optimizely
Module optimizely
API
Definitions
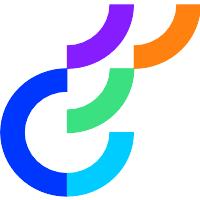
ballerinax/optimizely Ballerina library
Overview
This is a generated connector for Optimizely API v2 OpenAPI specification.
Optimizely Agent is a stand-alone, open-source microservice that provides major benefits over using Optimizely SDKs in certain use cases. Its REST API offers consolidated and simplified endpoints for accessing all the functionality of Optimizely Full Stack SDKs. Use this API the control experiments (such as a feature tests).
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create an Optimizely account.
- Obtain tokens by following this guide.
Quickstart
To use the Optimizely connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/optimizely
module into the Ballerina project.
import ballerinax/optimizely;
Step 2: Create a new connector instance
Create a optimizely:CredentialsConfig
with the <TOKEN>
obtained, and initialize the connector with it.
optimizely:ClientConfig clientConfig = { auth : { token: `<TOKEN>` } }; optimizely:Client baseClient = check new Client(clientConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example to list attributes under a project using the connector.
public function main() { int projectId = 231112; optimizely:Attribute[]|error response = baseClient->listAttributes(projectId); if (response is optimizely:Attribute[]) { log:printInfo(response.toString()); } else { log:printError(response.message()); } }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
optimizely: Client
Connects to Optimizely API v2 from Ballerina Optimizely Agent is a stand-alone, open-source microservice that provides major benefits over using Optimizely SDKs in certain use cases. Its REST API offers consolidated and simplified endpoints for accessing all the functionality of Optimizely Full Stack SDKs. Use this API the control experiments (such as a feature tests).
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Optimizely account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.optimizely.com/v2" - URL of the target service
listAttributes
List Attributes
Parameters
- projectId int - The ID of the Project you would like to list all Attributes for
- perPage int (default 25) - Optional pagination argument that specifies the maximum number of objects to return per request
- page int (default 1) - Optional pagination argument that specifies the page to return. If you have 140 objects and you choose to return 100 objects per page you will be able to access the last 40 objects on page 2. The default value is 1.
createAttribute
Create an Attribute
Parameters
- payload Attribute - A string in JSON format that includes all the fields to create a new Attribute
getAttribute
Read an Attribute
Parameters
- attributeId int - The Attribute ID of the Attribute you want to get
deleteAttribute
Delete an Attribute
Parameters
- attributeId int - The ID of the Attribute you'd like to delete
updateAttribute
function updateAttribute(int attributeId, AttributeUpdate payload) returns Attribute|error
Update an Attribute
Parameters
- attributeId int - The ID of the Attribute you'd like to update
- payload AttributeUpdate - A string in JSON format that includes all the fields you'd like to change for an Attribute
listAudiences
List Audiences
Parameters
- projectId int - The Project ID of the Project you would like to list all Audiences for
- perPage int (default 25) - Optional pagination argument that specifies the maximum number of objects to return per request
- page int (default 1) - Optional pagination argument that specifies the page to return. If you have 140 objects and you choose to return 100 objects per page you will be able to access the last 40 objects on page 2. The default value is 1.
createAudience
Create an Audience
Parameters
- payload Audience - A string in JSON format that includes all the fields to create an Audience
getAudience
Read an Audience
Parameters
- audienceId int - The Audience ID of the Audience you want to get
updateAudience
function updateAudience(int audienceId, AudienceUpdate payload) returns Audience|error
Update an Audience
Parameters
- audienceId int - The Audience ID of the Audience you want to change
- payload AudienceUpdate - A string in JSON format that includes all the fields you'd like to change for an Audience
getImpressionsUsageRequest
function getImpressionsUsageRequest(int accountId, string usageDateFrom, string usageDateTo, int perPage, int page, string[] platforms, string? query, string sort, string 'order) returns ImpressionsUsage[]|error
Get Impressions Usage
Parameters
- accountId int - The account ID of the customer
- usageDateFrom string - Start date of date range
- usageDateTo string - End date of date range
- perPage int (default 25) - Optional pagination argument that specifies the maximum number of objects to return per request
- page int (default 1) - Optional pagination argument that specifies the page to return. If you have 140 objects and you choose to return 100 objects per page you will be able to access the last 40 objects on page 2. The default value is 1.
- platforms string[] (default ["edge","fullstack","web"]) - The platform of the Project
- query string? (default ()) - Search by experiment_id, project_id.
- sort string (default "impression_count") - The property to sort by.
- 'order string (default "desc") - The property to sort by.
Return Type
- ImpressionsUsage[]|error - Return Impression Usage info for account
getImpressionsUsageSummaryRequest
function getImpressionsUsageSummaryRequest(int accountId) returns ImpressionsUsageSummary|error
Get Impressions Usage Summary
Parameters
- accountId int - The account ID of the customer
Return Type
- ImpressionsUsageSummary|error - Return Impression Usage Summary for account
listCampaigns
List Campaigns
Parameters
- projectId int - The Project ID of the Project you would like to list all Campaigns for
- perPage int (default 25) - Optional pagination argument that specifies the maximum number of objects to return per request
- page int (default 1) - Optional pagination argument that specifies the page to return. If you have 140 objects and you choose to return 100 objects per page you will be able to access the last 40 objects on page 2. The default value is 1.
createCampaign
Create a Campaign
Parameters
- payload Campaign - A string in JSON format that includes all the fields to create a Project
- action string? (default ()) - Action to change the state of the Campaign. 'publish' publishes your campaign, making any changes live to the world. Status will be 'paused'. 'start' publishes your campaign, making any changes live to the world. Status will be 'running'.
getCampaign
Read a Campaign
Parameters
- campaignId int - The Campaign ID of the Campaign you want to get
deleteCampaign
Delete a Campaign
Parameters
- campaignId int - The Campaign ID of the Campaign you want to delete
updateCampaign
function updateCampaign(int campaignId, CampaignUpdate payload, string? action) returns Campaign|error
Update a Campaign
Parameters
- campaignId int - The Campaign ID of the Campaign you want to change
- payload CampaignUpdate - A string in JSON format that includes all the fields you'd like to change for a Campaign
- action string? (default ()) - Action to change the state of the Campaign. 'publish' publishes your campaign, making any changes live to the world without changing the status of the campaign. 'start' publishes your campaign, making any changes live to the world. Status will be 'running'. 'pause' stops the campaign. Status will be 'paused'. No new visitors will see the campaign until it is resumed. 'resume' resumes the campaign from a paused status without publishing any new changes. Status will be 'running'. 'unarchive' unarchives an archived campaign. Status will be 'paused'.
getCampaignResults
function getCampaignResults(int campaignId, string? startTime, string? endTime, string? browser, string? device, string? 'source, int? attributeId, string? attributeValue, string? segmentConditions) returns CampaignResults|error?
Get Campaign results
Parameters
- campaignId int - The ID for the Campaign you want results for
- startTime string? (default ()) - The start time of the time interval (beginning time included) used to calculate the results over. If unspecified, defaults to the time that the Campaign was first activated. The start time will be rounded the smallest time modulo 5 minutes larger or equal to start_time.
- endTime string? (default ()) - The end of the time interval (end time excluded) used to calculate results over. If unspecified, defaults to the current time if the Experiment is still running, otherwise defaults to the time the experiment was last active. The end time will be rounded to the largest time modulo 5 minutes smaller or equal to end_time. The end_time in the response may be earlier than requested if fresher results are not available yet. In this case, the results will continue to calculate in the background and subsequent requests will eventually return the full results.
- browser string? (default ()) - Browser to segment results by. This parameter must not be sent with any other segmentation parameters, i.e. any parameters in [device, source, attribute_id, attribute_value].
- device string? (default ()) - Device to segment results by. This parameter must not be sent with any other segmentation parameters, i.e. any parameters in [browser, source, attribute_id, attribute_value].
- 'source string? (default ()) - Source to segment results by. This parameter must not be sent with any other segmentation parameters, i.e. any parameters in [browser, device, attribute_id, attribute_value]. Campaign: Contains users that arrive on a URL containing a 'utm_campaign,' 'utm_source,' 'gclid,' or 'otm_source' query parameter. If the URL contains one of these parameters, the visitor will count as "Campaign" traffic even if they arrived through search. Direct: Includes all users who do not have any external referrer in their URL. Referral: Includes all users that come from another URL that doesn't count as Campaign.
- attributeId int? (default ()) - ID of the attribute to segment results by. Requests containing attribute_id will return the results for all visitors that have attribute_value for the attribute represented by attribute_id. If present, the attribute_value parameter must also be present, and it cannot be sent with any other segmentation parameters, i.e. any parameters in [browser, device, source].
- attributeValue string? (default ()) - UTF-8 encoded value correlating to attribute_id. If present, the attribute_id parameter must also be present. This parameter also requires attribute_id to be set, and cannot be sent with any other segmentation parameters, i.e. any parameters in [browser, device, source].
- segmentConditions string? (default ()) - (BETA) A string representation of a JSON Segment Conditions Expression. This parameter can be either URL-escaped stringified JSON or Base64-encoded stringified JSON using URL-safe alphabet (preferred). Segment Conditions Expressions consist of Logical Expressions and Match Expressions. Logical Expressions are represented as an array of the format [<operator>, <expression>...], where the supported operators are "and", "or" and "not". Match Expressions are represented as an object of the format {"attribute_id": <attribute_id>, "attribute_value": <value>[, "match_type": <match_type>]}, where supported values for match_type are "exact" match type will match only an exact string match between "value" string and the attribute value. "substring" match type will match if "value" is a substring of the attribute value. "prefix" match type will match if "value" is a string prefix of the attribute value. "regex" match type will match if "value" is a regular expression match for the attribute value. The default match_type is "exact".
Return Type
- CampaignResults|error? - Return Campaign results
listChangeHistory
function listChangeHistory(int projectId, int[]? id, string? startTime, string? endTime, string[]? user, string[]? entityType, string[]? entity, int perPage, int page) returns ChangeHistoryChange[]|error
Retrieve changes for a project.
Parameters
- projectId int - ID of the Project you want to list changes for.
- id int[]? (default ()) - A specific Change ID to filter by. Can be specified multiple times to include multiple specific changes.
- startTime string? (default ()) - Start of the time interval (inclusive) to list changes. The time is formatted in ISO 8601.
- endTime string? (default ()) - End of the time interval (exclusive) to look for changes. The time is formatted in ISO 8601.
- user string[]? (default ()) - Email of the user who made the change. Can be specified multiple times to include changes from multiple users.
- entityType string[]? (default ()) - The type of the entity (with optional subtype delimited by a : (colon) you want to list changes for) e.g. experiment or experiment:feature. Can be specified multiple times to include changes for multiple entity types.
- entity string[]? (default ()) - Colon (:) delimited string containing the entity_type and entity_id of the entity wanted. e.g. experiment:123. Can be specified multiple times to filter changes to a specific set of entities.
- perPage int (default 25) - Optional pagination argument that specifies the maximum number of objects to return per request
- page int (default 1) - Optional pagination argument that specifies the page to return. If you have 140 objects and you choose to return 100 objects per page you will be able to access the last 40 objects on page 2. The default value is 1.
Return Type
- ChangeHistoryChange[]|error - Returns changes.
listEnvironments
function listEnvironments(int projectId, int perPage, int page) returns Environment[]|error
Get Environments by Project
Parameters
- projectId int - The ID of the project for which you would like to get Environments
- perPage int (default 25) - Optional pagination argument that specifies the maximum number of objects to return per request
- page int (default 1) - Optional pagination argument that specifies the page to return. If you have 140 objects and you choose to return 100 objects per page you will be able to access the last 40 objects on page 2. The default value is 1.
Return Type
- Environment[]|error - Return all Environments in a specified Project
createEnvironment
function createEnvironment(Environment payload) returns Environment|error
Create an Environment
Parameters
- payload Environment - A string in JSON format that includes all the fields to create an Environment
Return Type
- Environment|error - Return the created Environment
getEnvironment
function getEnvironment(int environmentId) returns Environment|error
Read an Environment
Parameters
- environmentId int - The unique identifier for the Environment
Return Type
- Environment|error - Successfully retrieved the Environment
deleteEnvironment
Archive an Environment
Parameters
- environmentId int - The ID of the Environment you'd like to archive
updateEnvironment
function updateEnvironment(int environmentId, EnvironmentUpdate payload) returns Environment|error
Update an Environment
Parameters
- environmentId int - The unique identifier for the Environment
- payload EnvironmentUpdate - A string in JSON format that includes all the fields you'd like to change for an Environment
Return Type
- Environment|error - Successfully updated the Environment
getDatafile
Read the datafile of an Environment
Parameters
- environmentId int - The Environment ID for the datafile you want to get.
