quickbooks.online
Module quickbooks.online
API
Definitions
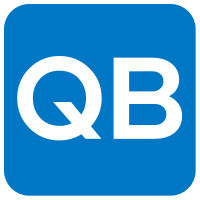
ballerinax/quickbooks.online Ballerina library
Overview
This is a generated connector for QuickBooks API v3 OpenAPI specification.
The QuickBooks Online Accounting API utilizes the REST architecture. It lets you seamlessly integrate your app with QuickBooks Online and the Intuit Financial Ecosystem
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a QuickBooks account.
- Obtain credentials by following Intuit Developer Documentation - Create and start developing your app. You can obtain tokens via the OAuth 2.0 Playground.
Quickstart
To use the QuickBooks Online connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
Import the ballerinax/quickbooks.online
module into the Ballerina project.
import ballerinax/quickbooks.online as quickbooks;
Step 2: Create a new connector instance
Create a configuration file named Config.toml
and add the tokens obtained through the OAuth 2.0 Playground to it as follows:
[authConfig] clientId=<CLIENT_ID> clientSecret=<CLIENT_SECRET> refreshToken=<REFRESH_TOKEN>
Create a quickbooks:ClientConfig and initialize the connector with it.
configurable quickbooks:OAuth2RefreshTokenGrantConfig & readonly authConfig = ?; quickbooks:ClientConfig clientConfig = {auth : authConfig}; quickbooks:Client baseClient = check new(clientConfig, "https://sandbox-quickbooks.api.intuit.com");
Step 3: Invoke connector operation
Now you can use the operations available within the connector. Note that they are available as remote operations.
For example, you can fetch a quickbook customer by ID as follows:
```ballerina public function main() returns error? { quickbooks:CustomerResponse response = check baseClient->readCustomer("<REALM_ID>", "<CUSTOMER_ID>"); log:printInfo("Customer Info : " + response.toString()); } ```
Use bal run
command to compile and run the Ballerina program.
Clients
quickbooks.online: Client
This is a generated connector for QuickBooks Online API v3 OpenAPI specification.
The QuickBooks Online Accounting API utilizes the REST architecture. It lets you seamlessly integrate your app with QuickBooks Online and the Intuit Financial Ecosystem
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Gets invoked to initialize the connector. During initialization you can pass either http:BearerTokenConfig if you have a bearer token or http:OAuth2RefreshTokenGrantConfig if you have Oauth tokens.
Create a QuickBooks account and obtain credentials following this guide.
Tokens can be obtained by using OAuth 2.0 Playground.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://quickbooks.api.intuit.com" - URL of the target service
createOrUpdateAccount
function createOrUpdateAccount(string realmId, AccountCreateObject payload, string accept) returns AccountResponse|error
Create or Update an Account. The ID of the object to update is specified in the request body.
Parameters
- realmId string - Realm ID.
- payload AccountCreateObject - Account creation data
- accept string (default "application/json") - Accept header
Return Type
- AccountResponse|error - Success
readAccount
function readAccount(string realmId, string accountId, string accept) returns AccountResponse|error
Retrieves the details of an Account object that has been previously created.
Parameters
- realmId string - Realm ID.
- accountId string - Account ID.
- accept string (default "application/json") - Accept header
Return Type
- AccountResponse|error - Success
createOrUpdateBill
function createOrUpdateBill(string realmId, BillCreateObject payload, string? operation, string accept) returns BillResponse|error
Create or Update a Bill. The ID of the object to update is specified in the request body.
Parameters
- realmId string - Realm ID.
- payload BillCreateObject - Bill creation data
- operation string? (default ()) - Opearation type
- accept string (default "application/json") - Accept header
Return Type
- BillResponse|error - Success
readBill
function readBill(string realmId, string billId, string accept) returns BillResponse|error
Retrieves the details of a bill that has been previously created.
Parameters
- realmId string - Realm ID.
- billId string - Bill ID.
- accept string (default "application/json") - Accept header
Return Type
- BillResponse|error - Success
createOrUpdateCustomer
function createOrUpdateCustomer(string realmId, CustomerCreateObject payload, string accept) returns CustomerResponse|error
Create or Update a Customer. The ID of the object to update is specified in the request body.
Parameters
- realmId string - Realm ID.
- payload CustomerCreateObject - Customer creation data
- accept string (default "application/json") - Accept header
Return Type
- CustomerResponse|error - Success
readCustomer
function readCustomer(string realmId, string customerId, string accept) returns CustomerResponse|error
Retrieves the details of a Customer object that has been previously created.
Parameters
- realmId string - Realm ID.
- customerId string - Customer ID.
- accept string (default "application/json") - Accept header
Return Type
- CustomerResponse|error - Success
createOrUpdatePayment
function createOrUpdatePayment(string realmId, PaymentCreateObject payload, string? operation, string accept) returns PaymentResponse|error
Create or Update a Payment. The ID of the object to update is specifiedin the request body.
Parameters
- realmId string - Realm ID.
- payload PaymentCreateObject - Payment creation data
- operation string? (default ()) - Opearation type
- accept string (default "application/json") - Accept header
Return Type
- PaymentResponse|error - Success
readPayment
function readPayment(string realmId, string paymentId, string accept) returns PaymentResponse|error
Retrieves the details of a Payment object that has been previously created.
Parameters
- realmId string - Realm ID.
- paymentId string - Payment ID.
- accept string (default "application/json") - Accept header
Return Type
- PaymentResponse|error - Success
createOrUpdateEstimate
function createOrUpdateEstimate(string realmId, EstimateCreateObject payload, string? operation, string accept) returns EstimateResponse|error
Create or Update an Estimate The ID of the object to update is specifiedin the request body.
Parameters
- realmId string - Realm ID.
- payload EstimateCreateObject - Estimate creation data
- operation string? (default ()) - Opearation type
- accept string (default "application/json") - Accept header
Return Type
- EstimateResponse|error - Success
readEstimate
function readEstimate(string realmId, string estimateId, string accept) returns EstimateResponse|error
Retrieves the details of an estimate that has been previously created.
Parameters
- realmId string - Realm ID.
- estimateId string - Estimate ID.
- accept string (default "application/json") - Accept header
Return Type
- EstimateResponse|error - Success
createOrUpdateInvoice
function createOrUpdateInvoice(string realmId, InvoiceCreateObject payload, string? operation, string accept) returns InvoiceResponse|error
Create or Update an Invoice The ID of the object to update is specifiedin the request body.
Parameters
- realmId string - Realm ID.
- payload InvoiceCreateObject - Invoice creation data
- operation string? (default ()) - Opearation type
- accept string (default "application/json") - Accept header
Return Type
- InvoiceResponse|error - Success
readInvoice
function readInvoice(string realmId, string invoiceId, string accept) returns InvoiceResponse|error
Retrieves the details of an invoice that has been previously created.
Parameters
- realmId string - Realm ID.
- invoiceId string - Invoice ID.
- accept string (default "application/json") - Accept header
Return Type
- InvoiceResponse|error - Success
createOrUpdateVendor
function createOrUpdateVendor(string realmId, VendorCreateObject payload, string accept) returns VendorResponse|error
Create or Update a vendor The ID of the object to update is specified in the request body. Either the DisplayName attribute or at least one of Title, GivenName, MiddleName, FamilyName, or Suffix attributes are required during create.
Parameters
- realmId string - Realm ID.
- payload VendorCreateObject - Vendor creation data
- accept string (default "application/json") - Accept header
Return Type
- VendorResponse|error - Success
readVendor
function readVendor(string realmId, string vendorId, string accept) returns VendorResponse|error
Retrieves the details of a vendor object that has been previously created.
Parameters
- realmId string - Realm ID.
- vendorId string - Vendor ID.
- accept string (default "application/json") - Accept header
Return Type
- VendorResponse|error - Success
queryEntity
Query an entity
Parameters
- realmId string - Realm ID.
- query string - Query statement.
- accept string (default "application/json") - Accept header
Return Type
- json|error - Success
Records
quickbooks.online: Account
Fields
- Id string? - Unique identifier for this object. Sort order is ASC by default.
- Name string? - User recognizable name for the Account. Account.Name attribute must not contain double quotes (") or colon (:).
- SyncToken string? - Version number of the object. It is used to lock an object for use by one app at a time. - As soon as an application modifies an object, its SyncToken is incremented. - Attempts to modify an object specifying an older SyncToken fails. - Only the latest version of the object is maintained by QuickBooks Online.
- AcctNum string? - User-defined account number to help the user in identifying the account within the chart-of-accounts and in deciding what should be posted to the account. The Account.AcctNum attribute must not contain colon (:). * Name must be unique. * For French Locales - - Length must be between 6 and 20 characters - Must start with the account number from the master category list. - Name limited to alpha-numeric characters. * Max length for Account.AcctNum - - AU & CA- 20 characters. - US, UK & IN- 7 characters
- CurrencyRef CurrencyRefType? - Currency reference type
- ParentRef Account_ParentRef? - Specifies the Parent AccountId if this represents a SubAccount.
- Description string? - User entered description for the account, which may include user entered information to guide bookkeepers/accountants in deciding what journal entries to post to the account.
- Active boolean? - Whether or not active inactive accounts may be hidden from most display purposes and may not be posted to.
- MetaData ModificationMetaData? - Modification metadata
- SubAccount boolean? - Specifies whether this object represents a parent (false) or subaccount (true). - Note that accounts of these types - OpeningBalanceEquity, UndepositedFunds, RetainedEarnings, CashReceiptIncome, CashExpenditureExpense, ExchangeGainOrLoss cannot have a sub account and cannot be a sub account of another account.
- Classification string? - The classification of an account. Not supported for non-posting accounts. - Valid values include- Asset, Equity, Expense, Liability, Revenue
- FullyQualifiedName string? - Fully qualified name of the object; derived from Name and ParentRef. - The fully qualified name prepends the topmost parent, followed by each subaccount separated by colons and takes the form of Parent:Account1:SubAccount1:SubAccount2. System generated. Limited to 5 levels.
- TxnLocationType string? - The account location. * Valid values include - WithinFrance - FranceOverseas - OutsideFranceWithEU - OutsideEU * For France locales, only.
- AccountType string? - A detailed account classification that specifies the use of this account. The type is based on the Classification.
- CurrentBalanceWithSubAccounts decimal? - Specifies the cumulative balance amount for the current Account and all its sub-accounts.
