId string - The book's id.
- customerId string - The customer's id.
- 'type string - The address's type by name or id.
updateCustomerAddress
function updateCustomerAddress(string bookId, string customerId, string 'type, UpdateAddressInput payload) returns Response|error
Updates a customer's address.
Parameters
- bookId string - The book's id.
- customerId string - The customer's id.
- 'type string - The address's type by name or id.
- payload UpdateAddressInput - The address.
deleteCustomerAddress
function deleteCustomerAddress(string bookId, string customerId, string 'type) returns Response|error
Deletes an existing customer address.
Parameters
- bookId string - The book's id.
- customerId string - The customer's id.
- 'type string - The address's type by name or id.
patchCustomerAddress
function patchCustomerAddress(string bookId, string customerId, string 'type, AddressPatch payload) returns Response|error
Updates selected fields of an existing customer address.
Parameters
- bookId string - The book's id.
- customerId string - The customer's id.
- 'type string - The address's type by name or id.
- payload AddressPatch - Details of the customer's address to change.
getCustomerElectronicAddress
function getCustomerElectronicAddress(string bookId, string customerId, string 'type) returns ElectronicAddress|error
Gets a customer's electronic address.
Parameters
- bookId string - The book's id.
- customerId string - The customer's id.
- 'type string - The electronic address's type by name or id.
Return Type
- ElectronicAddress|error - The customer's specific electronic address.
updateCustomerElectronicAddress
function updateCustomerElectronicAddress(string bookId, string customerId, string 'type, UpdateElectronicAddressInput payload) returns Response|error
Updates a customer's electronic address.
Parameters
- bookId string - The book's id.
- customerId string - The customer's id.
- 'type string - The electronic address's type by name or id.
- payload UpdateElectronicAddressInput - The electronic address.
deleteCustomerElectronicAddress
function deleteCustomerElectronicAddress(string bookId, string customerId, string 'type) returns Response|error
Deletes an existing customer electronic address.
Parameters
- bookId string - The book's id.
- customerId string - The customer's id.
- 'type string - The electronic address's type by name or id.
patchCustomerElectronicAddress
function patchCustomerElectronicAddress(string bookId, string customerId, string 'type, ElectronicAddressPatch payload) returns Response|error
Updates selected fields of an existing customer electronic address.
Parameters
- bookId string - The book's id.
- customerId string - The customer's id.
- 'type string - The electronic address type by name or id.
- payload ElectronicAddressPatch - Details of the customer's electronic address to update.
getCustomerElectronicAddresses
function getCustomerElectronicAddresses(string bookId, string customerId) returns ElectronicAddresses|error
Gets a customer's electronic addresses.
Return Type
- ElectronicAddresses|error - The requested customer's electronic addresses.
createCustomerElectronicAddress
function createCustomerElectronicAddress(string bookId, string customerId, ElectronicAddressInput payload) returns TypeResponse|error
Adds a new electronic address to an existing customer.
Parameters
- bookId string - The book's id.
- customerId string - The customer's id.
- payload ElectronicAddressInput - The details of the electronic address to add.
Return Type
- TypeResponse|error - The id and name of the electronic address type that has been created.
getDepartments
function getDepartments(string bookId, int page, int perPage) returns Departments|error
Gets a list of departments.
Parameters
- bookId string - The book's id.
- page int (default 1) - The page to retrieve.
- perPage int (default 0) - The number of departments per page.
Return Type
- Departments|error - A paged list of departments for the specified book.
createDepartment
function createDepartment(string bookId, DepartmentInput payload) returns IdResponse|error
Creates a new department.
Return Type
- IdResponse|error - The id of the department that has been created.
getDepartment
function getDepartment(string bookId, string departmentId) returns Department|error
Gets a department.
Return Type
- Department|error - The requested department.
updateDepartment
function updateDepartment(string bookId, string departmentId, DepartmentInput payload) returns Response|error
Updates an existing department.
Parameters
- bookId string - The book's id.
- departmentId string - The department's id.
- payload DepartmentInput - Details of the department.
deleteDepartment
Deletes an existing department.
patchDepartment
function patchDepartment(string bookId, string departmentId, DepartmentPatch payload) returns Response|error
Updates selected fields of an existing department.
Parameters
- bookId string - The book's id.
- departmentId string - The departments's id.
- payload DepartmentPatch - Details to update.
linkManagerToDepartment
function linkManagerToDepartment(string bookId, string departmentId, string employeeId) returns Response|error
Links a manager to a department.
Parameters
- bookId string - The book's id.
- departmentId string - The department's id.
- employeeId string - The manager's id to be linked to the department.
unlinkDepartmentLinkedManager
function unlinkDepartmentLinkedManager(string bookId, string departmentId, string employeeId) returns Response|error
Unlinks a manager from a department.
Parameters
- bookId string - The book's id.
- departmentId string - The department's id.
- employeeId string - The manager's id linked to the department.
linkEmployeeToDepartment
function linkEmployeeToDepartment(string bookId, string departmentId, string employeeId) returns Response|error
Links an employee to a department.
Parameters
- bookId string - The book's id.
- departmentId string - The department's id.
- employeeId string - The employees id to be linked to the department.
unlinkDepartmentLinkedEmployee
function unlinkDepartmentLinkedEmployee(string bookId, string departmentId, string employeeId) returns Response|error
Unlinks an employee from a department.
Parameters
- bookId string - The book's id.
- departmentId string - The department's id.
- employeeId string - The employees id linked to the department.
getPaymentMethods
function getPaymentMethods(string bookId) returns PaymentMethods|error
Gets a list of payment methods.
Parameters
- bookId string - The book's id.
Return Type
- PaymentMethods|error - A list of payment methods for the specified book.
getPayments
Get a list of payments.
Parameters
- bookId string - The book's id.
- page int (default 1) - The page to retrieve.
- perPage int (default 0) - The number of payments per page.
getPayment
Get a payment.
Parameters
- bookId string - The book's id.
- paymentId string - The payments id.
- format string? (default ()) - The format to use, e.g. pdf.
getUserPermissions
function getUserPermissions(string bookId) returns Permissions|error
Gets the current user's permissions.
Parameters
- bookId string - The book's id.
Return Type
- Permissions|error - An array of the current user's permissions.
getReceipts
Gets a list of receipts.
Parameters
- bookId string - The book's id.
- page int (default 1) - The page to retrieve.
- perPage int (default 0) - The number of receipts per page.
createReceipt
function createReceipt(string bookId, ReceiptInput payload) returns IdResponse|error
Creates a new receipt.
Return Type
- IdResponse|error - The id of the receipt that has been created.
getReceipt
Gets a receipt.
Parameters
- bookId string - The book's id.
- receiptId string - The receipt's id.
- format string? (default ()) - The format to use, e.g. pdf.
updateReceipt
function updateReceipt(string bookId, string receiptId, ReceiptInput payload) returns Response|error
Updates an existing receipt.
Parameters
- bookId string - The book's id.
- receiptId string - The receipt's id.
- payload ReceiptInput - Details of the updated receipt.
deleteReceipt
Deletes an existing receipt.
patchReceipt
function patchReceipt(string bookId, string receiptId, ReceiptPatch payload) returns Response|error
Updates selected fields of an existing receipt.
Parameters
- bookId string - The book's id.
- receiptId string - The receipt's id.
- payload ReceiptPatch - Details of the receipt to update.
getReceiptLineItem
function getReceiptLineItem(string bookId, string receiptId, string lineItemId) returns ReceiptLineItem|error
Gets a line item from a receipt.
Parameters
- bookId string - The book's id.
- receiptId string - The receipt's id.
- lineItemId string - The line item's id.
Return Type
- ReceiptLineItem|error - The line item.
updateReceiptLineItem
function updateReceiptLineItem(string bookId, string receiptId, string lineItemId, ReceiptLineItemInput payload) returns Response|error
Updates an existing line item within an existing receipt.
Parameters
- bookId string - The book's id.
- receiptId string - The receipt's id.
- lineItemId string - The line item's id.
- payload ReceiptLineItemInput - Details of the line item.
addLineItemToReceipt
function addLineItemToReceipt(string bookId, string receiptId, ReceiptLineItemInput payload) returns IdResponse|error
Creates a new line item within an existing receipt.
Parameters
- bookId string - The book's id.
- receiptId string - The receipt's id.
- payload ReceiptLineItemInput - Details of the new line item.
Return Type
- IdResponse|error - The id of the line item that has been created.
patchReceiptLineItem
function patchReceiptLineItem(string bookId, string receiptId, string lineItemId, ReceiptLineItemPatch payload) returns Response|error
Updates selected fields of an existing line item within an existing receipt.
Parameters
- bookId string - The book's id.
- receiptId string - The receipt's id.
- lineItemId string - The id of the line item to update.
- payload ReceiptLineItemPatch - Details to update.
deleteReceiptLineItem
function deleteReceiptLineItem(string bookId, string receiptId, string lineId) returns Response|error
Deletes an existing receipt's line item.
Parameters
- bookId string - The book's id.
- receiptId string - The receipt's id.
- lineId string - The receipt's line item id.
createReceiptTransactionLink
function createReceiptTransactionLink(string bookId, string receiptId, ReceiptTransactionLinkInput payload) returns StringIdResponse|error
Creates a new transaction link.
Parameters
- bookId string - The book's id.
- receiptId string - The receipt's id.
- payload ReceiptTransactionLinkInput - Details of the transaction to link.
Return Type
- StringIdResponse|error - The linked transaction's id.
deleteReceiptTransactionLink
function deleteReceiptTransactionLink(string bookId, string receiptId, string linkId) returns Response|error
Deletes an existing receipt's linked transaction.
Parameters
- bookId string - The book's id.
- receiptId string - The receipt's id.
- linkId string - The receipt's allocation link id.
patchReceiptTransactionLink
function patchReceiptTransactionLink(string bookId, string receiptId, string linkId, ReceiptTransactionLinkPatch payload) returns Response|error
Updates selected fields of an existing receipt's linked transaction.
Parameters
- bookId string - The book's id.
- receiptId string - The receipt's id.
- linkId string - The linked transaction's id.
- payload ReceiptTransactionLinkPatch - Details of the linked transaction to update.
getRoles
Gets a list of roles.
Parameters
- bookId string - The book's id.
- page int (default 1) - The page to retrieve.
- perPage int (default 0) - The number of roles per page.
getRole
Gets a role.
linkUserToRole
Links a user to a role.
Parameters
- bookId string - The book's id.
- roleId int - The role's id.
- userId string - The user's id to be linked to the role.
getSuperfundProviders
function getSuperfundProviders(string bookId, int page, int perPage) returns SuperfundProviders|error
Gets a list of super fund providers.
Parameters
- bookId string - The book's id.
- page int (default 1) - The page to retrieve.
- perPage int (default 0) - The number of super fund providers per page.
Return Type
- SuperfundProviders|error - A paged list of super fund providers for the specified book.
getSuperfundProvidersHavingUsi
function getSuperfundProvidersHavingUsi(string bookId, string usi, int page, int perPage) returns SuperfundProviders|error
Gets a list of super fund providers having a product with the provided USI.
Parameters
- bookId string - The book Id.
- usi string - The USI of a fund product.
- page int (default 1) - The page to retrieve.
- perPage int (default 0) - The number of super fund providers having a product with the provided USI per page.
Return Type
- SuperfundProviders|error - A paged list of super fund providers having a product with the provided USI for the specified book.
getSuperfundProvider
function getSuperfundProvider(string bookId, string superfundProviderId) returns SuperfundProvider|error
Gets a super fund provider.
Return Type
- SuperfundProvider|error - The requested super fund provider.
getSuperfundProviderProducts
function getSuperfundProviderProducts(string bookId, string superfundProviderId, int page, int perPage) returns SuperfundProviderProducts|error
Gets a list of a super fund provider's products.
Parameters
- bookId string - The book's id.
- superfundProviderId string - The super fund provider's id.
- page int (default 1) - The page to retrieve.
- perPage int (default 0) - The number of super fund provider products per page.
Return Type
- SuperfundProviderProducts|error - A paged list of a super fund provider's products for the specified book.
getReports
Gets a list of reports.
Parameters
- bookId string - The book's id.
getPayrollLeaveBalancesAndAccrualValueReport2
function getPayrollLeaveBalancesAndAccrualValueReport2(string bookId, PayrollLeaveBalancesAndAccrualValueReportParameters payload, string? format) returns PayrollLeaveBalancesAndAccrualValueReport|error
Gets the payroll leave balances and accrual value report.
Parameters
- bookId string - The book's id.
- payload PayrollLeaveBalancesAndAccrualValueReportParameters - The report parameters.
- format string? (default ()) - The format to use, e.g. pdf.
Return Type
- PayrollLeaveBalancesAndAccrualValueReport|error - The report.
getSuppliers
Gets a list of suppliers.
Parameters
- bookId string - The book's id.
- page int (default 1) - The page to retrieve.
- perPage int (default 0) - The number of suppliers per page.
createSupplier
function createSupplier(string bookId, SupplierInput payload) returns IdNameResponse|error
Creates a new supplier.
Return Type
- IdNameResponse|error - The id of the supplier that has been created.
getSupplier
Gets a supplier.
updateSupplier
function updateSupplier(string bookId, string supplierId, SupplierInput payload) returns Response|error
Updates an existing supplier.
Parameters
- bookId string - The book's id.
- supplierId string - The suppliers's id.
- payload SupplierInput - Details of the updated supplier.
deleteSupplier
Deletes an existing supplier.
patchSupplier
function patchSupplier(string bookId, string supplierId, SupplierPatch payload) returns Response|error
Updates selected fields of an existing supplier.
Parameters
- bookId string - The book's id.
- supplierId string - The supplier's id.
- payload SupplierPatch - Details of the supplier to change.
getSupplierAddresses
Gets a supplier's addresses.
createSupplierAddress
function createSupplierAddress(string bookId, string supplierId, AddressInput payload) returns Response|error
Adds a new address to an existing supplier.
Parameters
- bookId string - The book's id.
- supplierId string - The supplier's id.
- payload AddressInput - The address.
getSupplierAddress
Gets a supplier's address.
Parameters
- bookId string - The book's id.
- supplierId string - The supplier's id.
- 'type string - The type of address by name or id.
updateSupplierAddress
function updateSupplierAddress(string bookId, string supplierId, string 'type, UpdateAddressInput payload) returns Response|error
Updates an existing supplier address.
Parameters
- bookId string - The book's id.
- supplierId string - The supplier's id.
- 'type string - The address type by name or id.
- payload UpdateAddressInput - The address.
deleteSupplierAddress
function deleteSupplierAddress(string bookId, string supplierId, string 'type) returns Response|error
Deletes an existing supplier address.
Parameters
- bookId string - The book's id.
- supplierId string - The supplier's id.
- 'type string - The address's type by name or id.
patchSupplierAddress
function patchSupplierAddress(string bookId, string supplierId, string 'type, AddressPatch payload) returns Response|error
Updates selected fields of an existing supplier address.
Parameters
- bookId string - The book's id.
- supplierId string - The supplier's id.
- 'type string - The address type by name or id.
- payload AddressPatch - Details of the supplier's address to change.
getSuppliersLinkedContacts
function getSuppliersLinkedContacts(string bookId, string supplierId, int page, int perPage) returns Contacts|error
Gets the supplier's linked contacts.
Parameters
- bookId string - The book's id.
- supplierId string - The supplier's id.
- page int (default 1) - The page to retrieve.
- perPage int (default 0) - The number of supplier's contact per page.
addNewContactToSupplier
function addNewContactToSupplier(string bookId, string supplierId, LinkedContactInput payload) returns IdResponse|error
Creates a new contact and links it to the supplier.
Parameters
- bookId string - The book's id.
- supplierId string - The supplier's id.
- payload LinkedContactInput - Details of the new contact.
Return Type
- IdResponse|error - The id of the contact that has been created.
getSuppliersLinkedContact
function getSuppliersLinkedContact(string bookId, string supplierId, string contactId) returns Contact|error
Gets a supplier's linked contact details.
Parameters
- bookId string - The book's id.
- supplierId string - The supplier's id.
- contactId string - The supplier's linked contact id.
linkExistingContactToSupplier
function linkExistingContactToSupplier(string bookId, string supplierId, string contactId) returns Response|error
Links an existing contact to a supplier.
Parameters
- bookId string - The book's id.
- supplierId string - The supplier's id.
- contactId string - The contact's id to be linked to the supplier.
unlinkSupplierLinkedContact
function unlinkSupplierLinkedContact(string bookId, string supplierId, string contactId) returns Response|error
Unlinks a supplier's linked contact.
Parameters
- bookId string - The book's id.
- supplierId string - The supplier's id.
- contactId string - The supplier's linked contact's id.
getSupplierElectronicAddresses
function getSupplierElectronicAddresses(string bookId, string supplierId) returns ElectronicAddresses|error
Gets a supplier's electronic addresses.
Return Type
- ElectronicAddresses|error - The requested supplier's electronic addresses.
createSupplierElectronicAddress
function createSupplierElectronicAddress(string bookId, string supplierId, ElectronicAddressInput payload) returns TypeResponse|error
Adds a new electronic address to an existing supplier.
Parameters
- bookId string - The book's id.
- supplierId string - The supplier's id.
- payload ElectronicAddressInput - The details of the electronic address to add.
Return Type
- TypeResponse|error - The id and name of the electronic address type that has been created.
getSupplierElectronicAddress
function getSupplierElectronicAddress(string bookId, string supplierId, string 'type) returns ElectronicAddress|error
Gets a supplier's electronic address.
Parameters
- bookId string - The book's id.
- supplierId string - The supplier's id.
- 'type string - The electronic address type as either name or id.
Return Type
- ElectronicAddress|error - The supplier's requested electronic address.
updateSupplierElectronicAddress
function updateSupplierElectronicAddress(string bookId, string supplierId, string 'type, UpdateElectronicAddressInput payload) returns Response|error
Updates an existing supplier electronic address.
Parameters
- bookId string - The book's id.
- supplierId string - The supplier's id.
- 'type string - The electronic address's type by name or id.
- payload UpdateElectronicAddressInput - Details of the supplier's electronic address to update.
deleteElectronicAddress
function deleteElectronicAddress(string bookId, string supplierId, string 'type) returns Response|error
Deletes an existing supplier's electronic address.
Parameters
- bookId string - The book's id.
- supplierId string - The supplier's id.
- 'type string - The electronic address type's id or name.
patchSupplierElectronicAddress
function patchSupplierElectronicAddress(string bookId, string supplierId, string 'type, ElectronicAddressPatch payload) returns Response|error
Updates selected fields of an existing supplier electronic address.
Parameters
- bookId string - The book's id.
- supplierId string - The supplier's id.
- 'type string - The electronic address's type by name or id.
- payload ElectronicAddressPatch - Details of the supplier's electronic address to update.
getSupplierPhoneNumbers
function getSupplierPhoneNumbers(string bookId, string supplierId) returns PhoneNumbers|error
Gets a supplier's phone numbers.
Return Type
- PhoneNumbers|error - The requested supplier's phone numbers.
createSupplierPhoneNumber
function createSupplierPhoneNumber(string bookId, string supplierId, PhoneNumberInput payload) returns TypeResponse|error
Adds a new phone number to an existing supplier.
Parameters
- bookId string - The book's id.
- supplierId string - The supplier's id.
- payload PhoneNumberInput - Details of the phone number to add.
Return Type
- TypeResponse|error - The id and name of the phone number type that has been created.
getSupplierPhoneNumber
function getSupplierPhoneNumber(string bookId, string supplierId, string 'type) returns PhoneNumber|error
Get a supplier's phone number.
Parameters
- bookId string - The book's id.
- supplierId string - The supplier's id.
- 'type string - The phone number's type by name or id.
Return Type
- PhoneNumber|error - The supplier's specific phone number.
patchSupplierPhoneNumber
function patchSupplierPhoneNumber(string bookId, string supplierId, string 'type, PhoneNumberPatch payload) returns Response|error
Updates selected fields of an existing supplier phone number.
Parameters
- bookId string - The book's id.
- supplierId string - The supplier's id.
- 'type string - The phone number type by name or id.
- payload PhoneNumberPatch - Details of the phone number to change.
getSuperfunds
function getSuperfunds(string bookId, int page, int perPage) returns Superfunds|error
Gets a list of super funds.
Parameters
- bookId string - The book's id.
- page int (default 1) - The page to retrieve.
- perPage int (default 0) - The number of super funds per page.
Return Type
- Superfunds|error - A paged list of super funds for the specified book.
createSuperfund
function createSuperfund(string bookId, SuperfundInput payload) returns IdNameResponse|error
Creates a new super fund.
Return Type
- IdNameResponse|error - The id of the super fund that has been created.
getSuperfund
Gets a super fund.
updateSuperfund
function updateSuperfund(string bookId, string superfundId, SuperfundInput payload) returns Response|error
Updates an existing super fund.
Parameters
- bookId string - The book's id.
- superfundId string - The super fund's id.
- payload SuperfundInput - Details of the updated super fund.
deleteSuperfund
Deletes an existing super fund.
patchSuperfund
function patchSuperfund(string bookId, string superfundId, SuperfundPatch payload) returns Response|error
Updates selected fields of an existing super fund.
Parameters
- bookId string - The book's id.
- superfundId string - The super fund's id.
- payload SuperfundPatch - Details of the updated super fund to change.
getSuperfundsLinkedContacts
function getSuperfundsLinkedContacts(string bookId, string superfundId, int page, int perPage) returns Contacts|error
Gets a super fund's linked contacts.
Parameters
- bookId string - The book's id.
- superfundId string - The super fund's id.
- page int (default 1) - The page to retrieve.
- perPage int (default 0) - The number of super fund's contact per page.
addNewContactToSuperfund
function addNewContactToSuperfund(string bookId, string superfundId, LinkedContactInput payload) returns IdResponse|error
Creates a new contact and links it to the super fund.
Parameters
- bookId string - The book's id.
- superfundId string - The super fund's id.
- payload LinkedContactInput - Details of the new contact.
Return Type
- IdResponse|error - The id of the contact that has been created.
getSuperfundsLinkedContact
function getSuperfundsLinkedContact(string bookId, string superfundId, string contactId) returns Contact|error
Gets a super fund's linked contact.
Parameters
- bookId string - The book's id.
- superfundId string - The super fund's id.
- contactId string - The super fund's linked contact's id.
linkExistingContactToSuperfund
function linkExistingContactToSuperfund(string bookId, string superfundId, string contactId) returns Response|error
Links an existing contact to a specified super fund.
Parameters
- bookId string - The book's id.
- superfundId string - The super fund's id.
- contactId string - The contact's id to be linked to the super fund.
unlinkSuperfundsLinkedContact
function unlinkSuperfundsLinkedContact(string bookId, string superfundId, string contactId) returns Response|error
Unlinks a specified super fund's linked contact from that super fund.
Parameters
- bookId string - The book's id.
- superfundId string - The super fund's id.
- contactId string - The super fund's linked contact's id.
getSuperfundPhoneNumbers
function getSuperfundPhoneNumbers(string bookId, string superfundId) returns PhoneNumbers|error
Gets a super fund's phone numbers.
Return Type
- PhoneNumbers|error - The requested super fund's phone numbers.
createSuperfundPhoneNumber
function createSuperfundPhoneNumber(string bookId, string superfundId, PhoneNumberInput payload) returns TypeResponse|error
Adds a new phone number to an existing super fund.
Parameters
- bookId string - The book's id.
- superfundId string - The super fund's id.
- payload PhoneNumberInput - Details of the phone number to add.
Return Type
- TypeResponse|error - The id and name of the phone number type that has been created.
getSuperfundPhoneNumber
function getSuperfundPhoneNumber(string bookId, string superfundId, string 'type) returns PhoneNumber|error
Get a super fund's phone number.
Parameters
- bookId string - The book's id.
- superfundId string - The super fund's id.
- 'type string - The phone number's type by name or id.
Return Type
- PhoneNumber|error - The super fund's specific phone number.
patchSuperfundPhoneNumber
function patchSuperfundPhoneNumber(string bookId, string superfundId, string 'type, PhoneNumberPatch payload) returns Response|error
Updates selected fields of an existing super fund phone number.
Parameters
- bookId string - The book's id.
- superfundId string - The super fund's id.
- 'type string - The phone number's type by name or id.
- payload PhoneNumberPatch - Details of the phone number to change.
getSuperfundAddresses
Gets a super fund's addresses.
createSuperfundAddress
function createSuperfundAddress(string bookId, string superfundId, AddressInput payload) returns TypeResponse|error
Adds a new address to an existing super fund.
Parameters
- bookId string - The book's id.
- superfundId string - The super fund's id.
- payload AddressInput - The details of the address to add.
Return Type
- TypeResponse|error - The id and name of the address type that has been created.
getSuperfundAddress
Gets a super fund's address.
Parameters
- bookId string - The book's id.
- superfundId string - The super fund's id.
- 'type string - The type of address by name or id.
updateSuperfundAddress
function updateSuperfundAddress(string bookId, string superfundId, string 'type, UpdateAddressInput payload) returns Response|error
Updates an existing super fund address.
Parameters
- bookId string - The book's id.
- superfundId string - The super fund's id.
- 'type string - The address's type by name or id.
