paypal.payments
Module paypal.payments
API
Definitions
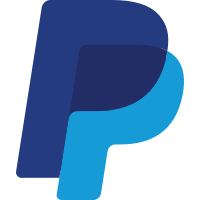
ballerinax/paypal.payments Ballerina library
Overview
This is a generated connector for Paypal Payments API v2 OpenAPI specification. Call the Payments API to authorize payments, capture authorized payments, refund payments that have already been captured, and show payment information. Use the Payments API in conjunction with the Orders API.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Paypal account
- Obtain tokens by following this guide
Quickstart
To use the Paypal Payments connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/paypal.payments
module into the Ballerina project.
import ballerinax/paypal.payments;
Step 2: Create a new connector instance
Create a payments:ClientConfig
with the Bearer_Token
obtained, and initialize the connector with it.
payments:ClientConfig clientConfig = { auth: { token: <Bearer_Token> } }; payments:Client baseClient = check new Client(clientConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to retrieve details for an authorized payment using the connector.
Get details for an authorized payment
public function main() returns error? { payments:AuthorizationDetails response = check baseClient->getAuthorization(<AuthorizationId>); log:printInfo(response.toString()); }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
paypal.payments: Client
This is a generated connector for Paypal Payments API v2 OpenAPI specification. Call the Payments API to authorize payments, capture authorized payments, refund payments that have already been captured, and show payment information. Use the Payments API in conjunction with the Orders API.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Paypal account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string - URL of the target service
getAuthorization
function getAuthorization(string authorizationId, int? count, int? endTime, int? page, int? pageSize, boolean? totalCountRequired, string? sortBy, string? sortOrder, string? startId, int? startIndex, string? startTime) returns AuthorizationDetails|error
Shows details for an authorized payment, by ID.
Parameters
- authorizationId string - The ID of the authorized payment for which to show details.
- count int? (default ()) - The number of items to list in the response.
- endTime int? (default ()) - The end date and time for the range to show in the response, in Internet date and time format. For example, end_time=2016-03-06T11:00:00Z.
- page int? (default ()) - The page number indicating which set of items will be returned in the response. So, the combination of page=1 and page_size=20 returns the first 20 items. The combination of page=2 and page_size=20 returns items 21 through 40.
- pageSize int? (default ()) - The number of items to return in the response.
- totalCountRequired boolean? (default ()) - Indicates whether to show the total count in the response.
- sortBy string? (default ()) - Sorts the payments in the response by a specified value, such as the create time or update time.
- sortOrder string? (default ()) - Sorts the items in the response in ascending or descending order.
- startId string? (default ()) - The ID of the starting resource in the response. When results are paged, you can use the next_id value as the start_id to continue with the next set of results.
- startIndex int? (default ()) - The start index of the payments to list. Typically, you use the start_index to jump to a specific position in the resource history based on its cart. For example, to start at the second item in a list of results, specify ?start_index=2.
- startTime string? (default ()) - The start date and time for the range to show in the response, in Internet date and time format. For example, start_time=2016-03-06T11:00:00Z.
Return Type
- AuthorizationDetails|error - Authorization details.
captureAuthorization
function captureAuthorization(string authorizationId, CaptureAuthorizationRequest payload, string? paypalRequestId, string? prefer) returns CapturedPaymentDetails|error
Captures an authorized payment, by ID.
Parameters
- authorizationId string - The PayPal-generated ID for the authorized payment to capture.
- payload CaptureAuthorizationRequest - The capture authorization request
- paypalRequestId string? (default ()) - The server stores keys for 45 days.
- prefer string? (default ()) - The preferred server response upon successful completion of the request. Value is return=minimal or return=representation.
Return Type
- CapturedPaymentDetails|error - Captured payment details
reauthorizeAuthorization
function reauthorizeAuthorization(string authorizationId, ReauthorizeAuthorizationRequest payload, string? paypalRequestId, string? prefer) returns ReauthorizedPaymentDetails|error
Reauthorizes an authorized PayPal account payment, by ID. To ensure that funds are still available, reauthorize a payment after its initial three-day honor period expires. Within the 29-day authorization period, you can issue multiple re-authorizations after the honor period expires. If 30 days have transpired since the date of the original authorization, you must create an authorized payment instead of reauthorizing the original authorized payment. A reauthorized payment itself has a new honor period of three days. You can reauthorize an authorized payment once for up to 115% of the original authorized amount, not to exceed an increase of $75 USD.
