Module ocpi
API
Definitions
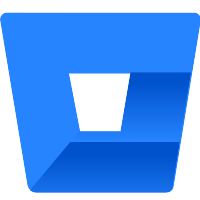
ballerinax/ocpi Ballerina library
Overview
This is a generated connector for Open Charge Point Interface(OCPI) v2.2 OpenAPI specification.
The Open Charge Point Interface (OCPI) enables a scalable, automated EV roaming setup between Charge Point Operators and e-Mobility Service Providers. It supports authorization, charge point information exchange (including live status updates and transaction events), charge detail record exchange, remote charge point commands and the exchange of smart-charging related information between parties.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Obtain your Open Charge Network(OCN) node endpoint URL that is based on OCPI v2.2 specification.
- Obtain tokens
- Follow this link to get OCPI specification detail for authorization and obtain tokens according to your OCN.
Quickstart
To Learn more on OCN, Follow this link or you can refer this link to learn how to test a local OCN. To use the OCPI connector in your Ballerina application, update the .bal file as follows: Follow the following steps to create a simple sample.
Step 1 - Import connector
import ballerinax/ocpi;
Step 2 - Create a new connector instance
ocpi:Client baseClient = check new ocpi:Client(serviceUrl = "<OCN node URL>");
Step 3 - Invoke connector operation
- Get health status of the OCN node
string response = check baseClient->getHealth();
- Get the ID of the node, Follow this link for more details.
json response = check baseClient->getMyNodeInfo();
Note: To Add a party to the OCN registry, Use below command if you test the connector using your locally configured OCN Follow this link for more information
//This should be done using the command line interface // Adding the party to the OCN registry // Execute the following in the command line // // ocn-registry set-party --credentials DE MSP \ // --roles EMSP \ // --operator <Operator ID> \ // --signer <Signer token>
string authorization = string `Token ${admin_API_key}`; ocpi:BasicRole[] payload = [ { country_code: "<Country code>", party_id: "<Party ID>" } ]; json response = check baseClient->generateRegistrationToken(authorization, payload);
string credentials_tokenA = "<Provide_your_credentials_token_A>"; string authorization = string `Token ${credentials_tokenA}`; cpi:OcpiResponseListVersion response = check baseClient->getVersions(authorization);
string credentials_tokenA = "<Provide_your_credentials_token_A>"; string authorization = string `Token ${credentials_tokenA}`; ocpi:OcpiResponseVersionDetail response = check baseClient->getVersionsDetail(authorization);
string credentials_tokenA = "<Provide_your_credentials_token_A>"; string authorization = string `Token ${credentials_tokenA}`; ocpi:Credentials credentials = { token: "<Token>", url: "<URL>", roles: [ { country_code: "<Country Code>", party_id: "<Party ID>", role: "<Role>", business_details: { name: "<Name>" } } ] }; ocpi:OcpiResponseCredentials response = check baseClient->postCredentials(authorization, credentials);
This Completes the registration to the OCN Node, Now you are ready to send requests to other parties on the network
string credentials_tokenC = "<Provide_your_credentials_token_C>"; string authorization = string `Token ${credentials_tokenC}`; string xRequestId = "1"; string xCorrelationId = "1"; string ocpiFromCountryCode = "<From Country Code>"; string ocpiFromPartyId = "<From Party ID>"; string ocpiToCountryCode = "<To Country Code>"; string ocpiToPartyId = "<To Party ID>"; ocpi:OcpiResponseLocationList response = check baseClient->getLocationListFromDataOwner( authorization, xRequestId, xCorrelationId, ocpiFromCountryCode, ocpiFromPartyId, ocpiToCountryCode, ocpiToPartyId);
- Get an array of tariffs provided by the CPO, with IDs matching those found on the Connector object.
string credentials_tokenC = "<Provide_your_credentials_token_C>"; string authorization = string `Token ${credentials_tokenC}`; string xRequestId = "1"; string xCorrelationId = "1"; string ocpiFromCountryCode = "<From Country Code>"; string ocpiFromPartyId = "<From Party ID>"; string ocpiToCountryCode = "<To Country Code>"; string ocpiToPartyId = "<To Party ID>"; ocpi:OcpiResponseTariffList response = check baseClient->getTariffsFromDataOwner(authorization, xRequestId, xCorrelationId, ocpiFromCountryCode, ocpiFromPartyId, ocpiToCountryCode, ocpiToPartyId);
- Tell the CPO a driver on the eMSP system wishes to start a session remotely.
string credentials_tokenC = "<Provide_your_credentials_token_C>"; string authorization = string `Token ${credentials_tokenC}`; string xRequestId = "1"; string xCorrelationId = "1"; string ocpiFromCountryCode = "<From Country Code>"; string ocpiFromPartyId = "<From Party ID>"; string ocpiToCountryCode = "<To Country Code>"; string ocpiToPartyId = "<To Party ID>"; ocpi:StartSession startSession = { response_url: "<Response URL>", token: { country_code: "<Country Code>", party_id: "<Party ID>", uid: "<UID>", 'type: "APP_USER", contract_id: "<Contract ID>", issuer: "<Issuer>", valid: true, whitelist: "ALWAYS", last_updated: "<Last Update date/time>" }, location_id: "<Location ID>" }; ocpi:OcpiResponseCommandResponse|error response = baseClient->postStartSession(authorization, xRequestId, xCorrelationId, ocpiFromCountryCode, ocpiFromPartyId, ocpiToCountryCode, ocpiToPartyId, startSession)
- Tell the CPO a driver on the eMSP system wishes to stop a session remotely. For more information, Follow this link
string credentials_tokenC = "<Provide_your_credentials_token_C>"; string authorization = string `Token ${credentials_tokenC}`; string xRequestId = "1"; string xCorrelationId = "1"; string ocpiFromCountryCode = "<From Country Code>"; string ocpiFromPartyId = "<From Party ID>"; string ocpiToCountryCode = "<To Country Code>"; string ocpiToPartyId = "<To Party ID>"; ocpi:StopSession stopSession = { "response_url": "<Response URL>", "session_id": "<Session ID>" }; ocpi:OcpiResponseCommandResponse response = check baseClient->postStopSession(authorization, xRequestId, xCorrelationId, ocpiFromCountryCode, ocpiFromPartyId, ocpiToCountryCode, ocpiToPartyId, stopSession);
Use bal run
command to compile and run the Ballerina program.
Clients
ocpi: Client
This is a generated connector for OCPI v2.2 OpenAPI specification. The Open Charge Point Interface (OCPI) enables a scalable, automated EV roaming setup between Charge Point Operators and e-Mobility Service Providers. It supports authorization, charge point information exchange (including live status updates and transaction events), charge detail record exchange, remote charge point commands and the exchange of smart-charging related information between parties.
Constructor
Gets invoked to initialize the connector
.
The connector initialization doesn't require setting the API credentials.
Follow the section OCPI v2.2 - 4. Transport and format section and obtain tokens according to your Open Charge Point network. Some operations may require setting the token as a parameter.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig {} - The configurations to be used when initializing the
connector
- serviceUrl string "http://localhost:8080" - URL of the target service
getConnectionStatus
function getConnectionStatus(string authorization, string countryCode, string partyID) returns string|error
Gets connection status
Parameters
- authorization string - Authorization token
- countryCode string - Country code
- partyID string - Party ID
generateRegistrationToken
Generates registration tokens
Return Type
- byte[]|error - OK
getHealth
Gets health of the OCN
postMessage
function postMessage(string xRequestId, string payload, string? ocnSignature) returns OcpiResponseObject|error
Communicates between CPO and eMSP
Parameters
- xRequestId string - Every request shall contain a unique request ID, the response to this request shall contain the same ID
- payload string - Request body
- ocnSignature string? (default ()) - OCN Signature
Return Type
- OcpiResponseObject|error - OK
getMyNodeInfo
function getMyNodeInfo() returns byte[]|error
Gets registry information
Return Type
- byte[]|error - OK
getNodeOf
Gets registry details of Providers and Operators by country code and party ID
Return Type
- byte[]|error - OK
getVersionsDetail
function getVersionsDetail(string authorization) returns OcpiResponseVersionDetail|error
Fetches information about the supported endpoints for this version
Parameters
- authorization string - Authorization token
Return Type
getVersions
function getVersions(string authorization) returns OcpiResponseListVersion|error
Fetches information about the supported versions.
Parameters
- authorization string - Authorization token
Return Type
getCdrsFromDataOwner
function getCdrsFromDataOwner(string authorization, string xRequestId, string xCorrelationId, string ocpiFromCountryCode, string ocpiFromPartyId, string ocpiToCountryCode, string ocpiToPartyId, string? ocnSignature, string? dateFrom, string? dateTo, int? offset, int? 'limit) returns OcpiResponseCDRList|error
Fetches charge detail records last updated (which in the current version of OCPI can only be the creation Date/Time) between the {date_from} and {date_to} (paginated).
Parameters
- authorization string - Authorization token
- xRequestId string - Every request shall contain a unique request ID, the response to this request shall contain the same ID
- xCorrelationId string - Every request/response shall contain a unique correlation ID, every response to this request shall contain the same ID
- ocpiFromCountryCode string - Country code of the connected party this message is sent from
- ocpiFromPartyId string - Party ID of the connected party this message is sent from
- ocpiToCountryCode string - Country code of the connected party this message is to be sent to
- ocpiToPartyId string - Party ID of the connected party this message is to be sent to
- ocnSignature string? (default ()) - OCN Signature
- dateFrom string? (default ()) - Only return CDRs that have last_updated after or equal to this Date/Time (inclusive)
- dateTo string? (default ()) - Only return CDRs that have last_updated up to this Date/Time, but not including (exclusive)
- offset int? (default ()) - The offset of the first object returned. Default is 0
- 'limit int? (default ()) - Maximum number of objects to GET
Return Type
- OcpiResponseCDRList|error - OK
getCdrPageFromDataOwner
function getCdrPageFromDataOwner(string authorization, string xRequestId, string xCorrelationId, string ocpiFromCountryCode, string ocpiFromPartyId, string ocpiToCountryCode, string ocpiToPartyId, string uid, string? ocnSignature) returns OcpiResponseCDRList|error
Gets CDR detail by ID from sender
Parameters
- authorization string - Authorization token
- xRequestId string - Every request shall contain a unique request ID, the response to this request shall contain the same ID
- xCorrelationId string - Every request/response shall contain a unique correlation ID, every response to this request shall contain the same ID
- ocpiFromCountryCode string - Country code of the connected party this message is sent from
- ocpiFromPartyId string - Party ID of the connected party this message is sent from
- ocpiToCountryCode string - Country code of the connected party this message is to be sent to
- ocpiToPartyId string - Party ID of the connected party this message is to be sent to
- uid string - UID
- ocnSignature string? (default ()) - OCN Signature
Return Type
- OcpiResponseCDRList|error - OK
getClientOwnedCdr
function getClientOwnedCdr(string authorization, string xRequestId, string xCorrelationId, string ocpiFromCountryCode, string ocpiFromPartyId, string ocpiToCountryCode, string ocpiToPartyId, string cdrID, string? ocnSignature) returns OcpiResponseCDR|error
Gets CDRs by ID from the receiver
Parameters
- authorization string - Authorization token
- xRequestId string - Every request shall contain a unique request ID, the response to this request shall contain the same ID
- xCorrelationId string - Every request/response shall contain a unique correlation ID, every response to this request shall contain the same ID
- ocpiFromCountryCode string - Country code of the connected party this message is sent from
- ocpiFromPartyId string - Party ID of the connected party this message is sent from
- ocpiToCountryCode string - Country code of the connected party this message is to be sent to
- ocpiToPartyId string - Party ID of the connected party this message is to be sent to
- cdrID string - Charge Detail Record ID
- ocnSignature string? (default ()) - OCN Signature
Return Type
- OcpiResponseCDR|error - OK
postClientOwnedCdr
function postClientOwnedCdr(string authorization, string xRequestId, string xCorrelationId, string ocpiFromCountryCode, string ocpiFromPartyId, string ocpiToCountryCode, string ocpiToPartyId, CDR payload, string? ocnSignature) returns OcpiResponseUnit|error
Creates a new CDR
Parameters
- authorization string - Authorization token
- xRequestId string - Every request shall contain a unique request ID, the response to this request shall contain the same ID
- xCorrelationId string - Every request/response shall contain a unique correlation ID, every response to this request shall contain the same ID
- ocpiFromCountryCode string - Country code of the connected party this message is sent from
- ocpiFromPartyId string - Party ID of the connected party this message is sent from
- ocpiToCountryCode string - Country code of the connected party this message is to be sent to
- ocpiToPartyId string - Party ID of the connected party this message is to be sent to
- payload CDR - CDR Details
- ocnSignature string? (default ()) - OCN Signature
Return Type
- OcpiResponseUnit|error - OK
postGenericChargingProfileResult
function postGenericChargingProfileResult(string authorization, string xRequestId, string xCorrelationId, string ocpiFromCountryCode, string ocpiFromPartyId, string ocpiToCountryCode, string ocpiToPartyId, string uid, GenericChargingProfileResult payload, string? ocnSignature) returns OcpiResponseUnit|error
Creates new charging profile
Parameters
- authorization string - Authorization token
- xRequestId string - Every request shall contain a unique request ID, the response to this request shall contain the same ID
- xCorrelationId string - Every request/response shall contain a unique correlation ID, every response to this request shall contain the same ID
- ocpiFromCountryCode string - Country code of the connected party this message is sent from
- ocpiFromPartyId string - Party ID of the connected party this message is sent from
- ocpiToCountryCode string - Country code of the connected party this message is to be sent to
- ocpiToPartyId string - Party ID of the connected party this message is to be sent to
- uid string - UID
- payload GenericChargingProfileResult - Charging profile details
- ocnSignature string? (default ()) - OCN Signature
Return Type
- OcpiResponseUnit|error - OK
putSenderChargingProfile
function putSenderChargingProfile(string authorization, string xRequestId, string xCorrelationId, string ocpiFromCountryCode, string ocpiFromPartyId, string ocpiToCountryCode, string ocpiToPartyId, string sessionId, ActiveChargingProfile payload, string? ocnSignature) returns OcpiResponseUnit|error
Sends or Updates a ChargingProfile by the Sender
Parameters
- authorization string - Authorization token
- xRequestId string - Every request shall contain a unique request ID, the response to this request shall contain the same ID
- xCorrelationId string - Every request/response shall contain a unique correlation ID, every response to this request shall contain the same ID
- ocpiFromCountryCode string - Country code of the connected party this message is sent from
- ocpiFromPartyId string - Party ID of the connected party this message is sent from
- ocpiToCountryCode string - Country code of the connected party this message is to be sent to
- ocpiToPartyId string - Party ID of the connected party this message is to be sent to
- sessionId string - Session ID
- payload ActiveChargingProfile - Active charging profile details
- ocnSignature string? (default ()) - OCN Signature
Return Type
- OcpiResponseUnit|error - OK
getReceiverChargingProfile
function getReceiverChargingProfile(string authorization, string xRequestId, string xCorrelationId, string ocpiFromCountryCode, string ocpiFromPartyId, string ocpiToCountryCode, string ocpiToPartyId, string sessionId, int duration, string responseUrl, string? ocnSignature) returns OcpiResponseChargingProfileResponse|error
Gets the active ActiveChargingProfile by receiver
Parameters
- authorization string - Authorization token
- xRequestId string - Every request shall contain a unique request ID, the response to this request shall contain the same ID
- xCorrelationId string - Every request/response shall contain a unique correlation ID, every response to this request shall contain the same ID
- ocpiFromCountryCode string - Country code of the connected party this message is sent from
- ocpiFromPartyId string - Party ID of the connected party this message is sent from
- ocpiToCountryCode string - Country code of the connected party this message is to be sent to
- ocpiToPartyId string - Party ID of the connected party this message is to be sent to
- sessionId string - Session ID
- duration int - Charging duration
- responseUrl string - URL that the ActiveChargingProfileResult POST should be send to. This URL might contain an unique ID to be able to distinguish between GETActiveChargingProfile requests
