peoplehr
Module peoplehr
API
Definitions
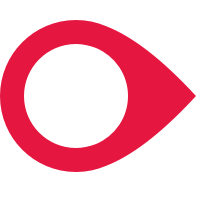
ballerinax/peoplehr Ballerina library
Overview
PeopleHR connector provides the capability to access the operation to manage employee details, holidays and salaries etc.
This module supports 3.1 version.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create PeopleHR account
- Obtain tokens by following this guide
Quickstart
To use the people connector in your Ballerina application, update the .bal file as follows: Add steps to create a simple sample
Step 1 - Import connector
import ballerinax/peoplehr;
Step 2 - Create a new connector instance
peoplehr:Client baseClient = check new ({apiKey: <API Key>, baseURL: "https://api.peoplehr.net"});
Step 3 - Invoke connector operation
peoplehr:QueryDetail response = check baseClient->getQueryByName({QueryName: <Query Name>});
Use bal run
command to compile and run the Ballerina program.
Clients
peoplehr: Client
This connector helps you easily integrate People with other systems and applications, for seamless cross-platform data sharing. The People API accepts and returns JSON data in the request body, with status indicating the outcome of the operation (sucess/failure).
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Follow this guide to obtain token for PeopleHR connector.
init (ConnectionConfig config)
- config ConnectionConfig - People connection configuration
createNewEmployee
function createNewEmployee(NewEmployeeRequest|json payload) returns OperationStatus|error
Creates new employee
Parameters
- payload NewEmployeeRequest|json - Request details on an new employee
Return Type
- OperationStatus|error - Successful response or an error
getEmployeeById
function getEmployeeById(EmployeeRequest payload) returns EmployeeResponse|error
Gets employee detail by id
Parameters
- payload EmployeeRequest - Request details on an employee
Return Type
- EmployeeResponse|error - Successful response or an error
updateEmployeeId
function updateEmployeeId(EmployeeIdUpdateRequest payload) returns OperationStatus|error
Updates employee id.
Parameters
- payload EmployeeIdUpdateRequest - Request details on an employee
Return Type
- OperationStatus|error - Successful response or an error
getAllEmployees
function getAllEmployees(AllEmployeesRequest payload) returns EmployeesResponse|error
Gets all employees.
Parameters
- payload AllEmployeesRequest - Request details on all employees
Return Type
- EmployeesResponse|error - Successful response or an error
updateEmployee
function updateEmployee(EmployeeUpdateRequest|json payload) returns OperationStatus|error
Updates employee details.
Parameters
- payload EmployeeUpdateRequest|json - Request details on an employee
Return Type
- OperationStatus|error - Successful response or an error
markAsLeaverById
function markAsLeaverById(EmployeeLeaverStatus payload) returns OperationStatus|error
Updates/marks employee leaver status by id.
Parameters
- payload EmployeeLeaverStatus - Request details on an employee
Return Type
- OperationStatus|error - Successful response or an error
getSalaryDetail
function getSalaryDetail(SalaryDetailRequest payload) returns SalaryDetailGetResponse|error
Gets employee salary details.
Parameters
- payload SalaryDetailRequest - Request detail on salary
Return Type
- SalaryDetailGetResponse|error - Successful response or an error
addNewHoliday
function addNewHoliday(NewHolidayRequest|json payload) returns OperationStatus|error
Add new holiday.