Parameters
- authorizationId string - The PayPal-generated ID for the authorized payment to capture.
- payload ReauthorizeAuthorizationRequest - The reauthorize authorization request
- paypalRequestId string? (default ()) - The server stores keys for 45 days.
- prefer string? (default ()) - The preferred server response upon successful completion of the request. Value is return=minimal or return=representation.
Return Type
- ReauthorizedPaymentDetails|error - Reauthorized payment details
voidAuthorization
function voidAuthorization(string authorizationId, string? paypalAuthAssertion) returns Response|error
Voids, or cancels, an authorized payment, by ID. You cannot void an authorized payment that has been fully captured.
Parameters
- authorizationId string - The PayPal-generated ID for the authorized payment to capture.
- paypalAuthAssertion string? (default ()) - An API-caller-provided JSON Web Token (JWT) assertion that identifies the merchant. For details, see PayPal-Auth-Assertion. For three party transactions in which a partner is managing the API calls on behalf of a merchant, the partner must identify the merchant using either a PayPal-Auth-Assertion header or an access token with target_subject.
getCaptures
function getCaptures(string captureId) returns CapturedPaymentDetails|error
Shows details for a captured payment, by ID.
Parameters
- captureId string - The PayPal-generated ID for the captured payment for which to show details.
Return Type
- CapturedPaymentDetails|error - Captured payment details.
refundCaptures
function refundCaptures(string captureId, RefundCaptureRequest payload, string? paypalAuthAssertion, string? paypalRequestId, string? prefer) returns RefundDetails|error
Refunds a captured payment, by ID. For a full refund, include an empty payload in the JSON request body. For a partial refund, include an amount object in the JSON request body.
Parameters
- captureId string - The PayPal-generated ID for the captured payment for which to show details.
- payload RefundCaptureRequest - The refund capture request
- paypalAuthAssertion string? (default ()) - An API-caller-provided JSON Web Token (JWT) assertion that identifies the merchant. For details, see PayPal-Auth-Assertion. For three party transactions in which a partner is managing the API calls on behalf of a merchant, the partner must identify the merchant using either a PayPal-Auth-Assertion header or an access token with target_subject.
- paypalRequestId string? (default ()) - The server stores keys for 45 days.
- prefer string? (default ()) - The preferred server response upon successful completion of the request. Value is return=minimal or return=representation.
Return Type
- RefundDetails|error - Refund details
getRefunds
function getRefunds(string refundId) returns RefundDetails|error
Shows details for a refund, by ID.
Parameters
- refundId string - The PayPal-generated ID for the refund for which to show details.
Return Type
- RefundDetails|error - Refund details.
Records
paypal.payments: Amount
The amount for this captured payment.
Fields
- reason string? - The reason why the captured payment status is PENDING or DENIED. The possible values are BUYER_COMPLAINT, CHARGEBACK, ECHECK, INTERNATIONAL_WITHDRAWAL, OTHER, PENDING_REVIEW, RECEIVING_PREFERENCE_MANDATES_MANUAL_ACTION, REFUNDED, TRANSACTION_APPROVED_AWAITING_FUNDING, UNILATERAL, VERIFICATION_REQUIRED.
paypal.payments: AuthorizationDetails
Authorization details.
Fields
- status string? - The status for the authorized payment. The possible values are CREATED, CAPTURED, DENIED, EXPIRED, PARTIALLY_CAPTURED, PARTIALLY_CREATED, VOIDED, PENDING.
- status_details AuthorizationStatusDetails? - The details of the authorized order pending status.
- id string? - The PayPal-generated ID for the authorized payment.
- amount Money? - The amount for this authorized payment.
- invoice_id string? - The API caller-provided external invoice number for this order. Appears in both the payer's transaction history and the emails that the payer receives.
