paypal.orders
Module paypal.orders
Definitions
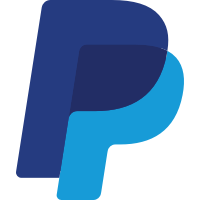
ballerinax/paypal.orders Ballerina library
Overview
This is a generated connector for Paypal Orders API v2 OpenAPI specification. An order represents a payment between two or more parties. Use the Orders API to create, update, retrieve, authorize, and capture orders.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Paypal account
- Obtain tokens by following this guide
Quickstart
To use the Paypal Orders connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/paypal.orders
module into the Ballerina project.
import ballerinax/paypal.orders;
Step 2: Create a new connector instance
Create a orders:ClientConfig
with the Bearer_Token
obtained, and initialize the connector with it.
orders:ClientConfig clientConfig = { auth: { token: <Bearer_Token> } }; orders:Client baseClient = check new Client(clientConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to create an order using the connector.
Creates an order
public function main() returns error? { orders:CreateOrderRequest createOrderRequest = { intent: "CAPTURE", purchase_units: [ { amount: { currency_code: "USD", value: "100.00" } } ] }; orders:CreatedOrderDetails response = check baseClient->createOrder(createOrderRequest); log:printInfo(response.toString()); }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
paypal.orders: Client
This is a generated connector for Paypal Orders API v2 OpenAPI specification. An order represents a payment between two or more parties. Use the Orders API to create, update, retrieve, authorize, and capture orders.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Paypal account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string - URL of the target service
createOrder
function createOrder(CreateOrderRequest payload, string? paypalRequestId, string? paypalPartnerAttributionId, string? paypalClientMetadataId, string? prefer) returns CreatedOrderDetails|error
Creates an order.
Parameters
- payload CreateOrderRequest - The create order request
- paypalRequestId string? (default ()) - The server stores keys for 6 hours. The API callers can request the times to up to 72 hours by speaking to their Account Manager.
- paypalPartnerAttributionId string? (default ()) - PayPal partner attribution ID
- paypalClientMetadataId string? (default ()) - PayPal client metadata ID
- prefer string? (default ()) - The preferred server response upon successful completion of the request. Value is return=minimal or return=representation.
Return Type
- CreatedOrderDetails|error - Created order details
getOrder
function getOrder(string id, string? fields) returns OrderDetails|error
Shows details for an order, by ID.
Parameters
- id string - The ID of the order for which to show details."
- fields string? (default ()) - A comma-separated list of fields that should be returned for the order. Valid filter field is payment_source.
Return Type
- OrderDetails|error - Order details.
updateOrder
function updateOrder(string id, UpdateOrderRequest payload) returns Response|error
Updates an order with a CREATED or APPROVED status. You cannot update an order with the COMPLETED status. To make an update, you must provide a reference_id. If you omit this value with an order that contains only one purchase unit, PayPal sets the value to default which enables you to use the path: /purchase_units/@reference_id=='default'/{attribute-or-object}.
Parameters
- id string - The ID of the order to update.
- payload UpdateOrderRequest - The update order request
authorizeOrder
function authorizeOrder(string id, AuthorizeOrderRequest payload, string? paypalRequestId, string? prefer, string? paypalClientMetadataId, string? paypalAuthAssertion) returns AuthorizedOrderDetails|error
Authorizes payment for an order. To successfully authorize payment for an order, the buyer must first approve the order or a valid payment_source must be provided in the request. A buyer can approve the order upon being redirected to the rel:approve URL that was returned in the HATEOAS links in the create order response.
Parameters
- id string - The ID of the order for which to authorize.
- payload AuthorizeOrderRequest - The authorize order request
- paypalRequestId string? (default ()) - The server stores keys for 6 hours. The API callers can request the times to up to 72 hours by speaking to their Account Manager.
- prefer string? (default ()) - The preferred server response upon successful completion of the request. Value is return=minimal or return=representation.