- AccountAlias string? - A user friendly name for the account. - It must be unique across all account categories. - For example, if an account is created under category 211 with AccountAlias of Terrains, then the system does not allow creation of an account with same AccountAlias of Terrains for any other category except 211. - In other words, 211001 and 215001 accounts cannot have same AccountAlias because both belong to different account category. - For France locales, only.
- TaxCodeRef ReferenceType? - Reference type
- AccountSubType string? - The account sub-type classification and is based on the AccountType value.
- CurrentBalance decimal? - Specifies the balance amount for the current Account. Valid for Balance Sheet accounts.
quickbooks.online: Account_ParentRef
Specifies the Parent AccountId if this represents a SubAccount.
Fields
- value string? - The ID for the referenced object as found in the Id field of the object payload. - The context is set by the type of reference and is specific to the QuickBooks company file.
- name string? - An identifying name for the object being referenced by value and is derived from the field that holds the common name of that object. - This varies by context and specific type of object referenced. - For example, references to a Customer object use Customer.DisplayName to populate this field. - Optionally returned in responses, implementation dependent.
quickbooks.online: AccountBasedExpenseLine
Fields
- Id string? - The Id of the line item. Its use in requests is as folllows - - If Id is greater than zero and exists for the company, the request is considered an update operation for a line item. - If no Id is provided, the Idprovided is less than or equal to zero, or the Idprovided is greater than zero and does not exist for the company then the request is considered a create operation for a line item. - Available in all objects that use lines and support the update operation.
- Amount decimal? - The amount of the line item.
- DetailType string? - Set to AccountBasedExpenseLineDetailfor this type of line.
- AccountBasedExpenseLineDetail AccountBasedExpenseLineDetail? - ItemBasedExpenseLineDetail
- LinkedTxn LinkedTxn[]? - Zero or more PurchaseOrder transactions linked to this Bill object. The LinkedTxn.TxnType should always be set to PurchaseOrder. Use LinkedTxn.TxnId as the ID of the PurchaseOrder. When updating an existing Bill to link to a PurchaseOrder a new Line must be created. This behavior matches the QuickBooks UI as it does not allow the linking of an existing line, but rather a new line must be added to link the PurchaseOrder. Over the API this is achieved by simply updating the Bill Line.Id to something new. This will ensure old bill line is deleted and the new line is linked to the PurchaseOrder.
- Description string? - Free form text description of the line item that appears in the printed record.
- LineNum decimal? - Specifies the position of the line in the collection of transaction lines. Positive Integer.
quickbooks.online: AccountBasedExpenseLineDetail
ItemBasedExpenseLineDetail
Fields
- TaxInclusiveAmt decimal? - The total amount of the line item including tax. Constraints- Available when endpoint is evoked with the minorversion=1query parameter.
- TaxAmount decimal? - Sales tax paid as part of the expense.
- AccountRef AccountBasedExpenseLineDetail_AccountRef? - Reference to the Expense account associated with this item. Query the Account name list resource to determine the appropriate Account object for this reference, where Account.AccountType=Expense. Use Account.Id and Account.Name from that object for AccountRef.value and AccountRef.name, respectively. For France locales- The account associated with the referenced Account object is looked up in the account category list. If this account has same location as specified in the transaction by the TransactionLocationType attribute and the same VAT as in the line item TaxCodeRef attribute, then this account is used. If there is a mismatch, then the account from the account category list that matches the transaction location and VAT is used. If this account is not present in the account category list, then a new account is created with the new location, new VAT code, and all other attributes as in the default account.
- CustomerRef ReferenceType? - Reference type
- PriceLevelRef ReferenceType? - Reference type
- ClassRef ReferenceType? - Reference type
- TaxCodeRef ReferenceType? - Reference type
- MarkupInfo record {}? - Reference to the TaxCodefor this item. Query the TaxCode name list resource to determine the appropriate TaxCode object for this reference. Use TaxCode.Id and TaxCode.Name from that object for TaxCodeRef.value and TaxCodeRef.name, respectively.
- BillableStatus string? - The billable status of the expense. Valid values- Billable, NotBillable, HasBeenBilled
quickbooks.online: AccountBasedExpenseLineDetail_AccountRef
Reference to the Expense account associated with this item. Query the Account name list resource to determine the appropriate Account object for this reference, where Account.AccountType=Expense. Use Account.Id and Account.Name from that object for AccountRef.value and AccountRef.name, respectively. For France locales- The account associated with the referenced Account object is looked up in the account category list. If this account has same location as specified in the transaction by the TransactionLocationType attribute and the same VAT as in the line item TaxCodeRef attribute, then this account is used. If there is a mismatch, then the account from the account category list that matches the transaction location and VAT is used. If this account is not present in the account category list, then a new account is created with the new location, new VAT code, and all other attributes as in the default account.
Fields
- value string? - The ID for the referenced object as found in the Id field of the object payload. The context is set by the type of reference and is specific to the QuickBooks company file.
- name string? - An identifying name for the object being referenced by value and is derived from the field that holds the common name of that object. This varies by context and specific type of object referenced. For example, references to a Customer object use Customer.DisplayName to populate this field. Optionally returned in responses, implementation dependent.
quickbooks.online: AccountCreateObject
Fields
- Name string - User recognizable name for the Account. Account.Name attribute must not contain double quotes (") or colon (:).
- AcctNum string? - User-defined account number to help the user in identifying the account within the chart-of-accounts and in deciding what should be posted to the account. The Account.AcctNum attribute must not contain colon (:). For France locales:Name must be unique. Length must be between 6 and 20 characters. Must start with the account number from the master category list. Name limited to alpha-numeric characters. Required for France locales.
- TaxCodeRef string? - Reference to the default tax code used by this account. Tax codes are referenced by the TaxCode. Id in the TaxCode object. Available when endpoint is invoked with the minorversion=3 query parameter. For global locales, only. Required for France locales
- AccountType string? - A detailed account classification that specifies the use of this account. The type is based on the Classification.
- AccountSubType string? - The account sub-type classification and is based on the AccountType value.
quickbooks.online: AccountResponse
Fields
- Account Account? -
quickbooks.online: Bill
Fields
- Id string? - Unique identifier for this object. Sort order is ASC by default.
- VendorRef ReferenceType? - Reference type
- Line (ItemBasedExpenseLine|AccountBasedExpenseLine)[]? - Individual line items of a transaction. Valid Line types include- ItemBasedExpenseLine and AccountBasedExpenseLine
- SyncToken string? - Version number of the object. It is used to lock an object for use by one app at a time. - As soon as an application modifies an object, its SyncToken is incremented. - Attempts to modify an object specifying an older SyncToken fails. - Only the latest version of the object is maintained by QuickBooks Online.
- CurrencyRef CurrencyRefType? - Currency reference type
- TxnDate string? - The date entered by the user when this transaction occurred. - For posting transactions, this is the posting date that affects the financial statements. - If the date is not supplied, the current date on the server is used. - Sort order is ASC by default.
- APAccountRef ReferenceType? - Reference type
- SalesTermRef ReferenceType? - Reference type
- LinkedTxn LinkedTxn[]? - Zero or more transactions linked to this Bill object. The LinkedTxn.TxnType can be set to PurchaseOrder, BillPaymentCheck or if using Minor Version 55 and above ReimburseCharge. Use LinkedTxn.TxnId as the ID of the transaction.
- GlobalTaxCalculation string? - Method in which tax is applied. Allowed values are- TaxExcluded, TaxInclusive, and NotApplicable.
- TotalAmt decimal? - Indicates the total amount of the transaction. This includes the total of all the charges, allowances, and taxes. Calculated by QuickBooks business logic; any value you supply is over-written by QuickBooks.
- TransactionLocationType string? - The account location. Valid values include- - WithinFrance - FranceOverseas - OutsideFranceWithEU - OutsideEU * For France locales, only.
- DueDate string? - Date when the payment of the transaction is due. If date is not provided, the number of days specified in SalesTermRef added the transaction date will be used.
- MetaData ModificationMetaData? - Modification metadata
- DocNumber string? - Reference number for the transaction. If not explicitly provided at create time, a custom value can be provided. - If no value is supplied, the resulting DocNumber is null. Throws an error when duplicate DocNumber is sent in the request. - Recommended best practice- check the setting of Preferences:OtherPrefs before setting DocNumber. - If a duplicate DocNumber needs to be supplied, add the query parameter name/value pair, include=allowduplicatedocnum to the URI. - Sort order is ASC by default.
- PrivateNote string? - User entered, organization-private note about the transaction. This note does not appear on the invoice to the customer. This field maps to the Memo field on the Invoice form.
- TxnTaxDetail record {}? - This data type provides information for taxes charged on the transaction as a whole. - It captures the details of all taxes calculated for the transaction based on the tax codes referenced by the transaction. - This can be calculated by QuickBooks business logic or you may supply it when adding a transaction. - If sales tax is disabled (Preferences.TaxPrefs.UsingSalesTax is set to false) then TxnTaxDetail is ignored and not stored.
- ExchangeRate decimal? - The number of home currency units it takes to equal one unit of currency specified by CurrencyRef. Applicable if multicurrency is enabled for the company.
- DepartmentRef ReferenceType? - Reference type
- IncludeInAnnualTPAR boolean? - Include the supplier in the annual TPAR. TPAR stands for Taxable Payments Annual Report. The TPAR is mandated by ATO to get the details payments that businesses make to contractors for providing services. Some government entities also need to report the grants they have paid in a TPAR.
- HomeBalance decimal? - Convenience field containing the amount in Balance expressed in terms of the home currency. Calculated by QuickBooks business logic. Value is valid only when CurrencyRef is specified and available when endpoint is evoked with the minorversion=3 query parameter. Applicable if multicurrency is enabled for the company.
- RecurDataRef ReferenceType? - Reference type
- Balance decimal? - The balance reflecting any payments made against the transaction. Initially set to the value of TotalAmt. A Balance of 0 indicates the bill is fully paid. Calculated by QuickBooks business logic; any value you supply is over-written by QuickBooks.
quickbooks.online: BillCreateObject
Fields
- VendorRef BillCreateObject_VendorRef - Reference to the vendor for this transaction. Query the Vendor name list resource to determine the appropriate Vendor object for this reference. Use Vendor.Id and Vendor.Name from that object for VendorRef.value and VendorRef.name, respectively.
