orbitcrm
Module orbitcrm
API
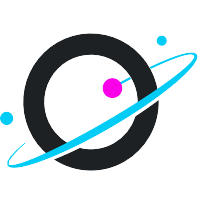
ballerinax/orbitcrm Ballerina library
Overview
This is a generated connector for Orbit API v1 OpenAPI specification.
Orbit API used to track activities of users and manage users.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create Orbit Account
- Obtaining tokens
- Log into Orbit Account and get token by navigating to
Account Settings
- Log into Orbit Account and get token by navigating to
Quickstart
Step 1: Import connector
Import the ballerinax/orbitcrm module into the Ballerina project.
import ballerinax/orbitcrm;
Step 2: Create a new connector instance
Add the project configuration file by creating a Config.toml
file. Config file should have following configurations. Add the tokens obtained in the previous step to the Config.toml
file.
[auth] token = "<Bearer_token">
configurable http:BearerTokenConfig & readonly auth = ?; orbitcrm:ClientConfig clientConfig = {auth : auth}; orbitcrm:Client myclient = check new orbitcrm:Client(clientConfig, {}, "https://app.orbit.love/api/v1");
Step 3: Invoke connector operation
- You can get activity details as follows with
getActivitiesById
method by passing Id of a work sheet as a parameter. Successful creation returns thejson
containing activity detail and the error cases returns anerror
object.orbitcrm:json result = check myclient->getActivitiesById(<Workspace_ID>);
- Use
bal run
command to compile and run the Ballerina program.
Clients
orbitcrm: Client
This is a generated connector for Orbit API v1 OpenAPI specification. Orbit API used to track activities of users and manage users.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create an Orbit Account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://app.orbit.love/api/v1" - URL of the target service
getActivityTypes
function getActivityTypes() returns json|error
Get all activity types
Return Type
- json|error - success
getCurrentUser
function getCurrentUser() returns json|error
Get info about the current user
Return Type
- json|error - success
getWorkspaces
function getWorkspaces() returns json|error
Get all workspaces for the current user
Return Type
- json|error - success
getWorkspaceById
Get a workspace
Parameters
- workspaceId string - Workspace ID
Return Type
- json|error - success
getActivitiesInWorkspace
function getActivitiesInWorkspace(string workspaceId, string? activityTags, string? affiliation, string? memberTags, string? orbitLevel, string? activityType, string? weight, string? identity, string? location, string? company, string? startDate, string? endDate, string? page, string? direction, string? items, string? sort, string? 'type) returns json|error
List activities for a workspace
Parameters
- workspaceId string - Workspace ID
- activityTags string? (default ()) - Activity tags allow to group activities
- affiliation string? (default ()) - Association with either 'member' or 'teammate'
- memberTags string? (default ()) - Member tags allow to group members
- orbitLevel string? (default ()) - Orbit level
- activityType string? (default ()) - Activity type related to activities
- weight string? (default ()) - Weighted score of activity
- identity string? (default ()) - Activity identity of platforms
- location string? (default ()) - Identifier for the location
- company string? (default ()) - Identifier for the company
- startDate string? (default ()) - Activity start date
- endDate string? (default ()) - Activity end date
- page string? (default ()) - Page of the activities
- direction string? (default ()) - Sort activities 'ASC' if Ascending and 'DESC' if Descending
- items string? (default ()) - Number of activities
- sort string? (default ()) - Sort activities by properties of activity
- 'type string? (default ()) - Type related to activities
Return Type
- json|error - success
postActivitiesById
function postActivitiesById(string workspaceId, ActivityAndIdentity payload) returns json|error
Create a Custom or a Content activity for a new or existing member
Return Type
- json|error - success
getActivitiesById
Get an activity in the workspace
Return Type
- json|error - success
getMembers
function getMembers(string workspaceId, string? activityTags, string? affiliation, string? memberTags, string? orbitLevel, string? activityType, string? weight, string? identity, string? location, string? company, string? startDate, string? endDate, string? query, string? page, string? direction, string? items, string? activitiesCountMin, string? activitiesCountMax, string? sort, string? 'type) returns json|error
List members in a workspace
Parameters
- workspaceId string - Workspace ID
- activityTags string? (default ()) - Activity tags allow to group activities
- affiliation string? (default ()) - Association with either 'member' or 'teammate'