Return Type
- json|error - Return Datafile for the Environment specified
listEvents
function listEvents(int projectId, int perPage, int page, boolean includeClassic) returns Event[]|error
List all Events
Parameters
- projectId int - The ID of the Project you would like to list all Events for
- perPage int (default 25) - Optional pagination argument that specifies the maximum number of objects to return per request
- page int (default 1) - Optional pagination argument that specifies the page to return. If you have 140 objects and you choose to return 100 objects per page you will be able to access the last 40 objects on page 2. The default value is 1.
- includeClassic boolean (default false) - Whether or not to include classic Events in the list of Events. If this parameter is not provided it will default to false
getEvent
Get Event by ID
Parameters
- eventId int - The Event ID of the Event you want to get
- includeClassic boolean? (default ()) - Whether or not to return a classic Event if the specified event_id belongs to a classic Event. If this parameter is not provided it will default to false
listExperiments
function listExperiments(int perPage, int page, int? projectId, int? campaignId, boolean includeClassic) returns Experiment[]|error
List Experiments
Parameters
- perPage int (default 25) - Optional pagination argument that specifies the maximum number of objects to return per request
- page int (default 1) - Optional pagination argument that specifies the page to return. If you have 140 objects and you choose to return 100 objects per page you will be able to access the last 40 objects on page 2. The default value is 1.
- projectId int? (default ()) - The Project ID of the Project you would like to list all Experiments for. You have to either use this argument or the campaign_id argument
- campaignId int? (default ()) - The Campaign ID of the Campaign you would like to list all Experiments for. You have to either use this argument or the project_id argument
- includeClassic boolean (default false) - Whether or not to include classic Experiments in the list of Experiments. If this parameter is not provided it will default to false
Return Type
- Experiment[]|error - Return all Experiments
createExperiment
function createExperiment(Experiment payload, string? action) returns Experiment|error
Create an Experiment
Parameters
- payload Experiment - A string in JSON format that includes all the fields to create an Experiment
- action string? (default ()) - Action to change the state of the experiment.
publish
saves and stages your experiment. If you have not started your experiment or the experiment is paused, your changes will not be visible to visitors when you publish.start
makes your experiment live to all visitors who are in your targeted audience and changes the status torunning
.pause
stops the experiment and changes the status topaused
. No new visitors will see apaused
experiment until you restart it. See Differences among publish, start, and pause for details.
Return Type
- Experiment|error - Return the created Experiment
getExperiment
function getExperiment(int experimentId) returns Experiment|error
Read an Experiment
Parameters
- experimentId int - The Experiment ID of the Experiment you want to get
Return Type
- Experiment|error - Return Experiment info
deleteExperiment
Delete an Experiment
Parameters
- experimentId int - The Experiment ID of the Experiment you want to delete
updateExperiment
function updateExperiment(int experimentId, ExperimentUpdate payload, string? action) returns Experiment|error
Update an Experiment
Parameters
- experimentId int - The Experiment ID of the Experiment you want to change
- payload ExperimentUpdate - A string in JSON format that includes all the fields you'd like to change for an Experiment
- action string? (default ()) - Action to change the state of the experiment.
publish
saves and stages your experiment. If you have not started your experiment or the experiment is paused, your changes will not be visible to visitors when you publish.start
makes your experiment live to all visitors who are in your targeted audience and changes the status torunning
.pause
stops the experiment and changes the status topaused
. No new visitors will see apaused
experiment until you restart it. See Differences among publish, start, and pause for details.resume
resumes the experiment from a paused status without publishing any new changes. Status will berunning
.unarchive
unarchives an archived experiment. Status will bepaused
.
Return Type
- Experiment|error - Successfully updated Experiment
getExperimentResults
function getExperimentResults(int experimentId, string? baselineVariationId, string? startTime, string? endTime, string? browser, string? device, string? 'source, int? attributeId, string? attributeValue, string? segmentConditions) returns ExperimentResults|error?
Get Experiment results
Parameters
- experimentId int - The ID for the Experiment you want results for
- baselineVariationId string? (default ()) - The ID of the variation to use as the baseline to compare against other variations. Defaults to the first variation if not provided. For an experience in a personalization campaign, the value can also be the string 'holdback'.
- startTime string? (default ()) - The start time of the time interval (beginning time included) used to calculate the results over. If unspecified, defaults to the time that the Experiment was first activated. The start time will be rounded the smallest time modulo 5 minutes larger or equal to start_time.
- endTime string? (default ()) - The end of the time interval (end time excluded) used to calculate results over. If unspecified, defaults to the current time if the Experiment is still running, otherwise defaults to the time the experiment was last active. The end time will be rounded to the largest time modulo 5 minutes smaller or equal to end_time. The end_time in the response may be earlier than requested if fresher results are not available yet. In this case, the results will continue to calculate in the background and subsequent requests will eventually return the full results.
- browser string? (default ()) - Browser to segment results by. This parameter must not be sent with any other segmentation parameters, i.e. any parameters in [device, source, attribute_id, attribute_value].
- device string? (default ()) - Device to segment results by. This parameter must not be sent with any other segmentation parameters, i.e. any parameters in [browser, source, attribute_id, attribute_value].
- 'source string? (default ()) - Source to segment results by. This parameter must not be sent with any other segmentation parameters, i.e. any parameters in [browser, device, attribute_id, attribute_value]. Campaign: Contains users that arrive on a URL containing a 'utm_campaign,' 'utm_source,' 'gclid,' or 'otm_source' query parameter. If the URL contains one of these parameters, the visitor will count as "Campaign" traffic even if they arrived through search. Direct: Includes all users who do not have any external referrer in their URL. Referral: Includes all users that come from another URL that doesn't count as Campaign.
- attributeId int? (default ()) - ID of the attribute to segment results by. Requests containing attribute_id will return the results for all visitors that have attribute_value for the attribute represented by attribute_id. If present, the attribute_value parameter must also be present, and it cannot be sent with any other segmentation parameters, i.e. any parameters in [browser, device, source].
- attributeValue string? (default ()) - UTF-8 encoded value correlating to attribute_id. If present, the attribute_id parameter must also be present. This parameter also requires attribute_id to be set, and cannot be sent with any other segmentation parameters, i.e. any parameters in [browser, device, source].
- segmentConditions string? (default ()) - (BETA) A string representation of a JSON Segment Conditions Expression. This parameter can be either URL-escaped stringified JSON or Base64-encoded stringified JSON using URL-safe alphabet (preferred). Segment Conditions Expressions consist of Logical Expressions and Match Expressions. Logical Expressions are represented as an array of the format [<operator>, <expression>...], where the supported operators are "and", "or" and "not". Match Expressions are represented as an object of the format {"attribute_id": <attribute_id>, "attribute_value": <value>[, "match_type": <match_type>]}, where supported values for match_type are "exact" match type will match only an exact string match between "value" string and the attribute value. "substring" match type will match if "value" is a substring of the attribute value. "prefix" match type will match if "value" is a string prefix of the attribute value. "regex" match type will match if "value" is a regular expression match for the attribute value. The default match_type is "exact".
Return Type
- ExperimentResults|error? - Return Experiment results
getExperimentSections
Read all the Sections in a Multivariate Test
Parameters
- experimentId int - The Experiment ID of the Multivariate Test you want to get Sections for
- perPage int (default 25) - Optional pagination argument that specifies the maximum number of objects to return per request
- page int (default 1) - Optional pagination argument that specifies the page to return. If you have 140 objects and you choose to return 100 objects per page you will be able to access the last 40 objects on page 2. The default value is 1.
createSection
Create a new Section in a Multivariate Test
Parameters
- experimentId int - The Experiment ID of the Multivariate Test you want to create a Section in
- payload Section - A string in JSON format that includes all the fields to create a Section
getSection
Read a Section of a Multivariate Test
Parameters
- sectionId int - The ID of the Section you want to read
- experimentId int - The ID of the Multivariate Test the requested Section is part of
deleteSection
Archive a Section by ID
Parameters
- sectionId int - The ID of the Section you want to archive within the given Experiment
- experimentId int - The ID of the Multivariate Test the requested Section is part of
updateSection
function updateSection(int sectionId, int experimentId, SectionUpdate payload) returns Section|error
Update a Section by ID
Parameters
- sectionId int - The ID of the Section you want to update
- experimentId int - The ID of the Multivariate Test the requested Section is part of
- payload SectionUpdate - A string in JSON format that includes all the fields to create a Section
getExperimentTimeseries
function getExperimentTimeseries(int experimentId, string? baselineVariationId, string? startTime, string? endTime, string? browser, string? device, string? 'source, int? attributeId, string? attributeValue, string? segmentConditions) returns ExperimentTimeseries|error?
Get Experiment results time series
Parameters
- experimentId int - The ID for the Experiment you want results for
- baselineVariationId string? (default ()) - The ID of the variation to use as the baseline to compare against other variations. Defaults to the first variation if not provided. For an experience in a personalization campaign, the value can also be the string 'holdback'.
- startTime string? (default ()) - The start time of the time interval (beginning time included) used to calculate the results over. If unspecified, defaults to the time that the Experiment was first activated. The start time will be rounded the smallest time modulo 5 minutes larger or equal to start_time.
- endTime string? (default ()) - The end of the time interval (end time excluded) used to calculate results over. If unspecified, defaults to the current time if the Experiment is still running, otherwise defaults to the time the experiment was last active. The end time will be rounded to the largest time modulo 5 minutes smaller or equal to end_time. The end_time in the response may be earlier than requested if fresher results are not available yet. In this case, the results will continue to calculate in the background and subsequent requests will eventually return the full results.
- browser string? (default ()) - Browser to segment results by. This parameter must not be sent with any other segmentation parameters, i.e. any parameters in [device, source, attribute_id, attribute_value].
- device string? (default ()) - Device to segment results by. This parameter must not be sent with any other segmentation parameters, i.e. any parameters in [browser, source, attribute_id, attribute_value].
- 'source string? (default ()) - Source to segment results by. This parameter must not be sent with any other segmentation parameters, i.e. any parameters in [browser, device, attribute_id, attribute_value]. Campaign: Contains users that arrive on a URL containing a 'utm_campaign,' 'utm_source,' 'gclid,' or 'otm_source' query parameter. If the URL contains one of these parameters, the visitor will count as "Campaign" traffic even if they arrived through search. Direct: Includes all users who do not have any external referrer in their URL. Referral: Includes all users that come from another URL that doesn't count as Campaign.
- attributeId int? (default ()) - ID of the attribute to segment results by. Requests containing attribute_id will return the results for all visitors that have attribute_value for the attribute represented by attribute_id. If present, the attribute_value parameter must also be present, and it cannot be sent with any other segmentation parameters, i.e. any parameters in [browser, device, source].
- attributeValue string? (default ()) - UTF-8 encoded value correlating to attribute_id. If present, the attribute_id parameter must also be present. This parameter also requires attribute_id to be set, and cannot be sent with any other segmentation parameters, i.e. any parameters in [browser, device, source].
- segmentConditions string? (default ()) - (BETA) A string representation of a JSON Segment Conditions Expression. This parameter can be either URL-escaped stringified JSON or Base64-encoded stringified JSON using URL-safe alphabet (preferred). Segment Conditions Expressions consist of Logical Expressions and Match Expressions. Logical Expressions are represented as an array of the format [<operator>, <expression>...], where the supported operators are "and", "or" and "not". Match Expressions are represented as an object of the format {"attribute_id": <attribute_id>, "attribute_value": <value>[, "match_type": <match_type>]}, where supported values for match_type are "exact" match type will match only an exact string match between "value" string and the attribute value. "substring" match type will match if "value" is a substring of the attribute value. "prefix" match type will match if "value" is a string prefix of the attribute value. "regex" match type will match if "value" is a regular expression match for the attribute value. The default match_type is "exact".
Return Type
- ExperimentTimeseries|error? - Return Experiment results time series
getCampaignResultsCsv
function getCampaignResultsCsv(int campaignId, string? startTime, string? endTime, string? browser, string? device, string? 'source, int? attributeId, string? attributeValue, string? segmentConditions) returns Response|error
Get Campaign results as a CSV
Parameters
- campaignId int - The ID for the Campaign you want results for
- startTime string? (default ()) - The start time of the time interval (beginning time included) used to calculate the results over. If unspecified, defaults to the time that the Campaign was first activated. The start time will be rounded the smallest time modulo 5 minutes larger or equal to start_time.
- endTime string? (default ()) - The end of the time interval (end time excluded) used to calculate results over. If unspecified, defaults to the current time if the Experiment is still running, otherwise defaults to the time the experiment was last active. The end time will be rounded to the largest time modulo 5 minutes smaller or equal to end_time. The end_time in the response may be earlier than requested if fresher results are not available yet. In this case, the results will continue to calculate in the background and subsequent requests will eventually return the full results.
- browser string? (default ()) - Browser to segment results by. This parameter must not be sent with any other segmentation parameters, i.e. any parameters in [device, source, attribute_id, attribute_value].
- device string? (default ()) - Device to segment results by. This parameter must not be sent with any other segmentation parameters, i.e. any parameters in [browser, source, attribute_id, attribute_value].
