prodpad
Module prodpad
API
Definitions
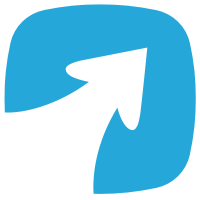
ballerinax/prodpad Ballerina library
Overview
This is a generated connector for ProdPad API v1.0 OpenAPI specification.
ProdPad is product management software that helps product managers develop product strategy. Easily manage teams, customers and roadmaps.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a ProdPad account
- Obtain token by following this guide.
Quickstart
To use the ProdPad connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/prodpad
module into the Ballerina project.
import ballerinax/prodpad;
Step 2: Create a new connector instance
Create a prodpad:ClientConfig
with the <API_KEY>
obtained, and initialize the connector with it.
prodpad:ClientConfig clientConfig = { auth: { token: <API_KEY> } }; prodpad:Client baseClient = check new Client(clientConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example to get a list of feedbacks using the connector.
public function main() { prodpad:FeedbackList|error response = baseClient->getFeedbacks(); if (response is prodpad:FeedbackList) { log:printInfo(response.toString()); } else { log:printError(response.message()); } }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
prodpad: Client
This is a generated connector for ProdPad API v1.0 OpenAPI specification. ProdPad is product management software that helps product managers develop product strategy. Easily manage teams, customers and roadmaps.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a ProdPad account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.prodpad.com/v1" - URL of the target service
getFeedbacks
function getFeedbacks(string? groupBy, string? state, int? page, int? size, string? company, string? companyCountry, string? companySize, string? companyValue, string? customer, string? product, string? persona, string? jobRole, string[]? tags, boolean? hasIdeas, string? externalId, string? externalUrl) returns FeedbackList|error
Get list of feedbacks
Parameters
- groupBy string? (default ()) - Setting to customer will group the feedback by customer
- state string? (default ()) - Set to value of active for active, archived for archived feedback, unsorted for unsorted feedback and all for all feedback. Default is active.
- page int? (default ()) - Set to page through the results. Default is 1.
- size int? (default ()) - For setting the number of results per page. Default is 100.
- company string? (default ()) - Set to filter the feedback results based on whether the feedback was entered for a contact linked to the company.
- companyCountry string? (default ()) - Set to filter the feedback results based on the country set for the associated company.
- companySize string? (default ()) - Set to filter the feedback results based on the size of the company for the associated company.
- companyValue string? (default ()) - Set to filter the feedback results based on the value of the company for the company assocaited to the feedback.
- customer string? (default ()) - Can be either numeric ID, UUID of a contac or contact email.
- product string? (default ()) - Filter results by the product associated to feedback. Can either be the product UUID or product ID.
- persona string? (default ()) - Filter results by the persona associated to feedback. Can either be the persona UUID or persona ID.
- jobRole string? (default ()) - Filter results by the job role of the contact associated to the feedback. Use the JobRole UUID.
- tags string[]? (default ()) - Filter feedback results by the tags associated to the feedback. Mulitple tags can be specified and acts as an OR. Use the tag ID or UUID.
- hasIdeas boolean? (default ()) - Whether the feedback is associated to one or more ideas. Default is either.
- externalId string? (default ()) - Filter feedback to return the feedback associated with a specific External ID. An example of an external ID is the ID of a record in a CRM or ID of a ticket in a customer support application.
- externalUrl string? (default ()) - Filter feedback to return the feedback associated with a specific external url. An example of an external url is that of a record in a CRM or a ticket in a customer support application
Return Type
- FeedbackList|error - Success response.
postFeedbacks
function postFeedbacks(FeedbackPost payload) returns ContactLinkWithFeedback|error
Create a new feedback.
Parameters
- payload FeedbackPost - Post body for the feedback.
Return Type
- ContactLinkWithFeedback|error - Success response.
getFeedback
function getFeedback(int id) returns ContactLinkWithFeedback|error
Get a piece of feedback.
Parameters
- id int - Feedback ID.
Return Type
- ContactLinkWithFeedback|error - Success response.
putFeedback
function putFeedback(int id, FeedbackPut payload) returns Response|error
Edit an existing piece of feedback.
getFeedbackIdeas
Get all ideas associated with a feedback
Parameters
- id int - Feedback ID
getContacts
function getContacts(string? company, string? persona, string? jobRole, string[]? tags, string? name, string? externalId, string? externalUrl, string? email, boolean feedbacks, int? page, int? size) returns ContactList|error
Get a list of contacts
Parameters
- company string? (default ()) - UUID of a company to filter contacts by.
- persona string? (default ()) - ID of a persona to filter contacts by.
- jobRole string? (default ()) - UUID of a job role to filter contacts by.
- tags string[]? (default ()) - ID, UUID or name of one or more tags to filter the contacts by.
- name string? (default ()) - Name of contact or partial name of contacts to filter the list by
- externalId string? (default ()) - Filter the contacts by an ID from another application such as a CRM
- externalUrl string? (default ()) - Filter the contacts by a URL from another application such as a CRM
- email string? (default ()) - Filter the contacts by an email.
- feedbacks boolean (default false) - Wheter to include the feedback for each contact in the results. Default is false.
- page int? (default ()) - Page number of results to return.
- size int? (default ()) - Number of results per page.
Return Type
- ContactList|error - Success response.
postContacts
function postContacts(ContactPost payload) returns Contact|error
Create a new contact
Parameters
- payload ContactPost - New contact details
getContact
function getContact(string id, boolean feedbacks) returns InlineResponse200|error
Get a contact
Parameters
- id string - Contact ID to fetch.
- feedbacks boolean (default true) - Whether to include the feedback provided by the contact in the response or not.
Return Type
- InlineResponse200|error - Success response.
putContact
function putContact(string id, ContactPost payload) returns Contact|error
Edit a contact.
getCompanies
function getCompanies(string? country, string? size, string? value, string? city, string[]? tags, string? name, string? externalId, string? externalUrl, boolean contacts, boolean feedbacks, int? page) returns CompanyList|error
Get a list of companies.
Parameters
- country string? (default ()) - Set to filter the companies based on the country. Use ISO Alpha-2 country codes. Only one country can be filtered at a time.
