powertoolsdeveloper.weather
Module powertoolsdeveloper.weather
API
Definitions
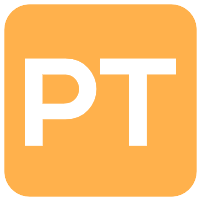
ballerinax/powertoolsdeveloper.weather Ballerina library
Overview
This is a generated connector from Apptigent PowerTools Developer API v2021.3.01 OpenAPI specification. Apptigent PowerTools Developer Edition is a powerful suite of API endpoints for custom applications running on any stack. Manipulate text, modify collections, format dates and times, convert currency, perform advanced mathematical calculations, shorten URL's, encode strings, convert text to speech, translate content into multiple languages, process images, and more. PowerTools is the ultimate developer toolkit. This connector provides the capability for weather conditions, forecasts and related operations.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create Apptigent account
- Obtain tokens
- Log into Apptigent Developer Portal by visiting https://portal.apptigent.com
- Create an app and obtain the
Client ID
which will be used as theAPI Key
by following the guidelines described here.
Quickstart
To use the Apptigent PowerTools Developer connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
Import the ballerinax/powertoolsdeveloper.weather
module into the Ballerina project.
import ballerinax/powertoolsdeveloper.weather as pw;
Step 2: Create a new connector instance
Create a text:ApiKeysConfig
with the Client ID obtained, and initialize the connector with it.
pw:ApiKeysConfig config = { xIbmClientId: "<CLIENT_ID>" } pw:Client baseClient = check new Client(config);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to get current weather conditions near an address or location using the connector.
Get current weather conditions near an address or location
public function main() returns error? { pw:InputWeatherConditions inputWeatherConditions = { 'source: "<Full or partial address>", units: "<Units>" }; pw:OutputWeatherConditions response = check baseClient->weatherConditions(inputWeatherConditions); log:printInfo(response.toString()); }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
powertoolsdeveloper.weather: Client
This is a generated connector for Apptigent PowerTools Developer API v2021.3.01 OpenAPI specification. 'Apptigent PowerTools Developer Edition is a powerful suite of API endpoints for custom applications running on any stack. Manipulate text, modify collections, format dates and times, convert currency, perform advanced mathematical calculations, shorten URL's, encode strings, convert text to speech, translate content into multiple languages, process images, and more. PowerTools is the ultimate developer toolkit.' This connector provides the capability for weather conditions, forecasts and related operations.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Apptigent account and obtain tokens by following this guide.
init (ApiKeysConfig apiKeyConfig, ConnectionConfig config, string serviceUrl)
- apiKeyConfig ApiKeysConfig - API keys for authorization
- config ConnectionConfig {} - The configurations to be used when initializing the
connector
- serviceUrl string "https://connect.apptigent.com/api/utilities" - URL of the target service
weatherConditions
function weatherConditions(InputWeatherConditions payload) returns OutputWeatherConditions|error
Weather - Weather Conditions
Parameters
- payload InputWeatherConditions - Input weather conditions
Return Type
weatherDailyForecast
function weatherDailyForecast(InputWeatherDailyForecast payload) returns OutputDailyForecast|error
Weather - Daily Forecast
Parameters
- payload InputWeatherDailyForecast - Input weather daily forecast
Return Type
- OutputDailyForecast|error - OK
weatherHourlyForecast
function weatherHourlyForecast(InputWeatherHourlyForecast payload) returns OutputHourlyForecast|error
Weather - Hourly Forecast
Parameters
- payload InputWeatherHourlyForecast - Input weather hourly forecast
Return Type
weatherActivityForecast
function weatherActivityForecast(InputWeatherActivityForecast payload) returns OutputActivityForecast|error
Weather - Activity Forecast
Parameters
- payload InputWeatherActivityForecast - Input weather activity forecast
Return Type
Records
powertoolsdeveloper.weather: ApiKeysConfig
Provides API key configurations needed when communicating with a remote HTTP endpoint.