- payload UpdateAddressInput - The address.
deleteSuperfundAddress
function deleteSuperfundAddress(string bookId, string superfundId, string 'type) returns Response|error
Deletes an existing super fund address.
Parameters
- bookId string - The book's id.
- superfundId string - The super fund's id.
- 'type string - The address's type by name or id.
patchSuperfundAddress
function patchSuperfundAddress(string bookId, string superfundId, string 'type, AddressPatch payload) returns Response|error
Updates selected fields of an existing super fund address.
Parameters
- bookId string - The book's id.
- superfundId string - The super fund's id.
- 'type string - The address's type by name or id.
- payload AddressPatch - Details of the super fund's address to change.
getSuperfundElectronicAddresses
function getSuperfundElectronicAddresses(string bookId, string superfundId) returns ElectronicAddresses|error
Gets a super fund's electronic addresses.
Return Type
- ElectronicAddresses|error - The requested super fund's electronic addresses.
createSuperfundElectronicAddress
function createSuperfundElectronicAddress(string bookId, string superfundId, ElectronicAddressInput payload) returns TypeResponse|error
Adds a new electronic address to an existing super fund.
Parameters
- bookId string - The book's id.
- superfundId string - The super fund's id.
- payload ElectronicAddressInput - The details of the electronic address to add.
Return Type
- TypeResponse|error - The id and name of the electronic address type that has been created.
getSuperfundElectronicAddress
function getSuperfundElectronicAddress(string bookId, string superfundId, string 'type) returns ElectronicAddress|error
Gets a super fund's electronic address.
Parameters
- bookId string - The book's id.
- superfundId string - The super fund's id.
- 'type string - The electronic address's type by name or id.
Return Type
- ElectronicAddress|error - The super fund's requested electronic address.
updateSuperfundElectronicAddress
function updateSuperfundElectronicAddress(string bookId, string superfundId, string 'type, UpdateElectronicAddressInput payload) returns Response|error
Updates an existing super fund electronic address.
Parameters
- bookId string - The book's id.
- superfundId string - The super fund's id.
- 'type string - The electronic address's type by name or id.
- payload UpdateElectronicAddressInput - Details of the super fund's electronic address to update.
deleteSuperfundPhoneNumber
function deleteSuperfundPhoneNumber(string bookId, string superfundId, string 'type) returns Response|error
Deletes an existing super fund electronic address.
Parameters
- bookId string - The book's id.
- superfundId string - The super fund's id.
- 'type string - The electronic address's type by name or id.
patchSuperfundElectronicAddress
function patchSuperfundElectronicAddress(string bookId, string superfundId, string 'type, ElectronicAddressPatch payload) returns Response|error
Updates selected fields of an existing super fund electronic address.
Parameters
- bookId string - The book's id.
- superfundId string - The super fund's id.
- 'type string - The electronic address's type by name or id.
- payload ElectronicAddressPatch - Details of the super fund's electronic address to update.
getPhoneTypes
function getPhoneTypes(string bookId) returns PhoneTypes|error
Gets a list of phone types.
Parameters
- bookId string - The book's id.
Return Type
- PhoneTypes|error - A list of phone types for the specified book.
createPhoneType
function createPhoneType(string bookId, PhoneTypeInput payload) returns IdResponse|error
Creates a new phone type.
Return Type
- IdResponse|error - The id of the phone type that has been created.
getPhoneType
Gets a phone type.
updatePhoneType
function updatePhoneType(string bookId, string phoneTypeId, PhoneTypeInput payload) returns IdResponse|error
Updates an existing phone type.
Parameters
- bookId string - The book's id.
- phoneTypeId string - The phone type's id.
- payload PhoneTypeInput - Details of the updated phone type.
Return Type
- IdResponse|error - The result of the update.
deletePhoneType
Deletes an existing phone type.
patchPhoneType
function patchPhoneType(string bookId, string phoneTypeId, PhoneTypePatch payload) returns Response|error
Updates selected fields of an existing phone type.
Parameters
- bookId string - The book's id.
- phoneTypeId string - The phone type's id.
- payload PhoneTypePatch - Details of the updated phone type.
getAddressTypes
function getAddressTypes(string bookId) returns AddressTypes|error
Gets a list of address types.
Parameters
- bookId string - The book's id.
Return Type
- AddressTypes|error - A list of address types for the specified book.
createAddressType
function createAddressType(string bookId, AddressTypeInput payload) returns IdResponse|error
Creates a new address type.
Return Type
- IdResponse|error - The id of the address type that has been created.
getAddressType
function getAddressType(string bookId, string addressTypeId) returns AddressType|error
Gets an address type.
Return Type
- AddressType|error - The requested address type.
updateAddressType
function updateAddressType(string bookId, string addressTypeId, AddressTypeInput payload) returns Response|error
Updates an existing address type.
Parameters
- bookId string - The book's id.
- addressTypeId string - The address type's id.
- payload AddressTypeInput - Details of the updated address type.
deleteAddressType
Deletes an existing address type.
patchAddressType
function patchAddressType(string bookId, string addressTypeId, AddressTypePatch payload) returns Response|error
Updates selected fields of an existing address type.
Parameters
- bookId string - The book's id.
- addressTypeId string - The address type's id.
- payload AddressTypePatch - Details of the updated address type.
getContacts
Gets a list of contacts.
Parameters
- bookId string - The book's id.
- page int (default 1) - The page to retrieve.
- perPage int (default 0) - The number of contacts per page.
createContact
function createContact(string bookId, ContactInput payload) returns IdResponse|error
Creates a new contact.
Return Type
- IdResponse|error - The id of the contact that has been created.
getContact
Gets a contact.
updateContact
function updateContact(string bookId, string contactId, ContactInput payload) returns Response|error
Updates an existing contact.
Parameters
- bookId string - The book's id.
- contactId string - The contact's id.
- payload ContactInput - Details of the updated contact.
deleteContact
Deletes an existing contact.
patchContact
function patchContact(string bookId, string contactId, ContactPatch payload) returns Response|error
Updates selected fields of an existing contact.
Parameters
- bookId string - The book's id.
- contactId string - The contact's id.
- payload ContactPatch - Details of the contact to change.
getContactElectronicAddresses
function getContactElectronicAddresses(string bookId, string contactId) returns ElectronicAddresses|error
Gets a contact's electronic addresses.
Return Type
- ElectronicAddresses|error - A list of contact's electronic addresses for the specified book.
createContactElectronicAddress
function createContactElectronicAddress(string bookId, string contactId, ElectronicAddressInput payload) returns TypeResponse|error
Adds a new electronic address to an existing contact.
Parameters
- bookId string - The book's id.
- contactId string - The contact's id.
- payload ElectronicAddressInput - Details of the electronic address to add.
Return Type
- TypeResponse|error - The id and name of the contact electronic address type that has been created.
getContactElectronicAddress
function getContactElectronicAddress(string bookId, string contactId, string 'type) returns ElectronicAddress|error
Gets a contact's electronic address.
Parameters
- bookId string - The book's id.
- contactId string - The contact's id.
- 'type string - The electronic address's type by name or id.
Return Type
- ElectronicAddress|error - A contact's electronic address.
updateContactElectronicAddress
function updateContactElectronicAddress(string bookId, string contactId, string 'type, UpdateElectronicAddressInput payload) returns Response|error
Updates an existing contact electronic address.
Parameters
- bookId string - The book's id.
- contactId string - The contact's id.
- 'type string - The electronic address's type by name or id.
- payload UpdateElectronicAddressInput - The electronic address.
deleteContactElectronicAddress
function deleteContactElectronicAddress(string bookId, string contactId, string 'type) returns Response|error
Deletes an existing contact electronic address.
Parameters
- bookId string - The book's id.
- contactId string - The contact's id.
- 'type string - The electronic address's type by name or id.
patchContactElectronicAddress
function patchContactElectronicAddress(string bookId, string contactId, string 'type, ElectronicAddressPatch payload) returns Response|error
Updates selected fields of an existing contact electronic address.
Parameters
- bookId string - The book's id.
- contactId string - The contact's id.
- 'type string - The electronic address's type by name or id
- payload ElectronicAddressPatch - Details of the contact electronic address to update.
getContactPhoneNumbers
function getContactPhoneNumbers(string bookId, string contactId) returns PhoneNumbers|error
Gets a contact's phone numbers.
Return Type
- PhoneNumbers|error - A list of contact's phone numbers.
createContactPhoneNumber
function createContactPhoneNumber(string bookId, string contactId, PhoneNumberInput payload) returns TypeResponse|error
Adds a new phone number to an existing contact.
Parameters
- bookId string - The book's id.
- contactId string - The contact's id.
- payload PhoneNumberInput - Details of the phone number to add.
Return Type
- TypeResponse|error - The id and name of the contact phone number type that has been created.
getContactPhoneNumber
function getContactPhoneNumber(string bookId, string contactId, string 'type) returns PhoneNumber|error
Gets a contact's phone number.
Parameters
- bookId string - The book's id.
- contactId string - The contact's id.
- 'type string - The phone number's type by name or id.
Return Type
- PhoneNumber|error - The contact's specific phone number.
updateContactPhoneNumber
function updateContactPhoneNumber(string bookId, string contactId, string 'type, UpdatePhoneNumberInput payload) returns Response|error
Updates an existing contact phone number.
Parameters
- bookId string - The book's id.
- contactId string - The contact's id.
- 'type string - The phone number's type by name or id.
- payload UpdatePhoneNumberInput - Details of the phone number to update.
deleteContactPhoneNumber
function deleteContactPhoneNumber(string bookId, string contactId, string 'type) returns Response|error
Delete an existing contact phone number.
Parameters
- bookId string - The book's id.
- contactId string - The contact's id.
- 'type string - The phone number's type by name or id.
patchContactPhoneNumber
function patchContactPhoneNumber(string bookId, string contactId, string 'type, PhoneNumberPatch payload) returns Response|error
Updates selected fields of an existing contact phone number.
Parameters
- bookId string - The book's id.
- contactId string - The contact's id.
- 'type string - The phone number's type by name or id.
- payload PhoneNumberPatch - Details of the contact phone number to update.
getContactAddresses
Gets a contact's addresses.
createContactAddress
function createContactAddress(string bookId, string contactId, AddressInput payload) returns TypeResponse|error
Create a new contact adress.
Parameters
- bookId string - The book's id.
- contactId string - The contact's id.
- payload AddressInput - Details of the new address.
Return Type
- TypeResponse|error - The id of the address that has been created.
getContactAddress
Get a contact's address.
Parameters
- bookId string - The book's id.
- contactId string - The contact's id.
- 'type string - The address's type by name or id.
updateContactAddress
function updateContactAddress(string bookId, string contactId, string 'type, UpdateAddressInput payload) returns Response|error
Updates an existing contact address.
Parameters
- bookId string - The book's id.
- contactId string - The contact's id.
- 'type string - The address's type by name or id.
- payload UpdateAddressInput - The address.
deleteContactAddress
Deletes an existing contact address.
Parameters
- bookId string - The book's id.
- contactId string - The contact's id.
- 'type string - The address's type by name or id.
patchContactAddress
function patchContactAddress(string bookId, string contactId, string 'type, AddressPatch payload) returns Response|error
Updates selected fields of an existing contact address.
Parameters
- bookId string - The book's id.
- contactId string - The contact's id.
- 'type string - The address's type by name or id.
- payload AddressPatch - Details of the contact address to update.
getElectronicAddressTypes
function getElectronicAddressTypes(string bookId) returns ElectronicAddressTypes|error
Gets a list of electronic address types.
Parameters
- bookId string - The book's id.
Return Type
- ElectronicAddressTypes|error - A list of electronic address types for the specified book.
createElectronicAddressType
function createElectronicAddressType(string bookId, ElectronicAddressTypeInput payload) returns IdResponse|error
Creates a new electronic address type.
Parameters
- bookId string - The book's id.
- payload ElectronicAddressTypeInput - Details of the new electronic address type.
Return Type
- IdResponse|error - The id of the electronic address type that has been created.
getElectronicAddressType
function getElectronicAddressType(string bookId, string electronicAddressTypeId) returns ElectronicAddressType|error
Gets an electronic address type.
Parameters
- bookId string - The book's id.
- electronicAddressTypeId string - The electronic address type's id.
Return Type
- ElectronicAddressType|error - The requested electronic address type.
updateElectronicAddressType
function updateElectronicAddressType(string bookId, string electronicAddressTypeId, ElectronicAddressTypeInput payload) returns Response|error
Updates an existing electronic address type.
Parameters
- bookId string - The book's id.
- electronicAddressTypeId string - The electronic address type's id.
- payload ElectronicAddressTypeInput - Details of the updated electronic address type.
deleteElectronicAddressType
function deleteElectronicAddressType(string bookId, string electronicAddressTypeId) returns Response|error
Deletes an existing electronic address type.
Parameters
- bookId string - The book's id.
- electronicAddressTypeId string - The electronic address type's id.
patchElectronicAddressType
function patchElectronicAddressType(string bookId, string electronicAddressTypeId, ElectronicAddressTypePatch payload) returns Response|error
Updates selected fields of an existing electronic address type.
Parameters
- bookId string - The book's id.
- electronicAddressTypeId string - The electronic address type's id.
- payload ElectronicAddressTypePatch - Details of the updated electronic address type.
getLedgerAccounts
function getLedgerAccounts(string bookId, int page, int perPage) returns LedgerAccounts|error
Gets a list of ledger accounts.
Parameters
- bookId string - The book's id.
- page int (default 1) - The page to retrieve.
- perPage int (default 0) - The number of accounts per page.
Return Type
- LedgerAccounts|error - A paged list of ledger accounts for the specified book.
createLedgerAccount
function createLedgerAccount(string bookId, LedgerAccountInput payload) returns IdResponse|error
Creates a new ledger account.
Return Type
- IdResponse|error - The id of the account that has been created.
getLedgerAccount
function getLedgerAccount(string bookId, string accountId) returns LedgerAccount|error
Gets a ledger account.
Return Type
- LedgerAccount|error - The requested ledger account.
updateLedgerAccount
function updateLedgerAccount(string bookId, string ledgerAccountId, LedgerAccountInput payload) returns Response|error
Updates an existing ledger account.
Parameters
- bookId string - The book's id.
- ledgerAccountId string - The ledger account's id.
- payload LedgerAccountInput - Details of the updated ledger account.
deleteLedgerAccount
Deletes an existing ledger account.
patchLedgerAccount
function patchLedgerAccount(string bookId, string ledgerAccountId, LedgerAccountPatch payload) returns Response|error
Updates selected fields of an existing ledger account.
Parameters
- bookId string - The book's id.
- ledgerAccountId string - The ledger account's id.
- payload LedgerAccountPatch - Details of the updated ledger account.
getFinancialInstitutions
function getFinancialInstitutions(string bookId) returns FinancialInstitutions|error
Gets a list of financial institutions.
Parameters
- bookId string - The book's id.
Return Type
- FinancialInstitutions|error - A list of financial institutions.
getEmployees
Gets a list of employees.
Parameters
- bookId string - The book's id.
- page int (default 1) - The page to retrieve.
- perPage int (default 0) - The number of employees per page.
createEmployee
function createEmployee(string bookId, EmployeeInput payload) returns IdResponse|error
Creates a new employee.
Return Type
- IdResponse|error - The id of the employee that has been created.
getEmployee
Gets an employee.
updateEmployee
function updateEmployee(string bookId, string employeeId, EmployeeInput payload) returns Response|error
Updates an existing employee.
Parameters
- bookId string - The book's id.
- employeeId string - The employee's id.
- payload EmployeeInput - Details of the updated employee.
deleteEmployee
Deletes an existing employee.
patchEmployee
function patchEmployee(string bookId, string employeeId, EmployeePatch payload) returns Response|error
Updates selected fields of an existing employee.
Parameters
- bookId string - The book's id.
- employeeId string - The employee's id.
- payload EmployeePatch - Details of the employee to change.
getExpenseClaims
function getExpenseClaims(string bookId, int page, int perPage) returns ExpenseClaims|error
Gets a list of expense claims.
Parameters
- bookId string - The book's id.
- page int (default 1) - The page to retrieve.
- perPage int (default 0) - The number of expense claims per page.
Return Type
- ExpenseClaims|error - A paged list of expense claims for the specified book.
createExpenseClaim
function createExpenseClaim(string bookId, ExpenseClaimInput payload) returns IdResponse|error
Creates a new expense claim.
Parameters
- bookId string - The book's id.
- payload ExpenseClaimInput - Details of the new expense claim.
Return Type
- IdResponse|error - The id of the expense claim that has been created.
getExpenseClaim
function getExpenseClaim(string bookId, string expenseClaimId, string? format) returns ExpenseClaim|error
Gets an expense claim.
Parameters
- bookId string - The book's id.
- expenseClaimId string - The expense claim's id.
- format string? (default ()) - The format to use, e.g. pdf.
Return Type
- ExpenseClaim|error - The requested expense claim.
updateExpensClaim
function updateExpensClaim(string bookId, string expenseClaimId, ExpenseClaimInput payload) returns Response|error
Updates an existing expense claim.
Parameters
- bookId string - The book's id.
- expenseClaimId string - The expense claim's id.
- payload ExpenseClaimInput - Details of the updated expense claim.
deleteExpenseClaim
Deletes an existing expense claim.
patchExpenseClaim
function patchExpenseClaim(string bookId, string expenseClaimId, ExpenseClaimPatch payload) returns Response|error
Updates selected fields of an existing expense claim.
Parameters
- bookId string - The book's id.
- expenseClaimId string - The expense claim's id.
- payload ExpenseClaimPatch - Details to update.
addLineItemToExpenseClaim
function addLineItemToExpenseClaim(string bookId, string expenseClaimId, ExpenseClaimLineItemInput payload) returns IdResponse|error
Creates a new line item within an existing expense claim.
Parameters
- bookId string - The book's id.
- expenseClaimId string - The expense claim's id.
- payload ExpenseClaimLineItemInput - Details of the new line item.
Return Type
- IdResponse|error - The id of the line item that has been created.
updateExpenseClaimLineItem
function updateExpenseClaimLineItem(string bookId, string expenseClaimId, string lineItemId, ExpenseClaimLineItemInput payload) returns Response|error
Updates an existing line item within an existing expense claim.
Parameters
- bookId string - The book's id.
- expenseClaimId string - The expense claim's id.
- lineItemId string - The id of the line item to update.
- payload ExpenseClaimLineItemInput - Details of the updated line item.
deleteExpenseClaimLineItem
function deleteExpenseClaimLineItem(string bookId, string expenseClaimId, string lineItemId) returns Response|error
Deletes an existing line item.
Parameters
- bookId string - The book's id.
- expenseClaimId string - The expense claim's id.
- lineItemId string - The id of the line item to delete.
patchExpenseClaimLineItem
function patchExpenseClaimLineItem(string bookId, string expenseClaimId, string lineItemId, ExpenseClaimLineItemPatch payload) returns Response|error
Updates selected fields of an existing line item within an existing expense claim.
Parameters
- bookId string - The book's id.
- expenseClaimId string - The expense claim's id.
- lineItemId string - The id of the line item to update.
- payload ExpenseClaimLineItemPatch - Details to update.
downloadAttachment
function downloadAttachment(string bookId, string expenseClaimId, string lineItemId, string attachmentId) returns Response|error
Downloads a document that is attached to an expense claim line item.
Parameters
- bookId string - The book's id.
- expenseClaimId string - The expense claim's id.
- lineItemId string - The line item's id.
- attachmentId string - The id of the attachment to download.
Return Type
deleteAttachment
function deleteAttachment(string bookId, string expenseClaimId, string lineItemId, string attachmentId) returns Response|error
Deletes an attachment.
Parameters
- bookId string - The book's id.
- expenseClaimId string - The expense claim's id.
- lineItemId string - The id of the line item to delete the document from.
- attachmentId string - The attachment's id.
addAttachment
function addAttachment(string bookId, string expenseClaimId, string lineItemId, string? fileName) returns AddAttachmentResponses|error
Attaches one or more documents to an expense claim line item.
Parameters
- bookId string - The book's id.
- expenseClaimId string - The expense claim's id.
- lineItemId string - The id of the line item to attach the document(s) to.
- fileName string? (default ()) - The name of the file when passing a single document in the body of the request.
Return Type
- AddAttachmentResponses|error - A list of document attachment responses.
getHeartbeat
function getHeartbeat() returns HeartbeatStatus|error
Reckon One API heartbeat.
Return Type
- HeartbeatStatus|error - The status of the API.
getClassifications
function getClassifications(string bookId, int page, int perPage) returns Classifications|error
Gets a list of classifications.
Parameters
- bookId string - The book's id.
- page int (default 1) - The page to retrieve.
- perPage int (default 0) - The number of classifications per page.
Return Type
- Classifications|error - A paged list of classifications for the specified book.
createClassification
function createClassification(string bookId, ClassificationInput payload) returns IdResponse|error
Creates a new classification.
Parameters
- bookId string - The book's id.
- payload ClassificationInput - Details of the new classification.
Return Type
- IdResponse|error - The id of the classification that has been created.
getClassification
function getClassification(string bookId, string classificationId) returns Classification|error
Gets a classification.
Return Type
- Classification|error - The requested classification.
updateClassification
function updateClassification(string bookId, string classificationId, ClassificationInput payload) returns Response|error
Updates an existing classification.
Parameters
- bookId string - The book's id.
- classificationId string - The classification's id.
- payload ClassificationInput - Details of the updated classification.
deleteClassification
Deletes an existing classification.
patchClassification
function patchClassification(string bookId, string classificationId, ClassificationPatch payload) returns Response|error
Updates selected fields of an existing classification.
Parameters
- bookId string - The book's id.
- classificationId string - The classification's id.
- payload ClassificationPatch - Details to update.
getPaymentTerms
function getPaymentTerms(string bookId, int page, int perPage) returns PaymentTerms|error
Gets a list of payment terms.
Parameters
- bookId string - The book's id.
- page int (default 1) - The page to retrieve.
- perPage int (default 0) - The number of payment term per page.
Return Type
- PaymentTerms|error - A paged list of payment terms for the specified book.
createPaymentTerm
function createPaymentTerm(string bookId, PaymentTermInput payload) returns IdResponse|error
Creates a new payment term.
Return Type
- IdResponse|error - The id of the payment term that has been created.
getPaymentTerm
function getPaymentTerm(string bookId, string termId) returns PaymentTerm|error
Gets a payment term.
Return Type
- PaymentTerm|error - The requested payment term.
updatePaymentTerm
function updatePaymentTerm(string bookId, string termId, PaymentTermInput payload) returns Response|error
Updates an existing payment term.
Parameters
- bookId string - The book's id.
- termId string - The term's id.
- payload PaymentTermInput - Details of the updated payment term.
deletePaymentTerm
Deletes an existing payment term.
patchPaymentTerm
function patchPaymentTerm(string bookId, string termId, PaymentTermPatch payload) returns Response|error
Updates an existing payment term.
Parameters
- bookId string - The book's id.
- termId string - The term's id.
- payload PaymentTermPatch - Details of the updated payment term.
getPaymentTermDueDate
function getPaymentTermDueDate(string bookId, string termId, string baseDate) returns PaymentTermDueDate|error
Gets a calculated due date from a specified base date.
Return Type
- PaymentTermDueDate|error - A calculated due date.
getInvoices
Gets a list of invoices.
Parameters
- bookId string - The book's id.
- page int (default 1) - The page to retrieve.
- perPage int (default 0) - The number of invoices per page.
createInvoice
function createInvoice(string bookId, InvoiceInput payload) returns IdResponse|error
Creates a new invoice.
Return Type
- IdResponse|error - The id of the invoice that has been created.
getInvoice
Gets an invoice.
Parameters
- bookId string - The book's id.
- invoiceId string - The invoice's id.
- format string? (default ()) - The format to use, e.g. pdf.
updateInvoice
function updateInvoice(string bookId, string invoiceId, InvoiceInput payload) returns Response|error
Updates an existing invoice.
Parameters
- bookId string - The book's id.
- invoiceId string - The invoice's id.
- payload InvoiceInput - Details of the updated invoice.
deleteInvoice
Deletes an existing invoice.
patchInvoice
function patchInvoice(string bookId, string invoiceId, InvoicePatch payload) returns Response|error
Updates selected fields of an existing invoice.
Parameters
- bookId string - The book's id.
- invoiceId string - The invoice's id.
- payload InvoicePatch - Details to update.
getInvoiceSummary
function getInvoiceSummary(string bookId, string? baseDate) returns InvoicesSummary|error
Gets a summary of a book's invoices.
Parameters
- bookId string - The book's id.
- baseDate string? (default ()) - The base date to use when checking for overdue invoices.
Return Type
- InvoicesSummary|error - A summary of a book's invoices.
getCustomerInvoiceSummary
function getCustomerInvoiceSummary(string bookId, string customerId, string? baseDate) returns InvoicesSummary|error
Gets a summary of a book's invoices for a given customer.
Parameters
- bookId string - The book's id.
- customerId string - The customer id to filter on.
- baseDate string? (default ()) - The base date to use when checking for overdue invoices.
Return Type
- InvoicesSummary|error - A summary of a book's invoices for a given customer.
convertInvoiceFrom
function convertInvoiceFrom(string bookId, InvoiceConvertFromInput payload) returns IdResponse|error
Creates a new invoice from an estimate.