- memberTags string? (default ()) - Member tags allow to group members
- orbitLevel string? (default ()) - Orbit level
- activityType string? (default ()) - Activity type related to activities
- weight string? (default ()) - Weighted score of activity
- identity string? (default ()) - Activity identity of platforms
- location string? (default ()) - Identifier for the location
- company string? (default ()) - Identifier for the company
- startDate string? (default ()) - Activity start date
- endDate string? (default ()) - Activity end date
- query string? (default ()) - Activity query
- page string? (default ()) - Page of the members
- direction string? (default ()) - Organise activities 'ASC' if Ascending and 'DESC' if Descending
- items string? (default ()) - Number of members
- activitiesCountMin string? (default ()) - Minumum count of activities
- activitiesCountMax string? (default ()) - Maximum count of activities
- sort string? (default ()) - Sort activities by properties of activity
- 'type string? (default ()) - Type related to activities
Return Type
- json|error - success
postMembers
function postMembers(string workspaceId, MemberAndIdentity payload) returns json|error
Create or update a member
Return Type
- json|error - success
findMembers
function findMembers(string workspaceId, string? 'source, string? sourceHost, string? uid, string? username, string? email, string? github) returns json|error
Find a member by an identity
Parameters
- workspaceId string - Workspace ID
- 'source string? (default ()) - Source of member in a workspace
- sourceHost string? (default ()) - Source host of member in a workspace
- uid string? (default ()) - Unique identifier of member in a workspace
- username string? (default ()) - Username of member in a workspace
- email string? (default ()) - Email of member in a workspace
- github string? (default ()) - Deprecated, please use source=github and username=<username> instead
Return Type
- json|error - success
getMembersById
Get a member
Return Type
- json|error - success
updateMembersById
Update a member
deleteMembersById
Delete a member
getActivities
function getActivities(string workspaceId, string memberId, string? page, string? direction, string? items, string? sort, string? activityType, string? 'type) returns json|error
List activities for a member
Parameters
- workspaceId string - Workspace ID
- memberId string - Member ID
- page string? (default ()) - Page of the activities
- direction string? (default ()) - Sort activities 'ASC' if Ascending and 'DESC' if Descending
- items string? (default ()) - Number of activities
- sort string? (default ()) - Sort activities by properties of activity
- activityType string? (default ()) - Activity type related to activities
- 'type string? (default ()) - Type related to activities
Return Type
- json|error - success
postActivities
Create a Custom or a Content activity for a member
Parameters
- workspaceId string - Workspace ID
- memberId string - Member ID
- payload Activity - Create member activity
Return Type
- json|error - success
updateActivityById
function updateActivityById(string workspaceId, string memberId, string id, Activity payload) returns Response|error
Update a custom activity for a member
Parameters
- workspaceId string - Workspace ID
- memberId string - Member ID
- id string - Activity ID
- payload Activity - Update member activity
deleteActivityById
Delete a post activity
postIdentities
Add identity to a member
Parameters
- workspaceId string - Workspace ID
- memberId string - Member ID
- payload Identity - Add member identity
Return Type
- json|error - success
deleteIdentities
Remove identity from a member
getNotes
Get the member's notes
Parameters
- workspaceId string - Workspace ID
- memberId string - Member ID
- page string? (default ()) - Page of the notes
Return Type
- json|error - success
postNotes
Create a note
Return Type
- json|error - note created
updateNoteById
function updateNoteById(string workspaceId, string memberId, string id, Note payload) returns Response|error
Update a note
Parameters
- workspaceId string - Workspace ID
- memberId string - Member ID
- id string - Note ID
- payload Note - Update note
getReports
function getReports(string workspaceId, string? startDate, string? endDate, string? group, string? activityType, string? 'type) returns json|error
Get a workspace stats
Parameters
- workspaceId string - Workspace ID
- startDate string? (default ()) - Report start date
- endDate string? (default ()) - Report end date
- group string? (default ()) - Group in report generation
- activityType string? (default ()) - Activity type related to activities
- 'type string? (default ()) - Report type to generate
Return Type
- json|error - success
Records
orbitcrm: Activity
Activities are instances of community participation and contribution.