- size string? (default ()) - Set to filter the companies based on their size.
- value string? (default ()) - Set to filter the companies based on their value.
- city string? (default ()) - Set to filter the companies based on city.
- tags string[]? (default ()) - Filter companies by the tags associated to the feedback. Mulitple tags can be specified and acts as an OR. Use the tag ID or UUID.
- name string? (default ()) - Filter the companies by the name or partial name of the companies.
- externalId string? (default ()) - Filter the companies by an ID from a 3rd party application associated to the companies in Prodpad
- externalUrl string? (default ()) - Filter the companies by a URL that is associated to a company.
- contacts boolean (default true) - Whether to include contacts associated with each company in the results. Default is true.
- feedbacks boolean (default true) - Whether to include the feedback for each contact associated to a company in the results. Default is true. Note if this is set to true then contacts will be returned whether or not contacts is set to true or false.
- page int? (default ()) - The page of results to return (size is always 100).
Return Type
- CompanyList|error - Success response.
postCompanies
function postCompanies(CompanyPost payload) returns Company|error
Create a new company
Parameters
- payload CompanyPost - Post body request with the company details used to create a new company.
getCompany
Get a company
Parameters
- id string - UUID of the company to fetch.
putCompany
function putCompany(string id, CompanyPost payload) returns Company|error
Edit a company.
Parameters
- id string - UUID of the company to edit.
- payload CompanyPost - Changes to be made to a company.
getJobRoles
function getJobRoles() returns JobRoleList|error
Get the job roles for the account.
Return Type
- JobRoleList|error - Success response.
getIdeas
function getIdeas(string[]? tags, string? product, string? persona, string? status, string? state, string? externalId, string? externalUrl, boolean? withfeedback, int page, int size) returns IdeaList|error
Get a list of ideas.
Parameters
- tags string[]? (default ()) - One or more tag names to filter the ideas by. These act as an OR not AND.
- product string? (default ()) - Name of a product to filter the ideas by.
- persona string? (default ()) - Name of a persona to filter the ideas by.
- status string? (default ()) - Name of a workflow status to filter the ideas by.
- state string? (default ()) - Filters the returned ideas based on their state. Active Public is same as portal in the UI. If not included then the endpoint returns all active and active_public the same as the UI.
- externalId string? (default ()) - Return ideas or idea with a specific external_id.
- externalUrl string? (default ()) - Return ideas or idea with a specific URL to an external resource
- withfeedback boolean? (default ()) - Return the associated feedback to the ideas
- page int (default 1) - Page of results to return
- size int (default 20) - The number of results per page
postIdeas
Create a new idea.
Parameters
- payload IdeaPost - Idea to add to the account
getIdeaByID
function getIdeaByID(int id, boolean expand, boolean byProjectId) returns InlineResponse2001|error
Get an idea.
Parameters
- id int - Numeric ID of the idea unless using the by_project_id then it is the numeric project ID (the Idea number seen in the UI).
- expand boolean (default false) - Whether to return the expanded version of the idea ojbect. This adds in business case, user stories, comments, etc.
- byProjectId boolean (default false) - Whether the ID represents the project_id instead of the numeric ID.
Return Type
- InlineResponse2001|error - Success response.
putIdea
Edit an existing idea.
getIdeaVotes
function getIdeaVotes(int id) returns IdeaThoughts|error
Get thoughts on an idea
Parameters
- id int - Numeric ID of the idea.
Return Type
- IdeaThoughts|error - Success response.
postIdeaVotes
function postIdeaVotes(int id, ThoughtPost payload) returns ThoughtPostResponse|error
Add a new thought to ideas. This is an alias for POST /votes.
Return Type
- ThoughtPostResponse|error - Success response.
postIdeaStatus
function postIdeaStatus(int id, IdeaStatusChangePost payload) returns IdeaStatusChangeResponse|error
Update workflow status of idea.
Parameters
- id int - Numeric ID of the idea.
- payload IdeaStatusChangePost - New workflow status of the idea.
Return Type
- IdeaStatusChangeResponse|error - Success response.
getIdeaUserstories
Get a list of user stories associated to the idea.
Parameters
- id int - Numeric ID of the idea.
Return Type
- UserStories|error - Success response.
getIdeaComments
Get comments for an idea.
Parameters
- id int - Numeric ID of the idea.
Return Type
- CommentList|error - Success response.
putIdeaComment
function putIdeaComment(int id, int commentId) returns InlineResponse2002|error
Get a specific comment on an idea.
Return Type
- InlineResponse2002|error - Success response.
getIdeaFeedback
Get feedback associated to an idea.
Parameters
- id int - Numeric ID of the idea.
Return Type
- FeedbackList|error - Success response.
getIdeaRelatedIdeas
function getIdeaRelatedIdeas(int id) returns RelatedIdeas|error
Get ideas linked to an idea.
Parameters
- id int - Numeric ID of the idea.
Return Type
- RelatedIdeas|error - Success response.
getUserStories
function getUserStories() returns UserStoryList|error
Get the user stories in an account.
Return Type
- UserStoryList|error - Success response.
getThoughts
function getThoughts() returns ThoughtList|error
Get the thoughts.
Return Type
- ThoughtList|error - Success response.
postThoughts
function postThoughts(ThoughtPost payload) returns ThoughtPostResponse|error
Create a new thought on an idea.
Parameters
- payload ThoughtPost - New thought to be added
Return Type
- ThoughtPostResponse|error - Success response.
getPersonas
function getPersonas() returns PersonaList|error
Get a list of Personas
Return Type
- PersonaList|error - Success response.
getPersona
Get a persona.
Parameters
- id int - Numeric ID of the persona.
getProducts
function getProducts(boolean group) returns InlineResponse2003|error
List products.
Parameters
- group boolean (default false) - Whether the returned list is grouped by product lines or not.
Return Type
- InlineResponse2003|error - Success response.
getProduct
Get a product.
Parameters
- id int - Numeric ID of the product.
getProductRoadmap
Get a product roadmap
Parameters
- id int - Numeric ID of the product.
Return Type
- Roadmap|error - Success response.
getRoadmaps
function getRoadmaps() returns RoadmapList|error
Get a list of the roadmaps.