Parameters
- bookId string - The book's id.
- payload InvoiceConvertFromInput - Details to convert into an invoice.
Return Type
- IdResponse|error - The id of the invoice that has been created.
unlinkInvoiceTransaction
function unlinkInvoiceTransaction(string bookId, string invoiceId, string linkId) returns Response|error
Unlinks an existing transaction from an invoice.
Parameters
- bookId string - The book's id.
- invoiceId string - The invoice's id.
- linkId string - The link's id.
getPopulatedEmail
Gets an email template populated with details from the specified invoice.
emailInvoice
function emailInvoice(string bookId, string invoiceId, EmailInput payload) returns Response|error
Emails an existing invoice.
Parameters
- bookId string - The book's id.
- invoiceId string - The invoice's id.
- payload EmailInput - Details of an email to send.
markInvoiceEmailAsSent
Marks an existing invoice as sent.
markInvoiceEmailAsUnsent
Marks an existing invoice as unsent.
getInvoiceLineItem
function getInvoiceLineItem(string bookId, string invoiceId, string lineItemId) returns InvoiceLineItem|error
Gets a line item from an invoice.
Parameters
- bookId string - The book's id.
- invoiceId string - The invoice's id.
- lineItemId string - The line item's id.
Return Type
- InvoiceLineItem|error - The line item.
updateInvoiceLineItem
function updateInvoiceLineItem(string bookId, string invoiceId, string lineItemId, InvoiceLineItemInput payload) returns Response|error
Updates an existing line item within an existing invoice.
Parameters
- bookId string - The book's id.
- invoiceId string - The invoice's id.
- lineItemId string - The line item's id.
- payload InvoiceLineItemInput - Details of the line item.
addLineItemToInvoice
function addLineItemToInvoice(string bookId, string invoiceId, InvoiceLineItemInput payload) returns IdResponse|error
Creates a new line item within an existing invoice.
Parameters
- bookId string - The book's id.
- invoiceId string - The invoice's id.
- payload InvoiceLineItemInput - Details of the new line item.
Return Type
- IdResponse|error - The id of the line item that has been created.
deleteInvoiceLineItem
function deleteInvoiceLineItem(string bookId, string invoiceId, string lineItemId) returns Response|error
Deletes an existing line item.
Parameters
- bookId string - The book's id.
- invoiceId string - The invoice's id.
- lineItemId string - The id of the line item to delete.
patchInvoiceLineItem
function patchInvoiceLineItem(string bookId, string invoiceId, string lineItemId, InvoiceLineItemPatch payload) returns Response|error
Updates selected fields of an existing line item within an existing invoice.
Parameters
- bookId string - The book's id.
- invoiceId string - The invoice's id.
- lineItemId string - The id of the line item to update.
- payload InvoiceLineItemPatch - Details to update.
getRecurringInvoiceTemplate
function getRecurringInvoiceTemplate(string bookId, string templateId) returns RecurringInvoiceTemplate|error
Gets a recurring invoice template.
Return Type
- RecurringInvoiceTemplate|error - The requested recurring invoice template.
getProjects
Gets a list of projects.
Parameters
- bookId string - The book's id.
- page int (default 1) - The page to retrieve.
- perPage int (default 0) - The number of projects per page.
createProject
function createProject(string bookId, ProjectInput payload) returns IdResponse|error
Creates a new project.
Return Type
- IdResponse|error - The id of the project that has been created.
getProject
Gets a project.
updateProject
function updateProject(string bookId, string projectId, ProjectInput payload) returns Response|error
Updates an existing project.
Parameters
- bookId string - The book's id.
- projectId string - The project's id.
- payload ProjectInput - Details of the updated project.
deleteProject
Deletes an existing project.
patchProject
function patchProject(string bookId, string projectId, ProjectPatch payload) returns Response|error
Updates selected fields of an existing project.
Parameters
- bookId string - The book's id.
- projectId string - The project's id.
- payload ProjectPatch - The project's details being altered.
deleteProjectItem
Deletes an existing project item.
Parameters
- bookId string - The book's id.
- projectId string - The project's id.
- lineId string - The id of the project item to delete.
patchProjectItem
function patchProjectItem(string bookId, string projectId, string lineId, ProjectItemPatch payload) returns Response|error
Updates selected fields of an existing project item within an existing project.
Parameters
- bookId string - The book's id.
- projectId string - The project's id.
- lineId string - The project item's id.
- payload ProjectItemPatch - The project item's details being altered.
deleteProjectCustomer
function deleteProjectCustomer(string bookId, string projectId, string lineId) returns Response|error
Deletes an existing project customer.
Parameters
- bookId string - The book's id.
- projectId string - The project's id.
- lineId string - The id of the project customer to delete.
patchProjectCustomer
function patchProjectCustomer(string bookId, string projectId, string lineId, ProjectCustomerPatch payload) returns Response|error
Updates selected fields of an existing project customer within an existing project.
Parameters
- bookId string - The book's id.
- projectId string - The project's id.
- lineId string - The project customer's id.
- payload ProjectCustomerPatch - The project customer's details being altered.
deleteProjectSupplier
function deleteProjectSupplier(string bookId, string projectId, string lineId) returns Response|error
Deletes an existing project supplier.
Parameters
- bookId string - The book's id.
- projectId string - The project's id.
- lineId string - The id of the project supplier to delete.
patchProjectSupplier
function patchProjectSupplier(string bookId, string projectId, string lineId, ProjectSupplierPatch payload) returns Response|error
Updates selected fields of an existing project supplier within an existing project.
Parameters
- bookId string - The book's id.
- projectId string - The project's id.
- lineId string - The project suppliers's id.
- payload ProjectSupplierPatch - The project suppliers's details being altered.
getSettings
function getSettings(string bookId) returns BookSettings|error
Get a book's general settings.
Parameters
- bookId string - The book's id.
Return Type
- BookSettings|error - The general settings for the specified book.
patchSettings
function patchSettings(string bookId, BookSettingsPatch payload) returns Response|error
Updates selected fields of a book's settings.
Parameters
- bookId string - The book's id.
- payload BookSettingsPatch - The book settings being altered.
patchSettingsAddress
function patchSettingsAddress(string bookId, string 'type, BookAddressPatch payload) returns Response|error
Updates selected fields of an address in a book's settings.
Parameters
- bookId string - The book's id.
- 'type string - The type of address. This can either be
legal
,physical
or the address type id.
- payload BookAddressPatch - The address being altered.
patchSettingsContactPhoneNumber
function patchSettingsContactPhoneNumber(string bookId, string 'type, BookPhoneNumberPatch payload) returns Response|error
Updates selected fields of a contact phone number in a book's settings.
Parameters
- bookId string - The book's id.
- 'type string - The type of phone number. This can either be
phone
,mobile
,fax
or the phone number type id.
- payload BookPhoneNumberPatch - The phone number being altered.
patchSettingsContactElectronicAddress
function patchSettingsContactElectronicAddress(string bookId, string 'type, BookElectronicAddressPatch payload) returns Response|error
Updates selected fields of a contact electronic address in a book's settings.
Parameters
- bookId string - The book's id.
- 'type string - The type of electronic address. This can either be
email
orweb
.
- payload BookElectronicAddressPatch - The electronic address being altered.
getTaxSettings
function getTaxSettings(string bookId) returns TaxSettings|error
Get a book's tax settings.
Parameters
- bookId string - The book's id.
Return Type
- TaxSettings|error - The tax settings for the specified book.
patchTaxSettings
function patchTaxSettings(string bookId, TaxSettingsPatch payload) returns Response|error
Updates selected fields of a book's tax settings.
Parameters
- bookId string - The book's id.
- payload TaxSettingsPatch - The book's tax settings being updated.
getInvoiceSettings
function getInvoiceSettings(string bookId) returns InvoiceSettings|error
Gets a book's invoice settings.
Parameters
- bookId string - The book's id.
Return Type
- InvoiceSettings|error - The invoice settings for the specified book.
patchInvoiceSettings
function patchInvoiceSettings(string bookId, InvoiceSettingsPatch payload) returns Response|error
Updates selected fields of a book's invoice settings.
Parameters
- bookId string - The book's id.
- payload InvoiceSettingsPatch - The book's invoice settings to update.
getCreditNoteSettings
function getCreditNoteSettings(string bookId) returns CreditNoteSettings|error
Gets a book's credit note settings.
Parameters
- bookId string - The book's id.
Return Type
- CreditNoteSettings|error - The credit note settings for the specified book.
patchCreditNoteSettings
function patchCreditNoteSettings(string bookId, CreditNoteSettingsPatch payload) returns Response|error
Updates selected fields of a book's credit note settings.
Parameters
- bookId string - The book's id.
- payload CreditNoteSettingsPatch - The book's credit note settings to update.
getReceiptSettings
function getReceiptSettings(string bookId) returns ReceiptSettings|error
Gets a book's receipt settings.
Parameters
- bookId string - The book's id.
Return Type
- ReceiptSettings|error - The receipt settings for the specified book.
patchReceiptSettings
function patchReceiptSettings(string bookId, ReceiptSettingsPatch payload) returns Response|error
Updates selected fields of a book's receipt settings.
Parameters
- bookId string - The book's id.
- payload ReceiptSettingsPatch - The book's receipt settings to update.
getPayrollSettings
function getPayrollSettings(string bookId) returns PayrollSettings|error
Get a book's payroll settings.
Parameters
- bookId string - The book's id.
Return Type
- PayrollSettings|error - The payroll settings for the specified book.
patchPayrollSettings
function patchPayrollSettings(string bookId, PayrollSettingsPatch payload) returns Response|error
Updates selected fields of a book's payroll settings.
Parameters
- bookId string - The book's id.
- payload PayrollSettingsPatch - The book's payroll settings being altered.
patchPayrollSettingsContactPhoneNumber
function patchPayrollSettingsContactPhoneNumber(string bookId, string 'type, BookPhoneNumberPatch payload) returns Response|error
Updates selected fields of a legal contact's phone number in a book's payroll settings.
Parameters
- bookId string - The book's id.
- 'type string - The type of phone number. This can either be
phone
or the phone number type id.
- payload BookPhoneNumberPatch - The phone number being altered.
patchPayrollSettingsElectronicAddress
function patchPayrollSettingsElectronicAddress(string bookId, string 'type, BookElectronicAddressPatch payload) returns Response|error
Updates selected fields of a legal contact's electronic address in a book's payroll settings.
Parameters
- bookId string - The book's id.
- 'type string - The type of electronic address. This can either be
email
or the email type id.
- payload BookElectronicAddressPatch - The electronic address being altered.
getTimeEntrySettings
function getTimeEntrySettings(string bookId) returns TimeEntrySettings|error
Get a book's time entry settings.
Parameters
- bookId string - The book's id.
Return Type
- TimeEntrySettings|error - The time entry settings for the specified book.
patchTimeEntrySettings
function patchTimeEntrySettings(string bookId, TimeEntrySettingsPatch payload) returns Response|error
Updates selected fields of a book's time entry settings.
Parameters
- bookId string - The book's id.
- payload TimeEntrySettingsPatch - The book's time entry settings being altered.
getTemplates
function getTemplates(string bookId, int page, int perPage) returns TemplateTypeRef|error
Gets a list of templates for the specified book.
Parameters
- bookId string - The book's id.
- page int (default 1) - The page to retrieve.
- perPage int (default 0) - The number of super funds per page.
Return Type
- TemplateTypeRef|error - A list of templates for the specified book.
getTemplateLogo
function getTemplateLogo(string bookId, string templateId) returns FileContentResult|error
Gets a template's logo for the specified book.
Return Type
- FileContentResult|error - The template.
getInvoiceTemplates
function getInvoiceTemplates(string bookId, int page, int perPage) returns InvoiceTemplates|error
Gets a list of invoice templates for the specified book.
Parameters
- bookId string - The book's id.
- page int (default 1) - The page to retrieve.
- perPage int (default 0) - The number of super funds per page.
Return Type
- InvoiceTemplates|error - A list of invoices templates for the specified book.
getInvoiceTemplate
function getInvoiceTemplate(string bookId, string templateId) returns InvoiceTemplate|error
Gets an invoice template for the specified book.
Return Type
- InvoiceTemplate|error - The template.
getEstimateTemplates
function getEstimateTemplates(string bookId, int page, int perPage) returns EstimateTemplates|error
Gets a list of estimate templates for the specified book.
Parameters
- bookId string - The book's id.
- page int (default 1) - The page to retrieve.
- perPage int (default 0) - The number of super funds per page.
Return Type
- EstimateTemplates|error - A list of estimates templates for the specified book.
getEstimateTemplate
function getEstimateTemplate(string bookId, string templateId) returns EstimateTemplate|error
Gets an estimate template for the specified book.
Return Type
- EstimateTemplate|error - The template.
getCustomerAdjustmentNotesTemplates
function getCustomerAdjustmentNotesTemplates(string bookId, int page, int perPage) returns Templates|error
Gets a list of customer adjustment note templates for the specified book.
Parameters
- bookId string - The book's id.
- page int (default 1) - The page to retrieve.
- perPage int (default 0) - The number of super funds per page.
getCustomerAdjustmentNotesTemplate
function getCustomerAdjustmentNotesTemplate(string bookId, string templateId) returns Template|error
Gets a customer adjustment note template for the specified book.
getBillTemplates
Gets a list of bill templates for the specified book.
Parameters
- bookId string - The book's id.
- page int (default 1) - The page to retrieve.
- perPage int (default 0) - The number of super funds per page.
getBillTemplate
Gets a bill template for the specified book.
getSupplierAdjustmentNotesTemplates
function getSupplierAdjustmentNotesTemplates(string bookId, int page, int perPage) returns Templates|error
Gets a list of supplier adjustment note templates for the specified book.
Parameters
- bookId string - The book's id.
- page int (default 1) - The page to retrieve.
- perPage int (default 0) - The number of super funds per page.
getSupplierAdjustmentNotesTemplate
function getSupplierAdjustmentNotesTemplate(string bookId, string templateId) returns Template|error
Gets a supplier adjustment note template for the specified book.
getExpenseclaimsTemplates
Gets a list of expense claim templates for the specified book.
Parameters
- bookId string - The book's id.
- page int (default 1) - The page to retrieve.
- perPage int (default 0) - The number of super funds per page.
getExpenseclaimssTemplate
Gets an expense claim template for the specified book.
getCustomerStatementsTemplates
function getCustomerStatementsTemplates(string bookId, int page, int perPage) returns CustomerStatementsTemplates|error
Gets a list of customer statement templates for the specified book.
Parameters
- bookId string - The book's id.
- page int (default 1) - The page to retrieve.
- perPage int (default 0) - The number of super funds per page.
Return Type
- CustomerStatementsTemplates|error - A list of customer statements templates for the specified book.
getCustomerStatementsTemplate
function getCustomerStatementsTemplate(string bookId, string templateId) returns CustomerStatementsTemplate|error
Gets a customer statement template for the specified book.
Return Type
- CustomerStatementsTemplate|error - The template.
getTaxGroups
function getTaxGroups(string bookId, string effectiveDate, int page, int perPage) returns TaxGroups|error
Gets a paged list of tax groups for the specified book.
Parameters
- bookId string - The book's id.
- effectiveDate string - The effective date for the tax rates.
- page int (default 1) - The page to retrieve.
- perPage int (default 0) - The number of groups per page.
getTaxGroup
function getTaxGroup(string bookId, string taxGroupId, string effectiveDate) returns TaxGroups|error
Gets a tax group with tax rates as at the supplied effective date.
Parameters
- bookId string - The book's id.
- taxGroupId string - The tax group's id.
- effectiveDate string - The effective date for the tax rates.
getTaxRates
function getTaxRates(string bookId, string effectiveDate, int page, int perPage) returns TaxRates|error
Gets tax rates as at the supplied effective date.
Parameters
- bookId string - The book's id.
- effectiveDate string - The effective date for the tax rates.
- page int (default 1) - The page to retrieve.
- perPage int (default 0) - The number of rates per page.
getTaxRate
Gets a tax rate as at the supplied effective date.
Parameters
- bookId string - The book's id.
- taxRateId string - The tax rate's id.
- effectiveDate string - The effective date for the tax rate.
getItems
Gets a list of items.
Parameters
- bookId string - The book's id.
- page int (default 1) - The page to retrieve.
- perPage int (default 0) - The number of items per page.
createItem
function createItem(string bookId, ItemInput payload) returns IdResponse|error
Creates a new item.
Return Type
- IdResponse|error - The id of the item that has been created.
getItem
Gets an item.
updateItem
Updates an existing item.
Parameters
- bookId string - The book's id.
- itemId string - The item's id.
- payload ItemInput - Details of the updated item.
deleteItem
Deletes an existing item.
patchItem
Updates selected fields of an existing item.
Parameters
- bookId string - The book's id.
- itemId string - The item's id.
- payload ItemPatch - Details to update.
getTimeEntries
function getTimeEntries(string bookId, int page, int perPage) returns TimeEntries|error
Gets a list of time entries.
Parameters
- bookId string - The book's id.
- page int (default 1) - The page to retrieve.
- perPage int (default 0) - The number of time entries per page.
Return Type
- TimeEntries|error - A paged list of time entries for the specified book.
getTimeEntry
Gets a time entry.
updateTimeEntry
function updateTimeEntry(string bookId, string timeEntryId, TimeEntryInput payload) returns Response|error
Updates an existing time entry.
Parameters
- bookId string - The book's id.
- timeEntryId string - The time entry's id.
- payload TimeEntryInput - Details of the updated time entry.
deleteTimeEntry
Deletes an existing time entry.
createTimeEntry
function createTimeEntry(string bookId, TimeEntryInput payload) returns IdResponse|error
Creates a new time entry.
Return Type
- IdResponse|error - The id of the time entry that has been created.
patchTimeEntry
function patchTimeEntry(string bookId, string timeEntryId, TimeEntryPatch payload) returns Response|error
Updates selected fields of a time entry.
Parameters
- bookId string - The book's id.
- timeEntryId string - The time entry's id.
- payload TimeEntryPatch - Details to update.
getUsers
Gets a list of users.
Records
reckon.one: AddAttachmentResponse
A response that contains the outcome of a document attachment request.
Fields
- fileName string? - The name of the document.
- code int - The HTTP status code for this particular document.
- 'error string? - The error message if a document could not be attached.
- id string? - The attached document's id.
- url string? - The URL to retrieve the document.
reckon.one: AddAttachmentResponses
A list of document attachment responses.
Fields
- list AddAttachmentResponse[]? - The list of responses for each document in the request.
reckon.one: Address
An address.
Fields
- id string? - The address's unique id.
- 'type AddressTypeRef? - The address type.
- line1 string? - Line 1 of the address.
- line2 string? - Line 2 of the address.
- line3 string? - Line 3 of the address.
- suburb string? - The suburb.
- town string? - The town.
- state string? - The state.
- postcode string? - The post code.
- country string? - The country.
reckon.one: Addresses
A list of electronic addresses.
Fields
- list Address[]? - The list of items.
reckon.one: AddressInput
Details of an address to create or update.
Fields
- line1 string? - Line 1 of the address.
- line2 string? - Line 2 of the address.
- line3 string? - Line 3 of the address.
- suburb string? - The suburb.
- town string? - The town.
- state string? - The state.
- postcode string? - The post code.
- country string? - The country.
- 'type string - The id or name of the address type.
reckon.one: AddressPatch
Details of an address to update.
Fields
- line1 string? - Line 1 of the address.
- line2 string? - Line 2 of the address.
- line3 string? - Line 3 of the address.
- suburb string? - The suburb.
- town string? - The town.
- state string? - The state.
- postcode string? - The post code.
- country string? - The country.
reckon.one: AddressType
Details of an address type.
Fields
- id string - The type's id.
- name string? - The type's name.
- description string? - The type's description.
- status TypeStatus - Type status.
- createdDateTime string - The creation UTC date time.
- lastModifiedDateTime string - The last modified UTC date time.
reckon.one: AddressTypeInput
Details of an address type to create or update.
Fields
- name string - The type's name.
- description string? - The type's description.
- status TypeStatus? - Type status.
reckon.one: AddressTypePatch
Details of an address type to create or update.
Fields
- name string? - The type's name.
- description string? - The type's description.
- status TypeStatus? - Type status.
reckon.one: AddressTypeRef
Reference to an address type.
Fields
- id string? - The unique id.
- name string? - The unique name.
reckon.one: AddressTypes
Address types.
Fields
- list AddressType[]? - The list of items.
reckon.one: BankAccount
Details of a bank account.
Fields
- dateOpened string? - When the account was opened.
- lockoffDate string? - The date when transactions are locked. Transactions dated on or before this date cannot be amended.
- bankBranchNumber string? - The branch number (BSB or Sort Code).
- bankAccountNumber string? - The account number.
- openingBalance decimal? - The opening balance, if there is one.
- isCash boolean - Whether the account is cash.
- financialInstitution FinancialInstitutionRef? - Details of the financial institution.
- includeBalancingTransaction boolean - Whether to include a balancing transaction.
- payerIdentifier string? - The APCA number to identify the payer.
reckon.one: BasReportSettings
BAS reporting preference details.
Fields
- reportingPeriodType string? - The BAS reporting period.
- simplerBAS boolean - Indicates whether its a simpler BAS. Simpler BAS is only applicable for business reporting GST on Monthly, Annual or quarterly basis (option 1: Calculate GST and report quarterly).
- amountTaxStatus SettingsAmountTaxStatus - The sales figures type of tax.
- fbt boolean - Indicates whether Fringe Benefits Tax (FBT) applies to the business.
- ftc boolean - Indicates whether Fuel Tax Credits (FTC) applies to the business.
- lct boolean - Indicates whether Luxury Car Tax (LCT) applies to the business.
- paygiPayQuarterly boolean - Indicates whether Pay As You Go Income Tax Instalment (PAYG I) applies to the business. Pay PAYG instalment quarterly.
- paygiCalculateUsingIncomeTimesRate boolean - Indicates whether Pay As You Go Income Tax Instalment (PAYG I) applies to the business. Calculate PAYG instalment using income times rate.
- paygw boolean - Indicates whether Pay As You Go Tax Withheld (PAYG W) applies to the business.
- wet boolean - Indicates whether Wine Equalisation Tax (WET) applies to the business.
reckon.one: BasReportSettingsPatch
BAS report settings to patch.
Fields
- reportingPeriodType string? - The BAS reporting period.
- simplerBAS boolean? - Indicates whether its a simpler BAS. Simpler BAS is only applicable for business reporting GST on Monthly, Annual or quarterly basis (option 1: Calculate GST and report quarterly).
- amountTaxStatus SettingsAmountTaxStatus? - The sales figures type of tax.
- fbt boolean? - Indicates whether Fringe Benefits Tax (FBT) applies to the business.
- ftc boolean? - Indicates whether Fuel Tax Credits (FTC) applies to the business.
- lct boolean? - Indicates whether Luxury Car Tax (LCT) applies to the business.
- paygiPayQuarterly boolean? - Indicates whether Pay As You Go Income Tax Instalment (PAYG I) applies to the business. Pay PAYG instalment quarterly.
- paygiCalculateUsingIncomeTimesRate boolean? - Indicates whether Pay As You Go Income Tax Instalment (PAYG I) applies to the business. Calculate PAYG instalment using income times rate.
- paygw boolean? - Indicates whether Pay As You Go Tax Withheld (PAYG W) applies to the business.
- wet boolean? - Indicates whether Wine Equalisation Tax (WET) applies to the business.
reckon.one: Book
Details of a book.
Fields
- id string - The book's id.
- name string? - The book's name.
- status BookStatus - A book's status.
- ownership BookOwnership - Book ownership.
- country string? - The book's country code.
- bookCreated boolean - Whether book creation has been completed.
- products BookProduct[]? - The products that are currently licensed.
reckon.one: BookAddress
An address.
Fields
- id string? - The address's unique id.
- 'type BookTypeRef? - The address type.
- line1 string? - First line of address.
- line2 string? - Second line of address.
- line3 string? - Third line of address.
- town string? - Town of address.
- suburb string? - Suburb of address.
- state string? - State of address.
- postcode string? - Postcode of address.
- country string? - Country of address.
reckon.one: BookAddressPatch
An address to patch.
Fields
- line1 string? - First line of address.
- line2 string? - Second line of address.
- line3 string? - Third line of address.
- town string? - Town of address.
- suburb string? - Suburb of address.
- state string? - State of address.
- postcode string? - Postcode of address.
- country string? - Country of address.
reckon.one: BookElectronicAddress
An electronic address.
Fields
- id string? - The electronic address's unique id.
- 'type BookTypeRef? - Type of electronic address.
- address string? - The electronic address.
reckon.one: BookElectronicAddressPatch
An electronic address.
Fields
- address string? - The electronic address.
reckon.one: BookPhoneNumber
A phone number.
Fields
- id string? - The phone number's unique id.
- 'type BookTypeRef? - The type of phone number.
- areaCode string? - The area code.
- number string? - The number.
reckon.one: BookPhoneNumberPatch
A phone number.
Fields
- areaCode string? - The area code.
- number string? - The number.
reckon.one: BookProduct
Details of a book product.