- paypalClientMetadataId string? (default ()) - PayPal client metadata ID
- paypalAuthAssertion string? (default ()) - PayPal auth assertion
Return Type
- AuthorizedOrderDetails|error - Authorized order details
captureOrder
function captureOrder(string id, CaptureOrderRequest payload, string? paypalRequestId, string? prefer, string? paypalClientMetadataId, string? paypalAuthAssertion) returns CapturedPaymentDetails|error
Captures payment for an order. To successfully capture payment for an order, the buyer must first approve the order or a valid payment_source must be provided in the request. A buyer can approve the order upon being redirected to the rel:approve URL that was returned in the HATEOAS links in the create order response.
Parameters
- id string - The ID of the order for which to capture a payment.
- payload CaptureOrderRequest - The capture order request
- paypalRequestId string? (default ()) - The server stores keys for 6 hours. The API callers can request the times to up to 72 hours by speaking to their Account Manager.
- prefer string? (default ()) - The preferred server response upon successful completion of the request. Value is return=minimal or return=representation.
- paypalClientMetadataId string? (default ()) - PayPal client metadata ID
- paypalAuthAssertion string? (default ()) - PayPal auth assertion
Return Type
- CapturedPaymentDetails|error - Captured payment details
Records
paypal.orders: Amount
The total order amount with an optional breakdown that provides details, such as the total item amount, total tax amount, shipping, handling, insurance, and discounts, if any. If you specify amount.breakdown, the amount equals item_total plus tax_total plus shipping plus handling plus insurance minus shipping_discount minus discount. The amount must be a positive number. For listed of supported currencies and decimal precision, see the PayPal REST APIs Currency Codes.
Fields
- currency_code string - The three-character ISO-4217 currency code that identifies the currency.
- value string - The value, which might be an integer for currencies like JPY that are not typically fractional. A decimal fraction for currencies like TND that are subdivided into thousandths. For the required number of decimal places for a currency code, see Currency Codes.
paypal.orders: AuthenticationResponse
Results of Authentication such as 3D Secure.
Fields
- liability_shift string? - Liability shift indicator. The outcome of the issuer's authentication. The possible values are YES, NO, POSSIBLE, UNKNOWN.
- three_d_secure ThreeDSecureAuthenticationResponse? - Results of 3D Secure Authentication.
paypal.orders: AuthorizationWithAdditionalData
An authorized payment for a purchase unit. A purchase unit can have zero or more authorized payments.
Fields
- processor_response ProcessorResponse? - The processor response for card transactions.
paypal.orders: AuthorizedOrderDetails
Authorized order details.
Fields
- create_time string? - The date and time when the transaction occurred, in Internet date and time format.
- update_time string? - The date and time when the transaction was last updated, in Internet date and time format.
- id string? - The ID of the order.
- payment_source PaymentSourceResponse? - The payment source used to fund the payment.
- intent string? - The intent to either capture payment immediately or authorize a payment for an order after order creation. The possible values are CAPTURE, AUTHORIZE.
- payer Payer? - The customer who approves and pays for the order. The customer is also known as the payer.
- purchase_units PurchaseUnit[]? - An array of purchase units. Each purchase unit establishes a contract between a customer and merchant. Each purchase unit represents either a full or partial order that the customer intends to purchase from the merchant.
- status string? - The order status. The possible values are CREATED, SAVED, APPROVED, VOIDED, COMPLETED, PAYER_ACTION_REQUIRED.
- links LinkDescription[]? - An array of request-related HATEOAS links. To complete payer approval, use the approve link to redirect the payer. The API caller has 3 hours (default setting, this which can be changed by your account manager to 24/48/72 hours to accommodate your use case) from the time the order is created, to redirect your payer. Once redirected, the API caller has 3 hours for the payer to approve the order and either authorize or capture the order. If you are not using the PayPal JavaScript SDK to initiate PayPal Checkout (in context) ensure that you include application_context.return_url is specified or you will get "We're sorry, Things don't appear to be working at the moment" after the payer approves the payment.
paypal.orders: AuthorizeOrderRequest
The source of payment for the order, which can be a token or a card. Use this object only if you have not redirected the user after order creation to approve the payment. In such cases, the user-selected payment method in the PayPal flow is implicitly used.