Parameters
- payload NewHolidayRequest|json - Request detail on new holiday
Return Type
- OperationStatus|error - Successful response or an error
getHolidayDetail
function getHolidayDetail(HolidayDetail payload) returns HolidayGetResponse|error
Gets holiday detail list
Parameters
- payload HolidayDetail - Request detail on a holiday
Return Type
- HolidayGetResponse|error - Successful response or an error
deleteHoliday
function deleteHoliday(HolidayDetail payload) returns OperationStatus|error
Deletes holiday detail
Parameters
- payload HolidayDetail - Request detail on a holiday
Return Type
- OperationStatus|error - Successful response or an error
createNewVacancy
function createNewVacancy(NewVacancy payload) returns OperationStatus|error
Create new vacancy
Parameters
- payload NewVacancy - Request detail on new vacancy
Return Type
- OperationStatus|error - Successful response or an error
getVacancy
function getVacancy(GetVacancyResultRequest|json payload) returns VacancyGetResponse|error
Gets vacancy detail
Parameters
- payload GetVacancyResultRequest|json - Request detail on a vacancy
Return Type
- VacancyGetResponse|error - Successful response or an error
getAllVacancies
function getAllVacancies() returns AllVacancies|error
Gets all vacancy detail
Return Type
- AllVacancies|error - Successful response or an error
createNewApplicant
function createNewApplicant(NewApplicant payload) returns OperationStatus|error
Creates New Applicant
Parameters
- payload NewApplicant - New applicant detail
Return Type
- OperationStatus|error - Successful response or an error
uploadApplicantDocument
function uploadApplicantDocument(NewDocument payload) returns OperationStatus|error
Upload applicant document
Parameters
- payload NewDocument - Request detail on new document
Return Type
- OperationStatus|error - Successful response or an error
checkDuplicateApplicant
function checkDuplicateApplicant(ApplicantInformation payload) returns OperationStatus|error
Checks duplicate applicant
Parameters
- payload ApplicantInformation - Request detail on an applicant
Return Type
- OperationStatus|error - Successful response or an error
getQueryByName
function getQueryByName(QueryResultGetRequest payload) returns QueryDetail|error
Gets query result By query name details.
Parameters
- payload QueryResultGetRequest - Request detail on a query
Return Type
- QueryDetail|error - Successful response or an error
checkAuthentication
function checkAuthentication(AuthenticationInfo payload) returns AuthenticationResponse|error
Checks if credentials of a given login user is valid.
Parameters
- payload AuthenticationInfo - Request details on authentication
Return Type
- AuthenticationResponse|error - Successful response or an error
getEmployeeScreenDetail
function getEmployeeScreenDetail() returns EmployeeScreenDetailResponse|error
Retrieves employee screen detail
Return Type
- EmployeeScreenDetailResponse|error - Successful response or an error
getEmployeeScreenDetailByEmployeeID
function getEmployeeScreenDetailByEmployeeID(ScreenDetailByEmployeeIDRequest payload) returns EmployeeScreenDetailResponse|error
Retrieves employee screen detail by employee ID
Parameters
- payload ScreenDetailByEmployeeIDRequest - Request details on employee screen
Return Type
- EmployeeScreenDetailResponse|error - Successful response or an error
getEmployeeScreenDetailByTransactionID
function getEmployeeScreenDetailByTransactionID(ScreenDetailByTransactionIDRequest payload) returns EmployeeScreenDetailResponse|error
Retrieves employee screen detail by transaction ID
Parameters
- payload ScreenDetailByTransactionIDRequest - Request details on employee screen
Return Type
- EmployeeScreenDetailResponse|error - Successful response or an error
addNewCustomScreenTransaction
function addNewCustomScreenTransaction(NewCustomScreenTransactionDetails payload) returns OperationStatus|error
New custom screen transaction details
Parameters
- payload NewCustomScreenTransactionDetails - New employee screen details
Return Type
- OperationStatus|error - Successful response or an error
updateCustomScreenTransaction
function updateCustomScreenTransaction(ExistingCustomScreenTransactionDetails payload) returns OperationStatus|error
Update custom screen transaction details
Parameters
- payload ExistingCustomScreenTransactionDetails - Employee screen details
Return Type
- OperationStatus|error - Successful response or an error
DeleteCustomScreenTransaction
function DeleteCustomScreenTransaction(ScreenDetailByTransactionIDRequest payload) returns OperationStatus|error
Delete custom screen transaction details
Parameters
- payload ScreenDetailByTransactionIDRequest - Employee screen details
Return Type
- OperationStatus|error - Successful response or an error
getAppraisalDetailsByEmployeeID
function getAppraisalDetailsByEmployeeID(AppraisalDetailsRequest payload) returns AppraisalDetailsResponse|error
Retrieves By Employee Id Appraisal details
Parameters
- payload AppraisalDetailsRequest - Employee screen details
Return Type
- AppraisalDetailsResponse|error - Successful response or an error
getAppraisalDetailsByAppraisalID
function getAppraisalDetailsByAppraisalID(AppraisalDetailsByAppraisalIDRequest payload) returns AppraisalDetailsResponse|error
Retrieves appraisal details by appraisal Id
Parameters
- payload AppraisalDetailsByAppraisalIDRequest - Employee screen details
Return Type
- AppraisalDetailsResponse|error - Successful response or an error
Records
peoplehr: AdditionalQuestion
Represents additional question
Fields
- QuestionId string - Question ID
- Value string - Value default value
peoplehr: AllEmployeesRequest
Represents the input of getting all employee data operation
Fields
- IncludeLeavers boolean - Indicates whether to include the leavers or not.