Fields
- id string - The products's id.
- name string? - The product's name.
- portalProductId string? - The Portal's product id.
reckon.one: Books
Books.
Fields
- list Book[]? - The list of items.
reckon.one: BookSettings
A book's general settings
Fields
- bookName string? - The book's name.
- startDate string - The first day of records for the book.
- bookCreatedDateTime string - Date and time when the book was created.
- lockOffDate string? - Transactions on or before this date cannot be changed.
- financialYearStartDay int - Day of month the financial year starts on.
- financialYearStartMonth int - Month as a number that the financial year starts in.
- entityType string? - Entity type of business.
- industryType IndustryTypeDetail? - Industry details of business.
- replyToEmailAddress string? - The email address used by clients when replying to emails sent from Reckon One.
- showEmailSentFrom string? - The displayed name of sender in emails.
- bankDataRetrievalDays int - The number of most recent days bank data is retrieved for.
- generalDetails GeneralDetail? - General company details
- addresses BookAddress[]? - List of addresses.
- contactDetails ContactDetail? - Business's contact details.
reckon.one: BookSettingsPatch
Details of a book's general settings to patch.
Fields
- startDate string? - The first day of records for the book.
- lockOffDate string? - Transactions on or before this date cannot be changed.
- entityType string? - Entity type of business.
- industryType IndustryTypeDetailPatch? - Industry details of business.
- replyToEmailAddress string? - The email address used by clients when replying to emails sent from Reckon One.
- showEmailSentFrom string? - The displayed name of sender in emails.
- bankDataRetrievalDays int? - The number of most recent days bank data is retrieved for.
- generalDetails GeneralDetailPatch? - General company details
- contactDetails ContactDetailPatch? - Business's contact details.
reckon.one: BookTypeRef
Type reference.
Fields
- id string? - The unique id.
- name string? - The unique name.
reckon.one: Classification
A classification
Fields
- id string - The classification's unique id.
- name string? - The classification's name.
- description string? - The classification's description.
- status ClassificationStatus - Classification status
- isDefault boolean - Whether the classification is the default for the transaction.
reckon.one: ClassificationInput
Details of a classification to create or update.
Fields
- name string - The classification's name.
- description string? - The classification's description.
- status ClassificationStatus? - Classification status
- isDefault boolean(default false) - Whether the classification is the default for the transaction.
reckon.one: ClassificationPatch
Details of the classification to patch.
Fields
- name string? - The classification's name.
- description string? - The classification's description.
- status ClassificationStatus? - Classification status
- isDefault boolean? - Whether the classification is the default for the transaction.
reckon.one: ClassificationRef
Reference to a classification.
Fields
- id string? - The unique id.
- name string? - The unique name.
reckon.one: Classifications
A list of classifications.
Fields
- list Classification[]? - The list of items.
reckon.one: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
reckon.one: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
reckon.one: Contact
A contact.
Fields
- id string - The contact's unique id.
- name string? - The contact's display name.
- salutation string? - The salutation to use in communications.
- department string? - The contact's department.
- position string? - The contact's position.
- organisation OrganisationRef? - The organisation that the contact is associated with.
- status ContactStatus - The status of a contact.
- notes string? - Notes for the contact.
- addresses Address[]? - List of addresses.
- phoneNumbers PhoneNumber[]? - List of phone numbers.
- electronicAddresses ElectronicAddress[]? - List of electronic addresses.
reckon.one: ContactDetail
Details on how to contact the business.
Fields
- contactName string? - Name of person who is primary contact within the business.
- phoneNumbers BookPhoneNumber[]? - List of phone numbers.
- electronicAddresses BookElectronicAddress[]? - List of electronic addresses.
reckon.one: ContactDetailPatch
Details on how to contact the business to be patched.
Fields
- contactName string? - Name of person who is primary contact within the business.
reckon.one: ContactInput
Details of a contact to create or update.
Fields
- name string - The contact's display name.
- salutation string? - The salutation to use in communications.
- department string? - The contact's department.
- position string? - The contact's position.
- status ContactStatus? - The status of a contact.
- notes string? - Notes for the contact.
- addresses AddressInput[]? - List of addresses.
- phoneNumbers PhoneNumberInput[]? - List of phone numbers.
- electronicAddresses ElectronicAddressInput[]? - List of electronic addresses.
- organisation string? - The id or name of the organisation that the contact is associated with.
reckon.one: ContactPatch
Details of a contact to patch.
Fields
- name string? - The contact's display name.
- salutation string? - The salutation to use in communications.
- department string? - The contact's department.
- position string? - The contact's position.
- organisation string? - The id or name of the organisation that the contact is associated with.
- status ContactStatus? - The status of a contact.
- notes string? - Notes for the contact.
reckon.one: ContactRef
Reference to a contact.
Fields
- id string? - The unique id.
- name string? - The unique name.
reckon.one: Contacts
A list of contacts.
Fields
- list Contact[]? - The list of items.
reckon.one: CreditAccount
Details of a credit account.
Fields
- dateOpened string? - When the account was opened.
- lockoffDate string? - The date when transactions are locked. Transactions dated on or before this date cannot be amended.
- openingBalance decimal? - The opening balance, if there is one.
reckon.one: CreditNote
A credit note.
Fields
- id string - The credit note's unique id.
- creditNoteNumber string? - The credit note's number.
- customer ContactRef? - The customer that the credit note was issued to.
- billingAddress Address? - The address that the credit note was sent to.
- shippingAddress Address? - The address that the credit note was sent to.
- creditNoteDate string - The date of the credit note.
- totalAmount decimal - The total amount of the credit note.
- balance decimal - The balance that has not yet been paid by the customer.
- amountTaxStatus AmountTaxStatus - The tax status of an amount.
- totalTaxAmount decimal? - The total tax amount of the credit note.
- status CreditNoteStatus - The status of a credit note.
- reference string? - The credit note reference.
- accountsReceivableLedgerAccount LedgerAccountRef? - The accounts receivable ledger account.
- classification ClassificationRef? - The classification.
- template TemplateRef? - The template used to print or email the credit note.
- transactionLinks TransactionLink[]? - Details of transactions (e.g. Invoices) that are linked to the credit note.
- lineItems CreditNoteLineItem[]? - The individual items that make up the credit note.
- notes string? - Notes related to the credit note.
- emailStatus EmailStatus - The status of an emailed invoice.
reckon.one: CreditNoteInput
Details of a credit note to create or update.
Fields
- customer string - The customer that the credit note will be issued to.
- creditNoteDate string - The date of the credit note.
- amountTaxStatus AmountTaxStatus - The tax status of an amount.
- reference string? - The credit note reference.
- accountsReceivableLedgerAccount string(default "The default Accounts Receivable account") - The accounts receivable ledger account. Note: It is not currently possible to create multiple Accounts Receivable accounts so this field is read-only and will be ignored.
- classification string? - The classification.
- template string(default "The default credit note template") - The template used to print or email the credit note.
- lineItems CreditNoteLineItemInput[]? - The individual items that make up the credit note.
- notes string? - Notes related to the credit note.
reckon.one: CreditNoteLineItem
A credit note line item.
Fields
- lineNumber int - The line number.
- lineId string - The line item's unique id.
- project ProjectRef? - The id and full name of the project that the line item relates to.
- itemDetails CreditNoteLineItemItemDetails? - Details of an item used on a credit note line item.
- accountDetails CreditNoteLineItemAccountDetails? - Details of an account used on a credit note line item.
- description string? - The description of the item.
- taxRate TaxRateRef? - The tax rate.
- taxAmount decimal? - The tax amount.
- taxIsModified boolean? - Whether the tax amount has been modified.
reckon.one: CreditNoteLineItemAccountDetails
Details of an account used on a credit note line item.
Fields
- ledgerAccount LedgerAccountRef? - The id and full name of the ledger account that the line relates to.
- quantity decimal? - The quantity sold.
- amount decimal? - The amount.
reckon.one: CreditNoteLineItemAccountDetailsInput
Details of an account used on a credit note line item.
Fields
- ledgerAccount string - The id or full name of the ledger account that the line relates to. Note that the full name includes the name(s) of any parent account(s) separated by colons.
- quantity decimal? - The quantity purchased.
- amount decimal? - The amount including or excluding tax (depending on the
amountTaxStatus
).
reckon.one: CreditNoteLineItemAccountDetailsPatch
Details of an account used on a credit note line item.
Fields
- ledgerAccount string? - The id or full name of the ledger account that the line relates to. Note that the full name includes the name(s) of any parent account(s) separated by colons.
- quantity decimal? - The quantity purchased.
- amount decimal? - The amount including or excluding tax (depending on the
amountTaxStatus
).
reckon.one: CreditNoteLineItemInput
Details of a credit note line item to create or update.
Fields
- lineNumber int - The line number.
- project string? - The id or full name of the project that the line item relates to. Note that the full name includes the name(s) of any parent project(s) separated by colons.
- itemDetails CreditNoteLineItemItemDetailsInput? - Details of an item used on an invoice line item.
This must be omitted if
accountDetails
have been provided.
- accountDetails CreditNoteLineItemAccountDetailsInput? - Details of an account used on an invoice line item.
This must be omitted if
itemDetails
have been provided.
- description string? - The description of the line.
- taxAmount decimal? - The tax amount.
- taxRate string? - The tax rate.
- taxIsModified boolean(default false) - Whether the tax amount has been modified.
reckon.one: CreditNoteLineItemItemDetails
Details of an item used on an credit note line item.
Fields
- item ItemRef? - The id and full name of the item that the line relates to.
- price decimal? - The price of each item.
- quantity decimal? - The quantity sold.
reckon.one: CreditNoteLineItemItemDetailsInput
Details of an item used on a credit note line item.
Fields
- item string - The id or full name of the item that the line relates to. Note that the full name includes the name(s) of any parent item(s) separated by colons.
- price decimal? - The price of each item including or excluding tax (depending on the
amountTaxStatus
).
- quantity decimal? - The quantity purchased.
reckon.one: CreditNoteLineItemItemDetailsPatch
Details of an item used on a credit note line item.
Fields
- item string? - The id or full name of the item that the line relates to. Note that the full name includes the name(s) of any parent item(s) separated by colons.
- price decimal? - The price of each item including or excluding tax (depending on the
amountTaxStatus
).
- quantity decimal? - The quantity purchased.
reckon.one: CreditNoteLineItemPatch
Details of a credit note line item to create or update.
Fields
- lineNumber int? - The line number.
- project string? - The id or full name of the project that the line item relates to. Note that the full name includes the name(s) of any parent project(s) separated by colons.
- itemDetails CreditNoteLineItemItemDetailsPatch? - Details of an item used on an invoice line item.
This must be omitted if
accountDetails
have been provided.
- accountDetails CreditNoteLineItemAccountDetailsPatch? - Details of an account used on an invoice line item.
This must be omitted if
itemDetails
have been provided.
- description string? - The description of the line.
- taxAmount decimal? - The tax amount.
- taxRate string? - The tax rate.
- taxIsModified boolean? - Whether the tax amount has been modified.
reckon.one: CreditNotePatch
Details of a credit note to patch.
Fields
- customer string? - The customer that the credit note will be issued to.
- creditNoteDate string? - The date of the credit note.
- status CreditNoteStatus? - The status of a credit note.
- reference string? - The credit note reference.
- accountsReceivableLedgerAccount string? - The accounts receivable ledger account. Note: It is not currently possible to create multiple Accounts Receivable accounts so this field is read-only and will be ignored.
- classification string? - The classification.
- template string? - The template used to print or email the credit note.
- notes string? - Notes related to the credit note.
reckon.one: CreditNotes
A list of credit notes.
Fields
- list CreditNote[]? - The list of items.
reckon.one: CreditNoteSettings
A book's credit note settings.
Fields
- prefix string? - The invoice prefix.
- defaultTemplate TemplateRef? - The default invoice template.
- emailSettings EmailTemplate? - Email settings.
reckon.one: CreditNoteSettingsPatch
Credit note settings to patch.
Fields
- prefix string? - The credit note prefix.
- defaultTemplate string? - The default credit note template.
- emailSettings EmailTemplatePatch? - Email settings.
reckon.one: CreditNoteTransactionLinkInput
Details of a transaction to link to a credit note.
Fields
- transactionId string - The unique id of the linked transaction.
- transactionAmount decimal - The transaction's amount.
- transactionType LinkedTransactionType - Type of transaction linked to an invoice.
reckon.one: CreditNoteTransactionLinkPatch
Selected fields to update in a credit note's transaction link.
Fields
- transactionAmount decimal - The transaction's amount.
reckon.one: Customer
A customer
Fields
- id string - The organisation's unique id.
- name string? - The organisation's display name.
- organisationName string? - The organisation's name.
- branch string? - The organisation's branch.
- notes string? - The organisation's notes.
- businessNumber1 string? - The organisation's business number 1.
- businessNumber2 string? - The organisation's business number 2.
- entityTypes EntityType? - The organisation's entity types.
- status string? - The organisation's status (Active or Inactive).
- electronicAddresses ElectronicAddress[]? - List of electronic addresses.
- phoneNumbers PhoneNumber[]? - List of phone numbers.
- addresses Address[]? - List of addresses.
- contacts ContactRef[]? - The organisation's contacts list.
- balance decimal? - The organisation's current balance.
- lastModifiedDateTime string - The organisation's last modified date.
- creditLimit decimal? - The customer's credit limit (null for other types).
- paymentTerms PaymentTermRef? - The customer's peyment term (null for other types).
- invoiceCount int? - The total number of invoices that have been issued to the organisation.
reckon.one: CustomerInput
Details of a customer to create or update.
Fields
- name string - The organisation's display name.
- organisationName string? - The organisation's name.
- branch string? - The organisation's branch.
- notes string? - Notes for the organisation.
- businessNumber1 string? - The organisation's business number 1.
- businessNumber2 string? - The organisation's business number 2.
- status string? - The status of the organisation. (Active/Inactive)
- addresses AddressInput[]? - List of addresses.
- phoneNumbers PhoneNumberInput[]? - List of phone numbers.
- electronicAddresses ElectronicAddressInput[]? - List of electronic addresses.
- entityTypes EntityType? - The organisation's entity types.
- creditLimit decimal? - The customer's credit limit.
- paymentTerms string? - The customer's payment term id.
reckon.one: CustomerPatch
Details of a customer to patch.
Fields
- name string? - The organisation's display name.
- organisationName string? - The organisation's name.
- branch string? - The organisation's branch.
- notes string? - Notes for the organisation.
- businessNumber1 string? - The organisation's business number 1.
- businessNumber2 string? - The organisation's business number 2.
- status string? - The status of the organisation. (Active/Inactive)
- entityTypes EntityTypePatch? - If the entity has other entity types.
- creditLimit decimal? - The customer's credit limit.
- paymentTerms string? - The customer's peyment term id.
reckon.one: Customers
A list of customers.
Fields
- list Customer[]? - The list of items.
reckon.one: CustomerStatementsTemplate
Details of a template.
Fields
- id string - The template's id.
- name string? - The template's name.
- header TemplateHeaderBase? - The header detail of a template.
reckon.one: CustomerStatementsTemplates
A list of templates.
Fields
- list CustomerStatementsTemplate[]? - The list of items.
reckon.one: Department
Details of a department.
Fields
- id string - The department's unique id.
- name string? - The department's unique name.
- code string? - The department's unique code.
- status DepartmentStatus - The status of a department.
- notes string? - Notes about the department.
- managers EmployeeRef[]? - A list of managers assigned to the department.
- employees EmployeeRef[]? - A list of employees assigned to the department.
- createdDateTime string - The date and time when the department was created.
- lastModifiedDateTime string - The date and time when the department was last modified.
reckon.one: DepartmentEmployeeInput
Employee belonging to department.
Fields
- id string - An employee's Id.
reckon.one: DepartmentInput
The department being created or updated.
Fields
- name string - The department's unique name.
- code string? - The department's unique code.
- status DepartmentStatus - The status of a department.
- notes string? - Notes about the department.
- managers DepartmentManagerInput[]? - A list of managers assigned to the department.
- employees DepartmentEmployeeInput[]? - A list of employees assigned to the department.
reckon.one: DepartmentManagerInput
Manager belonging to department.
Fields
- id string - A manager's Id.
reckon.one: DepartmentPatch
ThDetails of an department to patch.
Fields
- name string? - The department's unique name.
- code string? - The department's unique code.
- status DepartmentStatus? - The status of a department.
- notes string? - Notes about the department.
reckon.one: Departments
A list of departments.
Fields
- list Department[]? - The list of items.
reckon.one: DocumentAttachment
A document attachment.
Fields
- id string - The attachment's unique id.
- name string? - The attachment's name or file name.
- url string? - The URL to use to retrieve the attachment.
reckon.one: ElectronicAddress
An electronic address.
Fields
- id string? - The electronic address's unique id.
- 'type ElectronicAddressTypeRef? - Type of electronic address.
- address string? - The electronic address.
reckon.one: ElectronicAddresses
A list of electronic addresses.
Fields
- list ElectronicAddress[]? - The list of items.
reckon.one: ElectronicAddressInput
Details of an electronic address to create or update.
Fields
- 'type string - The id or name of the electronic address type.
- address string? - The electronic address.
reckon.one: ElectronicAddressPatch
Details of a electronic address to update.
Fields
- address string? - The electronic address.
reckon.one: ElectronicAddressType
Details of an electronic address type.
Fields
- id string - The type's id.
- name string? - The type's name.
- description string? - The type's description.
- status TypeStatus - Type status.
- createdDateTime string - The creation UTC date time.
- lastModifiedDateTime string - The last modified UTC date time.
reckon.one: ElectronicAddressTypeInput
Details of an electronic address type to create or update.
Fields
- name string - The type's name.
- description string? - The type's description.
- status TypeStatus? - Type status.
reckon.one: ElectronicAddressTypePatch
Details of an electronic address type to create or update.
Fields
- name string? - The type's name.
- description string? - The type's description.
- status TypeStatus? - Type status.
reckon.one: ElectronicAddressTypeRef
Reference to an electronic address type.
Fields
- id string? - The unique id.
- name string? - The unique name.
reckon.one: ElectronicAddressTypes
Electronic address types.
Fields
- list ElectronicAddressType[]? - The list of items.
reckon.one: Email
Details of an email.
Fields
- toAddresses string[]? - A list of "To" addresses.
- ccAddresses string[]? - A list of "CC" addresses.
- bccAddresses string[]? - A list of "BCC" addresses.
- subject string? - The email subject.
- body string? - The email body.
- documentNumber string? - The number of the document that the email relates to.
reckon.one: EmailInput
Details of an email to send.
Fields
- toAddresses string[]? - A list of "To" addresses.
- ccAddresses string[]? - A list of "CC" addresses.
- bccAddresses string[]? - A list of "BCC" addresses.
- subject string - The email subject.
- body string - The email body.
- documentNumber string? - The number of the document that the email relates to.
reckon.one: EmailSettings
The book's tax email settings.
Fields
- bas EmailTemplate? - BAS default email settings.
reckon.one: EmailSettingsPatch
The book's tax email settings to patch.
Fields
- bas EmailTemplatePatch? - BAS default email settings.
reckon.one: EmailTemplate
The email template.
Fields
- includeCc boolean - Indicates whether includes CC by default when emailing.
- ccAddress string? - Default CC email address.
- includeBcc boolean - Indicates whether includes BCC by default when emailing.
- bccAddress string? - Default BCC email address.
- emailSubject string? - Default email subject.
- emailContent string? - Default email content.
reckon.one: EmailTemplatePatch
The email template to patch.
Fields
- includeCc boolean? - Indicates whether includes CC by default when emailing.
- ccAddress string? - Default CC email address.
- includeBcc boolean? - Indicates whether includes BCC by default when emailing.
- bccAddress string? - Default BCC email address.
- emailSubject string? - Default email subject.
- emailContent string? - Default email content.
reckon.one: Employee
Details of an employee.
Fields
- id string - The employee's unique id.
- name string? - The employee's display name.
- firstName string? - The employee's first name.
- surname string? - The employee's last name.
- notes string? - Additional notes about the employee.
- businessNumber1 string? - The employee's business number 1.
- businessNumber2 string? - The employee's business number 2.
- addresses Address[]? - The employee's addresses.
- status ContactStatus - The status of a contact.
- electronicAddresses ElectronicAddress[]? - List of electronic addresses.
- phoneNumbers PhoneNumber[]? - List of phone numbers
- otherEntityTypes EntityType[]? - A list of other entity types this employee is assigned to.
- lastModifiedDateTime string - The UTC date and time when the employee's details were last modified.
reckon.one: EmployeeInput
Details of an employee.
Fields
- name string - The employee's display name.
- firstName string - The employee's first name.
- surname string - The employee's last name.
- notes string? - Additional notes about the employee.
- businessNumber1 string? - The employee's business number 1.
- businessNumber2 string? - The employee's business number 2.
- status ContactStatus? - The status of a contact.
- addresses AddressInput[]? - List of addresses.
- phoneNumbers PhoneNumberInput[]? - List of phone numbers.
- electronicAddresses ElectronicAddressInput[]? - List of electronic addresses.
reckon.one: EmployeePatch
Details of an employee.
Fields
- name string? - The employee's display name.
- firstName string? - The employee's first name.
- surname string? - The employee's last name.
- notes string? - Additional notes about the employee.
- businessNumber1 string? - The employee's business number 1.
- businessNumber2 string? - The employee's business number 2.
- status ContactStatus? - The status of a contact.
reckon.one: EmployeeRef
Reference to an employee.
Fields
- id string? - The unique id.
- name string? - The unique name.
reckon.one: Employees
A list of employees.
Fields
- list Employee[]? - The list of items.
reckon.one: EntityType
Contact's entity types.
Fields
- isCustomer boolean? - Is the entity a customer.
- isSupplier boolean? - Is the entity a suppleir.
- isEmployee boolean? - Is the entity an employee.
- isSuperfund boolean? - Is the entity a superfund.
reckon.one: EntityTypePatch
Contact's entity types.
Fields
- isCustomer boolean? - Is the entity a customer.
- isSupplier boolean? - Is the entity a supplier.
- isEmployee boolean? - Is the entity an employee.
- isSuperfund boolean? - Is the entity a superfund.
reckon.one: ErrorDetail
Details of a specific error.
Fields
- location string? - The location/path of the source of the error.
- message string? - The description of what was wrong at this location.
reckon.one: ErrorResponse
Response sent when an error occurs.
Fields
- message string? - The description of the error.
- code int - The HTTP status code of the error.
- errors ErrorDetail[]? - An array of specific errors where relevant.
reckon.one: ErrorResponseForbidden
Response sent when a 403 Forbidden error occurs.
Fields
- message string? - The description of the error.
- code int - The HTTP status code of the error.
reckon.one: ErrorResponseNotFound
Response sent when a 404 Forbidden error occurs.
Fields
- message string? - The description of the error.
- code int - The HTTP status code of the error.
reckon.one: ErrorResponseUnauthorized
Response sent when a 401 Unauthorized error occurs.
Fields
- message string? - The description of the error.
- code int - The HTTP status code of the error.
reckon.one: ErrorResponseUnsupportedMediaType
Response sent when a 415 Unsupported media type error occurs.
Fields
- message string? - The description of the error.
- code int - The HTTP status code of the error.
reckon.one: EstimateTemplate
Details of an estimate template.
Fields
- id string - The template's id.
- name string? - The template's name.
- font string? - The template's font.
- fontSize float - The template's font size.
- header EstimateTemplateHeader? - The header details of the template.
- content EstimateTemplateContent? - The content's details of the template.
- footer EstimateTemplateFooter? - The footer details of the template.
reckon.one: EstimateTemplateContent
Details of the content of a template.
Fields
- project boolean - Is Project included in the template's content.
- item boolean - Is Item included in the template's content.
- itemPrice boolean - Is ItemPrice included in the template's content.
- description boolean - Is Description included in the template's content.
- quantity boolean - Is Quantity included in the template's content.
- taxCode boolean - Is TaxCode included in the template's content.
- tax boolean - Is Tax included in the template's content.
- amount boolean - Is Amount included in the template's content.
reckon.one: EstimateTemplateFooter
Details of the footer of an estimate template.
Fields
- note boolean - Is Note included in the template's footer.
- subtotal boolean - Is Subtotal included in the template's footer.
- taxAmount boolean - Is TaxAmount included in the template's footer.
- totalExcludingTax boolean - Is total excluding tax included in the template's footer.
- total boolean - Is total included in the template's footer.
- termsAndConditions boolean - Is terms and conditions included in the template's footer.
- paymentNotes boolean - Is payment notes included in the template's footer.
- signature boolean - Is signature included in the template's footer.
reckon.one: EstimateTemplateHeader
Details of the header of an estimate template.
Fields
- companyName boolean - Is CompanyName included in the template's header.
- companyAddress boolean - Is CompanyAddress included in the template's header.
- taxNumber boolean - Is TaxNumber included in the template's header.
- phoneNumber boolean - Is PhoneNumber included in the template's header.
- email boolean - Is Email included in the template's header.
- website boolean - Is Website included in the template's header.
- logo Logo? - Logo of the template's header.
- billingAddress boolean - Is billing address included in the template's header.
- shippingAddress boolean - Is shipping address included in the template's header.