Fields
- token Token? - The tokenized payment source to fund a payment.
paypal.orders: Capture
A captured payment for a purchase unit. A purchase unit can have zero or more captured payments.
Fields
- status string? - The status of the captured payment.
- status_details CaptureStatusDetails? - The details of the captured payment status.
paypal.orders: CapturedPaymentDetails
Captured payment details.
Fields
- create_time string? - The date and time when the transaction occurred, in Internet date and time format.
- update_time string? - The date and time when the transaction was last updated, in Internet date and time format.
- id string? - The ID of the order.
- payment_source PaymentSourceResponse? - The payment source used to fund the payment.
- intent string? - The intent to either capture payment immediately or authorize a payment for an order after order creation. The possible values are CAPTURE, AUTHORIZE.
- payer Payer? - The customer who approves and pays for the order. The customer is also known as the payer.
- purchase_units PurchaseUnit[]? - An array of purchase units. Each purchase unit establishes a contract between a customer and merchant. Each purchase unit represents either a full or partial order that the customer intends to purchase from the merchant.
- status string? - The order status. The possible values are CREATED, SAVED, APPROVED, VOIDED, COMPLETED, PAYER_ACTION_REQUIRED.
- links LinkDescription[]? - An array of request-related HATEOAS links. To complete payer approval, use the approve link to redirect the payer. The API caller has 3 hours (default setting, this which can be changed by your account manager to 24/48/72 hours to accommodate your use case) from the time the order is created, to redirect your payer. Once redirected, the API caller has 3 hours for the payer to approve the order and either authorize or capture the order. If you are not using the PayPal JavaScript SDK to initiate PayPal Checkout (in context) ensure that you include application_context.return_url is specified or you will get "We're sorry, Things don't appear to be working at the moment" after the payer approves the payment.
paypal.orders: CaptureOrderRequest
The payment source definition.
Fields
- token Token? - The tokenized payment source to fund a payment.
paypal.orders: CaptureStatusDetails
The details of the captured payment status.
Fields
- reason string? - The reason why the captured payment status is PENDING or DENIED. The possible values are BUYER_COMPLAINT, CHARGEBACK, ECHECK, INTERNATIONAL_WITHDRAWAL, OTHER, PENDING_REVIEW, RECEIVING_PREFERENCE_MANDATES_MANUAL_ACTION, REFUNDED, TRANSACTION_APPROVED_AWAITING_FUNDING, UNILATERAL, VERIFICATION_REQUIRED.
paypal.orders: CardResponse
The payment card to use to fund a payment. Card can be a credit or debit card.
Fields
- name string? - The card holder's name as it appears on the card.
- billing_address CardResponseAddressPortable? - The billing address for this card. Supports only the address_line_1, address_line_2, admin_area_1, admin_area_2, postal_code, and country_code properties.
- last_digits string? - The last digits of the payment card.
- brand string? - The card brand or network. Typically used in the response. The possible values are VISA, MASTERCARD, DISCOVER, AMEX, SOLO, JCB, STAR, DELTA, SWITCH, MAESTRO, CB_NATIONALE, CONFIGOGA, CONFIDIS, ELECTRON, CETELEM, CHINA_UNION_PAY.
- 'type string? - The payment card type. The possible values are CREDIT, DEBIT, PREPAID, UNKNOWN.
- authentication_result AuthenticationResponse? - Results of Authentication such as 3D Secure.
paypal.orders: CardResponseAddressPortable
The billing address for this card. Supports only the address_line_1, address_line_2, admin_area_1, admin_area_2, postal_code, and country_code properties.
Fields
- address_line_1 string? - The first line of the address. For example, number or street. For example, 173 Drury Lane. Required for data entry and compliance and risk checks. Must contain the full address.
- address_line_2 string? - The second line of the address. For example, suite or apartment number.
- admin_area_2 string? - A city, town, or village. Smaller than admin_area_level_1.
- admin_area_1 string? - The highest level sub-division in a country, which is usually a province, state, or ISO-3166-2 subdivision. Format for postal delivery. For example, CA and not California.
