powertoolsdeveloper.math
Module powertoolsdeveloper.math
API
Definitions
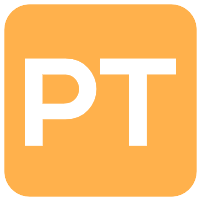
ballerinax/powertoolsdeveloper.math Ballerina library
Overview
This is a generated connector for Apptigent PowerTools Developer API v2021.1.01 OpenAPI specification. Apptigent PowerTools Developer Edition is a powerful suite of API endpoints for custom applications running on any stack. Manipulate text, modify collections, format dates and times, convert currency, perform advanced mathematical calculations, shorten URL's, encode strings, convert text to speech, translate content into multiple languages, process images, and more. PowerTools is the ultimate developer toolkit. This connector provides the capability for mathematical calculations and conversions.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create Apptigent account
- Obtain tokens
- Log into Apptigent Developer Portal by visiting https://portal.apptigent.com
- Create an app and obtain the
Client ID
which will be used as theAPI Key
by following the guidelines described here.
Quickstart
To use the Apptigent PowerTools Developer connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
Import the ballerinax/powertoolsdeveloper.math
module into the Ballerina project.
import ballerinax/powertoolsdeveloper.math as pm;
Step 2: Create a new connector instance
Create a math:ApiKeysConfig
with the Client ID obtained, and initialize the connector with it.
pm:ApiKeysConfig config = { xIbmClientId: "<CLIENT_ID>" } pm:Client baseClient = check new Client(config);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to calculate the standard deviation of two or more numbers using the connector by providing the
InputCalculateSeries
record with collection of values to calculate and number of decimal places to round.Calculate the standard deviation of two or more numbers
public function main() { pm:InputCalculateSeries inputCalculateSeries = { input: [1,2,3,4,5], decimals: 2 }; pm:OutputNumber|error response = baseClient->standardDeviation(inputCalculateSeries); if (response is pm:OutputNumber) { log:printInfo(response.toString()); } else { log:printError(response.message()); } }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
powertoolsdeveloper.math: Client
This is a generated connector for Apptigent PowerTools Developer API v2021.1.01 OpenAPI specification. 'Apptigent PowerTools Developer Edition is a powerful suite of API endpoints for custom applications running on any stack. Manipulate text, modify collections, format dates and times, convert currency, perform advanced mathematical calculations, shorten URL's, encode strings, convert text to speech, translate content into multiple languages, process images, and more. PowerTools is the ultimate developer toolkit.' This connector provides the capability for mathematical calculations and conversions.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create an Apptigent account and obtain tokens following this guide.
init (ApiKeysConfig apiKeyConfig, ConnectionConfig config, string serviceUrl)
- apiKeyConfig ApiKeysConfig - API keys for authorization
- config ConnectionConfig {} - The configurations to be used when initializing the
connector
- serviceUrl string "https://connect.apptigent.com/api/utilities" - URL of the target service
calculateAverage
function calculateAverage(InputCalculateSeries payload) returns OutputNumber|error
Math - Calculate average
Parameters
- payload InputCalculateSeries - Series calculation parameters
Return Type
- OutputNumber|error - OK
calculateMedian
function calculateMedian(InputCalculateSeries payload) returns OutputNumber|error
Math - Calculate median
Parameters
- payload InputCalculateSeries - Series calculation parameters
Return Type
- OutputNumber|error - OK
calculateMinMax
function calculateMinMax(InputCalculateMinMax payload) returns OutputNumber|error
Math - Calculate minimum or maximum
Parameters
- payload InputCalculateMinMax - Series calculation parameters
Return Type
- OutputNumber|error - OK
calculateSum
function calculateSum(InputCalculateSeries payload) returns OutputNumber|error
Math - Calculate sum
Parameters
- payload InputCalculateSeries - Series calculation parameters
Return Type
- OutputNumber|error - OK
calculatePower
function calculatePower(InputCalculatePower payload) returns OutputNumber|error
Math - Calculate power
Parameters
- payload InputCalculatePower - Power calculation parameters
Return Type
- OutputNumber|error - OK
calculateVariance
function calculateVariance(InputCalculateSeries payload) returns OutputNumber|error
Math - Calculate variance
Parameters
- payload InputCalculateSeries - Series calculation parameters
Return Type
- OutputNumber|error - OK
calculateAddition
function calculateAddition(InputCalculateNumbers payload) returns OutputNumber|error
Math - Calculate Addition
Parameters
- payload InputCalculateNumbers - Number calculation parameters
Return Type
- OutputNumber|error - OK
calculateSubtraction
function calculateSubtraction(InputCalculateNumbers payload) returns OutputNumber|error
Math - Calculate Subtraction
Parameters
- payload InputCalculateNumbers - Number calculation parameters
Return Type
- OutputNumber|error - OK
calculateMultiplication
function calculateMultiplication(InputCalculateNumbers payload) returns OutputNumber|error
Math - Calculate Multiplication