- Line (ItemBasedExpenseLine|AccountBasedExpenseLine)[] - Individual line items of a transaction. Valid Line types include- ItemBasedExpenseLine and AccountBasedExpenseLine
- CurrencyRef CurrencyRefType? - Currency reference type
quickbooks.online: BillCreateObject_VendorRef
Reference to the vendor for this transaction. Query the Vendor name list resource to determine the appropriate Vendor object for this reference. Use Vendor.Id and Vendor.Name from that object for VendorRef.value and VendorRef.name, respectively.
Fields
- value string? - The ID for the referenced object as found in the Id field of the object payload. The context is set by the type of reference and is specific to the QuickBooks company file.
- name string? - An identifying name for the object being referenced by value and is derived from the field that holds the common name of that object. This varies by context and specific type of object referenced. For example, references to a Customer object use Customer.DisplayName to populate this field. Optionally returned in responses, implementation dependent.
quickbooks.online: BillResponse
Fields
- Bill Bill? -
quickbooks.online: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
quickbooks.online: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_1_1) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
quickbooks.online: CurrencyRefType
Currency reference type
Fields
- value string? - A three letter string representing the ISO 4217 code for the currency. For example, USD, AUD, EUR, and so on.
- name string? - The full name of the currency.
quickbooks.online: Customer
Fields
- Id string? - Unique identifier for this object. Sort order is ASC by default.
- SyncToken string? - Version number of the object. It is used to lock an object for use by one app at a time. As soon as an application modifies an object, its SyncToken is incremented. Attempts to modify an object specifying an older SyncToken fails. Only the latest version of the object is maintained by QuickBooks Online.
- DisplayName string? - The name of the person or organization as displayed. Must be unique across all Customer, Vendor, and Employee objects. Cannot be removed with sparse update. If not supplied, the system generates DisplayName by concatenating customer name components supplied in the request from the following list- Title, GivenName, MiddleName, FamilyName, and Suffix.
- Title string? - Title of the person. This tag supports i18n, all locales. The DisplayName attribute or at least one of Title, GivenName, MiddleName, FamilyName, or Suffix attributes is required.
- GivenName string? - Given name or first name of a person. The DisplayName attribute or at least one of Title, GivenName, MiddleName, FamilyName, or Suffix attributes is required.
- MiddleName string? - Middle name of the person. The person can have zero or more middle names. The DisplayName attribute or at least one of Title, GivenName, MiddleName, FamilyName, or Suffix attributes is required.
- Suffix string? - Suffix of the name. For example, Jr. The DisplayName attribute or at least one of Title, GivenName, MiddleName, FamilyName, or Suffix attributes is required.
- FamilyName string? - Family name or the last name of the person. The DisplayName attribute or at least one of Title, GivenName, MiddleName, FamilyName, or Suffix attributes is required.
- PrimaryEmailAddr EmailAddress? - Email address
- ResaleNum string? - Resale number or some additional info about the customer.
- SecondaryTaxIdentifier string? - Also called UTR No. in ( UK ) , CST Reg No. ( IN ) also represents the tax registration number of the Person or Organization. This value is masked in responses, exposing only last five characters. For example, the ID of 123-45-6789 is returned as XXXXXX56789.
- ARAccountRef ReferenceType? - Reference type
- DefaultTaxCodeRef ReferenceType? - Reference type
- PreferredDeliveryMethod string? - Preferred delivery method. Values are Print, Email, or None.
- GSTIN string? - GSTIN is an identification number assigned to every GST registered business.
- SalesTermRef ReferenceType? - Reference type
- CustomerTypeRef CustomerTypeRefType? - Reference to the customer type assigned to a customer. This field is only returned if the customer is assigned a customer type.
- Fax TelephoneNumber? - Telephone number
- BillWithParent boolean? - If true, this Customer object is billed with its parent. If false, or null the customer is not to be billed with its parent. This attribute is valid only if this entity is a Job or sub Customer.
- Mobile TelephoneNumber? - Telephone number
- PrimaryPhone TelephoneNumber? - Telephone number
- AlternatePhone TelephoneNumber? - Telephone number
- MetaData ModificationMetaData? - Modification metadata
- ParentRef ReferenceType? - Reference type
- WebAddr WebSiteAddress? - Website address
- ShipAddr PhysicalAddress? - Physical address
- PaymentMethodRef ReferenceType? - Reference type
- BillAddr PhysicalAddress? - Physical address
- Job boolean? - If true, this is a Job or sub-customer. If false or null, this is a top level customer, not a Job or sub-customer.
- BalanceWithJobs decimal? - Cumulative open balance amount for the Customer (or Job) and all its sub-jobs. Cannot be written to QuickBooks.
- OpenBalanceDate string? - Date of the Open Balance for the create operation. Write-on-create.
- Taxable boolean? - If true, transactions for this customer are taxable. Default behavior with minor version 10 and above- true, if DefaultTaxCodeRef is defined or false if TaxExemptionReasonId is set.
- Notes string? - Free form text describing the Customer.
- Active boolean? - If true, this entity is currently enabled for use by QuickBooks. If there is an amount in Customer.Balance when setting this Customer object to inactive through the QuickBooks UI, a CreditMemo balancing transaction is created for the amount.
- CompanyName string? - The name of the company associated with the person or organization.
- Balance decimal? - Specifies the open balance amount or the amount unpaid by the customer. For the create operation, this represents the opening balance for the customer. When returned in response to the query request it represents the current open balance (unpaid amount) for that customer. Write-on-create.
- IsProject boolean? - If true, indicates this is a Project.
- Source string? - The Source type of the transactions created by QuickBooks Commerce. Valid values include QBCommerce
- PrimaryTaxIdentifier string? - Also called Tax Reg. No in ( UK ) , ( CA ) , ( IN ) , ( AU ) represents the tax ID of the Person or Organization. This value is masked in responses, exposing only last five characters. For example, the ID of 123-45-6789 is returned as XXXXXX56789.
- GSTRegistrationType string? - For the filing of GSTR, transactions need to be classified depending on the type of customer to whom the sale is done. To facilitate this, we have introduced a new field as 'GST registration type'. Possible values are listed below- GST_REG_REG GST registered- Regular. Customer who has a business which is registered under GST and has a GSTIN (doesn’t include customers registered under composition scheme, as an SEZ or as EOU's, STP's EHTP's etc.). GST_REG_COMP GST registered-Composition. Customer who has a business which is registered under the composition scheme of GST and has a GSTIN. GST_UNREG GST unregistered. Customer who has a business which is not registered under GST and does not have a GSTIN. CONSUMER Consumer. Customer who is not registered under GST and is the final consumer of the service or product sold. OVERSEAS Overseas. Customer who has a business which is located out of India. SEZ SEZ. Customer who has a business which is registered under GST, has a GSTIN and is located in a SEZ or is a SEZ Developer. DEEMED Deemed exports- EOU's, STP's EHTP's etc. Customer who has a business which is registered under GST and falls in the category of companies (EOU's, STP's EHTP's etc.), to which supplies are made they are termed as deemed exports.
- PrintOnCheckName string? - Name of the person or organization as printed on a check. If not provided, this is populated from DisplayName. Constraints- Cannot be removed with sparse update.
- FullyQualifiedName string? - Fully qualified name of the object. The fully qualified name prepends the topmost parent, followed by each sub element separated by colons. Takes the form of Customer:Job:Sub-job. System generated. Limited to 5 levels.
- Level int? - Specifies the level of the hierarchy in which the entity is located. Zero specifies the top level of the hierarchy; anything above will be level with respect to the parent. Constraints:up to 5 levels
- TaxExemptionReasonId string? - The tax exemption reason associated with this customer object. Applicable if automated sales tax is enabled (Preferences.TaxPrefs.PartnerTaxEnabled is set to true) for the company. Set TaxExemptionReasonId to one of the following- - Id Reason 1. Federal government 2. State government 3. Local government 4. Tribal government 5. Charitable organization 6. Religious organization 7. Educational organization 8. Hospital 9. Resale 10. Direct pay permit 11. Multiple points of use 12. Direct mail 13. Agricultural production 14. Industrial production / manufacturing 15. Foreign diplomat
quickbooks.online: CustomerCreateObject
Fields
- Id string? - Unique identifier for this object. Sort order is ASC by default.
- SyncToken string? - Version number of the object. It is used to lock an object for use by one app at a time. As soon as an application modifies an object, its SyncToken is incremented. Attempts to modify an object specifying an older SyncToken fails. Only the latest version of the object is maintained by QuickBooks Online.
- DisplayName string? - The name of the person or organization as displayed. Must be unique across all Customer, Vendor, and Employee objects. Cannot be removed with sparse update. If not supplied, the system generates DisplayName by concatenating customer name components supplied in the request from the following list- Title, GivenName, MiddleName, FamilyName, and Suffix.
- Title string? - Title of the person. This tag supports i18n, all locales. The DisplayName attribute or at least one of Title, GivenName, MiddleName, FamilyName, or Suffix attributes is required.
- GivenName string? - Given name or first name of a person. The DisplayName attribute or at least one of Title, GivenName, MiddleName, FamilyName, or Suffix attributes is required.
- MiddleName string? - Middle name of the person. The person can have zero or more middle names. The DisplayName attribute or at least one of Title, GivenName, MiddleName, FamilyName, or Suffix attributes is required.
- Suffix string? - Suffix of the name. For example, Jr. The DisplayName attribute or at least one of Title, GivenName, MiddleName, FamilyName, or Suffix attributes is required.
- FamilyName string? - Family name or the last name of the person. The DisplayName attribute or at least one of Title, GivenName, MiddleName, FamilyName, or Suffix attributes is required.
- PrimaryEmailAddr EmailAddress? - Email address
- ResaleNum string? - Resale number or some additional info about the customer.
- SecondaryTaxIdentifier string? - Also called UTR No. in ( UK ) , CST Reg No. ( IN ) also represents the tax registration number of the Person or Organization. This value is masked in responses, exposing only last five characters. For example, the ID of 123-45-6789 is returned as XXXXXX56789.
- ARAccountRef ReferenceType? - Reference type
- DefaultTaxCodeRef ReferenceType? - Reference type
- PreferredDeliveryMethod string? - Preferred delivery method. Values are Print, Email, or None.
- GSTIN string? - GSTIN is an identification number assigned to every GST registered business.
- SalesTermRef ReferenceType? - Reference type
- CustomerTypeRef CustomerTypeRefType? - Reference to the customer type assigned to a customer. This field is only returned if the customer is assigned a customer type.
