openair
Module openair
API
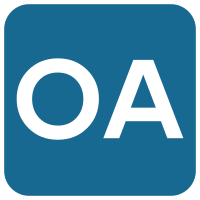
ballerinax/openair Ballerina library
Overview
This is a generated connector for OpenAir API v1.0 OpenAPI specification. The OpenAir REST API provides an interface for integration applications to exchange information with OpenAir. OpenAir REST API currently supports the following resources: Expense reports, Receipts, Attachments, Job codes, Contacts
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a OpenAir account.
- Obtain token by following this guide.
Quickstart
To use the OpenAir connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/openair
module into the Ballerina project.
import ballerinax/openair;
Step 2: Create a new connector instance
Create a openair:ClientConfig
with bearer token obtained, then initialize the connector with it and the service URL according to the OpenAir
REST API guide.
configurable http:BearerTokenConfig & readonly authConfig = ?; openair:ClientConfig clientConfig = {auth : authConfig}; openair:Client baseClient = check new Client(clientConfig, serviceUrl = "<Service URL>");
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example to get a list of contacts using the connector.
public function main() { openair:OpenAirResponse|error response = baseClient->getContacts(); if (response is openair:OpenAirResponse) { log:printInfo(response.toString()); } else { log:printError(response.message()); } }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
openair: Client
This is a generated connector for OpenAir API v1.0 OpenAPI specification. The OpenAir REST API provides an interface for integration applications to exchange information with OpenAir. OpenAir REST API currently supports the following resources: Expense reports, Receipts, Attachments, Job codes, Contacts
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a OpenAir account and obtain tokens by following this guide.
init (ClientConfig clientConfig, string serviceUrl)
- clientConfig ClientConfig - The configurations to be used when initializing the
connector
- serviceUrl string - URL of the target service
getContacts
function getContacts() returns OpenAirResponse|error
Retrieves the list of contacts.
Return Type
- OpenAirResponse|error - An OpenAir type record or an error
addContact
function addContact(Contact payload, string? fields, boolean? returnObject) returns OpenAirResponse|error
Creates a new contact record
Parameters
- payload Contact - A contact is a person working for, or associated with a customer
- fields string? (default ()) - A comma-separated list of attributes to include in the response. If not specified, the response includes all attributes for the contact returned
- returnObject boolean? (default ()) - If set to 1, the response will return the contact created. Otherwise, the response will include only the internal ID of the contact created
Return Type
- OpenAirResponse|error - An OpenAir type record or an error
discoverMethods
Discover the methods available
getContactByID
function getContactByID(int id, string? fields) returns OpenAirResponse|error
Retrieves the contact record with the specified internal ID
Parameters
- id int - The internal id of the contact
- fields string? (default ()) - A comma-separated list of attributes to include in the response. If not specified, the response includes all attributes for the contact returned
Return Type
- OpenAirResponse|error - An OpenAir type record or an error
updateContact
function updateContact(int id, Contact payload, string? fields, boolean? returnObject) returns OpenAirResponse|error
Updates the contact record with the specified internal ID
Parameters
- id int - The internal id of the contact
- payload Contact - If set to any value other than 0 (zero), the response will include the contact updated. Otherwise, the response will include only the internal ID of the contact updated
- fields string? (default ()) - A comma-separated list of attributes to include in the response. If not specified, the response includes all attributes for the contact returned
- returnObject boolean? (default ()) - If set to any value other than 0 (zero), the response will include the contact updated. Otherwise, the response will include only the internal ID of the contact updated
Return Type
- OpenAirResponse|error - An OpenAir type record or an error
deleteContact
function deleteContact(int id) returns OpenAirResponse|error
Deletes the contact record with the specified internal ID
Parameters
- id int - The internal id of the contact
Return Type
- OpenAirResponse|error - An OpenAir type record or an error
getListOfExpenseReports
function getListOfExpenseReports(string? expand, string? fields, int? 'limit, int? offset, string? q) returns OpenAirResponse|error
Get the list of Expense Report
Parameters
- expand string? (default ()) - A comma-separated list of attributes available for expansion. The comma-separated list may include spaces (or %20 in the URL encoded string)
- fields string? (default ()) - A comma-separated list of attributes to include in the response. If not specified, the response includes all attributes for the expense report returned