- custom_id string? - The API caller-provided external ID. Used to reconcile API caller-initiated transactions with PayPal transactions. Appears in transaction and settlement reports.
- seller_protection SellerProtection? - The level of protection offered as defined by PayPal Seller Protection for Merchants.
- expiration_time string? - The date and time when the authorized payment expires, in Internet date and time format.
- links LinkDescription[]? - An array of related HATEOAS links.
- create_time string? - The date and time when the transaction occurred, in Internet date and time format.
- update_time string? - The date and time when the transaction was last updated, in Internet date and time format.
paypal.payments: AuthorizationStatusDetails
The details of the authorized order pending status.
Fields
- reason string? - The reason why the authorized status is PENDING. The possible values are PENDING_REVIEW.
paypal.payments: CaptureAuthorizationRequest
Capture authorization request
Fields
- invoice_id string? - The API caller-provided external invoice number for this order. Appears in both the payer's transaction history and the emails that the payer receives.
- note_to_payer string? - An informational note about this settlement. Appears in both the payer's transaction history and the emails that the payer receives.
- amount MoneyForCaptureAuthorizationRequest? - The amount to capture. To capture a portion of the full authorized amount, specify an amount. If amount is not specified, the full authorized amount is captured. The amount must be a positive number and in the same currency as the authorization against which the payment is being captured.
- final_capture boolean? - Indicates whether you can make additional captures against the authorized payment. Set to true if you do not intend to capture additional payments against the authorization. Set to false if you intend to capture additional payments against the authorization.
- payment_instruction PaymentInstruction? - Any additional payment instructions to be consider during payment processing. This processing instruction is applicable for Capturing an order or Authorizing an Order.
- soft_descriptor string? - The payment descriptor on the payer's account statement.
paypal.payments: CapturedPaymentDetails
Captured payment details.
Fields
- status string? - The status of the captured payment. The possible values are COMPLETED, DECLINED, PARTIALLY_REFUNDED, PENDING, REFUNDED, FAILED.
- status_details CaptureStatusDetails? - The details of the captured payment status.
- id string? - The PayPal-generated ID for the captured payment.
- amount MoneyForAmount? - The amount for this captured payment.
- invoice_id string? - The API caller-provided external invoice number for this order. Appears in both the payer's transaction history and the emails that the payer receives.
- custom_id string? - The API caller-provided external ID. Used to reconcile API caller-initiated transactions with PayPal transactions. Appears in transaction and settlement reports.
- seller_protection SellerProtectionForCaptureAuthorizationResponse? - The level of protection offered as defined by PayPal Seller Protection for Merchants
- final_capture boolean? - Indicates whether you can make additional captures against the authorized payment. Set to true if you do not intend to capture additional payments against the authorization. Set to false if you intend to capture additional payments against the authorization.
- seller_receivable_breakdown SellerReceivableBreakdown? - The detailed breakdown of the capture activity. This is not available for transactions that are in pending state.
- disbursement_mode string? - The funds that are held on behalf of the merchant. The possible values are INSTANT, DELAYED.
- links LinkDescription[]? - An array of related HATEOAS links.
- processor_response ProcessorResponse? - An object that provides additional processor information for a direct credit card transaction.
- create_time string? - The date and time when the transaction occurred, in Internet date and time format.
- update_time string? - The date and time when the transaction was last updated, in Internet date and time format.
paypal.payments: CaptureStatusDetails
The details of the captured payment status.
Fields
- reason string? - The reason why the captured payment status is PENDING or DENIED. The possible values are BUYER_COMPLAINT, CHARGEBACK, ECHECK, INTERNATIONAL_WITHDRAWAL, OTHER, PENDING_REVIEW, RECEIVING_PREFERENCE_MANDATES_MANUAL_ACTION, REFUNDED, TRANSACTION_APPROVED_AWAITING_FUNDING, UNILATERAL, VERIFICATION_REQUIRED.
paypal.payments: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
paypal.payments: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
paypal.payments: DisputeCategory
The condition that is covered for the transaction.