Fields
- activity_type string? - The type of activity - what action was done by the member
- description string? - A description of the activity; displayed in the timeline
- 'key string? - Supply a key that must be unique or leave blank to have one generated.
- link string? - A URL for the activity; displayed in the timeline
- link_text string? - The text for the timeline link
- occurred_at string? - The date and time the activity occurred; defaults to now
- tags string[]? - [EXPERIMENTAL] Capture facets of the activity to group by later e.g. locations or channels; replaces existing value
- title string - A title for the activity; displayed in the timeline
- weight string? - A custom weight to be used in filters and reports; defaults to 1.
orbitcrm: ActivityAndIdentity
Fields
- activity ActivityWithMember|PostActivityWithMember? -
- identity Identity? - Represents an email address, a profile on networks like github and twitter, or a record in another system.
orbitcrm: ActivityWithMember
Fields
- Fields Included from *Activity
- member Member? - Members are the people in the community.
orbitcrm: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
orbitcrm: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
orbitcrm: Identity
Represents an email address, a profile on networks like github and twitter, or a record in another system.
Fields
- email string? - The email of the person in the source system
- name string? - The name of the person in the source system
- 'source string - The type of source: known values include github, twitter, discourse, email, linkedin, devto. Custom values can also be used
- source_host string? - Specifies the location of the source, such as the host of a Discourse instance
- uid string? - The uid of the person in the source system
- url string? - For custom identities, an optional link to the profile on the source system
- username string? - The username of the person in the source system
orbitcrm: Member
Members are the people in the community.
Fields
- bio string? - The member’s bio
- birthday string? - The member’s birthday, e.g. August 1
- company string? - The member’s company
- devto string? - The member's dev.to username
- email string? - The member's email
- github string? - The member's GitHub username
- linkedin string? - The member's LinkedIn username, without the in/ or pub/
- location string? - The member’s location. That field is not normalized and reflects what can be found in the member’s identities.
- name string? - The member’s name
- pronouns string? - The member’s pronouns
- shipping_address string? - The member’s shipping address
- slug string? - The member’s slug in the Orbit app
- tag_list string? - Deprecated: Please use the tags attribute instead
- tags string? - Replaces all tags for the member; comma-separated string or array
- tags_to_add string? - Adds tags to member; comma-separated string or array
- teammate boolean? - Whether the member is a teammate
- title string? - The member’s title
- tshirt string? - The member’s tshirt size
- twitter string? - The member’s Twitter username, if available. null otherwise
- url string? - The URL listed by the member in one of their profiles
orbitcrm: MemberAndIdentity
Fields
- identity Identity? - Represents an email address, a profile on networks like github and twitter, or a record in another system.
- member Member? - Members are the people in the community.
orbitcrm: Note
A member's notes are free-form areas to attach information to a member's profile.
Fields
- body string - The body of member's note
orbitcrm: PostActivity
Fields
- activity_type string - Activity type related to activities
- occurred_at string? - The date and time at which the content was published; defaults to now
- url string - The url representing the post
orbitcrm: PostActivityWithMember
Fields
- Fields Included from *PostActivity
- member Member? - Members are the people in the community.
orbitcrm: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
Union types
orbitcrm: CustomOrPostActivity
CustomOrPostActivity
Import
import ballerinax/orbitcrm;
Metadata
Released date: over 1 year ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 2
Current verison: 1
Weekly downloads
Keywords
Sales & CRM/Customer Relationship Management
Cost/Freemium
Contributors
Dependencies