- 'source string? (default ()) - Source to segment results by. This parameter must not be sent with any other segmentation parameters, i.e. any parameters in [browser, device, attribute_id, attribute_value]. Campaign: Contains users that arrive on a URL containing a 'utm_campaign,' 'utm_source,' 'gclid,' or 'otm_source' query parameter. If the URL contains one of these parameters, the visitor will count as "Campaign" traffic even if they arrived through search. Direct: Includes all users who do not have any external referrer in their URL. Referral: Includes all users that come from another URL that doesn't count as Campaign.
- attributeId int? (default ()) - ID of the attribute to segment results by. Requests containing attribute_id will return the results for all visitors that have attribute_value for the attribute represented by attribute_id. If present, the attribute_value parameter must also be present, and it cannot be sent with any other segmentation parameters, i.e. any parameters in [browser, device, source].
- attributeValue string? (default ()) - UTF-8 encoded value correlating to attribute_id. If present, the attribute_id parameter must also be present. This parameter also requires attribute_id to be set, and cannot be sent with any other segmentation parameters, i.e. any parameters in [browser, device, source].
- segmentConditions string? (default ()) - (BETA) A string representation of a JSON Segment Conditions Expression. This parameter can be either URL-escaped stringified JSON or Base64-encoded stringified JSON using URL-safe alphabet (preferred). Segment Conditions Expressions consist of Logical Expressions and Match Expressions. Logical Expressions are represented as an array of the format [<operator>, <expression>...], where the supported operators are "and", "or" and "not". Match Expressions are represented as an object of the format {"attribute_id": <attribute_id>, "attribute_value": <value>[, "match_type": <match_type>]}, where supported values for match_type are "exact" match type will match only an exact string match between "value" string and the attribute value. "substring" match type will match if "value" is a substring of the attribute value. "prefix" match type will match if "value" is a string prefix of the attribute value. "regex" match type will match if "value" is a regular expression match for the attribute value. The default match_type is "exact".
Return Type
- Response|error - <p>Return Campaign results in CSV format</p> <p>Name of the columns returned: 'Start Time (UTC)', 'End Time (UTC)', 'Is Baseline Variation', 'Metric Event Name', 'Metric Event ID', 'Metric Numerator Type', 'Metric Denominator Type', 'Metric Winning Direction', 'Numerator Value', 'Denominator Value', 'Metric Value', 'Improvement Status from Baseline', 'Improvement Value from Baseline', 'Statistical Significance', 'Is Improvement Significant', 'Confidence Interval - Low', 'Confidence Interval - High', 'Samples Remaining to Significance'</p> <p>(<a href="https://help.optimizely.com/Export_to_CSV_column_reference_for_campaigns_and_experiments">Column reference guide for CSV export</a>)</p>
getEnrichedEventsExportCredentials
function getEnrichedEventsExportCredentials(string duration) returns E3Credentials|error
Get aws credentials to access enriched events dataset
Parameters
- duration string (default "1h") - Duration of the aws credentials token. Please use [H,h] for hours or [M,m] for minutes. Minimum is 15m and Maximum is 1h. Usage 1h.
Return Type
- E3Credentials|error - Return aws credentials
getExperimentResultsCsv
function getExperimentResultsCsv(int experimentId, string? baselineVariationId, string? startTime, string? endTime, string? browser, string? device, string? 'source, int? attributeId, string? attributeValue, string? segmentConditions) returns Response|error
Get Experiment results as a CSV
Parameters
- experimentId int - The ID for the Experiment you want results for
- baselineVariationId string? (default ()) - The ID of the variation to use as the baseline to compare against other variations. Defaults to the first variation if not provided. For an experience in a personalization campaign, the value can also be the string 'holdback'.
- startTime string? (default ()) - The start time of the time interval (beginning time included) used to calculate the results over. If unspecified, defaults to the time that the Experiment was first activated. The start time will be rounded the smallest time modulo 5 minutes larger or equal to start_time.
- endTime string? (default ()) - The end of the time interval (end time excluded) used to calculate results over. If unspecified, defaults to the current time if the Experiment is still running, otherwise defaults to the time the experiment was last active. The end time will be rounded to the largest time modulo 5 minutes smaller or equal to end_time. The end_time in the response may be earlier than requested if fresher results are not available yet. In this case, the results will continue to calculate in the background and subsequent requests will eventually return the full results.
- browser string? (default ()) - Browser to segment results by. This parameter must not be sent with any other segmentation parameters, i.e. any parameters in [device, source, attribute_id, attribute_value].
- device string? (default ()) - Device to segment results by. This parameter must not be sent with any other segmentation parameters, i.e. any parameters in [browser, source, attribute_id, attribute_value].
- 'source string? (default ()) - Source to segment results by. This parameter must not be sent with any other segmentation parameters, i.e. any parameters in [browser, device, attribute_id, attribute_value]. Campaign: Contains users that arrive on a URL containing a 'utm_campaign,' 'utm_source,' 'gclid,' or 'otm_source' query parameter. If the URL contains one of these parameters, the visitor will count as "Campaign" traffic even if they arrived through search. Direct: Includes all users who do not have any external referrer in their URL. Referral: Includes all users that come from another URL that doesn't count as Campaign.
- attributeId int? (default ()) - ID of the attribute to segment results by. Requests containing attribute_id will return the results for all visitors that have attribute_value for the attribute represented by attribute_id. If present, the attribute_value parameter must also be present, and it cannot be sent with any other segmentation parameters, i.e. any parameters in [browser, device, source].
- attributeValue string? (default ()) - UTF-8 encoded value correlating to attribute_id. If present, the attribute_id parameter must also be present. This parameter also requires attribute_id to be set, and cannot be sent with any other segmentation parameters, i.e. any parameters in [browser, device, source].
- segmentConditions string? (default ()) - (BETA) A string representation of a JSON Segment Conditions Expression. This parameter can be either URL-escaped stringified JSON or Base64-encoded stringified JSON using URL-safe alphabet (preferred). Segment Conditions Expressions consist of Logical Expressions and Match Expressions. Logical Expressions are represented as an array of the format [<operator>, <expression>...], where the supported operators are "and", "or" and "not". Match Expressions are represented as an object of the format {"attribute_id": <attribute_id>, "attribute_value": <value>[, "match_type": <match_type>]}, where supported values for match_type are "exact" match type will match only an exact string match between "value" string and the attribute value. "substring" match type will match if "value" is a substring of the attribute value. "prefix" match type will match if "value" is a string prefix of the attribute value. "regex" match type will match if "value" is a regular expression match for the attribute value. The default match_type is "exact".
Return Type
- Response|error - <p>Return Experiment results in CSV format</p> <p>Name of the columns returned: 'Start time (UTC)', 'End time (UTC)', 'Variation Name', 'Variation ID', 'Is Baseline Variation', 'Metric Event Name', 'Metric Event ID', 'Metric Numerator Type', 'Metric Denominator Type', 'Metric Winning Direction', 'Numerator Value', 'Denominator Value', 'Metric Value', 'Improvement Status from Baseline', 'Improvement Value from Baseline', 'Statistical Significance', 'Is Improvement Significant', 'Confidence Interval - Low', 'Confidence Interval - High', 'Samples Remaining to Significance'</p> <p>(<a href="https://help.optimizely.com/Export_to_CSV_column_reference_for_campaigns_and_experiments">Column reference guide for CSV export</a>)</p>
listExtensions
List Extensions
Parameters
- projectId int - The ID of the project you would like to list all extensions for
- perPage int (default 25) - Optional pagination argument that specifies the maximum number of objects to return per request
- page int (default 1) - Optional pagination argument that specifies the page to return. If you have 140 objects and you choose to return 100 objects per page you will be able to access the last 40 objects on page 2. The default value is 1.
createExtension
Create an Extension
Parameters
- payload Extension - A string in JSON format that includes all the fields to create an extension
getExtension
Get an Extension
Parameters
- extensionId int - The ID of the extension you'd like to get
deleteExtension
Archive an Extension
Parameters
- extensionId int - The ID of the extension you'd like to archive
updateExtension
function updateExtension(int extensionId, ExtensionUpdate payload) returns Extension|error
Update an Extension
Parameters
- extensionId int - The extension ID of the extension you want to change
- payload ExtensionUpdate - A string in JSON format that includes all the fields you'd like to change for an extension
listFeatures
Get Features by Project
Parameters
- projectId int - The ID of the project for which you would like to get Features
- perPage int (default 25) - Optional pagination argument that specifies the maximum number of objects to return per request
- page int (default 1) - Optional pagination argument that specifies the page to return. If you have 140 objects and you choose to return 100 objects per page you will be able to access the last 40 objects on page 2. The default value is 1.
createFeature
Create a Feature
Parameters
- payload Feature - A string in JSON format that includes all the fields to create a new Feature. Note this endpoint is incompatible with Full Stack Targeted Rollouts projects
getFeature
Read a Feature
Parameters
- featureId int - The unique identifier for the Feature
deleteFeature
Archive a Feature
Parameters
- featureId int - The ID of the Feature you'd like to archive
updateFeature
function updateFeature(int featureId, FeatureUpdate payload) returns Feature|error
Update a Feature
Parameters
- featureId int - The unique identifier for the Feature
- payload FeatureUpdate - A string in JSON format that includes all the fields you'd like to change for a Feature
listGroups
List Exclusion Groups
Parameters
- projectId int - The ID of the Project you would like to list all Exclusion Groups for
- perPage int (default 25) - Optional pagination argument that specifies the maximum number of objects to return per request
- page int (default 1) - Optional pagination argument that specifies the page to return. If you have 140 objects and you choose to return 100 objects per page you will be able to access the last 40 objects on page 2. The default value is 1.
createGroup
Create an Exclusion Group
Parameters
- payload Group - A string in JSON format that includes all the fields to create an Exclusion Group
getGroup
Get an Exclusion Group
Parameters
- groupId int - The ID of the Exclusion Group you'd like to get
deleteGroup
Archive an Exclusion Group
Parameters
- groupId int - The ID of the Exclusion Group you'd like to archive
updateGroup
function updateGroup(int groupId, GroupUpdate payload) returns Group|error
Update an Exclusion Group
Parameters
- groupId int - The group ID of the Exclusion Group you want to change
- payload GroupUpdate - A string in JSON format that includes all the fields you'd like to change for an Exclusion Group
listListAttributes
function listListAttributes(int projectId) returns ListAttribute[]|error
Get list attributes by project
Parameters
- projectId int - The ID of the project for which you would like to get List Attributes
Return Type
- ListAttribute[]|error - Return all List Attributes in the specified Project
createListAttribute
function createListAttribute(ListAttribute payload) returns ListAttribute|error
Create a List Attribute
Parameters
- payload ListAttribute - A string in JSON format that includes all the fields to create a new List Attribute
Return Type
- ListAttribute|error - Successfully created the List Attribute
getListAttribute
function getListAttribute(int listAttributeId) returns ListAttribute|error
Read a List Attribute
Parameters
- listAttributeId int - the unique identifier for the List Attribute
Return Type
- ListAttribute|error - Successfully retrieved List Attribute
archiveListAttribute
Archive a List Attribute
Parameters
- listAttributeId int - The ID of the List Attribute you'd like to archive
updateListAttribute
function updateListAttribute(int listAttributeId, ListAttributeUpdate payload) returns ListAttribute|error
Update a List Attribute
Parameters
- listAttributeId int - The ID of the List Attribute you'd like to update
- payload ListAttributeUpdate - A string in JSON format that includes all the fields you'd like to change for a List Attribute
Return Type
- ListAttribute|error - Successfully updated the List Attribute
listPages
List Pages
Parameters
- projectId int - The Project ID of the Project you would like to list all Pages for
- perPage int (default 25) - Optional pagination argument that specifies the maximum number of objects to return per request
- page int (default 1) - Optional pagination argument that specifies the page to return. If you have 140 objects and you choose to return 100 objects per page you will be able to access the last 40 objects on page 2. The default value is 1.
createPage
Create a Page
Parameters
- payload Page - A string in JSON format that includes all the fields to create a Page
getPage
Read a page
Parameters
- pageId int - The Page ID of the Page you want to get
deletePage
Delete a Page
Parameters
- pageId int - The Page ID of the Page you want to delete
updatePage
function updatePage(int pageId, PageUpdate payload) returns Page|error
Update a Page
Parameters
- pageId int - The Page ID of the Page you want to change
- payload PageUpdate - A string in JSON format that includes all the fields you'd like to change for a Page
createInPageEvent
function createInPageEvent(int pageId, InPageEvent payload) returns InPageEvent|error
Create an In-Page Event
Parameters
- pageId int - The Page ID of the Page you want to create an In-Page Event for
- payload InPageEvent - A string in JSON format that includes all the fields to create an In-Page Event
Return Type
- InPageEvent|error - Return the created In-Page event
deleteInPageEvent
Delete an In-Page Event
Parameters
- pageId int - The Page ID of the In-Page Event you want to delete
- eventId int - The In-Page Event ID of the In-Page Event you want to delete
updateInPageEvent
function updateInPageEvent(int pageId, int eventId, InPageEventUpdate payload) returns InPageEvent|error
Update an In-Page Event
Parameters
- pageId int - The Page ID of the In-Page Event you want to change
- eventId int - The In-Page Event ID of the In-Page Event you want to change
- payload InPageEventUpdate - A string in JSON format that includes all the fields you'd like to change for an In-Page Event
Return Type
- InPageEvent|error - Successfully updated the In-Page event
getPlan
Get Plan & Usage information for all products
listProjects
List Projects
Parameters
- perPage int (default 25) - Optional pagination argument that specifies the maximum number of objects to return per request
- page int (default 1) - Optional pagination argument that specifies the page to return. If you have 140 objects and you choose to return 100 objects per page you will be able to access the last 40 objects on page 2. The default value is 1.