Return Type
- RoadmapList|error - Success response.
getRoadmap
Get an individual roadmap.
Parameters
- id int - Numeric ID of the roadmap.
Return Type
- Roadmap|error - Success response.
postRoadmapCard
function postRoadmapCard(int id, RoadmapCardPost payload) returns RoadmapCardPostResponse|error
Create roadmap card.
Return Type
- RoadmapCardPostResponse|error - Success response.
getRoadmapCard
function getRoadmapCard(int id, int cardid) returns RoadmapCardColumn|error
Get a roadmap card.
Return Type
- RoadmapCardColumn|error - Success response.
putRoadmapCard
function putRoadmapCard(int id, int cardid, RoadmapCardPost payload) returns RoadmapCardColumn|error
Edit a roadmap card.
Parameters
- id int - Numeric ID of the roadmap.
- cardid int - Numeric ID of the roadmap card.
- payload RoadmapCardPost - The card details to change.
Return Type
- RoadmapCardColumn|error - Success response.
getObjectives
function getObjectives() returns ObjectiveList|error
Get list of objectives.
Return Type
- ObjectiveList|error - Success response.
postObjectives
function postObjectives(ObjectivePost payload) returns Objective|error
Create a new objective.
Parameters
- payload ObjectivePost - Objective details.
getObjective
Get an objective.
Parameters
- id string - UUID of the objective.
putObjective
function putObjective(string id, ObjectivePost payload) returns Objective|error
Edit an objective.
Parameters
- id string - UUID of the objective.
- payload ObjectivePost - Details of objective to be changed.
getTags
function getTags() returns TagList|error
Get a list of tags.
Return Type
- TagList|error - Success response.
getTag
Get a tag.
Parameters
- id int - Numeric ID of the tag.
getStatuses
function getStatuses() returns StatusList|error
Get a list of workflow statuses.
Return Type
- StatusList|error - Success response.
getStatus
Get a workflow status.
Parameters
- id int - Numeric ID of the workflow status.
getSearch
Search across ideas, products, personas and feedback.
Parameters
- q string - Query to search for.
- page int? (default ()) - Page of results.
- size int? (default ()) - Number of entries per page of results.
- 'type string? (default ()) - Limit the search results to ideas, products, personas or feedback. Default is all.
Return Type
- SearchResults|error - Success response.
getUsers
function getUsers() returns UserList|error
Get a list of users.
Return Type
- UserList|error - Success response.
postUsers
Create a new user.
Parameters
- payload UserPost - User details.
getUser
Get a user.
Parameters
- id int - Numeric ID of the user.
Records
prodpad: AccountLink
Fields
- id int? - ID of the account.
- slug string? - Slug for the account (not used).
- name string? - The name of the account.
prodpad: Attachment
Fields
- id int? - ID of the file attachment.
- name string? - Name of the file attachment.
- url string? - Url to the file attachment.
- added string? - When the attachment was added to another object.
prodpad: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
prodpad: Comment
Fields
- id int? - ID of the comment.
- comment string? - The comment. This field can contain HTML markup and UTF-8 character codes.
- created_by UserLink? - User link information.
- created_at string? - When the comment was created.
- replies Comment[]? - Replies to the original comment.
prodpad: CommentWithDesign
Fields
- Fields Included from *Comment
- mockup CommentwithdesignMockup? -
prodpad: CommentwithdesignMockup
Fields
- id int? - ID of the mockup.
- name string? - Name of the mockup.
prodpad: CommentWithThought
Fields
- Fields Included from *Comment
- vote CommentwiththoughtVote? -
prodpad: CommentwiththoughtVote
Fields
- id string? - ID of the thought.
- vote string? - The thought associated to the comment.
- added_at string? - When the vote was added.
prodpad: Company
Fields
- id string? - UUID of the company.
- name string? - Name of the company.
- city string? - The city of the company.
- country string? - ISO Alpha-2 two letter country code.
- size string? - The number of employees.
- value string? - The value of the company.
- image string? - The URL to the logo/image that represents the company in the UI.
- tags TagLink[]? -
- external_links ExternalLink[]? -
- created_at string? - When the company was created.
- updated_at string? - When the company details were last updated.
prodpad: CompanyContact
Fields
- id string? - ID of the contact.
- name string? - Name of the contact.
- email string? - Email of the contact.
- about string? - Details about the contact. This field can contain HTML markup and UTF-8 character codes.
- phone string? - Contact's phone number.
- twitter_url string? - Contact's twitter handle
- image CompanycontactImage? - Photo or image of contact.
- created_at string? - When the contact was added to the account.
- updated_at string? - When the contact was last updated.
prodpad: CompanycontactImage
Photo or image of contact.
Fields
- original string? - URL to the original image of contact.
- large string? - Resized image of the contact.
prodpad: CompanyContactWithFeedback
Fields
- Fields Included from *CompanyContact
- feedbacks FeedbackLink[] -
prodpad: CompanyLink
Fields
- id string? - UUID of the company.
- name string? - Name of the company.
- city string? - City the company is in.
- country string? - The ISO Alpha-2 country code.
- size string? - The relative size of the company.
- value string? - The value of the company as a client.
- image string? - URL to the company logo.
- created_at string? - When when the company was added to the account.
- updated_at string? - When when the company details were last updated.
prodpad: CompanyList
Fields
- success string? - Whether the API call worked.
- company_count int? - Number of companies that match the filters.
- page int? - Page number of results.
- size int? - Number of items per page. Default is 100
- filters CompanylistFilters? -
- include CompanylistInclude? -
- companies (Company|CompanyWithContacts|CompanyWithContactsFeedback)[]? -
prodpad: CompanylistFilters
Fields
- tags string? - Comma separate list of tags filtered on.
- size string? - the size of the company filtered on.
- value string? - the value of the company filtered on.
- country string? - ISO Alpha-2 two letter country code filtered on.
- city string? - City filtered on.
prodpad: CompanylistInclude
Fields
- feedbacks boolean? - Whether the feedback associated to the company via the contacts associated to the company are included in the results.
- contacts boolean? - Whether the company contacts are included in the results.
prodpad: CompanyPost
Fields
- name string? - Name of the company.
- city string? - City the company is located in or you want to tag with.