- ocnSignature string? (default ()) - OCN Signature
Return Type
putReceiverChargingProfile
function putReceiverChargingProfile(string authorization, string xRequestId, string xCorrelationId, string ocpiFromCountryCode, string ocpiFromPartyId, string ocpiToCountryCode, string ocpiToPartyId, string sessionId, SetChargingProfile payload, string? ocnSignature) returns OcpiResponseChargingProfileResponse|error
Creates/updates a ChargingProfile for a specific charging session
Parameters
- authorization string - Authorization token
- xRequestId string - Every request shall contain a unique request ID, the response to this request shall contain the same ID
- xCorrelationId string - Every request/response shall contain a unique correlation ID, every response to this request shall contain the same ID
- ocpiFromCountryCode string - Country code of the connected party this message is sent from
- ocpiFromPartyId string - Party ID of the connected party this message is sent from
- ocpiToCountryCode string - Country code of the connected party this message is to be sent to
- ocpiToPartyId string - Party ID of the connected party this message is to be sent to
- sessionId string - Session ID
- payload SetChargingProfile - Charging profile details
- ocnSignature string? (default ()) - OCN Signature
Return Type
deleteReceiverChargingProfile
function deleteReceiverChargingProfile(string authorization, string xRequestId, string xCorrelationId, string ocpiFromCountryCode, string ocpiFromPartyId, string ocpiToCountryCode, string ocpiToPartyId, string sessionId, string responseUrl, string? ocnSignature) returns OcpiResponseChargingProfileResponse|error
Cancels an existing ChargingProfile for a specific charging session
Parameters
- authorization string - Authorization token
- xRequestId string - Every request shall contain a unique request ID, the response to this request shall contain the same ID
- xCorrelationId string - Every request/response shall contain a unique correlation ID, every response to this request shall contain the same ID
- ocpiFromCountryCode string - Country code of the connected party this message is sent from
- ocpiFromPartyId string - Party ID of the connected party this message is sent from
- ocpiToCountryCode string - Country code of the connected party this message is to be sent to
- ocpiToPartyId string - Party ID of the connected party this message is to be sent to
- sessionId string - The unique id that identifies the session in the CPO platform
- responseUrl string - URL that the ClearProfileResult POST should be send to. This URL might contain an unique ID to be able to distinguish between DELETE ChargingProfile requests.
- ocnSignature string? (default ()) - OCN Signature
Return Type
postAsyncResponse
function postAsyncResponse(string authorization, string xRequestId, string xCorrelationId, string ocpiFromCountryCode, string ocpiFromPartyId, string ocpiToCountryCode, string ocpiToPartyId, string command, string uid, CommandResult payload, string? ocnSignature) returns OcpiResponseUnit|error
Receive the asynchronous response from the Charge Point.
Parameters
- authorization string - Authorization token
- xRequestId string - Every request shall contain a unique request ID, the response to this request shall contain the same ID
- xCorrelationId string - Every request/response shall contain a unique correlation ID, every response to this request shall contain the same ID
- ocpiFromCountryCode string - Country code of the connected party this message is sent from
- ocpiFromPartyId string - Party ID of the connected party this message is sent from
- ocpiToCountryCode string - Country code of the connected party this message is to be sent to
- ocpiToPartyId string - Party ID of the connected party this message is to be sent to
- command string - Command of the request
- uid string - Unique id as a URL segment
- payload CommandResult - command details
- ocnSignature string? (default ()) - OCN Signature
Return Type
- OcpiResponseUnit|error - OK
postCancelReservation
function postCancelReservation(string authorization, string xRequestId, string xCorrelationId, string ocpiFromCountryCode, string ocpiFromPartyId, string ocpiToCountryCode, string ocpiToPartyId, CancelReservation payload, string? ocnSignature) returns OcpiResponseCommandResponse|error
Cancel an existing reservation
Parameters
- authorization string - Authorization token
- xRequestId string - Every request shall contain a unique request ID, the response to this request shall contain the same ID
- xCorrelationId string - Every request/response shall contain a unique correlation ID, every response to this request shall contain the same ID
- ocpiFromCountryCode string - Country code of the connected party this message is sent from
- ocpiFromPartyId string - Party ID of the connected party this message is sent from
- ocpiToCountryCode string - Country code of the connected party this message is to be sent to
- ocpiToPartyId string - Party ID of the connected party this message is to be sent to
- payload CancelReservation - Reservation detail for cancellation
- ocnSignature string? (default ()) - OCN Signature
Return Type
postReserveNow
function postReserveNow(string authorization, string xRequestId, string xCorrelationId, string ocpiFromCountryCode, string ocpiFromPartyId, string ocpiToCountryCode, string ocpiToPartyId, ReserveNow payload, string? ocnSignature) returns OcpiResponseCommandResponse|error
Reserves a (specific) connector of a Charge Point for a given Token
Parameters
- authorization string - Authorization token
- xRequestId string - Every request shall contain a unique request ID, the response to this request shall contain the same ID
- xCorrelationId string - Every request/response shall contain a unique correlation ID, every response to this request shall contain the same ID
- ocpiFromCountryCode string - Country code of the connected party this message is sent from
- ocpiFromPartyId string - Party ID of the connected party this message is sent from
- ocpiToCountryCode string - Country code of the connected party this message is to be sent to
- ocpiToPartyId string - Party ID of the connected party this message is to be sent to
- payload ReserveNow - Reservation details
- ocnSignature string? (default ()) - OCN Signature
Return Type
postStartSession
function postStartSession(string authorization, string xRequestId, string xCorrelationId, string ocpiFromCountryCode, string ocpiFromPartyId, string ocpiToCountryCode, string ocpiToPartyId, StartSession payload, string? ocnSignature) returns OcpiResponseCommandResponse|error
Starts a session.
Parameters
- authorization string - Authorization token
- xRequestId string - Every request shall contain a unique request ID, the response to this request shall contain the same ID
- xCorrelationId string - Every request/response shall contain a unique correlation ID, every response to this request shall contain the same ID
- ocpiFromCountryCode string - Country code of the connected party this message is sent from
- ocpiFromPartyId string - Party ID of the connected party this message is sent from
- ocpiToCountryCode string - Country code of the connected party this message is to be sent to
- ocpiToPartyId string - Party ID of the connected party this message is to be sent to
- payload StartSession - Session details
- ocnSignature string? (default ()) - OCN Signature
Return Type
postStopSession
function postStopSession(string authorization, string xRequestId, string xCorrelationId, string ocpiFromCountryCode, string ocpiFromPartyId, string ocpiToCountryCode, string ocpiToPartyId, StopSession payload, string? ocnSignature) returns OcpiResponseCommandResponse|error
Stops a session
Parameters
- authorization string - Authorization token
- xRequestId string - Every request shall contain a unique request ID, the response to this request shall contain the same ID
- xCorrelationId string - Every request/response shall contain a unique correlation ID, every response to this request shall contain the same ID
- ocpiFromCountryCode string - Country code of the connected party this message is sent from
- ocpiFromPartyId string - Party ID of the connected party this message is sent from
- ocpiToCountryCode string - Country code of the connected party this message is to be sent to
- ocpiToPartyId string - Party ID of the connected party this message is to be sent to
- payload StopSession - Session details
- ocnSignature string? (default ()) - OCN Signature
Return Type
postUnlockConnector
function postUnlockConnector(string authorization, string xRequestId, string xCorrelationId, string ocpiFromCountryCode, string ocpiFromPartyId, string ocpiToCountryCode, string ocpiToPartyId, UnlockConnector payload, string? ocnSignature) returns OcpiResponseCommandResponse|error
Unlocks a connector of a Charge Point
Parameters
- authorization string - Authorization token
- xRequestId string - Every request shall contain a unique request ID, the response to this request shall contain the same ID
- xCorrelationId string - Every request/response shall contain a unique correlation ID, every response to this request shall contain the same ID
- ocpiFromCountryCode string - Country code of the connected party this message is sent from
- ocpiFromPartyId string - Party ID of the connected party this message is sent from
- ocpiToCountryCode string - Country code of the connected party this message is to be sent to
- ocpiToPartyId string - Party ID of the connected party this message is to be sent to
- payload UnlockConnector - Charge connector details
- ocnSignature string? (default ()) - OCN Signature
Return Type
getCredentials
function getCredentials(string authorization) returns OcpiResponseCredentials|error
Retrieves the credentials object to access the server’s platform
Parameters
- authorization string - Authorization token
Return Type
putCredentials
function putCredentials(string authorization, Credentials payload) returns OcpiResponseCredentials|error
Provides the server with an updated credentials object to access the client’s system
Return Type
postCredentials
function postCredentials(string authorization, Credentials payload) returns OcpiResponseCredentials|error
Provides the server with a credentials object to access the client’s system (i.e. register)
Return Type
deleteCredentials
function deleteCredentials(string authorization) returns OcpiResponse|error
Informs the server that its credentials to the client’s system are now invalid (i.e. unregister)
Parameters
- authorization string - Authorization token
Return Type
- OcpiResponse|error - OK
getLocationListFromDataOwner
function getLocationListFromDataOwner(string authorization, string xRequestId, string xCorrelationId, string ocpiFromCountryCode, string ocpiFromPartyId, string ocpiToCountryCode, string ocpiToPartyId, string? ocnSignature, string? dateFrom, string? dateTo, int? offset, int? 'limit) returns OcpiResponseLocationList|error
Fetches a list of Locations, last updated between the {date_from} and {date_to} (paginated), or get a specificLocation, EVSE or Connector
Parameters
- authorization string - Authorization token
- xRequestId string - Every request shall contain a unique request ID, the response to this request shall contain the same ID
- xCorrelationId string - Every request/response shall contain a unique correlation ID, every response to this request shall contain the same ID
- ocpiFromCountryCode string - Country code of the connected party this message is sent from
- ocpiFromPartyId string - Party ID of the connected party this message is sent from
- ocpiToCountryCode string - Country code of the connected party this message is to be sent to
- ocpiToPartyId string - Party ID of the connected party this message is to be sent to
- ocnSignature string? (default ()) - OCN Signature
- dateFrom string? (default ()) - Only return CDRs that have last_updated after or equal to this Date/Time(inclusive)
- dateTo string? (default ()) - Only return CDRs that have last_updated up to this Date/Time, but not including (exclusive)
- offset int? (default ()) - The offset of the first object returned. Default is 0
- 'limit int? (default ()) - Maximum number of objects to GET
Return Type
getLocationPageFromDataOwner
function getLocationPageFromDataOwner(string authorization, string xRequestId, string xCorrelationId, string ocpiFromCountryCode, string ocpiFromPartyId, string ocpiToCountryCode, string ocpiToPartyId, string uid, string? ocnSignature) returns OcpiResponseLocationList|error
Lists of all available Locations with valid EVSEs
Parameters
- authorization string - Authorization token
- xRequestId string - Every request shall contain a unique request ID, the response to this request shall contain the same ID
- xCorrelationId string - Every request/response shall contain a unique correlation ID, every response to this request shall contain the same ID
- ocpiFromCountryCode string - Country code of the connected party this message is sent from
- ocpiFromPartyId string - Party ID of the connected party this message is sent from
- ocpiToCountryCode string - Country code of the connected party this message is to be sent to
- ocpiToPartyId string - Party ID of the connected party this message is to be sent to
- uid string - Page ID
- ocnSignature string? (default ()) - OCN Signature
Return Type
getLocationObjectFromDataOwner
function getLocationObjectFromDataOwner(string authorization, string xRequestId, string xCorrelationId, string ocpiFromCountryCode, string ocpiFromPartyId, string ocpiToCountryCode, string ocpiToPartyId, string locationID, string? ocnSignature) returns OcpiResponseLocation|error
Retrieves information about one specific Location
Parameters
- authorization string - Authorization token
- xRequestId string - Every request shall contain a unique request ID, the response to this request shall contain the same ID
- xCorrelationId string - Every request/response shall contain a unique correlation ID, every response to this request shall contain the same ID
- ocpiFromCountryCode string - Country code of the connected party this message is sent from
- ocpiFromPartyId string - Party ID of the connected party this message is sent from
- ocpiToCountryCode string - Country code of the connected party this message is to be sent to
- ocpiToPartyId string - Party ID of the connected party this message is to be sent to
- locationID string - ID of the charging location of an operator
- ocnSignature string? (default ()) - OCN Signature
Return Type
getEvseObjectFromDataOwner
function getEvseObjectFromDataOwner(string authorization, string xRequestId, string xCorrelationId, string ocpiFromCountryCode, string ocpiFromPartyId, string ocpiToCountryCode, string ocpiToPartyId, string locationID, string evseUID, string? ocnSignature) returns OcpiResponseEvse|error
Retrieves a Location, EVSE by ID
Parameters
- authorization string - Authorization token
- xRequestId string - Every request shall contain a unique request ID, the response to this request shall contain the same ID
- xCorrelationId string - Every request/response shall contain a unique correlation ID, every response to this request shall contain the same ID
- ocpiFromCountryCode string - Country code of the connected party this message is sent from
- ocpiFromPartyId string - Party id of the connected party this message is sent from
- ocpiToCountryCode string - Country code of the connected party this message is to be sent to
- ocpiToPartyId string - Party ID of the connected party this message is to be sent to
- locationID string - ID of the charging location of an operator
- evseUID string - ID of the EVSE
- ocnSignature string? (default ()) - OCN Signature
Return Type
- OcpiResponseEvse|error - OK
getConnectorObjectFromDataOwner
function getConnectorObjectFromDataOwner(string authorization, string xRequestId, string xCorrelationId, string ocpiFromCountryCode, string ocpiFromPartyId, string ocpiToCountryCode, string ocpiToPartyId, string locationID, string evseUID, string connectorID, string? ocnSignature) returns OcpiResponseConnector|error
Retrieves a Location, EVSE or Connector by ID
Parameters
- authorization string - Authorization token
- xRequestId string - Every request shall contain a unique request ID, the response to this request shall contain the same ID
- xCorrelationId string - Every request/response shall contain a unique correlation ID, every response to this request shall contain the same ID
- ocpiFromCountryCode string - Country code of the connected party this message is sent from
- ocpiFromPartyId string - Party ID of the connected party this message is sent from
- ocpiToCountryCode string - Country code of the connected party this message is to be sent to
- ocpiToPartyId string - Party ID of the connected party this message is to be sent to
- locationID string - ID of the charging location of an operator
- evseUID string - ID of the EVSE
- connectorID string - Connector ID
- ocnSignature string? (default ()) - OCN Signature
Return Type
getClientOwnedLocation
function getClientOwnedLocation(string authorization, string xRequestId, string xCorrelationId, string ocpiFromCountryCode, string ocpiFromPartyId, string ocpiToCountryCode, string ocpiToPartyId, string countryCode, string partyID, string locationID, string? ocnSignature) returns OcpiResponseLocation|error
Retrieves a Location as it is stored in the eMSP system.