- Fax TelephoneNumber? - Telephone number
- BillWithParent boolean? - If true, this Customer object is billed with its parent. If false, or null the customer is not to be billed with its parent. This attribute is valid only if this entity is a Job or sub Customer.
- Mobile TelephoneNumber? - Telephone number
- PrimaryPhone TelephoneNumber? - Telephone number
- AlternatePhone TelephoneNumber? - Telephone number
- MetaData ModificationMetaData? - Modification metadata
- ParentRef ReferenceType? - Reference type
- WebAddr WebSiteAddress? - Website address
- ShipAddr PhysicalAddress? - Physical address
- PaymentMethodRef ReferenceType? - Reference type
- BillAddr PhysicalAddress? - Physical address
- Job boolean? - If true, this is a Job or sub-customer. If false or null, this is a top level customer, not a Job or sub-customer.
- BalanceWithJobs decimal? - Cumulative open balance amount for the Customer (or Job) and all its sub-jobs. Cannot be written to QuickBooks.
- OpenBalanceDate string? - Date of the Open Balance for the create operation. Write-on-create.
- Taxable boolean? - If true, transactions for this customer are taxable. Default behavior with minor version 10 and above- true, if DefaultTaxCodeRef is defined or false if TaxExemptionReasonId is set.
- Notes string? - Free form text describing the Customer.
- Active boolean? - If true, this entity is currently enabled for use by QuickBooks. If there is an amount in Customer.Balance when setting this Customer object to inactive through the QuickBooks UI, a CreditMemo balancing transaction is created for the amount.
- CompanyName string? - The name of the company associated with the person or organization.
- Balance decimal? - Specifies the open balance amount or the amount unpaid by the customer. For the create operation, this represents the opening balance for the customer. When returned in response to the query request it represents the current open balance (unpaid amount) for that customer. Write-on-create.
- Source string? - The Source type of the transactions created by QuickBooks Commerce. Valid values include QBCommerce
- PrimaryTaxIdentifier string? - Also called Tax Reg. No in ( UK ) , ( CA ) , ( IN ) , ( AU ) represents the tax ID of the Person or Organization. This value is masked in responses, exposing only last five characters. For example, the ID of 123-45-6789 is returned as XXXXXX56789.
- GSTRegistrationType string? - For the filing of GSTR, transactions need to be classified depending on the type of customer to whom the sale is done. To facilitate this, we have introduced a new field as 'GST registration type'. Possible values are listed below- GST_REG_REG GST registered- Regular. Customer who has a business which is registered under GST and has a GSTIN (doesn’t include customers registered under composition scheme, as an SEZ or as EOU's, STP's EHTP's etc.). GST_REG_COMP GST registered-Composition. Customer who has a business which is registered under the composition scheme of GST and has a GSTIN. GST_UNREG GST unregistered. Customer who has a business which is not registered under GST and does not have a GSTIN. CONSUMER Consumer. Customer who is not registered under GST and is the final consumer of the service or product sold. OVERSEAS Overseas. Customer who has a business which is located out of India. SEZ SEZ. Customer who has a business which is registered under GST, has a GSTIN and is located in a SEZ or is a SEZ Developer. DEEMED Deemed exports- EOU's, STP's EHTP's etc. Customer who has a business which is registered under GST and falls in the category of companies (EOU's, STP's EHTP's etc.), to which supplies are made they are termed as deemed exports.
- PrintOnCheckName string? - Name of the person or organization as printed on a check. If not provided, this is populated from DisplayName. Constraints- Cannot be removed with sparse update.
- Level int? - Specifies the level of the hierarchy in which the entity is located. Zero specifies the top level of the hierarchy; anything above will be level with respect to the parent. Constraints:up to 5 levels
- TaxExemptionReasonId string? - The tax exemption reason associated with this customer object. Applicable if automated sales tax is enabled (Preferences.TaxPrefs.PartnerTaxEnabled is set to true) for the company. Set TaxExemptionReasonId to one of the following- - Id Reason 1. Federal government 2. State government 3. Local government 4. Tribal government 5. Charitable organization 6. Religious organization 7. Educational organization 8. Hospital 9. Resale 10. Direct pay permit 11. Multiple points of use 12. Direct mail 13. Agricultural production 14. Industrial production / manufacturing 15. Foreign diplomat
quickbooks.online: CustomerResponse
Fields
- Customer Customer? -
quickbooks.online: CustomerTypeRefType
Reference to the customer type assigned to a customer. This field is only returned if the customer is assigned a customer type.
Fields
- value string? - The unique numeric Id of the customer type. This maps to the CustomerType entity- CustomerType.Id.
quickbooks.online: EmailAddress
Email address
Fields
- Address string? - An email address. The address format must follow the RFC 822 standard.
quickbooks.online: Estimate
Fields
- Id string? - Unique identifier for this object. Sort order is ASC by default.
- CustomerRef ReferenceType? - Reference type
- SyncToken string? - Version number of the object. It is used to lock an object for use by one app at a time. As soon as an application modifies an object, its SyncToken is incremented. Attempts to modify an object specifying an older SyncToken fails. Only the latest version of the object is maintained by QuickBooks Online.
- CurrencyRef CurrencyRefType? - Currency reference type
- BillEmail EmailAddress? - Email address
- TxnDate string? - The date entered by the user when this transaction occurred. For posting transactions, this is the posting date that affects the financial statements. If the date is not supplied, the current date on the server is used. Sort order is ASC by default.
- ShipFromAddr PhysicalAddress? - Physical address
- ShipDate string? - Date for delivery of goods or services.
- ClassRef ReferenceType? - Reference type
- PrintStatus string? - Printing status of the invoice. Valid values- NotSet, NeedToPrint, PrintComplete .
- CustomField record {}? - One of, up to three custom fields for the transaction. Available for custom fields so configured for the company. Check Preferences.SalesFormsPrefs.CustomField and Preferences.VendorAndPurchasesPrefs.POCustomField for custom fields currenly configured. Click here to learn about managing custom fields.
- SalesTermRef ReferenceType? - Reference type
- TxnStatus string? - One of the following status settings- Accepted, Closed, Pending, Rejected, Converted
- LinkedTxn LinkedTxn[]? - Zero or more Invoice objects related to this transaction. Use LinkedTxn.TxnId as the ID in a separate Invoice read request to retrieve details of the linked object.
- GlobalTaxCalculation string? - TaxExcluded Method in which tax is applied. Allowed values are- TaxExcluded, TaxInclusive, and NotApplicable.
- AcceptedDate string? - Date estimate was accepted.
- ExpirationDate string? - Date by which estimate must be accepted before invalidation.
- TransactionLocationType string? - The account location. Valid values include- - WithinFrance - FranceOverseas - OutsideFranceWithEU - OutsideEU - For France locales, only.
- DueDate string? - Date when the payment of the transaction is due. If date is not provided, the number of days specified in SalesTermRef added the transaction date will be used.
- MetaData ModificationMetaData? - Modification metadata
- DocNumber string? - Reference number for the transaction. If not explicitly provided at create time, this field is populated based on the setting of Preferences:CustomTxnNumber as follows- If Preferences:CustomTxnNumber is true a custom value can be provided. If no value is supplied, the resulting DocNumber is null. If Preferences:CustomTxnNumber is false, resulting DocNumber is system generated by incrementing the last number by 1. If Preferences:CustomTxnNumber is false then do not send a value as it can lead to unwanted duplicates. If a DocNumber value is sent for an Update operation, then it just updates that particular invoice and does not alter the internal system DocNumber. Note- DocNumber is an optional field for all locales except France. For France locale if Preferences:CustomTxnNumber is enabled it will not be automatically generated and is a required field.
- PrivateNote string? - User entered, organization-private note about the transaction. This note does not appear on the invoice to the customer. This field maps to the Memo field on the Invoice form.
- Line anydata[]? - Individual line items of a transaction. Valid Line types include- SalesItemLine, GroupLine, DescriptionOnlyLine (also used for inline Subtotal lines), DiscountLine and SubTotalLine (used for the overall transaction)
- CustomerMemo MemoRef? -
- EmailStatus string? - Email status of the invoice. Valid values- NotSet, NeedToSend, EmailSent
- TxnTaxDetail record {}? - This data type provides information for taxes charged on the transaction as a whole. It captures the details sales taxes calculated for the transaction based on the tax codes referenced by the transaction. This can be calculated by QuickBooks business logic or you may supply it when adding a transaction. See Global tax model for more information about this element. If sales tax is disabled (Preferences.TaxPrefs.UsingSalesTax is set to false) then TxnTaxDetail is ignored and not stored.
- AcceptedBy string? - Name of customer who accepted the estimate.
- ExchangeRate decimal? - The number of home currency units it takes to equal one unit of currency specified by CurrencyRef. Applicable if multicurrency is enabled for the company.
- ShipAddr PhysicalAddress? - Physical address
- DepartmentRef ReferenceType? - Reference type
- ShipMethodRef ReferenceType? - Reference type
- BillAddr PhysicalAddress? - Physical address
- ApplyTaxAfterDiscount boolean? - If false or null, calculate the sales tax first, and then apply the discount. If true, subtract the discount first and then calculate the sales tax.
- TotalAmt decimal? - Indicates the total amount of the transaction. This includes the total of all the charges, allowances, and taxes. Calculated by QuickBooks business logic; any value you supply is over-written by QuickBooks.
- RecurDataRef ReferenceType? - Reference type
- TaxExemptionRef ReferenceType? - Reference type
- HomeTotalAmt decimal? - Total amount of the transaction in the home currency. Includes the total of all the charges, allowances and taxes. Calculated by QuickBooks business logic. Value is valid only when CurrencyRef is specified. Applicable if multicurrency is enabled for the company.
- FreeFormAddress boolean? - Denotes how ShipAddr is stored- formatted or unformatted. The value of this flag is system defined based on format of shipping address at object create time. If set to false, shipping address is returned in a formatted style using City, Country, CountrySubDivisionCode, Postal code. If set to true, shipping address is returned in an unformatted style using Line1 through Line5 attributes.
quickbooks.online: EstimateCreateObject
Fields
- Id string? - Unique identifier for this object. Sort order is ASC by default.
- CustomerRef ReferenceType - Reference type
- SyncToken string? - Version number of the object. It is used to lock an object for use by one app at a time. As soon as an application modifies an object, its SyncToken is incremented. Attempts to modify an object specifying an older SyncToken fails. Only the latest version of the object is maintained by QuickBooks Online.