- 'limit int? (default ()) - A limit on the length of the page
- offset int? (default ()) - A cursor for use in pagination
- q string? (default ()) - A URL-encoded query expression used to filter the resource collection and return the objects matching the specified search criteria
Return Type
- OpenAirResponse|error - An OpenAir type record or an error
getExpenseReport
function getExpenseReport(int id, string? expand, string? fields) returns OpenAirResponse|error
Retrieves the expense report with the specified internal
Parameters
- id int - The internal id of the expense report
- expand string? (default ()) - A comma-separated list of attributes available for expansion. The comma-separated list may include spaces (or %20 in the URL encoded string)
- fields string? (default ()) - A comma-separated list of attributes to include in the response. If not specified, the response includes all attributes for the expense report returned
Return Type
- OpenAirResponse|error - An OpenAir type record or an error
updateExpneseReport
function updateExpneseReport(int id, string fields, boolean returnObject, ExpenseReport payload, string? expand) returns OpenAirResponse|error
Updates an Expense Report
Parameters
- id int - The internal id of the expense report
- fields string - A comma-separated list of attributes to include in the response. If not specified, the response includes all attributes for the expense report returned
- returnObject boolean - If set to any value other than 0 (zero), the response will include the expense report updated. Otherwise, the response will include only the internal ID of the expense report updated
- payload ExpenseReport - An object including valid key-value pairs for the fields to be updated
- expand string? (default ()) - A comma-separated list of attributes available for expansion. The comma-separated list may include spaces (or %20 in the URL encoded string)
Return Type
- OpenAirResponse|error - An OpenAir type record or an error
deleteExpenseReports
function deleteExpenseReports(int id) returns InlineResponse200|error
Deletes e the expense report record with the specified internal ID
Parameters
- id int - The internal id of the contact
Return Type
- InlineResponse200|error - An OpenAir type record or an error with ID and a message
insertOverlappingExpenseReport
function insertOverlappingExpenseReport(ExpenseReport payload, string? expand, string? fields, boolean? returnObject) returns OpenAirResponse|error
Inserts an Overlapping Expense Report
Parameters
- payload ExpenseReport - An expense report is a collection of expense items (receipts) that employees can use in OpenAir to claim reimbursement
- expand string? (default ()) - A comma-separated list of attributes available for expansion. The comma-separated list may include spaces (or %20 in the URL encoded string)
- fields string? (default ()) - A comma-separated list of attributes to include in the response. If not specified, the response includes all attributes for the expense report returned
- returnObject boolean? (default ()) - If set to any value other than 0 (zero), the response will return the expense report created. Otherwise, the response will include only the internal ID of the expense report created
Return Type
- OpenAirResponse|error - An OpenAir type record or an error
getListOfReceiptsInExpenseReport
function getListOfReceiptsInExpenseReport(int id, string? expand, string? fields, int? 'limit, int? offset, string? q) returns OpenAirResponse|error
retrieve the collection of receipts in the expense report with the specified internal ID
Parameters
- id int - The internal id of the expense report
- expand string? (default ()) - A comma-separated list of attributes available for expansion. The comma-separated list may include spaces (or %20 in the URL encoded string)
- fields string? (default ()) - A comma-separated list of attributes to include in the response. If not specified, the response includes all attributes for the expense report returned
- 'limit int? (default ()) - A limit on the length of the page
- offset int? (default ()) - A cursor for use in pagination
- q string? (default ()) - A URL-encoded query expression used to filter the resource collection and return the objects matching the specified search criteria
Return Type
- OpenAirResponse|error - An OpenAir type record or an error
getReceiptForExpenseReport
function getReceiptForExpenseReport(int id, int ticketId, string? expand, string? fields) returns OpenAirResponse|error
Gets a Receipt associated with an Expense Report
Parameters
- id int - The internal id of the expense report
- ticketId int - The internal id of the receipt
- expand string? (default ()) - A comma-separated list of attributes available for expansion. The comma-separated list may include spaces (or %20 in the URL encoded string)
- fields string? (default ()) - A comma-separated list of attributes to include in the response. If not specified, the response includes all attributes for the expense report returned
Return Type
- OpenAirResponse|error - An OpenAir type record or an error
getAttachmentListWithExpenseReport