- estimateDate boolean - Is estimate date included in the template's header.
- estimateNumber boolean - Is estimate number included in the template's header.
- expiryDate boolean - Is expiry date included in the template's header.
- referenceCode boolean - Is reference code included in the template's header.
- customText boolean - Is custom text included in the template's header.
- customTextValue string? - The custom text value of the template's header.
reckon.one: EstimateTemplates
A list of estimate templates.
Fields
- list EstimateTemplate[]? - The list of items.
reckon.one: ExpenseClaim
An expense claim.
Fields
- id string - The expense claim's unique id.
- claimNumber string? - The expense claim's number.
- employee ContactRef? - The employee who lodged the expense claim.
- claimDate string - The date of the expense claim.
- reference string? - The reference entered by the employee.
- project ProjectRef? - The project that the expense claim relates to.
- customer ContactRef? - The customer that the expense claim relates to.
- totalAmount decimal - The total amount of the expense claim.
- balance decimal - The balance that has not yet been reimbursed to the employee.
- amountTaxStatus AmountTaxStatus - The tax status of an amount.
- totalTaxAmount decimal? - The total tax amount of the expense claim.
- status ExpenseClaimStatus - The status of an expense claim.
- transactionLinks TransactionLink[]? - Details of transactions (e.g. payments) that are linked to the expense claim.
- lineItems ExpenseClaimLineItem[]? - The individual items that make up the expense claim.
- notes string? - The notes entered by the employee.
- declineReason string? - When an expense claim has been declined, this field will provide the reason.
reckon.one: ExpenseClaimInput
Details of an expense claim to create or update.
Fields
- employee string - The employee who lodged the expense claim.
- claimDate string - The date of the expense claim.
- reference string? - The reference entered by the employee.
- project string? - The project that the expense claim relates to.
- customer string? - The customer that the expense claim relates to.
- amountTaxStatus AmountTaxStatus - The tax status of an amount.
- status ExpenseClaimStatus? - The status of an expense claim.
- lineItems ExpenseClaimLineItemInput[]? - The individual items that make up the expense claim.
- notes string? - The notes entered by the employee.
reckon.one: ExpenseClaimLineItem
An expense claim line item.
Fields
- lineNumber int - The line number.
- lineId string - The line item's unique id.
- date string - The date of the line item.
- project ProjectRef? - The id and full name of the project that the line item relates to.
- itemDetails ExpenseClaimLineItemItemDetails? - Details of an item used on an expense claim line item.
- accountDetails ExpenseClaimLineItemAccountDetails? - Details of an account used on an expense claim line item.
- supplier ContactRef? - The supplier that the line item relates to.
- customer ContactRef? - The customer that the line item relates to.
- isBillable boolean? - Whether the line item is billable to the customer.
- billableStatus BillableStatus - The billable status.
- description string? - The description of the expense.
- taxAmount decimal? - The tax amount.
- taxRate TaxRateRef? - The tax rate.
- taxIsModified boolean? - Whether the tax amount has been modified.
- notes string? - Notes relating to the expense.
- attachments DocumentAttachment[]? - Document attachments related to the expense.
reckon.one: ExpenseClaimLineItemAccountDetails
Details of an account used on an expense claim line item.
Fields
- ledgerAccount LedgerAccountRef? - The id and full name of the ledger account that the expense claim line relates to.
- quantity decimal? - The quantity purchased.
- amount decimal? - The amount.
reckon.one: ExpenseClaimLineItemAccountDetailsInput
Details of an account used on an expense claim line item.
Fields
- ledgerAccount string - The id or full name of the ledger account that the expense claim line relates to. Note that the full name includes the name(s) of any parent account(s) separated by colons.
- quantity decimal? - The quantity purchased.
- amount decimal? - The amount including or excluding tax (depending on the expense claim's
amountTaxStatus
).
reckon.one: ExpenseClaimLineItemAccountDetailsPatch
Details of an account used on an expense claim line item.
Fields
- ledgerAccount string? - The id or full name of the ledger account that the expense claim line relates to. Note that the full name includes the name(s) of any parent account(s) separated by colons.
- quantity decimal? - The quantity purchased.
- amount decimal? - The amount including or excluding tax (depending on the expense claim's
amountTaxStatus
).
reckon.one: ExpenseClaimLineItemInput
Details of an expense claim line item to create or update.
Fields
- lineNumber int - The line number.
- date string - The date of the line item.
- project string(default "The project from the expense claim header") - The id or full name of the project that the line item relates to. Note that the full name includes the name(s) of any parent project(s) separated by colons.
- supplier string? - The supplier that the line item relates to.
- customer string(default "The customer from the expense claim header") - The customer that the line item relates to.
- isBillable boolean(default false) - Whether the line item is billable to the customer.
- billableStatus BillableStatus? - The billable status.
- itemDetails ExpenseClaimLineItemItemDetailsInput? - Details of an item used on an expense claim line item.
This must be omitted if
accountDetails
have been provided.
- accountDetails ExpenseClaimLineItemAccountDetailsInput? - Details of an account used on an expense claim line item.
This must be omitted if
itemDetails
have been provided.
- description string? - The description of the expense.
- taxAmount decimal? - The tax amount.
- taxRate string? - The tax rate.
- taxIsModified boolean(default false) - Whether the tax amount has been modified.
- notes string? - Notes relating to the expense.
reckon.one: ExpenseClaimLineItemItemDetails
Details of an item used on an expense claim line item.
Fields
- item ItemRef? - The id and full name of the item that the expense claim line relates to.
- price decimal? - The price of each item.
- quantity decimal? - The quantity purchased.
reckon.one: ExpenseClaimLineItemItemDetailsInput
Details of an item used on an expense claim line item.
Fields
- item string - The id or full name of the item that the expense claim line relates to. Note that the full name includes the name(s) of any parent item(s) separated by colons.
- price decimal? - The price of each item.
- quantity decimal? - The quantity sold.
reckon.one: ExpenseClaimLineItemItemDetailsPatch
Details of an item used on an expense claim line item.
Fields
- item string? - The id or full name of the item that the expense claim line relates to. Note that the full name includes the name(s) of any parent item(s) separated by colons.
- price decimal? - The price of each item.
- quantity decimal? - The quantity sold.
reckon.one: ExpenseClaimLineItemPatch
Details of an expense claim line item to patch.
Fields
- lineNumber int? - The line number.
- date string? - The date of the line item.
- project string? - The id or full name of the project that the line item relates to. Note that the full name includes the name(s) of any parent project(s) separated by colons.
- supplier string? - The supplier that the line item relates to.
- customer string? - The customer that the line item relates to.
- isBillable boolean? - Whether the line item is billable to the customer.
- billableStatus BillableStatus? - The billable status.
- itemDetails ExpenseClaimLineItemItemDetailsPatch? - Details of an item used on an expense claim line item.
This must be omitted if
accountDetails
have been provided.
- accountDetails ExpenseClaimLineItemAccountDetailsPatch? - Details of an account used on an expense claim line item.
This must be omitted if
itemDetails
have been provided.
- description string? - The description of the expense.
- taxAmount decimal? - The tax amount.
- taxRate string? - The tax rate.
- taxIsModified boolean? - Whether the tax amount has been modified.
- notes string? - Notes relating to the expense.
reckon.one: ExpenseClaimPatch
Details of an expense claim to patch.
Fields
- employee string? - The employee who lodged the expense claim.
- claimDate string? - The date of the expense claim.
- reference string? - The reference entered by the employee.
- project string? - The project that the expense claim relates to.
- customer string? - The customer that the expense claim relates to.
- status ExpenseClaimStatus? - The status of an expense claim.
- declineReason string? - The decline reason when the status is set to Declined. This cannot be used with any other status.
- notes string? - The notes entered by the employee.
reckon.one: ExpenseClaims
A list of expense claims.
Fields
- list ExpenseClaim[]? - The list of items.
reckon.one: FileContentResult
A file content result.
reckon.one: FinancialInstitution
Details of a financial institution.
Fields
- id string - The financial institution's unique id.
- code string? - The financial institution's unique code.
- name string? - The financial institution's name.
reckon.one: FinancialInstitutionRef
Reference to a financial institution.
Fields
- id string? - The unique id.
- name string? - The unique name.
reckon.one: FinancialInstitutions
A list of financial institutions.
Fields
- list FinancialInstitution[]? - The list of financial institutions.
reckon.one: FundDetails
Class for the fund details of the superfund provider
Fields
- id string? - The super fund provider's unique id.
- name string? - The name of the superfund
reckon.one: GeneralDetail
Basic business details
Fields
- companyName string? - The company's business/trading name.
- legalName string? - The name of the company that appears on legal documents.
- taxNumber string? - The tax number of the company.
- branchNumber string? - The branch number associated with the tax number.
reckon.one: GeneralDetailPatch
Basic business details to patch.
Fields
- companyName string? - The company's business/trading name.
- legalName string? - The name of the company that appears on legal documents.
- taxNumber string? - The tax number of the company.
- branchNumber string? - The branch number associated with the tax number.
reckon.one: HeartbeatStatus
The current API status.
Fields
- status string? - The status of the API.
- 'version string? - The current API version.
- description string? - The description of the API.
reckon.one: IdNameResponse
A response that contains the resource's id and display name.
Fields
- id string - The resource's id.
- name string? - The resource's display name.
reckon.one: IdResponse
A response that contains the resource's id.
Fields
- id string - The resource's id.
reckon.one: IndustryTypeDetail
Business's industry details.
Fields
- industry string? - Type of industry business belongs to.
- category string? - Category of industry.
- businessType string? - Type of business activity within specified industry.
reckon.one: IndustryTypeDetailPatch
Business's industry details to patch.
Fields
- industry string? - Type of industry business belongs to.
- category string? - Category of industry.
- businessType string? - Type of business activity within specified industry.
reckon.one: Invoice
An invoice
Fields
- id string - The invoice's unique id.
- invoiceNumber string? - The invoice's number.
- customer ContactRef? - The customer that was invoiced.
- billingAddress Address? - The address that the invoice was sent to.
- shippingAddress Address? - The address that the invoice was sent to.
- invoiceDate string - The date of the invoice.
- dueDate string? - The date payment is due by.
- totalAmount decimal - The total amount of the invoice.
- invoiceDiscountAmount decimal? - The invoice discount amount.
- invoiceDiscountPercent decimal? - The invoice discount percentage.
- balance decimal - The balance that has not yet been paid by the customer.
- amountTaxStatus AmountTaxStatus - The tax status of an amount.
- totalTaxAmount decimal? - The total tax amount of the invoice.
- status InvoiceStatus - The status of an invoice.
- paymentTerms PaymentTermRef? - Payment terms.
- reference string? - The invoice reference.
- accountsReceivableLedgerAccount LedgerAccountRef? - The accounts receivable ledger account.
- classification ClassificationRef? - The classification.
- template TemplateRef? - The template used to print or email the invoice.
- recurringTemplate RecurringTemplateRef? - Details of the recurring template.
- transactionLinks TransactionLink[]? - Details of transactions (e.g. Estimates) that are linked to the invoice.
- lineItems InvoiceLineItem[]? - The individual items that make up the invoice.
- notes string? - Notes related to the invoice.
- paymentDetails string? - Details of how to pay the invoice.
- emailStatus EmailStatus - The status of an emailed invoice.
reckon.one: InvoiceConvertFromInput
Details of an invoice to create from an estimate, sales order and etc.
Fields
- id string - The Id of the object that needs to be converted into an invoice.
- 'type InvoiceConvertFromType - Types of an invoice to create from, which can be an estimate, sales order and etc.
reckon.one: InvoiceInput
Details of an invoice to create or update.
Fields
- customer string - The customer that is being invoiced.
- invoiceDate string - The date of the invoice.
- dueDate string? - The date payment is due by.
- invoiceDiscountAmount decimal? - The invoice discount amount.
- invoiceDiscountPercent decimal? - The invoice discount percentage.
- amountTaxStatus AmountTaxStatus - The tax status of an amount.
- paymentTerms string(default "The customer's default payment terms") - Payment terms.
- reference string? - The invoice reference.
- accountsReceivableLedgerAccount string(default "The default Accounts Receivable account") - The accounts receivable ledger account. Note: It is not currently possible to create multiple Accounts Receivable accounts so this field is read-only and will be ignored.
- classification string? - The classification.
- template string(default "The default invoice template") - The template used to print or email the invoice.
- lineItems InvoiceLineItemInput[]? - The individual items that make up the invoice.
- notes string? - Notes related to the invoice.
- paymentDetails string? - Details of how to pay the invoice.
reckon.one: InvoiceLineItem
An invoice line item.
Fields
- lineNumber int - The line number.
- lineId string - The line item's unique id.
- parentLineId string? - The parent line item's unique id.
- serviceDate string? - The date when the service was provided.
- project ProjectRef? - The id and full name of the project that the line item relates to.
- itemDetails InvoiceLineItemItemDetails? - Details of an item used on an invoice line item.
- accountDetails InvoiceLineItemAccountDetails? - Details of an account used on an invoice line item.
- description string? - The description of the item.
- taxRate TaxRateRef? - The tax rate.
- taxAmount decimal? - The tax amount.
- taxIsModified boolean? - Whether the tax amount has been modified.
- transactionLinks TransactionLink[]? - Details of transactions (e.g. expenses and timesheets) that are linked to the invoice's line items.
reckon.one: InvoiceLineItemAccountDetails
Details of an account used on an invoice line item.
Fields
- ledgerAccount LedgerAccountRef? - The id and full name of the ledger account that the line relates to.
- quantity decimal? - The quantity sold.
- amount decimal? - The amount.
reckon.one: InvoiceLineItemAccountDetailsInput
Details of an account used on an invoice line item.
Fields
- ledgerAccount string - The id or full name of the ledger account that the line relates to. Note that the full name includes the name(s) of any parent account(s) separated by colons.
- quantity decimal? - The quantity purchased.
- amount decimal? - The amount including or excluding tax (depending on the
amountTaxStatus
).
reckon.one: InvoiceLineItemAccountDetailsPatch
Details of an account used on an invoice line item.
Fields
- ledgerAccount string? - The id or full name of the ledger account that the invoice line relates to. Note that the full name includes the name(s) of any parent account(s) separated by colons.
- quantity decimal? - The quantity purchased.
- amount decimal? - The amount including or excluding tax (depending on the invoice's
amountTaxStatus
).
reckon.one: InvoiceLineItemInput
Details of an invoice line item to create or update.
Fields
- lineNumber int - The line number.
- serviceDate string? - The date when the service was provided.
- project string? - The id or full name of the project that the line item relates to. Note that the full name includes the name(s) of any parent project(s) separated by colons.
- itemDetails InvoiceLineItemItemDetailsInput? - Details of an item used on an invoice line item.
This must be omitted if
accountDetails
have been provided.
- accountDetails InvoiceLineItemAccountDetailsInput? - Details of an account used on an invoice line item.
This must be omitted if
itemDetails
have been provided.
- description string? - The description of the line.
- taxAmount decimal? - The tax amount.
- taxRate string? - The tax rate.
- taxIsModified boolean(default false) - Whether the tax amount has been modified.
reckon.one: InvoiceLineItemItemDetails
Details of an item used on an invoice line item.
Fields
- item ItemRef? - The id and full name of the item that the line relates to.
- price decimal? - The price of each item.
- quantity decimal? - The quantity sold.
- discountAmount decimal? - The total discount for the line item.
- discountPercent decimal? - The discount percentage for the line item.
reckon.one: InvoiceLineItemItemDetailsInput
Details of an item used on an invoice line item.
Fields
- item string - The id or full name of the item that the line relates to. Note that the full name includes the name(s) of any parent item(s) separated by colons.
- price decimal? - The price of each item including or excluding tax (depending on the
amountTaxStatus
).
- quantity decimal? - The quantity purchased.
- discountAmount decimal? - The total discount for the line item.
- discountPercent decimal? - The discount percentage for the line item.
reckon.one: InvoiceLineItemItemDetailsPatch
Details of an item used on an invoice line item.
Fields
- item string? - The id or full name of the item that the invoice line relates to. Note that the full name includes the name(s) of any parent item(s) separated by colons.
- price decimal? - The price of each item including or excluding tax (depending on the invoice's
amountTaxStatus
).
- quantity decimal? - The quantity purchased.
- discountAmount decimal? - The total discount for the line item.
- discountPercent decimal? - The discount percentage for the line item.
reckon.one: InvoiceLineItemPatch
Details of an invoice to patch.
Fields
- lineNumber int? - The line number.
- serviceDate string? - The date when the service was provided.
- project string? - The id or full name of the project that the line item relates to. Note that the full name includes the name(s) of any parent project(s) separated by colons.
- itemDetails InvoiceLineItemItemDetailsPatch? - Details of an item used on an invoice line item.
This must be omitted if
accountDetails
have been provided.
- accountDetails InvoiceLineItemAccountDetailsPatch? - Details of an account used on an invoice line item.
This must be omitted if
itemDetails
have been provided.
- description string? - The description of the line.
- taxAmount decimal? - The tax amount.
- taxRate string? - The tax rate.
- taxIsModified boolean? - Whether the tax amount has been modified.
reckon.one: InvoicePatch
Details of an invoice to patch.
Fields
- customer string? - The customer that is being invoiced.
- invoiceDate string? - The date of the invoice.
- dueDate string? - The date payment is due by.
- invoiceDiscountAmount decimal? - The invoice discount amount.
- invoiceDiscountPercent decimal? - The invoice discount percentage.
- status InvoiceStatus? - The status of an invoice.
- paymentTerms string? - Payment terms.
- reference string? - The invoice reference.
- accountsReceivableLedgerAccount string? - The accounts receivable ledger account. Note: It is not currently possible to create multiple Accounts Receivable accounts so this field is read-only and will be ignored.
- classification string? - The classification.
- template string? - The template used to print or email the invoice.
- notes string? - Notes related to the invoice.
- paymentDetails string? - Details of how to pay the invoice.
reckon.one: Invoices
A list of invoices.
Fields
- list Invoice[]? - The list of items.
reckon.one: InvoiceSettings
A book's invoice settings.
Fields
- approvalEnabled boolean - Indicates whether invoice approval is enabled.
- prefix string? - The invoice prefix.
- defaultTemplate TemplateRef? - The default invoice template.
- nextInvoiceNumber int - The number that will be used for the next invoice.
- defaultPaymentDetails string? - The default payment details.
- serviceDateOnInvoice boolean - Indicates whether service date can be used on invoices.
- emailSettings EmailTemplate? - Email settings.
reckon.one: InvoiceSettingsPatch
Invoice settings to patch.
Fields
- approvalEnabled boolean - Indicates whether invoice approval is enabled.
- prefix string? - The invoice prefix.
- defaultTemplate string? - The default invoice template.
- nextInvoiceNumber int - The number that will be used for the next invoice.
- defaultPaymentDetails string? - The default payment details.
- serviceDateOnInvoice boolean - Indicates whether service date can be used on invoices.
- emailSettings EmailTemplatePatch? - Email settings.
reckon.one: InvoicesSummary
Summary of a book's invoices.
Fields
- totalInvoiceCount int - The total number of invoices.
- totalInvoiceAmount decimal - The total monetary amount of included invoices.
- unpaidInvoiceCount int - The total number of unpaid invoices.
- unpaidInvoiceAmount decimal - The total monetary amount of unpaid invoices.
- overdueInvoiceCount int - The total number of unpaid invoices that are overdue.
- overdueInvoiceAmount decimal - The total monetary amount of unpaid invoices that are overdue.
reckon.one: InvoiceTemplate
Details of an invoice template.
Fields
- id string - The template's id.
- name string? - The template's name.
- font string? - The template's font.
- fontSize float - The template's font size.
- header InvoiceTemplateHeader? - The header details of the template.
- content InvoiceTemplateContent? - The content's details of the template.
- footer InvoiceTemplateFooter? - The footer details of the template.
reckon.one: InvoiceTemplateContent
Details of the content of an invoice template.
Fields
- project boolean - Is Project included in the template's content.
- item boolean - Is Item included in the template's content.
- itemPrice boolean - Is ItemPrice included in the template's content.
- description boolean - Is Description included in the template's content.
- quantity boolean - Is Quantity included in the template's content.
- taxCode boolean - Is TaxCode included in the template's content.
- tax boolean - Is Tax included in the template's content.
- amount boolean - Is Amount included in the template's content.
- serviceDate boolean - Is ServiceDate included in the template's content.
- account boolean - Is Account included in the template's content.
- discount boolean - Is Discount included in the template's content.
reckon.one: InvoiceTemplateFooter
Details of the footer of an invoice template.
Fields
- note boolean - Is Note included in the template's footer.
- subtotal boolean - Is Subtotal included in the template's footer.
- taxAmount boolean - Is TaxAmount included in the template's footer.
- invoiceDiscount boolean - Is InvoiceDiscount included in the template's footer.
- balanceDue boolean - Is BalanceDue included in the template's footer.
- totalExcludingTax boolean - Is TotalExcludingTax included in the template's footer.
- howToPay boolean - Is HowToPay included in the template's footer.
reckon.one: InvoiceTemplateHeader
Details of the header of an invoice template.
Fields
- companyName boolean - Is CompanyName included in the template's header.
- companyAddress boolean - Is CompanyAddress included in the template's header.
- taxNumber boolean - Is TaxNumber included in the template's header.
- phoneNumber boolean - Is PhoneNumber included in the template's header.
- email boolean - Is Email included in the template's header.
- website boolean - Is Website included in the template's header.
- logo Logo? - Logo of the template's header.
- invoiceTo boolean - Is InvoiceTo included in the template's header.
- shipTo boolean - Is ShipTo included in the template's header.
- invoiceDate boolean - Is InvoiceDate included in the template's header.
- invoiceNumber boolean - Is InvoiceNumber included in the template's header.
- dueDate boolean - Is DueDate included in the template's header.
- invoiceDiscount boolean - Is InvoiceDiscount included in the template's header.
- paymentTerms boolean - Is PaymentTerms included in the template's header.
- referenceCode boolean - Is reference code included in the template's header.
- customText boolean - Is custom text included in the template's header.
- customTextValue string? - The custom text value of the template's header.
reckon.one: InvoiceTemplates
A list of invoice templates.
Fields
- list InvoiceTemplate[]? - The list of items.
reckon.one: Item
Details of an item.
Fields
- id string - The item's unique id.
- name string? - The name of the item.
- parentItem ItemRef? - The parent item if there is one.
- itemType ItemType - The item type.
- itemCode string? - The item code.
- amountTaxStatus ItemAmountTaxStatus - The tax status of an item's amount.
- status ItemStatus - The status of an item.
- fullName string? - The full name of the item.
- purchase ItemUse? - Details for the item when it is purchased.
- sale ItemUse? - Details for the item when it is sold.
- createdDateTime string - The date and time when the item was created.
- lastModifiedDateTime string - The date and time when the item was last modified.
reckon.one: ItemInput
Details of an item to create or update.
Fields
- name string - The name of the item.
- parentItem string? - The parent item if there is one.
- itemType ItemType? - The item type.
- itemCode string? - The item code.
- status ItemStatus? - The status of an item.
- purchase ItemUseInput? - Details for the item when it is purchased.
- sale ItemUseInput? - Details for the item when it is sold.
- amountTaxStatus ItemAmountTaxStatus - The tax status of an item's amount.
reckon.one: ItemPatch
Details of an item to patch.
Fields
- name string? - The name of the item.
- parentItem string? - The parent item if there is one.
- itemType ItemType? - The item type.
- itemCode string? - The item code.
- status ItemStatus? - The status of an item.
- purchase ItemUsePatch? - Details for the item when it is purchased.
- sale ItemUsePatch? - Details for the item when it is sold.
- amountTaxStatus ItemAmountTaxStatus? - The tax status of an item's amount.
reckon.one: ItemRef
Reference to an item.
Fields
- id string? - The unique id.
- name string? - The unique name.
reckon.one: Items
A list of items.
Fields
- list Item[]? - The list of items.
reckon.one: ItemUse
Item usage details.
Fields
- price decimal? - The item's price.
If the item's
amountTaxStatus
isInclusive
then the price includes tax (gross). If the item'samountTaxStatus
isExclusive
then the price excludes tax (net).
- priceAccuracy int? - The accuracy (number of decimal places) of the price.
- ledgerAccount LedgerAccountRef? - The ledger account.
- description string? - The description.
- taxRate TaxRateRef? - The tax rate.
reckon.one: ItemUseInput
Item usage details.
Fields
- price decimal? - The item's price.
If the item's
amountTaxStatus
isInclusive
then the price includes tax (gross). If the item'samountTaxStatus
isExclusive
then the price excludes tax (net).
- priceAccuracy int? - The accuracy (number of decimal places) of the price.
- ledgerAccount string - The ledger account.
- description string? - The description.
- taxRate string? - The tax rate.
reckon.one: ItemUsePatch
Item usage details.
Fields
- price decimal? - The item's price.
If the item's
amountTaxStatus
isInclusive
then the price includes tax (gross). If the item'samountTaxStatus
isExclusive
then the price excludes tax (net).
- priceAccuracy int? - The accuracy (number of decimal places) of the price.
- ledgerAccount string? - The ledger account.
- description string? - The description.