createProject
Create a Project
Parameters
- payload Project - A string in JSON format that includes all the fields to create a Project
getProject
Read a Project
Parameters
- projectId int - The Project ID of the Project you want to get
updateProject
function updateProject(int projectId, ProjectUpdate payload) returns Project|error
Update a Project
Parameters
- projectId int - The Project ID of the Project you want to change
- payload ProjectUpdate - A string in JSON format that includes all the fields you'd like to change for a Project
createCustomEvent
function createCustomEvent(int projectId, CustomEvent payload) returns CustomEvent|error
Create a Custom Event
Parameters
- projectId int - The Project ID of the Project you want to create an Custom Event in
- payload CustomEvent - A string in JSON format that includes all the fields to create a Custom Event
Return Type
- CustomEvent|error - Return the created Custom Event
deleteCustomEvent
Delete a Custom Event
Parameters
- projectId int - The project ID of the Project the Custom Event is stored in
- eventId int - The Event ID of the Custom Event you'd like to delete
updateCustomEvent
function updateCustomEvent(int projectId, int eventId, CustomEventUpdate payload) returns CustomEvent|error
Update a Custom Event
Parameters
- projectId int - The project ID of the Project the Custom Event is stored in
- eventId int - The Event ID of the Custom Event you'd like to change
- payload CustomEventUpdate - A string in JSON format that includes all the fields you'd like to change for a Custom Event
Return Type
- CustomEvent|error - Return the updated Event
getRecsCatalogCsv
Download a CSV with all current catalog data
Parameters
- date string - A string in JSON format that includes all the fields to create a Custom Event
- catalogId string - The Catalog ID of the Catalog you want to download
getRecsOutputCsv
function getRecsOutputCsv(string date, string catalogId, string recommenderId) returns Response|error
Download a CSV with all computed recommendations output data
Parameters
- date string - A string in JSON format that includes all the fields to create a Custom Event
- catalogId string - The Catalog ID of the Catalog which contains the Recommender
- recommenderId string - The Recommender ID of the Recommender you want to get output from
getRecsStatsCsv
Download a CSV with summary stats data
Parameters
- date string - A string in JSON format that includes all the fields to create a Custom Event
- catalogId string - The Catalog ID of the Catalog you want to download stats for
listSarRequestsByAccount
function listSarRequestsByAccount(int perPage, int page) returns SubjectAccessRequest[]|error
List Subject Access Requests
Parameters
- perPage int (default 25) - Optional pagination argument that specifies the maximum number of objects to return per request
- page int (default 1) - Optional pagination argument that specifies the page to return. If you have 140 objects and you choose to return 100 objects per page you will be able to access the last 40 objects on page 2. The default value is 1.
Return Type
- SubjectAccessRequest[]|error - Return Subject Access Request info
createSarRequest
function createSarRequest(SubjectAccessRequest payload) returns SubjectAccessRequest|error
Create a Subject Access Request
Parameters
- payload SubjectAccessRequest - A JSON string containing the fields needed to create a subject access request.
Return Type
- SubjectAccessRequest|error - Return the created SubjectAccessRequest
getSarRequest
function getSarRequest(int requestId) returns SubjectAccessRequest|error
Get Subject Access Request
Parameters
- requestId int - The ID of the Subject Access Request
Return Type
- SubjectAccessRequest|error - Return Subject Access Request info
Records
optimizely: Account
Fields
- id int? - The unique identifier of the Account
- name string? - The name of the Account
optimizely: AccountFeature
Fields
- feature_id string? -
- sources AccountFeatureSource[]? -
optimizely: AccountFeatureSource
Fields
- 'type string? -
- value string? -
optimizely: Action
Fields
- changes Change[]? - The list of changes to apply to the Page. If 'dependencies' is supplied in a Change within 'changes', each ID in 'dependencies' must also be in 'changes'.
- page_id int - The ID of the Page to apply changes to
- share_link string? - The share link for the provided Variation and Page combination
optimizely: Attribute
Fields
- archived boolean(default false) - Whether or not the Attribute has been archived
- condition_type string(default "custom_attribute") - Whether this Attribute is a custom dimension or custom attribute. If this is a custom dimension, it belongs to an Optimizely Classic experiment and is read-only.
- description string? - A short description of the Attribute
- id int? - The unique identifier for the Attribute
- 'key string - Unique string identifier for this Attribute within the project
- last_modified string? - The last time the Attribute was modified
- name string? - The name of the Attribute. For Full Stack projects, the name will be set to the value of the key.
- project_id int? - The ID of the project the Attribute belongs to
optimizely: AttributeUpdate
Fields
- archived boolean? - Whether or not the Attribute has been archived
- description string? - A short description of the Attribute
- 'key string? - Unique string identifier for this Attribute within the project
- name string? - The name of the Attribute
optimizely: Audience
Fields
- archived boolean(default false) - Whether the Audience has been archived
- conditions string? - A string defining the targeting rules for an Audience
- created string? - The time the Audience was initially created
- description string? - A short description of the Audience
- id int? - The unique identifier for the Audience
- is_classic boolean? - Whether or not Audience is a classic Audience. If true, the Audience is only compatible with classic Experiments. Otherwise, the Audience may be used in Optimizely X Campaigns.
- last_modified string? - The last time the Audience was modified
- name string? - The name of the Audience
- project_id int - The ID of the Project the Audience was created in
- segmentation boolean(default false) - True if the Audience is available for segmentation on the results page (Audiences can only be used for segmentation in Optimizely Classic). Set to False if you intend to use this Audience only in Optimizely X. Note that a maximum of 10 Audiences can have segmentation set to True in any given Optimizely Classic project.
optimizely: AudienceUpdate
Fields
- archived boolean? - True if the Audience has been archived
- conditions string? - A string defining the targeting rules for an Audience
- description string? - A short description of the Audience
- name string? - The name of the Audience
- segmentation boolean? - True if the Audience is available for segmentation on the results page (Enterprise plans only)
optimizely: BucketingInformation
Fields
- account_id int? - ID of the Account
- bucketing_history BucketingInformationItem[]? - Array of bucketing information items
- visitor_id string? - The unique identifier of the visitor
optimizely: BucketingInformationItem
Fields
- campaign_id int? - The unique identifier of the Campaign
- experiment_id int? - The unique identifier of the Experiment
- is_holdback boolean? - Is the visitor bucketed into a variation or a holdback
- timestamp int? - The timestamp of the bucketing decision on the client side
- variation_id int? - The unique identifier of the Variation
optimizely: Campaign
Fields
- changes SharedCodeChange[]? - A list of changes to apply to the Campaign. This corresponds to the Campaign's Shared Code in the application. Only supports 'custom_css' or 'custom_code' type changes.
- created string? - The time the Campaign was initially created
- description string? - The description or goal for a Campaign
- earliest string? - The first time the Campaign was activated
- experiment_priorities int[]? - A list of lists of Experiment IDs that indicate the relative priority of how to show those Experiments in the context of the Campaign. Each list inside of the list represents a group of Experiments of equal priority where groups that appear earlier in the list are of higher priority to be shown.
- holdback int? - Percentage of visitors to exclude from personalization, measured in basis points. 100 basis points = 1% traffic. For example, a value of 500 would mean that 95% of visitors will see a personalized experience and 5% will see the holdback.
- id int? - The unique identifier for the Campaign
- last_modified string? - The last time the Campaign was modified
- latest string? - The last time the Campaign was paused (not present if the Campaign still running)
- metrics Metric[]? - An ordered list of metrics to track for the Campaign
- name string? - The name of the Campaign
- page_ids int[]? - A list of Page IDs used in the Campaign
- project_id int - The Project ID the Campaign is in
- status string? - Current state of the Campaign. not_started means the Campaign has never been published to the world. running means the Campaign is currently live to the world. paused means the Campaign has been published, but is currently not running. archived means the Campaign is paused and not visible in the web UI.
- 'type string(default "personalization") - Indicates the type of this campaign. Campaigns created or fetched via the API should currently all have a type of
personalization
, but if you get a campaign_id for an experiment and look it up, you may get another
value.
- url_targeting URLTargeting? -
optimizely: CampaignMetricResults
Results specific to an Event
Fields
- aggregator string? - The aggregation function for the numerator of the metric. 'unique' measures the number of unique visitors/sessions that include the specified Event. 'count' measures the total number of occurrences of Event for the scope (visitor/session). 'sum' is the sum of the 'field' value
- event_id int? - The ID for the Event to select data from. Omitted for global metrics that are not relative to a specific Event, i.e. "overall revenue"
- 'field string? - The field to aggregate for the numerator of the metric. Required when 'aggregator' = 'sum', otherwise omitted
- name string? - The name of the Metric
- results record {}? - A map of results for the variants affected by the Campaign. Variants may represent aggregated results scoped to the Campaign and/or individual Experiment results scoped to just that Experiment. The special variant 'baseline' represents visitors that have been held back from any change in experience across all Experiments in the Campaign. The special variant 'campaign' represents the aggregated effect of all Experiments included in the Campaign.
- scope string? - Specifies how Events should be grouped together. Can also be thought of as the denonimator of the metric. 'session' divides by the number of sessions. "Influenced sessions", or sessions that do not contain a decision Event but carry a decision from a previous session are not included in counts for numerator or denominator. 'visitor' divides by the number of visitors. 'event' divides by the total occurrences (impressions) of the specified Event
- winning_direction string? - The winning direction of this metric
optimizely: CampaignResults
Fields
- campaign_id int? - The unique identifier for the Campaign
- confidence_threshold decimal? - The significance level at which you would like to declare winning and losing variations. A lower number minimizes the time needed to declare a winning or losing variation, but increases the risk that your results aren't true winners and losers.
- end_time string? - End of the time interval (exclusive) used to calculate the results. The time is formatted in ISO 8601.
- metrics CampaignMetricResults[]? - The breakdown of Campaign results by metric. CampaignMetricResults object ordering in the array is consistent with the order that metrics are attached to the Experiment in the Optimizely UI and REST API (i.e. index 0 is the primary metric, indices 1-4 are secondary metrics, indices 5+ are monitoring metrics). See here for an explantion of the impact of metric ordering on results calculations.
- start_time string? - Start of the time interval (inclusive) used to calculate the results. The time is formatted in ISO 8601.
optimizely: CampaignUpdate
Fields
- changes SharedCodeChange[]? - A list of changes to apply to the Campaign
- description string? - The description or goal for a Campaign
- experiment_priorities int[]? - A list of lists of Experiment IDs that indicate the relative priority of how to show those Experiments in the context of the Campaign. Each list inside of the list represents a group of Experiments of equal priority where groups that appear earlier in the list are of higher priority to be shown.
- holdback int? - Percentage of visitors to exclude from personalization, measured in basis points. 100 basis points = 1% traffic. For example, a value of 500 would mean that 95% of visitors will see a personalized experience and 5% will see the holdback.
- metrics Metric[]? - An ordered list of metrics to track for the Campaign
- name string? - The name of the Campaign
- page_ids int[]? - A list of Page IDs used in the Campaign. Only
url_targeting
orpage_ids
can be used when updating a Campaign, but not both.
- url_targeting URLTargeting? -
optimizely: Change
Fields
- allow_additional_redirect boolean? - Whether or not to allow additional redirects after redirecting to destination. Required for changes of type 'redirect'
- async boolean? - Indicates whether or not to execute the change asyncronously. If true, src will be returned in the response. Otherwise, it will be not included.
- attributes ChangeAttribute? -
- config record {}? - Configuration properties for the extension
- css CSSAttribute? -
- dependencies string[]? - A list of dependent change IDs that must happen before this change
- destination string? - URL to redirect to. Required for changes of type 'redirect'.
destination
anddestination_function
cannot be used at the same time
- destination_function string? - A function string to redirect to. Required for changes of type 'redirect'.
destination
anddestination_function
cannot be used at the same time
- extension_id string? - ID of the extension to insert. Required for changes of type 'extension'
- id string? - The ID of the change
- name string? - Name of the change
- operator string? - Where to instert HTML or image for types 'insert_html' and 'insert_image' with respect to the element(s) matched by 'selector'
- preserve_parameters boolean? - Whether or not to preserve parameters from original request when redirecting to new destination URL. Required for changes of type 'redirect'. For redirects using
destination_function
,preserve_parameters
must be false.