function getAttachmentListWithExpenseReport(int id, string? expand, string? fields, int? 'limit, int? offset, string? q) returns OpenAirResponse|error
Get the List of Attachments Associated with an Expense Report
Parameters
- id int - The internal id of the expense report
- expand string? (default ()) - A comma-separated list of attributes available for expansion. The comma-separated list may include spaces (or %20 in the URL encoded string)
- fields string? (default ()) - A comma-separated list of attributes to include in the response. If not specified, the response includes all attributes for the expense report returned
- 'limit int? (default ()) - A limit on the length of the page
- offset int? (default ()) - A cursor for use in pagination
- q string? (default ()) - A URL-encoded query expression used to filter the resource collection and return the objects matching the specified search criteria
Return Type
- OpenAirResponse|error - An OpenAir type record or an error
addAttachmentToExpenseReport
function addAttachmentToExpenseReport(int id, AttachmentTypeJson payload, string? expand, string? fields) returns OpenAirResponse|error
Add an Attachment to an Expense Report
Parameters
- id int - The internal id of the expense report
- payload AttachmentTypeJson - This method accepts application/json content types
- expand string? (default ()) - A comma-separated list of attributes available for expansion. The comma-separated list may include spaces (or %20 in the URL encoded string)
- fields string? (default ()) - A comma-separated list of attributes to include in the response. If not specified, the response includes all attributes for the expense report returned
Return Type
- OpenAirResponse|error - An OpenAir type record or an error
getAttachmentByIDWithExpenseReport
function getAttachmentByIDWithExpenseReport(int id, int attachmentId, string? expand, string? fields) returns OpenAirResponse|error
Retrieves the attachment record with the specified attachment ID associated with the expense report with the specified internal ID
Parameters
- id int - The internal id of the expense report
- attachmentId int - The internal id of the attachment
- expand string? (default ()) - A comma-separated list of attributes available for expansion. The comma-separated list may include spaces (or %20 in the URL encoded string)
- fields string? (default ()) - A comma-separated list of attributes to include in the response. If not specified, the response includes all attributes for the expense report returned
Return Type
- OpenAirResponse|error - An OpenAir type record or an error
deleteAttachmentOfExpenseReport
function deleteAttachmentOfExpenseReport(int id, int attachmentId) returns InlineResponse2001|error
delete the attachment with the specified attachment ID associated with the expense report with the specified internal ID, or clear the association between the workspace document with the specified attachment ID and the expense report with the specified internal ID
Parameters
- id int - The internal id of the expense report
- attachmentId int - The internal id of the attachment
Return Type
- InlineResponse2001|error - A successful or failed request returns a JSON object
getJobs
function getJobs() returns OpenAirResponse|error
Retrieves the list of job codes
Return Type
- OpenAirResponse|error - An OpenAir type record or an error
addJob
function addJob(JobCode payload, string? fields, boolean? returnObject) returns OpenAirResponse|error
Creates a new job code record
Parameters
- payload JobCode - The JobCode object to be created
- fields string? (default ()) - A comma-separated list of attributes to include in the response. If not specified, the response includes all attributes for the expense report returned
- returnObject boolean? (default ()) - If set to any value other than 0 (zero), the response will return the job code created. Otherwise, the response will include only the internal ID of the job code created
Return Type
- OpenAirResponse|error - An OpenAir type record or an error
getJobById
function getJobById(int id, string? fields) returns OpenAirResponse|error
Retrieves the job code record with the specified internal ID
Parameters
- id int - The internal id of the contact
- fields string? (default ()) - A comma-separated list of attributes to include in the response. If not specified, the response includes all attributes for the job code returned
Return Type
- OpenAirResponse|error - An OpenAir type record or an error
updateJobCodeById
function updateJobCodeById(int id, JobCode payload, string? fields, boolean? returnObject) returns OpenAirResponse|error
Update the job code record with the specified internal ID
Parameters
- id int - The internal id of the contact
- payload JobCode - An object including valid key-value pairs for the fields to be updated
- fields string? (default ()) - A comma-separated list of attributes to include in the response. If not specified, the response includes all attributes for the job code returned
- returnObject boolean? (default ()) - If set to any value other than 0 (zero), the response will include the job code updated. Otherwise, the response will include only the internal ID of the job code updated.