- CurrencyRef CurrencyRefType? - Currency reference type
- BillEmail EmailAddress? - Email address
- TxnDate string? - The date entered by the user when this transaction occurred. For posting transactions, this is the posting date that affects the financial statements. If the date is not supplied, the current date on the server is used. Sort order is ASC by default.
- ShipFromAddr PhysicalAddress? - Physical address
- ShipDate string? - Date for delivery of goods or services.
- ClassRef ReferenceType? - Reference type
- PrintStatus string? - Printing status of the invoice. Valid values- NotSet, NeedToPrint, PrintComplete .
- CustomField record {}? - One of, up to three custom fields for the transaction. Available for custom fields so configured for the company. Check Preferences.SalesFormsPrefs.CustomField and Preferences.VendorAndPurchasesPrefs.POCustomField for custom fields currenly configured. Click here to learn about managing custom fields.
- SalesTermRef ReferenceType? - Reference type
- TxnStatus string? - One of the following status settings- Accepted, Closed, Pending, Rejected, Converted
- LinkedTxn LinkedTxn[]? - Zero or more Invoice objects related to this transaction. Use LinkedTxn.TxnId as the ID in a separate Invoice read request to retrieve details of the linked object.
- GlobalTaxCalculation string? - TaxExcluded Method in which tax is applied. Allowed values are- TaxExcluded, TaxInclusive, and NotApplicable.
- AcceptedDate string? - Date estimate was accepted.
- ExpirationDate string? - Date by which estimate must be accepted before invalidation.
- TransactionLocationType string? - The account location. Valid values include- - WithinFrance - FranceOverseas - OutsideFranceWithEU - OutsideEU - For France locales, only.
- DueDate string? - Date when the payment of the transaction is due. If date is not provided, the number of days specified in SalesTermRef added the transaction date will be used.
- MetaData ModificationMetaData? - Modification metadata
- DocNumber string? - Reference number for the transaction. If not explicitly provided at create time, this field is populated based on the setting of Preferences:CustomTxnNumber as follows- If Preferences:CustomTxnNumber is true a custom value can be provided. If no value is supplied, the resulting DocNumber is null. If Preferences:CustomTxnNumber is false, resulting DocNumber is system generated by incrementing the last number by 1. If Preferences:CustomTxnNumber is false then do not send a value as it can lead to unwanted duplicates. If a DocNumber value is sent for an Update operation, then it just updates that particular invoice and does not alter the internal system DocNumber. Note- DocNumber is an optional field for all locales except France. For France locale if Preferences:CustomTxnNumber is enabled it will not be automatically generated and is a required field.
- PrivateNote string? - User entered, organization-private note about the transaction. This note does not appear on the invoice to the customer. This field maps to the Memo field on the Invoice form.
- Line anydata[]? - Individual line items of a transaction. Valid Line types include- SalesItemLine, GroupLine, DescriptionOnlyLine (also used for inline Subtotal lines), DiscountLine and SubTotalLine (used for the overall transaction)
- CustomerMemo MemoRef? -
- EmailStatus string? - Email status of the invoice. Valid values- NotSet, NeedToSend, EmailSent
- TxnTaxDetail record {}? - This data type provides information for taxes charged on the transaction as a whole. It captures the details sales taxes calculated for the transaction based on the tax codes referenced by the transaction. This can be calculated by QuickBooks business logic or you may supply it when adding a transaction. See Global tax model for more information about this element. If sales tax is disabled (Preferences.TaxPrefs.UsingSalesTax is set to false) then TxnTaxDetail is ignored and not stored.
- AcceptedBy string? - Name of customer who accepted the estimate.
- ExchangeRate decimal? - The number of home currency units it takes to equal one unit of currency specified by CurrencyRef. Applicable if multicurrency is enabled for the company.
- ShipAddr PhysicalAddress? - Physical address
- DepartmentRef ReferenceType? - Reference type
- ShipMethodRef ReferenceType? - Reference type
- BillAddr PhysicalAddress? - Physical address
- ApplyTaxAfterDiscount boolean? - If false or null, calculate the sales tax first, and then apply the discount. If true, subtract the discount first and then calculate the sales tax.
- FreeFormAddress boolean? - Denotes how ShipAddr is stored- formatted or unformatted. The value of this flag is system defined based on format of shipping address at object create time. If set to false, shipping address is returned in a formatted style using City, Country, CountrySubDivisionCode, Postal code. If set to true, shipping address is returned in an unformatted style using Line1 through Line5 attributes.
quickbooks.online: EstimateResponse
Fields
- Estimate Estimate? -
quickbooks.online: Invoice
Fields
- Id string? - Unique identifier for this object. Sort order is ASC by default.
- Line anydata[]? - Individual line items of a transaction. Valid Line types include SalesItemLine, GroupLine, DescriptionOnlyLine (also used for inline Subtotal lines), DiscountLine and SubTotalLine (used for the overall transaction)
- CustomerRef ReferenceType? - Reference type
- SyncToken string? - Version number of the object. It is used to lock an object for use by one app at a time. As soon as an application modifies an object, its SyncToken is incremented. Attempts to modify an object specifying an older SyncToken fails. Only the latest version of the object is maintained by QuickBooks Online.
- CurrencyRef CurrencyRefType? - Currency reference type
- DocNumber string? - Reference number for the transaction. If not explicitly provided at create time, this field is populated based on the setting of Preferences:CustomTxnNumber as follows- If Preferences:CustomTxnNumber is true a custom value can be provided. If no value is supplied, the resulting DocNumber is null. If Preferences:CustomTxnNumber is false, resulting DocNumber is system generated by incrementing the last number by 1. If Preferences:CustomTxnNumber is false then do not send a value as it can lead to unwanted duplicates. If a DocNumber value is sent for an Update operation, then it just updates that particular invoice and does not alter the internal system DocNumber. Note- DocNumber is an optional field for all locales except France. For France locale if Preferences:CustomTxnNumber is enabled it will not be automatically generated and is a required field. If a duplicate DocNumber needs to be supplied, add the query parameter name/value pair, include=allowduplicatedocnum to the URI.
- BillEmail EmailAddress? - Email address
- TxnDate string? - The date entered by the user when this transaction occurred. yyyy/MM/dd is the valid date format. For posting transactions, this is the posting date that affects the financial statements. If the date is not supplied, the current date on the server is used. Sort order is ASC by default.
- ShipFromAddr PhysicalAddress? - Physical address
- ShipDate string? - Date for delivery of goods or services.
- TrackingNum string? - Shipping provider's tracking number for the delivery of the goods associated with the transaction.
- ClassRef ReferenceType? - Reference type
- PrintStatus string? - Printing status of the invoice. Valid values- NotSet, NeedToPrint, PrintComplete .
- SalesTermRef ReferenceType? - Reference type
- TxnSource string? - Used internally to specify originating source of a credit card transaction.
- LinkedTxn anydata[]? - Zero or more related transactions to this Invoice object. The following linked relationships are supported- Links to Estimate and TimeActivity objects can be established directly to this Invoice object with UI or with the API. Create, Read, Update, and Query operations are avaialble at the API level for these types of links. Only one link can be made to an Estimate. Progress Invoicing is not supported via the API. Links to expenses incurred on behalf of the customer are returned in the response with LinkedTxn.TxnType set to ReimburseCharge, ChargeCredit or StatementCharge corresponding to billable customer expenses of type Cash, Delayed Credit, and Delayed Charge, respectively. Links to these types of transactions are established within the QuickBooks UI, only, and are available as read-only at the API level. Links to payments applied to an Invoice object are returned in the response with LinkedTxn.TxnType set to Payment. Links to Payment transactions are established within the QuickBooks UI, only, and are available as read-only at the API level. Use LinkedTxn.TxnId as the ID in a separate read request for the specific resource to retrieve details of the linked object.
- DepositToAccountRef ReferenceType? - Reference type
- GlobalTaxCalculation string? - Method in which tax is applied. Allowed values are- TaxExcluded, TaxInclusive, and NotApplicable.
- AllowOnlineACHPayment boolean? - Specifies if this invoice can be paid with online bank transfers and corresponds to the Free bank transfer online payment check box on the QuickBooks UI. Active when Preferences.SalesFormsPrefs.ETransactionPaymentEnabled is set to true. If set to true, allow invoice to be paid with online bank transfers. The Free bank transfer online payment check box is checked on the QuickBooks UI for this invoice. If set to false, online bank transfers are not allowed. The Free bank transfer online payment check box is not checked on the QuickBooks UI for this invoice.
- TransactionLocationType string? - The account location. For France locale valid values include- WithinFrance FranceOverseas OutsideFranceWithEU OutsideEU For UAE, valid values include ABUDHABI AJMAN SHARJAH DUBAI FUJAIRAH RAS_AL_KHAIMAH UMM_AL_QUWAIN OTHER_GCC
- DueDate string? - Date when the payment of the transaction is due. If date is not provided, the number of days specified in SalesTermRef added the transaction date will be used.
- MetaData ModificationMetaData? - Modification metadata
- PrivateNote string? - User entered, organization-private note about the transaction. This note does not appear on the invoice to the customer. This field maps to the Statement Memo field on the Invoice form in the QuickBooks Online UI.
- BillEmailCc EmailAddress? - Email address
- CustomerMemo MemoRef? -
- EmailStatus string? - Email status of the invoice. Valid values- NotSet, NeedToSend, EmailSent
- ExchangeRate decimal? - The number of home currency units it takes to equal one unit of currency specified by CurrencyRef. Applicable if multicurrency is enabled for the company.
- Deposit decimal? - The deposit made towards this invoice.
- TxnTaxDetail record {}? - This data type provides information for taxes charged on the transaction as a whole. It captures the details sales taxes calculated for the transaction based on the tax codes referenced by the transaction. This can be calculated by QuickBooks business logic or you may supply it when adding a transaction. See Global tax model for more information about this element. If sales tax is disabled (Preferences.TaxPrefs.UsingSalesTax is set to false) then TxnTaxDetail is ignored and not stored.
- AllowOnlineCreditCardPayment boolean? - Specifies if online credit card payments are allowed for this invoice and corresponds to the Cards online payment check box on the QuickBooks UI. Active when Preferences.SalesFormsPrefs.ETransactionPaymentEnabled is set to true. If set to true, allow invoice to be paid with online credit card payments. The Cards online payment check box is checked on the QuickBooks UI. If set to false, online credit card payments are not allowed. The Cards online payment check box is not checked on the QuickBooks UI.