- taxRate string? - The tax rate.
reckon.one: LeaveBalanceItem
Details of a leave balance item.
Fields
- payItem PayItemRef? - The pay item.
- employee EmployeeRef? - The employee.
- leaveBalance decimal - The leave balance.
- leaveLoadingValue decimal - The leave loading currency value.
- oteValue decimal - The ordinary time earnings value.
- totalValue decimal - The total value.
reckon.one: LedgerAccount
Details of a ledger account.
Fields
- id string - The account's unique id.
- name string? - The name of the account.
- description string? - The description of the account.
- fullName string? - The full name of the account.
- isDebit boolean - Whether amounts in this account are usually debits.
- parentLedgerAccount LedgerAccountRef? - The parent ledger account if there is one.
- sortOrder int - The order in which the user would like to sort the account relative to other accounts.
- status LedgerAccountStatus - The status of a ledger account.
- defaultTaxRate TaxRef? - The default tax rate for the account.
- accountType LedgerAccountType - The ledger account type.
- accountCode string? - The account code.
- exportCode string? - The export code for the account.
- bankAccount BankAccount? - The bank account details when the
accountType
isCurrentAsset_Bank
.
- creditAccount CreditAccount? - The credit account details when the
accountType
isCurrentLiability_CreditCard
.
- balance decimal? - The account's balance as of now.
- lastModifiedDateTime string - The date and time when the account was last modified.
reckon.one: LedgerAccountBankInput
Bank account details.
Fields
- dateOpened string? - When the bank account was opened.
- lockoffDate string? - The date when transactions are locked. Transactions dated on or before this date cannot be amended.
- bankBranchNumber string? - The bank branch number (BSB in Australia, Sort Code in UK).
- bankAccountNumber string? - The bank account number.
- openingBalance decimal? - The bank account's opening balance.
- financialInstitution string? - The id of the financial institution.
- includeBalancingTransaction boolean - Whether to include a balancing transaction.
- payerIdentifier string? - The APCA number to identify the payer.
reckon.one: LedgerAccountBankPatch
Bank account details.
Fields
- dateOpened string? - When the bank account was opened.
- lockoffDate string? - The date when transactions are locked. Transactions dated on or before this date cannot be amended.
- bankBranchNumber string? - The bank branch number (BSB in Australia, Sort Code in UK).
- bankAccountNumber string? - The bank account number.
- openingBalance decimal? - The bank account's opening balance.
- financialInstitution string? - The id of the financial institution.
- includeBalancingTransaction boolean - Whether to include a balancing transaction.
- payerIdentifier string? - The APCA number to identify the payer.
reckon.one: LedgerAccountCreditInput
Credit account details.
Fields
- dateOpened string? - When the credit account was opened.
- lockoffDate string? - The date when transactions are locked. Transactions dated on or before this date cannot be amended.
- openingBalance decimal? - The credit account's opening balance.
reckon.one: LedgerAccountCreditPatch
Credit account details.
Fields
- dateOpened string? - When the credit account was opened.
- lockoffDate string? - The date when transactions are locked. Transactions dated on or before this date cannot be amended.
- openingBalance decimal? - The credit account's opening balance.
reckon.one: LedgerAccountInput
Details of a ledger account to create or update.
Fields
- name string - The name of the account.
- description string(default "empty string") - The description of the account.
- parentLedgerAccount string? - The parent ledger account if there is one.
- sortOrder int(default 0) - The order in which the user would like to sort the account relative to other accounts.
- status LedgerAccountStatus? - The status of a ledger account.
- defaultTaxRate string? - The default tax rate for the account.
- accountType LedgerAccountType - The ledger account type.
- accountCode string? - The account code.
- exportCode string? - The export code for the account.
- bankAccount LedgerAccountBankInput? - The bank account details - only when the
accountType
isCurrentAsset_Bank
.
- creditAccount LedgerAccountCreditInput? - The credit account details - only when the
accountType
isCurrentLiability_CreditCard
.
reckon.one: LedgerAccountPatch
Details of a ledger account to patch.
Fields
- name string? - The name of the account.
- description string? - The description of the account.
- parentLedgerAccount string? - The parent ledger account if there is one.
- sortOrder int? - The order in which the user would like to sort the account relative to other accounts.
- status LedgerAccountStatus? - The status of a ledger account.
- defaultTaxRate string? - The default tax rate for the account.
- accountCode string? - The account code.
- exportCode string? - The export code for the account.
- bankAccount LedgerAccountBankPatch? - The bank account details - only when the
accountType
isCurrentAsset_Bank
.
- creditAccount LedgerAccountCreditPatch? - The credit account details - only when the
accountType
isCurrentLiability_CreditCard
.
reckon.one: LedgerAccountRef
Reference to a ledger account.
Fields
- id string? - The unique id.
- name string? - The unique name.
reckon.one: LedgerAccounts
A list of ledger accounts.
Fields
- list LedgerAccount[]? - The list of items.
reckon.one: LinkedContactInput
Details of a contact to create or update within an existing organisation.
Fields
- name string - The contact's display name.
- salutation string? - The salutation to use in communications.
- department string? - The contact's department.
- position string? - The contact's position.
- status ContactStatus? - The status of a contact.
- notes string? - Notes for the contact.
- addresses AddressInput[]? - List of addresses.
- phoneNumbers PhoneNumberInput[]? - List of phone numbers.
- electronicAddresses ElectronicAddressInput[]? - List of electronic addresses.
reckon.one: Logo
Details of a logo.
Fields
- name string? - Name of the logo.
- url string? - Url of the logo.
reckon.one: OrganisationRef
Link to an organisation.
Fields
- id string? - The unique id.
- name string? - The unique name.
reckon.one: PayItemRef
Reference to a payroll pay item.
Fields
- id string? - The unique id.
- name string? - The unique name.
reckon.one: Payment
A Payment.
Fields
- id string - The payments unique id.
- paymentNumber string? - The payments number.
- supplier ContactRef? - The supplier that made the payment.
- paymentDate string - The date of the payment.
- ledgerAccount LedgerAccountRef? - The id of the bank/cash/credit account where the money was paid from.
- allocateFullAmount boolean - Whether the full amount of the payment has been allocated.
- paymentMethod PaymentMethodRef? - The payment method.
- reference string? - The payment reference.
- notes string? - Notes related to the payment.
- totalAmount decimal - The total amount of the bill.
- reconciliationStatus ReconciliationStatus - Reconciliation status.
- internalNotes string? - Internal Notes related to the payment.
- recurring RecurringTransactionRef? - Details of the recurring transaction.
- accountsPayableLedgerAccount LedgerAccountRef? - The accounts receivable ledger account.
- classification ClassificationRef? - The classification.
- transactionLinks TransactionLink[]? - Details of transactions that the payment has been allocated to.
- lineItems PaymentLineItem[]? - The individual items that make up the payment.
- lastModifiedDateTime string - The last modified date of the payment
reckon.one: PaymentLineItem
A payment line item.
Fields
- lineNumber int - The line number.
- lineId string - The line item's unique id.
- project ProjectRef? - The id and full name of the project that the line item relates to.
- itemDetails PaymentLineItemItemDetails? - Details of an item used on a payment line item.
- accountDetails PaymentLineItemAccountDetails? - Details of an account used on a payment line item.
- description string? - The description of the item.
- taxRate TaxRateRef? - The tax rate.
- taxAmount decimal? - The tax amount.
reckon.one: PaymentLineItemAccountDetails
Details of an account used on a payment line item.
Fields
- ledgerAccount LedgerAccountRef? - The id and full name of the ledger account that the line relates to.
- quantity decimal? - The quantity sold.
- amount decimal? - The amount.
reckon.one: PaymentLineItemItemDetails
Details of an item used on a payment line item.
Fields
- item ItemRef? - The id and full name of the item that the line relates to.
- price decimal? - The price of each item.
- quantity decimal? - The quantity sold.
- discountAmount decimal? - The total discount for the line item.
- discountPercent decimal? - The discount percentage for the line item.
reckon.one: PaymentMethod
Details of a payment method.
Fields
- id string - The payment method's id.
- name string? - The payment method's name.
reckon.one: PaymentMethodRef
Reference to a payment method.
Fields
- id string? - The unique id.
- name string? - The unique name.
reckon.one: PaymentMethods
Payment methods.
Fields
- list PaymentMethod[]? - The list of items.
reckon.one: Payments
A list of payments.
Fields
- list Payment[]? - The list of items.
reckon.one: PaymentTerm
A payment term
Fields
- id string - The payment term's unique id.
- name string? - The payment term's name.
- description string? - The payment term's description.
- status PaymentTermStatus - The status of a payment term.
- isDefault boolean - Whether the payment term is the default for the transaction type.
- useForInvoice boolean - Whether the payment term is used for invoice type transaction.
- netDueDay int - Net Due day of the payment term.
- netDueDaySelection NetDueDateOptions - Net due date options.
- isDueDateWeekend boolean - Whether the due date falls on weekend. If due date is on a weekend, push due date to the first Monday.
- isIssuedWithinDays boolean - Whether push invoice issue date to following month if invoice issued within x day(s) of the due date.
- issuedWithinDays int - Invoice issued within x day(s) of the due date
reckon.one: PaymentTermDueDate
A payment term's due date
Fields
- dueDate string - The due day of the payment term.
reckon.one: PaymentTermInput
Details of a payment term to create or update.
Fields
- name string - The payment term's name.
- description string(default "empty string") - The payment term's description.
- status PaymentTermStatus? - The status of a payment term.
- isDefault boolean(default false) - Whether the payment term is the default for the transaction type.
- useForInvoice boolean(default true) - Whether the payment term is used for invoice type transaction.
- netDueDay int - Net Due day of the payment term.
- netDueDaySelection NetDueDateOptions - Net due date options.
- isDueDateWeekend boolean(default false) - Whether the due date falls on weekend. If due date is on a weekend, push due date to the first Monday.
- isIssuedWithinDays boolean(default false) - Whether push invoice issue date to following month if invoice issued within x day(s) of the due date.
- issuedWithinDays int(default 0) - Invoice issued within x day(s) of the due date
reckon.one: PaymentTermPatch
Details of the payment term to patch.
Fields
- name string? - The payment term's name.
- description string? - The payment term's description.
- status PaymentTermStatus? - The status of a payment term.
- isDefault boolean? - Whether the payment term is the default for the transaction type.
- useForInvoice boolean? - Whether the payment term is used for invoice type transaction.
- netDueDay int? - Net Due day of the payment term.
- netDueDaySelection NetDueDateOptions? - Net due date options.
- isDueDateWeekend boolean? - Whether the due date falls on weekend. If due date is on a weekend, push due date to the first Monday.
- isIssuedWithinDays boolean? - Whether push invoice issue date to following month if invoice issued within x day(s) of the due date.
- issuedWithinDays int? - Invoice issued within x day(s) of the due date
reckon.one: PaymentTermRef
Reference to a payment term.
Fields
- id string? - The unique id.
- name string? - The unique name.
reckon.one: PaymentTerms
A list of payment terms.
Fields
- list PaymentTerm[]? - The list of items.
reckon.one: PayrollDefaultAccounts
The payroll settings' default accounts.
Fields
- expenseAccount LedgerAccountRef? - The expense account.
- expenseAccountForCompanySuperContributions LedgerAccountRef? - The expense account for company super contributions.
- liabilityAccount LedgerAccountRef? - The liability account.
- liabilityAccountForWithholdingAmounts LedgerAccountRef? - The liability account for withholding amount.
- payWitholdingAmountToSupplier boolean - Indicates whether pays to supplier through accounts payable.
- supplierForWithholdingAmounts OrganisationRef? - Supplier for withholding amounts.
reckon.one: PayrollDefaultAccountsPatch
The payroll settings' default accounts to patch.
Fields
- payWitholdingAmountToSupplier boolean? - Indicates whether pays to supplier through accounts payable.
- expenseAccount string? - The expense account.
- expenseAccountForCompanySuperContributions string? - The expense account for company super contributions.
- liabilityAccount string? - The liability account.
- liabilityAccountForWithholdingAmounts string? - The liability account for withholding amount.
- supplierForWithholdingAmounts string? - Supplier for withholding amounts.
reckon.one: PayrollDefaultBankAccounts
The details of payroll default bank accounts.
Fields
- directCreditPaymentsBankAccount LedgerAccountRef? - The direct credit payment bank account.
- cashPaymentsBankAccount LedgerAccountRef? - The cash payments account.
- superPaymentsBankAccount LedgerAccountRef? - The super payment bank account.
reckon.one: PayrollDefaultBankAccountsPatch
The details of payroll default bank accounts to patch.
Fields
- directCreditPaymentsBankAccount string? - The direct credit payment bank account.
- cashPaymentsBankAccount string? - The cash payments account.
- superPaymentsBankAccount string? - The super payment bank account.
reckon.one: PayrollDefaultPayments
The details of payroll default payments.
Fields
- roundToNearest decimal - Rounding settings of payments.
- expenseAccountForRounding LedgerAccountRef? - Expense account for rounding of cash payments.
reckon.one: PayrollDefaultPaymentsPatch
The details of payroll default payments to patch.
Fields
- roundToNearest decimal? - Rounding settings of payments.
- expenseAccountForRounding string? - Expense account for rounding of cash payments.
reckon.one: PayrollLeaveBalancesAndAccrualValueMetaData
Meta data for the Payroll Leave Balances and Accrual report.
Fields
- id string - The report's unique id.
- reportName string? - The report's name.
- bookName string? - The book's name.
- companyName string? - The company's name.
- period PayrollLeaveBalancesAndAccrualValueReportPeriod? - Periods that can be used for the Payroll Leave Balances And Accrual Value report.
- asAtDate string? - When using AsAtDate, the date that was used.
reckon.one: PayrollLeaveBalancesAndAccrualValueReport
The Payroll Leave Balances and Accrual report.
Fields
- data LeaveBalanceItem[]? - The report's data.
- metaData PayrollLeaveBalancesAndAccrualValueMetaData? - Data about the report.
- message string? - A message that might contain warnings or additional information about the report contents.
reckon.one: PayrollLeaveBalancesAndAccrualValueReportParameters
The parameters for the Payroll Leave Balances And Accrual Value report.
Fields
- period PayrollLeaveBalancesAndAccrualValueReportPeriod? - Periods that can be used for the Payroll Leave Balances And Accrual Value report.
- asAtDate string? - When using AsAtDate, the date to use.
- leaveItems string[]? - A list of leave item IDs to include in the report. If omitted, all leave items will be included.
- employees string[]? - A list of employee IDs to include in the report. If omitted, all employees will be included.
reckon.one: PayrollLegalContact
The payroll settings' ledgal contact details.
Fields
- firstName string? - The first name of legal contact.
- lastName string? - The last name of legal contact.
- title string? - The legal contact's title.
- phoneNumbers BookPhoneNumber[]? - List of phone numbers.
- electronicAddresses BookElectronicAddress[]? - List of electronic addresses.
reckon.one: PayrollLegalContactPatch
The payroll settings' legal contact details to patch.
Fields
- firstName string? - The first name of legal contact.
- lastName string? - The last name of legal contact.
- title string? - The legal contact's title.
reckon.one: PayrollSettings
A book's payroll settings
Fields
- legalContact PayrollLegalContact? - The payroll settings' legal contact.
- payrollSetup PayrollSetup? - The payroll setup details.
- defaultAccounts PayrollDefaultAccounts? - The default payroll accounts.
- defaultPayments PayrollDefaultPayments? - The default payroll payments.
- automaticPayrollPayments boolean - Indicates whether makes payroll salary and wages payments when users finish each pay run.
- defaultBankAccounts PayrollDefaultBankAccounts? - The default payroll bank accounts.
reckon.one: PayrollSettingsPatch
A book's payroll settings to patch.
Fields
- legalContact PayrollLegalContactPatch? - The payroll settings' legal contact.
- payrollSetup PayrollSetupPatch? - The payroll setup details.
- defaultAccounts PayrollDefaultAccountsPatch? - The default payroll accounts.
- defaultPayments PayrollDefaultPaymentsPatch? - The default payroll payments.
- automaticPayrollPayments boolean? - Indicates whether makes payroll salary and wages payments when users finish each pay run.
- defaultBankAccounts PayrollDefaultBankAccountsPatch? - The default payroll bank accounts.
reckon.one: PayrollSetup
A book's payroll setup.
Fields
- copyPayItemsFromPreviousPay boolean - Indicates whether the pay run copies pay items from previous pay (overrides default pay items).
- trackProjectCost boolean - Indicates whether it tracks project cost.
- allowPayRatesEditInPayRuns boolean - Indicates whether it allows editing of pay rates on pay runs.
- optionalEmailPasswordProtection boolean - Indicates whether it enables password protection for emailing pay slips and payment summaries.
- displayYTDOnPayrun boolean - Indicates whether it displays YTD amounts on pay run.
reckon.one: PayrollSetupPatch
A book's payroll setup to patch.
Fields
- copyPayItemsFromPreviousPay boolean? - Indicates whether the pay run copies pay items from previous pay (overrides default pay items).
- trackProjectCost boolean? - Indicates whether it tracks project cost.
- allowPayRatesEditInPayRuns boolean? - Indicates whether it allows editing of pay rates on pay runs.
- optionalEmailPasswordProtection boolean? - Indicates whether it enables password protection for emailing pay slips and payment summaries.
- displayYTDOnPayrun boolean? - Indicates whether it displays YTD amounts on pay run.
reckon.one: Permissions
List of permissions.
Fields
- list string[]? - The list of items.
reckon.one: PhoneNumber
A phone number.
Fields
- id string? - The phone number's unique id.
- 'type PhoneTypeRef? - The type of phone number.
- countryCode string? - The country code.
- areaCode string? - The area code.
- number string? - The number.
- extension string? - The extension.
reckon.one: PhoneNumberInput
Details of a phone number to create or update.
Fields
- countryCode string? - The country code.
- areaCode string? - The area code.
- number string? - The number.
- extension string? - The extension.
- 'type string - The id or name of the phone number type.
reckon.one: PhoneNumberPatch
Details of a phone number to update.
Fields
- countryCode string? - The country code.
- areaCode string? - The area code.
- number string? - The number.
- extension string? - The extension.
reckon.one: PhoneNumbers
A list of phone numbers.
Fields
- list PhoneNumber[]? - The list of items.
reckon.one: PhoneType
Details of a phone type.
Fields
- id string - The type's id.
- name string? - The type's name.
- description string? - The type's description.
- status TypeStatus - Type status.
- createdDateTime string - The creation UTC date time.
- lastModifiedDateTime string - The last modified UTC date time.
reckon.one: PhoneTypeInput
Details of a phone type to create or update.
Fields
- name string - The type's name.
- description string? - The type's description.
- status TypeStatus? - Type status.
reckon.one: PhoneTypePatch
Details of a phone type to create or update.
Fields
- name string? - The type's name.
- description string? - The type's description.
- status TypeStatus? - Type status.
reckon.one: PhoneTypeRef
Reference to a phone type.
Fields
- id string? - The unique id.
- name string? - The unique name.
reckon.one: PhoneTypes
Phone types.
Fields
- list PhoneType[]? - The list of items.
reckon.one: Project
A project
Fields
- id string - The project's unique id.
- name string? - The project's name.
- fullName string? - The project's full name.
- parentProject ProjectRef? - The parent project.
- status ProjectStatus - The status of a project.
- startDate string? - The date the project starts.
- endDate string? - The date the project ends.
- description string? - A description of the project.
- amountTaxStatus ProjectAmountTaxStatus - The tax status of an item's amount.
- customers ProjectCustomer[]? - Customers linked to the project.
- suppliers ProjectSupplier[]? - Suppliers linked to the project.
- items ProjectItem[]? - Items used in the project.
- lastModifiedDateTime string - Timestamp of when project record was last modified.
reckon.one: ProjectCustomer
A customer linked to a project.
Fields
- lineId string - Record identifier.
- customer ContactRef? - The customer.
- weighting decimal - Weighting.
reckon.one: ProjectCustomerInput
Details of a project customer to create or update.
Fields
- weighting decimal? - Weighting assigned to contact.
- customer string - The id or name of the customer.
reckon.one: ProjectCustomerPatch
Details of a project customer to patch.
Fields
- customer string? - The id or name of the customer.
reckon.one: ProjectInput
Details of a project to create or update.
Fields
- name string - Name of project.
- status ProjectStatus? - The status of a project.
- amountTaxStatus ProjectAmountTaxStatus? - The tax status of an item's amount.
- parentProject string? - The parent project.
- startDate string? - The date the project starts.
- endDate string? - The date the project ends.
- description string? - A description of the project.
- items ProjectItemInput[]? - Items used in the project.
- customers ProjectCustomerInput[]? - Customers linked to the project.
- suppliers ProjectSupplierInput[]? - Suppliers linked to the project.
reckon.one: ProjectItem
An item linked to a project.
Fields
- lineId string - Record identifier.
- item ItemRef? - The item.
- description string? - Description of the linked item.
- regularRate decimal? - Rate at which item is usually charged.
- projectRate decimal? - Rate at which item is charged for this project.
- taxRate TaxRateRef? - Tax rate of item.
reckon.one: ProjectItemInput
Details of a project item to create or update.
Fields
- item string - The id or name of the item.
- projectRate decimal? - Rate at which item is charged for this project.
reckon.one: ProjectItemPatch
Details of a project item to patch.
Fields
- item string? - The id or name of the item.
- projectRate decimal? - Rate at which item is charged for this project.
reckon.one: ProjectPatch
Details of a project to patch.
Fields
- name string? - Name of project.
- status ProjectStatus? - The status of a project.
- amountTaxStatus ProjectAmountTaxStatus? - The tax status of an item's amount.
- parentProject string? - The parent project.
- startDate string? - The date the project starts.
- endDate string? - The date the project ends.
- description string? - A description of the project.
reckon.one: ProjectRef
Reference to a project.
Fields
- id string? - The unique id.
- name string? - The unique name.
reckon.one: Projects
A list of projects.
Fields
- list Project[]? - The list of items.
reckon.one: ProjectSupplier
A supplier linked to a project.
Fields
- lineId string - Record identifier.
- supplier ContactRef? - The supplier.
- weighting decimal - Weighting.
reckon.one: ProjectSupplierInput
Details of a project supplier to create or update.
Fields
- weighting decimal? - Weighting assigned to contact.
- supplier string - The id or name of the supplier.
reckon.one: ProjectSupplierPatch
Details of a project supplier to patch.
Fields
- supplier string? - The id or name of the suplier.
reckon.one: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
reckon.one: Receipt
A receipt.
Fields
- id string - The receipt's unique id.
- receiptNumber string? - The receipt's number.
- customer ContactRef? - The customer that made the payment.
- receiptDate string - The date of the receipt.
- ledgerAccount LedgerAccountRef? - The id of the bank/cash/credit account where the money was received.
- allocateFullAmount boolean - Whether the full amount of the receipt has been allocated.
- paymentMethod PaymentMethodRef? - The payment method.
- reference string? - The receipt reference.
- notes string? - Notes related to the receipt.
- totalAmount decimal - The total amount of the invoice.
- reconciliationStatus ReconciliationStatus - Reconciliation status.
- internalNotes string? - Internal Notes related to the receipt.
- recurring RecurringTransactionRef? - Details of the recurring transaction.
- accountsReceivableLedgerAccount LedgerAccountRef? - The accounts receivable ledger account.
- classification ClassificationRef? - The classification.
- transactionLinks TransactionLink[]? - Details of transactions that the receipt has been allocated to.
- lineItems ReceiptLineItem[]? - The individual items that make up the receipt.
reckon.one: ReceiptInput
Details of a receipt to create or update.
Fields
- customer string - The customer that is being invoiced.
- receiptDate string - The date of the receipt.
- ledgerAccount string(default "The default bank account.") - The id or name of the bank/cash/credit account where the money was received.
- paymentMethod string? - The id or name of the payment method used.
- reference string? - The receipt reference.
- notes string? - Notes related to the receipt.
- totalAmount decimal - The total amount of the invoice.
- reconciliationStatus ReconciliationStatus? - Reconciliation status.
- internalNotes string? - Internal Notes related to the receipt.
- accountsReceivableLedgerAccount string(default "The default Accounts Receivable account") - The accounts receivable ledger account. Note: It is not currently possible to create multiple Accounts Receivable accounts so this field is read-only and will be ignored.
- classification string? - The classification.
- lineItems ReceiptLineItemInput[]? - The individual items that make up the receipt.
- transactionLinks ReceiptTransactionLinkInput[]? - Details of transactions that the receipt should be allocated to.
reckon.one: ReceiptLineItem
A receipt line item.
Fields
- lineNumber int - The line number.
- lineId string - The line item's unique id.
- project ProjectRef? - The id and full name of the project that the line item relates to.
- itemDetails ReceiptLineItemItemDetails? - Details of an item used on a receipt line item.
- accountDetails ReceiptLineItemAccountDetails? - Details of an account used on a receipt line item.
- description string? - The description of the item.
- taxRate TaxRateRef? - The tax rate.
- taxAmount decimal? - The tax amount.
reckon.one: ReceiptLineItemAccountDetails
Details of an account used on a receipt line item.
Fields
- ledgerAccount LedgerAccountRef? - The id and full name of the ledger account that the line relates to.