Fields
- dispute_category string? - The condition that is covered for the transaction. The possible values are ITEM_NOT_RECEIVED, UNAUTHORIZED_TRANSACTION.
paypal.payments: ExchangeRate
The exchange rate that determines the amount that is credited to the payee's PayPal account. Returned when the currency of the captured payment is different from the currency of the PayPal account where the payee wants to credit the funds.
Fields
- source_currency string? - The source currency from which to convert an amount.
- target_currency string? - The target currency to which to convert an amount.
- value string? - The target currency amount. Equivalent to one unit of the source currency. Formatted as integer or decimal value with one to 15 digits to the right of the decimal point.
paypal.payments: ExchangeRateForNetAmountBreakdown
The exchange rate that determines the amount that was debited from the merchant's PayPal account.
Fields
- source_currency string? - The source currency from which to convert an amount.
- target_currency string? - The target currency to which to convert an amount.
- value string? - The target currency amount. Equivalent to one unit of the source currency. Formatted as integer or decimal value with one to 15 digits to the right of the decimal point.
paypal.payments: LinkDescription
Related HATEOAS links.
Fields
- href string - The complete target URL. To make the related call, combine the method with this URI Template-formatted link. For pre-processing, include the $, (, and ) characters. The href is the key HATEOAS component that links a completed call with a subsequent call.
- rel string - The link relation type, which serves as an ID for a link that unambiguously describes the semantics of the link. See Link Relations.
- method string? - The HTTP method required to make the related call. Possible values are GET,POST,PUT,DELETE,HEAD,CONNECT,OPTIONS,PATCH.
paypal.payments: Money
The amount for this authorized payment.
Fields
- currency_code string - The three-character ISO-4217 currency code that identifies the currency.
- value string - The value, which might be an integer for currencies like JPY that are not typically fractional. A decimal fraction for currencies like TND that are subdivided into thousandths. For the required number of decimal places for a currency code, see Currency Codes.
paypal.payments: MoneyForAmount
The amount for this captured payment.
Fields
- currency_code string - The three-character ISO-4217 currency code that identifies the currency.
- value string - The value, which might be an integer for currencies like JPY that are not typically fractional. A decimal fraction for currencies like TND that are subdivided into thousandths. For the required number of decimal places for a currency code, see Currency Codes.
paypal.payments: MoneyForCaptureAuthorizationRequest
The amount to capture. To capture a portion of the full authorized amount, specify an amount. If amount is not specified, the full authorized amount is captured. The amount must be a positive number and in the same currency as the authorization against which the payment is being captured.
Fields
- currency_code string - The three-character ISO-4217 currency code that identifies the currency.
- value string - The value, which might be an integer for currencies like JPY that are not typically fractional. A decimal fraction for currencies like TND that are subdivided into thousandths. For the required number of decimal places for a currency code, see Currency Codes.
paypal.payments: MoneyForConvertedAmount
The converted payable amount.
Fields
- currency_code string - The three-character ISO-4217 currency code that identifies the currency.
- value string - The value, which might be an integer for currencies like JPY that are not typically fractional. A decimal fraction for currencies like TND that are subdivided into thousandths. For the required number of decimal places for a currency code, see Currency Codes.
paypal.payments: MoneyForGrossAmount
The amount for this captured payment in the currency of the transaction.
Fields
- currency_code string - The three-character ISO-4217 currency code that identifies the currency.
- value string - The value, which might be an integer for currencies like JPY that are not typically fractional. A decimal fraction for currencies like TND that are subdivided into thousandths. For the required number of decimal places for a currency code, see Currency Codes.
paypal.payments: MoneyForGrossAmountForSellerPayableBreakdown
The amount that the payee refunded to the payer.
Fields
- currency_code string - The three-character ISO-4217 currency code that identifies the currency.
- value string - The value, which might be an integer for currencies like JPY that are not typically fractional. A decimal fraction for currencies like TND that are subdivided into thousandths. For the required number of decimal places for a currency code, see Currency Codes.
paypal.payments: MoneyForNetAmount
The net amount that is credited to the payee's PayPal account. Returned only when the currency of the captured payment is different from the currency of the PayPal account where the payee wants to credit the funds. The amount is computed as net_amount times exchange_rate.