Parameters
- payload InputCalculateNumbers - Number calculation parameters
Return Type
- OutputNumber|error - OK
calculateDivision
function calculateDivision(InputCalculateNumbers payload) returns OutputNumber|error
Math - Calculate Division
Parameters
- payload InputCalculateNumbers - Number calculation parameters
Return Type
- OutputNumber|error - OK
calculateModulo
function calculateModulo(InputCalculateNumbers payload) returns OutputNumber|error
Math - Calculate Modulo
Parameters
- payload InputCalculateNumbers - Number calculation parameters
Return Type
- OutputNumber|error - OK
calculateNthRoot
function calculateNthRoot(InputCalculateNumbers payload) returns OutputNumber|error
Math - Calculate Nth Root
Parameters
- payload InputCalculateNumbers - Number calculation parameters
Return Type
- OutputNumber|error - OK
calculateSquareRoot
function calculateSquareRoot(InputCalculateNumber payload) returns OutputNumber|error
Math - Calculate Square Root
Parameters
- payload InputCalculateNumber - Number calculation parameters
Return Type
- OutputNumber|error - OK
calculateLogarithm
function calculateLogarithm(InputCalculateNumber payload) returns OutputNumber|error
Math - Calculate Logarithm
Parameters
- payload InputCalculateNumber - Number calculation parameters
Return Type
- OutputNumber|error - OK
calculateSine
function calculateSine(InputCalculateNumber payload) returns OutputNumber|error
Math - Calculate Sine
Parameters
- payload InputCalculateNumber - Number calculation parameters
Return Type
- OutputNumber|error - OK
calculateCosine
function calculateCosine(InputCalculateNumber payload) returns OutputNumber|error
Math - Calculate Cosine
Parameters
- payload InputCalculateNumber - Number calculation parameters
Return Type
- OutputNumber|error - OK
calculateTangent
function calculateTangent(InputCalculateNumber payload) returns OutputNumber|error
Math - Calculate Tangent
Parameters
- payload InputCalculateNumber - Number calculation parameters
Return Type
- OutputNumber|error - OK
calculateAbsolute
function calculateAbsolute(InputCalculateNumber payload) returns OutputNumber|error
Math - Calculate Absolute
Parameters
- payload InputCalculateNumber - Number calculation parameters
Return Type
- OutputNumber|error - OK
convertAngle
function convertAngle(InputConvertAngle payload) returns OutputNumber|error
Math - Convert angle
Parameters
- payload InputConvertAngle - Input convert angle parameters
Return Type
- OutputNumber|error - OK
convertArea
function convertArea(InputConvertArea payload) returns OutputNumber|error
Math - Convert area
Parameters
- payload InputConvertArea - Input convert area parameters
Return Type
- OutputNumber|error - OK
convertDistance
function convertDistance(InputConvertDistance payload) returns OutputNumber|error
Math - Convert distance
Parameters
- payload InputConvertDistance - Input convert distance parameters
Return Type
- OutputNumber|error - OK
convertDuration
function convertDuration(InputConvertDuration payload) returns OutputNumber|error
Math - Convert duration
Parameters
- payload InputConvertDuration - Input convert duration parameters
Return Type
- OutputNumber|error - OK
convertEnergy
function convertEnergy(InputConvertEnergy payload) returns OutputNumber|error
Math - Convert energy
Parameters
- payload InputConvertEnergy - Input convert energy parameters
Return Type
- OutputNumber|error - OK
convertPower
function convertPower(InputConvertPower payload) returns OutputNumber|error
Math - Convert power
Parameters
- payload InputConvertPower - Input convert power parameters
Return Type
- OutputNumber|error - OK
convertSpeed
function convertSpeed(InputConvertSpeed payload) returns OutputNumber|error
Math - Convert speed
Parameters
- payload InputConvertSpeed - Input convert speed parameters
Return Type
- OutputNumber|error - OK
convertTemperature
function convertTemperature(InputConvertTemperature payload) returns OutputNumber|error
Math - Convert temperature
Parameters
- payload InputConvertTemperature - Input convert temperature parameters
Return Type
- OutputNumber|error - OK
convertVolume
function convertVolume(InputConvertVolume payload) returns OutputNumber|error
Math - Convert volume
Parameters
- payload InputConvertVolume - Input convert volume parameters
Return Type
- OutputNumber|error - OK
convertWeight
function convertWeight(InputConvertWeight payload) returns OutputNumber|error
Math - Convert weight
Parameters
- payload InputConvertWeight - Input convert weight parameters
Return Type
- OutputNumber|error - OK
randomNumber
function randomNumber(InputNumberRange payload) returns OutputNumber|error
Math - Random number
Parameters
- payload InputNumberRange - Input number range parameters
Return Type
- OutputNumber|error - OK
roundNumber
function roundNumber(InputCalculateNumber payload) returns OutputNumber|error
Math - Round number
Parameters
- payload InputCalculateNumber - Numeric calculation parameters
Return Type
- OutputNumber|error - OK
standardDeviation
function standardDeviation(InputCalculateSeries payload) returns OutputNumber|error
Math - Calculate standard deviation
Parameters
- payload InputCalculateSeries - Series calculation parameters
Return Type
- OutputNumber|error - OK
Records
powertoolsdeveloper.math: ApiKeysConfig
Provides API key configurations needed when communicating with a remote HTTP endpoint.