Fields
- xIbmClientId string - Represents API Key
X-IBM-Client-Id
powertoolsdeveloper.weather: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
powertoolsdeveloper.weather: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
powertoolsdeveloper.weather: InputCalculateMinMax
Fields
- input decimal[] - Colllection of values to calculate
- 'type string - Minimum or Maximum
powertoolsdeveloper.weather: InputCalculateNumber
Fields
- input decimal - Numeric value to calculate
- decimals decimal - Round to number of decimal places
powertoolsdeveloper.weather: InputCalculateNumbers
Fields
- input decimal - Numeric value
- value decimal - Addend, subtrahend, factor, divisor or radicand
- decimals decimal - Round to number of decimal places
powertoolsdeveloper.weather: InputCalculatePower
Fields
- input decimal - Number to raise
- power decimal - Power
- decimals decimal - Round to number of decimal places
powertoolsdeveloper.weather: InputCalculateSeries
Fields
- input decimal[] - Colllection of values to calculate
- decimals decimal - Round to number of decimal places
powertoolsdeveloper.weather: InputCaseConversion
Fields
- input string - String containing the text to convert
- alphacase string - Case of conversion result
powertoolsdeveloper.weather: InputCollectionConversion
Fields
- input string[] - Collection containing strings to convert
- name string - Collection name
powertoolsdeveloper.weather: InputCollectionConversionXML
Fields
- input string[] - Collection containing strings to convert
- root string - Name of root XML node
- child string - Name of child XML node(s)
powertoolsdeveloper.weather: InputCollectionCount
Fields
- input string[] - Collection of items to count
powertoolsdeveloper.weather: InputCollectionFilter
Fields
- input string[] - Collection of strings to filter
- 'match string - Match type
- keywords string - Keywords (separate multiple values with commas)
powertoolsdeveloper.weather: InputCollectionModify
Fields
- input string[] - Collection of values or objects to modify
- item string? - Item (for multiple items, leave blank and use Items)
- items string[]? - Items (Collection, for a single item leave blank and use Item)
- index string? - Index position for operation (leave blank to specify end of collection)
powertoolsdeveloper.weather: InputCollectionReplace
Fields
- input string[] - Collection of strings
- 'match string - Match value
- replacement string - Replacement value
- ignoreCase string - Ignore case
powertoolsdeveloper.weather: InputCollectionSearch
Fields
- input string[] - Collection of strings to search
- 'match string - Text to match
- trim string? - Trim white space from comparison string
- ignorecase string? - Ignore case when performing comparison
powertoolsdeveloper.weather: InputCollectionSearchNumeric
Fields
- input decimal[] - Collection of strings to search
- 'match decimal - Number to match
- 'type string(default "Integer") - Type of number - integer or decimal
powertoolsdeveloper.weather: InputCollectionSort
Fields
- input string[] - Collection of strings to sort
- 'order string - Sort order
powertoolsdeveloper.weather: InputCollectionSplit
Fields
- input string[] - Collection of items to split
- 'match string? - String to match (explicit, case-insensitive, leave empty to use Index)
- index string? - Index location to split (leave empty to use Match value)
powertoolsdeveloper.weather: InputConvertAngle
Fields
- input decimal -
- 'source string -
- target string -
powertoolsdeveloper.weather: InputConvertArea
Fields
- input decimal -
- 'source string -
- target string -
powertoolsdeveloper.weather: InputConvertDistance
Fields
- input decimal -
- 'source string -
- target string -
powertoolsdeveloper.weather: InputConvertDuration
Fields
- input decimal -
- 'source string -
- target string -
powertoolsdeveloper.weather: InputConvertEnergy
Fields
- input decimal -
- 'source string -
- target string -
powertoolsdeveloper.weather: InputConvertPower
Fields
- input decimal -
- 'source string -
- target string -
powertoolsdeveloper.weather: InputConvertSpeed
Fields
- input decimal -
- 'source string -
- target string -
powertoolsdeveloper.weather: InputConvertTemperature
Fields
- input decimal -
- 'source string -