Parameters
- authorization string - Authorization token
- xRequestId string - Every request shall contain a unique request ID, the response to this request shall contain the same ID
- xCorrelationId string - Every request/response shall contain a unique correlation ID, every response to this request shall contain the same ID
- ocpiFromCountryCode string - Country code of the connected party this message is sent from
- ocpiFromPartyId string - Party ID of the connected party this message is sent from
- ocpiToCountryCode string - Country code of the connected party this message is to be sent to
- ocpiToPartyId string - Party ID of the connected party this message is to be sent to
- countryCode string - Country code of the CPO requesting data from the eMSP system
- partyID string - Party ID (Provider ID) of the CPO requesting data from the eMSP system
- locationID string - Location.id of the Location object to retrieve
- ocnSignature string? (default ()) - OCN Signature
Return Type
putClientOwnedLocation
function putClientOwnedLocation(string authorization, string xRequestId, string xCorrelationId, string ocpiFromCountryCode, string ocpiFromPartyId, string ocpiToCountryCode, string ocpiToPartyId, string countryCode, string partyID, string locationID, Location payload, string? ocnSignature) returns OcpiResponseUnit|error
Pushes new/updated Location and/or Connector to the eMSP
Parameters
- authorization string - Authorization token
- xRequestId string - Every request shall contain a unique request ID, the response to this request shall contain the same ID
- xCorrelationId string - Every request/response shall contain a unique correlation ID, every response to this request shall contain the same ID
- ocpiFromCountryCode string - Country code of the connected party this message is sent from
- ocpiFromPartyId string - Party ID of the connected party this message is sent from
- ocpiToCountryCode string - Country code of the connected party this message is to be sent to
- ocpiToPartyId string - Party ID of the connected party this message is to be sent to
- countryCode string - Country code of the CPO requesting this PUT to the eMSP system. This shall be the same value as the country_code in the Location object being pushed
- partyID string - Party ID (Provider ID) of the CPO requesting this PUT to the eMSP system. This shall be the same value as the party_id in the Location object being pushed.
- locationID string - Location.id of the new Location object, or the Location of which an EVSE orConnector object is pushed
- payload Location - Location details
- ocnSignature string? (default ()) - OCN Signature
Return Type
- OcpiResponseUnit|error - OK
patchClientOwnedLocation
function patchClientOwnedLocation(string authorization, string xRequestId, string xCorrelationId, string ocpiFromCountryCode, string ocpiFromPartyId, string ocpiToCountryCode, string ocpiToPartyId, string countryCode, string partyID, string locationID, json payload, string? ocnSignature) returns OcpiResponseUnit|error
Notifies the eMSP of partial updates to a Location, EVSE or Connector (such as the status).
Parameters
- authorization string - Authorization token
- xRequestId string - Every request shall contain a unique request ID, the response to this request shall contain the same ID
- xCorrelationId string - Every request/response shall contain a unique correlation ID, every response to this request shall contain the same ID
- ocpiFromCountryCode string - Country code of the connected party this message is sent from
- ocpiFromPartyId string - Party ID of the connected party this message is sent from
- ocpiToCountryCode string - Country code of the connected party this message is to be sent to
- ocpiToPartyId string - Party ID of the connected party this message is to be sent to
- countryCode string - Country code
- partyID string - Party ID
- locationID string - ID of the charging location of an operator
- payload json - Location details
- ocnSignature string? (default ()) - OCN Signature
Return Type
- OcpiResponseUnit|error - OK
getClientOwnedEvse
function getClientOwnedEvse(string authorization, string xRequestId, string xCorrelationId, string ocpiFromCountryCode, string ocpiFromPartyId, string ocpiToCountryCode, string ocpiToPartyId, string countryCode, string partyID, string locationID, string evseUID, string? ocnSignature) returns OcpiResponseEvse|error
Retrieves a Location as it is stored in the eMSP system by EVSE UID
Parameters
- authorization string - Authorization token
- xRequestId string - Every request shall contain a unique request ID, the response to this request shall contain the same ID
- xCorrelationId string - Every request/response shall contain a unique correlation ID, every response to this request shall contain the same ID
- ocpiFromCountryCode string - Country code of the connected party this message is sent from
- ocpiFromPartyId string - Party ID of the connected party this message is sent from
- ocpiToCountryCode string - Country code of the connected party this message is to be sent to
- ocpiToPartyId string - Party ID of the connected party this message is to be sent to
- countryCode string - Country code of the CPO requesting data from the eMSP system.
- partyID string - Party ID (Provider ID) of the CPO requesting data from the eMSP system.
- locationID string - Location.id of the Location object to retrieve
- evseUID string - Evse.uid, required when requesting an EVSE or Connector object
- ocnSignature string? (default ()) - OCN Signature
Return Type
- OcpiResponseEvse|error - OK
putClientOwnedEvse
function putClientOwnedEvse(string authorization, string xRequestId, string xCorrelationId, string ocpiFromCountryCode, string ocpiFromPartyId, string ocpiToCountryCode, string ocpiToPartyId, string countryCode, string partyID, string locationID, string evseUID, Evse payload, string? ocnSignature) returns OcpiResponseUnit|error
Pushes new/updated Location, EVSE and/or Connector to the eMSP by EVSE UID
Parameters
- authorization string - Authorization token
- xRequestId string - Every request shall contain a unique request ID, the response to this request shall contain the same ID
- xCorrelationId string - Every request/response shall contain a unique correlation ID, every response to this request shall contain the same ID
- ocpiFromCountryCode string - Country code of the connected party this message is sent from
- ocpiFromPartyId string - Party ID of the connected party this message is sent from
- ocpiToCountryCode string - Country code of the connected party this message is to be sent to
- ocpiToPartyId string - Party ID of the connected party this message is to be sent to
- countryCode string - Country code of the CPO requesting this PUT to the eMSP system. This shall be the same value as the country_code in the Location object being pushed
- partyID string - Party ID (Provider ID) of the CPO requesting this PUT to the eMSP system. ThisSHALL be the same value as the party_id in the Location object being pushed.
- locationID string - Location.id of the new Location object, or the Location of which an EVSE orConnector object is pushed
- evseUID string - Evse.uid, required when an EVSE or Connector object is pushed
- payload Evse - EVSE details
- ocnSignature string? (default ()) - OCN Signature
Return Type
- OcpiResponseUnit|error - OK
patchClientOwnedEvse
function patchClientOwnedEvse(string authorization, string xRequestId, string xCorrelationId, string ocpiFromCountryCode, string ocpiFromPartyId, string ocpiToCountryCode, string ocpiToPartyId, string countryCode, string partyID, string locationID, string evseUID, json payload, string? ocnSignature) returns OcpiResponseUnit|error
Notifies the eMSP of partial updates to a Location, EVSE or Connector (such as the status) by EVSE UID
Parameters
- authorization string - Authorization token
- xRequestId string - Every request shall contain a unique request ID, the response to this request shall contain the same ID
- xCorrelationId string - Every request/response shall contain a unique correlation ID, every response to this request shall contain the same ID
- ocpiFromCountryCode string - Country code of the connected party this message is sent from
- ocpiFromPartyId string - Party ID of the connected party this message is sent from
- ocpiToCountryCode string - Country code of the connected party this message is to be sent to
- ocpiToPartyId string - Party ID of the connected party this message is to be sent to
- countryCode string - Country code of the CPO requesting this PATCH to the eMSP system
- partyID string - Party ID (Provider ID) of the CPO requesting this PATCH to the eMSP system
- locationID string - ID of the charging location of an operator
- evseUID string - ID of the EVSE
- payload json - Request body details
- ocnSignature string? (default ()) - OCN Signature
Return Type
- OcpiResponseUnit|error - OK
getClientOwnedConnector
function getClientOwnedConnector(string authorization, string xRequestId, string xCorrelationId, string ocpiFromCountryCode, string ocpiFromPartyId, string ocpiToCountryCode, string ocpiToPartyId, string countryCode, string partyID, string locationID, string evseUID, string connectorID, string? ocnSignature) returns OcpiResponseConnector|error
Retrieves a Location as it is stored in the eMSP system by EVSE UID and Connector ID
Parameters
- authorization string - Authorization token
- xRequestId string - Every request shall contain a unique request ID, the response to this request shall contain the same ID
- xCorrelationId string - Every request/response shall contain a unique correlation ID, every response to this request shall contain the same ID
- ocpiFromCountryCode string - Country code of the connected party this message is sent from
- ocpiFromPartyId string - Party ID of the connected party this message is sent from
- ocpiToCountryCode string - Country code of the connected party this message is to be sent to
- ocpiToPartyId string - Party ID of the connected party this message is to be sent to
- countryCode string - Country code of the CPO requesting this PUT to the eMSP system. This shall be the same value as the country_code in the Location object being pushed.
- partyID string - Party ID (Provider ID) of the CPO requesting this PUT to the eMSP system. ThisSHALL be the same value as the party_id in the Location object being pushed
- locationID string - Location.id of the new Location object, or the Location of which an EVSE orConnector object is pushed
- evseUID string - Evse.uid, required when an EVSE or Connector object is pushed.
- connectorID string - Connector.id, required when a Connector object is pushed.
- ocnSignature string? (default ()) - OCN Signature
Return Type
putClientOwnedConnector
function putClientOwnedConnector(string authorization, string xRequestId, string xCorrelationId, string ocpiFromCountryCode, string ocpiFromPartyId, string ocpiToCountryCode, string ocpiToPartyId, string countryCode, string partyID, string locationID, string evseUID, string connectorID, Connector payload, string? ocnSignature) returns OcpiResponseUnit|error
Pushes new/updated Location, EVSE and/or Connector to the eMSP by EVSE UID and connector ID
Parameters
- authorization string - Authorization token
- xRequestId string - Every request shall contain a unique request ID, the response to this request shall contain the same ID
- xCorrelationId string - Every request/response shall contain a unique correlation ID, every response to this request shall contain the same ID
- ocpiFromCountryCode string - Country code of the connected party this message is sent from
- ocpiFromPartyId string - Party ID of the connected party this message is sent from
- ocpiToCountryCode string - Country code of the connected party this message is to be sent to
- ocpiToPartyId string - Party ID of the connected party this message is to be sent to
- countryCode string - Country code of the CPO requesting this PUT to the eMSP system. This shall be the same value as the country_code in the Location object being pushed
- partyID string - Party ID (Provider ID) of the CPO requesting this PUT to the eMSP system. This shall be the same value as the party_id in the Location object being pushed.
- locationID string - Location.id of the new Location object, or the Location of which an EVSE orConnector object is pushed
- evseUID string - Evse.uid, required when an EVSE or Connector object is pushed.