- postal_code string? - The postal code, which is the zip code or equivalent. Typically required for countries with a postal code or an equivalent.
- country_code string - The two-character ISO 3166-1 code that identifies the country or region.
paypal.orders: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
paypal.orders: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
paypal.orders: CreatedOrderDetails
Created order details.
Fields
- create_time string? - The date and time when the transaction occurred, in Internet date and time format.
- update_time string? - The date and time when the transaction was last updated, in Internet date and time format.
- id string? - The ID of the order.
- payment_source PaymentSourceResponse? - The payment source used to fund the payment.
- intent string? - The intent to either capture payment immediately or authorize a payment for an order after order creation. The possible values are CAPTURE, AUTHORIZE.
- payer Payer? - The customer who approves and pays for the order. The customer is also known as the payer.
- purchase_units PurchaseUnit[]? - An array of purchase units. Each purchase unit establishes a contract between a customer and merchant. Each purchase unit represents either a full or partial order that the customer intends to purchase from the merchant.
- status string? - The order status. The possible values are CREATED, SAVED, APPROVED, VOIDED, COMPLETED, PAYER_ACTION_REQUIRED.
- links LinkDescription[]? - An array of request-related HATEOAS links. To complete payer approval, use the approve link to redirect the payer. The API caller has 3 hours (default setting, this which can be changed by your account manager to 24/48/72 hours to accommodate your use case) from the time the order is created, to redirect your payer. Once redirected, the API caller has 3 hours for the payer to approve the order and either authorize or capture the order. If you are not using the PayPal JavaScript SDK to initiate PayPal Checkout (in context) ensure that you include application_context.return_url is specified or you will get "We're sorry, Things don't appear to be working at the moment" after the payer approves the payment.
paypal.orders: CreateOrderRequest
The create order request
Fields
- intent string - The intent to either capture payment immediately or authorize a payment for an order after order creation. The possible values are CAPTURE, AUTHORIZE.
- payer Payer? - The customer who approves and pays for the order. The customer is also known as the payer.
- purchase_units PurchaseUnitRequest[] - An array of purchase units. Each purchase unit establishes a contract between a payer and the payee. Each purchase unit represents either a full or partial order that the payer intends to purchase from the payee.
- application_context OrderApplicationContext? - Customize the payer experience during the approval process for the payment with PayPal.
paypal.orders: Item
Item that the customer purchases from the merchant.
Fields
- name string - The item name or title.
- unit_amount UnitAmount - The item price or rate per unit. If you specify unit_amount, purchase_units[].amount.breakdown.item_total is required. Must equal unit_amount * quantity for all items. unit_amount.value can not be a negative number.
- tax Tax? - The item tax for each unit. If tax is specified, purchase_units[].amount.breakdown.tax_total is required. Must equal tax * quantity for all items. tax.value can not be a negative number.
- quantity string - The item quantity. Must be a whole number.
- description string? - The detailed item description.
- sku string? - The stock keeping unit (SKU) for the item.
- category string? - The item category type. The possible values are DIGITAL_GOODS, PHYSICAL_GOODS, DONATION.
paypal.orders: LinkDescription
Related HATEOAS links.
Fields
- href string - The complete target URL. To make the related call, combine the method with this URI Template-formatted link. For pre-processing, include the $, (, and ) characters. The href is the key HATEOAS component that links a completed call with a subsequent call.
- rel string - The link relation type, which serves as an ID for a link that unambiguously describes the semantics of the link. See Link Relations.
- method string? - The HTTP method required to make the related call. Possible values are GET,POST,PUT,DELETE,HEAD,CONNECT,OPTIONS,PATCH.
paypal.orders: MoneyForAmountForPlatformFee
The fee for this transaction.
Fields
- currency_code string - The three-character ISO-4217 currency code that identifies the currency.
- value string - The value, which might be an integer for currencies like JPY that are not typically fractional. A decimal fraction for currencies like TND that are subdivided into thousandths. For the required number of decimal places for a currency code, see Currency Codes.
paypal.orders: NetworkTransactionReference
Reference values used by the card network to identify a transaction. The possible values are VISA, MASTERCARD, DISCOVER, AMEX, SOLO, JCB, STAR, DELTA, SWITCH, MAESTRO, CB_NATIONALE, CONFIGOGA, CONFIDIS, ELECTRON, CETELEM, CHINA_UNION_PAY.