- rearrange record {}? - A directive to place the DOM element(s) matched by 'selector' to the position of the element matched by 'insertSelector', with the relation specified by 'operator'. The supplied example moves element matched by 'selector' above the element of class .greyBox
- selector string? - CSS selector to determine where changes are applied. Required for changes of type 'attribute', 'insert_html', and 'insert_image'
- src string? - The path to the change payload on the CDN. Only present if 'async' is True.
- 'type string - The type of this change.
- Changes of type 'attribute' have required fields 'selector' and 'attributes'
- Changes of type 'custom_code' have required field 'value'
- Changes of type 'custom_css' have required field 'value'
- Changes of type 'extension' have required field 'extension_id'
- Changes of type 'insert_html' have required field 'selector'
- Changes of type 'insert_image' have required field 'selector'
- Changes of type 'redirect' have required fields 'destination', 'preserve_parameters', and 'allow_additional_redirect'
- value string? - The value for the change can be custom Javascript or CSS as a string. Required for changes of type 'custom_css' and 'custom_code'
optimizely: ChangeAttribute
Fields
- 'class string? - Name of the class to set the element(s) matched by a selector to
- hide boolean? - Whether or not to hide the element(s) matched by a selector
- href string? - Value of href attribute to add to element(s) matched by a selector
- html string? - Value of HTML attribute to add to element(s) matched by a selector
- remove boolean? - Whether or not to remove the element(s) matched by a selector
- src string? - Value of src attribute to add to element(s) matched by a selector
- style string? - Value of style attribute to add to element(s) matched by a selector
- text string? - Value of text attribute to add to the element(s) matched by a selector
optimizely: ChangeDetail
Fields
- after record {}? - Value of the property after modification.
- before record {}? - Value of the property before modification.
- description string? - Human readable description of the change.
- property string? - The name of the property modified.
optimizely: ChangeHistoryChange
Fields
- change_type string? - Action performed on the entity.
- changes ChangeDetail[]? - List of change details.
- created string? - Created time of the current revision. Format is ISO 8601.
- entity Entity? -
- id int? - ID of the change.
- project_id int - ID of the project related to the entity.
- revisions ChangeRevision? -
- 'source string? - Origin of the current revision.
- summary string? - Short human readable description of the change.
- user User? -
optimizely: ChangeRevision
Fields
- current Revision? -
- previous Revision? -
optimizely: Choices
Fields
- label string? - label of the choice
- value string? - value of the choice
optimizely: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
optimizely: CollaboratorEntry
Fields
- id string? - The unique identifier of the user
- profile UserProfile? -
- project_roles ProjectRole[]? -
optimizely: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
optimizely: CredentialsItem
Credentials item containing information on access key and secrets
Fields
- accessKeyId string? - AWS access key
- expiration int? - expiration time of the token in epoch time
- secretAccessKey string? - AWS secret access key
- sessionToken string? - AWS session token
optimizely: CSSAttribute
Fields
- 'background\-color string? -
- 'background\-image string? -
- 'border\-color string? -
- 'border\-style string? -
- 'border\-width string? -
- color string? -
- 'font\-size string? -
- 'font\-weight string? -
- height string? -
- position string? -
- width string? -
optimizely: CurrentAccount
Fields
- features record {}? - The available features for the Account
- id int? - The unique identifier of the Account
optimizely: CustomEvent
Fields
- archived boolean? - Whether or not to archive this Event
- category EventCategories? - Event categories
- created string? - Creation date for this Event
- description string? - A description of this Event
- event_type string? - The type of this Event
- id int? - The unique identifier of the Event
- is_classic boolean(default false) - Whether or not this Event is a classic Event. If true, this Event is read-only
- is_editable boolean? - Whether or not this Event may be edited
- 'key string - Unique string identifier for this Event within the Project
- name string? - A human readable name for this Event. If unspecified, defaults to the key
- project_id int? - The ID of this Event's parent Project
optimizely: CustomEventUpdate
Fields
- archived boolean? - Whether or not to archive this Event
- category EventCategories? - Event categories
- description string? - A description of this Event
- 'key string? - Unique string identifier for this Event within the Project
- name string? - A human readable name for this Event
optimizely: Datafile
Fields
- id int? - ID of Datafile.
- latest_file_size int? - The current size in bytes of the Datafile.
- other_urls string[]? - List of other URLs where this Datafile is also available.
- revision int? - Current revision number.
- sdk_key string? - Unique key to identify this specific Environment and Datafile programmatically in our SDKs.
- url string? - URL where this Datafile is available.
optimizely: Datapoint
A value with statistical context
Fields
- confidence_interval decimal[]? - The confidence interval measures the uncertainty around improvement. It starts out wide and shrinks as more data comes in. Significance means that the confidence interval is completely above or completely below 0. If the result is significant and positive, the confidence interval will be above 0. If the result is significant and negative, confidence interval will be below 0. If the result is inconclusive, confidence interval includes 0
- confidence_interval_scaled decimal[]? - The confidence interval with bounds that are scaled by the baseline conversion rate.
- end_of_epoch boolean? - End of epoch
- is_most_conclusive boolean? - Indicates that this is the best performing variant for this metric. Also referred to as the 'Winner'
- is_significant boolean? - Indicates if significance is above your confidence threshold
- lift_status string? - Indicates whether a variation is doing better/worse than the baseline after taking the metric's winning direction into account.
- significance decimal? - The likelihood that the observed difference in conversion rate is not due to chance
- value decimal? - The estimated improvement for this variant compared to the baseline
- value_scaled decimal? - The estimated improvement scaled by the baseline conversion rate.
- visitors_remaining int? - The number of estimated visitors remaining before result becomes statistically significant. A value of 9223372036854775807 means the value is not available.
optimizely: E3Credentials
Fields
- credentials CredentialsItem? - Credentials item containing information on access key and secrets
- s3Path string? - s3 path of the enriched events dataset of the account
optimizely: Entity
Fields
- api_url string? - Generated URL to access the entity by using the public API.
- id int? - ID of the entity.
- name string? - Name of the entity.
- sub_type string? - Optional field for entities that have sub_types (projects, experiments, extensions, pages, etc)
- 'type string? - Type of the entity as defined by the public API.
- ui_url string? - Generated URL to access the entity using Optimizely UI.
optimizely: Environment
Fields
- archived boolean? - Boolean representing whether the Environment is archived.
- created string? - Created time.
- datafile Datafile? -
- description string? - A short description of the Environment.
- has_restricted_permissions boolean(default false) - Boolean representing whether starting experiments should be restricted to publishers and above in this Environment.
- id int? - ID of this Environment.
- is_primary boolean? - Boolean representing if this is the primary (default) Environment.
- 'key string - Unique string identifier for this Environment within the Project.
- last_modified string? - Last modification time.
- name string - Name of the Environment.
- priority int? - Integer representing the priority of the Environment. This is used for ordering in the UI.
- project_id int - ID of the project of the Environment.
optimizely: EnvironmentUpdate
Fields
- archived boolean? - Boolean representing whether the Environment is archived.
- description string? - Text description of the Environment.
- has_restricted_permissions boolean? - Boolean representing whether starting experiments should be restricted to publishers and above in this Environment.
- 'key string? - Unique string identifier for this Environment within the Project.
- name string? - Name of the Environment.
- priority int? - Integer representing the priority of the Environment. This is used for ordering in the UI.
optimizely: Error
Fields
- code string? -
- message string? -
- messages record {}? -
- uuid string? -
optimizely: Event
Fields
- archived boolean? - Whether or not this Event is archived
- category EventCategories? - Event categories
- config InPageEventConfig? - Inpage event config
- created string? - Creation date for this Event
- description string? - A description for this Event
- event_type string? - The type of this Event
- id int? - The unique identifier of the Event
- is_classic boolean? - Whether or not this Event is a classic Event. If true, this Event is read-only
- is_editable boolean? - Whether this Event may be edited
- 'key string? - Unique string identifier for this Event within the Project
- last_modified string? - The last time the Event was modified
- name string? - A human readable name for this Event
- page_id int? - The Page ID associated with this Event
- project_id int? - The ID of this Event's parent Project
optimizely: EventCategories
Event categories
Fields
- name string? - Event name
optimizely: Experiment
Fields
- allocation_policy string? - Traffic allocation policy across variations in this experiment
- audience_conditions string(default "everyone") - The audiences that should see this experiment. To target everyone, use the string "everyone" or omit this field. Multiple audiences can be combined with "and" or "or" using the same structure as audience conditions
- campaign_id int? - For Personalization experiences, this ID corresponds to the parent Campaign. For standalone experiments this campaign_id does not correspond to a campaign object.
- changes SharedCodeChange[]? - Custom CSS or JavaScript that will run before all variations in the Experiment (for Experiments in Web Projects only)
- created string? - The time when the Experiment was initially created
- description string? - The description or hypothesis for an Experiment
- earliest string? - The first time the Experiment was activated
- environments record {}? - String identifier for the Experiment's status in each Environment based on the environment key.
- feature_id int? - The ID of a Feature to attach to the Experiment. This turns an Experiment into a Feature Test.
- feature_key string? - The key for the Feature attached to the Experiment. Applies to Feature Tests only. Valid keys contain alphanumeric characters, hyphens, and underscores, and are limited to 64 characters.
- feature_name string? - The feature flag name to display in the Optimizely app. Whitespaces and other non-alphanumeric characters allowed. Defaults to feature key if left empty.
- holdback int? - Percent of traffic to exclude from the experiment, measured in basis points. 100 basis points = 1% traffic. For example, a value of 9900 would mean that 1% of visitors will be eligible for the experiment. This is only applicable for Web.
- id int? - The unique identifier for the Experiment
- is_classic boolean(default false) - Whether or not the Experiment is a classic Experiment. If true, the Experiment is read-only
- 'key string? - Unique string identifier for this Experiment within the Project. Only applicable for Full Stack and Mobile projects.
- last_modified string? - The last time the Experiment was modified
- latest string? - The last time the Experiment was paused (not present if the Experiment is still running). For campaign experiences, this field represents the last time the Campaign was paused.
- metrics Metric[]? - An ordered list of metrics to track for the Experiment. Required for Web, Full Stack, and Mobile Experimentation. Not applicable for Web Personalization Experiences.
- multivariate_traffic_policy string? - For Experiments of
multivariate
type, this specifies how the weights and statuses of combinations will be decided. Infull_factorial
mode, | combination weights are read-only, and are generated by multiplying together weights of section variations.
- name string? - Name of the Experiment. Required for Web Experimentation. Optional for Web Personalization experiences and Full Stack experiments. Not applicable for Mobile Experiments.
- page_ids int[]? - A list of Page IDs used in the Experiment.
url_targeting
orpage_ids
, but not both.
- project_id int - The Project the Experiment is in
- results_token string? - temporary token based on experiment id, used to access data platform services from other parts of the product
- schedule Schedule? -
- status string? - Current state of the Experiment.<br> In Full Stack, this is the Experiment's state in the primary (production) environment.
- traffic_allocation int? - Percent of traffic allocated for the experiment, measured in basis points. 100 basis points = 1% traffic. For example, a value of 5500 would mean that 55% of visitors will be eligible for the experiment. This is only applicable for Full Stack.
- 'type string(default "a/b") - Indicates whether this is an
a/b
,multivariate
,feature
, ormultiarmed_bandit
test or an experience within apersonalization
campaign. Note that the default for this field isa/b
. If another test type is desired, populate this field with the appropriate string (from one of the possible values).
- url_targeting URLTargeting? -
- variations Variation[]? - A list of variations that each define an experience to show in the context of the Experiment for the purpose of comparison against each other
- whitelist ExperimentWhitelist[]? - A list containing the user IDs and variations of users who have been whitelisted
optimizely: ExperimentEnvironment
Fields
- status string - The Experiment's status in different Environments based on the environment key.
optimizely: ExperimentEnvironmentUpdate
Fields
- status string - Update the Experiment's status in different Environments based on the environment key.
optimizely: ExperimentMetricConclusion
Experiment metric conclusion.
Fields
- loser string? - ID of the variation that has reached statistical significance and has performed worse than all other variations.
- winner string? - ID of the variation that has reached statistical significance and has performed better than all other variations.
optimizely: ExperimentMetricResults
Results specific to an Event
Fields
- aggregator string? - The aggregation function for the numerator of the metric. 'unique' measures the number of unique visitors/sessions that include the specified Event. 'count' measures the total number of occurrences of Event for the scope (visitor/session). 'sum' is the sum of the 'field' value. 'exit' measures the ratio of sessions with last activation occurring on the target page to the sessions that activated the target page at least once during the session. 'bounce' measures the ratio of sessions that with first and last activation occurring on the target page to the sessions with first activation on the target page. For both 'exit' and 'bounce', the eventId must be the ID of a Page.
- conclusion ExperimentMetricConclusion? - Experiment metric conclusion.