- CustomField anydata[]? - One of, up to three custom fields for the transaction. Available for custom fields so configured for the company. Check Preferences.SalesFormsPrefs.CustomField and Preferences.VendorAndPurchasesPrefs.POCustomField for custom fields currenly configured. Click here to learn about managing custom fields.
- ShipAddr PhysicalAddress? - Physical address
- DepartmentRef ReferenceType? - Reference type
- BillEmailBcc EmailAddress? - Email address
- ShipMethodRef ReferenceType? - Reference type
- BillAddr PhysicalAddress? - Physical address
- ApplyTaxAfterDiscount boolean? - If false or null, calculate the sales tax first, and then apply the discount. If true, subtract the discount first and then calculate the sales tax.
- HomeBalance decimal? - Convenience field containing the amount in Balance expressed in terms of the home currency. Calculated by QuickBooks business logic. Value is valid only when CurrencyRef is specified and available when endpoint is evoked with the minorversion=3 query parameter. Applicable if multicurrency is enabled for the company
- DeliveryInfo record {}? - Email delivery information. Returned when a request has been made to deliver email with the send operation.
- TotalAmt decimal? - Indicates the total amount of the transaction. This includes the total of all the charges, allowances, and taxes. Calculated by QuickBooks business logic; any value you supply is over-written by QuickBooks.
- InvoiceLink string? - Sharable link for the invoice sent to external customers. The link is generated only for invoices with online payment enabled and having a valid customer email address. Include query param
include=invoiceLink
to get the link back on query response.
- RecurDataRef ReferenceType? - Reference type
- TaxExemptionRef ReferenceType? - Reference type
- Balance decimal? - The balance reflecting any payments made against the transaction. Initially set to the value of TotalAmt. A Balance of 0 indicates the invoice is fully paid. Calculated by QuickBooks business logic; any value you supply is over-written by QuickBooks.
- HomeTotalAmt decimal? - Total amount of the transaction in the home currency. Includes the total of all the charges, allowances and taxes. Calculated by QuickBooks business logic. Value is valid only when CurrencyRef is specified. Applicable if multicurrency is enabled for the company.
- FreeFormAddress boolean? - Denotes how ShipAddr is stored- formatted or unformatted. The value of this flag is system defined based on format of shipping address at object create time. If set to false, shipping address is returned in a formatted style using City, Country, CountrySubDivisionCode, Postal code. If set to true, shipping address is returned in an unformatted style using Line1 through Line5 attributes.
quickbooks.online: InvoiceCreateObject
Fields
- Id string? - Unique identifier for this object. Sort order is ASC by default.
- Line anydata[] - Individual line items of a transaction. Valid Line types include SalesItemLine, GroupLine, DescriptionOnlyLine (also used for inline Subtotal lines), DiscountLine and SubTotalLine (used for the overall transaction)
- CustomerRef ReferenceType - Reference type
- SyncToken string? - Version number of the object. It is used to lock an object for use by one app at a time. As soon as an application modifies an object, its SyncToken is incremented. Attempts to modify an object specifying an older SyncToken fails. Only the latest version of the object is maintained by QuickBooks Online.
- CurrencyRef CurrencyRefType? - Currency reference type
- DocNumber string? - Reference number for the transaction. If not explicitly provided at create time, this field is populated based on the setting of Preferences:CustomTxnNumber as follows- If Preferences:CustomTxnNumber is true a custom value can be provided. If no value is supplied, the resulting DocNumber is null. If Preferences:CustomTxnNumber is false, resulting DocNumber is system generated by incrementing the last number by 1. If Preferences:CustomTxnNumber is false then do not send a value as it can lead to unwanted duplicates. If a DocNumber value is sent for an Update operation, then it just updates that particular invoice and does not alter the internal system DocNumber. Note- DocNumber is an optional field for all locales except France. For France locale if Preferences:CustomTxnNumber is enabled it will not be automatically generated and is a required field. If a duplicate DocNumber needs to be supplied, add the query parameter name/value pair, include=allowduplicatedocnum to the URI.
- BillEmail EmailAddress? - Email address
- TxnDate string? - The date entered by the user when this transaction occurred. yyyy/MM/dd is the valid date format. For posting transactions, this is the posting date that affects the financial statements. If the date is not supplied, the current date on the server is used. Sort order is ASC by default.
- ShipFromAddr PhysicalAddress? - Physical address
- ShipDate string? - Date for delivery of goods or services.
- TrackingNum string? - Shipping provider's tracking number for the delivery of the goods associated with the transaction.
- ClassRef ReferenceType? - Reference type
- PrintStatus string? - Printing status of the invoice. Valid values- NotSet, NeedToPrint, PrintComplete .
- SalesTermRef ReferenceType? - Reference type
- TxnSource string? - Used internally to specify originating source of a credit card transaction.
- LinkedTxn anydata[]? - Zero or more related transactions to this Invoice object. The following linked relationships are supported- Links to Estimate and TimeActivity objects can be established directly to this Invoice object with UI or with the API. Create, Read, Update, and Query operations are avaialble at the API level for these types of links. Only one link can be made to an Estimate. Progress Invoicing is not supported via the API. Links to expenses incurred on behalf of the customer are returned in the response with LinkedTxn.TxnType set to ReimburseCharge, ChargeCredit or StatementCharge corresponding to billable customer expenses of type Cash, Delayed Credit, and Delayed Charge, respectively. Links to these types of transactions are established within the QuickBooks UI, only, and are available as read-only at the API level. Links to payments applied to an Invoice object are returned in the response with LinkedTxn.TxnType set to Payment. Links to Payment transactions are established within the QuickBooks UI, only, and are available as read-only at the API level. Use LinkedTxn.TxnId as the ID in a separate read request for the specific resource to retrieve details of the linked object.
- DepositToAccountRef ReferenceType? - Reference type
- GlobalTaxCalculation string? - Method in which tax is applied. Allowed values are- TaxExcluded, TaxInclusive, and NotApplicable.
- AllowOnlineACHPayment boolean? - Specifies if this invoice can be paid with online bank transfers and corresponds to the Free bank transfer online payment check box on the QuickBooks UI. Active when Preferences.SalesFormsPrefs.ETransactionPaymentEnabled is set to true. If set to true, allow invoice to be paid with online bank transfers. The Free bank transfer online payment check box is checked on the QuickBooks UI for this invoice. If set to false, online bank transfers are not allowed. The Free bank transfer online payment check box is not checked on the QuickBooks UI for this invoice.
- TransactionLocationType string? - The account location. For France locale valid values include- WithinFrance FranceOverseas OutsideFranceWithEU OutsideEU For UAE, valid values include ABUDHABI AJMAN SHARJAH DUBAI FUJAIRAH RAS_AL_KHAIMAH UMM_AL_QUWAIN OTHER_GCC
- DueDate string? - Date when the payment of the transaction is due. If date is not provided, the number of days specified in SalesTermRef added the transaction date will be used.
- MetaData ModificationMetaData? - Modification metadata
- PrivateNote string? - User entered, organization-private note about the transaction. This note does not appear on the invoice to the customer. This field maps to the Statement Memo field on the Invoice form in the QuickBooks Online UI.
- BillEmailCc EmailAddress? - Email address
- CustomerMemo MemoRef? -
- EmailStatus string? - Email status of the invoice. Valid values- NotSet, NeedToSend, EmailSent
- ExchangeRate decimal? - The number of home currency units it takes to equal one unit of currency specified by CurrencyRef. Applicable if multicurrency is enabled for the company.
- Deposit decimal? - The deposit made towards this invoice.
- TxnTaxDetail record {}? - This data type provides information for taxes charged on the transaction as a whole. It captures the details sales taxes calculated for the transaction based on the tax codes referenced by the transaction. This can be calculated by QuickBooks business logic or you may supply it when adding a transaction. See Global tax model for more information about this element. If sales tax is disabled (Preferences.TaxPrefs.UsingSalesTax is set to false) then TxnTaxDetail is ignored and not stored.
- AllowOnlineCreditCardPayment boolean? - Specifies if online credit card payments are allowed for this invoice and corresponds to the Cards online payment check box on the QuickBooks UI. Active when Preferences.SalesFormsPrefs.ETransactionPaymentEnabled is set to true. If set to true, allow invoice to be paid with online credit card payments. The Cards online payment check box is checked on the QuickBooks UI. If set to false, online credit card payments are not allowed. The Cards online payment check box is not checked on the QuickBooks UI.
- CustomField record {}? - One of, up to three custom fields for the transaction. Available for custom fields so configured for the company. Check Preferences.SalesFormsPrefs.CustomField and Preferences.VendorAndPurchasesPrefs.POCustomField for custom fields currenly configured. Click here to learn about managing custom fields.
- ShipAddr PhysicalAddress? - Physical address
- DepartmentRef ReferenceType? - Reference type
- BillEmailBcc EmailAddress? - Email address
- ShipMethodRef ReferenceType? - Reference type
- BillAddr PhysicalAddress? - Physical address
- ApplyTaxAfterDiscount boolean? - If false or null, calculate the sales tax first, and then apply the discount. If true, subtract the discount first and then calculate the sales tax.
- FreeFormAddress boolean? - Denotes how ShipAddr is stored- formatted or unformatted. The value of this flag is system defined based on format of shipping address at object create time. If set to false, shipping address is returned in a formatted style using City, Country, CountrySubDivisionCode, Postal code. If set to true, shipping address is returned in an unformatted style using Line1 through Line5 attributes.
quickbooks.online: InvoiceResponse
Fields
- Invoice Invoice? -
quickbooks.online: ItemBasedExpenseLine
Fields
- Id string? - The Id of the line item. Its use in requests is as folllows - - If Id is greater than zero and exists for the company, the request is considered an update operation for a line item. - If no Id is provided, the Idprovided is less than or equal to zero, or the Idprovided is greater than zero and does not exist for the company then the request is considered a create operation for a line item. - Available in all objects that use lines and support the update operation.
- Amount decimal? - The amount of the line item.
- DetailType string? - Set to ItemBasedExpenseLineDetail for this type of line.