- target string -
powertoolsdeveloper.weather: InputConvertVolume
Fields
- input decimal -
- 'source string -
- target string -
powertoolsdeveloper.weather: InputConvertWeight
Fields
- input decimal -
- 'source string -
- target string -
powertoolsdeveloper.weather: InputCsvConversionJSON
Fields
- input string - CSV string
- header boolean - Include header row
powertoolsdeveloper.weather: InputCurrencyConversion
Fields
- input decimal - Amount to convert
- 'source string -
- target string -
powertoolsdeveloper.weather: InputCurrencyFormat
Fields
- input decimal - Amount to format
- target string -
powertoolsdeveloper.weather: InputDataQuery
Fields
- input string - XML or JSON string
- query string - XPath or JSONPath query
powertoolsdeveloper.weather: InputDateTimeConversion
Fields
- input string - Source date and time
- 'source string -
- target string -
- format string? - Display format (defaults to 'yyyy-MM-dd HH:mm:ss')
powertoolsdeveloper.weather: InputDateTimeDifference
Fields
- dateTime1 string - First date/time value
- dateTime2 string - Second date/time value
powertoolsdeveloper.weather: InputDateTimeFormat
Fields
- input string - Source date and time
- culture string - Language culture
- format string - Output format
powertoolsdeveloper.weather: InputDateTimeInfo
Fields
- input string - Source date and time
- culture string - Language culture
powertoolsdeveloper.weather: InputGenerateHash
Fields
- input string - Hash source string
- algorithm string - Hash algorithm
powertoolsdeveloper.weather: InputGeneratePassword
Fields
- length decimal - Number of characters
- lowercase boolean - Include lowercase characters
- uppercase boolean - Include uppercase characters
- numeric boolean - Include single-digit numbers
- special boolean - Include non-alphanumeric characters
powertoolsdeveloper.weather: InputGenerateUniqueID
Fields
- uppercase string - All uppercase alpha characters
powertoolsdeveloper.weather: InputJoinStrings
Fields
- input string[] - Collection of strings to be joined
- separator string - Separator character
- lower string - Convert strings in collection to lowercase
- trim string - Trim strings in collection
powertoolsdeveloper.weather: InputJsonConversionCSV
Fields
- input string - JSON array object
- header boolean - Include header row
- omit string? - Columns to omit (comma separated)
- 'order string? - Column order (comma separated)
powertoolsdeveloper.weather: InputJsonConversionHTML
Fields
- input string - JSON array object
- header boolean - Include header row
- alternate string? - Alternate header row markup
- attributes string? - Optional table attributes (single quoted values)
- omit string? - Columns to omit (comma separated)
- 'order string? - Column order (comma separated)
powertoolsdeveloper.weather: InputJsonConversionXML
Fields
- input string - JSON array object
- root string - Name of root node
powertoolsdeveloper.weather: InputMapImage
Fields
- 'source string - Full or partial address
- zoom decimal - Map image zoom level
- height decimal - Image height
- width decimal - Image width
powertoolsdeveloper.weather: InputMarketIndex
Fields
- symbol string - Market index
- date string? - Date (yyyy-MM-dd, leave empty for last trading day)
powertoolsdeveloper.weather: InputNumberRange
Fields
- 'start decimal - Start of range
- end decimal - End of range
powertoolsdeveloper.weather: InputQRCode
Fields
- input string - Text value(s) (vertical bar delimited by type)
- payload string - Payload type
powertoolsdeveloper.weather: InputRedactString
Fields
- 'source string - String containing the complete text
- value string? - Individual string to redact
- values string[]? - Collection of strings to redact
- regex string? - Regular expression pattern for matching strings
powertoolsdeveloper.weather: InputReplaceString
Fields
- 'source string - String containing the text to be replaced
- value string - Text to replace
- replacement string - Replacement text
powertoolsdeveloper.weather: InputSplitString
Fields
- input string - Text to split
- characters string - One or more characters that will be used to split the text
powertoolsdeveloper.weather: InputStockPrices
Fields
- symbols string - Stock ticker symbols (comma-separated, max 20)