Fields
- id string - Transaction reference id returned by the scheme. For Visa and Amex, this is the "Tran id" field in response. For MasterCard, this is the "BankNet reference id" field in response. For Discover, this is the "NRID" field in response.
- date string? - The date that the transaction was authorized by the scheme. For MasterCard, this is the "BankNet reference date" field in response.
- network string - Name of the card network through which the transaction was routed.
paypal.orders: OrderApplicationContext
Customize the payer experience during the approval process for the payment with PayPal.
Fields
- brand_name string? - The label that overrides the business name in the PayPal account on the PayPal site.
- locale string? - The BCP 47-formatted locale of pages that the PayPal payment experience shows. PayPal supports a five-character code. For example, da-DK, he-IL, id-ID, ja-JP, no-NO, pt-BR, ru-RU, sv-SE, th-TH, zh-CN, zh-HK, or zh-TW.
- landing_page string? - The type of landing page to show on the PayPal site for customer checkout. The possible values are LOGIN, BILLING, NO_PREFERENCE.
- shipping_preference string? - The shipping preference. Displays the shipping address to the customer. Enables the customer to choose an address on the PayPal site. Restricts the customer from changing the address during the payment-approval process. The possible values are GET_FROM_FILE, NO_SHIPPING, SET_PROVIDED_ADDRESS.
- user_action string? - Configures a Continue or Pay Now checkout flow. The possible values are CONTINUE, PAY_NOW.
- payment_method PaymentMethod? - The customer and merchant payment preferences.
- return_url string? - The URL where the customer is redirected after the customer approves the payment.
- cancel_url string? - The URL where the customer is redirected after the customer cancels the payment.
- stored_payment_source StoredPaymentSource? - Provides additional details to process a payment using a payment_source that has been stored or is intended to be stored (also referred to as stored_credential or card-on-file).
paypal.orders: OrderDetails
Order details.
Fields
- create_time string? - The date and time when the transaction occurred, in Internet date and time format.
- update_time string? - The date and time when the transaction was last updated, in Internet date and time format.
- id string? - The ID of the order.
- payment_source PaymentSourceResponse? - The payment source used to fund the payment.
- intent string? - The intent to either capture payment immediately or authorize a payment for an order after order creation. The possible values are CAPTURE, AUTHORIZE.
- payer Payer? - The customer who approves and pays for the order. The customer is also known as the payer.
- purchase_units PurchaseUnit[]? - An array of purchase units. Each purchase unit establishes a contract between a customer and merchant. Each purchase unit represents either a full or partial order that the customer intends to purchase from the merchant.
- status string? - The order status. The possible values are CREATED, SAVED, APPROVED, VOIDED, COMPLETED, PAYER_ACTION_REQUIRED.
- links LinkDescription[]? - An array of request-related HATEOAS links. To complete payer approval, use the approve link to redirect the payer. The API caller has 3 hours (default setting, this which can be changed by your account manager to 24/48/72 hours to accommodate your use case) from the time the order is created, to redirect your payer. Once redirected, the API caller has 3 hours for the payer to approve the order and either authorize or capture the order. If you are not using the PayPal JavaScript SDK to initiate PayPal Checkout (in context) ensure that you include application_context.return_url is specified or you will get "We're sorry, Things don't appear to be working at the moment" after the payer approves the payment.
paypal.orders: Patch
A JSON patch object to apply partial updates to resources.
Fields
- op string - The operation. The possible values are add, remove, replace, move, copy, test.
- path string? - The JSON Pointer to the target document location at which to complete the operation.
- 'from string? - The JSON Pointer to the target document location from which to move the value. Required for the move operation.
paypal.orders: Payee
The merchant who receives payment for this transaction.
Fields
- email_address string? - The email address of merchant.