- ItemBasedExpenseLineDetail ItemBasedExpenseLineDetail? - Item Based Expense Line Detail
- LinkedTxn LinkedTxn[]? - Zero or more PurchaseOrder transactions linked to this Bill object. The LinkedTxn.TxnType should always be set to PurchaseOrder. Use LinkedTxn.TxnId as the ID of the PurchaseOrder. When updating an existing Bill to link to a PurchaseOrder a new Line must be created. This behavior matches the QuickBooks UI as it does not allow the linking of an existing line, but rather a new line must be added to link the PurchaseOrder. Over the API this is achieved by simply updating the Bill Line.Id to something new. This will ensure old bill line is deleted and the new line is linked to the PurchaseOrder.
- Description string? - Free form text description of the line item that appears in the printed record.
- LineNum decimal? - Specifies the position of the line in the collection of transaction lines. Positive Integer.
quickbooks.online: ItemBasedExpenseLineDetail
Item Based Expense Line Detail
Fields
- TaxInclusiveAmt decimal? - The total amount of the line item including tax. Constraints- Available when endpoint is evoked with the minorversion=1query parameter.
- ItemRef ReferenceType? - Reference type
- CustomerRef ReferenceType? - Reference type
- PriceLevelRef ReferenceType? - Reference type
- ClassRef ReferenceType? - Reference type
- TaxCodeRef ReferenceType? - Reference type
- MarkupInfo record {}? - Reference to the TaxCodefor this item. Query the TaxCode name list resource to determine the appropriate TaxCode object for this reference. Use TaxCode.Id and TaxCode.Name from that object for TaxCodeRef.value and TaxCodeRef.name, respectively.
- BillableStatus string? - The billable status of the expense. Valid values- Billable, NotBillable, HasBeenBilled
- Qty decimal? - Number of items for the line.
- UnitPrice decimal? - Unit price of the subject item as referenced by ItemRef. Corresponds to the Rate column on the QuickBooks Online UI to specify either unit price, a discount, or a tax rate for item. If used for unit price, the monetary value of the service or product, as expressed in the home currency. If used for a discount or tax rate, express the percentage as a fraction. For example, specify 0.4 for 40% tax.
quickbooks.online: LinkedTxn
Fields
- TxnId string? - Transaction Id of the related transaction.
- TxnType string? - Transaction type of the linked object.
- TxnLineId string? - Required for Deposit and Bill entities. -The line number of a specific line of the linked transaction. - If supplied, the TxnId and TxnType attributes of the linked transaction must also be populated.
quickbooks.online: MemoRef
Fields
- value string? - User-entered message to the customer; this message is visible to the end user on their transactions.
quickbooks.online: ModificationMetaData
Modification metadata
Fields
- CreateTime string? - Time the entity was created in the source domain.
- LastUpdatedTime string? - Time the entity was last updated in the source domain.
quickbooks.online: OAuth2RefreshTokenGrantConfig
OAuth2 Refresh Token Grant Configs
Fields
- Fields Included from *OAuth2RefreshTokenGrantConfig
- refreshUrl string(default "https://oauth.platform.intuit.com/oauth2/v1/tokens/bearer") - Refresh URL
quickbooks.online: Payment
Fields
- Id string? - Unique identifier for this object. Sort order is ASC by default.
- TotalAmt decimal? - Indicates the total amount of the transaction. This includes the total of all the charges, allowances, and taxes.
- CustomerRef ReferenceType? - Reference type
- SyncToken string? - Version number of the object. It is used to lock an object for use by one app at a time. As soon as an application modifies an object, its SyncToken is incremented. Attempts to modify an object specifying an older SyncToken fails. Only the latest version of the object is maintained by QuickBooks Online.
- CurrencyRef CurrencyRefType? - Currency reference type
- PrivateNote string? - User entered, organization-private note about the transaction.
- PaymentMethodRef ReferenceType? - Reference type
- UnappliedAmt decimal? - Indicates the amount that has not been applied to pay amounts owed for sales transactions.
- DepositToAccountRef record {}? - Identifies the account to be used for this payment. Query the Account name list resource to determine the appropriate Account object for this reference, where Account.AccountType is Other Current Asset or Bank. Use Account.Id and Account.Name from that object for DepositToAccountRef.value and DepostiToAccountRef.name, respectively. If you do not specify this account, payment is applied to the Undeposited Funds account.
- ExchangeRate decimal? - The number of home currency units it takes to equal one unit of currency specified by CurrencyRef. Applicable if multicurrency is enabled for the company
- Line (ItemBasedExpenseLine|AccountBasedExpenseLine)[]? - Zero or more transactions accounting for this payment. Values for Line.LinkedTxn.TxnTypecan be one of the following- - Expense--Payment is reimbursement for expense paid by cash made on behalf of the customer - Check--Payment is reimbursement for expense paid by check made on behalf of the customer - CreditCardCredit--Payment is reimbursement for a credit card credit made on behalf of the customer - JournalEntry--Payment is linked to the representative journal entry - CreditMemo--Payment is linked to the credit memo the customer has with the business - Invoice--The invoice to which payment is applied - Use Line.LinkedTxn.TxnId as the ID in a separate read request for the specific resource to retrieve details of the linked object.
- TxnSource string? - Used internally to specify originating source of a credit card transaction.
- ARAccountRef ReferenceType? - Reference type
- TxnDate string? - The date entered by the user when this transaction occurred. For posting transactions, this is the posting date that affects the financial statements. If the date is not supplied, the current date on the server is used. Sort order is ASC by default.
- CreditCardPayment record {}? - Information about a payment received by credit card. Inject with data only if the payment was transacted through Intuit Payments API.
- TransactionLocationType string? - The account location. Valid values include- WithinFrance FranceOverseas OutsideFranceWithEU OutsideEU For France locales, only.
- MetaData ModificationMetaData? - Modification metadata
- PaymentRefNum string? - The reference number for the payment received. For example, Â Check number for a check, envelope number for a cash donation. Required for France locales.
- TaxExemptionRef ReferenceType? - Reference type
quickbooks.online: PaymentCreateObject
Fields
- Id string? - Unique identifier for this object. Sort order is ASC by default.
- TotalAmt decimal - Indicates the total amount of the transaction. This includes the total of all the charges, allowances, and taxes.
- CustomerRef ReferenceType - Reference type
- SyncToken string? - Version number of the object. It is used to lock an object for use by one app at a time. As soon as an application modifies an object, its SyncToken is incremented. Attempts to modify an object specifying an older SyncToken fails. Only the latest version of the object is maintained by QuickBooks Online.
- CurrencyRef CurrencyRefType? - Currency reference type
- PrivateNote string? - User entered, organization-private note about the transaction.
- PaymentMethodRef ReferenceType? - Reference type
- DepositToAccountRef record {}? - Identifies the account to be used for this payment. Query the Account name list resource to determine the appropriate Account object for this reference, where Account.AccountType is Other Current Asset or Bank. Use Account.Id and Account.Name from that object for DepositToAccountRef.value and DepostiToAccountRef.name, respectively. If you do not specify this account, payment is applied to the Undeposited Funds account.
- ExchangeRate decimal? - The number of home currency units it takes to equal one unit of currency specified by CurrencyRef. Applicable if multicurrency is enabled for the company
- Line (ItemBasedExpenseLine|AccountBasedExpenseLine)[]? - Zero or more transactions accounting for this payment. Values for Line.LinkedTxn.TxnTypecan be one of the following- - Expense--Payment is reimbursement for expense paid by cash made on behalf of the customer - Check--Payment is reimbursement for expense paid by check made on behalf of the customer - CreditCardCredit--Payment is reimbursement for a credit card credit made on behalf of the customer - JournalEntry--Payment is linked to the representative journal entry - CreditMemo--Payment is linked to the credit memo the customer has with the business - Invoice--The invoice to which payment is applied - Use Line.LinkedTxn.TxnId as the ID in a separate read request for the specific resource to retrieve details of the linked object.
- TxnSource string? - Used internally to specify originating source of a credit card transaction.
- ARAccountRef ReferenceType? - Reference type
- TxnDate string? - The date entered by the user when this transaction occurred. For posting transactions, this is the posting date that affects the financial statements. If the date is not supplied, the current date on the server is used. Sort order is ASC by default.
- CreditCardPayment record {}? - Information about a payment received by credit card. Inject with data only if the payment was transacted through Intuit Payments API.
- TransactionLocationType string? - The account location. Valid values include- WithinFrance FranceOverseas OutsideFranceWithEU OutsideEU For France locales, only.
- MetaData ModificationMetaData? - Modification metadata
- PaymentRefNum string? - The reference number for the payment received. For example, Â Check number for a check, envelope number for a cash donation. Required for France locales.
quickbooks.online: PaymentResponse
Fields
- Payment Payment? -
quickbooks.online: PhysicalAddress
Physical address
Fields
- Id string? - Unique identifier of the QuickBooks object for the address, used for modifying the address.
- PostalCode string? - Postal code. For example, zip code for USA and Canada
- City string? - City name.
- Country string? - Country name. For international addresses - countries should be passed as 3 ISO alpha-3 characters or the full name of the country.
- Line5 string? - Fifth line of the address.
- Line4 string? - Fourth line of the address.
- Line3 string? - Third line of the address.
- Line2 string? - Second line of the address.
- Line1 string? - First line of the address.
- Lat string? - Latitude coordinate of Geocode (Geospacial Entity Object Code). INVALIDis returned for invalid addresses.
- Long string? - Longitude coordinate of Geocode (Geospacial Entity Object Code). INVALIDis returned for invalid addresses.
- CountrySubDivisionCode string? - Region within a country. For example, state name for USA, province name for Canada.
quickbooks.online: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
quickbooks.online: ReferenceType
Reference type
Fields
- value string? - The ID for the referenced object as found in the Id field of the object payload. The context is set by the type of reference and is specific to the QuickBooks company file.
- name string? - An identifying name for the object being referenced by value and is derived from the field that holds the common name of that object. This varies by context and specific type of object referenced. For example, references to a Customer object use Customer.DisplayName to populate this field. Optionally returned in responses, implementation dependent.
quickbooks.online: TelephoneNumber
Telephone number
Fields
- FreeFormNumber string? - Specifies the telephone number in free form.
quickbooks.online: Vendor
Fields
- Id string? - Unique identifier for this object. Sort order is ASC by default.
- SyncToken string? - Version number of the object. It is used to lock an object for use by one app at a time. As soon as an application modifies an object, its SyncToken is incremented. Attempts to modify an object specifying an older SyncToken fails. Only the latest version of the object is maintained by QuickBooks Online.
- Title string? - Title of the person. This tag supports i18n, all locales. The DisplayName attribute or at least one of Title, GivenName, MiddleName, FamilyName, or Suffix attributes are required during create.