- country string? - ISO Alpha-2 two letter country code.
- size string? - The size of the company by employees
- value string? - The value of the company.
- tags (TagIdPostLink|TagNamePostLink)[]? -
- external_links ExternalUrlPost[]? -
- contacts ContactIdPostLink[]? -
prodpad: CompanyWithContacts
Fields
- Fields Included from *Company
- contacts CompanyContact[] -
prodpad: CompanyWithContactsFeedback
Fields
- Fields Included from *Company
- contacts CompanyContactWithFeedback[] -
prodpad: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
prodpad: Contact
Fields
- id string? - ID of the contact.
- name string? - Name of the contact.
- email string? - Email of the contact.
- about string? - Details about the contact. This field can contain HTML markup and UTF-8 character codes.
- phone string? - Contact's phone number.
- twitter_url string? - Contact's twitter handle.
- image ContactImage? - Photo or image of contact
- job_role JobRoleLink? -
- company CompanyLink? -
- tags TagLink[]? -
- personas PersonaLink[]? -
- external_links ExternalLink[]? -
- created_at string? - When the contact was added to the account.
- updated_at string? - When the contact was last updated
prodpad: ContactIdPostLink
Fields
- id string? - ID of the contact
prodpad: ContactImage
Photo or image of contact
Fields
- original string? - URL to the original image of contact.
- large string? - Resized image of the contact.
prodpad: ContactLink
Fields
- id int? - ID of the contact.
- name string? - Name of the contact.
- email string? - Email of the contact.
- about string? - Details about the contact.
- company CompanyLink? -
- job_role JobRoleLink? -
- created_at string? - When the contact was added to the account.
- updated_at string? - When the contact was last updated.
prodpad: ContactLinkWithFeedback
Fields
- Fields Included from *ContactLink
- id int
- name string
- email string
- about string
- company CompanyLink|()
- job_role JobRoleLink|()
- created_at string
- updated_at string
- anydata...
- feedbacks Feedback[]? -
prodpad: ContactList
Fields
- success boolean? - Whether the fetch worked.
- contact_count string? - Number of contacts found.
- page int? - Which page of results.
- size int? - Number of results on each page.
- filters ContactlistFilters? -
- includes ContactlistIncludes? -
- contacts (Contact|ContactWithFeedback)[]? -
prodpad: ContactlistFilters
Fields
- tags string? - Comma separate list of tags filtered on.
- persona string? - Comma separated list of personas filtered on.
- company string? - ID of the company filtered on.
- job_role string? - ID of the job role filtered on.
prodpad: ContactlistIncludes
Fields
- feedbacks boolean? - Whether to return the feedbacks for each contact.
- numeric_id boolean? - Depreciated
prodpad: ContactPost
Fields
- name string? - Name of the contact.
- email string? - Can be email or other unique identifier. This is used to de-duplicate contacts.
- about string? - Details on the contact. This field accepts HTML and is stored as UTF-8.
- phone string? - Phone number of the contact.
- twitter_url string? - Twitter handle of the contact.
- tags (TagIdPostLink|TagNamePostLink)[]? -
- external_links ExternalUrlPost[]? -
- personas (PersonaIdPostLink|PersonaNamePostLink)[]? -
- company string? - UUID of the company to link the contact to. The UUID can be determined using /GET companies endpoint. Only used in the creation of a new contact.
- job_role string? - The UUID of the job role. The UUID can be /GET job_roles endpoint
prodpad: ContactWithFeedback
Fields
- Fields Included from *Contact
- id string
- name string
- email string
- about string|()
- phone string|()
- twitter_url string|()
- image ContactImage|()
- job_role JobRoleLink|()
- company CompanyLink|()
- tags TagLink[]
- personas PersonaLink[]
- external_links ExternalLink[]
- created_at string
- updated_at string
- anydata...
- feedbacks FeedbackLink[] -
prodpad: Design
A design or mockup.
Fields
- id int? - ID of the design.
- name string? - Name of the design.
- previous_versions DesignVersion[]? - List of previous versions of the design.
prodpad: DesignVersion
Previous version of a design.
Fields
- id int? - ID of the design version.
- name string? - Name of the design version.
- 'version string? - Version of the design.
prodpad: Error4xx
Fields
- success boolean? -
- developer_message string? - Error message for developers.
- user_message string? - Error message that can be displayed to users.
prodpad: ExternalLink
Fields
- id int? - ID of the external link.
- name string? - Name of the external link.
- url string? - The URL of the external link.
- external_id string? - An ID from another 3rd party application
- added string? - When the external link was added to another object.
prodpad: ExternalUrlIdeaPost
Fields
- title string - Name/title of the URL.
- url string - The URL of the external link.
prodpad: ExternalUrlPost
Fields
- name string - Name/title of the URL.
- url string - The URL of the external link.
- external_id string? - The ID for the object in a 3rd party application
prodpad: Feedback
Fields
- id int? - Feedback id.
- feedback string? - The feedback. This field can contain HTML markup and UTF-8 character codes.
- 'source string? - The source or channel that the feedback was received through.
- created_at string? - When the feedback was added to the account.
- updated_at string? - When the feedback was last updated.
- tags TagLink[]? - List of associated tags.
- ideas IdeaLinkWithAdded[]? - List of associated ideas.
- products ProductLink[]? - List of associated products.
- personas PersonaLink[]? - List of associated personas.
- attachments Attachment[]? - List of attachments.
- external_links ExternalLink[]? - List of external links.
prodpad: FeedbackAttachment
Fields
- id int? - Numeric id of the file attachment.
- file_id string? - UUID of the file attachment.
- url string? - URL to the file.
- filename string? - Name of the file attachment.
- extension string? - The file attachment extension.
- created_at string? - When the attachment was created.
- updated_at string? - When the attachment was last updated.
prodpad: FeedbackLink
Fields
- id int? - Feedback id.
- feedback string? - The feedback. This field can contain HTML markup and UTF-8 character codes.
- 'source string? - The source or channel that the feedback was received by.
- state string? - Whether the feedback is unsorted, active or archived.
- created_at string? - When the feedback was added to the account.