Fields
- xIbmClientId string - Represents API Key
X-IBM-Client-Id
powertoolsdeveloper.math: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
powertoolsdeveloper.math: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
powertoolsdeveloper.math: InputCalculateMinMax
Fields
- input decimal[] - Colllection of values to calculate
- 'type string - Minimum or Maximum
powertoolsdeveloper.math: InputCalculateNumber
Fields
- input decimal - Numeric value to calculate
- decimals decimal - Round to number of decimal places
powertoolsdeveloper.math: InputCalculateNumbers
Fields
- input decimal - Numeric value
- value decimal - Addend, subtrahend, factor, divisor or radicand
- decimals decimal - Round to number of decimal places
powertoolsdeveloper.math: InputCalculatePower
Fields
- input decimal - Number to raise
- power decimal - Power
- decimals decimal - Round to number of decimal places
powertoolsdeveloper.math: InputCalculateSeries
Fields
- input decimal[] - Colllection of values to calculate
- decimals decimal - Round to number of decimal places
powertoolsdeveloper.math: InputCaseConversion
Fields
- input string - String containing the text to convert
- alphacase string - Case of conversion result
powertoolsdeveloper.math: InputCollectionConversion
Fields
- input string[] - Collection containing strings to convert
- name string - Collection name
powertoolsdeveloper.math: InputCollectionConversionXML
Fields
- input string[] - Collection containing strings to convert
- root string - Name of root XML node
- child string - Name of child XML node(s)
powertoolsdeveloper.math: InputCollectionCount
Fields
- input string[] - Collection of items to count
powertoolsdeveloper.math: InputCollectionFilter
Fields
- input string[] - Collection of strings to filter
- 'match string - Match type
- keywords string - Keywords (separate multiple values with commas)
powertoolsdeveloper.math: InputCollectionModify
Fields
- input string[] - Collection of values or objects to modify
- item string? - Item (for multiple items, leave blank and use Items)
- items string[]? - Items (Collection, for a single item leave blank and use Item)
- index string? - Index position for operation (leave blank to specify end of collection)
powertoolsdeveloper.math: InputCollectionReplace
Fields
- input string[] - Collection of strings
- 'match string - Match value
- replacement string - Replacement value
- ignoreCase string - Ignore case
powertoolsdeveloper.math: InputCollectionSearch
Fields
- input string[] - Collection of strings to search
- 'match string - Text to match
- trim string? - Trim white space from comparison string
- ignorecase string? - Ignore case when performing comparison
powertoolsdeveloper.math: InputCollectionSearchNumeric
Fields
- input decimal[] - Collection of strings to search
- 'match decimal - Number to match
- 'type string(default "Integer") - Type of number - integer or decimal
powertoolsdeveloper.math: InputCollectionSort
Fields
- input string[] - Collection of strings to sort
- 'order string - Sort order
powertoolsdeveloper.math: InputCollectionSplit
Fields
- input string[] - Collection of items to split
- 'match string? - String to match (explicit, case-insensitive, leave empty to use Index)
- index string? - Index location to split (leave empty to use Match value)
powertoolsdeveloper.math: InputConvertAngle
Fields
- input decimal -
- 'source string -
- target string -
powertoolsdeveloper.math: InputConvertArea
Fields
- input decimal -
- 'source string -
- target string -
powertoolsdeveloper.math: InputConvertDistance
Fields
- input decimal -
- 'source string -
- target string -
powertoolsdeveloper.math: InputConvertDuration
Fields
- input decimal -
- 'source string -
- target string -
powertoolsdeveloper.math: InputConvertEnergy
Fields
- input decimal -
- 'source string -
- target string -
powertoolsdeveloper.math: InputConvertPower
Fields
- input decimal -
- 'source string -
- target string -
powertoolsdeveloper.math: InputConvertSpeed
Fields
- input decimal -
- 'source string -
- target string -
powertoolsdeveloper.math: InputConvertTemperature