Return Type
- OpenAirResponse|error - An OpenAir type record or an error
deleteJob
function deleteJob(int id) returns OpenAirResponse|error
Deletes the job code record with the specified internal ID
Parameters
- id int - The internal id of the job code
Return Type
- OpenAirResponse|error - An OpenAir type record or an error
getReceipts
function getReceipts(string? expand, string? fields, int? 'limit, int? offset, string? q) returns OpenAirResponse|error
Retrieves a list of receipts
Parameters
- expand string? (default ()) - A comma-separated list of attributes available for expansion. The comma-separated list may include spaces (or %20 in the URL encoded string)
- fields string? (default ()) - A comma-separated list of attributes to include in the response. If not specified, the response includes all attributes for the expense report returned
- 'limit int? (default ()) - A limit on the length of the page
- offset int? (default ()) - A cursor for use in pagination
- q string? (default ()) - A URL-encoded query expression used to filter the resource collection and return the objects matching the specified search criteria
Return Type
- OpenAirResponse|error - An OpenAir type record or an error
insertReceipt
function insertReceipt(Receipts payload, string? expand, string? fields, boolean? returnObject) returns OpenAirResponse|error
Create a new receipt
Parameters
- payload Receipts - An expense report is a collection of expense items (receipts) that employees can use in OpenAir to claim reimbursement
- expand string? (default ()) - A comma-separated list of attributes available for expansion. The comma-separated list may include spaces (or %20 in the URL encoded string)
- fields string? (default ()) - A comma-separated list of attributes to include in the response. If not specified, the response includes all attributes for the expense report returned
- returnObject boolean? (default ()) - If set to any value other than 0 (zero), the response will return the expense report created. Otherwise, the response will include only the internal ID of the expense report created
Return Type
- OpenAirResponse|error - An OpenAir type record or an error with ID and a message
getReceiptById
function getReceiptById(int id, string fields, string? expand) returns OpenAirResponse|error
Retrieves a receipt with the specified internal ID.
Parameters
- id int - The internal id of the receipt
- fields string - A comma-separated list of attributes to include in the response. If not specified, the response includes all attributes for the expense report returned
- expand string? (default ()) - A comma-separated list of attributes available for expansion. The comma-separated list may include spaces (or %20 in the URL encoded string)
Return Type
- OpenAirResponse|error - An OpenAir type record or an error
updateReceipt
function updateReceipt(int id, Receipts payload, string? expand, string? fields, boolean? returnObject) returns OpenAirResponse|error
Updates the receipt with the specified internal ID
Parameters
- id int - The internal id of the receipt
- payload Receipts - An object including valid key-value pairs for the fields to be updated
- expand string? (default ()) - A comma-separated list of attributes available for expansion. The comma-separated list may include spaces (or %20 in the URL encoded string)
- fields string? (default ()) - A comma-separated list of attributes to include in the response. If not specified, the response includes all attributes for the job code returned
- returnObject boolean? (default ()) - If set to any value other than 0 (zero), the response will include the job code updated. Otherwise, the response will include only the internal ID of the job code updated.
Return Type
- OpenAirResponse|error - An OpenAir type record or an error
getListOfAttachmentsOfReceipt
function getListOfAttachmentsOfReceipt(int id, string? expand, string? fields, int? 'limit, int? offset, string? q) returns OpenAirResponse|error
Gets the List of Attachments Associated with a Receipt
Parameters
- id int - The internal id of the expense receipts
- expand string? (default ()) - A comma-separated list of attributes available for expansion. The comma-separated list may include spaces (or %20 in the URL encoded string)
- fields string? (default ()) - A comma-separated list of attributes to include in the response. If not specified, the response includes all attributes for the expense report returned
- 'limit int? (default ()) - A limit on the length of the page
- offset int? (default ()) - A cursor for use in pagination
- q string? (default ()) - A URL-encoded query expression used to filter the resource collection and return the objects matching the specified search criteria
Return Type
- OpenAirResponse|error - An OpenAir type record or an error
addAttachmentReceipt
function addAttachmentReceipt(int id, AttachmentTypeJson payload, string? expand, string? fields, boolean? returnObject) returns OpenAirResponse|error
Add an attachment to the receipt with the specified internal ID
Parameters
- id int - The internal id of the receipt
- payload AttachmentTypeJson - This method accepts application/json content types
- expand string? (default ()) - A comma-separated list of attributes available for expansion. The comma-separated list may include spaces (or %20 in the URL encoded string)
- fields string? (default ()) - A comma-separated list of attributes to include in the response. If not specified, the response includes all attributes for the job code returned
- returnObject boolean? (default ()) - If set to any value other than 0 (zero), the response will include the job code updated. Otherwise, the response will include only the internal ID of the job code updated.