- event_id int? - The ID for the Event to select data from. Omitted for global metrics that are not relative to a specific Event, i.e. "overall revenue"
- 'field string? - The field to aggregate for the numerator of the metric. Required when 'aggregator' = 'sum', otherwise omitted
- name string? - The name of the Metric
- results record {}? - A map of results for each variation in the Experiment keyed by variation ID. For Personalization Campaigns, the special variant 'baseline' represents visitors that have been held back from any change in experience for the Experiment
- scope string? - Specifies how Events should be grouped together. Can also be thought of as the denonimator of the metric. 'session' divides by the number of sessions. "Influenced sessions", or sessions that do not contain a decision Event but carry a decision from a previous session are not included in counts for numerator or denominator. 'visitor' divides by the number of visitors. 'event' divides by the total occurrences (impressions) of the specified Event
- winning_direction string? - The winning direction of this metric
optimizely: ExperimentMetricTimeseries
Results time series specific to an Event
Fields
- aggregator string? - The aggregation function for the numerator of the metric. 'unique' measures the number of unique visitors/sessions that include the specified Event. 'count' measures the total number of occurrences of Event for the scope (visitor/session). 'sum' is the sum of the 'field' value
- event_id int? - The ID for the Event to select data from. Omitted for global metrics that are not relative to a specific Event, i.e. "overall revenue"
- 'field string? - The field to aggregate for the numerator of the metric. Required when 'aggregator' = 'sum', otherwise omitted
- name string? - The name of the Metric
- results record {}? - A map of results for each variation in the Experiment keyed by variation ID. For Personalization Campaigns, the special variant 'baseline' represents visitors that have been held back from any change in experience for the Experiment
- scope string? - Specifies how Events should be grouped together. Can also be thought of as the denonimator of the metric. 'session' divides by the number of sessions. "Influenced sessions", or sessions that do not contain a decision Event but carry a decision from a previous session are not included in counts for numerator or denominator. 'visitor' divides by the number of visitors. 'event' divides by the total occurrences (impressions) of the specified Event
- winning_direction string? - The winning direction of this metric
optimizely: ExperimentResults
Fields
- confidence_threshold decimal? - The significance level at which you would like to declare winning and losing variations. A lower number minimizes the time needed to declare a winning or losing variation, but increases the risk that your results aren't true winners and losers.
- end_time string? - End of the time interval (exclusive) used to calculated the results. The time is formatted in ISO 8601.
- experiment_id int? - The unique identifier for the Experiment
- metrics ExperimentMetricResults[]? - The breakdown of Experiment results by metric. ExperimentMetricResults object ordering in the array is consistent with the order that metrics are attached to the Experiment in the Optimizely UI and REST API (i.e. index 0 is the primary metric, indices 1-4 are secondary metrics, indices 5+ are monitoring metrics). See here for an explantion of the impact of metric ordering on results calculations.
- reach ExperimentVariationReach? -
- start_time string? - Start of the time interval (inclusive) used to calculate the results. The time is formatted in ISO 8601.
- stats_config StatsConfig? - Stats Engine configuration settings
optimizely: ExperimentResultsReport
Fields
- end_time string? - End of the time interval (exclusive) used to generate the report. The time is formatted in ISO 8601.
- experiment_id int? - The unique identifier for the Experiment for which the report is to be generated.
- inferred_outcome string? - Outcome of the experiment as inferred by the Optimizely system upon the evaluation of variations that may or may not have reached statistical significance.
- start_time string? - Start of the time interval (inclusive) used to generate the reports. The time is formatted in ISO 8601.
optimizely: ExperimentSummary
Fields
- archived boolean? - Whether or not the entity is archived.
- created string? - The time that the entity was created.
- description string? - Description for the entity if it has one.
- experiment_type string? - The type of experiment
- id int? - ID of the entity
- last_modified string? - The time that the entity was last modified.
- name string? - Name of the entity. This value will be the 'key' of the entity if there is no name.
- project_id int? - ID of the project that contains the entity (if applicable)
- project_name string? - Name of the project that contains the entity (if applicable)
- status string? - The current status for the entity if it has one.
- 'type string? - Type of the entity.
optimizely: ExperimentTimeseries
Fields
- confidence_threshold decimal? - The significance level at which you would like to declare winning and losing variations. A lower number minimizes the time needed to declare a winning or losing variation, but increases the risk that your results aren't true winners and losers.
- end_time string? - End of the time interval (exclusive) used to calculated the results. The time is formatted in ISO 8601.
- experiment_id int? - The unique identifier for the Experiment
- metrics ExperimentMetricTimeseries[]? - The breakdown of Experiment results time series by metric
- start_time string? - Start of the time interval (inclusive) used to calculate the results. The time is formatted in ISO 8601.
- stats_config StatsConfig? - Stats Engine configuration settings
optimizely: ExperimentUpdate
Fields
- audience_conditions string? - The audiences that should see this experiment. To target everyone, use the string "everyone". Multiple audiences can be combined with "and" or "or" using the same structure as audience conditions
- changes SharedCodeChange[]? - Custom CSS or JavaScript that will run before all variations in the Experiment (for Experiments in Web Projects only)
- description string? - The description or hypothesis for an Experiment
- environments record {}? - String identifier for the Experiment's status in each Environment based on the environment key.
- feature_id int? - The ID of a Feature to attach to the Experiment. This turns an Experiment into a Feature Test.
- holdback int? - Percent of traffic to exclude from the experiment, measured in basis points. 100 basis points = 1% traffic. For example, a value of 9900 would mean that 1% of visitors will be eligible for the experiment. This is only applicable for Web.
- 'key string? - Unique string identifier for this Experiment within the Project. Only applicable for Full Stack and Mobile projects.
- metrics Metric[]? - An ordered list of metrics to track for the Experiment
- name string? - Name of the Experiment
- page_ids int[]? - A list of Page IDs used in the Experiment. Only
url_targeting
orpage_ids
can be used when updating an experiment, but not both.
- schedule ScheduleUpdate? -
- traffic_allocation int? - Percent of traffic allocated for the experiment, measured in basis points. 100 basis points = 1% traffic. For example, a value of 5500 would mean that 55% of visitors will be eligible for the experiment. This is only applicable for Full Stack.
- url_targeting URLTargeting? -
- variations VariationUpdate[]? - List of IDs of all variations in the Experiment
- whitelist ExperimentWhitelist[]? - A list containing the user IDs and variations of users who have been whitelisted
optimizely: ExperimentVariationReach
Fields
- baseline_count int? - Baseline count
- baseline_reach decimal? - Baseline reach
- total_count int? - Total number of visitors exposed to the Experiment
- treatment_count int? - Treatment count
- treatment_reach decimal? - Treatment reach
- variations record {}? - A map of reach for each variation keyed by variation ID
optimizely: ExperimentWhitelist
Fields
- user_id string - The ID of the user being whitelisted
- variation_id int - The unique identifier for the variation
optimizely: Extension
Fields
- archived boolean? - Whether the extension is archived
- created string? - The time when the extension was initially created
- description string? - The description for the extension
- edit_url string - The URL to load when editing the extension
- enabled boolean? - Whether the extension is enabled. A disabled extension won't appear in the editor and won't be built into the snippet
- fields Field[]? - Array of editable fields in the extension
- id int? - The unique identifier for the extension
- implementation Implementation -
- last_modified string? - The last time when the extension was modified
- name string - Name of the extension
- project_id int - The project the extension is in
optimizely: ExtensionUpdate
Fields
- archived boolean? - Whether the extension is archived
- description string? - The description for the extension
- edit_url string? - The URL to load when editing the extension
- enabled boolean? - Whether the extension is enabled
- fields Field[]? - Array of editable fields in the extension
- implementation Implementation? -
- name string? - Name of the extension
optimizely: Feature
Fields
- archived boolean(default false) - Whether the Feature has been archived
- created string? - Time when the Feature was created
- description string? - A short description of this Feature
- environments record {}? - The configuration for this Feature's Rollout within each Environment, keyed by Environment key
- id int? - The ID of this Feature
- 'key string - Unique string identifier for this Feature within the Project
- last_modified string? - Date last modified
- name string? - Name of the Feature
- project_id int - The ID of the Project this Feature belongs to
- variables FeatureVariable[]? - Variables define the dynamic configuration of a feature, and each variable can take on a different value on a per-variation basis within a feature test.
optimizely: FeatureEnvironment
Fields
- id int? - The ID of the Environment this set of Rollout Rules applies to
- is_primary boolean? - Whether the Environment this set of Rollout Rules applies to is the primary Environment
- rollout_rules RolloutRule[] - Rollout Rules allow you to define groups of users and a percentage of those users that will see a Feature by default. You are currently limited to a single rollout rule. Audience IDs sent in any environment must always match that of the primary environment.
optimizely: FeatureUpdate
Fields
- archived boolean? - Whether the Feature has been archived
- description string? - A short description of this Feature
- environments record {}? - The configuration for this Feature's Rollout within each Environment, keyed by Environment key.
- 'key string? - Unique string identifier for this Feature within the Project
- name string? - Name of the Feature
- variables FeatureVariableUpdate[]? - Variables define the dynamic configuration of a feature, and each variable can take on a different value on a per-variation basis within a feature test.
optimizely: FeatureVariable
Fields
- archived boolean(default false) - Whether or not this Feature Variable is archived
- default_value string - The stringified default value for this Feature Variable. The default value is the value Optimizely SDKs will return when this Feature Variable is accessed by getFeatureVariableValue unless the Feature Variable's value is a part of a feature test variation.
- id int? - The ID of this Feature Variable
- 'key string - Unique string identifier for this Feature Variable within the Feature
- 'type string - The datatype for this Feature Variable
optimizely: FeatureVariableUpdate
Fields
- archived boolean? - Whether or not this Feature Variable is archived
- default_value string? - The stringified default value for this Feature Variable. The default value is the value Optimizely SDKs will return when this Feature Variable is accessed by getFeatureVariableValue unless the Feature Variable's value is a part of a feature test variation.
- description string? - A short description of this Feature Variable
- id int? - The ID of this Feature Variable
- 'key string? - Unique string identifier for this Feature Variable within the Feature
- 'type string? - The datatype for this Feature Variable
optimizely: Field
Fields
- api_name string - The API name of the field
- default_value string - A string in JSON format that corresponds to the default_value of the field
- field_type string - The type of the field
- label string - The label of the field
- options Options? -
optimizely: Group
Fields
- archived boolean? - Whether the group is archived
- created string? - The time when the Exclusion Group was initially created
- description string? - The description for an Exclusion Group
- entities GroupEntity[]? - Array of Group Entities in the Exclusion Group
- id int? - The unique identifier for the Exclusion Group
- last_modified string? - The last time the Exclusion Group was modified
- name string - Name of the Exclusion Group
- project_id int - The Project the Exclusion Group is in
optimizely: GroupEntity
Fields
- id int - The unique identifier for the Group Entity
- kind string - The kind of the Group Entity
- weight int - The weight of the Group Entity. The weight is a value between 0 and 10000. An entity with weight 5000 will receive 50% of this group's traffic
optimizely: GroupUpdate
Fields
- archived boolean? - Whether the Exclusion Group has been archived
- description string? - The updated description of the Exclusion Group
- entities GroupEntity[]? - Array of experiments or campaigns in the Exclusion Group, represented as a GroupEntity object
- name string? - The updated name of the Exclusion Group
optimizely: Implementation
Fields
- apply_js string? - The Apply JS code is used to inject the extension onto the page
- css string? - Some extensions may not need any CSS because they inherit styles from the page itself. However, you can add additional styling here. This will be injected on the page through a <style> tag
- html string? - The html implementation of the field
- reset_js string? - Reset JS is used to "clean up" after a extension. It's used in the editor, when changing field values or removing an existing extension. Reset JS should remove the element and any other side-effects
optimizely: ImpressionsUsage
Fields
- experiment_id int - The ID for the experiment.
- experiment_name string - The Experiment name to display in the Optimizely app. Whitespaces and other non-alphanumeric characters allowed. Defaults to experiment key if left empty.
- experiment_status string - The status of the Experiment.
- impression_count int - The Impressions count at experiment level.
- platform string - The platform of the Project
- project_id int - The ID for the project.
- project_name string - The name of the Project.
optimizely: ImpressionsUsageSummary
Fields
- allowance int - Total allowance purchased for the account.
- end_date string - Current subscription end date.
- last_update_date string - Last impressions usage updated date.
- start_date string - Current subscription start date.
- usage int - Usage for the account.