- APIKey string -
- Action string -
- anydata... -
peoplehr: AllVacancies
Represents the response of GetAllVacancies
operation
Fields
- Fields Included from *PeopleHRGenericResponse
- Result Vacancy[] - The response of the operation
peoplehr: ApplicantInformation
Applicant information for duplicate checking
Fields
- FirstName string - First name for check duplicate applicant(<= 50 characters)
- LastName string - Last name for check duplicate applicant(<= 50 characters)
- VacancyReference string - Vacancy reference for check duplicate applicant
- Email string? - Email for check duplicate applicant
- APIKey string -
- Action string -
- anydata... -
peoplehr: AppraisalDetail
Represents appraisal details response results
Fields
- AppraisalId int - Appraisal ID
- AppraisalReviewDate string - Appraisal Review Date
- Reviewer string - Reviewer Details
- Note string - Note on appraisal
- ActionPlan string - Action Plan
- Objectives string - Objective detail
- Customfields Customfield[]? - Custom columns details
- Files File[]? - File Details
peoplehr: AppraisalDetailsByAppraisalIDRequest
Represents appraisal details request by appraisal ID
Fields
- EmployeeId string - Employee id for get by employee id
- AppraisalId int - Start date for get by employee id
peoplehr: AppraisalDetailsRequest
Represents appraisal details request
Fields
- EmployeeId string - Employee id for get by employee id
- StartDate string - Start date for get by employee id
- EndDate string - EndDate date for get by employee id
peoplehr: AppraisalDetailsResponse
Represents appraisal details response record
Fields
- Fields Included from *PeopleHRGenericResponse
- Result AppraisalDetail[]|record {} - Field Description
peoplehr: AuthenticationInfo
Represents the inputs of checkAuthentication
operation
Fields
- EmailAddress string - Email Address of the user
- Password string - The password of the user
- APIKey string -
- Action string -
- anydata... -
peoplehr: AuthenticationResponse
Represents the response type of updateEmployeeDetail
operation
Fields
- Fields Included from *PeopleHRGenericResponse
- Result EmployeeVerification - The response details of the operation
peoplehr: BackgroundDetail
Represents the background details
Fields
- CheckType ValueDetails? - The type of the check
- CompletionDate ValueDetails? - The completion date
- ExpiryDate ValueDetails? - The expiry date
- BackgroundDetailComments ValueDetails? - The background detail comments
peoplehr: BankDetail
Represents bank details
Fields
- BankName ValueDetails? - The name of the bank
- BankAddress ValueDetails? - The address of the bank
- BankCode ValueDetails? - The code of the bank
- AccountNumber ValueDetails? - Account number
- AccountName ValueDetails? - Account name
peoplehr: ConnectionConfig
Client configuration details.
Fields
- Fields Included from *ConnectionConfig
- auth AuthConfig
- httpVersion HttpVersion
- http1Settings ClientHttp1Settings
- http2Settings ClientHttp2Settings
- timeout decimal
- forwarded string
- poolConfig PoolConfiguration
- cache CacheConfig
- compression Compression
- circuitBreaker CircuitBreakerConfig
- retryConfig RetryConfig
- responseLimits ResponseLimitConfigs
- secureSocket ClientSecureSocket
- proxy ProxyConfig
- validation boolean
- anydata...