- updated_at string? - When the feedback was last updated.
prodpad: FeedbackListing
Fields
- Fields Included from *Feedback
- id int
- feedback string
- source string
- created_at string
- updated_at string
- tags TagLink[]
- ideas IdeaLinkWithAdded[]
- products ProductLink[]
- personas PersonaLink[]
- attachments Attachment[]
- external_links ExternalLink[]
- anydata...
- state string? - State of the feedback
- customer ContactLink? -
- added_by UserLink? - User link information.
prodpad: FeedbackPost
Fields to create a feedback. Note either the contact ID or contact name is required. Otherwise a 400 error will be returned. If a name and email field are used, the value in the email field will be used to see if the contact already exists and if it does the feedback will be automatically added to the existing contact.
Fields
- contact_id string? - ID of the contact providing the feedback. Either Contact ID or Contact name is required.
- name string? - Name of the contact providing the feedback. Either Contact ID or Contact name is required.
- company_id string? - UUID of the company to link the contact to. The UUID can be determined using /GET companies endpoint.
- feedback string - The feedback. This field accepts HTML and is stored as UTF-8.
- email string? - The email of the contact. This is used to avoid duplication of contacts. This can be any unique ID for each contact.
- about string? - Text field about the contact. This will overright the existing about if the contact already exists. This field accepts HTML and is stored as UTF-8.
- ideas IdeaPostLink[]? - Ihe one or more ideas associated to the feedback.
- tags (TagIdPostLink|TagNamePostLink)[]? - The tags associated to the feedback. Mulitple tags can be specified and acts as an OR. Use the tag ID or UUID.
- personas (PersonaIdPostLink|PersonaNamePostLink)[]? - The persona associated to feedback. Can either be the persona UUID or persona ID.
- products (ProductIdPostLink|ProductNamePostLink)[]? - The product associated to feedback. Can either be the product UUID or product ID.
- 'source string? - The source from where the feedback was gathered. If none is supplied the default is API.
- external_links ExternalUrlPost[]? - The external url associated with the feedback. An example of an external url is that of a record in a CRM or a ticket in a customer support application
prodpad: FeedbackPostLink
Fields
- id string - Numeric id of the feedback to link to an object when creating.
prodpad: FeedbackPut
Fields
- feedback string? - Update to the actual feedback. This field accepts HTML and is stored as UTF-8.
- state string? - The state of the feedback.
- external_links ExternalUrlPost[]? -
prodpad: File
A file.
Fields
- id int? - ID of the file.
- name string? - Name of the file.
prodpad: Idea
Fields
- id string? - Data store ID of the idea.
- project_id string? - The displayed ID for the idea in the UI.
- account AccountLink? -
- web_url string? - The URL to the idea in the ProdPad UI. Useful for providing a link to the original source in other applications.
- impact string? - The raw impact value.
- impact_scaled string? - The impact value in the scale.
- effort string? - the raw effort value.
- effort_scaled string? - The effort value in the scale.
- popularity int? - The raw popularity value.
- engagement int? - The raw engagement value.
- created_at string? - When the idea was added to the account.
- updated_at string? - When the idea was last updated.
- title string? - Title for the idea.
- description string? - The details of the idea. This field can contain HTML markup and UTF-8 character codes.
- state string? - State of the idea.
- creator UserLink? - User link information.
prodpad: IdeaCommentPost
Fields
- comment string? - The comment someone has made on the idea. This field accepts HTML and is stored as UTF-8.
- user_id int? - Numeric ID of the user in ProdPad who is making the comment.
prodpad: IdeaExpanded
Fields
- Fields Included from *Idea
- business_case IdeaexpandedBusinessCase? -
- functional_spec string? - The functional specs for this idea. It is a free text field. This field can contain HTML markup and UTF-8 character codes.
- notes string? - Notes on the idea. It is a free text field. This field can contain HTML markup and UTF-8 character codes.
- status Status? -
- owner UserLink? - User link information.
- author UserLink? - User link information.
- tags TagLink[]? - List of associated tags.
- products ProductLink[]? - List of associated products.
- personas PersonaLink[]? - List of associated personas.
- comments Comment[]? - List of comments made on the idea.
- userstories UserStory[]? - List of associated user stories.
- external_links ExternalLink[]? - List of associated external links.
- roadmap_cards RoadmapCard[]? - List of roadmap cards the idea is associated to.
- votes IdeaThoughts? - List of thoughts on an idea.
- mockups Design[]? - List of all the associated mockups and designs.
- files File[]? - List of files associated to the idea.
prodpad: IdeaexpandedBusinessCase
Fields
- problem string? - What problem is this idea solving. This field can contain HTML markup and UTF-8 character codes.
- value string? - What is the value of solving this problem. This field can contain HTML markup and UTF-8 character codes.
prodpad: IdeaLink
Fields
- id int? - ID of the idea.
prodpad: IdeaLinkPipeline
Fields
- id string? - ID of idea.
- title string? - Title of the idea.
- description string? - Description of the idea. This field can contain HTML markup and UTF-8 character codes.
- state string? - State of the idea.
- status StatusLink? -
- added string? - When the idea was added to roadmap card.
- 'order int? - Order of idea on the card.
prodpad: IdeaLinkWithAdded
Fields
- Fields Included from *IdeaLink
- id int
- anydata...
- added string? - When the idea was associated to the object.
prodpad: IdeaList
Fields
- success boolean? - Whether the call worked or not.
- idea_count int? - The number of ideas that match the filters.
- page int? - Page number of the results.
- size int? - The number of results per page.
- ideas Idea[]? -
prodpad: IdeaPost
Fields
- title string? - The title of the idea. Either the title or description is required.
- description string? - The description of the idea. This field accepts HTML and is stored as UTF-8.
- creator int? - Numeric ID of a user that has a role in the account.
- business_case IdeapostBusinessCase? -
- functional string? - The functional specs of how this idea could be implemented. This field accepts HTML and is stored as UTF-8.
- notes string? - Free text field for notes on the idea. This field accepts HTML and is stored as UTF-8.