- date string? - Date (yyyy-MM-dd, leave empty for latest)
- exchange string? - Stock exchange
powertoolsdeveloper.weather: InputString
Fields
- 'source string - String variable or text value
powertoolsdeveloper.weather: InputStringComparison
Fields
- input string - Original string
- compare string - Comparison string
- lower string - Convert strings to lowercase before comparison
- trim string - Trim strings before comparison
powertoolsdeveloper.weather: InputStringContains
Fields
- find string - Text to match
- input string - Text to search
- lower string - Convert strings to lowercase
powertoolsdeveloper.weather: InputStringToFile
Fields
- input string - Text string (body of file)
- extension string - File extension
- filename string - Name of file (without extension)
powertoolsdeveloper.weather: InputTextToSpeech
Fields
- text string - Text to convert (10,000 characters max)
- 'type string - Text or file type
- voice string - Voice locale (must match language of input text)
powertoolsdeveloper.weather: InputTranslateString
Fields
- input string - String containing the text to be translated
- language string - Translation language
powertoolsdeveloper.weather: InputTrimString
Fields
- 'source string - String containing the text to be trimmed
- 'type string - Type of white space to remove
powertoolsdeveloper.weather: InputVerifyHash
Fields
- input string - Original source string
- algorithm string - Hash algorithm
- hash string - Hashed result
powertoolsdeveloper.weather: InputWeatherActivityForecast
Fields
- 'source string - Full or partial address
- activity string - Weather-related activity
powertoolsdeveloper.weather: InputWeatherConditions
Fields
- 'source string - Full or partial address
- units string - Units
powertoolsdeveloper.weather: InputWeatherDailyForecast
Fields
- 'source string - Full or partial address
- units string - Units
- duration decimal - Duration
powertoolsdeveloper.weather: InputWeatherHourlyForecast
Fields
- 'source string - Full or partial address
- units string - Units
- duration decimal - Duration
powertoolsdeveloper.weather: InputXmlConversionJSON
Fields
- input string - XML string
powertoolsdeveloper.weather: OutputActivityForecast
Fields
- result OutputactivityforecastResult[]? - Current weather-related activity forecast
powertoolsdeveloper.weather: OutputactivityforecastResult
Fields
- name string? - Name
- date string? - Date
- quality string? - Quality of weather for specified activity
- rating decimal? - General quality rating
- description string? - Description
powertoolsdeveloper.weather: OutputBoolean
Fields
- result boolean? - Result
powertoolsdeveloper.weather: OutputCollectionNumber
Fields
- status boolean? - Success
- item decimal? - First Value
- items decimal[]? - All Values
powertoolsdeveloper.weather: OutputCollectionResult
Fields
- result string[]? - Modified collection result
powertoolsdeveloper.weather: OutputCollectionString
Fields
- status boolean? - Success
- item string? - First Value
- items string[]? - All Values
powertoolsdeveloper.weather: OutputDailyForecast
Fields
- result OutputdailyforecastResult[]? - Daily weather forecast
powertoolsdeveloper.weather: OutputdailyforecastResult
Fields
- date string? - Date
- low string? - Low temperature
- high string? - High temperature
- sunlight decimal? - Hours of Sunlight
- airQuality string? - Relative air quality
- description string? - Description
- precipitation boolean? - Observable precipitation
- precipitationProbability decimal? - Chance of precipitiation
- precipitationType string? - Type of precipitation
- hoursPrecipitation decimal? - Hours of precipitation
- rainProbability decimal? - Probability of rain
- snowProbability decimal? - Probability of snow
- iceProbability decimal? - Probability of ice
- thunderstormProbability decimal? - Probability of thunderstorms
- windDirection string? - Wind direction
- windSpeed string? - Wind speed
- windGust string? - Wind gust
- totalRain string? - Total rain
- totalSnow string? - Total snow
- totalIce string? - Total ice
- cloudCover decimal? - Cloud cover
powertoolsdeveloper.weather: OutputDateDifference
Fields
- years decimal? - Years
- months decimal? - Months
- days decimal? - Days