- GivenName string? - Given name or first name of a person. The DisplayName attribute or at least one of Title, GivenName, MiddleName, FamilyName, or Suffix attributes is required for object create.
- MiddleName string? - Middle name of the person. The person can have zero or more middle names. The DisplayName attribute or at least one of Title, GivenName, MiddleName, FamilyName, or Suffix attributes is required for object create.
- Suffix string? - Suffix of the name. For example, Jr. The DisplayName attribute or at least one of Title, GivenName, MiddleName, FamilyName, or Suffix attributes is required for object create.
- FamilyName string? - Family name or the last name of the person. The DisplayName attribute or at least one of Title, GivenName, MiddleName, FamilyName, or Suffix attributes is required for object create.
- PrimaryEmailAddr EmailAddress? - Email address
- DisplayName string? - The name of the vendor as displayed. Must be unique across all Vendor, Customer, and Employee objects. Cannot be removed with sparse update. If not supplied, the system generates DisplayName by concatenating vendor name components supplied in the request from the following list- Title, GivenName, MiddleName, FamilyName, and Suffix.
- OtherContactInfo record {}? - List of ContactInfo entities of any contact info type.
- APAccountRef ReferenceType? - Reference type
- TermRef ReferenceType? - Reference type
- Source string? - The Source type of the transactions created by QuickBooks Commerce. Valid values include- QBCommerce
- GSTIN string? - GSTIN is an identification number assigned to every GST registered business.
- T4AEligible string? - True if vendor is T4A eligible. Valid for CA locale
- Fax TelephoneNumber? - Telephone number
- BusinessNumber string? - Also called, PAN (in India) is a code that acts as an identification for individuals, families and corporates, especially for those who pay taxes on their income.
- CurrencyRef CurrencyRefType? - Currency reference type
- HasTPAR boolean? - Indicate if the vendor has TPAR enabled. TPAR stands for Taxable Payments Annual Report. The TPAR is mandated by ATO to get the details payments that businesses make to contractors for providing services. Some government entities also need to report the grants they have paid in a TPAR.
- TaxReportingBasis string? - The method in which the supplier tracks their income. Applicable for France companies, only. Available when endpoint is evoked with the minorversion=3 query parameter. Valid values include- Cash and Accrual.
- Mobile TelephoneNumber? - Telephone number
- PrimaryPhone TelephoneNumber? - Telephone number
- Active boolean? - If true, this object is currently enabled for use by QuickBooks.
- AlternatePhone TelephoneNumber? - Telephone number
- MetaData ModificationMetaData? - Modification metadata
- Vendor1099 boolean? - This vendor is an independent contractor; someone who is given a 1099-MISC form at the end of the year. A 1099 vendor is paid with regular checks, and taxes are not withheld on their behalf.
- BillRate decimal? - BillRate can be set to specify this vendor's hourly billing rate.
- WebAddr WebSiteAddress? - Website address
- T5018Eligible boolean? - True if vendor is T5018 eligible. Valid for CA locale
- CompanyName string? - The name of the company associated with the person or organization.
- VendorPaymentBankDetail record {}? - Vendor Payment Bank Detail.
- TaxIdentifier string? - The tax ID of the Person or Organization. The value is masked in responses, exposing only last four characters. For example, the ID of 123-45-6789 is returned as XXXXXXX6789.
- AcctNum string? - Name or number of the account associated with this vendor.
- GSTRegistrationType string? - For the filing of GSTR, transactions need to be classified depending on the type of vendor from whom the purchase is made. To facilitate this, we have introduced a new field as 'GST registration type'. Possible values are listed below- GST_REG_REG GST registered- Regular. Customer who has a business which is registered under GST and has a GSTIN (doesn’t include customers registered under composition scheme, as an SEZ or as EOU's, STP's EHTP's etc.). GST_REG_COMP GST registered-Composition. Customer who has a business which is registered under the composition scheme of GST and has a GSTIN. GST_UNREG GST unregistered. Customer who has a business which is not registered under GST and does not have a GSTIN. CONSUMER Consumer. Customer who is not registered under GST and is the final consumer of the service or product sold. OVERSEAS Overseas. Customer who has a business which is located out of India. SEZ SEZ. Customer who has a business which is registered under GST, has a GSTIN and is located in a SEZ or is a SEZ Developer. DEEMED Deemed exports- EOU's, STP's EHTP's etc. Customer who has a business which is registered under GST and falls in the category of companies (EOU's, STP's EHTP's etc.), to which supplies are made they are termed as deemed exports.
- PrintOnCheckName string? - Name of the person or organization as printed on a check. If not provided, this is populated from DisplayName. Cannot be removed with sparse update.
- BillAddr PhysicalAddress? - Physical address
- Balance decimal? - Specifies the open balance amount or the amount unpaid by the customer. For the create operation, this represents the opening balance for the customer. When returned in response to the query request it represents the current open balance (unpaid amount) for that customer. Write-on-create, read-only otherwise.
quickbooks.online: VendorCreateObject
Fields
- Id string? - Unique identifier for this object. Sort order is ASC by default.
- SyncToken string? - Version number of the object. It is used to lock an object for use by one app at a time. As soon as an application modifies an object, its SyncToken is incremented. Attempts to modify an object specifying an older SyncToken fails. Only the latest version of the object is maintained by QuickBooks Online.
- Title string? - Title of the person. This tag supports i18n, all locales. The DisplayName attribute or at least one of Title, GivenName, MiddleName, FamilyName, or Suffix attributes are required during create.
- GivenName string? - Given name or first name of a person. The DisplayName attribute or at least one of Title, GivenName, MiddleName, FamilyName, or Suffix attributes is required for object create.
- MiddleName string? - Middle name of the person. The person can have zero or more middle names. The DisplayName attribute or at least one of Title, GivenName, MiddleName, FamilyName, or Suffix attributes is required for object create.
- Suffix string? - Suffix of the name. For example, Jr. The DisplayName attribute or at least one of Title, GivenName, MiddleName, FamilyName, or Suffix attributes is required for object create.
- FamilyName string? - Family name or the last name of the person. The DisplayName attribute or at least one of Title, GivenName, MiddleName, FamilyName, or Suffix attributes is required for object create.
- PrimaryEmailAddr EmailAddress? - Email address
- DisplayName string? - The name of the vendor as displayed. Must be unique across all Vendor, Customer, and Employee objects. Cannot be removed with sparse update. If not supplied, the system generates DisplayName by concatenating vendor name components supplied in the request from the following list- Title, GivenName, MiddleName, FamilyName, and Suffix.
- OtherContactInfo record {}? - List of ContactInfo entities of any contact info type.
- APAccountRef ReferenceType? - Reference type
- TermRef ReferenceType? - Reference type
- Source string? - The Source type of the transactions created by QuickBooks Commerce. Valid values include- QBCommerce
- GSTIN string? - GSTIN is an identification number assigned to every GST registered business.
- T4AEligible string? - True if vendor is T4A eligible. Valid for CA locale
- Fax TelephoneNumber? - Telephone number
- BusinessNumber string? - Also called, PAN (in India) is a code that acts as an identification for individuals, families and corporates, especially for those who pay taxes on their income.
- HasTPAR boolean? - Indicate if the vendor has TPAR enabled. TPAR stands for Taxable Payments Annual Report. The TPAR is mandated by ATO to get the details payments that businesses make to contractors for providing services. Some government entities also need to report the grants they have paid in a TPAR.
- TaxReportingBasis string? - The method in which the supplier tracks their income. Applicable for France companies, only. Available when endpoint is evoked with the minorversion=3 query parameter. Valid values include- Cash and Accrual.
- Mobile TelephoneNumber? - Telephone number
- PrimaryPhone TelephoneNumber? - Telephone number
- Active boolean? - If true, this object is currently enabled for use by QuickBooks.
- AlternatePhone TelephoneNumber? - Telephone number
- MetaData ModificationMetaData? - Modification metadata
- Vendor1099 boolean? - This vendor is an independent contractor; someone who is given a 1099-MISC form at the end of the year. A 1099 vendor is paid with regular checks, and taxes are not withheld on their behalf.
- BillRate decimal? - BillRate can be set to specify this vendor's hourly billing rate.
- WebAddr WebSiteAddress? - Website address
- T5018Eligible boolean? - True if vendor is T5018 eligible. Valid for CA locale
- CompanyName string? - The name of the company associated with the person or organization.
- VendorPaymentBankDetail record {}? - Vendor Payment Bank Detail.
- TaxIdentifier string? - The tax ID of the Person or Organization. The value is masked in responses, exposing only last four characters. For example, the ID of 123-45-6789 is returned as XXXXXXX6789.
- AcctNum string? - Name or number of the account associated with this vendor.
- GSTRegistrationType string? - For the filing of GSTR, transactions need to be classified depending on the type of vendor from whom the purchase is made. To facilitate this, we have introduced a new field as 'GST registration type'. Possible values are listed below- GST_REG_REG GST registered- Regular. Customer who has a business which is registered under GST and has a GSTIN (doesn’t include customers registered under composition scheme, as an SEZ or as EOU's, STP's EHTP's etc.). GST_REG_COMP GST registered-Composition. Customer who has a business which is registered under the composition scheme of GST and has a GSTIN. GST_UNREG GST unregistered. Customer who has a business which is not registered under GST and does not have a GSTIN. CONSUMER Consumer. Customer who is not registered under GST and is the final consumer of the service or product sold. OVERSEAS Overseas. Customer who has a business which is located out of India. SEZ SEZ. Customer who has a business which is registered under GST, has a GSTIN and is located in a SEZ or is a SEZ Developer. DEEMED Deemed exports- EOU's, STP's EHTP's etc. Customer who has a business which is registered under GST and falls in the category of companies (EOU's, STP's EHTP's etc.), to which supplies are made they are termed as deemed exports.
- PrintOnCheckName string? - Name of the person or organization as printed on a check. If not provided, this is populated from DisplayName. Cannot be removed with sparse update.
- BillAddr PhysicalAddress? - Physical address
quickbooks.online: VendorResponse
Fields
- Vendor Vendor? -
quickbooks.online: WebSiteAddress
Website address
Fields
- URI string? - Uniform Resource Identifier for the web site.
Import
import ballerinax/quickbooks.online;
Metadata
Released date: about 2 years ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 1885
Current verison: 342
Weekly downloads
Keywords
Finance/Accounting
Cost/Paid
Contributors
Dependencies