- user_stories IdeaStoryPost[]? -
- comments IdeaCommentPost[]? -
- Feedbacks FeedbackPostLink[]? -
- products (ProductIdPostLink|ProductNamePostLink)[]? -
- personas (PersonaIdPostLink|PersonaNamePostLink)[]? -
- tags (TagIdPostLink|TagNamePostLink)[]? -
- external_links ExternalUrlIdeaPost[]? -
- status IdeapostStatus? -
- state string? - Set the state of the idea to active, archived or unsorted.
prodpad: IdeapostBusinessCase
Fields
- problem string? - The problem or hypothesis this idea is aiming to address. This field accepts HTML and is stored as UTF-8.
- value string? - The value of solving this problem or hypothesis to the user and the company. This field accepts HTML and is stored as UTF-8.
prodpad: IdeaPostLink
Fields
- id string - Numeric id of the idea to link to an object when creating.
prodpad: IdeapostStatus
Fields
- id int? - ID of the workflow status. This can be retrieved from GET /statuses.
prodpad: IdeaPut
Fields
- title string? - The title of the idea.
- description string? - The description of the idea. This field accepts HTML and is stored as UTF-8.
- business_case IdeaputBusinessCase? -
- functional string? - Functional specs for the idea. This field accepts HTML and is stored as UTF-8.
- notes string? - General notes on the idea. This field accepts HTML and is stored as UTF-8.
- state string? - The state of the idea.
prodpad: IdeaputBusinessCase
Fields
- problem string? - The problem or hypothesis this idea focuses on. This field accepts HTML and is stored as UTF-8.
- value string? - The value to user and company to address the problem. This field accepts HTML and is stored as UTF-8.
prodpad: IdeaStatusChangePost
Fields
- idea_id int? - Numeric ID of the idea to change the status on.
- user_id int? - Numeric ID of the user changing the workflow status of an idea.
- status_id int? - Numeric ID of the status to change the idea to. Can be found using GET /statuses.
prodpad: IdeaStatusChangeResponse
Fields
- id int? - Numeric ID of the idea
- statuses IdeastatuschangeresponseStatuses? -
- comments IdeastatuschangeresponseComments? - Any comment added when changing the status.
prodpad: IdeastatuschangeresponseComments
Any comment added when changing the status.
Fields
- id int? - ID of the comment.
- comment string? - The comment. This field may contain HTML and UTF-8 characters.
- created_at string? - When the comment was created.
prodpad: IdeastatuschangeresponseStatuses
Fields
- id int? - Numeric ID of the status.
- added string? - When the idea was switched to the workflow status.
prodpad: IdeaStoryPost
Fields
- story string? - The main body of a user story. This field accepts HTML and is stored as UTF-8.
- acceptance_criteria string? - The acceptance criteria for passing tests. This field accepts HTML and is stored as UTF-8.
prodpad: IdeaThoughts
List of thoughts on an idea.
Fields
- yea Thought[]? - All the users who indicated yea on the idea.
- nay Thought[]? - All the users who indicated nay on the idea.
- maybe Thought[]? - All the users who indicated maybe on the idea.
prodpad: JobRole
Fields
- id string? - UUID of the job role.
- name string? - Name of the job role.
- created_at string? - When the job role was added.
- updated_at string? - When the job role was last updated.
prodpad: JobRoleLink
Fields
- id string? - UUID of the job role.
- name string? - Name of job role.
prodpad: Objective
Fields
- id string? - UUID of objective.
- name string? - Objective name.
- state string? - Whether the objective is active or archived.
- product ObjectiveProduct? -
- created_at string? - When the objective was added.
- updated_at string? - When the objective was last updated.
prodpad: ObjectiveIdLink
Fields
- id string? - The UUID of the objective.
prodpad: ObjectiveLink
Fields
- id string? - UUID of objective.
- name string? - Name of objective.
- state string? - State of objective (active or archived).
- added string? - When the objective was linked.
prodpad: ObjectivePost
Fields
- name string? - Name of the objective
- state string? - The state of the objective
- product ObjectivepostProduct? -
prodpad: ObjectivepostProduct
Fields
- id int? - Numeric poduct ID to link the objective too.
prodpad: ObjectiveProduct
Fields
- id int? - The ID of the product the objective belongs too.
prodpad: Persona
Fields
- id string? - ID of the persona.
- persona_id string? - UUID of the persona.
- name string? - Persona name.
- description string? - Description of the persona. This field can contain HTML markup and UTF-8 character codes.
- image PersonaImage? -
- created_at string? - When the persona was created.
- updated_at string? - When the persona details were last updated.
- behaviours string? - Key behaviours of the persona. This field can contain HTML markup and UTF-8 character codes.
- goals string? - Key goals of the persona. This field can contain HTML markup and UTF-8 character codes.
- constraints string? - Key constraints of the persona. This field can contain HTML markup and UTF-8 character codes.
prodpad: PersonaIdPostLink
Fields
- id int - Numeric ID of the persona to link to an object when creating.
prodpad: PersonaImage
Fields
- original string? - URL to persona image.
prodpad: PersonaLink
Fields
- id int? - ID of the persona.
- persona string? - name of the persona.
- added string? - When the presona was linked to the object.
prodpad: PersonaListing
Fields
- id string? - ID of the persona.
- persona_id string? - UUID of the persona.
- name string? - Persona name.
- description string? - Description of the persona. This field can contain HTML markup and UTF-8 character codes.
- image PersonalistingImage? -
- created_at string? - When the persona was created.
- updated_at string? - When the persona details were last updated.
prodpad: PersonalistingImage
Fields
- original string? - URL of the persona image.
prodpad: PersonaNamePostLink
Fields
- name string - Name of the persona to link to an object when creating. It must match exactly.
prodpad: Product
Fields
- id int? - ID of product.
- name string? - Name of product.
- description string? - Description of the product. This field can contain HTML markup and UTF-8 character codes.
- vision string? - Vision for the product. This field can contain HTML markup and UTF-8 character codes.
- kpis string? - KPIs for the product. This field can contain HTML markup and UTF-8 character codes.
- value string? - Value of the product to end users and the business. This field can contain HTML markup and UTF-8 character codes.
- documentation string? - Documentation related to the product. This field can contain HTML markup and UTF-8 character codes.
- image ProductImage? -
- roadmaps ProductRoadmaps? -
- created_at string? - When the product was created.