- hours decimal? - Hours
- minutes decimal? - Minutes
- milliseconds decimal? - Milliseconds
- totalYears decimal? - Total Years
- totalMonths decimal? - Total Months
- totalDays decimal? - Total Days
- totalHours decimal? - Total Hours
- totalMinutes decimal? - Total Minutes
- totalSeconds decimal? - Total Seconds
- totalMilliseconds decimal? - Total Milliseconds
- ticks decimal? - Ticks
powertoolsdeveloper.weather: OutputDateInfo
Fields
- DayOfYear decimal? - DayOfYear
- DayOfWeek decimal? - DayOfWeek
- WeekOfYear decimal? - WeekOfYear
- SecondsInDay decimal? - SecondsInDay
- MinutesInDay decimal? - MinutesInDay
- Ticks decimal? - Ticks
powertoolsdeveloper.weather: OutputFileByte
Fields
- result string? - Result
powertoolsdeveloper.weather: OutputHourlyForecast
Fields
- result OutputhourlyforecastResult[]? - Daily weather forecast
powertoolsdeveloper.weather: OutputhourlyforecastResult
Fields
- date string? - Date
- description string? - Description
- temperature string? - Temperature
- dewPoint string? - Dew point
- relativeHumidity decimal? - Relative humidity
- visibility string? - Visibility
- uvIndex string? - UV Index
- precipitation boolean? - Observable precipitation
- precipitationProbability decimal? - Chance of precipitiation
- rainProbability decimal? - Probability of rain
- snowProbability decimal? - Probability of snow
- iceProbability decimal? - Probability of ice
- windDirection string? - Wind direction
- windSpeed string? - Wind speed
- windGust string? - Wind gust
- totalRain string? - Total rain
- totalSnow string? - Total snow
- totalIce string? - Total ice
- ceiling string? - Ceiling
- cloudCover decimal? - Cloud cover
powertoolsdeveloper.weather: OutputImageMetadata
Fields
- result OutputimagemetadataResult[]? - Image EXIF Metadata
powertoolsdeveloper.weather: OutputimagemetadataResult
Fields
- apertureValue string? - Aperture Value
- artist string? - Artist
- backgroundColor string? - Background Color
- bitsPerSample string? - Bits Per Sample
- brightnessValue string? - Brightness Value
- cameraElevationAngle string? - Camera Elevation Angle
- clipPath string? - Clip Path
- colorMap string? - Color Map
- colorSpace string? - Color Space
- colorType string? - Color Type
- compressedBitsPerPixel string? - Compressed Bits Per Pixel
- compression string? - Compression Type
- contrast string? - Contrast
- copyright string? - Copyright
- customRendered string? - Custom Rendered
- dateTime string? - Date/Time
- dateTimeDigitized string? - DateTime Digitized
- dateTimeOriginal string? - DateTime Original
- decode string? - Decode
- defaultImageColor string? - Default Image Color
- deviceSettingDescription string? - Device Setting Description
- digitalZoomRatio string? - Digital Zoom Ratio
- documentName string? - Document Name
- exifVersion string? - ExifVersion
- exposureBiasValue string? - Exposure Bias Value
- exposureIndex string? - Exposure Index
- exposureMode string? - Exposure Mode
- exposureTime string? - Exposure Time
- extraSamples string? - Extra Samples
- fileSource string? - File Source
- fillOrder string? - Fill Order
- flash string? - Flash
- flashEnergy string? - Flash Energy
- fNumber string? - F Number
- focalLength string? - Focal Length
- gainControl string? - Gain Control
- gpsAltitude string? - GPS Altitude
- gpsAltitudeRef string? - GPS Altitude Ref
- gpsAreaInformation string? - GPS Area Information
- gpsDateStamp string? - GPS Date Stamp
- gpsDifferential string? - GPS Differential
- gpsLatitude string? - GPS Latitude
- gpsLongitude string? - GPS Longitude
- gpsSpeed string? - GPS Speed
- gpsStatus string? - GPS Status
- gpsTrack string? - GPS Track
- hostComputer string? - Host Computer
- imageDescription string? - Image Description
- imageHistory string? - Image History
- imageID string? - Image ID
- imageHeight string? - Image Height
- imageLength string? - Image Length
- imageNumber string? - Image Number
- imageWidth string? - Image Width
- isoSpeed string? - ISO Speed
- jpegInterchangeFormat string? - JPEG Interchange Format
- maxApertureValue string? - Max Aperture Value
- maxSampleValue string? - Max Sample Value
- meteringMode string? - Metering Mode
- model string? - Model
- modelNumber string? - Model Number