- connectorID string - Connector.id, required when a Connector object is pushed
- payload Connector - Connector Details
- ocnSignature string? (default ()) - OCN Signature
Return Type
- OcpiResponseUnit|error - OK
patchClientOwnedConnector
function patchClientOwnedConnector(string authorization, string xRequestId, string xCorrelationId, string ocpiFromCountryCode, string ocpiFromPartyId, string ocpiToCountryCode, string ocpiToPartyId, string countryCode, string partyID, string locationID, string evseUID, string connectorID, json payload, string? ocnSignature) returns OcpiResponseUnit|error
Notifies the eMSP of partial updates to a Location, EVSE or Connector (such as the status) by EVSE UID and connector ID
Parameters
- authorization string - Authorization token
- xRequestId string - Every request shall contain a unique request ID, the response to this request shall contain the same ID
- xCorrelationId string - Every request/response shall contain a unique correlation ID, every response to this request shall contain the same ID
- ocpiFromCountryCode string - Country code of the connected party this message is sent from
- ocpiFromPartyId string - Party ID of the connected party this message is sent from
- ocpiToCountryCode string - Country code of the connected party this message is to be sent to
- ocpiToPartyId string - Party ID of the connected party this message is to be sent to
- countryCode string - Country code of the CPO requesting this PATCH to the eMSP system
- partyID string - Party ID (Provider ID) of the CPO requesting this PATCH to the eMSP system
- locationID string - ID of the charging location of an operator
- evseUID string - ID of the EVSE
- connectorID string - ID of the Connector
- payload json - OCPI request details
- ocnSignature string? (default ()) - OCN Signature
Return Type
- OcpiResponseUnit|error - OK
updateWhitelist
function updateWhitelist(string authorization, OcnRulesListParty[] payload) returns OcpiResponseUnit|error
Updates OCN rules - whitelist
Parameters
- authorization string - Authorization token
- payload OcnRulesListParty[] - OCN Rules List Details
Return Type
- OcpiResponseUnit|error - OK
appendToWhitelist
function appendToWhitelist(string authorization, OcnRulesListParty payload) returns OcpiResponseUnit|error
Parameters
- authorization string - Authorization token
- payload OcnRulesListParty - OCN Rules List Details
Return Type
- OcpiResponseUnit|error - OK
updateBlacklist
function updateBlacklist(string authorization, OcnRulesListParty[] payload) returns OcpiResponseUnit|error
Updates OCN rules - blacklist
Parameters
- authorization string - Authorization token
- payload OcnRulesListParty[] - OCN Rules List Details
Return Type
- OcpiResponseUnit|error - OK
appendToBlacklist
function appendToBlacklist(string authorization, OcnRulesListParty payload) returns OcpiResponseUnit|error
Parameters
- authorization string - Authorization token
- payload OcnRulesListParty - OCN Rules List Details
Return Type
- OcpiResponseUnit|error - OK
updateSignatures
function updateSignatures(string authorization) returns OcpiResponseUnit|error
Updates OCN Rules - signature
Parameters
- authorization string - Authorization token
Return Type
- OcpiResponseUnit|error - OK
blockAll
function blockAll(string authorization) returns OcpiResponseUnit|error
Updates OCN Rules - block-all
Parameters
- authorization string - Authorization token
Return Type
- OcpiResponseUnit|error - OK
deleteFromWhitelist
function deleteFromWhitelist(string authorization, string countryCode, string partyID) returns OcpiResponseUnit|error
Deletes OCN Rules - whitelist
by country code and party ID
Parameters
- authorization string - Authorization token
- countryCode string - Country code
- partyID string - Party ID
Return Type
- OcpiResponseUnit|error - OK
deleteFromBlacklist
function deleteFromBlacklist(string authorization, string countryCode, string partyID) returns OcpiResponseUnit|error
Deletes OCN Rules - blacklist
by country code and party ID
Parameters
- authorization string - Authorization token
- countryCode string - Country code
- partyID string - Party ID
Return Type
- OcpiResponseUnit|error - OK
getRules
function getRules(string authorization) returns OcpiResponseOcnRules|error
Gets all OCN Rules
Parameters
- authorization string - Authorization token
Return Type
getSessionsFromDataOwner
function getSessionsFromDataOwner(string authorization, string xRequestId, string xCorrelationId, string ocpiFromCountryCode, string ocpiFromPartyId, string ocpiToCountryCode, string ocpiToPartyId, string? ocnSignature, string? dateFrom, string? dateTo, int? offset, int? 'limit) returns OcpiResponseSessionList|error
Fetches Session objects of charging sessions last updated between the {date_from} and {date_to}
Parameters
- authorization string - Authorization token
- xRequestId string - Every request shall contain a unique request ID, the response to this request shall contain the same ID
- xCorrelationId string - Every request/response shall contain a unique correlation ID, every response to this request shall contain the same ID
- ocpiFromCountryCode string - Country code of the connected party this message is sent from
- ocpiFromPartyId string - Party ID of the connected party this message is sent from
- ocpiToCountryCode string - Country code of the connected party this message is to be sent to
- ocpiToPartyId string - Party ID of the connected party this message is to be sent to
- ocnSignature string? (default ()) - OCN Signature
- dateFrom string? (default ()) - Only return Sessions that have last_updated after or equal to this Date/Time(inclusive)
- dateTo string? (default ()) - Only return Sessions that have last_updated up to this Date/Time, but not including (exclusive)
- offset int? (default ()) - The offset of the first object returned. Default is 0
- 'limit int? (default ()) - Maximum number of objects to GET
Return Type
getSessionsPageFromDataOwner
function getSessionsPageFromDataOwner(string authorization, string xRequestId, string xCorrelationId, string ocpiFromCountryCode, string ocpiFromPartyId, string ocpiToCountryCode, string ocpiToPartyId, string uid, string? ocnSignature) returns OcpiResponseSessionList|error
Fetches Session objects of charging sessions
Parameters
- authorization string - Authorization token
- xRequestId string - Every request shall contain a unique request ID, the response to this request shall contain the same ID
- xCorrelationId string - Every request/response shall contain a unique correlation ID, every response to this request shall contain the same ID
- ocpiFromCountryCode string - Country code of the connected party this message is sent from
- ocpiFromPartyId string - Party ID of the connected party this message is sent from
- ocpiToCountryCode string - Country code of the connected party this message is to be sent to
- ocpiToPartyId string - Party ID of the connected party this message is to be sent to
- uid string - Page ID
- ocnSignature string? (default ()) - OCN Signature
Return Type
putChargingPreferences
function putChargingPreferences(string authorization, string xRequestId, string xCorrelationId, string ocpiFromCountryCode, string ocpiFromPartyId, string ocpiToCountryCode, string ocpiToPartyId, string sessionID, ChargingPreferences payload, string? ocnSignature) returns OcpiResponseChargingPreferencesResponse|error
Sets/Updates the driver’s Charging Preferences for the charging session
Parameters
- authorization string - Authorization token
- xRequestId string - Every request shall contain a unique request ID, the response to this request shall contain the same ID
- xCorrelationId string - Every request/response shall contain a unique correlation ID, every response to this request shall contain the same ID
- ocpiFromCountryCode string - Country code of the connected party this message is sent from
- ocpiFromPartyId string - Party ID of the connected party this message is sent from
- ocpiToCountryCode string - Country code of the connected party this message is to be sent to
- ocpiToPartyId string - Party ID of the connected party this message is to be sent to
- sessionID string - Charging session ID
- payload ChargingPreferences - Charging preferences details
- ocnSignature string? (default ()) - OCN Signature
Return Type
getClientOwnedSession
function getClientOwnedSession(string authorization, string xRequestId, string xCorrelationId, string ocpiFromCountryCode, string ocpiFromPartyId, string ocpiToCountryCode, string ocpiToPartyId, string countryCode, string partyID, string sessionID, string? ocnSignature) returns OcpiResponseSession|error
Retrieves a Session object from the eMSP’s system with Session.id equal to {session_id}
Parameters
- authorization string - Authorization token
- xRequestId string - Every request shall contain a unique request ID, the response to this request shall contain the same ID
- xCorrelationId string - Every request/response shall contain a unique correlation ID, every response to this request shall contain the same ID
- ocpiFromCountryCode string - Country code of the connected party this message is sent from
- ocpiFromPartyId string - Party ID of the connected party this message is sent from
- ocpiToCountryCode string - Country code of the connected party this message is to be sent to
- ocpiToPartyId string - Party ID of the connected party this message is to be sent to
- countryCode string - Country code of the CPO performing the GET on the eMSP’s system
- partyID string - Party ID (Provider ID) of the CPO performing the GET on the eMSP’s system
- sessionID string - ID of the Session object to get from the eMSP’s system.
- ocnSignature string? (default ()) - OCN Signature
Return Type
- OcpiResponseSession|error - OK
putClientOwnedSession
function putClientOwnedSession(string authorization, string xRequestId, string xCorrelationId, string ocpiFromCountryCode, string ocpiFromPartyId, string ocpiToCountryCode, string ocpiToPartyId, string countryCode, string partyID, string sessionID, Session payload, string? ocnSignature) returns OcpiResponseUnit|error
Informs the eMSP’s system about a new/updated Session object in the CPO’s system
Parameters
- authorization string - Authorization token
- xRequestId string - Every request shall contain a unique request ID, the response to this request shall contain the same ID
- xCorrelationId string - Every request/response shall contain a unique correlation ID, every response to this request shall contain the same ID
- ocpiFromCountryCode string - Country code of the connected party this message is sent from
- ocpiFromPartyId string - Party ID of the connected party this message is sent from
- ocpiToCountryCode string - Country code of the connected party this message is to be sent to
- ocpiToPartyId string - Party ID of the connected party this message is to be sent to
- countryCode string - Country code of the CPO performing this PUT on the eMSP’s system. This shall be the same value as the country_code in the Session object being pushed.
- partyID string - Party ID (Provider ID) of the CPO performing this PUT on the eMSP’s system.This shall be the same value as the party_id in the Session object being pushed.
- sessionID string - ID of the new or updated Session object.
- payload Session - Session details
- ocnSignature string? (default ()) - OCN Signature
Return Type
- OcpiResponseUnit|error - OK
patchClientOwnedSession
function patchClientOwnedSession(string authorization, string xRequestId, string xCorrelationId, string ocpiFromCountryCode, string ocpiFromPartyId, string ocpiToCountryCode, string ocpiToPartyId, string countryCode, string partyID, string sessionID, json payload, string? ocnSignature) returns OcpiResponseUnit|error
Updates the Session object with Session.id equal to {session_id}
Parameters
- authorization string - Authorization token
- xRequestId string - Every request shall contain a unique request ID, the response to this request shall contain the same ID
- xCorrelationId string - Every request/response shall contain a unique correlation ID, every response to this request shall contain the same ID
- ocpiFromCountryCode string - Country code of the connected party this message is sent from
- ocpiFromPartyId string - Party ID of the connected party this message is sent from
- ocpiToCountryCode string - Country code of the connected party this message is to be sent to
- ocpiToPartyId string - Party ID of the connected party this message is to be sent to
- countryCode string - Country code of the CPO
- partyID string - Country code of the CPO
- sessionID string - ID of the charging session
- payload json - Request Body detail
- ocnSignature string? (default ()) - OCN Signature
Return Type
- OcpiResponseUnit|error - OK
getClientOwnedTariff
function getClientOwnedTariff(string authorization, string xRequestId, string xCorrelationId, string ocpiFromCountryCode, string ocpiFromPartyId, string ocpiToCountryCode, string ocpiToPartyId, string countryCode, string partyID, string tariffID, string? ocnSignature) returns OcpiResponseTariff|error
Retrieves a Tariff as it is stored in the eMSP’s system
Parameters
- authorization string - Authorization token
- xRequestId string - Every request shall contain a unique request ID, the response to this request shall contain the same ID
- xCorrelationId string - Every request/response shall contain a unique correlation ID, every response to this request shall contain the same ID
- ocpiFromCountryCode string - Country code of the connected party this message is sent from
- ocpiFromPartyId string - Party ID of the connected party this message is sent from
- ocpiToCountryCode string - Country code of the connected party this message is to be sent to
- ocpiToPartyId string - Party ID of the connected party this message is to be sent to
- countryCode string - Country code of the CPO performing the PUT request on the eMSP’s system
- partyID string - Party ID (Provider ID) of the CPO performing the PUT request on the eMSP’ssystem
- tariffID string - Tariff.id of the Tariff object to retrieve
- ocnSignature string? (default ()) - OCN Signature
Return Type
- OcpiResponseTariff|error - OK
putClientOwnedTariff
function putClientOwnedTariff(string authorization, string xRequestId, string xCorrelationId, string ocpiFromCountryCode, string ocpiFromPartyId, string ocpiToCountryCode, string ocpiToPartyId, string countryCode, string partyID, string tariffID, Tariff payload, string? ocnSignature) returns OcpiResponseUnit|error
Pushes new/updated Tariff object to the eMSP.