Fields
- currency_code string - The three-character ISO-4217 currency code that identifies the currency.
- value string - The value, which might be an integer for currencies like JPY that are not typically fractional. A decimal fraction for currencies like TND that are subdivided into thousandths. For the required number of decimal places for a currency code, see Currency Codes.
paypal.payments: MoneyForNetAmountForSellerPayableBreakdown
The net amount that the payee's account is debited in the transaction currency. The net amount is calculated as gross_amount minus paypal_fee minus platform_fees.
Fields
- currency_code string - The three-character ISO-4217 currency code that identifies the currency.
- value string - The value, which might be an integer for currencies like JPY that are not typically fractional. A decimal fraction for currencies like TND that are subdivided into thousandths. For the required number of decimal places for a currency code, see Currency Codes.
paypal.payments: MoneyForNetAmountInReceivableCurrencyForSellerPayableBreakdown
The net amount that the payee's account is debited in the receivable currency. Returned only in cases when the receivable currency is different from transaction currency. Example 'CNY'.
Fields
- currency_code string - The three-character ISO-4217 currency code that identifies the currency.
- value string - The value, which might be an integer for currencies like JPY that are not typically fractional. A decimal fraction for currencies like TND that are subdivided into thousandths. For the required number of decimal places for a currency code, see Currency Codes.
paypal.payments: MoneyForPayableAmount
The net amount debited from the merchant's PayPal account.
Fields
- currency_code string - The three-character ISO-4217 currency code that identifies the currency.
- value string - The value, which might be an integer for currencies like JPY that are not typically fractional. A decimal fraction for currencies like TND that are subdivided into thousandths. For the required number of decimal places for a currency code, see Currency Codes.
paypal.payments: MoneyForPaypalFee
The applicable fee for this captured payment in the currency of the transaction.
Fields
- currency_code string - The three-character ISO-4217 currency code that identifies the currency.
- value string - The value, which might be an integer for currencies like JPY that are not typically fractional. A decimal fraction for currencies like TND that are subdivided into thousandths. For the required number of decimal places for a currency code, see Currency Codes.
paypal.payments: MoneyForPaypalFeeForSellerPayableBreakdown
The PayPal fee that was refunded to the payer in the currency of the transaction. This fee might not match the PayPal fee that the payee paid when the payment was captured.
Fields
- currency_code string - The three-character ISO-4217 currency code that identifies the currency.
- value string - The value, which might be an integer for currencies like JPY that are not typically fractional. A decimal fraction for currencies like TND that are subdivided into thousandths. For the required number of decimal places for a currency code, see Currency Codes.
paypal.payments: MoneyForPaypalFeeInReceivableCurrency
The applicable fee for this captured payment in the receivable currency. Returned only in cases the fee is charged in the receivable currency. Example 'CNY'.
Fields
- currency_code string - The three-character ISO-4217 currency code that identifies the currency.
- value string - The value, which might be an integer for currencies like JPY that are not typically fractional. A decimal fraction for currencies like TND that are subdivided into thousandths. For the required number of decimal places for a currency code, see Currency Codes.
paypal.payments: MoneyForPaypalFeeInReceivableCurrencyForSellerPayableBreakdown
The PayPal fee that was refunded to the payer in the receivable currency. Returned only in cases when the receivable currency is different from transaction currency. Example 'CNY'.
Fields
- currency_code string - The three-character ISO-4217 currency code that identifies the currency.
- value string - The value, which might be an integer for currencies like JPY that are not typically fractional. A decimal fraction for currencies like TND that are subdivided into thousandths. For the required number of decimal places for a currency code, see Currency Codes.
paypal.payments: MoneyForPlatformFee
The fee for this transaction.
Fields
- currency_code string - The three-character ISO-4217 currency code that identifies the currency.
- value string - The value, which might be an integer for currencies like JPY that are not typically fractional. A decimal fraction for currencies like TND that are subdivided into thousandths. For the required number of decimal places for a currency code, see Currency Codes.
paypal.payments: MoneyForReceivableAmount
The net amount that the payee receives for this captured payment in their PayPal account. The net amount is computed as gross_amount minus the paypal_fee minus the platform_fees.
