openweathermap
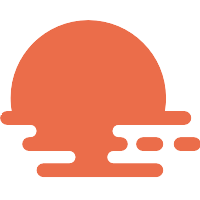
ballerinax/openweathermap Ballerina library
Overview
This is a generated connector from Open Weather Map API v2.5 OpenAPI Specification.
The Open Weather Map API provides access to current weather data and weather forecast of any location worldwide including 200,000 cities by consuming the Current Weather Data
and One Call
API endpoints.
Prerequisites
- Create OpenWeatherMap account.
- Choose a subscription that matches with your requirements
- Obtain Api Key
- Visit My API Keys and generate a new API Key
Quickstart
To use the Open Weather Map connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
import ballerinax/openweathermap;
Step 2: Create a new connector instance
Provide the obtained API Key in client configuration.
ApiKeysConfig config = { appid : "<your appid>" } openweathermap:Client openweatherClient = check new openweathermap:Client(config); };
Step 3: Invoke connector operation
- Obtain current weather data by city name.
CurrentWeatherData result = check weatherclient->getCurretWeatherData("Colombo"); log:printInfo("Colombo Current Weather data : " + result.toString());
- Use
bal run
command to compile and run the Ballerina program.
Clients
openweathermap: Client
This is a generated connector from Open Weather Map API version 2.5 OpenAPI Specification.
The Open Weather Map API provides access to current weather data and weather forecast of any location worldwide including 200,000 cities by consuming the Current Weather Data
and One Call
API endpoints.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Please create an account at https://openweathermap.org and an API key following this guide. Choose a subscription that matches with your requirements.
init (ApiKeysConfig apiKeyConfig, ClientConfiguration clientConfig, string serviceUrl)
- apiKeyConfig ApiKeysConfig - API keys for authorization
- clientConfig ClientConfiguration {} - The configurations to be used when initializing the
connector
- serviceUrl string "http://api.openweathermap.org/data/2.5/" - URL of the target service
getCurretWeatherData
function getCurretWeatherData(string? q, string? id, string? lat, string? lon, string? zip, string units, string lang, string mode) returns CurrentWeatherData|error
Access current weather data for any location. Note: All parameters are optional, but you must provide at least one parameter. Calling the API by city ID (using the id
parameter) will provide the most precise location results.
Parameters
- q string? (default ()) - City name, or city name and country code. For the query value, type the city name and optionally the country code divided by comma; use ISO 3166 country codes.
- lat string? (default ()) - Latitude
- lon string? (default ()) - Longtitude
- zip string? (default ()) - Zip code. Search by zip code. Example: 95050,us.
- units string (default "imperial") - Temperature in Fahrenheit use
units=imperial
, temperature in Celsius useunits=metric
, temperature inKelvin
is used by default.
- lang string (default "en") - Language
- mode string (default "json") - Format of response. Possible values are
xml
andhtml
. If mode parameter is empty the format isjson
by default.
Return Type
- CurrentWeatherData|error - Current weather data of the given location
getWeatherForecast
function getWeatherForecast(string lat, string lon, string? exclude, string? units, string? lang) returns WeatherForecast|error
Access to current weather, minute forecast for 1 hour, hourly forecast for 48 hours, daily forecast for 7 days and government weather alerts.
Parameters
- lat string - Latitude
- lon string - Longtitude
- exclude string? (default ()) - Exclude parts of the weather data from the API response. It should be a comma-delimited list (without spaces).
- units string? (default ()) - For temperature in Fahrenheit and wind speed in miles/hour, use
units=imperial
. For temperature in Celsius and wind speed in meter/sec, useunits=metric
. Temperature in Kelvin and wind speed in meter/sec is used by default, so there is no need to use the units parameter in the API call if you want this.
- lang string? (default ()) - Language
Return Type
- WeatherForecast|error - Weather forecast of the given location
Records
openweathermap: Alerts
Government weather alerts
Fields
- sender_name string? - Name of the alert source.
- event string? - Alert event name
- description string? - Description of the alert
- 'start decimal? - Date and time of the start of the alert, Unix, UTC
- end decimal? - Date and time of the end of the alert, Unix, UTC
- tags string[]? - Tags related to alerts
openweathermap: ApiKeysConfig
Provides API key configurations needed when communicating with a remote HTTP endpoint.
Fields
- appid string - Represents API Key
appid
openweathermap: Clouds
Nature of the clouds
Fields
- 'all int? - Cloudiness, %
openweathermap: Coord
City geo location
Fields
- lon decimal? - Longitude
- lat decimal? - Latitude
openweathermap: CurrentWeatherData
Current weather data
Fields
- coord Coord? - City geo location
- weather Weather[]? - More info Weather condition codes
- base string? - Internal parameter
- main Main? - Basic weather data
- visibility int? - Visibility, meter
- wind Wind? - Nature of the wind
- clouds Clouds? - Nature of the clouds
- rain Rain? - Nature of the rain
- snow Snow? - Snow volume information
- dt int? - Time of data calculation, unix, UTC
- sys Sys? - System data
- id int? - City ID
- name string? - City name
- cod int? - Internal parameter
openweathermap: Daily
Daily forecast weather data API response
Fields
- dt decimal? - Time of the forecasted data, Unix, UTC
- temp Temp? - Temperature data
- feels_like FeelsLike? - Human perception of temperature each time of the day
- moonrise decimal? - The time of when the moon rises for this day, Unix, UTC
- moonset decimal? - The time of when the moon sets for this day, Unix, UTC
- moon_phase decimal? - Moon phase. 0 and 1 are 'new moon', 0.25 is 'first quarter moon', 0.5 is 'full moon' and 0.75 is 'last quarter moon.