- merchant_id string? - The encrypted PayPal account ID of the merchant.
paypal.orders: PayeeBase
The recipient of the fee for this transaction. If you omit this value, the default is the API caller.
Fields
- email_address string? - The email address of merchant.
- merchant_id string? - The encrypted PayPal account ID of the merchant.
paypal.orders: Payer
The customer who approves and pays for the order. The customer is also known as the payer.
Fields
- email_address string? - The email address of the payer.
- payer_id string? - The PayPal-assigned ID for the payer.
paypal.orders: PaymentCollection
The comprehensive history of payments for the purchase unit.
Fields
- authorizations AuthorizationWithAdditionalData[]? - An array of authorized payments for a purchase unit. A purchase unit can have zero or more authorized payments.
- captures Capture[]? - An array of captured payments for a purchase unit. A purchase unit can have zero or more captured payments.
- refunds Refund[]? - An array of refunds for a purchase unit. A purchase unit can have zero or more refunds.
paypal.orders: PaymentInstruction
Any additional payment instructions to be consider during payment processing. This processing instruction is applicable for Capturing an order or Authorizing an Order.
Fields
- platform_fees PlatformFee[]? - An array of various fees, commissions, tips, or donations. This field is only applicable to merchants that been enabled for PayPal Commerce Platform for Marketplaces and Platforms capability.
- disbursement_mode string? - The funds that are held on behalf of the merchant. The possible values are INSTANT, DELAYED.
- payee_pricing_tier_id string? - This field is only enabled for selected merchants/partners to use and provides the ability to trigger a specific pricing rate/plan for a payment transaction. The list of eligible 'payee_pricing_tier_id' would be provided to you by your Account Manager. Specifying values other than the one provided to you by your account manager would result in an error.
paypal.orders: PaymentMethod
The customer and merchant payment preferences.
Fields
- payer_selected string? - The customer-selected payment method on the merchant site.
- payee_preferred string? - The merchant-preferred payment methods. The possible values are UNRESTRICTED, IMMEDIATE_PAYMENT_REQUIRED.
- standard_entry_class_code string? - NACHA (the regulatory body governing the ACH network) requires that API callers (merchants, partners) obtain the consumer’s explicit authorization before initiating a transaction. To stay compliant, you’ll need to make sure that you retain a compliant authorization for each transaction that you originate to the ACH Network using this API. ACH transactions are categorized (using SEC codes) by how you capture authorization from the Receiver (the person whose bank account is being debited or credited). PayPal supports the following SEC codes. The possible values are TEL, WEB, CCD, PPD.
paypal.orders: PaymentSourceResponse
The payment source used to fund the payment.
Fields
- card CardResponse? - The payment card to use to fund a payment. Card can be a credit or debit card.
paypal.orders: PlatformFee
Various fees, commissions, tips, or donations. This field is only applicable to merchants that been enabled for PayPal Commerce Platform for Marketplaces and Platforms capability.
Fields
- amount MoneyForAmountForPlatformFee - The fee for this transaction.
- payee PayeeBase? - The recipient of the fee for this transaction. If you omit this value, the default is the API caller.
paypal.orders: ProcessorResponse
The processor response for card transactions.
Fields
- avs_code string? - The address verification code for Visa, Discover, Mastercard, or American Express transactions.
- cvv_code string? - The card verification value code for for Visa, Discover, Mastercard, or American Express.
- response_code string? - Processor response code for the non-PayPal payment processor errors.
- payment_advice_code string? - The declined payment transactions might have payment advice codes. The card networks, like Visa and Mastercard, return payment advice codes.
paypal.orders: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
paypal.orders: PurchaseUnit
A purchase unit. Each purchase unit establishes a contract between a customer and merchant. Each purchase unit represents either a full or partial order that the customer intends to purchase from the merchant.
Fields
- reference_id string? - The API caller-provided external ID for the purchase unit. Required for multiple purchase units when you must update the order through PATCH. If you omit this value and the order contains only one purchase unit, PayPal sets this value to default.