Fields
- currency_code string - The three-character ISO-4217 currency code that identifies the currency.
- value string - The value, which might be an integer for currencies like JPY that are not typically fractional. A decimal fraction for currencies like TND that are subdivided into thousandths. For the required number of decimal places for a currency code, see Currency Codes.
paypal.payments: MoneyForRefundCaptureRequest
The amount to refund. To refund a portion of the captured amount, specify an amount. If amount is not specified, an amount equal to captured amount - previous refunds is refunded. The amount must be a positive number and in the same currency as the one in which the payment was captured.
Fields
- currency_code string - The three-character ISO-4217 currency code that identifies the currency.
- value string - The value, which might be an integer for currencies like JPY that are not typically fractional. A decimal fraction for currencies like TND that are subdivided into thousandths. For the required number of decimal places for a currency code, see Currency Codes.
paypal.payments: MoneyForRefundDetails
The amount that the payee refunded to the payer.
Fields
- currency_code string - The three-character ISO-4217 currency code that identifies the currency.
- value string - The value, which might be an integer for currencies like JPY that are not typically fractional. A decimal fraction for currencies like TND that are subdivided into thousandths. For the required number of decimal places for a currency code, see Currency Codes.
paypal.payments: MoneytForReauthorizeAuthorizationRequest
The amount to reauthorize for an authorized payment.
Fields
- currency_code string - The three-character ISO-4217 currency code that identifies the currency.
- value string - The value, which might be an integer for currencies like JPY that are not typically fractional. A decimal fraction for currencies like TND that are subdivided into thousandths. For the required number of decimal places for a currency code, see Currency Codes.
paypal.payments: NetAmountBreakdown
Breakdown value for the net amount. Returned when the currency of the refund is different from the currency of the PayPal account where the payee holds their funds.
Fields
- payable_amount MoneyForPayableAmount? - The net amount debited from the merchant's PayPal account.
- converted_amount MoneyForConvertedAmount? - The converted payable amount.
- exchange_rate ExchangeRateForNetAmountBreakdown? - The exchange rate that determines the amount that was debited from the merchant's PayPal account.
paypal.payments: PayeeBase
The recipient of the fee for this transaction. If you omit this value, the default is the API caller.
Fields
- email_address string? - The email address of merchant.
- merchant_id string? - The encrypted PayPal account ID of the merchant.
paypal.payments: PaymentInstruction
Any additional payment instructions to be consider during payment processing. This processing instruction is applicable for Capturing an order or Authorizing an Order.
Fields
- platform_fees PlatformFee[]? - An array of various fees, commissions, tips, or donations. This field is only applicable to merchants that been enabled for PayPal Commerce Platform for Marketplaces and Platforms capability.
- disbursement_mode string? - The funds that are held on behalf of the merchant.
- payee_pricing_tier_id string? - This field is only enabled for selected merchants/partners to use and provides the ability to trigger a specific pricing rate/plan for a payment transaction. The list of eligible 'payee_pricing_tier_id' would be provided to you by your Account Manager. Specifying values other than the one provided to you by your account manager would result in an error.
paypal.payments: PlatformFee
Various fees, commissions, tips, or donations.
Fields
- amount MoneyForPlatformFee - The fee for this transaction.
- payee PayeeBase? - The recipient of the fee for this transaction. If you omit this value, the default is the API caller.
paypal.payments: PlatformFeeForGrossAmount
Platform or partner fees, commissions, or brokerage fees that associated with the captured payment.
Fields
- amount MoneyForPlatformFee - The fee for this transaction.
- payee PayeeBase? - The recipient of the fee for this transaction. If you omit this value, the default is the API caller.
paypal.payments: PlatformFeeForGrossAmountForSellerPayableBreakdown
Platform or partner fees, commissions, or brokerage fees for the refund.
Fields
- amount MoneyForPlatformFee - The fee for this transaction.
- payee PayeeBase? - The recipient of the fee for this transaction. If you omit this value, the default is the API caller.
paypal.payments: ProcessorResponse
An object that provides additional processor information for a direct credit card transaction.