- orientation string? - Orientation
- ownerName string? - Owner Name
- pixelScale string? - Pixel Scale
- primaryChromaticities string? - Primary Chromaticities
- profileType string? - Profile Type
- rating string? - Rating
- resolutionUnit string? - Resolution Units
- sampleFormat string? - Sample Format
- sceneType string? - Scene Type
- securityClassification string? - Security Classification
- sensingMethod string? - Sensing Method
- serialNumber string? - Serial Number
- sharpness string? - Sharpness
- shutterSpeedValue string? - Shutter Speed Value
- software string? - Software
- userComment string? - User Comment
- 'version string? - Version
- whiteBalance string? - WhiteBalance
- whitePoint string? - White Point
- whitePointX string? - White Point X
- whitePointY string? - White Point Y
- xResolution string? - X Resolution
- yResolution string? - Y Resolution
powertoolsdeveloper.weather: OutputIPLocation
Fields
- countryName string? - Country Name
- countryCode string? - Country Code
- region string? - Region
- locale string? - Locale
powertoolsdeveloper.weather: OutputMapCoordinates
Fields
- latitude decimal? - Latitude
- longitude decimal? - Longitude
powertoolsdeveloper.weather: OutputMarketIndex
Fields
- date string? - Date
- symbol string? - Index symbol
- exchange string? - Market exchange
- open decimal? - Open value
- high decimal? - High value
- low decimal? - Low value
- close decimal? - Close value
- volume decimal? - Trading volume
- adj_open decimal? - Adjusted open value
- adj_high decimal? - Adjusted high value
- adj_low decimal? - Adjusted low value
- adj_close decimal? - Adjusted close value
- adj_volume decimal? - Adjusted trading volume
powertoolsdeveloper.weather: OutputMultiCollection
Fields
- result1 string[]? - First collection result
- result2 string[]? - Second collection result
powertoolsdeveloper.weather: OutputNumber
Fields
- result decimal? - Result
powertoolsdeveloper.weather: OutputStockPrice
Fields
- result OutputstockpriceResult[]? - Stock price information
powertoolsdeveloper.weather: OutputstockpriceResult
Fields
- date string? - Date
- symbol string? - Ticker symbol
- exchange string? - Stock exchange
- open decimal? - Open
- high decimal? - High
- low decimal? - Low
- close decimal? - Close
- volume decimal? - Volume
powertoolsdeveloper.weather: OutputString
Fields
- result string? - Result
powertoolsdeveloper.weather: OutputStringArray
Fields
- data string[]? - data
powertoolsdeveloper.weather: OutputTimezoneInfo
Fields
- id string? - Timezone ID
- countryName string? - Full country name
- countryCode string? - Two-letter country code
- shortName string? - Short timezone name
- standardName string? - Standard timezone name
- dstName string? - Daylight Savings timezone name
- standardOffset string? - Standard timezone offset from GMT
- dstOffset string? - Daylight savings timezone offset from GMT
- dstStart string? - Daylight savings time start date
- dstEnd string? - Daylight savings time end date
- sunrise string? - Time of sunrise in target timezone
- sunset string? - Time of sunset in target timezone
powertoolsdeveloper.weather: OutputWeatherConditions
Fields
- description string? - Description
- precipitation boolean? - Precipitation
- accumulatedPrecipitation string? - Accumulated precipitation (24 hours)
- temperature decimal? - Temperature
- windChill decimal? - Wind Chill
- humidity decimal? - Relative humidity
- dewPoint decimal? - Dew point
- windDirection string? - Wind direction
- dstStart string? - Daylight savings time start date
- windSpeed string? - Wind speed
- windGust string? - Wind gusts (max)
- uvIndex decimal? - UV Index
- uvDescription string? - UV Level
- visibility string? - Visbility
- cloudCover decimal? - Cloud cover
- ceiling string? - Ceiling
- pressure string? - Barometric pressure
- pressureTendency string? - Barometric pressure trend
powertoolsdeveloper.weather: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
Import
import ballerinax/powertoolsdeveloper.weather;
Metadata
Released date: almost 2 years ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 2
Current verison: 2
Weekly downloads
Keywords
Website & App Building/App Builders
Cost/Freemium
Contributors
Dependencies