Parameters
- authorization string - Authorization token
- xRequestId string - Every request shall contain a unique request ID, the response to this request shall contain the same ID
- xCorrelationId string - Every request/response shall contain a unique correlation ID, every response to this request shall contain the same ID
- ocpiFromCountryCode string - Country code of the connected party this message is sent from
- ocpiFromPartyId string - Party ID of the connected party this message is sent from
- ocpiToCountryCode string - Country code of the connected party this message is to be sent to
- ocpiToPartyId string - Party ID of the connected party this message is to be sent to
- countryCode string - Country code of the CPO performing the PUT request on the eMSP’s system.This shall be the same value as the country_code in the Tariff object being pushed
- partyID string - Party ID (Provider ID) of the CPO performing the PUT request on the eMSP’ssystem. This shall be the same value as the party_id in the Tariff object being pushed
- tariffID string - Tariff.id of the Tariff object to create or replace
- payload Tariff - Tariff detail
- ocnSignature string? (default ()) - OCN Signature
Return Type
- OcpiResponseUnit|error - OK
deleteClientOwnedTariff
function deleteClientOwnedTariff(string authorization, string xRequestId, string xCorrelationId, string ocpiFromCountryCode, string ocpiFromPartyId, string ocpiToCountryCode, string ocpiToPartyId, string countryCode, string partyID, string tariffID, string? ocnSignature) returns OcpiResponseUnit|error
Deletes a Tariff object which is not used any more and will not be used in future either
Parameters
- authorization string - Authorization token
- xRequestId string - Every request shall contain a unique request ID, the response to this request shall contain the same ID
- xCorrelationId string - Every request/response shall contain a unique correlation ID, every response to this request shall contain the same ID
- ocpiFromCountryCode string - Country code of the connected party this message is sent from
- ocpiFromPartyId string - Party ID of the connected party this message is sent from
- ocpiToCountryCode string - Country code of the connected party this message is to be sent to
- ocpiToPartyId string - Party ID of the connected party this message is to be sent to
- countryCode string - Country code of the CPO performing the PUT request on the eMSP’s system
- partyID string - Party ID (Provider ID) of the CPO performing the PUT request on the eMSP’ssystem
- tariffID string - Tariff.id of the Tariff object to delete
- ocnSignature string? (default ()) - OCN Signature
Return Type
- OcpiResponseUnit|error - OK
getTariffsFromDataOwner
function getTariffsFromDataOwner(string authorization, string xRequestId, string xCorrelationId, string ocpiFromCountryCode, string ocpiFromPartyId, string ocpiToCountryCode, string ocpiToPartyId, string? ocnSignature, string? dateFrom, string? dateTo, int? offset, int? 'limit) returns OcpiResponseTariffList|error
Returns Tariff objects from the CPO, last updated between the {date_from} and {date_to}
Parameters
- authorization string - Authorization token
- xRequestId string - Every request shall contain a unique request ID, the response to this request shall contain the same ID
- xCorrelationId string - Every request/response shall contain a unique correlation ID, every response to this request shall contain the same ID
- ocpiFromCountryCode string - Country code of the connected party this message is sent from
- ocpiFromPartyId string - Party ID of the connected party this message is sent from
- ocpiToCountryCode string - Country code of the connected party this message is to be sent to
- ocpiToPartyId string - Party ID of the connected party this message is to be sent to
- ocnSignature string? (default ()) - OCN Signature
- dateFrom string? (default ()) - Only return Tariffs that have last_updated after or equal to this Date/Time(inclusive).
- dateTo string? (default ()) - Only return Tariffs that have last_updated up to this Date/Time, but notincluding (exclusive)
- offset int? (default ()) - The offset of the first object returned. Default is 0
- 'limit int? (default ()) - Maximum number of objects to GET
Return Type
getTariffsPageFromDataOwner
function getTariffsPageFromDataOwner(string authorization, string xRequestId, string xCorrelationId, string ocpiFromCountryCode, string ocpiFromPartyId, string ocpiToCountryCode, string ocpiToPartyId, string uid, string? ocnSignature) returns OcpiResponseTariffList|error
Returns Tariff objects from the CPO by page ID
Parameters
- authorization string - Authorization token
- xRequestId string - Every request shall contain a unique request ID, the response to this request shall contain the same ID
- xCorrelationId string - Every request/response shall contain a unique correlation ID, every response to this request shall contain the same ID
- ocpiFromCountryCode string - Country code of the connected party this message is sent from
- ocpiFromPartyId string - Party ID of the connected party this message is sent from
- ocpiToCountryCode string - Country code of the connected party this message is to be sent to
- ocpiToPartyId string - Party ID of the connected party this message is to be sent to
- uid string - Page ID
- ocnSignature string? (default ()) - OCN Signature
Return Type
getTokensFromDataOwner
function getTokensFromDataOwner(string authorization, string xRequestId, string xCorrelationId, string ocpiFromCountryCode, string ocpiFromPartyId, string ocpiToCountryCode, string ocpiToPartyId, string? ocnSignature, string? dateFrom, string? dateTo, int? offset, int? 'limit) returns OcpiResponseTokenList|error
Gets the list of known Tokens, last updated between the {date_from} and {date_to}(Paginated)
Parameters
- authorization string - Authorization token
- xRequestId string - Every request shall contain a unique request ID, the response to this request shall contain the same ID
- xCorrelationId string - Every request/response shall contain a unique correlation ID, every response to this request shall contain the same ID
- ocpiFromCountryCode string - Country code of the connected party this message is sent from
- ocpiFromPartyId string - Party ID of the connected party this message is sent from
- ocpiToCountryCode string - Country code of the connected party this message is to be sent to
- ocpiToPartyId string - Party ID of the connected party this message is to be sent to
- ocnSignature string? (default ()) - OCN Signature
- dateFrom string? (default ()) - Only return Tokens that have last_updated after or equal to this Date/Time(inclusive)
- dateTo string? (default ()) - Only return Tokens that have last_updated up to this Date/Time, but not including (exclusive)
- offset int? (default ()) - The offset of the first object returned. Default is 0
- 'limit int? (default ()) - Maximum number of objects to GET
Return Type
getTokensPageFromDataOwner
function getTokensPageFromDataOwner(string authorization, string xRequestId, string xCorrelationId, string ocpiFromCountryCode, string ocpiFromPartyId, string ocpiToCountryCode, string ocpiToPartyId, string uid, string? ocnSignature) returns OcpiResponseTokenList|error
Gets the list of known Tokens Page ID
Parameters
- authorization string - Authorization token
- xRequestId string - Every request shall contain a unique request ID, the response to this request shall contain the same ID
- xCorrelationId string - Every request/response shall contain a unique correlation ID, every response to this request shall contain the same ID
- ocpiFromCountryCode string - Country code of the connected party this message is sent from
- ocpiFromPartyId string - Party ID of the connected party this message is sent from
- ocpiToCountryCode string - Country code of the connected party this message is to be sent to
- ocpiToPartyId string - Party ID of the connected party this message is to be sent to
- uid string - Page ID
- ocnSignature string? (default ()) - OCN Signature
Return Type
postRealTimeTokenAuthorization
function postRealTimeTokenAuthorization(string authorization, string xRequestId, string xCorrelationId, string ocpiFromCountryCode, string ocpiFromPartyId, string ocpiToCountryCode, string ocpiToPartyId, string tokenUID, LocationReferences payload, string? ocnSignature, string 'type) returns OcpiResponseAuthorizationInfo|error
Does a 'real-time' authorization request to the eMSP system, validating if a Token might be used (at the optionally given Location)
Parameters
- authorization string - Authorization token
- xRequestId string - Every request shall contain a unique request ID, the response to this request shall contain the same ID
- xCorrelationId string - Every request/response shall contain a unique correlation ID, every response to this request shall contain the same ID
- ocpiFromCountryCode string - Country code of the connected party this message is sent from
- ocpiFromPartyId string - Party ID of the connected party this message is sent from
- ocpiToCountryCode string - Country code of the connected party this message is to be sent to
- ocpiToPartyId string - Party ID of the connected party this message is to be sent to
- tokenUID string - Token.uid of the Token for which authorization is requested.
- payload LocationReferences - Location references details
- ocnSignature string? (default ()) - OCN Signature
- 'type string (default "RFID") - Token type of the Token for which this authorization is. Default if omitted- RFID
Return Type
getClientOwnedToken
function getClientOwnedToken(string authorization, string xRequestId, string xCorrelationId, string ocpiFromCountryCode, string ocpiFromPartyId, string ocpiToCountryCode, string ocpiToPartyId, string countryCode, string partyID, string tokenUID, string? ocnSignature, string 'type) returns OcpiResponseToken|error
Retrieves a Token as it is stored in the CPO system
Parameters
- authorization string - Authorization token
- xRequestId string - Every request shall contain a unique request ID, the response to this request shall contain the same ID
- xCorrelationId string - Every request/response shall contain a unique correlation ID, every response to this request shall contain the same ID
- ocpiFromCountryCode string - Country code of the connected party this message is sent from
- ocpiFromPartyId string - Party ID of the connected party this message is sent from
- ocpiToCountryCode string - Country code of the connected party this message is to be sent to
- ocpiToPartyId string - Party ID of the connected party this message is to be sent to
- countryCode string - Country code of the eMSP requesting this GET from the CPO system
- partyID string - Party ID (Provider ID) of the eMSP requesting this GET from the CPO system
- tokenUID string - Token.uid of the Token object to retrieve
- ocnSignature string? (default ()) - OCN Signature
- 'type string (default "RFID") - Token.type of the Token to retrieve. Default if omitted- RFID
Return Type
- OcpiResponseToken|error - OK
putClientOwnedToken
function putClientOwnedToken(string authorization, string xRequestId, string xCorrelationId, string ocpiFromCountryCode, string ocpiFromPartyId, string ocpiToCountryCode, string ocpiToPartyId, string countryCode, string partyID, string tokenUID, Token payload, string? ocnSignature, string 'type) returns OcpiResponseUnit|error
Pushes new/updated Token object to the CPO
Parameters
- authorization string - Authorization token
- xRequestId string - Every request shall contain a unique request ID, the response to this request shall contain the same ID
- xCorrelationId string - Every request/response shall contain a unique correlation ID, every response to this request shall contain the same ID
- ocpiFromCountryCode string - Country code of the connected party this message is sent from
- ocpiFromPartyId string - Party ID of the connected party this message is sent from
- ocpiToCountryCode string - Country code of the connected party this message is to be sent to
- ocpiToPartyId string - Party ID of the connected party this message is to be sent to
- countryCode string - Country code of the eMSP sending this PUT request to the CPO system. This shall be the same value as the country_code in the Token object being pushed.
- partyID string - Party ID (Provider ID) of the eMSP sending this PUT request to the CPO system. This shall be the same value as the party_id in the Token object being pushed.
- tokenUID string - Token.uid of the (new) Token object (to replace)
- payload Token - Token details
- ocnSignature string? (default ()) - OCN Signature
- 'type string (default "RFID") - Token.type of the Token of the (new) Token object (to replace). Default if omitted:RFID
Return Type
- OcpiResponseUnit|error - OK
patchClientOwnedToken
function patchClientOwnedToken(string authorization, string xRequestId, string xCorrelationId, string ocpiFromCountryCode, string ocpiFromPartyId, string ocpiToCountryCode, string ocpiToPartyId, string countryCode, string partyID, string tokenUID, json payload, string? ocnSignature, string 'type) returns OcpiResponseUnit|error
Notifies the CPO of partial updates to a Token
Parameters
- authorization string - Authorization token
- xRequestId string - Every request shall contain a unique request ID, the response to this request shall contain the same ID
- xCorrelationId string - Every request/response shall contain a unique correlation ID, every response to this request shall contain the same ID
- ocpiFromCountryCode string - Country code of the connected party this message is sent from
- ocpiFromPartyId string - Party ID of the connected party this message is sent from
- ocpiToCountryCode string - Country code of the connected party this message is to be sent to
- ocpiToPartyId string - Party ID of the connected party this message is to be sent to
- countryCode string - Country code of the eMSP sending this PATCH request to the CPO system
- partyID string - Party ID (Provider ID) of the eMSP sending this PATCH request to the CPO system
- tokenUID string - Token.uid of the (new) Token object (to replace)
- payload json - Request body details
- ocnSignature string? (default ()) - OCN Signature
- 'type string (default "RFID") - Token.type of the Token of the Token object
Return Type
- OcpiResponseUnit|error - OK
Records
ocpi: ActiveChargingProfile
The charging profile as calculated by the EVSE.
Fields
- start_date_time string - Date and time at which the Charge Point has calculated this ActiveChargingProfile. All time measurements within the profile are relative to this timestamp.
- charging_profile ChargingProfile - A list of charging periods
ocpi: AdditionalGeoLocation
An additional geo location that is relevant for the Charge Point. The geodetic system to be used is WGS 84.
Fields
- latitude string - Latitude of the point in decimal degree. Example- 50.770774. Decimal separator- "." Regex- -?[0-9]{1,2}.[0-9]{5,7}
- longitude string - Longitude of the point in decimal degree. Example- -126.104965. Decimal separator- "." Regex- -?[0-9]{1,3}.[0-9]{5,7}
- name DisplayText? - A List of multi-language alternative tariff info texts
ocpi: AuthorizationInfo
Contains information about the authorization, if the Token is allowed to charge and optionally which EVSEs are allowed to be used.
Fields
- allowed string - Status of the Token, and whether charging is allowed at the optionally given location
- token Token - Represents a token information
- location LocationReferences? - References to location details.
- authorization_reference string? - Reference to the authorization given by the eMSP. When the eMSP provided an authorization_reference in either- real-time authorization or StartSession, this field shall contain the same value. When different authorization_reference values havebeen given by the eMSP that are relevant to this Session, the lastgiven value shall be used here.
- info DisplayText? - A List of multi-language alternative tariff info texts
ocpi: BasicRole
OCPI basic role details
Fields
- party_id string - CPO, eMSP (or other role) ID of this party (following the ISO-15118standard)
- country_code string - ISO-3166 alpha-2 country code of the country this party is operating in
ocpi: BusinessDetails
Details of a party
Fields
- name string - Name of the operator
- website string? - Link to the operator’s website
- logo Image? - References an image related to an EVSE in terms of a file name or url.
ocpi: CancelReservation
The cancel of an existing Reservation
Fields
- response_url string - URL that the CommandResult POST should be send to. This URL might contain an unique ID to be able to distinguish between CancelReservation requests.
- reservation_id string - Reservation id, unique for this reservation. If the Charge Point already has a reservation that matches this reservationId the Charge Point will replace the reservation.
ocpi: CDR
Charge detail record
Fields
- country_code string - ISO-3166 alpha-2 country code of the CPO that 'owns' this CDR
- party_id string - CPO ID of the CPO that 'owns' this CDR (following the ISO-15118standard)
- id string - Uniquely identifies the CDR within the CPO’s platform (and sub operator platforms). This field is longer than the usual 36characters to allow for credit CDRs to have something appended to the original ID. Normal (non-credit) CDRs SHALL only have an ID with a maximum length of 36.