Return Type
- OpenAirResponse|error - An OpenAir type record or an error
getAttachmentOfReceipt
function getAttachmentOfReceipt(int id, int attachmentId, string? expand, string? fields) returns OpenAirResponse|error
Gets an Attachment Associated with a Receipt
Parameters
- id int - The internal id of the expense receipts
- attachmentId int - The internal id of the attachment
- expand string? (default ()) - A comma-separated list of attributes available for expansion. The comma-separated list may include spaces (or %20 in the URL encoded string)
- fields string? (default ()) - A comma-separated list of attributes to include in the response. If not specified, the response includes all attributes for the expense report returned
Return Type
- OpenAirResponse|error - An OpenAir type record or an error
deleteAttachmentOfReceipt
function deleteAttachmentOfReceipt(int id, int attachmentId) returns OpenAirResponse|error
Deletes an Attachment Associated with a Receipt
Parameters
- id int - The internal id of the expense receipts
- attachmentId int - The internal id of the attachment
Return Type
- OpenAirResponse|error - An OpenAir type record or an error
Records
openair: Attachment
Attachments are files uploaded and stored in OpenAir and associated with records
Fields
- attachmentCategoryId int? - The internal ID of the attachment category
- created string? - The date the attachment was created
- fileName string? - The filename of the attached file
- fileType string? - The file type. This is used for tracking purposes
- id int? - The unique internal identifier of the attachment
- isExcludedFromAlert boolean? - A 1/0 field indicating if the attachment is excluded from the alert system
- isFolder boolean? - A 1/0 field indicating if other attachment records have this attachment record as a parent
- isLocked boolean? - A 1/0 field indicating if the attachment is locked for editing
- lockedBy int? - The internal ID of the employee who uploaded the file. The value is 0 (zero) if unlocked.
- name string? - The display name of the attachment
- notes string? - Notes about the attachment. This attribute is used for keyword search.
- size decimal? - The file size in bytes
- updated string? - The date the attachment was last updated or modified
- uploadedBy int? - The internal ID of the employee who uploaded the file.
- workspaceId int? - The internal ID of the workspace associated with the attachment. The value is 0 (zero) if the attachment is not associated with a workspace.
openair: AttachmentTypeJson
Fields
- id int? - The internal ID of the workspace document to be associated with the expense report.
openair: ClientConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig - Configurations related to client authentication
- httpVersion string(default "1.1") - The HTTP version understood by the client
- http1Settings ClientHttp1Settings(default {}) - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings(default {}) - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- followRedirects FollowRedirects?(default ()) - Configurations associated with Redirection
- poolConfig PoolConfiguration?(default ()) - Configurations associated with request pooling
- cache CacheConfig(default {}) - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig?(default ()) - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig?(default ()) - Configurations associated with retrying
- cookieConfig CookieConfig?(default ()) - Configurations associated with cookies
- responseLimits ResponseLimitConfigs(default {}) - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket?(default ()) - SSL/TLS-related options
openair: Contact
Contacts details
Fields
- accountingCode int? - Optional accounting code that can be used for integration with external accounting systems.