- auth never? -
- apiKey string - PeopleHR API key
- baseURL string(default "https://api.peoplehr.net") - Base URL
peoplehr: ContactDetail
Represents the contact detail
Fields
- Address ValueDetails? - The address of the contact details
- WorkPhoneNumber ValueDetails? - The work phone number
- PersonalPhoneNumber ValueDetails? - The personal phone number
- PersonalEmail ValueDetails? - The personal email
- Mobile ValueDetails? - The mobile
peoplehr: Customfield
Represents the Custom Fields
Fields
- ColumnName string - The name of the custom field
- ColumnValue string - The value of the custom field
peoplehr: DeductionsOrEntitlements
Represents deductions Or entitlements type record
Fields
- Deduction string? - deduction value
- Entitlement string? - entitlement value
- Amount string? - The amount
- Percentage string? - The percentage
- Comments string? - The comments
- IncludeInTotalSalary string? - Indicates whether includes in total salary
- DisplayAsAmountOrPercentage string? - The display as amount or percentage
peoplehr: Document
Represents Document detail
Fields
- DocumentName string - Document name
- Url string - URL for the document
peoplehr: Employee
Represents an employee type record
Fields
- EmployeeId ValueDetails - The employee ID
- Title ValueDetails? - The title of the employee
- FirstName ValueDetails? - The first name of the employee
- LastName ValueDetails? - The last name of the employee
- OtherName ValueDetails? - Other names
- KnownAs ValueDetails? - The employee is known as
- EmailId ValueDetails - Email ID
- StartDate ValueDetails? - The start date
- DateOfBirth ValueDetails? - The date of birth
- JobRole ValueDetails? - The job role
- Company ValueDetails - The company
- CompanyEffectiveDate ValueDetails? - The effective date
- Location ValueDetails? - The location
- LocationEffectiveDate ValueDetails? - The effective date of the location
- Department ValueDetails? - The department of the employee
- DepartmentEffectiveDate ValueDetails? - The effective date of the department
- JobRoleChangeDate ValueDetails? - The job role change date
- ReportsTo ValueDetails? - Employee reports to
- ReportsToEffectiveDate ValueDetails? - Reports to effective date
- ReportsToEmployeeId ValueDetails? - The ID of the employee whom should be reported
- ReportsToEmailAddress ValueDetails? - The email address of the employee whom should be reported
- NISNumber ValueDetails? - NIS number
- Nationality ValueDetails? - The nationality of the employee
- EmploymentType ValueDetails? - The type of the employee
- EmploymentTypeEffectiveDate ValueDetails? - The effective date of employment type
- EmployeeStatus ValueDetails? - The status of employee
- HolidayAllowanceDays ValueDetails? - The holiday allowance days
- HolidayAllowanceMins ValueDetails? - The holiday allowance mins
- NoticePeriod ValueDetails? - The notice period
- ProbationEndDate ValueDetails? - The end date of the probation
- Gender ValueDetails? - The gender
- ContactDetail ContactDetail - The contact detail
- OtherContact OtherContact[]? - The other contact
- RightToWork RightToWork[]? - Right to work
- BackgroundDetail ValueDetails[]? - background detail
- BankDetail ValueDetails? - The bank detail
- EmploymentDetail ValueDetails? - The employment detail
- lstFieldHistoryJobrole FieldHistoryForJobRole[]? - The last field history job role
- LeavingDate string? - The leaving date
- ReasonForLeaving string? - Reason for leaving
- EmployeeImage string? - The employee image
- APIColumn1 string? - API column 1
- APIColumn2 string? - API column 2
- APIColumn3 string? - API column 3
- APIColumn4 string? - API column 4
- APIColumn5 string? - API column 5
peoplehr: EmployeeIdUpdateRequest
Represents the input of UpdateEmployeeId
operation
Fields
- ReasonForChange string - The reason for change
- OldEmployeeId string - The old employee ID
- NewEmployeeId string - The new employee ID
- APIKey string -
- Action string -
- anydata... -
peoplehr: EmployeeLeaverStatus
Represent employee leaver status type record
Fields
- EmployeeId string - employee ID
- ReasonforLeaving string - Reason for leaving
- AdditionalComments string? - Additional comments