- start_date_time string - Start timestamp of the charging session, or in-case of a reservation(before the start of a session) the start of the reservation
- end_date_time string - The timestamp when the session was completed/finished, charging might have finished before the session ends, for example- EV is full,but parking cost also has to be paid
- session_id string? - Unique ID of the Session for which this CDR is sent. Is only allowed to be omitted when the CPO has not implemented the Sessions module or this CDR is the result of a reservation that never became a charging session, thus no OCPI Session
- cdr_token CdrToken - CDR Token details
- auth_method string - Method used for authentication
- authorization_reference string? - Reference to the authorization given by the eMSP. When the eMSP provided an authorization_reference in either- real-time authorization or StartSession, this field shall contain the same value. When different authorization_reference values have been given by the eMSP that are relevant to this Session, the last given value shall be used here.
- cdr_location CdrLocation - CDR location
- meter_id string? - Identification of the Meter inside the Charge Point
- currency string - Currency of the CDR in ISO 4217 Code
- charging_periods ChargingPeriod[] - A list of Charging Periods that make up this charging session. A session consists of 1 or more periods, where each period has a different relevant Tariff
- signed_data SignedData? - All the information of the signed data. Which encoding method is used, if needed, the public key and a list of signed values.
- total_cost Price - The total cost of the session in the specified currency
- total_fixed_cost Price? - The total cost of the session in the specified currency
- total_energy float - Total energy charged, in kWh
- total_energy_cost Price? - The total cost of the session in the specified currency
- total_time float - Total duration of the charging session (including the duration of charging and not charging), in hours
- total_time_cost Price? - The total cost of the session in the specified currency
- total_parking_time float? - Total duration of the charging session where the EV was not charging (no energy was transferred between EVSE and EV), in hours
- total_parking_cost Price? - The total cost of the session in the specified currency
- total_reservation_cost Price? - The total cost of the session in the specified currency
- remark string? - Optional remark, can be used to provide additional human readable information to the CDR, for example- reason why a transaction was stopped
- invoice_reference_id string? - This field can be used to reference an invoice, that will later be sent for this CDR. Making it easier to link a CDR to a given invoice.Maybe even group CDRs that will be on the same invoice
- credit boolean? - When set to true, this is a Credit CDR, and the field credit_reference_id needs to be set as well
- credit_reference_id string? - Whether it is required to be set for a Credit CDR. This shall contain the id of the CDR for which this is a Credit CDR
- last_updated string - Timestamp when this CDR was last updated (or created)
ocpi: CdrDimension
CDR dimension
Fields
- 'type string - Type of CDR dimension
- volume float - Volume of the dimension consumed, measured according to the dimension type
ocpi: CdrLocation
CDR location
Fields
- id string - Uniquely identifies the location within the CPO’s platform (and sub operator platforms). This field can never be changed, modified or renamed
- name string? - Display name of the location
- address string - Street/block name and house number if available
- city string - City or town
- postal_code string - Postal code of the location
- country string - ISO 3166-1 alpha-3 code for the country of this location
- coordinates GeoLocation - The geo location of the Charge Point. The geodetic system to be used is WGS 84.
- evse_uid string - Uniquely identifies the EVSE within the CPO’s platform (and sub operator platforms). For example a database unique ID or the actual EVSE ID. This field can never be changed, modified or renamed. This is the technical identification of the EVSE, not to be used as human readable identification, use the field:evse_id for that
- evse_id string - Compliant with the following specification for EVSE ID from "eMI3 standard version V1.0" (http://emi3group.com/documents-links/) "Part 2- business objects"
- connector_id string - Identifier of the connector within the EVSE
- connector_standard string - The standard of the installed connector
- connector_format string - The format (socket/cable) of the installed connector
- connector_power_type string - The power type of the connector
ocpi: CdrToken
CDR Token details
Fields
- uid string - Unique ID by which this Token can be identified.This is the field used by the CPO’s system (RFID reader on the Charge Point) to identify this token.Currently, in most cases- type=RFID, this is the RFID hidden ID as read by the RFID reader, but that is not a requirement. If this is a type=APP_USER Token, it will be a unique, by the eMSP, generatedID.
- 'type string - Type of the token
- contract_id string - Uniquely identifies the EV driver contract token within the eMSP’s platform (and sub operator platforms). Recommended to follow the specification for eMA ID from "eMI3 standard version V1.0" (http://emi3group.com/documents-links/)"Part 2- business objects."
ocpi: ChargingPeriod
Charging period details
Fields
- start_date_time string - Start timestamp of the charging period. A period ends when the next period starts. The last period ends when the session ends
- dimensions CdrDimension[] - A list of relevant values for this charging period
- tariff_id string? - Unique identifier of the Tariff that is relevant for this Charging Period. If not provided, no Tariff is relevant during this period
ocpi: ChargingPreferences
Contains the charging preferences of an EV driver
Fields
- profile_type string - Type of Smart Charging Profile selected by the driver. The ProfileType has to be supported at the Connector and for every supported ProfileType, a Tariff MUSTbe provided. This gives the EV driver the option between different pricing options.
- departure_time string? - Expected departure. The driver has given this Date/Time as expected departure moment. It is only an estimation and not necessarily the Date/Time of the actual departure.
- energy_need float? - Requested amount of energy in kWh. The EV driver wants to have this amountof energy charged
- discharge_allowed boolean? - The driver allows their EV to be discharged when needed, as long as the other preferences are met- EV is charged with the preferred energy (energy_need)until the preferred departure moment (departure_time). Default if omitted:false
ocpi: ChargingProfile
A list of charging periods
Fields
- start_date_time string? - Starting point of an absolute profile. If absent the profile will be relative to start of charging.
- duration int? - Duration of the charging profile in seconds. If the duration is left empty, the last period will continue in definitely or until end of the transaction in case startProfile is absent.
- charging_rate_unit string - The unit of measure
- min_charging_rate float? - Minimum charging rate supported by the EV. The unit of measure is defined by the chargingRateUnit. This parameter is intended to be used by a local smart charging algorithm to optimize the power allocation for in the case a charging process is inefficient at lower charging rates. Accepts at most one digit fraction.
- charging_profile_period ChargingProfilePeriod[]? - A list of ChargingProfilePeriod elements defining maximum power or current usage over time
ocpi: ChargingProfilePeriod
A time period in a charging profile, as used in ChargingProfile
Fields
- start_period int - Start of the period, in seconds from the start of profile. The value of StartPeriod also defines the stop time of the previous period.
- 'limit float - Charging rate limit during the profile period, in the applicable chargingRateUnit,for example in Amperes (A) or Watts (W). Accepts at most one digit fraction.
ocpi: ChargingProfileResponse
Response to the ChargingProfile request from the eMSP to the CPO
Fields
- result string - Response types to the ChargingProfile request from the eMSP to the CPO
- timeout int - timeout value
ocpi: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
ocpi: CommandResponse
The CommandResponse object is sent in the HTTP response body
Fields
- result string - Response from the CPO on the command request
- timeout int - Timeout for this command in seconds. When the Result is not received within this timeout, the eMSP can assume that the message might never be sent.
- message DisplayText? - A List of multi-language alternative tariff info texts
ocpi: CommandResult
Result of the command request, from the Charge Point
Fields
- result string - Result of the command request as sent by the Charge Point to the CPO.
- message DisplayText? - A List of multi-language alternative tariff info texts
ocpi: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
ocpi: Connector
The socket or cable and plug available for the EV to use. A single EVSE may provide multiple Connectors but onlyone of them can be in use at the same time. A Connector always belongs to an EVSE object.
Fields
- id string - Identifier of the Connector within the EVSE. Two Connectors may have the same id as long as they do not belong to the same EVSE object
- standard string - The standard of the installed connector
- format string - The format (socket/cable) of the installed connector
- power_type string - Power type
- max_voltage int - Maximum voltage of the connector (line to neutral for AC_3_PHASE), in volt [V]. For example- DC Chargers might vary the voltage during charging when battery almost full
- max_amperage int - Maximum amperage of the connector, in ampere [A]
- max_electric_power int? - Maximum electric power that can be delivered by this connector, inWatts (W)
- tariff_ids string[]? - Identifiers of the currently valid charging tariffs. Multiple tariffs are possible, but only one of each Tariff.type can be active at the same time.Tariffs with the same type are only allowed if they are not active at the same time- start_date_time and end_date_time period not overlapping.When preference-based smart charging is supported, one tariff forevery possible ProfileType should be provided. These tell the user aboutthe options they have at this Connector, and what the tariff is for every option.For a "free of charge" tariff, this field should be set and point to a defined "free of charge" tariff.
- terms_and_conditions string? - URL to the operator’s terms and conditions
- last_updated string - Timestamp when this Connector was last updated (or created)
ocpi: Credentials
Credentials detail
Fields
- token string - Case Sensitive, ASCII only. The credentials token for the other party to authenticate in your system. Not encoded in Base64 or any other encoding.
- url string - The URL to your API versions endpoint
- roles CredentialsRole[] - List of the roles this party provides
ocpi: CredentialsRole
A list of the roles this party provides.
Fields
- role string - Type of role
- business_details BusinessDetails - Details of a party
- party_id string - CPO, eMSP (or other role) ID of this party (following the ISO-15118standard)
- country_code string - ISO-3166 alpha-2 country code of the country this party is operating in
ocpi: DisplayText
A List of multi-language alternative tariff info texts
Fields
- language string - A language Code ISO 639-1
- text string - Text to be displayed to a end user. No markup, html etc. allowed
ocpi: Endpoint
OCPI module endpoint details
Fields
- identifier string - Endpoint identifier
- role string - Interface role this endpoint implements
- url string - URL to the endpoint
ocpi: EnergyContract
Information about a energy contract that belongs to a Token so a driver could use his/her own energy contract when charging at a Charge Point
Fields
- supplier_name string - Name of the energy supplier for this token
- contract_id string? - Contract ID at the energy supplier, that belongs to the owner of this token
ocpi: EnergyMix
Details on the energy supplied at this location
Fields
- is_green_energy boolean - True if 100% from regenerative sources. (CO2 and nuclear waste is zero)
- energy_sources EnergySource[]? - Key-value pairs (enum + percentage) of energy sources of this location’s tariff
- environ_impact EnvironmentalImpact[]? - Key-value pairs (enum + percentage) of nuclear waste and CO2exhaust of this location’s tariff
- supplier_name string? - Name of the energy supplier, delivering the energy for this location or tariff.*
- energy_product_name string? - Name of the energy suppliers product/tariff plan used at this location.*
ocpi: EnergySource
Key-value pairs (enum + percentage) of energy sources
Fields
- 'source string - The type of energy source
- percentage float - Percentage of this source (0-100) in the mix
ocpi: EnvironmentalImpact
Amount of waste produced/emitted per kWh.
Fields
- category string - The environmental impact category of this value
- amount float - Amount of this portion in g/kWh
ocpi: Evse
The part that controls the power supply to a single EV in a single session
Fields
- uid string - Uniquely identifies the EVSE within the CPO's platform (and sub operator platforms)
- evse_id string? - Compliant with the following specification for EVSE ID from "eMI3 standard version V1.0" (http://emi3group.com/documents-links/) "Part 2:business objects." Optional because- if an evse_id is to be re-used in the real world, the evse_id can be removed from an EVSE object if the status is set to REMOVED.
- status string - Indicates the current status of the EVSE
- status_schedule StatusSchedule[]? - Indicates a planned status update of the EVSE
- capabilities string[]? - List of functionalities that the EVSE is capable of
- connectors Connector[] - List of available connectors on the EVSE
- floor_level string? - Level on which the Charge Point is located (in garage buildings) in the locally displayed numbering scheme.
- coordinates GeoLocation? - The geo location of the Charge Point. The geodetic system to be used is WGS 84.
- physical_reference string? - A number/string printed on the outside of the EVSE for visual identification.
- directions DisplayText[]? - Multi-language human-readable directions when more detailed information on how to reach the EVSE from the Location is required.
- parking_restrictions string[]? - The restrictions that apply to the parking spot.
- images Image[]? - Links to images related to the EVSE such as photos or logos
- last_updated string - Timestamp when this EVSE or one of its Connectors was last updated(or created)
ocpi: ExceptionalPeriod
Specifies one exceptional period for opening or access hour
Fields
- period_begin string - Begin of the exception. In UTC, time_zone field can be used to convert to local time.
- period_end string - End of the exception. In UTC, time_zone field can be used to convert to local time.
ocpi: GenericChargingProfileResult
Result of a ChargingProfile request that the EVSE sends via the CPO to the eMSP
Fields
- result string - charging profile request results
- profile ActiveChargingProfile? - The charging profile as calculated by the EVSE.
ocpi: GeoLocation
The geo location of the Charge Point. The geodetic system to be used is WGS 84.
Fields
- latitude string - Latitude of the point in decimal degree. Example- 50.770774. Decimal separator- "." Regex- -?[0-9]{1,2}.[0-9]{5,7}
- longitude string - Longitude of the point in decimal degree. Example- -126.104965. Decimal separator- "." Regex- -?[0-9]{1,3}.[0-9]{5,7}
ocpi: Hours
Opening and access hours of the location
Fields
- twentyfourseven boolean - True to represent 24 hours a day and 7 days a week, except the givenexceptions.
- regular_hours RegularHours? - Regular recurring operation or access hours
- exceptional_openings ExceptionalPeriod? - Specifies one exceptional period for opening or access hour
- exceptional_closings ExceptionalPeriod? - Specifies one exceptional period for opening or access hour
ocpi: Image
References an image related to an EVSE in terms of a file name or url.
Fields
- url string - URL from where the image data can be fetched through a web browser
- thumbnail string? - URL from where a thumbnail of the image can be fetched through a web browser
- category string - Describes what the image is used for
- 'type string - Image type like- gif, jpeg, png, svg
- width int? - Width of the full scale image
- height int? - Height of the full scale image
ocpi: Location
The location and its properties where a group of EVSEs that belong together are installed
Fields
- country_code string - ISO-3166 alpha-2 country code of the CPO that 'owns' thisLocation.