- pressure decimal? - Atmospheric pressure on the sea level, hPa
- humidity decimal? - Humidity, %
- dew_point decimal? - Atmospheric temperature below which water droplets begin to condense and dew can form.
- uvi decimal? - The maximum value of UV index for the day
- clouds decimal? - Cloudiness, %
- visibility decimal? - Visibility of the atmosphere
- wind_deg decimal? - Wind direction, degrees (meteorological)
- wind_gust decimal? - (where available) Wind gust. Units – default: metre/sec, metric: metre/sec, imperial: miles/hour.
- pop decimal? - Probability of precipitation
- weather Weather[]? - More info Weather condition codes
- rain decimal? - where available) Precipitation volume, mm
openweathermap: FeelsLike
Human perception of temperature each time of the day
Fields
- day decimal? - Day temperature.
- night decimal? - Night temperature.
- eve decimal? - Evening temperature.
- morn decimal? - Morning temperature.
openweathermap: ForecastCurrent
Current weather data API response
Fields
- dt decimal? - Current time, Unix, UTC
- sunrise decimal? - Sunrise time, Unix, UTC
- sunset decimal? - Sunset time, Unix, UTC
- temp decimal? - Temperature. Units - default: kelvin, metric: Celsius, imperial: Fahrenheit.
- feels_like decimal? - Temperature. This temperature parameter accounts for the human perception of weather.
- pressure decimal? - Atmospheric pressure on the sea level, hPa
- humidity decimal? - Humidity, %
- dew_point decimal? - Atmospheric temperature below which water droplets begin to condense and dew can form.
- uvi decimal? - Current UV index
- clouds decimal? - Cloudiness, %
- visibility decimal? - Average visibility, metres
- wind_speed decimal? - Wind speed. Wind speed. Units – default: metre/sec, metric: metre/sec, imperial: miles/hour.
- wind_deg decimal? - Wind direction, degrees (meteorological)
- weather Weather[]? - More info Weather condition codes
- rain Rain? - Nature of the rain
- snow Snow? - Snow volume information
openweathermap: Hourly
Hourly forecast weather data API response
Fields
- dt decimal? - Time of the forecasted data, Unix, UTC
- temp decimal? - Temperature. Units – default: kelvin, metric: Celsius, imperial: Fahrenheit.
- feels_like decimal? - Temperature. This accounts for the human perception of weather.
- pressure decimal? - Atmospheric pressure on the sea level, hPa
- humidity decimal? - Humidity, %
- dew_point decimal? - Atmospheric temperature below which water droplets begin to condense and dew can form.
- uvi decimal? - UV index
- clouds decimal? - Cloudiness, %
- visibility decimal? - Average visibility, metres
- wind_deg decimal? - Wind direction, degrees (meteorological)
- wind_gust decimal? - (where available) Wind gust. Units – default: metre/sec, metric: metre/sec, imperial: miles/hour.
- pop decimal? - Probability of precipitatio
- weather Weather[]? - More info Weather condition codes
- rain Rain? - Nature of the rain
openweathermap: Main
Basic weather data
Fields
- temp decimal? - Temperature. Unit Default: Kelvin, Metric: Celsius, Imperial: Fahrenheit.
- pressure int? - Atmospheric pressure (on the sea level, if there is no sea_level or grnd_level data), hPa
- humidity int? - Humidity, %
- temp_min decimal? - Minimum temperature at the moment.
- temp_max decimal? - Maximum temperature at the moment.
- sea_level decimal? - Atmospheric pressure on the sea level, hPa
- grnd_level decimal? - Atmospheric pressure on the ground level, hPa
openweathermap: Minutely
Minute forecast weather data API response
Fields
- dt decimal? - Time of the forecasted data, unix, UTC
- precipitation decimal? - Precipitation volume, mm
openweathermap: Rain
Nature of the rain
Fields
- '3h int? - Rain volume for the last 3 hours
openweathermap: Snow
Snow volume information
Fields
- '3h decimal? - Snow volume for the last 3 hours
openweathermap: Sys
System data
Fields
- 'type int? - Internal parameter
- id int? - Internal parameter
- message decimal? - Internal parameter
- country string? - Country code (GB, JP etc.)
- sunrise int? - Sunrise time, unix, UTC
- sunset int? - Sunset time, unix, UTC
openweathermap: Temp
Temperature data
Fields
- day decimal? - Day temperature.
- min decimal? - Min daily temperature.
- max decimal? - Max daily temperature.
- night decimal? - Night temperature.
- eve decimal? - Evening temperature.
- morn decimal? - Morning temperature
openweathermap: Weather
Weather metadata
Fields
- id int? - Weather condition id
- main string? - Group of weather parameters (Rain, Snow, Extreme etc.)
- description string? - Weather condition within the group
- icon string? - Weather icon id
openweathermap: WeatherForecast
Weather forecast data
Fields
- lat decimal? - Latitude
- lon decimal? - Longtitude
- timezone string? - Timezone name for the requested location
- timezone_offset decimal? - Shift in seconds from UTC
- current ForecastCurrent? - Current weather data API response
- minutely Minutely[]? - Minutely weather forecast
- hourly Hourly[]? - Hourly weather forecast
- daily Daily[]? - Daily weather forecast
- alerts Alerts[]? - Government weather alerts
openweathermap: Wind
Nature of the wind
Fields
- speed decimal? - Wind speed. Unit Default: meter/sec, Metric: meter/sec, Imperial: miles/hour.
- deg int? - Wind direction, degrees (meteorological)
Import
import ballerinax/openweathermap;
Metadata
Released date: over 3 years ago
Version: 1.1.0
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: slbeta6
GraalVM compatible: Yes
Pull count
Total: 5180
Current verison: 105
Weekly downloads
Keywords
Lifestyle & Entertainment/News & Lifestyle
Cost/Freemium
Contributors
Dependencies