- usage_percentage decimal - Utilised usage percentage.
optimizely: InPageEvent
An Event that can occur only within the context of a specific Page (allowed in Web Projects only)
Fields
- archived boolean? - Whether or not this Event is archived
- category EventCategories? - Event categories
- config InPageEventConfig? - Inpage event config
- created string? - Creation date for this Event
- description string? - A description of this Event
- event_type string - The type of this Event
- id int? - The unique identifier of the Event
- is_classic boolean(default false) - Whether or not this Event is a classic Event. If true, the Event is read-only
- is_editable boolean? - Whether or not this Event may be edited
- 'key string? - Unique string identifier for this Event within the Project
- name string - A human readable name for this Event
- page_id int? - The Page ID associated with this Event
- project_id int? - The ID of this Event's parent Project
optimizely: InPageEventConfig
Inpage event config
Fields
- selector string - CSS selector for the page element(s) that trigger an Event
optimizely: InPageEventUpdate
Fields
- archived boolean? - Whether or not to archive this Event
- category EventCategories? - Event categories
- config InPageEventConfig? - Inpage event config
- description string? - A description of this Event
- 'key string? - Unique string identifier for this Event within the Project
- name string? - A human readable name for this Event
- page_id int? - The Page ID associated with this Event
optimizely: ListAttribute
Fields
- account_id int? - The unique identifier for the Optimizely account
- archived boolean(default false) - Whether or not the List Attribute has been archived
- aws_access_key string? - AWS access key to upload List Attribute source file to S3
- aws_secret_key string? - AWS secret key to upload List Attribute source file to S3
- created string? - Time when the List Attribute was created
- description string? - A short description of the List Attribute
- id int? - The unique identifier for the List Attribute
- key_field string - The name of the object which holds targeting ids on your website
- last_modified string? - The last time the List Attribute was modified
- list_content string? - A comma separated string of IDs or ZIP Codes. Items will be matched against the key_field to determine if an active visitor should be targeted by the list. Note that if the list currently contains data, providing this value will overwrite the previous data.
- list_type string? - The type of data object which holds targeting ids on your website (cookies, query parameters, zip codes, Global JS variables)
- name string - A unique, human-readable name for the List Attribute
- project_id int - The ID of the project the List Attribute belongs to
- s3_path string? - S3 path containing files used to populate the List Attribute with data. Can be used with an S3 client or CLI to upload data.
optimizely: ListAttributeUpdate
Fields
- archived boolean? - Whether or not the List Attribute has been archived
- description string? - A short description of the List Attribute
- key_field string? - The name of the object which holds targeting ids on your website
- list_content string? - A comma separated string of IDs or ZIP Codes. Items will be matched against the key_field to determine if an active visitor should be targeted by the list. Note that if the list currently contains data, providing this value will overwrite the previous data.
- list_type string? - The type of data object which holds targeting ids on your website (cookies, query parameters, zip codes, Global JS variables)
- name string? - A unique, human-readable name for the List Attribute
optimizely: Me
Fields
- accounts Account[]? - List of Accounts this user can access
- current_account CurrentAccount? -
- id string? - The unique identifier of the current User
- profile UserProfile? -
- project_roles ProjectRole[]? - List of Project IDs in the current Account with the current User's role information
optimizely: Metric
Fields
- aggregator string? - The aggregation function for the numerator of the metric. 'unique' measures the number of unique visitors/sessions that include the specified Event. 'count' measures the total number of occurrences of Event for the scope (visitor/session). 'sum' is the sum of the 'field' value
- event_id int? - The ID for the Event to select data from. Omitted for global metrics that are not relative to a specific Event, i.e. "overall revenue"
- 'field string? - The field to aggregate for the numerator of the metric. Required when 'aggregator' = 'sum', otherwise omitted
- scope string? - Specifies how Events should be grouped together. Can also be thought of as the denonimator of the metric. 'session' divides by the number of sessions. "Influenced sessions", or sessions that do not contain a decision Event but carry a decision from a previous session are not included in counts for numerator or denominator. 'visitor' divides by the number of visitors. 'event' divides by the total occurrences (impressions) of the specified Event
- winning_direction string? - The winning direction of this metric
optimizely: OAuth2RefreshTokenGrantConfig
OAuth2 Refresh Token Grant Configs
Fields
- Fields Included from *OAuth2RefreshTokenGrantConfig
- refreshUrl string(default "https://app.optimizely.com/oauth2/token") - Refresh URL
optimizely: Options
Fields
- choices Choices[]? - The choices for a field
optimizely: Page
Fields
- activation_code string? - Stringified Javascript function that determines when the Page is activated. Only required when activation_type is 'polling' or 'callback'.
- activation_type string? - Page activation type is a trigger that determines when the page is activated. Triggers tell Optimizely when to start checking whether certain conditions are true 'Immediate' activation mode activates the page as soon as the snippet loads. 'Polling' activation mode polls every 50ms until 'activation_code' evaluates to True, then activates the page. 'Callback' activation mode activates the page when the event defined by 'activation_code' is triggered. 'Manual' activation mode requires code within the subject app to explicitly trigger page activation. 'DOM Changed' sets the page to trigger when the DOM changes Learn more. 'URL Changed' sets the page to trigger when the URL changes Learn more.
- archived boolean(default false) - Whether the Page has been archived
- category string(default "other") - The category this Page is grouped under
- conditions string? - Stringified array of the conditions that activate the Page. The array contains Page Condition JSON dicts joined by "and" and "or". Each individual Page Condition dict has format {"type": "url", "match_type": <match_type>, "value": <value>} where match_types are: "simple" match type will match if "value" matches the hostname and path of the Page URL. "exact" match type will match only an exact string match between "value" and the Page URL. "substring" match type will match if "value" is a substring of the Page URL. "regex" match type will match if "value" is a regular expression match for the Page URL.
- created string? - Date created
- edit_url string - URL of the Page
- id int? - The unique identifier of the Page
- 'key string? - Unique string identifier for this Page within the Project
- last_modified string? - Date last modified
- name string - Name of the Page
- page_type string? - Type of Page
- project_id int - ID of the Page's Project
optimizely: PageUpdate
Fields
- activation_code string? - Stringified Javascript function that determines when the Page is activated. Only required when activation_type is 'polling' or 'callback'.
- activation_type string? - Page activation type is a trigger that determines when the page is activated. Triggers tell Optimizely when to start checking whether certain conditions are true 'Immediate' activation mode activates the page as soon as the snippet loads. 'Polling' activation mode polls every 50ms until 'activation_code' evaluates to True, then activates the page. 'Callback' activation mode activates the page when the event defined by 'activation_code' is triggered. 'Manual' activation mode requires code within the subject app to explicitly trigger page activation. 'DOM Changed' sets the page to trigger when the DOM changes Learn more. 'URL Changed' sets the page to trigger when the URL changes Learn more.
- archived boolean? - Whether the Page is archived
- category string? - The category this Page is grouped under
- conditions string? - Stringified array of the conditions that activate the Page. The array contains Page Condition JSON dicts joined by "and" and "or". Each individual Page Condition dict has format {"type": "url", "match_type": <match_type>, "value": <value>} where match_types are: "simple" match type will match if "value" matches the hostname and path of the Page URL. "exact" match type will match only an exact string match between "value" and the Page URL. "substring" match type will match if "value" is a substring of the Page URL. "regex" match type will match if "value" is a regular expression match for the Page URL.
- edit_url string? - URL of the Page
- 'key string? - Unique string identifier for this Page within the Project
- name string? - Page Name
- page_type string? - Type of Page
optimizely: Plan
Fields
- account_id int? - The account ID of the account that this Plan & Usage information is associated with
- plan_name string? - The name of the plan for the current account
- product_usages ProductUsage[]? - Array of products under this account
- status string? - The status of the plan for the current account
- unit_of_measurement string? - The unit by which we measure the
usage
of this account
optimizely: ProductUsage
Fields
- allocation_term_in_months int? - The current allocation term length in months. The allocation term is the time between the
start_time
and theend_time
. For example theusage
for an account with anallocation_term_in_months
of 6 has started accumulating on thestart_time
and will reset to 0 atend_time
(6 months later)
- end_time string? - The end date of the current allocation term period. For monthly paying accounts, the current allocation term period means the current billing month
- last_update_time string? - The last time that the
unit_of_measurement
count was updated
- overage_cents_per_visitor float? - (optional) The cost in cents for every visitor when the number of
unit_of_measurement
has exceeded theusage_allowance
. This value is only set for accounts with a limitedusage_allowance
- product_name string? - The product name
- projects record {}? - Key-value map of
usage
per project under this account and this product. Keys are project IDs, values areusage
numbers. Only available for the Impressions metrics, otherwise omitted.
- start_time string? - The start date of the current allocation term period. For monthly paying accounts, the current allocation term period means the current billing month
- usage int? - The total
usage
in theunit_of_measurement
within the current allocation term
- usage_allowance int? - (optional) The total
usage
allowed in theunit_of_measurement
for the current allocation term. This value is only set for accounts with a limitedusage_allowance
optimizely: Project
Fields
- account_id int? - The account the Project is associated with
- confidence_threshold decimal? - The significance level at which you would like to declare winning and losing variations. A lower number minimizes the time needed to declare a winning or losing variation, but increases the risk that your results aren't true winners and losers. The precision for this number is up to 4 decimal places
- created string? - The time that the Project was originally created
- dcp_service_id int? - The ID of a Dynamic Customer Profile Service associated with this Project
- description string? - A short description of the Project
- id int? - The unique identifier for the Project
- is_classic boolean? - If
is_classic
is true it means that the project doesn't have Optimizely X enabled. In other words: this project is Optimizely Classic only. The v2 REST API can only be used for Optimizely X objects (with the exception of Audiences and Projects, which can be shared between Optimizely X and Classic), so whenis_classic
is set to true you will need to use the v1 API
- last_modified string? - The time the Project was last modified
- name string - The name of the Project
- platform string(default "web") - The platform of the Project
- sdks string[]? - For Full Stack, Mobile, and OTT projects, the language used for the SDK
- socket_token string? - The token used to identify your mobile app to Optimizely (mobile only)
- status string(default "active") - The current status of the Project
- third_party_platform string? - The third party platform with which the project is intended to be used. When this is set, a project might have special restrictions. This can have a value of "salesforce" but defaults to null. In order to set this field, an account must have the third party platforms feature and be a fullstack project.
- web_snippet WebSnippet? -
optimizely: ProjectRole
Fields
- invitation_status string? -
- project_id int? - The unique identifier of the Project
- role string? -
optimizely: ProjectUpdate
Fields
- account_id int? - The ID of the account the Project is associated with
- confidence_threshold decimal? - The significance level at which you would like to declare winning and losing variations. A lower number minimizes the time needed to declare a winning or losing variation, but increases the risk that your results aren't true winners and losers. The precision for this number is up to 4 decimal places.
- dcp_service_id int? - The ID of a Dynamic Customer Profile Service associated with this Project
- description string? - A short description of the Project
- name string? - The name of the Project
- status string? - The current status of the Project
- web_snippet WebSnippetUpdate? -
optimizely: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
optimizely: ResultsShareLink
Fields
- url string? - The share link for the results of this Campaign
optimizely: Revision
Fields
- id int? - Reference to a revision of the entity.
optimizely: RolloutRule
Fields
- audience_conditions string? - The audiences that should see this feature. To target everyone, use the string "everyone". Multiple audiences can be combined with "and" or "or" using the same structure as audience conditions. <code>audience_conditions</code> sent in any environment must always match those of the primary environment.
- enabled boolean? - Whether or not the Rollout Rule is applied in this Environment. You can toggle this on and off by sending True or False.
- percentage_included int? - The percentage of the designated audiences that should get this Feature, measured in basis points. 100 basis points = 1% traffic.
optimizely: Schedule
Fields
- start_time string? - The start time for the Experiment, in date-time or date format (as defined by ISO 8601), and rounded to the nearest minute. If only date is supplied without time, the start time defaults to 00:00 on the specified start date.
- stop_time string? - The stop time for the Experiment, in date-time or full-date format (as defined by ISO 8601), and rounded to the nearest minute. If only date is supplied without time, the stop time defaults to 00:00 on the specified stop date.
- time_zone string? - The time zone to use for Experiment start and stop times with respect to an IANA time zone (ex. "America/New_York"). The time zones expressed by GMT (e.g. "GMT-08:00") are no longer supported.
optimizely: ScheduledJob
Fields
- account_id int? - The account that the job is associated with
- archived boolean(default false) - Whether the Scheduled Job is archived
- campaign_ids int[]? - A list of campaign_ids that should be considered during the job
- experiment_ids int[]? - A list of experiment_ids that should be considered during the job
- frequency string - How often the job should be performed
- id int? - The unique identifier of the Scheduled Job
- job_type string - The type of job to be performed
- output_channels record {}? - An object describing how the output of the job should be communicated
- project_id int - The project that the job is associated with
optimizely: ScheduledJobUpdate
Fields
- archived boolean? - Whether the Scheduled Job is archived
- campaign_ids int[]? - A list of campaign_ids that should be considered during the job
- experiment_ids int[]? - A list of experiment_ids that should be considered during the job
- frequency string? -
- job_type string? - The type of job to be performed
- output_channels record {}? - An object describing how the output of the job should be communicated
- project_id int? - The project that the job is associated with
optimizely: ScheduleUpdate
Fields
- start_time string? - The start time for the Experiment, in date-time or date format (as defined by ISO 8601), and rounded to the nearest minute. If only date is supplied without time, the start time defaults to 00:00 on the specified start date.