- party_id string - CPO ID of the CPO that 'owns' this Location (following the ISO-15118 standard)
- id string - Uniquely identifies the location within the CPO's platform (and sub operator platforms). This field can never be changed,modified or renamed
- publish boolean - Defines if a Location may be published on an website or app etc
- publish_allowed_to PublishTokenType[]? - This field may only be used when the publish field is set to false Only owners of Tokens that match all the set fields of one Publish Token in the list are allowed to be shown this location
- name string? - Display name of the location
- address string - Street/block name and house number if available
- city string - City or town
- postal_code string? - Postal code of the location, may only be omitted when the location has no postal code- in some countries charging locations at highways don’t have postal codes
- state string? - State or province of the location, only to be used when relevant
- country string - ISO 3166-1 alpha-3 code for the country of this location
- coordinates GeoLocation - The geo location of the Charge Point. The geodetic system to be used is WGS 84.
- related_locations AdditionalGeoLocation[]? - Geographical location of related points relevant to the user
- parking_type string? - The general type of parking at the charge point location
- evses Evse[]? - List of EVSEs that belong to this Location
- directions DisplayText[]? - Human-readable directions on how to reach the location
- operator BusinessDetails? - Details of a party
- suboperator BusinessDetails? - Details of a party
- owner BusinessDetails? - Details of a party
- facilities string[]? - Optional list of facilities this charging location directly belongsto.
- time_zone string? - One of IANA tzdata’s TZ-values representing the time zone of the location. Examples- "Europe/Oslo", "Europe/Zurich".(http://www.iana.org/time-zones)
- opening_times Hours? - Opening and access hours of the location
- charging_when_closed string? - Indicates if the EVSEs are still charging outside the opening hours of the location. E.g. when the parking garage closes its barriers over night, is it allowed to charge till the next morning? Default- true
- images Image[]? - Links to images related to the location such as photos or logos
- energy_mix EnergyMix? - Details on the energy supplied at this location
- last_updated string - Timestamp when this Location or one of its EVSEs orConnectors were last updated (or created)
ocpi: LocationReferences
References to location details.
Fields
- location_id string - Unique identifier for the location
- evse_uids string[]? - Unique identifiers for EVSEs within the CPO’s platform for the EVSE within the given location
- connector_ids string[]? - Connector IDs
ocpi: OcnRules
OCN rules
Fields
- signatures boolean - Signatures
- whitelist OcnRulesList - OCN Rules List
- blacklist OcnRulesList - OCN Rules List
ocpi: OcnRulesList
OCN Rules List
Fields
- active boolean - Indicates the activations
- list OcnRulesListParty[] - An array of OCN rules list parties
ocpi: OcnRulesListParty
OCN rules list party
Fields
- party_id string - Party ID
- country_code string - Country ID
- modules string[] - an array of Modules
ocpi: OcpiResponse
OCPI response
Fields
- status_code int - OCPI status code, as listed in Status Codes, indicates how the request was handled. To avoid confusion with HTTP codes, OCPI status codes consist of four digits.
- status_message string? - An optional status message which may help when debugging
- data record {}? - Response data
- timestamp string - The time this message was generated
- ocn_signature string? - OCN signature
ocpi: OcpiResponseAuthorizationInfo
OCPI response - Authorization Information
Fields
- status_code int - OCPI status code, as listed in Status Codes, indicates how the request was handled. To avoid confusion with HTTP codes, OCPI status codes consist of four digits.
- status_message string? - An optional status message which may help when debugging
- data AuthorizationInfo? - Contains information about the authorization, if the Token is allowed to charge and optionally which EVSEs are allowed to be used.
- timestamp string - The time this message was generated
- ocn_signature string? - OCN signature
ocpi: OcpiResponseCDR
OCPI response - CDR
Fields
- status_code int - OCPI status code, as listed in Status Codes, indicates how the request was handled. To avoid confusion with HTTP codes, OCPI status codes consist of four digits.
- status_message string? - An optional status message which may help when debugging
- data CDR? - Charge detail record
- timestamp string - The time this message was generated
- ocn_signature string? - OCN signature
ocpi: OcpiResponseCDRList
OCPI response CDR List
Fields
- status_code int - OCPI status code, as listed in Status Codes, indicates how the request was handled. To avoid confusion with HTTP codes, OCPI status codes consist of four digits.
- status_message string? - An optional status message which may help when debugging
- data CDR[]? - An array of CDRs
- timestamp string - The time this message was generated
- ocn_signature string? - OCN signature
ocpi: OcpiResponseChargingPreferencesResponse
OCPI response - Charging preference reponse
Fields
- status_code int - OCPI status code, as listed in Status Codes, indicates how the request was handled. To avoid confusion with HTTP codes, OCPI status codes consist of four digits.
- status_message string? - An optional status message which may help when debugging
- data string? - Charging preference response data
- timestamp string - The time this message was generated
- ocn_signature string? - OCN signature
ocpi: OcpiResponseChargingProfileResponse
OCPI response charging profile response
Fields
- status_code int - OCPI status code, as listed in Status Codes, indicates how the request was handled. To avoid confusion with HTTP codes, OCPI status codes consist of four digits.
- status_message string? - An optional status message which may help when debugging
- data ChargingProfileResponse? - Response to the ChargingProfile request from the eMSP to the CPO
- timestamp string - The time this message was generated
- ocn_signature string? - OCN signature
ocpi: OcpiResponseCommandResponse
OCPI response - command response
Fields
- status_code int - OCPI status code, as listed in Status Codes, indicates how the request was handled. To avoid confusion with HTTP codes, OCPI status codes consist of four digits.
- status_message string? - An optional status message which may help when debugging
- data CommandResponse? - The CommandResponse object is sent in the HTTP response body
- timestamp string - The time this message was generated
- ocn_signature string? - OCN signature
ocpi: OcpiResponseConnector
OCPI response - Connector
Fields
- status_code int - OCPI status code, as listed in Status Codes, indicates how the request was handled. To avoid confusion with HTTP codes, OCPI status codes consist of four digits.
- status_message string? - An optional status message which may help when debugging
- data Connector? - The socket or cable and plug available for the EV to use. A single EVSE may provide multiple Connectors but onlyone of them can be in use at the same time. A Connector always belongs to an EVSE object.
- timestamp string - The time this message was generated
- ocn_signature string? - OCN signature
ocpi: OcpiResponseCredentials
OCPI response credentials detail
Fields
- status_code int - OCPI status code, as listed in Status Codes, indicates how the request was handled. To avoid confusion with HTTP codes, OCPI status codes consist of four digits.
- status_message string? - An optional status message which may help when debugging
- data Credentials? - Credentials detail
- timestamp string - The time this message was generated
- ocn_signature string? - OCN signature
ocpi: OcpiResponseEvse
OCPI response - EVSE
Fields
- status_code int - OCPI status code
- status_message string? - An optional status message which may help when debugging
- data Evse? - The part that controls the power supply to a single EV in a single session
- timestamp string - The time this message was generated
- ocn_signature string? - OCN signature
ocpi: OcpiResponseListVersion
Fields
- status_code int - OCPI status code, as listed in Status Codes, indicates how the request was handled. To avoid confusion with HTTP codes, OCPI status codes consist of four digits.
- status_message string? - An optional status message which may help when debugging
- data Version[]? -
- timestamp string - The time this message was generated
- ocn_signature string? -
ocpi: OcpiResponseLocation
OCPI response - 'Location'
Fields
- status_code int - OCPI status code, as listed in Status Codes, indicates how the request was handled. To avoid confusion with HTTP codes, OCPI status codes consist of four digits.
- status_message string? - An optional status message which may help when debugging
- data Location? - The location and its properties where a group of EVSEs that belong together are installed
- timestamp string - The time this message was generated
- ocn_signature string? - OCN signature
ocpi: OcpiResponseLocationList
OCPI response - Location list
Fields
- status_code int - OCPI status code, as listed in Status Codes, indicates how the request was handled. To avoid confusion with HTTP codes, OCPI status codes consist of four digits.
- status_message string? - An optional status message which may help when debugging
- data Location[]? - An array of locations
- timestamp string - The time this message was generated
- ocn_signature string? - OCN signature
ocpi: OcpiResponseObject
OCPI response object
Fields
- status_code int - OCPI status code, as listed in Status Codes, indicates how the request was handled. To avoid confusion with HTTP codes, OCPI status codes consist off our digits.
- status_message string? - An optional status message which may help when debugging
- data record {}? - Contains the actual response data object or list of objects from each request,depending on the cardinality of the response data, this is an array (card. * or +),or a single object (card. 1 or ?)
- timestamp string - The time this message was generated
- ocn_signature string? - OCN signature
ocpi: OcpiResponseOcnRules
OCPI response - OCN Rules
Fields
- status_code int - OCPI status code, as listed in Status Codes, indicates how the request was handled. To avoid confusion with HTTP codes, OCPI status codes consist of four digits.
- status_message string? - An optional status message which may help when debugging
- data OcnRules? - OCN rules
- timestamp string - The time this message was generated
- ocn_signature string? - OCN signature
ocpi: OcpiResponseSession
OCPI response - Session
Fields
- status_code int - OCPI status code, as listed in Status Codes, indicates how the request was handled. To avoid confusion with HTTP codes, OCPI status codes consist of four digits.
- status_message string? - An optional status message which may help when debugging
- data Session? - Describes one charging session
- timestamp string - The time this message was generated
- ocn_signature string? - OCN signature
ocpi: OcpiResponseSessionList
OCPI response - Session List
Fields
- status_code int - OCPI status code, as listed in Status Codes, indicates how the request was handled. To avoid confusion with HTTP codes, OCPI status codes consist of four digits.
- status_message string? - An optional status message which may help when debugging
- data Session[]? - An array of sessions
- timestamp string - The time this message was generated
- ocn_signature string? - OCN signature
ocpi: OcpiResponseTariff
OCPI response - Tariff
Fields
- status_code int - OCPI status code, as listed in Status Codes, indicates how the request was handled. To avoid confusion with HTTP codes, OCPI status codes consist of four digits.
- status_message string? - An optional status message which may help when debugging
- data Tariff? - Consists of a list of one or more Tariff Elements, which can be used to create complex Tariff structures.
- timestamp string - The time this message was generated
- ocn_signature string? - OCN signature
ocpi: OcpiResponseTariffList
OPCI response - Tariff list
Fields
- status_code int - OCPI status code, as listed in Status Codes, indicates how the request was handled. To avoid confusion with HTTP codes, OCPI status codes consist of four digits.
- status_message string? - An optional status message which may help when debugging
- data Tariff[]? - An array of tariffs
- timestamp string - The time this message was generated
- ocn_signature string? - OCN signature
ocpi: OcpiResponseToken
OCPI response - Token
Fields
- status_code int - OCPI status code, as listed in Status Codes, indicates how the request was handled. To avoid confusion with HTTP codes, OCPI status codes consist of four digits.
- status_message string? - An optional status message which may help when debugging
- data Token? - Represents a token information
- timestamp string - The time this message was generated
- ocn_signature string? - OCN signature
ocpi: OcpiResponseTokenList
OCPI response - Token List
Fields
- status_code int - OCPI status code, as listed in Status Codes, indicates how the request was handled. To avoid confusion with HTTP codes, OCPI status codes consist of four digits.
- status_message string? - An optional status message which may help when debugging
- data Token[]? - An array of tokens
- timestamp string - The time this message was generated
- ocn_signature string? - OCN signature
ocpi: OcpiResponseUnit
OCPI response - Unit
Fields
- status_code int - OCPI status code, as listed in Status Codes, indicates how the request was handled. To avoid confusion with HTTP codes, OCPI status codes consist of four digits.
- status_message string? - An optional status message which may help when debugging
- data Unit? - Unit
- timestamp string - The time this message was generated
- ocn_signature string? - OCN signature
ocpi: OcpiResponseVersionDetail
OCPI response version details
Fields
- status_code int - OCPI status code, as listed in Status Codes, indicates how the request was handled. To avoid confusion with HTTP codes, OCPI status codes consist of four digits.
- status_message string? - An optional status message which may help when debugging
- data VersionDetail? - Version details
- timestamp string - The time this message was generated
- ocn_signature string? - OCN Signature
ocpi: Price
The total cost of the session in the specified currency
Fields
- excl_vat float - Price/Cost excluding VAT
- incl_vat float - Price/Cost including VAT
ocpi: PriceComponent
Describes the pricing of a tariff
Fields
- 'type string - Type of tariff dimension
- price float - Price per unit (excl. VAT) for this tariff dimension
- vat float? - Applicable VAT percentage for this tariff dimension. If omitted, no VATis applicable. Not providing a VAT is different from 0% VAT, which would be a value of 0.0 here.
- step_size int - Minimum amount to be billed. This unit will be billed in this step_size blocks. Amounts that are less then this step_size are rounded up to the given step_size. For example- if type is TIME and step_size has a value of 300, then time will be billed in blocks of 5 minutes. If 6 minutes were used, 10 minutes (2 blocks of step_size) will be billed.
ocpi: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
ocpi: PublishTokenType
Defines the set of values that identify a token to which a Location might be published
Fields
- uid string? - Unique ID by which this Token can be identified
- 'type string? - Type of the token
- visual_number string? - Visual readable number/identification as printed on the Token (RFID card)
- issuer string? - Issuing company, most of the times the name of the company printed on the token (RFID card), not necessarily the eMSP
- group_id string? - This ID groups a couple of tokens. This can be used to make two or more tokens work as one.
ocpi: RegularHours
Regular recurring operation or access hours
Fields
- weekday int - Number of day in the week, from Monday (1) till Sunday (7)
- period_begin string - Begin of the regular period, in local time, given in hours and minutes. Must be in24h format with leading zeros. Examples- "18:15". Hour/Minute separator- ":"Regex- ([0-1][0-9]|2[0-3]):[0-5][0-9].