Fields
- input decimal -
- 'source string -
- target string -
powertoolsdeveloper.math: InputConvertVolume
Fields
- input decimal -
- 'source string -
- target string -
powertoolsdeveloper.math: InputConvertWeight
Fields
- input decimal -
- 'source string -
- target string -
powertoolsdeveloper.math: InputCsvConversionJSON
Fields
- input string - CSV string
- header boolean - Include header row
powertoolsdeveloper.math: InputCurrencyConversion
Fields
- input decimal - Amount to convert
- 'source string -
- target string -
powertoolsdeveloper.math: InputCurrencyFormat
Fields
- input decimal - Amount to format
- target string -
powertoolsdeveloper.math: InputDataQuery
Fields
- input string - XML or JSON string
- query string - XPath or JSONPath query
powertoolsdeveloper.math: InputDateTimeConversion
Fields
- input string - Source date and time
- 'source string -
- target string -
- format string? - Display format (defaults to 'yyyy-MM-dd HH:mm:ss')
powertoolsdeveloper.math: InputDateTimeDifference
Fields
- dateTime1 string - First date/time value
- dateTime2 string - Second date/time value
powertoolsdeveloper.math: InputDateTimeFormat
Fields
- input string - Source date and time
- culture string - Language culture
- format string - Output format
powertoolsdeveloper.math: InputDateTimeInfo
Fields
- input string - Source date and time
- culture string - Language culture
powertoolsdeveloper.math: InputGenerateHash
Fields
- input string - Hash source string
- algorithm string - Hash algorithm
powertoolsdeveloper.math: InputGenerateUniqueID
Fields
- uppercase string - All uppercase alpha characters
powertoolsdeveloper.math: InputJoinStrings
Fields
- input string[] - Collection of strings to be joined
- separator string - Separator character
- lower string - Convert strings in collection to lowercase
- trim string - Trim strings in collection
powertoolsdeveloper.math: InputJsonConversionCSV
Fields
- input string - JSON array object
- header boolean - Include header row
- omit string? - Columns to omit (comma separated)
- 'order string? - Column order (comma separated)
powertoolsdeveloper.math: InputJsonConversionHTML
Fields
- input string - JSON array object
- header boolean - Include header row
- alternate string? - Alternate header row markup
- attributes string? - Optional table attributes (single quoted values)
- omit string? - Columns to omit (comma separated)
- 'order string? - Column order (comma separated)
powertoolsdeveloper.math: InputJsonConversionXML
Fields
- input string - JSON array object
- root string - Name of root node
powertoolsdeveloper.math: InputMarketIndex
Fields
- symbol string - Market index
- date string? - Date (yyyy-MM-dd, leave empty for last trading day)
powertoolsdeveloper.math: InputNumberRange
Fields
- 'start decimal - Start of range
- end decimal - End of range
powertoolsdeveloper.math: InputQRCode
Fields
- input string - Text value(s) (vertical bar delimited by type)
- payload string - Payload type
powertoolsdeveloper.math: InputRedactString
Fields
- 'source string - String containing the complete text
- value string? - Individual string to redact
- values string[]? - Collection of strings to redact
- regex string? - Regular expression pattern for matching strings
powertoolsdeveloper.math: InputReplaceString
Fields
- 'source string - String containing the text to be replaced
- value string - Text to replace
- replacement string - Replacement text
powertoolsdeveloper.math: InputSplitString
Fields
- input string - Text to split
- characters string - One or more characters that will be used to split the text
powertoolsdeveloper.math: InputStockPrices
Fields
- symbols string - Stock ticker symbols (comma-separated, max 20)
- date string? - Date (yyyy-MM-dd, leave empty for latest)
- exchange string? - Stock exchange
powertoolsdeveloper.math: InputString
Fields
- 'source string - String variable or text value
powertoolsdeveloper.math: InputStringComparison
Fields
- input string - Original string
- compare string - Comparison string
- lower string - Convert strings to lowercase before comparison