Fields
- avs_code string? - The address verification code for Visa, Discover, Mastercard, or American Express transactions.
- cvv_code string? - The card verification value code for for Visa, Discover, Mastercard, or American Express.
- response_code string? - Processor response code for the non-PayPal payment processor errors.
- payment_advice_code string? - The declined payment transactions might have payment advice codes. The card networks, like Visa and Mastercard, return payment advice codes.
paypal.payments: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
paypal.payments: ReauthorizeAuthorizationRequest
Reauthorize authorization request
Fields
- amount MoneytForReauthorizeAuthorizationRequest? - The amount to reauthorize for an authorized payment.
paypal.payments: ReauthorizedPaymentDetails
Reauthorized payment details.
Fields
- status string? - The status for the authorized payment. The possible values are CREATED, CAPTURED, DENIED, EXPIRED, PARTIALLY_CAPTURED, PARTIALLY_CREATED, VOIDED, PENDING.
- status_details AuthorizationStatusDetails? - The details of the authorized order pending status.
- id string? - The PayPal-generated ID for the authorized payment.
- amount Money? - The amount for this authorized payment.
- invoice_id string? - The API caller-provided external invoice number for this order. Appears in both the payer's transaction history and the emails that the payer receives.
- custom_id string? - The API caller-provided external ID. Used to reconcile API caller-initiated transactions with PayPal transactions. Appears in transaction and settlement reports.
- seller_protection SellerProtection? - The level of protection offered as defined by PayPal Seller Protection for Merchants.
- expiration_time string? - The date and time when the authorized payment expires, in Internet date and time format.
- links LinkDescription[]? - An array of related HATEOAS links.
- create_time string? - The date and time when the transaction occurred, in Internet date and time format.
- update_time string? - The date and time when the transaction was last updated, in Internet date and time format.
paypal.payments: RefundCaptureRequest
Refund capture request
Fields
- amount MoneyForRefundCaptureRequest? - The amount to refund. To refund a portion of the captured amount, specify an amount. If amount is not specified, an amount equal to captured amount - previous refunds is refunded. The amount must be a positive number and in the same currency as the one in which the payment was captured.
- invoice_id string? - The API caller-provided external invoice number for this order. Appears in both the payer's transaction history and the emails that the payer receives.
- note_to_payer string? - The reason for the refund. Appears in both the payer's transaction history and the emails that the payer receives.
paypal.payments: RefundDetails
Refund details.
Fields
- status string? - The status of the refund. The possible values are CANCELLED, PENDING, COMPLETED.
- status_details RefundStatusDetails? - The details of the refund status.
- id string? - The PayPal-generated ID for the refund.
- amount MoneyForRefundDetails? - The amount that the payee refunded to the payer.
- invoice_id string? - The API caller-provided external invoice number for this order. Appears in both the payer's transaction history and the emails that the payer receives.
- note_to_payer string? - The reason for the refund. Appears in both the payer's transaction history and the emails that the payer receives.
- seller_payable_breakdown SellerPayableBreakdown? - The breakdown of the refund.
- links LinkDescription[]? - An array of related HATEOAS links.
- create_time string? - The date and time when the transaction occurred, in Internet date and time format.
- update_time string? - The date and time when the transaction was last updated, in Internet date and time format.
paypal.payments: RefundStatusDetails
The details of the refund status.
Fields
- reason string? - The reason why the refund has the PENDING or FAILED status. The possible values are ECHECK.
paypal.payments: SellerPayableBreakdown
The breakdown of the refund.
Fields
- gross_amount MoneyForGrossAmountForSellerPayableBreakdown - The amount that the payee refunded to the payer.
- paypal_fee MoneyForPaypalFeeForSellerPayableBreakdown? - The PayPal fee that was refunded to the payer in the currency of the transaction. This fee might not match the PayPal fee that the payee paid when the payment was captured.
- paypal_fee_in_receivable_currency MoneyForPaypalFeeInReceivableCurrencyForSellerPayableBreakdown? - The PayPal fee that was refunded to the payer in the receivable currency. Returned only in cases when the receivable currency is different from transaction currency. Example 'CNY'.