- stop_time string? - The stop time for the Experiment, in date-time or full-date format (as defined by ISO 8601), and rounded to the nearest minute. If only date is supplied without time, the stop time defaults to 00:00 on the specified stop date.
- time_zone string? - The time zone to use for Experiment start and stop times with respect to an IANA time zone (ex. "America/New_York"). The time zones expressed by GMT (e.g. "GMT-08:00") are no longer supported.
optimizely: SearchResult
Fields
- archived boolean? - Whether or not the entity is archived.
- created string? - The time that the entity was created.
- description string? - Description for the entity if it has one.
- experiment_type string? - The type of experiment
- feature_key string? - The feature flag key
- feature_name string? - The feature flag name to display in the Optimizely app. Whitespaces and other non-alphanumeric characters allowed. Defaults to feature key if left empty.
- id int? - ID of the entity
- 'key string? - Key of the entity.
- last_modified string? - The time that the entity was last modified.
- name string? - Name of the entity. This value will be the 'key' of the entity if there is no name.
- project_id int? - ID of the project that contains the entity (if applicable)
- project_name string? - Name of the project that contains the entity (if applicable)
- status string? - The current status for the entity if it has one.
- 'type string? - Type of the entity.
optimizely: Section
Fields
- archived boolean(default false) - Whether or not the Section has been archived
- description string? - A short description of this Section
- experiment_id int? - The ID of the Multivariate Test this Section belongs to
- id int? - The ID of this Section
- name string? - The name of this Section
- project_id int? - The ID of the project that this Section belongs to
- variations Variation[] -
optimizely: SectionUpdate
Fields
- archived boolean? - Whether or not the Section has been archived
- description string? - A short description of this Section
- name string? - The name of this Section
- variations Variation[]? -
optimizely: SharedCodeChange
Fields
- async boolean? - Indicates whether or not to execute the change asyncronously. If true, src will be returned in the response. Otherwise, it will be not included.
- dependencies string[]? - A list of dependent change IDs that must happen before this change
- id string? - The ID of the change
- name string? - Name of the change
- selector string? - CSS selector to determine where changes are applied. Required for changes of type 'custom_css'.
- src string? - The path to the change payload on the CDN. Only present if 'async' is True.
- 'type string - The type of this change.
- value string - The value for the change can be JavaScript or CSS as a string.
optimizely: StatsConfig
Stats Engine configuration settings
Fields
- confidence_level decimal? - Confidence level
- difference_type string? - The type of test to compare the variant to baseline
- epoch_enabled boolean? - Indicates if epoch-based statistics were used
optimizely: SubjectAccessRequest
Fields
- account_id int? - The Account ID for the account the Subject Access Request will be executed.
- completed_at_time string? - The time when the request was completed.
- data_type string - <p>The type of data to be accessed or deleted. The two options are:</p> <ul> <li><code>user</code> - End users (also known as <em>Collaborators</em>) that are added to the accounts of our customers. A user can be a <a href="https://help.optimizely.com/Set_Up_Optimizely/Manage_collaborators_in_Optimizely_X" rel="internal"><u>collaborator</u></a> on multiple accounts.</li> <li><code>visitor</code> - Visitors who visit or use our customers’ websites, apps and other digital products. Optimizely stores visitor data to calculate experiment results and to tailor content.</li> </ul>
- expired_at_time string? - Time when the url expires (7 days from the completed_at_time)
- export_location string? - The location to which the data will be exported. The data will be made accessible in a AWS S3 bucket.
- id int? - The Subject Access Request ID.
- identifier string - The identifier value that you would like us to use when searching. If <code>user</code> was selected in the previous step, the identifier will be the email address for the User.
- identifier_type string - <p>User data is identified by the email address used to create the end user account. The endpoint only accepts the <code>email</code> datatype if you selected <code>user</code> for <strong>Datatype</strong>.<br> <br> If you selected <code>visitor</code> for <strong>data_type</strong>, you can select 5 options for personal identifier types:</p> <ul> <li> <p><code>dcp_id</code> - Any ID used to identify targeting records in Optimizely.</p> </li> <li> <p><code>email</code> - The email address of a visitor.</p> </li> <li> <p><code>fullstack_id</code> - The unique identifier used for Full Stack experiments.</p> </li> <li> <p><code>optimizely_end_user_id</code> - An Optimizely generated user cookie.</p> </li> <li> <p><code>other</code> - Any other identifier that was uploaded to Optimizely.</p> </li> </ul>
- processing_started_time string? - The time when the processing of the Subject Access Request started.
- request_type string - <code>delete</code> - Removes all data within an account that is associated to the identifier defined in the identifier field. <br> <code>access</code> - Finds all data stored in Optimizely systems associated to the identifier defined in the identifier field and exports it to an AWS S3 bucket for you to access.
- requested_at_time string? - The time when the Subject Access Request was submitted.
- sla_deadline_time string? - The time by which the Subject Access Request must be completed.
- status string? - The status of the Subject Access Request.
optimizely: SubjectAccessRequestInternal
Fields
- account_id int? - Admin account id
- completed_at_time string? - Time when the request was completed
- data_source string - Subject Access Request Data Source
- data_type string - Subject Access Request Data Type
- expired_at_time string? - Time when the url expires (7 days from the completed_at_time)
- export_location string? - The location to which the data will be exported.
- id int? - Subject Access Request ID
- identifier string - Subject Access Request Identifier
- identifier_type string - Subject Access Request Identifier Type
- parent_request_id int? - Parent Subject Access Request id
- processing_started_time string? - Time when the processing of the request started
- request_type string - Subject Access Request Request Type
- requested_at_time string? - Time when the request was submitted
- sla_deadline_time string? - Time by which the request must be deleted
- status string? - Status of the Subject Access Request
optimizely: SubjectAccessRequestUpdate
Fields
- completed_at_time string? - Time when the request was completed
- export_location string? - s3 path to the Subject Access Request's ZIP file in the optimizely-sar-prod bucket
- processing_started_time string? - Time when the processing of the request started
- status string - Status of the Subject Access Request
optimizely: TimeseriesDatapoint
Fields
- lift Datapoint? - A value with statistical context
- rate decimal? - The value of the metric after dividing the numerator value over the denominator value
- samples decimal? - The value of the denominator
- time string? - Time of this data point formatted in ISO 8601
- value decimal? - The value of the numerator
- variance decimal? - The variance of the metric value
optimizely: URLTargeting
Fields
- activation_code string? - Stringified Javascript function that determines when the Page is activated. Only required when activation_type is 'polling' or 'callback'.
- activation_type string? - How this page is activated. See the full documentation on the Page object.
- conditions string? - Conditions to activate the experiment; our knowledge base article on Activation Types is the best guide for how to set up this data.
- edit_url string - URL to load in the editor for this page
- 'key string? - Unique string identifier for this Page within the Project
- page_id int? - The unique identifier of the Page that represents the experiment or campaign's URL Targeting.
optimizely: User
Fields
- display_name string? - Display name of the user, most commonly the Optimizely user's email.
- email string - Email of the user.
- first_name string? - First name of the user.
- id string - ID of the user.
- last_name string? - Last name of the user.
- profile_image_url string? - URL to the user profile image.
optimizely: UserProfile
Fields
- email string? - User's email
- first_name string? - User's first name
- last_name string? - User's last name
- profile_image string? - User's profile image URL
optimizely: UserProxy
Fields
- email string? - Email of the user proxy.
- id string - ID of the user proxy.
- proxy_type string - Type of user proxy.
optimizely: VariantResults
Fields
- experiment_id int? - The unique identifier for the Experiment this entity contains results for (if applicable)
- is_baseline boolean? - Indicates that this variant is the baseline that all other entities will be compared against. Also referred to as the 'Control' or 'Control Group'
- level string? - The level of entity that this variant represents
- lift Datapoint? - A value with statistical context
- name string? - The name of the variant
- rate decimal? - The value of the metric after dividing the numerator value over the denominator value
- samples decimal? - The value of the denominator
- total_increase Datapoint? - A value with statistical context
- value decimal? - The value of the numerator
- variance decimal? - The variance of the metric value
- variation_id string? - The unique identifier for the variation this entity contains results for (if applicable)
optimizely: VariantTimeseries
Fields
- experiment_id int? - The unique identifier for the Experiment this entity contains results for (if applicable)
- is_baseline boolean? - Indicates that this variant is the baseline that all other entities will be compared against. Also referred to as the 'Control' or 'Control Group'
- level string? - The level of entity that this variant represents
- name string? - The name of the variant
- timeseries TimeseriesDatapoint[]? - The results time series data points
- variation_id string? - The unique identifier for the variation this entity contains results for (if applicable)
optimizely: Variation
Fields
- actions Action[]? - A collection of changes to run for each page in an experiment. Only applicable to Optimizely X Web.
- archived boolean? - Whether the variation is archived
- description string? - A description of the variation.
- feature_enabled boolean? - For Feature Tests, indicates if the feature should be enabled for the variation
- 'key string? - Unique string identifier for this variation within the Experiment. Only applicable for Full Stack and Mobile projects.
- name string? - The name of the variation. Required for Web Experiments and Personalization experiences. Not required for Full Stack Experiments.
- status string? - Current status of the variation
- variable_values record {}? - For Feature Tests, the variable values for the variation represented as a map of Variable keys to their values.
- variation_id int? - The unique identifier for the variation
- weight int - The percentage of your visitors that should see this variation, measured in basis points. 100 basis points = 1% traffic. Variation weights must add up to 10000.
optimizely: VariationReach
Fields
- count int? - Total number of visitors exposed to this particular variation
- name string? - The name of the variation
- variation_id string? - The unique identifier for the variation
- variation_reach decimal? -
optimizely: VariationUpdate
Fields
- actions Action[]? - A collection of changes to run for each page in an experiment. Only applicable to Optimizely X Web.
- archived boolean? - Whether the variation is archived
- description string? - A description for the variation.
- feature_enabled boolean? - For Feature Tests, indicates if the feature should be enabled for the variation
- 'key string? - Unique string identifier for this variation within the Experiment. Only applicable for Full Stack and Mobile projects.
- name string? - The name of the variation. Required for Web Experiments and Personalization experiences. Not required for Full Stack Experiments.
- status string? - Current status of the variation
- variable_values record {}? - For Feature Tests, the variable values for the variation represented as a map of Variable keys to their values.
- variation_id int? - An immutable unique identifier for the variation. Required to update an existing variation.
- weight int - The percentage of your visitors that should see this variation, measured in basis points. 100 basis points = 1% traffic. Variation weights must add up to 10000.
optimizely: WebSnippet
Fields
- code_revision int? - The current revision number of the Project snippet
- enable_force_variation boolean? - Enables the option to force yourself into a specific variation on any page
- exclude_disabled_experiments boolean? - Set to true to remove paused and draft Experiment from the snippet
- exclude_names boolean? - Set to true to mask descriptive names
- include_jquery boolean? - Set to true to include jQuery in your snippet
- ip_anonymization boolean? - Set to true to change the last octet of IP addresses to 0 prior to logging
- ip_filter string? - A regular expression (max 1500 characters) matching ip addresses for filtering out visitors. Matching visitors will still see the Experiment, but they won't be counted in results.
- js_file_size int? - The current size in bytes of the Project snippet
- library string? - The prefered jQuery library version you would like to use with your snippet. If you do not want to include jQuery, set include_jquery to false
- project_javascript string? - The javascript code which runs before Optimizely on all pages, regardless of whether or not there is a running Experiment
- visitor_id_locator_name string? - (BETA) The name of said identifier that locates the visitor id
- visitor_id_locator_type string? - (BETA) The type of identifer where the visitor id is located (e.g. cookies, query param)
optimizely: WebSnippetUpdate
Fields
- enable_force_variation boolean? - Enables the option to force yourself into a specific variation on any page
- exclude_disabled_experiments boolean? - Set to true to remove paused and draft Experiment from the snippet
- exclude_names boolean? - Set to true to mask descriptive names
- include_jquery boolean? - Set to true to include jQuery in your snippet
- ip_anonymization boolean? - Set to true to change the last octet of IP addresses to 0 prior to logging
- ip_filter string? - A regular expression (max 1500 characters) matching ip addresses for filtering out visitors. Matching visitors will still see the Experiment, but they won't be counted in results.
- library string? - The prefered jQuery library version you would like to use with your snippet. If you do not want to include jQuery, set include_jquery to false
- project_javascript string? - The javascript code which runs before Optimizely on all pages, regardless of whether or not there is a running Experiment
- visitor_id_locator_name string? - (BETA) The name of said identifier that locates the visitor id
- visitor_id_locator_type string? - (BETA) The type of identifer where the visitor id is located (e.g. cookies, query param)
Import
import ballerinax/optimizely;
Metadata
Released date: about 1 year ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 0
Current verison: 0
Weekly downloads
Keywords
IT Operations/Testing Tools
Cost/Paid
Contributors