- FinalEmploymentDate string - final employment date
- FinalWorkingDate string? - Final working date
- MarkasLeaverImmediately string? - Mark as leaver immediately
- ReportsTo string? - Reports to
- ReEmployable string? - Re-employable
- SupportingComments string? - The supporting comments
- APIKey string -
- Action string -
- anydata... -
peoplehr: EmployeeRequest
Represents the input of getting employee detail operation
Fields
- EmployeeId string - The employee ID
- APIKey string -
- Action string -
- anydata... -
peoplehr: EmployeeResponse
Represents the response type of getEmployeeDetailById
operation
Fields
- Fields Included from *PeopleHRGenericResponse
- Result Employee?|record {} - The response details of the operation
peoplehr: EmployeeScreenDetail
Represents Employee Screen Details
Fields
- ScreenId int? - ScreenId is the unique identifier of the employee custom screen
- ScreenName string? - ScreenName is the name of the employee custom screen
- TxnId int? - Field Description
- Customfields Customfield[]? - Custom fields details
- Files File[]? - Files details
peoplehr: EmployeeScreenDetailResponse
Represents the response of GetEmployeeScreenDetail
operation
Fields
- Fields Included from *PeopleHRGenericResponse
- Result EmployeeScreenDetail[]|record {} - The response of the operation
peoplehr: EmployeesResponse
Represents the response type of getAllEmployeeDetail
operation
Fields
- Fields Included from *PeopleHRGenericResponse
- Result Employee[] - All employee detail
peoplehr: EmployeeUpdateRequest
Represents a new employee type record
Fields
- EmployeeId string - The employee ID
- Title string? - The title of the employee
- FirstName string? - The first name of the employee
- LastName string? - The last name of the employee
- Email string? - The email address
- Gender string? - The gender
- DateOfBirth string? - The date of birth
- StartDate string? - The start date
- ReportsTo string? - Employee reports to
- ReportsToEffectiveDate string? - The reports to effective date
- Company string? - The company
- CompanyEffectiveDate string? - The company effective date
- JobRole string? - The job role
- JobRoleEffectiveDate string? - The job role effective date
- Location string? - The location
- LocationEffectiveDate string? - The effective date of location
- Department string? - The department of the employee
- DepartmentEffectiveDate string? - The effective date of department
- NationalInsuranceNumber string? - The national insurance number
- Nationality string? - The nationality of the employee
- EmploymentType string? - The type of the employee
- EmploymentTypeEffectiveDate string? - The effective date of employee type
- Address string? - The address of the employee
- PersonalPhoneNumber string? - The personal phone number
- Payroll string? - The payroll
- Time string? - The time
- Rota string? - Rota
- CRM string? - CRM value
- ATS string? - ATS value
- Performance string? - The performance
- Benefits string? - The benefits
- System1\ ID string? - The system1 ID
- System2\ ID string? - The system2 ID
- System3\ ID string? - The system3 ID
- APIColumn1 string? - API column 1
- APIColumn2 string? - API column 2
- APIColumn3 string? - API column 3
- APIColumn4 string? - API column 4
- APIColumn5 string? - API column 5
- PersonalEmail string? - Personal email
- MethodOfRecruitment string? - Method of recruitment
- ReasonForChange string - Reason For Change
- APIKey string -
- Action string -
- anydata... -
peoplehr: EmployeeVerification
The Results of the employee verification
Fields
- EmployeeId string? - The employee Id of the verified user
peoplehr: ExistingCustomScreenTransactionDetails
Represents Existing custom screen transaction details
Fields
- Fields Included from *ScreenDetailByTransactionIDRequest
- CustomColumns Customfield[] - Field Description
- AddFiles NewFile[] - Field Description
peoplehr: FieldHistory
Represents the field history
Fields
- OldValue string? - The old value
- NewValue string? - The new value
- ChangedOn string? - The changed on
- ReasonForChange string? - The reason for change
peoplehr: FieldHistoryForEffectiveDate
Represents the field history for effective date
Fields
- FieldData string - The field date