- updated_at string? - When the product details were last updatd.
prodpad: ProductIdPostLink
Fields
- id int - Numeric ID of the product to link to an object when creating.
prodpad: ProductImage
Fields
- original string? - URL of the product image.
prodpad: Productline
Fields
- id string? - ID of the product line.
- name string? - Name of the product line.
- created_at string? - When the product line was created.
- products ProductListing[]? -
prodpad: ProductlineLink
Fields
- id int? - ID of productline.
- name string? - Name of productline.
- roadmaps RoadmapLink[]? -
- created_at string? - When the product line was created.
prodpad: ProductLink
Fields
- id int? - ID of the product.
- product string? - Name of the product.
- added string? - When the product was linked to the other object
prodpad: ProductListGroup
Fields
- productlines Productline[]? -
- products ProductListing[]? -
- product_count string? - Count of products.
prodpad: ProductListing
Fields
- id int? - Numeric product ID.
- product_id string? - UUID of the product.
- name string? - Name of the product.
- description string? - Description of the product. This field can contain HTML markup and UTF-8 character codes.
- image ProductImage? -
- roadmaps ProductlistingRoadmaps? -
- productlines ProductlistingProductlines? -
- 'order string? - Order of product in portfolio .
- created_at string? - When the product was created.
- updated_at string? - When the product details were last updated.
prodpad: ProductlistingProductlines
Fields
- id int? - ID of productline a product is in.
- name string? - Name of product line.
- 'order string? - Order of the product in the product line.
prodpad: ProductlistingRoadmaps
Fields
- id int? - ID of the product roadmap.
prodpad: ProductNamePostLink
Fields
- name string - Name of the product to link to an object when creating. It must match exactly.
prodpad: ProductRoadmaps
Fields
- id string? - ID of the product roadmap.
prodpad: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
prodpad: RelatedIdeas
Fields
- related Idea[]? -
- duplicate Idea[]? -
prodpad: RoadmapCard
Fields
- id int? - ID of the Roamdap card.
- title string? - Title of the roadmap card.
- description string? - Roadmap card description. This field can contain HTML markup and UTF-8 character codes.
- column RoadmapcardColumn? -
- roadmap RoadmapcardRoadmap? -
prodpad: RoadmapCardColumn
Fields
- id string? - ID of roadmap card.
- numeric_product_id string? - ID of product.
- title string? - Title of roadmap card.
- description string? - Description of roadmap card. This field can contain HTML markup and UTF-8 character codes.
- 'public string? - Whether the roadmap card is visible on embedded roadmaps.
- position string? - Position in the roadmap colum. 0 is top.
- effort int? - Raw effort score.
- effort_scaled string? - Effort value on the scale.
- impact int? - Raw impact score.
- impact_scaled string? - Impact value on the scale.
- status string? - Current status.
- status_raw int? - Raw status value.
- state string? - Current state of roadmap card.
- state_raw int? - Raw state value.
- created_at string? - When the card was created.
- updated_at string? - When the card was last updated.
- tags TagLink[]? -
- objectives ObjectiveLink[]? -
- owners UserLink[]? -
- pipeline RoadmapcardcolumnPipeline? -
prodpad: RoadmapcardColumn
Fields
- id int? - ID of the column.
- title string? - Title of the roadmap column.
- column_number string? - Number of the column on the roadmap.
prodpad: RoadmapcardcolumnPipeline
Fields
- ideas IdeaLinkPipeline[]? -
- specs SpecLinkPipeline[]? -
prodpad: RoadmapCardPost
Fields
- title string? - The title of the roadmap card.
- roadmap_id int? - Numeric roadmap ID.
- column_id int? - Numeric column ID. This can be found using GET /roadmaps/{id}.
- description string? - The description of the problem(s) and/or hypothesis this card is about. This field accepts HTML and is stored as UTF-8.
- tags (TagIdPostLink|TagNamePostLink)[]? -
- objectives RoadmapcardpostObjectives[]? -
prodpad: RoadmapcardpostObjectives
Fields
- id string? - UUID of the objective to link to the roadmap card.
prodpad: RoadmapCardPostResponse
Fields
- Fields Included from *RoadmapCard
- id int
- title string
- description string
- column RoadmapcardColumn
- roadmap RoadmapcardRoadmap
- anydata...
- account RoadmapcardpostresponseAccount? -
- product RoadmapcardpostresponseProduct? -
- tags TagLink[]? -
- ojectives ObjectiveIdLink[]? -
prodpad: RoadmapcardpostresponseAccount
Fields
- id string? - Account ID for the roadmap card.
prodpad: RoadmapcardpostresponseProduct
Fields
- id int? - ID of the product that the roadmap belongs to.
prodpad: RoadmapcardRoadmap
Fields
- id string? - ID of the roadmap.
prodpad: RoadmapColumn
Fields
- id int? - ID of the roadmap column.
- title string? - Column title.
- cards RoadmapCardColumn[]? -
- column_number int? - Column position on the roadmap.
- products RoadmapcolumnProducts? -
- created_at string? - When the column was created.
- updated_at string? - When the column details were last updated.
prodpad: RoadmapcolumnProducts
Fields
- id int? - Product ID.
prodpad: RoadmapLink
Fields
- id string? - ID of roadmap.
- name string? - Name of roadmap.
- products RoadmaplinkProducts? -
- created_at string? - When the roadmap was created.
prodpad: RoadmaplinkProducts
Fields
- id int? - ID of the associated product.
prodpad: RoadmapList
Fields
- roadmap_count int? - Number of roadmaps.
- productline_roadmaps ProductlineLink[]? -
- roadmaps RoadmapLink[]? -
prodpad: SearchFeedback
Fields
- id int? - ID of the feedback.
- feedback string? - The feedback. This field can contain HTML markup and UTF-8 character codes.
- customer SearchfeedbackCustomer? -
prodpad: SearchfeedbackCustomer
Fields
- id int? - The contact ID.
- name string? - The contact Name.
prodpad: SearchIdea
Search idea
Fields
- id int? - ID of the idea.
- project_id int? - The displayed ID of the idea.
- title string? - Title of the idea.
- summary string? - The description of the idea. This field can contain HTML markup and UTF-8 character codes.
prodpad: SearchPersona
Fields
- id int? - ID of the persona.