- trim string - Trim strings before comparison
powertoolsdeveloper.math: InputStringContains
Fields
- find string - Text to match
- input string - Text to search
- lower string - Convert strings to lowercase
powertoolsdeveloper.math: InputStringToFile
Fields
- input string - Text string (body of file)
- extension string - File extension
- filename string - Name of file (without extension)
powertoolsdeveloper.math: InputTextToSpeech
Fields
- text string - Text to convert (10,000 characters max)
- 'type string - Text or file type
- voice string - Voice locale (must match language of input text)
powertoolsdeveloper.math: InputTranslateString
Fields
- input string - String containing the text to be translated
- language string - Translation language
powertoolsdeveloper.math: InputTrimString
Fields
- 'source string - String containing the text to be trimmed
- 'type string - Type of white space to remove
powertoolsdeveloper.math: InputVerifyHash
Fields
- input string - Original source string
- algorithm string - Hash algorithm
- hash string - Hashed result
powertoolsdeveloper.math: InputXmlConversionJSON
Fields
- input string - XML string
powertoolsdeveloper.math: OutputBoolean
Fields
- result boolean? - Result
powertoolsdeveloper.math: OutputCollectionNumber
Fields
- status boolean? - Success
- item decimal? - First Value
- items decimal[]? - All Values
powertoolsdeveloper.math: OutputCollectionResult
Fields
- result string[]? - Modified collection result
powertoolsdeveloper.math: OutputCollectionString
Fields
- status boolean? - Success
- item string? - First Value
- items string[]? - All Values
powertoolsdeveloper.math: OutputDateDifference
Fields
- years decimal? - Years
- months decimal? - Months
- days decimal? - Days
- hours decimal? - Hours
- minutes decimal? - Minutes
- milliseconds decimal? - Milliseconds
- totalYears decimal? - Total Years
- totalMonths decimal? - Total Months
- totalDays decimal? - Total Days
- totalHours decimal? - Total Hours
- totalMinutes decimal? - Total Minutes
- totalSeconds decimal? - Total Seconds
- totalMilliseconds decimal? - Total Milliseconds
- ticks decimal? - Ticks
powertoolsdeveloper.math: OutputDateInfo
Fields
- DayOfYear decimal? - DayOfYear
- DayOfWeek decimal? - DayOfWeek
- WeekOfYear decimal? - WeekOfYear
- SecondsInDay decimal? - SecondsInDay
- MinutesInDay decimal? - MinutesInDay
- Ticks decimal? - Ticks
powertoolsdeveloper.math: OutputFileByte
Fields
- result string? - Result
powertoolsdeveloper.math: OutputMarketIndex
Fields
- date string? - Date
- symbol string? - Index symbol
- exchange string? - Market exchange
- open decimal? - Open value
- high decimal? - High value
- low decimal? - Low value
- close decimal? - Close value
- volume decimal? - Trading volume
- adj_open decimal? - Adjusted open value
- adj_high decimal? - Adjusted high value
- adj_low decimal? - Adjusted low value
- adj_close decimal? - Adjusted close value
- adj_volume decimal? - Adjusted trading volume
powertoolsdeveloper.math: OutputMultiCollection
Fields
- result1 string[]? - First collection result
- result2 string[]? - Second collection result
powertoolsdeveloper.math: OutputNumber
Fields
- result decimal? - Result
powertoolsdeveloper.math: OutputStockPrice
Fields
- result OutputstockpriceResult[]? - Stock price information
powertoolsdeveloper.math: OutputstockpriceResult
Fields
- date string? - Date
- symbol string? - Ticker symbol
- exchange string? - Stock exchange
- open decimal? - Open
- high decimal? - High
- low decimal? - Low
- close decimal? - Close
- volume decimal? - Volume
powertoolsdeveloper.math: OutputString
Fields
- result string? - Result
powertoolsdeveloper.math: OutputStringArray
Fields
- data string[]? - data
powertoolsdeveloper.math: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
Import
import ballerinax/powertoolsdeveloper.math;
Metadata
Released date: over 2 years ago
Version: 1.4.0
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.2.1
GraalVM compatible: Yes
Pull count
Total: 5
Current verison: 0
Weekly downloads
Keywords
Website & App Building/App Builders
Cost/Freemium
Contributors
Dependencies