- quantity decimal? - The quantity sold.
- amount decimal? - The amount.
reckon.one: ReceiptLineItemAccountDetailsInput
Details of an account used on an receipt line item.
Fields
- ledgerAccount string - The id or full name of the ledger account that the line relates to. Note that the full name includes the name(s) of any parent account(s) separated by colons.
- quantity decimal? - The quantity purchased.
- amount decimal? - The amount including or excluding tax (depending on the
amountTaxStatus
).
reckon.one: ReceiptLineItemAccountDetailsPatch
Details of an account used on an receipt line item.
Fields
- ledgerAccount string? - The id or full name of the ledger account that the invoice line relates to. Note that the full name includes the name(s) of any parent account(s) separated by colons.
- quantity decimal? - The quantity purchased.
- amount decimal? - The amount including or excluding tax (depending on the invoice's
amountTaxStatus
).
reckon.one: ReceiptLineItemInput
Details of a receipt line item to create or update.
Fields
- lineNumber int - The line number.
- project string? - The id or full name of the project that the line item relates to. Note that the full name includes the name(s) of any parent project(s) separated by colons.
- itemDetails ReceiptLineItemItemDetailsInput? - Details of an item used on an invoice line item.
This must be omitted if
accountDetails
have been provided.
- accountDetails ReceiptLineItemAccountDetailsInput? - Details of an account used on an invoice line item.
This must be omitted if
itemDetails
have been provided.
- description string? - The description of the line.
- taxAmount decimal? - The tax amount.
- taxRate string? - The tax rate.
- taxIsModified boolean(default false) - Whether the tax amount has been modified.
reckon.one: ReceiptLineItemItemDetails
Details of an item used on a receipt line item.
Fields
- item ItemRef? - The id and full name of the item that the line relates to.
- price decimal? - The price of each item.
- quantity decimal? - The quantity sold.
- discountAmount decimal? - The total discount for the line item.
- discountPercent decimal? - The discount percentage for the line item.
reckon.one: ReceiptLineItemItemDetailsInput
Details of an item used on an receipt line item.
Fields
- item string - The id or full name of the item that the line relates to. Note that the full name includes the name(s) of any parent item(s) separated by colons.
- price decimal? - The price of each item including or excluding tax (depending on the
amountTaxStatus
).
- quantity decimal? - The quantity purchased.
- discountAmount decimal? - The total discount for the line item.
- discountPercent decimal? - The discount percentage for the line item.
reckon.one: ReceiptLineItemItemDetailsPatch
Details of an item used on an receipt line item.
Fields
- item string? - The id or full name of the item that the invoice line relates to. Note that the full name includes the name(s) of any parent item(s) separated by colons.
- price decimal? - The price of each item including or excluding tax (depending on the invoice's
amountTaxStatus
).
- quantity decimal? - The quantity purchased.
- discountAmount decimal? - The total discount for the line item.
- discountPercent decimal? - The discount percentage for the line item.
reckon.one: ReceiptLineItemPatch
Details of a receipt line item to update.
Fields
- lineNumber int? - The line number.
- project string? - The id or full name of the project that the line item relates to. Note that the full name includes the name(s) of any parent project(s) separated by colons.
- itemDetails ReceiptLineItemItemDetailsPatch? - Details of an item used on an receipt line item.
This must be omitted if
accountDetails
have been provided.
- accountDetails ReceiptLineItemAccountDetailsPatch? - Details of an account used on an receipt line item.
This must be omitted if
itemDetails
have been provided.
- description string? - The description of the line.
- taxAmount decimal? - The tax amount.
- taxRate string? - The tax rate.
reckon.one: ReceiptPatch
Details of a receipt to update.
Fields
- customer string? - The customer that is being invoiced.
- receiptDate string? - The date of the receipt.
- ledgerAccount string? - The id or name of the bank/cash/credit account where the money was received.
- paymentMethod string? - The id or name of the payment method used.
- reference string? - The receipt reference.
- notes string? - Notes related to the receipt.
- totalAmount decimal - The total amount of the invoice.
- reconciliationStatus ReconciliationStatus? - Reconciliation status.
- internalNotes string? - Internal Notes related to the receipt.
- classification string? - The classification.
reckon.one: Receipts
A list of receipts.
Fields
- list Receipt[]? - The list of items.
reckon.one: ReceiptSettings
A book's receipt settings.
Fields
- prefix string? - The receipt prefix.
- defaultBankAccount LedgerAccountRef? - The default invoice bank account.
- emailSettings EmailTemplate? - Email settings.
reckon.one: ReceiptSettingsPatch
Receipt settings to patch.
Fields
- prefix string? - The receipt prefix.
- defaultBankAccount string? - The default bank account.
- emailSettings EmailTemplatePatch? - Email settings.
reckon.one: ReceiptTransactionLinkInput
Details of a transaction to link to a receipt.
Fields
- transactionId string - The unique id of the linked transaction.
- transactionAmount decimal - The transaction's amount.
- transactionType LinkedTransactionType - Type of transaction linked to an invoice.
reckon.one: ReceiptTransactionLinkPatch
Selected fields to update in receipt's transaction link.
Fields
- transactionAmount decimal - The transaction's amount.
reckon.one: RecurringInvoiceLineItemTemplateDetails
Details of a recurring invoice line item.
Fields
- lineNumber int - The line number.
- lineId string - The line item's unique id.
- parentLineId string? - The parent line item's unique id.
- project ProjectRef? - The id and full name of the project that the line item relates to.
- itemDetails InvoiceLineItemItemDetails? - Details of an item used on an invoice line item.
- accountDetails InvoiceLineItemAccountDetails? - Details of an account used on an invoice line item.
- description string? - The description of the item.
- taxRate TaxRateRef? - The tax rate.
- taxAmount decimal? - The tax amount.
- taxIsModified boolean? - Whether the tax amount has been modified.
reckon.one: RecurringInvoiceTemplate
Recurring invoice template.
Fields
- id string - The recurring template's unique id.
- name string? - The recurring template's name.
- frequencyQuantity int - The number of days, weeks or months between each occurrence.
- frequencyPeriod FrequencyPeriod - Frequency period for a recurring template.
- startDate string - The date of the first occurrence.
- endOfSchedule FrequencyEndType - Frequency end type for a recurring template.
- endAfterOccurrences int? - Ends once the defined number of occurrences have occurred.
- endAfterDate string? - Ends once the defined date has been reached.
- useLastDayOfEachMonth boolean - Whether to always use the last day of each month for each occurrence.
- numberOfOccurrencesRemaining int? - The number of occurrences remaining.
- nextTransactionDate string? - The date of the next occurrence.
- status RecurringTemplateStatus - Recurring template status.
- emailAutomatically boolean - Whether to automatically email each occurrence.
- invoice RecurringInvoiceTemplateDetails? - Details of the recurring invoice.
reckon.one: RecurringInvoiceTemplateDetails
Details of the recurring invoice.
Fields
- customer ContactRef? - The customer that will be invoiced.
- billingAddress Address? - The address that the invoice will be sent to.
- shippingAddress Address? - The address that the goods/service will be sent to.
- totalAmount decimal - The total amount of the invoice.
- invoiceDiscountAmount decimal? - The invoice discount amount.
- invoiceDiscountPercent decimal? - The invoice discount percentage.
- amountTaxStatus AmountTaxStatus - The tax status of an amount.
- totalTaxAmount decimal? - The total tax amount of the invoice.
- paymentTerms PaymentTermRef? - Payment terms.
- reference string? - The invoice reference.
- accountsReceivableLedgerAccount LedgerAccountRef? - The accounts receivable ledger account.
- classification ClassificationRef? - The classification.
- template TemplateRef? - The template used to print or email the invoice.
- transactionLinks TransactionLink[]? - Details of transactions (e.g. Invoices) that are linked to the recurring template.
- lineItems RecurringInvoiceLineItemTemplateDetails[]? - The individual items that make up the invoice.
- notes string? - Notes related to the invoice.
- paymentDetails string? - Details of how to pay the invoice.
reckon.one: RecurringTemplateRef
Reference to a recurring template.
Fields
- id string? - The unique id.
- name string? - The unique name.
reckon.one: RecurringTransactionRef
Reference to a recurring transaction.
Fields
- id string? - The unique id.
- name string? - The unique name.
reckon.one: ReportDefinition
Details of a report definition.
Fields
- id string - The report's unique id.
- name string? - The reports name.
- description string? - A description of the report.
- favourite boolean - Whether the report is a favourite of the user.
- category ReportCategory - Report categories.
reckon.one: Reports
Reports
Fields
- list ReportDefinition[]? - The list of items.
reckon.one: Role
A role.
Fields
- id int - The role's unique id.
- name string? - The role's unique name.
- description string? - A description of the role.
- systemRole boolean - Whether or not the role is a system role. System roles cannot be modified or deleted.
- permissions RolePermissions? - The role's detailed permissions.
- users UserRef[]? - The users that are assigned to the role.
reckon.one: RolePermissions
Role permissions.
Fields
- accountEnquiryReport RolePermissionsForReport? - Permissions for the Account Enquiry report.
- accountListReport RolePermissionsForReport? - Permissions for the Account List report.
- accounts RolePermissionsCommon? - Permissions for the Chart of Accounts.
- activityStatements RolePermissionsWithPrintEmailLodge? - Permissions for Activity Statements.
- agedCreditorsSummaryReport RolePermissionsForReport? - Permissions for the Aged Creditors summary report.
- agedCreditorsTransactionsReport RolePermissionsForReport? - Permissions for the Aged Creditors transactions report.
- agedDebtorsSummaryReport RolePermissionsForReport? - Permissions for the Aged Debtors summary report.
- agedDebtorsTransactionsReport RolePermissionsForReport? - Permissions for the Aged Debtors transactions report.
- balanceSheetReport RolePermissionsForReport? - Permissions for the Balance Sheet report.
- bankAccounts RolePermissionsForBankAccounts? - Permissions for bank accounts.
- bankPayments RolePermissionsCommon? - Permissions for bank payments.
- bankReconciliationReport RolePermissionsForReport? - Permissions for the Bank Reconciliation report.
- bankStatementReport RolePermissionsForReport? - Permissions for the Bank Statement report.
- bankTransfers RolePermissionsCommon? - Permissions for bank transfers.
- billListReport RolePermissionsForReport? - Permissions for the Bill List report.
- bills RolePermissionsWithPrintEmailApprove? - Permissions for bills.
- bookSettings RolePermissionsForSettings? - Permissions for book settings.
- budgets RolePermissionsCommon? - Permissions for budgets.
- budgetsReport RolePermissionsForReport? - Permissions for the Budgets report.
- classifications RolePermissionsCommon? - Permissions for classifications.
- contactDetailTypes RolePermissionsCommon? - Permissions for contact detail types.
- contacts RolePermissionsCommon? - Permissions for contacts.
- creditNotes RolePermissionsWithPrintEmailApprove? - Permissions for credit notes.
- customerListReport RolePermissionsForReport? - Permissions for the Customer List report.
- customers RolePermissionsCommon? - Permissions for customers.
- customerStatements RolePermissionsForCustomerStatements? - Permissions for customer statements.
- customerTransactionsReport RolePermissionsForReport? - Permssions for the Customer Transactions report.
- departments RolePermissionsCommon? - Permissions for departments.
- documentStorage RolePermissionsForDocumentStorage? - Permissions for document storage.
- emails RolePermissionsForEmails? - Permissions for emails.
- emailSettings RolePermissionsForSettings? - Permissions for email settings.
- employees RolePermissionsForEmployees? - Permissions for employees.
- employeeListReport RolePermissionsForReport? - Permissions for the Employee List report.
- estimateListReport RolePermissionsForReport? - Permissions for the Estimate List report.
- estimates RolePermissionsWithPrintEmail? - Permissions for estimates.
- expenseClaimListReport RolePermissionsForReport? - Permissions for the Expense Claim List report.
- expenseClaims RolePermissionsWithPrintEmailApproveManage? - Permissions for expense claims.
- expenseClaimSettings RolePermissionsForSettings? - Permissions for expense claim settings.
- expenseClaimTransactionsReport RolePermissionsForReport? - Permissions for the Expense Claim Transactions report.
- gstSummaryReport RolePermissionsForReport? - Permissions for the GST Summary report.
- invoiceListReport RolePermissionsForReport? - Permissions for the Invoice List report.
- invoices RolePermissionsWithPrintEmailApprove? - Permissions for invoices.
- itemListReport RolePermissionsForReport? - Permissions for the Item List report.
- items RolePermissionsCommon? - Permissions for items.
- journalListReport RolePermissionsForReport? - Permissions for the Journal List report.
- journals RolePermissionsWithPrintApprove? - Permissions for journals.
- ledgerTransactionsReport RolePermissionsForReport? - Permissions for the Ledger Transactions report.
- moneyInSettings RolePermissionsForSettings? - Settings for money in settings (invoices, receipts, credit notes etc.).
- moneyOutSettings RolePermissionsForSettings? - Permissions for money out settings (bills, payments, supplier credit notes etc.).
- paymentListReport RolePermissionsForReport? - Permissions for the Payment List report.
- payments RolePermissionsWithPrintEmail? - Permissions for payments.
- payrollAdjustments RolePermissionsCommon? - Permissions for payroll adjustments.
- payrollDetailReport RolePermissionsForReport? - Permissions for the Payroll Detail report.
- payrollEmployeeListReport RolePermissionsForReport? - Permissions for the Payroll Employee List report.
- payrollItems RolePermissionsCommon? - Permissions for payroll pay items.
- payrollLeaveBalancesReport RolePermissionsForReport? - Permissions for the Payroll Leave Balances report.
- payrollPayments RolePermissionsCommon? - Permissions for payroll payments.
- payrollPaymentScheduleReport RolePermissionsForReport? - Permissions for the Payroll Payment Schedule report.
- payrollSettings RolePermissionsCommon? - Permissions for payroll settings.
- payrollSummaryReport RolePermissionsForReport? - Permissions for the Payroll Summary report.
- payrollTransactionSummaryReport RolePermissionsForReport? - Permissions for the Payroll Transaction Summary report.
- payrollPaymentSummaries RolePermissionsCommon? - Permissions for payroll payment summaries.
- payruns RolePermissionsWithApproveManage? - Permissions for pay runs.
- paySlips RolePermissionsForPaySlips? - Permissions for pay slips.
- profitAndLossReport RolePermissionsForReport? - Permissions for the Profit And Loss report.
- projectListReport RolePermissionsForReport? - Permissions for the Project List report.
- projects RolePermissionsCommon? - Permissions for projects.
- receiptListReport RolePermissionsForReport? - Permissions for the Receipts List report.
- receipts RolePermissionsWithPrintEmail? - Permissons for receipts.
- recurringTransactions RolePermissionsCommon? - Permissions for recurring transactions.
- reportSettings RolePermissionsForSettings? - Permissions for settings.
- restHooks RolePermissionsForRestHooks? - Permissions for REST hooks.
- roles RolePermissionsCommon? - Permissions for roles.
- salesByItemReport RolePermissionsForReport? - Permissions for the Sales By Item report.
- singleTouchPayroll RolePermissionsForStp? - Permissions for Single Touch Payroll.
- statementSettings RolePermissionsForSettings? - Permissions for statements.
- superContributionsByEmployeeReport RolePermissionsForReport? - Permissions for the Super Contributions By Employee report.
- superFunds RolePermissionsCommon? - Permissions for super funds.
- superStream RolePermissionsForSuperStream? - Permissions for SuperStream
- superTransactionsReport RolePermissionsForReport? - Permissions for the Super Transactions report.
- supplierCreditNotes RolePermissionsWithPrintApprove? - Permissions for supplier credit notes.
- supplierListReport RolePermissionsForReport? - Permissions for the Supplier List report.
- suppliers RolePermissionsCommon? - Permissions for suppliers.
- supplierTransactionsReport RolePermissionsForReport? - Permissions for the Supplier Transactions report.
- taxCodeExceptionReport RolePermissionsForReport? - Permissions for the Tax Code Exception report.
- taxCodeListReport RolePermissionsForReport? - Permissions for the Tax Code List report.
- taxCodeTransactionsReport RolePermissionsForReport? - Permissions for the Tax Code Transactions report.
- taxSettings RolePermissionsForTaxSettings? - Permissions for tax settings.
- terms RolePermissionsCommon? - Permissions for payment terms.
- timeEntries RolePermissionsForTimeEntries? - Permissions for time entries.
- timeEntriesReport RolePermissionsForReport? - Permissions for the Time Entries report.
- timeSettings RolePermissionsForSettings? - Permissions for time settings.
- topCustomersReport RolePermissionsForReport? - Permissions for the Top 10 Customers report.
- topExpenseAccountsReport RolePermissionsForReport? - Permissions for the Top 10 Expense Accounts report.
- topIncomeAccountsReport RolePermissionsForReport? - Permissions for the Top 10 Income Accounts report.
- topSuppliersReport RolePermissionsForReport? - Permissions for the Top 10 Suppliers report.
- tpar RolePermissionsWithPrintEmailLodge? - Permissions for TPAR.
- transactionLineItemsReport RolePermissionsForReport? - Permissions for the Transaction Line Items report.
- trialBalanceReport RolePermissionsForReport? - Permissions for the Trial Balance report.
- uninvoicedAmountsByCustomerReport RolePermissionsForReport? - Permissions for the Uninvoiced Amounts By Customer report.
- uninvoicedAmountsByProjectReport RolePermissionsForReport? - Permissions for the Uninvoiced Amounts By Project report.
- uninvoicedTransactionsByCustomerReport RolePermissionsForReport? - Permissions for the Uninvoiced Amounts By Customer report.
- uninvoicedTransactionsByProjectReport RolePermissionsForReport? - Permissions for the Uninvoiced Amounts By Project report.
- users RolePermissionsForUsers? - Permissions for users.
- vat RolePermissionsForVat? - Permissions for UK VAT.
reckon.one: RolePermissionsCommon
Common role permissions.
Fields
- view boolean - Whether the role has permission to view.
- create boolean - Whether the role has permission to create.
- edit boolean - Whether the role has permission to edit/update/modify.
- delete boolean - Whether the role has permission to delete.
reckon.one: RolePermissionsForBankAccounts
Role permissions for bank accounts.
Fields
- view boolean - Whether the role has permission to view.
- create boolean - Whether the role has permission to create.
- edit boolean - Whether the role has permission to edit/update/modify.
- delete boolean - Whether the role has permission to delete.
- 'import boolean - Whether the role has permission to import bank data.
- reconcile boolean - Whether the role has permission to reconcile a bank account.
reckon.one: RolePermissionsForCustomerStatements
Role permissions for customer statements.
Fields
- create boolean - Whether the role has permission to create.
reckon.one: RolePermissionsForDocumentStorage
Role permissions for document storage.
Fields
- upload boolean - Whether the role has permission to upload.
- download boolean - Whether the role has permission to download.
- delete boolean - Whether the role has permission to delete.
reckon.one: RolePermissionsForEmails
Role permissions for emails.
Fields
- viewHistory boolean - Whether the role has permission to view history.
- resend boolean - Whether the role has permission to resend.
reckon.one: RolePermissionsForEmployees
Role permissions for employees.
Fields
- view boolean - Whether the role has permission to view.
- create boolean - Whether the role has permission to create.
- edit boolean - Whether the role has permission to edit/update/modify.
- delete boolean - Whether the role has permission to delete.
- managePayrollEmployeeDetails boolean - Whether the role can manage payroll employee's details.
- viewOwnDetails boolean - Whether the role can view own employee details.
- editOwnDetails boolean - Whether the role can edit own employee details.
reckon.one: RolePermissionsForPaySlips
Role permissions for pay slips.
Fields
- view boolean - Whether the role has permission to view.
- manage boolean - Whether the role has permission to manage.
- print boolean - Whether the role has permission to print.
- email boolean - Whether the role has permission to email.
reckon.one: RolePermissionsForReport
Role permissions for a report.
Fields
- view boolean - Whether the role has permission to view the report.
- export boolean - Whether the role has permission to export the report.
reckon.one: RolePermissionsForRestHooks
Role permissions for REST hooks.
Fields
- modify boolean - Whether the role can modify REST hooks.
reckon.one: RolePermissionsForSettings
Role permissions for settings.
Fields
- view boolean - Whether the role has permission to view.
- edit boolean - Whether the role has permission to edit/update/modify.
reckon.one: RolePermissionsForStp
Role permissions for Single Touch Payroll.
Fields
- view boolean - Whether the role has permission to view.
- create boolean - Whether the role has permission to create.
- edit boolean - Whether the role has permission to edit/update/modify.
- send boolean - Whether the role has permission to send.
reckon.one: RolePermissionsForSuperStream
Role permissions for SuperStream.
Fields
- view boolean - Whether the role has permission to view.
- create boolean - Whether the role has permission to create.
- edit boolean - Whether the role has permission to edit/update/modify.
- delete boolean - Whether the role has permission to delete.
- canLodge boolean - Whether the role has permission to lodge SuperStream.
reckon.one: RolePermissionsForTaxSettings
Role permissions for tax settings.
Fields
- view boolean - Whether the role has permission to view.
- edit boolean - Whether the role has permission to edit/update/modify.
- canDeleteCodeGroup boolean - Whether the role has permission to delete tax codes and tax groups.
reckon.one: RolePermissionsForTimeEntries
Role permissions for time entries.
Fields
- approve boolean - Whether the role has permission to approve.
- print boolean - Whether the role has permission to print.
- view boolean - Whether the role has permission to view.
- create boolean - Whether the role has permission to create.
- edit boolean - Whether the role has permission to edit/update/modify.
- delete boolean - Whether the role has permission to delete.
- manage boolean - Whether the role has permission to manage.
- assignPayItem boolean - Whether the role has permission to assign a payroll pay item to a time entry.
reckon.one: RolePermissionsForUsers
Role permissions for users.
Fields
- view boolean - Whether the role has permission to view.
- create boolean - Whether the role has permission to create.
- edit boolean - Whether the role has permission to edit/update/modify.
- delete boolean - Whether the role has permission to delete.
- editOtherUser boolean - Whether the role has permission to edit another user's details.
- invite boolean - Whether the role has permission to invite a user.
reckon.one: RolePermissionsForVat
Role permissions for UK VAT.
Fields
- authorise boolean - Whether the role has permission to authorise.
- print boolean - Whether the role has permission to print.
- submit boolean - Whether the role has permission to submit.
- view boolean - Whether the role has permission to view.
reckon.one: RolePermissionsWithApproveManage
Common role permissions plus approve and manage actions.
Fields
- view boolean - Whether the role has permission to view.
- create boolean - Whether the role has permission to create.
- edit boolean - Whether the role has permission to edit/update/modify.
- delete boolean - Whether the role has permission to delete.
- approve boolean - Whether the role has permission to approve.
- manage boolean - Whether the role has permission to manage.
reckon.one: RolePermissionsWithPrintApprove
Common role permissions plus print and approve actions.
Fields
- print boolean - Whether the role has permission to print.
- view boolean - Whether the role has permission to view.
- create boolean - Whether the role has permission to create.
- edit boolean - Whether the role has permission to edit/update/modify.
- delete boolean - Whether the role has permission to delete.
- approve boolean - Whether the role has permission to approve.
reckon.one: RolePermissionsWithPrintEmail
Common role permissions plus print and email actions.
Fields
- print boolean - Whether the role has permission to print.
- view boolean - Whether the role has permission to view.
- create boolean - Whether the role has permission to create.
- edit boolean - Whether the role has permission to edit/update/modify.
- delete boolean - Whether the role has permission to delete.
- email boolean - Whether the role has permission to email.
reckon.one: RolePermissionsWithPrintEmailApprove
Common role permissions plus print, email and approve actions.
Fields
- email boolean - Whether the role has permission to email.
- print boolean - Whether the role has permission to print.
- view boolean - Whether the role has permission to view.
- create boolean - Whether the role has permission to create.
- edit boolean - Whether the role has permission to edit/update/modify.
- delete boolean - Whether the role has permission to delete.
- approve boolean - Whether the role has permission to approve.
reckon.one: RolePermissionsWithPrintEmailApproveManage
Common role permissions plus print, email, approve and manage actions.
Fields
- approve boolean - Whether the role has permission to approve.
- email boolean - Whether the role has permission to email.
- print boolean - Whether the role has permission to print.
- view boolean - Whether the role has permission to view.
- create boolean - Whether the role has permission to create.
- edit boolean - Whether the role has permission to edit/update/modify.
- delete boolean - Whether the role has permission to delete.
- manage boolean - Whether the role has permission to manage.
reckon.one: RolePermissionsWithPrintEmailLodge
Common role permissions plus print, email and lodge actions.
Fields
- email boolean - Whether the role has permission to email.
- print boolean - Whether the role has permission to print.
- view boolean - Whether the role has permission to view.
- create boolean - Whether the role has permission to create.
- edit boolean - Whether the role has permission to edit.
- delete boolean - Whether the role has permission to delete.
- lodge boolean - Whether the role has permission to lodge.
reckon.one: RoleRef
Reference to a role.
Fields
- id int? - The unique id.
- name string? - The unique name.
reckon.one: Roles
A list of roles.
Fields
- list Role[]? - The list of items.
reckon.one: SelfManagedSuperfund
A superfund’s details.