- address1 string? - First line of the contact's address
- address2 string? - Second line of the contact's address
- address3 string? - Third line of the contact's address
- address4 string? - Forth line of the contact's address
- canBill boolean? - A 1/0 field indicating if the contact can be used for billing
- canSell boolean? - A 1/0 field indicating if the contact an be used for selling
- canShip boolean? - A 1/0 field indicating if the contact can be used for shipping
- city string? - The contact's city
- country string? - The contact's country
- created string? - The date the contact record was created
- customerId int? - The internal ID of the associated customer
- email string? - The contact's Email address
- externalId string? - The unique external ID of the contact, if the record was imported from an external system
- fax string? - The contact's fax number
- firstName string? - The contact's first name
- id int? - The unique internal identifier of the contact
- isActive boolean? - A 1/0 field indicating if the contact is active
- jobTitle string? - The contact's job title
- lastName string? - The contact's last name
- mobile string? - The contact's mobile phone number
- nickname string? - The nickname used to identify the contact
- notes string? - Notes about the contact
- phone string? - The contact's phone number
- state string? - The contact's state
- title string? - The contact's title
- updated string? - The date the contact record was last updated or modified
- zip string? - The contact's ZIP code or postal code
openair: ExpenseReport
collections of expense items (receipts) that employees can use in OpenAir to claim reimbursement
Fields
- accountingDate string? - The accounting period date of the expense report
- advance string? - The amount of any cash advance on the expense report
- approveDate string? - The date the expense report was approved
- attachments IDs[]? - The attachments associated with this expense report. Array of internal IDs for attachment objects
- balance decimal? - The outstanding balance on the expense report
- created string? - The date the expense report was created
- currency string? - The currency for monetary values in the expense report. Three-letter currency code
- customerId int? - The internal ID of the associated customer
- date string? - The date of the expense report
- description string? - The description of the expense report (e.g. reason for the trip)
- endDate string? - The ending date of the expense report (only used with auto-naming)
- exported string? - The date and time the record was marked as "exported"
- externalId string? - The unique external ID of the expense report, if the record was imported from an external "system"
- id int? - The unique internal identifier of the expense report
- isAccounting boolean? - A 1/0 field indicating if an envelope was submitted to an accounting partner
- isAdjusting boolean? - A 1/0 field indicating if the expense report is an adjusting expense report
- isForeignCurrencyExchangeIntolerance boolean? - A 1/0 field indicating if the record is within the specified foreign currency tolerance as defined in database data definitions
- name string? - A 1/0 field indicating if the record is within the specified foreign currency tolerance as defined in database data definitions
- notes string? - Notes about the expense report
- projectId int? - The internal ID of the associated project
- startDate string? - The starting date of the expense report (only used with auto-naming)
- status string? - The status of the expense report. Possible values: O — open, S — submitted, A — approved, R — rejected
- submitDate string? - The date the expense report was submitted
- taxLocationId int? - Default tax location for this expense report
- total decimal? - The total value of all the receipts in the expense report
- totalReceipts int? - The total number of receipts in the expense report
- totalReimburse decimal? - The total amount of reimbursable expenses in the expense report
- trackingNumber string? - The expense report tracking number
- updated string? - The date the expense report was last updated or modified
- userId int? - The internal ID of the associated employee
openair: IDs
IDs of the objects
Fields
- id string? - ID of the object
openair: InlineResponse200
Fields
- message string? - A string containing a brief message about the status of your request
openair: InlineResponse2001
Fields
- message string? - A string containing a brief message about the status of your request — e.g. "Success" or "Attachment #4985 not found"
openair: JobCode
Job Code
Fields
- accountingCode string? - Optional accounting code that can be used for integration with external accounting systems
- created string? - The date the job code was created
- currency string? - The currency for monetary values in the job code record. Three-letter currency code
- externalId string? - The unique external record ID, if the record was imported from an external system
- genericResourceId int? - The internal ID of the generic resource used in full-time equivalent (FTE) forecasting
- id int? - The unique internal identifier of the job code
- isActive boolean? - A 1/0 field indicating if the job code is active
- loadedCost decimal? - The cost per hour
- name string? - The display name of the job code
- notes boolean? - Notes about the job code
- updated string? - The date the job code was last updated or modified
openair: OpenAirResponse
An OpenAir type record or an error
Fields
- data (IDs|ExpenseReport|Attachment|JobCode)[]? - An array containing the relevant objects requested or an IDs
- included record {}? - An array of expanded objects, if the expand parameter was set in the request
- meta record {}? - An object containing information about objects referenced by internal ID in the data array (object type and internal ID). Only returned if the return_object parameter was set to any value other than 0 (zero) in the request
- message string? - A string containing a brief message about the status of your request
openair: Receipts
Fields
- accountingDate string? - The accounting period date of the receipt
- attachments IDs[]? - The attachments associated with the receipt. Array of internal IDs for attachment objects.