- net_amount MoneyForNetAmountForSellerPayableBreakdown? - The net amount that the payee's account is debited in the transaction currency. The net amount is calculated as gross_amount minus paypal_fee minus platform_fees.
- net_amount_in_receivable_currency MoneyForNetAmountInReceivableCurrencyForSellerPayableBreakdown? - The net amount that the payee's account is debited in the receivable currency. Returned only in cases when the receivable currency is different from transaction currency. Example 'CNY'.
- platform_fees PlatformFeeForGrossAmountForSellerPayableBreakdown[]? - An array of platform or partner fees, commissions, or brokerage fees for the refund.
- net_amount_breakdown NetAmountBreakdown[]? - An array of breakdown values for the net amount. Returned when the currency of the refund is different from the currency of the PayPal account where the payee holds their funds.
- total_refunded_amount TotalRefundedAmountForSellerPayableBreakdown? - The total amount refunded from the original capture to date. For example, if a payer makes a $100 purchase and was refunded $20 a week ago and was refunded $30 in this refund, the gross_amount is $30 for this refund and the total_refunded_amount is $50.
paypal.payments: SellerProtection
The level of protection offered as defined by PayPal Seller Protection for Merchants.
Fields
- status string? - Indicates whether the transaction is eligible for seller protection. For information, see PayPal Seller Protection for Merchants. The possible values are ELIGIBLE, PARTIALLY_ELIGIBLE, NOT_ELIGIBLE.
- dispute_categories DisputeCategory[]? - An array of conditions that are covered for the transaction.
paypal.payments: SellerProtectionForCaptureAuthorizationResponse
The level of protection offered as defined by PayPal Seller Protection for Merchants
Fields
- status string? - Indicates whether the transaction is eligible for seller protection. For information, see PayPal Seller Protection for Merchants. The possible values are ELIGIBLE, PARTIALLY_ELIGIBLE, NOT_ELIGIBLE.
- dispute_categories DisputeCategory[]? - An array of conditions that are covered for the transaction.
paypal.payments: SellerReceivableBreakdown
The detailed breakdown of the capture activity. This is not available for transactions that are in pending state.
Fields
- gross_amount MoneyForGrossAmount - The amount for this captured payment in the currency of the transaction.
- paypal_fee MoneyForPaypalFee? - The applicable fee for this captured payment in the currency of the transaction.
- paypal_fee_in_receivable_currency MoneyForPaypalFeeInReceivableCurrency? - The applicable fee for this captured payment in the receivable currency. Returned only in cases the fee is charged in the receivable currency. Example 'CNY'.
- net_amount MoneyForNetAmount? - The net amount that is credited to the payee's PayPal account. Returned only when the currency of the captured payment is different from the currency of the PayPal account where the payee wants to credit the funds. The amount is computed as net_amount times exchange_rate.
- receivable_amount MoneyForReceivableAmount? - The net amount that the payee receives for this captured payment in their PayPal account. The net amount is computed as gross_amount minus the paypal_fee minus the platform_fees.
- exchange_rate ExchangeRate? - The exchange rate that determines the amount that is credited to the payee's PayPal account. Returned when the currency of the captured payment is different from the currency of the PayPal account where the payee wants to credit the funds.
- platform_fees PlatformFeeForGrossAmount[]? - An array of platform or partner fees, commissions, or brokerage fees that associated with the captured payment.
paypal.payments: TotalRefundedAmountForSellerPayableBreakdown
The total amount refunded from the original capture to date. For example, if a payer makes a $100 purchase and was refunded $20 a week ago and was refunded $30 in this refund, the gross_amount is $30 for this refund and the total_refunded_amount is $50.
Fields
- amount MoneyForPlatformFee - The fee for this transaction.
- payee PayeeBase? - The recipient of the fee for this transaction. If you omit this value, the default is the API caller.
Import
import ballerinax/paypal.payments;
Metadata
Released date: about 2 years ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 7
Current verison: 7
Weekly downloads
Keywords
Finance/Payment
Cost/Freemium
Contributors
Dependencies