Fields
- fundName string? - The self-managed super fund’s (SMSF) name.
- electronicServiceAddress string? - The electronic address for service
reckon.one: SelfManagedSuperfundInput
Superfund’s details to create or update.
Fields
- fundName string? - The SelfManagedSuperfund (SMSF) Name
- electronicServiceAddress string? - The electronic address for service
reckon.one: SelfManagedSuperfundPatch
Superfund’s details to patch.
Fields
- fundName string? - The SelfManagedSuperfund (SMSF) Name
- electronicServiceAddress string? - The electronic address for service
reckon.one: StringIdResponse
A response that contains the resource's id.
Fields
- id string? - The resource's id.
reckon.one: Superfund
An organisations superfund.
Fields
- id string - The organisation's unique id.
- name string? - The organisation's display name.
- organisationName string? - The organisation's name.
- branch string? - The organisation's branch.
- notes string? - The organisation's notes.
- businessNumber1 string? - The organisation's business number 1.
- businessNumber2 string? - The organisation's business number 2.
- entityTypes EntityType? - The organisation's entity types.
- status string? - The organisation's status (Active or Inactive).
- electronicAddresses ElectronicAddress[]? - List of electronic addresses.
- phoneNumbers PhoneNumber[]? - List of phone numbers.
- addresses Address[]? - List of addresses.
- contacts ContactRef[]? - The organisation's contacts list.
- balance decimal? - The organisation's current balance.
- lastModifiedDateTime string - The organisation's last modified date.
- bankDetails SuperfundBankDetails? - The superfund's bank account details.
- superfundDetails SuperfundDetails? - The superfund fund details
- employees SuperfundEmployee[]? - The linked employee's.
reckon.one: SuperfundBankDetails
Details of a superfund's bank account.
Fields
- name string? - The bank account's name.
- bankBranchNumber string? - The bank account's branch number.
- bankAccountNumber string? - The bank account's number.
reckon.one: SuperfundBankDetailsInput
Details of a superfund's bank account to create or update.
Fields
- name string? - The bank account's name.
- bankBranchNumber string? - The bank account's branch number.
- bankAccountNumber string? - The bank account's number.
reckon.one: SuperfundBankDetailsPatch
Details of a superfund's bank account to patch.
Fields
- name string? - The bank account's name.
- bankBranchNumber string? - The bank account's branch number.
- bankAccountNumber string? - The bank account's number.
reckon.one: SuperfundDetails
Details of a superfund to create or update.
Fields
- superfundProvider SuperfundProvider2? - The superfund provider details
- smsf SelfManagedSuperfund? - The SMSF provider details
reckon.one: SuperfundDetailsInput
Details of a superfund to create or update.
Fields
- superfundProvider SuperfundProviderInput? - The superfundProvider details
- smsf SelfManagedSuperfundInput? - The SMSF provider details
reckon.one: SuperfundDetailsPatch
Details of a superfund to patch.
Fields
- superfundProvider SuperfundProviderPatch? - The superfundProvider details
- smsf SelfManagedSuperfundPatch? - The SMSF provider details
reckon.one: SuperfundEmployee
An employee linked to a superfund.
Fields
- id string - Id of an employee.
- name string? - Name of an employee.
reckon.one: SuperfundInput
Details of a superfund to create or update.
Fields
- name string - The organisation's display name.
- organisationName string? - The organisation's name.
- branch string? - The organisation's branch.
- notes string? - Notes for the organisation.
- businessNumber1 string? - The organisation's business number 1.
- businessNumber2 string? - The organisation's business number 2.
- status string? - The status of the organisation. (Active/Inactive)
- addresses AddressInput[]? - List of addresses.
- phoneNumbers PhoneNumberInput[]? - List of phone numbers.
- electronicAddresses ElectronicAddressInput[]? - List of electronic addresses.
- entityTypes EntityType? - The organisation's entity types.
- bankDetails SuperfundBankDetailsInput? - The superfund's bank account details.
- superFundDetails SuperfundDetailsInput? - The superfund's details.
reckon.one: SuperfundPatch
Details of the superfund to patch.
Fields
- name string? - The organisation's display name.
- organisationName string? - The organisation's name.
- branch string? - The organisation's branch.
- notes string? - Notes for the organisation.
- businessNumber1 string? - The organisation's business number 1.
- businessNumber2 string? - The organisation's business number 2.
- status string? - The status of the organisation. (Active/Inactive)
- entityTypes EntityTypePatch? - If the entity has other entity types.
- bankDetails SuperfundBankDetailsPatch? - The superfund's bank account details.
- superFundDetails SuperfundDetailsPatch? - The superfund's details.
reckon.one: SuperfundProvider
A super fund provider.
Fields
- id string - The super fund provider's unique id.
- name string? - The super fund provider's name.
- abn string? - The super fund provider's ABN.
- status SuperfundProviderStatus - The status of a super fund provider.
reckon.one: SuperfundProvider2
Superfund’s details
Fields
- fund FundDetails? - The superfund provider details
- employerNumber string? - APRA type employee number
reckon.one: SuperfundProviderInput
Superfund’s details to create or update
Fields
- fund string? - The superfund provider id
- employerNumber string? - APRA type employee number
reckon.one: SuperfundProviderPatch
Superfund’s details to patch
Fields
- fund string? - The superfund provider id
- employerNumber string? - APRA type employee number
reckon.one: SuperfundProviderProduct
A super fund provider's product.
Fields
- id string - The super fund provider product's unique id.
- name string? - The super fund provider product's name.
- usi string? - The unique superannuation identifier (USI).
- spin string? - The superannuation product identification number (SPIN).
reckon.one: SuperfundProviderProducts
A list of a super fund provider's products.
Fields
- list SuperfundProviderProduct[]? - The list of items.
reckon.one: SuperfundProviders
A list of super fund providers.
Fields
- list SuperfundProvider[]? - The list of items.
reckon.one: Superfunds
A list of super funds.
Fields
- list Superfund[]? - The list of items.
reckon.one: Supplier
A supplier.
Fields
- id string - The organisation's unique id.
- name string? - The organisation's display name.
- organisationName string? - The organisation's name.
- branch string? - The organisation's branch.
- notes string? - The organisation's notes.
- businessNumber1 string? - The organisation's business number 1.
- businessNumber2 string? - The organisation's business number 2.
- entityTypes EntityType? - The organisation's entity types.
- status string? - The organisation's status (Active or Inactive).
- electronicAddresses ElectronicAddress[]? - List of electronic addresses.
- phoneNumbers PhoneNumber[]? - List of phone numbers.
- addresses Address[]? - List of addresses.
- contacts ContactRef[]? - The organisation's contacts list.
- balance decimal? - The organisation's current balance.
- lastModifiedDateTime string - The organisation's last modified date.
- isTpar boolean? - If the supplier is Tpar (null for other types).
- billCount int? - The total number of bills that have been received from the organisation.
- bankDetails SupplierBankDetails? - The supplier's bank account details.
reckon.one: SupplierBankDetails
Details of a supplier's bank account.
Fields
- name string? - The bank account's name.
- bankBranchNumber string? - The bank account's branch number.
- bankAccountNumber string? - The bank account's number.
reckon.one: SupplierBankDetailsInput
Details of a supplier's bank account to create or update.
Fields
- name string? - The bank account's name.
- bankBranchNumber string? - The bank account's branch number.
- bankAccountNumber string? - The bank account's number.
reckon.one: SupplierBankDetailsPatch
Details of a supplier's bank account to patch.
Fields
- name string? - The bank account's name.
- bankBranchNumber string? - The bank account's branch number.
- bankAccountNumber string? - The bank account's number.
reckon.one: SupplierInput
Details of a supplier to create or update.
Fields
- name string - The organisation's display name.
- organisationName string? - The organisation's name.
- branch string? - The organisation's branch.
- notes string? - Notes for the organisation.
- businessNumber1 string? - The organisation's business number 1.
- businessNumber2 string? - The organisation's business number 2.
- status string? - The status of the organisation. (Active/Inactive)
- addresses AddressInput[]? - List of addresses.
- phoneNumbers PhoneNumberInput[]? - List of phone numbers.
- electronicAddresses ElectronicAddressInput[]? - List of electronic addresses.
- entityTypes EntityType? - The organisation's entity types.
- isTpar boolean - If the supplier is Tpar.
- bankDetails SupplierBankDetailsInput? - The supplier's bank account details.
reckon.one: SupplierPatch
Details of a supplier to patch.
Fields
- name string? - The organisation's display name.
- organisationName string? - The organisation's name.
- branch string? - The organisation's branch.
- notes string? - Notes for the organisation.
- businessNumber1 string? - The organisation's business number 1.
- businessNumber2 string? - The organisation's business number 2.
- status string? - The status of the organisation. (Active/Inactive)
- entityTypes EntityTypePatch? - If the entity has other entity types.
- isTpar boolean? - If the supplier is Tpar.
- bankDetails SupplierBankDetailsPatch? - The supplier's bank account details.
reckon.one: Suppliers
A list of suppliers.
Fields
- list Supplier[]? - The list of items.
reckon.one: TaxCode
Details of a tax code.
Fields
- id string - The tax code's id.
- name string? - The tax code's name.
- description string? - The description of the tax code.
- rate decimal - The rate for the tax code.
- purchaseTaxLedgerAccount LedgerAccount? - The ledger account to use for purchases.
- saleTaxLedgerAccount LedgerAccount? - The ledger account to use for sales.
- taxType TaxType? - Type of tax.
- useCodeOnPurchases boolean - Whether the tax code is used for purchases.
- useCodeOnSales boolean - Whether the tax code is used for sales.
- isCodeForEuropeanCommunityGoods boolean? - Whether the tax code is used for European community goods (UK only).
- isWholeAmountTax boolean - Whether the whole amount is tax.
reckon.one: TaxGroup
Details of a tax group.
Fields
- id string - The tax group's id.
- name string? - The tax group's name.
- description string? - The description of the tax group.
- rate decimal - The rate for the tax group.
- exportCode string? - The export code for the tax group.
- status TaxStatus - The status of an accounting category.
- useOnPurchases boolean - Whether the tax group is used for purchases.
- useOnSales boolean - Whether the tax group is used for sales.
- taxCodes TaxCode[]? - Tax codes assigned to the tax group.
- reportingLocations TaxReportingLocation[]? - Locations where the tax group will be included in reports.
- purchaseTaxLedgerAccounts LedgerAccount[]? - Accounts to use for purchases.
- saleTaxLedgerAccounts LedgerAccount[]? - Accounts to use for sales.
- lastModifiedDateTime string - Timestamp of when tax group record was last modified.
reckon.one: TaxGroups
A list of tax groups.
Fields
- list TaxGroup[]? - The list of items.
reckon.one: TaxRate
A tax rate.
Fields
- id string - The tax rate's Id
- name string? - The tax rate's name.
- description string? - The description of the tax rate.
- rate decimal - The rate of tax.
- status TaxStatus - The status of an accounting category.
- useOnPurchases boolean - Whether the tax rate can be used on purchases.
- useOnSales boolean - Whether the tax rate can be used on sales.
reckon.one: TaxRateRef
Reference to a tax rate.
Fields
- id string? - The unique id.
- name string? - The unique name.
reckon.one: TaxRates
A list of tax rates.
Fields
- list TaxRate[]? - The list of items.
reckon.one: TaxRef
Reference to a tax rate.
Fields
- id string? - The unique id.
- name string? - The unique name.
reckon.one: TaxReportingLocation
Tax reporting location.
Fields
- id string - The tax reporting location's id.
- taxCodeId string? - The tax code's id.
- taxGroupId string? - The tax group's id.
- location string? - The location in the report.
- appliesToPurchases boolean - Whether it applies to purchases.
- appliesToSales boolean - Whether it applies to sales.
- reportAmountType AmountType - The type of an amount.
- reportLocationType TaxCodeReportLocationType - Tax code reporting location.
reckon.one: TaxSettings
A book's tax settings
Fields
- taxRegistered boolean - Indicates whether you registered for tax.
- reportingBasis AccountingMethodType - The sales figures type of tax.
- amountTaxStatus SettingsAmountTaxStatus - The sales figures type of tax.
- defaultSalesTaxLedgerAccount LedgerAccountRef? - Default tax account ledger for sales.
- defaultPurchasesTaxLedgerAccount LedgerAccountRef? - Default tax account ledger for purchases.
- taxAmountEditable boolean - Indicates whether allows users to edit tax amounts.
- chooseAmountTaxStatus boolean - Indicates whether a user can choose if amounts include or exclude tax.
- basSettings BasReportSettings? - The BAS reporting preference details.
- emailSettings EmailSettings? - The email settings for tax.
reckon.one: TaxSettingsPatch
Tax settings to patch.
Fields
- taxRegistered boolean? - Indicates whether you registered for tax.
- reportingBasis AccountingMethodType? - The sales figures type of tax.
- amountTaxStatus SettingsAmountTaxStatus? - The sales figures type of tax.
- defaultSalesTaxLedgerAccount string? - Default tax account ledger for sales.
- defaultPurchasesTaxLedgerAccount string? - Default tax account ledger for purchases.
- taxAmountEditable boolean? - Indicates whether allows users to edit tax amounts.
- chooseAmountTaxStatus boolean? - Indicates whether a user can choose if amounts include or exclude tax.
- basSettings BasReportSettingsPatch? - The BAS reporting preference details.
- emailSettings EmailSettingsPatch? - The email settings for tax.
reckon.one: TaxType
The tax type.
Fields
- id string - The tax type's id.
- description string? - The description of the tax type.
- isPrimary boolean - Whether this is a primary tax.
- systemType TaxSystemType - The system tax type.
reckon.one: Template
Details of a template.
Fields
- id string - The template's id.
- name string? - The template's name.
- header TemplateHeader? - The header detail of a template.
reckon.one: TemplateHeader
Details of the header of a template.
Fields
- logo Logo? - Logo of the template's header.
- companyName boolean - Is CompanyName included in the template's header.
- companyAddress boolean - Is CompanyAddress included in the template's header.
- taxNumber boolean - Is TaxNumber included in the template's header.
- phoneNumber boolean - Is PhoneNumber included in the template's header.
- email boolean - Is Email included in the template's header.
- website boolean - Is Website included in the template's header.
reckon.one: TemplateHeaderBase
Base details of the header of a template.
Fields
- logo Logo? - Logo of the template's header.
reckon.one: TemplateRef
Reference to a template.
Fields
- id string? - The unique id.
- name string? - The unique name.
reckon.one: Templates
A list of templates.
Fields
- list Template[]? - The list of items.
reckon.one: TemplateTypeRef
Reference to a template.
Fields
- id string? - The unique id.
- name string? - The unique name.
- 'type string? - The resource's type.
reckon.one: TimeDetails
Time details for a time entry.
Fields
- startTime string? - The start time.
- endTime string? - The end time.
- duration int - The duration in minutes.
reckon.one: TimeDetailsInput
Time details for a time entry being created or updated.
Fields
- startTime string? - The start time. Required if duration is not specified.
- endTime string? - The end time. Required if duration is not specified.
- duration int? - The duration in minutes. Used if specified, otherwise calculated from startTime and endTime.
reckon.one: TimeDetailsPatch
Time details for a time entry being patched.
Fields
- startTime string? - The start time. Required if duration is not specified.
- endTime string? - The end time. Required if duration is not specified.
- duration int? - The duration in minutes. Used if specified, otherwise calculated from startTime and endTime.
reckon.one: TimeEntries
A list of time entries.
Fields
- list TimeEntry[]? - The list of items.
reckon.one: TimeEntry
A time entry.
Fields
- id string - The time entry's id.
- employee EmployeeRef? - The employee.
- timeEntryDate string - The date of the time entry.
- project ProjectRef? - The project.
- customer OrganisationRef? - The customer.
- classification ClassificationRef? - The classification.
- billable boolean - Whether the time entry is billable.
- billableStatus TimeEntryBillableStatus - Time entry billable status.
- approvalStatus TimeEntryApprovalStatus - Time entry approval status.
- declineReason string? - The reason why a time entry has been declined.
- item ItemRef? - The item.
- payrollItem PayItemRef? - The payroll pay item.
- time TimeDetails? - Details of the time.
- notes string? - Notes.
- transactionLinks TransactionLink[]? - Transactions linked to the time entry.
reckon.one: TimeEntryInput
Details of a time entry to create or update.
Fields
- employee string - The id or name of the employee that spent the time.
- timeEntryDate string - Date of the time entry.
- project string? - The id or full name of the project on which the time was spent on. Note that the full name includes the name(s) of any parent project(s) separated by colons.
- customer string? - The id or name of the customer for whom the time was spent on.
- classification string? - The id or name of the classification.
- billable boolean? - Whether the time can be billed to a customer.
- approvalStatus TimeEntryApprovalStatus - Time entry approval status.
- item string? - The id or full name of the item that the time entry relates to. Note that the full name includes the name(s) of any parent item(s) separated by colons.
- payrollItem string? - The id or full name of the payroll pay item that the time entry relates to.
- time TimeDetailsInput - Details of the time spent.
- notes string? - Notes related to the time entry.
- declineReason string? - Reason why time entry approval was declined. Note that this cannot be set via POST.
reckon.one: TimeEntryPatch
Details of a time entry to patch.
Fields
- employee string? - The id or name of the employee that spent the time.
- timeEntryDate string - Date of the time entry.
- project string? - The id or full name of the project on which the time was spent on. Note that the full name includes the name(s) of any parent project(s) separated by colons.
- customer string? - The id or name of the customer for whom the time was spent on.
- classification string? - The id or name of the classification.
- billable boolean? - Whether the time can be billed to a customer.
- approvalStatus TimeEntryApprovalStatus - Time entry approval status.
- item string? - The id or full name of the item that the time entry relates to. Note that the full name includes the name(s) of any parent item(s) separated by colons.
- payrollItem string? - The id or full name of the payroll pay item that the time entry relates to.
- time TimeDetailsPatch? - Details of the time spent.
- notes string? - Notes related to the time entry.
- declineReason string? - Reason why time entry approval was declined.
reckon.one: TimeEntrySettings
A book's time entry settings.
Fields
- approvalEnabled boolean - Indicates whether time entry approval is enabled.
- firstDayOfWeek TimeStartOfWeek - The start time of a week.
- transferBillableTimeAs TransferBillableTimeSettings? - The transfer billable time settings.
reckon.one: TimeEntrySettingsPatch
Time entry settings to patch.
Fields
- approvalEnabled boolean? - Indicates whether time entry approval is enabled.
- firstDayOfWeek TimeStartOfWeek? - The start time of a week.
- transferBillableTimeAs TransferBillableTimeSettingsPatch? - The transfer billable time settings.
reckon.one: TransactionLink
A transaction linked to another transaction.
Fields
- linkId string? - The link's unique id.
- transactionType LinkedTransactionType - Type of transaction linked to an invoice.
- transactionId string - The unique id of the linked transaction.
- transactionNumber string? - The transaction number of the linked transaction.
- transactionDate string? - The transaction's date.
- transactionReference string? - The transaction's reference.
- transactionAmount decimal? - The transacion's amount.
- href string? - The URL to use to retrieve the linked transaction.
reckon.one: TransferBillableTimeSettings
The billable time transfer settings.
Fields
- combineItemAndRate boolean - Indicates whether combines time item and rate when transferring billable time.
- transferNotes boolean - Indicates whether use time sheet entry note as line item description when transferring billable time separately.
- transferDescriptions boolean - Indicates whether use chargeable item description as line item description when transferring billable time separately.
reckon.one: TransferBillableTimeSettingsPatch
Transfer billable time settings to patch.
Fields
- combineItemAndRate boolean? - Indicates whether to combine the item and the rate when transferring billable time.
- transferNotes boolean? - Indicates whether to use time sheet entry notes as line item descriptions when transferring billable time separately.
- transferDescriptions boolean? - Indicates whether to use chargeable item descriptions as line item descriptions when transferring billable time separately.
reckon.one: TypeRef
Reference to a type.
Fields
- id string? - The unique id.
- name string? - The unique name.
reckon.one: TypeResponse
A response that contains the resource's type id and name.
Fields
- id string? - The resource's id.
- 'type TypeRef? - Reference to a type.
reckon.one: UpdateAddressInput
Details of an address to update.
Fields
- line1 string? - Line 1 of the address.
- line2 string? - Line 2 of the address.
- line3 string? - Line 3 of the address.
- suburb string? - The suburb.
- town string? - The town.
- state string? - The state.
- postcode string? - The post code.
- country string? - The country.
reckon.one: UpdateElectronicAddressInput
Details of a electronic address to update.
Fields
- address string? - The electronic address.
reckon.one: UpdatePhoneNumberInput
Details of a phone number to update.
Fields
- countryCode string? - The country code.
- areaCode string? - The area code.
- number string? - The number.
- extension string? - The extension.
reckon.one: User
A user.
Fields
- id string - Then user's unique id.
- name string? - The user's name.
- email string? - The user's email address.
- portalUserId string? - The user's unique Reckon Id.
- employee EmployeeRef? - The user's employee reference.
- roles RoleRef[]? - List of roles user is assigned to.
- currentUser boolean - Indicates whether this is the currently logged in user.
- lastLoggedInDateTime string? - The last time the user logged in.
- invitationStatus UserEmployeeInvitationStatus - Status of user employee invitation.
reckon.one: UserRef
Reference to a user.
Fields
- id string? - The unique id.
- name string? - The unique name.
reckon.one: Users
A list of users.
Fields
- list User[]? - The list of items.
String types
reckon.one: AccountingMethodType
AccountingMethodType
The sales figures type of tax.
reckon.one: AmountTaxStatus
AmountTaxStatus
The tax status of an amount.
reckon.one: AmountType
AmountType
The type of an amount.
reckon.one: BillableStatus
BillableStatus
The billable status.
reckon.one: BookOwnership
BookOwnership
Book ownership.
reckon.one: BookStatus
BookStatus
A book's status.
reckon.one: ClassificationStatus
ClassificationStatus
Classification status
reckon.one: ContactStatus
ContactStatus
The status of a contact.
reckon.one: CreditNoteStatus
CreditNoteStatus
The status of a credit note.
reckon.one: DepartmentStatus
DepartmentStatus
The status of a department.
reckon.one: EmailStatus
EmailStatus
The status of an emailed invoice.
reckon.one: ExpenseClaimStatus
ExpenseClaimStatus
The status of an expense claim.
reckon.one: FrequencyEndType
FrequencyEndType
Frequency end type for a recurring template.
reckon.one: FrequencyPeriod
FrequencyPeriod
Frequency period for a recurring template.
reckon.one: InvoiceConvertFromType
InvoiceConvertFromType
Types of an invoice to create from, which can be an estimate, sales order and etc.
reckon.one: InvoiceStatus
InvoiceStatus
The status of an invoice.
reckon.one: ItemAmountTaxStatus
ItemAmountTaxStatus
The tax status of an item's amount.
reckon.one: ItemStatus
ItemStatus
The status of an item.
reckon.one: ItemType
ItemType
The item type.
reckon.one: LedgerAccountStatus
LedgerAccountStatus
The status of a ledger account.
reckon.one: LedgerAccountType
LedgerAccountType
The ledger account type.
reckon.one: LinkedTransactionType
LinkedTransactionType
Type of transaction linked to an invoice.
reckon.one: NetDueDateOptions
NetDueDateOptions
Net due date options.
reckon.one: PaymentTermStatus
PaymentTermStatus
The status of a payment term.
reckon.one: PayrollLeaveBalancesAndAccrualValueReportPeriod
PayrollLeaveBalancesAndAccrualValueReportPeriod
Periods that can be used for the Payroll Leave Balances And Accrual Value report.
reckon.one: ProjectAmountTaxStatus
ProjectAmountTaxStatus
The tax status of an item's amount.
reckon.one: ProjectStatus
ProjectStatus
The status of a project.
reckon.one: ReconciliationStatus
ReconciliationStatus
Reconciliation status.
reckon.one: RecurringTemplateStatus
RecurringTemplateStatus
Recurring template status.
reckon.one: ReportCategory
ReportCategory
Report categories.
reckon.one: SettingsAmountTaxStatus
SettingsAmountTaxStatus
The sales figures type of tax.
reckon.one: SuperfundProviderStatus
SuperfundProviderStatus
The status of a super fund provider.
reckon.one: TaxCodeReportLocationType
TaxCodeReportLocationType
Tax code reporting location.
reckon.one: TaxStatus
TaxStatus
The status of an accounting category.
reckon.one: TaxSystemType
TaxSystemType
The system tax type.
reckon.one: TimeEntryApprovalStatus
TimeEntryApprovalStatus
Time entry approval status.
reckon.one: TimeEntryBillableStatus
TimeEntryBillableStatus
Time entry billable status.
reckon.one: TimeStartOfWeek
TimeStartOfWeek
The start time of a week.
reckon.one: TypeStatus
TypeStatus
Type status.
reckon.one: UserEmployeeInvitationStatus
UserEmployeeInvitationStatus
Status of user employee invitation.
Import
import ballerinax/reckon.one;
Metadata
Released date: about 2 years ago
Version: 1.3.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 0
Current verison: 7
Weekly downloads
Keywords
Finance/Accounting
Cost/Freemium
Contributors