- period_end string - End of the regular period, in local time, syntax as for period_begin. Must be later than period_begin.
ocpi: ReserveNow
The RESERVE_NOW command, with information needed to reserve a (specific) connector of a Charge Point for a given Token
Fields
- response_url string - URL that the CommandResult POST should be send to. This URL might contain an unique ID to be able to distinguish between ReserveNow requests.
- token Token - Represents a token information
- expiry_date string - The Date/Time when this reservation ends, in UTC
- reservation_id string - Reservation id, unique for this reservation. If the Receiver (typicallyCPO) Point already has a reservation that matches this reservationId for that Location it will replace the reservation.
- location_id string - Location.id of the Location (belonging to the CPO this request is send to) for which to reserve an EVSE
- evse_uid string? - Optional EVSE.uid of the EVSE of this Location if a specific EVSE has to be reserved.
- authorization_reference string? - Reference to the authorization given by the eMSP, when given, this reference will be provided in the relevant Session and/or CDR
ocpi: Session
Describes one charging session
Fields
- country_code string - ISO-3166 alpha-2 country code of the CPO that 'owns' thisSession
- party_id string - CPO ID of the CPO that 'owns' this Session (following the ISO-15118 standard)
- id string - The unique id that identifies the charging session in the CPO platform
- start_date_time string - The timestamp when the session became ACTIVE in the ChargePoint.When the session is still PENDING, this field shall be set to the time the Session was created at the Charge Point. When a Session goes from PENDING to ACTIVE, this field SHALL be update to the moment the Session went to ACTIVE in the Charge Point.
- end_date_time string? - The timestamp when the session was completed/finished, charging might have finished before the session ends, for example- EV is full,but parking cost also has to be paid.
- kwh float - How many kWh were charged
- cdr_token CdrToken - CDR Token details
- auth_method string - Method used for authentication
- authorization_reference string? - Reference to the authorization given by the eMSP. When the eMSP provided an authorization_reference in either- real-time authorization or StartSession, this field SHALL contain the samevalue. When different authorization_reference values have been given by the eMSP that are relevant to this Session, the last given value SHALL be used here.
- location_id string - Location.id of the Location object of this CPO, on which the charging session is/was happening.
- evse_uid string - Location.id of the Location object of this CPO, on which the charging session is/was happening.
- connector_id string - Connector.id of the Connector of this Location the charging session is/was happening.
- meter_id string? - Optional identification of the kWh meter.
- currency string - ISO 4217 code of the currency used for this session
- charging_periods ChargingPeriod[]? - An optional list of Charging Periods that can be used to calculateand verify the total cost.
- total_cost Price? - The total cost of the session in the specified currency
- status string - The status of the session
- last_updated string - Timestamp when this Session was last updated (or created)
ocpi: SetChargingProfile
Object set to a CPO to set a Charging Profile
Fields
- charging_profile ChargingProfile - A list of charging periods
- response_url string - URL that the ChargingProfileResult POST should be send to. ThisURL might contain an unique ID to be able to distinguish between GET ActiveChargingProfile requests.
ocpi: SignedData
All the information of the signed data. Which encoding method is used, if needed, the public key and a list of signed values.
Fields
- encoding_method string - The name of the encoding used in the SignedData field. This is the name given to the encoding by a company or group of companies.
- encoding_method_version int? - Version of the EncodingMethod (when applicable)
- public_key string? - Public key used to sign the data, base64 encoded
- signed_values SignedValue[] - One or more signed values
- url string - URL that can be shown to an EV driver. This URL gives the EV driver the possibility to check the signed data from a charging session
ocpi: SignedValue
This class contains the signed and the plain/unsigned data. By decoding the data, the receiver can check if the content has not been altered.
Fields
- nature string - Nature of the value, in other words, the event this value belongs to.Possible values at moment of writing:- Start (value at the start of the Session)- End (signed value at the end of the Session)- Intermediate (signed values take during the Session, after Start, before End)Others might be added later.
- plain_data string - The unencoded string of data. The format of the content depends on theEncodingMethod field.
- signed_data string - Blob of signed data, base64 encoded. The format of the content depends on theEncodingMethod field.
ocpi: StartSession
StartSession object, for the START_SESSION command, with information needed to start a sessions
Fields
- response_url string - URL that the CommandResult POST should be sent to. This URL might contain an unique ID to be able to distinguish between StartSession requests.
- token Token - Represents a token information
- location_id string - Location.id of the Location (belonging to the CPO this request is send to) on which a session is to be started
- evse_uid string? - Optional EVSE.uid of the EVSE of this Location on which a session is to be started.
- authorization_reference string? - Reference to the authorization given by the eMSP, when given, this reference will be provided in the relevant Session and/or CDR.
ocpi: StatusSchedule
This type is used to schedule status periods in the future. The eMSP can provide this information to the EV user for trip planning purposes. A period MAY have no end. Example- "This station will be running as of tomorrow. Today it is still planned and under construction.
Fields
- period_begin string - Begin of the scheduled period
- period_end string? - End of the scheduled period, if known
- status string - Status value during the scheduled period
ocpi: StopSession
StopSession object, for the STOP_SESSION command, with information needed to stop a sessions.
Fields
- response_url string - URL that the CommandResult POST should be sent to. This URL might contain an unique ID to be able to distinguish between StopSession requests.
- session_id string - Session.id of the Session that is requested to be stopped
ocpi: Tariff
Consists of a list of one or more Tariff Elements, which can be used to create complex Tariff structures.
Fields
- country_code string - ISO-3166 alpha-2 country code of the CPO that owns this Tariff
- party_id string - CPO ID of the CPO that owns this Tariff (following the ISO-15118 standard)
- id string - Uniquely identifies the tariff within the CPO’s platform (and sub operator platforms)
- currency string - ISO-4217 code of the currency of this tariff
- 'type string? - Defines the type of the tariff. This allows for distinction in case of given ChargingPreferences. When omitted, this tariff is valid for all sessions.
- tariff_alt_text DisplayText[]? - A list of multi-language alternative tariff info texts
- tariff_alt_url string? - URL to a web page that contains an explanation of the tariff information inhuman readable form
- min_price Price? - The total cost of the session in the specified currency
- max_price Price? - The total cost of the session in the specified currency
- elements TariffElement[] - An array of tariff elements
- start_date_time string? - The time when this tariff becomes active, in UTC, time_zone field of theLocation can be used to convert to local time. Typically used for a new tariff that is already given with the location, before it becomes active.
- end_date_time string? - The time after which this tariff is no longer valid, in UTC, time_zone field if theLocation can be used to convert to local time. Typically used when this tariff is going to be replaced with a different tariff in the near future.
- energy_mix EnergyMix? - Details on the energy supplied at this location
- last_updated string - Timestamp when this Tariff was last updated (or created)
ocpi: TariffElement
OCPI Tariff element
Fields
- price_components PriceComponent[] - List of price components that describe the pricing of a tariff
- restrictions TariffRestrictions? - These restrictions are not for the entire Charging Session. They only describe if and when a TariffElement becomes active or inactive during a Charging Session.
ocpi: TariffRestrictions
These restrictions are not for the entire Charging Session. They only describe if and when a TariffElement becomes active or inactive during a Charging Session.
Fields
- start_time string? - Start time of day in local time, the time zone is defined in the time_zone field of the Location, for example 13:30, valid from this time of the day. Must be in 24h format with leading zeros. Hour/Minute separator:- ":" Regex- ([0-1][0-9]|2[0-3]):[0-5][0-9]
- end_time string? - End time of day in local time, the time zone is defined in the time_zone field of the Location, for example 19:45, valid until this time of the day. Same syntax asstart_time. If end_time < start_time then the period wraps around to the next day. To stop at end of the day use- 00:00
- start_date string? - Start date in local time, the time zone is defined in the time_zone field of theLocation, for example- 2015-12-24, valid from this day (inclusive). Regex-([12][0-9]{3})-(0[1-9]|1[0-2])-(0[1-9]|[12][0-9]|3[01])
- end_date string? - End date in local time, the time zone is defined in the time_zone field of theLocation, for example- 2015-12-27, valid until this day (exclusive). Same syntax as start_date.
- min_kwh float? - Minimum consumed energy in kWh, for example 20, valid from this amount of energy (inclusive) being used.
- max_kwh float? - Maximum consumed energy in kWh, for example 50, valid until this amount of energy (exclusive) being used
- min_current float? - Sum of the minimum current (in Amperes) over all phases, for example 5. When the EV is charging with more than, or equal to, the defined amount of current,this TariffElement is/becomes active. If the charging current is or becomes lower,this TariffElement is not or no longer valid and becomes inactive. This describesNOT the minimum current over the entire Charging Session. This restriction can make a TariffElement become active when the charging current is above the defined value, but the TariffElement MUST no longer be active when the charging current drops below the defined value.
- max_current float? - Sum of the maximum current (in Amperes) over all phases, for example 20.When the EV is charging with less than the defined amount of current, thisTariffElement becomes/is active. If the charging current is or becomes higher,this TariffElement is not or no longer valid and becomes inactive. This describesNOT the maximum current over the entire Charging Session. This restriction can make a TariffElement become active when the charging current is below this value, but the TariffElement MUST no longer be active when the charging current raises above the defined value.
- min_power float? - Minimum power in kW, for example 5. When the EV is charging with more than,or equal to, the defined amount of power, this TariffElement is/becomes active. Ifthe charging power is or becomes lower, this TariffElement is not or no longer valid and becomes inactive. This describes NOT the minimum power over the entire Charging Session. This restriction can make a TariffElement become active when the charging power is above this value, but the TariffElement MUSTno longer be active when the charging power drops below the defined value.
- max_power float? - Maximum power in kW, for example 20. When the EV is charging with less than the defined amount of power, this TariffElement becomes/is active. If the charging power is or becomes higher, this TariffElement is not or no longer valid and becomes inactive. This describes NOT the maximum power over the entireCharging Session. This restriction can make a TariffElement become active when the charging power is below this value, but the TariffElement MUST no longer be active when the charging power raises above the defined value.
- min_duration int? - Minimum duration in seconds the Charging Session MUST last (inclusive).When the duration of a Charging Session is longer than the defined value, thisTariffElement is or becomes active. Before that moment, this TariffElement is not yet active.
- max_duration int? - Maximum duration in seconds the Charging Session MUST last (exclusive).When the duration of a Charging Session is shorter than the defined value, thisTariffElement is or becomes active. After that moment, this TariffElement is no longer active.
- day_of_week string[]? - Which day(s) of the week this TariffElement is active
- reservation string? - When this field is present, the TariffElement describes reservation costs. A reservation starts when the reservation is made, and ends when the driverstarts charging on the reserved EVSE/Location, or when the reservationexpires. A reservation can only have- FLAT and TIME TariffDimensions, whereTIME is for the duration of the reservation.
ocpi: Token
Represents a token information
Fields
- country_code string - ISO-3166 alpha-2 country code of the MSP that 'owns' this Token
- party_id string - CPO ID of the MSP that 'owns' this Token (following the ISO-15118 standard)
- uid string - Unique ID by which this Token can be identified. This is the field used by CPO system (RFID reader on the Charge Point) to identify this token.Currently, in most cases- type=RFID, this is the RFID hidden ID as read by theRFID reader, but that is not a requirement.If this is a APP_USER or AD_HOC_USER Token, it will be a uniquely, by the eMSP,generated ID.This field is named uid instead of id to prevent confusion with- contract_id.
- 'type string - Type of the token
- contract_id string - Uniquely identifies the EV Driver contract token within the eMSP’s platform (and sub operator platforms). Recommended to follow the specification for eMA IDfrom "eMI3 standard version V1.0" (http://emi3group.com/documents-links/)"Part 2- business objects."
- visual_number string? - Visual readable number/identification as printed on the Token (RFID card), might be equal to the contract_id.
- issuer string - Issuing company, most of the times the name of the company printed on the token (RFID card), not necessarily the eMSP.
- group_id string? - This ID groups a couple of tokens. This can be used to make two or more tokens work as one, so that a session can be started with one token andstopped with another, handy when a card and key-fob are given to the EV-driver.Beware that OCPP 1.5/1.6 only support group_ids (it is called parentId in OCPP 1.5/1.6) with a maximum length of 20
- valid boolean - Indicates whether this Token valid is or not.
- whitelist string - Indicates what type of white-listing is allowed
- language string? - Language Code ISO 639-1. This optional field indicates the Token owner’s preferred interface language. If the language is not provided or not supported then the CPO is free to choose its own language.
- default_profile_type string? - The default Charging Preference. When this is provided, and a charging session is started on an Charge Point that support Preference base Smart Charging and support this ProfileType, the Charge Point can start using this ProfileType,without this having to be set via- Set Charging Preferences
- energy_contract EnergyContract? - Information about a energy contract that belongs to a Token so a driver could use his/her own energy contract when charging at a Charge Point
- last_updated string - Timestamp when this Token was last updated (or created)
ocpi: UnlockConnector
UnlockConnector object, for the UNLOCK_CONNECTOR command, with information needed to unlock a connector of a Charge Point.
Fields
- response_url string - URL that the CommandResult POST should be sent to. This URL might contain an unique ID to be able to distinguish between UnlockConnector requests.
- location_id string - Location.id of the Location (belonging to the CPO this request is send to) of which it is requested to unlock the connector
- evse_uid string - EVSE.uid of the EVSE of this Location of which it is requested to unlock the connector
- connector_id string - Connector.id of the Connector of this Location of which it is requested to unlock
ocpi: Version
A list of supported OCPI versions
Fields
- 'version string - The version number
- url string - URL to the endpoint containing version specific information
ocpi: VersionDetail
Version details
Fields
- 'version string - The version number
- endpoints Endpoint[] - An array of supported endpoints for this version
String types
ocpi: Unit
Unit
Unit
Import
import ballerinax/ocpi;
Metadata
Released date: over 1 year ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 0
Current verison: 0
Weekly downloads
Keywords
IT Operations/Cloud Services
Cost/Free
Contributors
Dependencies