- EffectiveDate string - The effective date
- ChangedOn string - The changed on
- ReasonForChange string - The reason for change
peoplehr: FieldHistoryForJobRole
Represents field history for job role type record
Fields
- JobRole string - The job role
- EffectiveDate string - The effective date
- ChangedOn string - The changed on
- ReasonForChange string - The reason for change
peoplehr: File
Represents Files Details
Fields
- DocumentName string - Name of the document name
- Description string - Description of the file
- FileUrl string - URL of the file
- Category string - File category
- SignatureRequired boolean - Whether a signature is required for the document
peoplehr: GetVacancyResultRequest
Represents the request payload of GetVacancyResult
operation
Fields
- VacancyReference string - Field Description
- APIKey string -
- Action string -
- anydata... -
peoplehr: Holiday
Represents holiday type record
Fields
- StartDate string? - The start date
- EndDate string? - The end date
- DurationType int? - The duration type
- DurationInDays int? - The duration in days
- DurationInMinutes int? - The duration in minutes
- DurationInDaysThisPeriod int? - The duration in days this period
- DurationInMinutesThisPeriod int? - The duration in minutes this period
- PartOfDay string? - Part of day
- RequesterComments string? - The requester comments
- ApproverComments string? - The approver comments
- Approver string? - Approver detail
- Status string? - The status
- LastActionDateTime string? - The last action date time
- IsToilHoliday boolean? - indicates whether isToil Holiday
- APIKey string -
- Action string -
- anydata... -
peoplehr: HolidayDetail
Represents holiday detail
Fields
- EmployeeId string - The employee ID
- StartDate string? - Start date
- EndDate string? - End date
- APIKey string -
- Action string -
- anydata... -
peoplehr: HolidayGetResponse
Represents the response type of GetHolidayDetail
operation
Fields
- Fields Included from *PeopleHRGenericResponse
- Result Holiday[] - Holiday Detail
peoplehr: HolidayUpdateRequest
Represents holiday update type record
Fields
- EmployeeId string - The employee ID
- OldStartDate string - The old start date
- OldEndDate string - The old end date
- DurationType string - The duration type
- StartDate string - The start date
- EndDate string - The end date
- DurationInDays string - The duration in days
- DurationInMinutes string - The duration in minutes
- PartOfDay string? - The part of day
- Comments string? - Comments
- APIKey string -
- Action string -
- anydata... -
peoplehr: InternalQuestion
Internal questions for create new applicant
Fields
- Fields Included from *AdditionalQuestion
peoplehr: NewApplicant
Represents new applicant
Fields
- VacancyReference string? - Vacancy reference for create new applicant
- FirstName string - First name for create new applicant
- LastName string - Last name for create new applicant
- Email string? - Email for create new applicant
- Gender string? - Gender for create new applicant
- DateOfBirth string? - Date of birth for create new applicant
- PostCode string? - Post code for create new applicant
- Address string? - Address for create new applicant
- PhoneNumber string? - PhoneNumber for create new applicant
- OtherContactDetails string? - Other contact details for create new applicant
- Source string? - Source for create new applicant
- AdditionalQuestions AdditionalQuestion[]? - Source for create new applicant
- InternalQuestions InternalQuestion[]? - Internal questions for create new applicant
- Documents Document[] - Documents for create new applicant
- Skills string - Skills for create new applicant
- RecruitmentCost string? - Recruitment cost for create new applicant
- DateLastContacted string? - Date last contacted for create new applicant
- APIKey string -
- Action string -
- anydata... -
- json... - Rest field
peoplehr: NewCustomScreenTransactionDetails
Represents new custom screen details
Fields
- Fields Included from *ScreenDetailByEmployeeIDRequest
- CustomColumns Customfield[] - CustomColumns for add new custom screen transaction
- AddFiles NewFile[] - AddFiles for add new custom screen transaction
peoplehr: NewDocument