- name string? - Name of the persona.
prodpad: SearchProduct
Fields
- id int? - ID of the product.
- name string? - Name of the product.
prodpad: SearchResults
Fields
- query string? - The query that was searched on.
- filter record {}? - The filters applied.
- page int? - The page number of results.
- size int? - The number of results per page.
- ideas SearchresultsIdeas? - The list of ideas that match the query and filters.
- products SearchresultsProducts? - The list of products that match the query and filters.
- personas SearchresultsPersonas? - The list of personas of that match the query and filters.
- feedback SearchresultsFeedback? - The feedback that matches the query and filters.
prodpad: SearchresultsFeedback
The feedback that matches the query and filters.
Fields
- count int? - The count of pieces of feedback that match the query and filters.
- results SearchFeedback[]? - Search feedback
prodpad: SearchresultsIdeas
The list of ideas that match the query and filters.
Fields
- count int? - Count of ideas that match the query and filters.
- results SearchIdea[]? - An array of search ideas.
prodpad: SearchresultsPersonas
The list of personas of that match the query and filters.
Fields
- count int? - The count of personas that match the query and filters.
- results SearchPersona[]? - Search persona result.
prodpad: SearchresultsProducts
The list of products that match the query and filters.
Fields
- count int? - Count of products that match the query and filters.
- results SearchProduct[]? - Searched product result.
prodpad: SpecLinkPipeline
Fields
- id string? - ID of the user story.
- story string? - The user story. This field can contain HTML markup and UTF-8 character codes.
- acceptance_critiera string? - Acceptance criteria for the story. This field can contain HTML markup and UTF-8 character codes.
- added string? - When the user story was added to the roadmap card.
- 'order int? - Order of the user story on the card.
prodpad: Status
Fields
- id int? - ID of the status.
- status string? - The status name.
- state string? - Whether the status is active or archived.
- created_at string? - When the status was added.
- updated_at string? - When the status was updated.
prodpad: StatusLink
Fields
- id int? - ID of the status.
- status string? - Status name.
prodpad: StatusListing
Fields
- id int? - ID of the status.
- status string? - The status name.
- created_at string? - When the status was added.
- updated_at string? - When the status was updated.
prodpad: Tag
Fields
- id string? - ID of the tag.
- tag string? - Tag name.
- created_at string? - When the tag was created.
- updated_at string? - When the tag was last updated.
prodpad: TagIdPostLink
Fields
- id string - Numeric id of the tag to link to an object when creating.
prodpad: TagLink
Fields
- id string? - ID of the tag.
- tag string? - Tag name.
- added string? - Date time when the tag was added to the object.
prodpad: TagNamePostLink
Fields
- name string - Name of the tag to link to an object when creating.
prodpad: Thought
Quick yay, nay or maybe on an idea.
Fields
- id int? - ID of the thought.
- voter UserLink? - User link information.
- comments ThoughtComments? - Comment
- created_at string? - When the thought was added.
prodpad: ThoughtComments
Comment
Fields
- id int? - ID of the comment associated to the thought.
- comment string? - The comment with the though. This field can contain HTML markup and UTF-8 character codes.
prodpad: ThoughtList
List of all thoughts made in an account.
Fields
- total_votes string? - Total number of votes.
- yea ThoughtWithIdea[]? - All the users who indicated yea on the idea.
- nay ThoughtWithIdea[]? - All the users who indicated nay on the idea.
- maybe ThoughtWithIdea[]? - All the users who indicated maybe on the idea.
prodpad: ThoughtPost
Fields
- vote string? - The thought type.
- idea_id int? - Numeric ID of the idea to associate the vote too. This can be retrieved from GET /ideas
- user_id int? - Numeric ID of the user with a role in the account who is making the thought.
- comment string? - Comment made when adding the thought. This field accepts HTML and is stored as UTF-8.
prodpad: ThoughtPostResponse
Fields
- id int? - Numeric ID of the thought
- vote string? - The thought type
- ideas IdeaLink? -
- comments ThoughtpostresponseComments? -
- created_at string? - When the thought was created
- updated_at string? - When the thought was last updated.
prodpad: ThoughtpostresponseComments
Fields
- id int? - ID of the comment associated to the thought.
- ideas IdeaLink? -
- comment string? - The comment with the though. May include HTML and UTF-8 characters.
- created_at string? - When the comment was created.
prodpad: ThoughtWithIdea
Fields
- Fields Included from *Thought
- id int
- voter UserLink|()
- comments ThoughtComments
- created_at string
- anydata...
- ideas IdeaLink -
prodpad: User
Fields
- id int? - ID of user.
- username string? - Username of user. (depreciated and not used)
- display_name string? - Displayable name for the user.
- email string? - Email of user.
- role_id string? - Role ID.
- role_type string? - The role type of the user.
- image UserImage? -
prodpad: UserImage
Fields
- original string? - URL to user image.
prodpad: UserLink
User link information.
Fields
- id int? - Numeric ID of the user.
- username string? - User name of the user.
- display_name string? - Name of the user that can be shown in an UI.
prodpad: UserPost
Fields
- email string? - The email of the user.
- role_type string? - Role of the user in the account.
- name string? - The name to display for the user in the UI.
- timezone string? - Timezone of the user using the format like Europe/London.
prodpad: UserStory
Fields
- id int? - ID of the user story.
- title string? - Title of the user story.
- story string? - The details of the user story. This field can contain HTML markup and UTF-8 character codes.
- acceptance_criteria string? - The acceptance criteria that indicate the user story has been achieved. This field can contain HTML markup and UTF-8 character codes.
- created_at string? - When the user story was created.
- updated_at string? - When the user story was last updated.
prodpad: UserStoryWithIdea
Fields
- Fields Included from *UserStory
- ideas IdeaLink? -
Union types
prodpad: InlineResponse200
InlineResponse200
prodpad: InlineResponse2001
InlineResponse2001
prodpad: InlineResponse2002
InlineResponse2002
prodpad: InlineResponse2003
InlineResponse2003
Import
import ballerinax/prodpad;
Metadata
Released date: about 2 years ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 5
Current verison: 5
Weekly downloads
Keywords
Productivity/Product Management
Cost/Paid
Contributors