- costCenterId int? - The internal ID of the cost center associated with the receipt
- costPerUnit decimal? - The cost per unit of measure
- created string? - The date the receipt was created
- currency string? - The currency for monetary values in the receipt record. Three-letter currency code
- customerId int? - The internal ID of the customer associated with the receipt
- date string? - The date of the receipt
- description string? - The description of the receipt
- expenseReportId int? - The internal ID of the expense report associated with the receipt
- externalId string? - The unique external ID of the receipt, if the record was imported from an external system
- federalTax decimal? - The total federal tax for the receipt
- federalTaxRate decimal? - The federal tax rate for the receipt
- foreignCurrencyCost decimal? - The cost per unit of measure in the selected foreign currency, if this is a foreign currency receipt
- foreignCurrencyRate decimal? - The cost per unit of measure in the selected foreign currency, if this is a foreign currency receipt.
- foreignCurrencySymbol string? - The foreign currency, if this is a foreign currency receipt. Three-letter currency code
- foreignCurrencyTotalTaxPaid decimal? - The tax paid in the foreign currency, if this is a foreign currency receipt
- gst decimal? - The total GST tax for the receipt
- gstRate decimal? - The total GST tax for the receipt
- hst decimal? - The total GST tax for the receipt
- hstRate decimal? - The total GST tax for the receipt
- id int? - The total GST tax for the receipt
- isForeignCurrencyExchangeIntolerance boolean? - A 1/0 field indicating if the record is within the specified foreign currency tolerance
- isMissingPaperReceipt boolean? - A 1/0 field indicating if the paper receipt is missing for this receipt
- isNonBillable boolean? - A 1/0 field indicating if the receipt is not billable
- isReimbursable boolean? - A 1/0 field indicating if the receipt is reimbursable
- isTaxIncludedInCost boolean? - A 1/0 field indicating if the cost includes the tax
- itemId int? - The internal ID of the item associated with the receipt. The type of item can be used to determine the receipt subtype
- notes string? - Notes about the receipt
- paymentTypeId int? - The internal ID of the payment type associated with the receipt. The payment type indicates how the payment was made and may determine if the receipt is reimbursable
- projectId int? - The internal ID of the project associated with the receipt
- projectTaskId int? - The internal ID of the project task associated with the receipt. Requires an option to be enabled in OpenAir (Administration > Application settings > Expenses > Other settings)
- pst decimal? - The total PST tax for the receipt
- pstRate decimal? - The total PST tax for the receipt
- receiptLocation string? - The city or location where the cost was incurred
- slipId int? - The internal ID of the slip associated with the receipt, if the expense was billed
- stateTax decimal? - The total state tax for the receipt
- quantity int? - The quantity (number of units of measure)
- taxLocationId int? - The internal ID of the tax location associated with the receipt
- total decimal? - The internal ID of the tax location associated with the receipt
- totalNoTax decimal? - The total value of the receipt excluding tax
- totalTaxPaid decimal? - The total tax paid
- trackingNumber string? - The unique reference number of the receipt within the associated expense report. This attribute is used to cross reference digital receipts with paper receipts
- updated string? - The date the receipt was last updated or modified
- userId int? - The internal ID of the employee associated with the receipt
- userLocationId int? - The internal ID of the employee location associated with the receipt
- vehicleId int? - The internal ID of the vehicle associated with the receipt
- vendorId int? - The internal ID of the vendor associated with the receipt.
Import
import ballerinax/openair;
Metadata
Released date: over 3 years ago
Version: 1.1.0
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: slbeta6
GraalVM compatible: Yes
Pull count
Total: 7
Current verison: 0
Weekly downloads
Keywords
Productivity/Project Management
Cost/Paid
Contributors
Dependencies