Represents document detail
Fields
- ApplicantId string - ApplicantId for upload applicant document
- DocumentName string - Document name for upload applicant document(<= 100 characters)
- Description string - Description for upload applicant document(<= 256 characters)
- File string - File for upload applicant document
- APIKey string -
- Action string -
- anydata... -
peoplehr: NewEmployeeRequest
Represents a new employee type record
Fields
- EmployeeId string - The employee ID
- Title string? - The title of the employee
- FirstName string - The first name of the employee
- LastName string - The last name of the employee
- Email string? - The email address
- Gender string? - The gender
- StartDate string - The start date
- DateOfBirth string? - The date of birth
- ReportsTo string? - Employee reports to
- JobRole string - The job role
- JobRoleEffectiveDate string - Field Description
- Company string? - The company
- Location string - The location
- Department string - The department of the employee
- NationalInsuranceNumber string? - Field Description
- Nationality string? - The nationality of the employee
- EmploymentType string? - The type of the employee
- EntitlementThisYear string? - The entitlement this year
- EntitlementNextYear string? - The entitlement next year
- Address string? - The address of the employee
- PersonalPhoneNumber string? - The personal phone number
- Payroll string? - The payroll
- Time string? - The time
- Rota string? - Rota
- CRM string? - CRM value
- ATS string? - ATS value
- Performance string? - The performance
- Benefits string? - The benefits
- System1\ ID string? - The system1 ID
- System2\ ID string? - The system2 ID
- System3\ ID string? - The system3 ID
- APIColumn1 string? - API column 1
- APIColumn2 string? - API column 2
- APIColumn3 string? - API column 3
- APIColumn4 string? - API column 4
- APIColumn5 string? - API column 5
- PersonalEmail string? - Personal email
- MethodOfRecruitment string? - Method of recruitment
- APIKey string -
- Action string -
- anydata... -
peoplehr: NewFile
Represents Files Details
Fields
- DocumentName string - Name of the document name
- Description string - Description of the file
- File string - The file content in Base64 string
- DocumentCategory string - File category
- SignatureRequired boolean - Whether a signature is required for the document
peoplehr: NewHolidayRequest
Represents new holiday type record
Fields
- EmployeeId string - The employee ID
- DurationType string? - The duration type
- StartDate string? - The start date
- EndDate string? - The end date
- DurationInDays string? - The duration in days
- DurationInMinutes string? - The duration in minutes
- PartOfDay string? - The part of day
- Comments string? - The comments
- APIKey string -
- Action string -
- anydata... -
peoplehr: NewVacancy
Represents the details of the new vacancy
Fields
- VacancyName string? - The name of the vacancy
- VacancyDescription string? - The description of the vacancy
- Company string? - The company
- Location string? - The location
- Department string? - The department
- ClosingDate string? - The closing date
- Reference string? - The reference
- Comment string? - The comment
- SalaryRange string? - The salary range
- JobDescription string? - The job description
- IsCoverLetterMandatory boolean? - Indicates whether the cover letter is mandatory or not
- IsResumeMandatory boolean? - Indicates whether the resume is mandatory or not
- IsHideSalary boolean? - Indicates whether to hide the salary of the vacancy
- VacancyType string? - The vacancy type
- JobTitle string? - The title of the job
- City string? - The city of the job
- Country string? - The country of the job
- Experience string? - The experience expected
- APIKey string -
- Action string -
- anydata... -
peoplehr: OperationStatus
Represents the response type of an operation
Fields
- Fields Included from *PeopleHRGenericResponse
- Result json - The response of the operation
peoplehr: OtherContact
Represents the other contact
Fields
- OtherContactName ValueDetails? - The other contact name
- Relationship ValueDetails? - The relationship
- OtherContactAddress ValueDetails? - The other contact address