- amount Amount? - The total order amount with an optional breakdown that provides details, such as the total item amount, total tax amount, shipping, handling, insurance, and discounts, if any. If you specify amount.breakdown, the amount equals item_total plus tax_total plus shipping plus handling plus insurance minus shipping_discount minus discount. The amount must be a positive number. For listed of supported currencies and decimal precision, see the PayPal REST APIs Currency Codes.
- payee Payee? - The merchant who receives payment for this transaction.
- payment_instruction PaymentInstruction? - Any additional payment instructions to be consider during payment processing. This processing instruction is applicable for Capturing an order or Authorizing an Order.
- description string? - The purchase description.
- custom_id string? - The API caller-provided external ID. Used to reconcile API caller-initiated transactions with PayPal transactions. Appears in transaction and settlement reports.
- invoice_id string? - The API caller-provided external invoice ID for this order.
- id string? - The PayPal-generated ID for the purchase unit. This ID appears in both the payer's transaction history and the emails that the payer receives. In addition, this ID is available in transaction and settlement reports that merchants and API callers can use to reconcile transactions. This ID is only available when an order is saved by calling v2/checkout/orders/id/save.
- soft_descriptor string? - The payment descriptor on account transactions on the customer's credit card statement, that PayPal sends to processors. The maximum length of the soft descriptor information that you can pass in the API field is 22 characters, in the following format:22 - len(PAYPAL * (8)) - len(Descriptor in Payment Receiving Preferences of Merchant account + 1)The PAYPAL prefix uses 8 characters.
- items Item[]? - An array of items that the customer purchases from the merchant.
- shipping ShippingDetail? - The name and address of the person to whom to ship the items.
- payments PaymentCollection? - The comprehensive history of payments for the purchase unit.
paypal.orders: PurchaseUnitRequest
A purchase unit. Each purchase unit establishes a contract between a payer and the payee. Each purchase unit represents either a full or partial order that the payer intends to purchase from the payee.
Fields
- reference_id string? - The API caller-provided external ID for the purchase unit. Required for multiple purchase units when you must update the order through PATCH. If you omit this value and the order contains only one purchase unit, PayPal sets this value to default.
- amount Amount - The total order amount with an optional breakdown that provides details, such as the total item amount, total tax amount, shipping, handling, insurance, and discounts, if any. If you specify amount.breakdown, the amount equals item_total plus tax_total plus shipping plus handling plus insurance minus shipping_discount minus discount. The amount must be a positive number. For listed of supported currencies and decimal precision, see the PayPal REST APIs Currency Codes.
- payee Payee? - The merchant who receives payment for this transaction.
- payment_instruction PaymentInstruction? - Any additional payment instructions to be consider during payment processing. This processing instruction is applicable for Capturing an order or Authorizing an Order.
- description string? - The purchase description.
- custom_id string? - The API caller-provided external ID. Used to reconcile client transactions with PayPal transactions. Appears in transaction and settlement reports but is not visible to the payer.
- invoice_id string? - The API caller-provided external invoice number for this order. Appears in both the payer's transaction history and the emails that the payer receives.
- soft_descriptor string? - The soft descriptor is the dynamic text used to construct the statement descriptor that appears on a payer's card statement. If an Order is paid using the "PayPal Wallet", the statement descriptor will appear in following format on the payer's card statement, PAYPAL_prefix+(space)+merchant_descriptor+(space)+ soft_descriptor
- items Item[]? - An array of items that the customer purchases from the merchant.
- shipping ShippingDetail? - The name and address of the person to whom to ship the items.
paypal.orders: Refund
A refund for a purchase unit. A purchase unit can have zero or more refunds.
Fields
- status string? - The status of the refund.
- status_details RefundStatusDetails? - The details of the refund status.
paypal.orders: RefundStatusDetails
The details of the refund status.
Fields
- reason string? - The reason why the refund has the PENDING or FAILED status. The possible values are ECHECK.
paypal.orders: ShippingDetail
The name and address of the person to whom to ship the items.
Fields
- name ShippingDetailName? - The name of the person to whom to ship the items. Supports only the full_name property.
