pushcut
Module pushcut
API
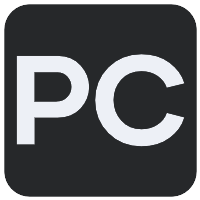
ballerinax/pushcut Ballerina library
Overview
This is a generated connector for Pushcut API v1 OpenAPI specification. API to send Pushcut notifications, execute Automation Server actions, and register custom webhooks as online actions.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Pushcut account
- Obtain tokens by following this guide
Quickstart
To use the Optirtc connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/pushcut
module into the Ballerina project.
import ballerinax/pushcut;
Step 2: Create a new connector instance
Create a pushcut:ApiKeysConfig
with the API key obtained, and initialize the connector with it.
pushcut:ApiKeysConfig config = { apiKey: "<API_KEY>" } pushcut:Client baseClient = check new Client(config);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to get a list of all active devices using the connector.
Gets a list of all active devices
public function main() returns error? { pushcut:InlineResponse200[] response = check baseClient->getDevices(); log:printInfo(response.toString()); }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
pushcut: Client
This is a generated connector for Pushcut API v1 OpenAPI specification. API to send Pushcut notifications, execute Automation Server actions, and register custom webhooks as online actions.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Pushcut account and obtain tokens by following this guide.
init (ApiKeysConfig apiKeyConfig, ConnectionConfig config, string serviceUrl)
- apiKeyConfig ApiKeysConfig - API keys for authorization
- config ConnectionConfig {} - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.pushcut.io/v1" - URL of the target service
getDevices
function getDevices() returns InlineResponse200[]|error
Get devices
Return Type
- InlineResponse200[]|error - Success
getNotifications
function getNotifications() returns InlineResponse2001[]|error
Get defined notifications
Return Type
- InlineResponse2001[]|error - Success
sendNotification
function sendNotification(string notificationName, Notification payload) returns Response|error
Send a smart notification
Parameters
- notificationName string - Notification Name
- payload Notification - Extend and customize the defined notification by providing dynamic content.
getSubscriptions
function getSubscriptions() returns SubscriptionData[]|error
Get a list of all online action subscriptions
Return Type
- SubscriptionData[]|error - Success
actionExecuted
function actionExecuted(Subscription payload) returns InlineResponse2002|error
Subscribe to an online action
Parameters
- payload Subscription - Subscription request
Return Type
- InlineResponse2002|error - Success
deleteSubscription
Remove an online action subscription
Parameters
- subscriptionId string - Id that was returned when creating the subscription.
execute
function execute(Execute payload, string? shortcut, string? homekit, string? timeout, string? delay, string? identifier) returns Response|error
Execute an Automation Server action.
Parameters
- payload Execute - Pass an input or optional configuration with the request.
- shortcut string? (default ()) - Shortcut
- homekit string? (default ()) - HomeKit scene
- timeout string? (default ()) - Timout in seconds, or 'nowait'
- delay string? (default ()) - Duration in which this request should be executed. Eg: 10s, 15m, 6h
- identifier string? (default ()) - Only used for delayed requests. Use an identifier to overwrite or cancel a scheduled execution.
cancelExecution
Cancel a scheduled Automation Server action.
Parameters
- identifier string? (default ()) - Identifier of the request.
Records
pushcut: ApiKeysConfig
Provides API key configurations needed when communicating with a remote HTTP endpoint.
Fields
- apiKey string - API-Keys can be managed in the Account view of the Pushcut app.
pushcut: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
pushcut: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
pushcut: EventData
Fields
- id string? - Unique id of trigger event
- actionName string? - Name of the triggered action.
- input string? - Input passed with the action.
- timestamp decimal? - Timestamp of the event
pushcut: Execute
Fields
- input string? - Value that is passed as input to the shortcut.
pushcut: GeneralError
Fields
- 'error string? - Error message
pushcut: InlineResponse200
Fields
- id string? - Name of the device
pushcut: InlineResponse2001
Fields
- id string? - Name of the notification definition
- title string? - Title of the notification
pushcut: InlineResponse2002
Fields
- id string? - ID of the subscription
pushcut: Notification
Fields
- text string? - Text that is used instead of the one defined in the app.
- title string? - Title that is used instead of the one defined in the app.
- input string? - Value that is passed as input to the notification action.
- defaultAction NotificationAction? -
- image string? - Name of importet image, or URL to an image. (https or local network)
- sound string? - Name of a sound that is used to the notification.
- actions NotificationAction[]? - List of dynamic actions that will added or merged with the static ones.
- devices string[]? - List of devices this notification is sent to. (default is all devices)
pushcut: NotificationAction
Fields
- name string - Name of the action.
- input string? - Value that will be passed as input to this action when executed.
- url string? - URL that will be opened.
- homekit string? - HomeKit scene that will be set.
- runOnServer boolean? - If true, run the Shortcut or HomeKit scenes on the Automation Server.
- online string? - Online Action that will be executed.
- urlBackgroundOptions NotificationactionUrlbackgroundoptions? - Configuration for a web request.
- shortcut string? - Shortcut that will be run.
- keepNotification boolean? - If true, this action will not dismiss the notification
pushcut: NotificationactionUrlbackgroundoptions
Configuration for a web request.
Fields
- httpMethod string? - HTTP Method (GET, POST, PUT)
- httpContentType string? - HTTP Content Type
- httpBody string? - Request body
- httpHeader NotificationactionUrlbackgroundoptionsHttpheader[]? - HTTP Headers
pushcut: NotificationactionUrlbackgroundoptionsHttpheader
Fields
- 'key string? -
- value string? -
pushcut: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
pushcut: Subscription
Fields
- actionName string - A unique name to identify the online action.
- url string - The URL to which EventData will be posted to.
- isLocalUrl boolean? - If set to true, the device will call the webhook on its local network.
pushcut: SubscriptionData
Fields
- id string? - ID of the subscription.
- serviceName string? - Name of the service that registered it.
- actionName string? - Identifier of the online action.
- url string? - URL of the registered webhook
- isLocalUrl boolean? - True if the URL should be triggered locally.
Import
import ballerinax/pushcut;
Metadata
Released date: about 2 years ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 5
Current verison: 5
Weekly downloads
Keywords
Internet of Things/Device Management
Cost/Paid
Contributors
Dependencies