- Telephone ValueDetails? - The telephone
- Mobile ValueDetails? - The mobile
- Comments ValueDetails? - The comments
peoplehr: PeopleHRGenericResponse
Represents common fields of the response
Fields
- isError boolean - Indicates whether the response is an error or not
- Status int - The status of the error
- Message string - The message of the response
peoplehr: QueryDetail
Represents the response type of getQueryByName
operation
Fields
- Fields Included from *PeopleHRGenericResponse
- Result json - The results as an json array
peoplehr: QueryResultGetRequest
Represents the input of GetQueryResult
operation
Fields
- QueryName string - The Query Name
- APIKey string -
- Action string -
- anydata... -
peoplehr: RightToWork
Represents right to work type record
Fields
- DocumentType ValueDetails? - The document type
- DocumentId ValueDetails? - The document ID
- ValidFrom ValueDetails? - The valid from
- ValidTo ValueDetails? - The valid to
- Duration ValueDetails? - The duration
- RightToWorkComments ValueDetails? - The right to work comments
peoplehr: Salary
Represents salary detail type record
Fields
- EffectiveFrom string? - The effective from
- SalaryType string? - The salary type
- PaymentFrequency string? - The payment frequency
- SalaryAmount string? - The salary amount
- TotalSalaryAmount string? - The total salary amount
- Currency string? - The currency
- ChangeReason string? - The change reason
- Comments string? - The comments
- Deductions DeductionsOrEntitlements[]? - The deductions
- Entitlements DeductionsOrEntitlements[]? - The entitlements
peoplehr: SalaryDetailGetResponse
Represents the response of GetSalaryDetail
operation
Fields
- Fields Included from *PeopleHRGenericResponse
- Result Salary[] - Salary Detail
peoplehr: SalaryDetailRequest
Represents salary detail request type record
Fields
- EmployeeId string - The employee ID
- IsIncludeHistory boolean - Indicates whether to include the history
- APIKey string -
- Action string -
- anydata... -
peoplehr: ScreenDetailByEmployeeIDRequest
Represents request details for retrieving screen details by employee ID
Fields
- EmployeeId string - EmployeeId for employee custom screen
- ScreenId int - ScreenId for employee custom screen
peoplehr: ScreenDetailByTransactionIDRequest
Represents request details for retrieving screen details by transaction ID
Fields
- Fields Included from *ScreenDetailByEmployeeIDRequest
- CustomScreenTransactionId int - CustomScreenTransactionId for employee custom screen
peoplehr: Vacancy
Represents a vacancy record
Fields
- VacancyName string? - The vacancy Name
- VacancyDescription string? - The vacancy detail
- Company string? - The company
- Location string? - The location of the vacancy
- Department string? - The department of the vacancy
- Status int? - The status of the vacancy
- ClosingDate string? - The closing date
- Reference string? - The reference
- SalaryRange string? - The salary range of the job
- JobDescription string? - The job detail
- IsHideSalary boolean? - Indicates whether to hide the salary
- JobTitle string? - The job title
- VacancyType string? - The vacancy type
- City string? - The city of the vacancy
- Country string? - The country of the vacancy
- Experience string? - The experience expected
- JobBordUrl string? - The URL for job details
- AdditionalQuestions json[]? - Additional questions
- InternalQuestions json[]? - Internal questions on the vacancy
peoplehr: VacancyGetResponse
Represents the response of GetVacancy
operation
Fields
- Fields Included from *PeopleHRGenericResponse
- Result Vacancy - The response of the operation
peoplehr: ValueDetails
Represents valueDetails type
Fields
- DisplayValue string? - The display value
- FieldHistory FieldHistory[]? - The field history
- FieldHistoryForJobRole FieldHistory[]? - The field history for job role
- FieldHistoryForEffectiveDate FieldHistoryForEffectiveDate[]? - The field history for effective date
Import
import ballerinax/peoplehr;
Metadata
Released date: about 1 year ago
Version: 2.2.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
Pull count
Total: 211
Current verison: 5
Weekly downloads
Keywords
Human Resources/HRMS
Cost/Paid
Contributors