- 'type string? - The method by which the payer wants to get their items from the payee e.g shipping, in-person pickup. Either type or options but not both may be present. The possible values are SHIPPING, PICKUP_IN_PERSON.
- address ShippingDetailAddressPortable? - The address of the person to whom to ship the items. Supports only the address_line_1, address_line_2, admin_area_1, admin_area_2, postal_code, and country_code properties.
paypal.orders: ShippingDetailAddressPortable
The address of the person to whom to ship the items. Supports only the address_line_1, address_line_2, admin_area_1, admin_area_2, postal_code, and country_code properties.
Fields
- address_line_1 string? - The first line of the address. For example, number or street. For example, 173 Drury Lane. Required for data entry and compliance and risk checks. Must contain the full address.
- address_line_2 string? - The second line of the address. For example, suite or apartment number.
- admin_area_2 string? - A city, town, or village. Smaller than admin_area_level_1.
- admin_area_1 string? - The highest level sub-division in a country, which is usually a province, state, or ISO-3166-2 subdivision. Format for postal delivery. For example, CA and not California.
- postal_code string? - The postal code, which is the zip code or equivalent. Typically required for countries with a postal code or an equivalent.
- country_code string - The two-character ISO 3166-1 code that identifies the country or region.
paypal.orders: ShippingDetailName
The name of the person to whom to ship the items. Supports only the full_name property.
Fields
- full_name string? - When the party is a person, the party's full name.
paypal.orders: StoredPaymentSource
Provides additional details to process a payment using a payment_source that has been stored or is intended to be stored (also referred to as stored_credential or card-on-file).
Fields
- payment_initiator string - The person or party who initiated or triggered the payment. The possible values are CUSTOMER, MERCHANT.
- payment_type string - Indicates the type of the stored payment_source payment. The possible values are ONE_TIME, RECURRING, UNSCHEDULED.
- usage string? - Indicates if this is a first or subsequent payment using a stored payment source (also referred to as stored credential or card on file). The possible values are FIRST, SUBSEQUENT, DERIVED.
- previous_network_transaction_reference NetworkTransactionReference? - Reference values used by the card network to identify a transaction. The possible values are VISA, MASTERCARD, DISCOVER, AMEX, SOLO, JCB, STAR, DELTA, SWITCH, MAESTRO, CB_NATIONALE, CONFIGOGA, CONFIDIS, ELECTRON, CETELEM, CHINA_UNION_PAY.
paypal.orders: Tax
The item tax for each unit. If tax is specified, purchase_units[].amount.breakdown.tax_total is required. Must equal tax * quantity for all items. tax.value can not be a negative number.
Fields
- currency_code string - The three-character ISO-4217 currency code that identifies the currency.
- value string - The value, which might be an integer for currencies like JPY that are not typically fractional. A decimal fraction for currencies like TND that are subdivided into thousandths. For the required number of decimal places for a currency code, see Currency Codes.
paypal.orders: ThreeDSecureAuthenticationResponse
Results of 3D Secure Authentication.
Fields
- authentication_status string? - The outcome of the issuer's authentication. The possible values are Y, N, U, A, C, R, D, I.
- enrollment_status string? - Status of authentication eligibility. The possible values are Y, N, U, B.
paypal.orders: Token
The tokenized payment source to fund a payment.
Fields
- id string - The PayPal-generated ID for the token.
- 'type string - The tokenization method that generated the ID. The possible values are BILLING_AGREEMENT.
paypal.orders: UnitAmount
The item price or rate per unit. If you specify unit_amount, purchase_units[].amount.breakdown.item_total is required. Must equal unit_amount * quantity for all items. unit_amount.value can not be a negative number.
Fields
- currency_code string - The three-character ISO-4217 currency code that identifies the currency.
- value string - The value, which might be an integer for currencies like JPY that are not typically fractional. A decimal fraction for currencies like TND that are subdivided into thousandths. For the required number of decimal places for a currency code, see Currency Codes.
Import
import ballerinax/paypal.orders;
Metadata
Released date: over 1 year ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 1301
Current verison: 248
Weekly downloads
Keywords
Finance/Payment
Cost/Freemium
Contributors
Dependencies