nytimes.books
Module nytimes.books
API
Definitions
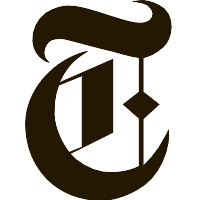
ballerinax/nytimes.books Ballerina library
Overview
This is a generated connector for New York Times Books API v3.0.0 OpenAPI specification.
The Books API provides information about book reviews and The New York Times bestsellers lists. For additional help getting started with the API, visit New York Times Books API.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create NYTimes developer account
- Obtain tokens
- Log into NYTimes Developer Portal by visiting https://developer.nytimes.com/accounts/login
- Register an app and obtain the API Key following the process summarized here.
Quickstart
To use the New York Times Books connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
Import the ballerinax/nytimes.books module into the Ballerina project.
import ballerinax/nytimes.books as books;
Step 2: Create a new connector instance
You can initialize the client as follows. You can now provide the API key obtained from the NYTimes Developer Portal in the configuration.
books:ApiKeysConfig config = { apiKey: "<API_KEY>" } books:Client baseClient = check new Client(config);
Step 3: Invoke connector operation
- You can use this function to get list of all the New York Times Best Sellers Lists. Some lists are published weekly and others monthly. The response includes when each list was first published and last published.
books:InlineResponse2002 listsBestSellersHistoryJson = check baseClient->getListsFormat("json"); if (listsBestSellersHistoryJson is books:InlineResponse2002) { books:InlineResponse2002Results[]? Results = listsBestSellersHistoryJson?.results; if !(Results is ()) { foreach var result in Results { log:printInfo(result.toString() + "\n"); } } }
- Use
bal run
command to compile and run the Ballerina program
Clients
nytimes.books: Client
This is a generated connector for New York Times Books API v3.0.0 OpenAPI specification. The Books API provides information about book reviews and The New York Times bestsellers lists. For additional help getting started with the API, visit New York Times Books API.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create an New York Times Account and obtain tokens following this guide.
init (ApiKeysConfig apiKeyConfig, ConnectionConfig config, string serviceUrl)
- apiKeyConfig ApiKeysConfig - API keys for authorization
- config ConnectionConfig {} - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.nytimes.com/svc/books/v3" - URL of the target service
getListsFormat
function getListsFormat(string format, string? list, int? weeksOnList, string? bestsellersDate, string? date, string? isbn, string? publishedDate, int? rank, int? rankLastWeek, int? offset, string? sortOrder) returns InlineResponse200|error
Best Seller List
Parameters
- format string - Type format
- list string? (default ()) - The name of the Times best-seller list. To get valid values, use a list names request. Be sure to replace spaces with hyphens (e.g., e-book-fiction or hardcover-fiction, not E-Book Fiction or Hardcover Fiction). (The parameter is not case sensitive.)
- weeksOnList int? (default ()) - The number of weeks that the best seller has been on list-name, as of bestsellers-date
- bestsellersDate string? (default ()) - YYYY-MM-DD The week-ending date for the sales reflected on list-name. Times best-seller lists are compiled using available book sale data. The bestsellers-date may be significantly earlier than published-date. For additional information, see the explanation at the bottom of any best-seller list page on NYTimes.com (example: Hardcover Fiction, published Dec. 5 but reflecting sales to Nov. 29).
- date string? (default ()) - YYYY-MM-DD The date the best-seller list was published on NYTimes.com (compare bestsellers-date)
- isbn string? (default ()) - International Standard Book Number, 10 or 13 digits
- publishedDate string? (default ()) - YYYY-MM-DD The date the best-seller list was published on NYTimes.com (compare bestsellers-date)
- rank int? (default ()) - The rank of the best seller on list-name as of bestsellers-date
- rankLastWeek int? (default ()) - The rank of the best seller on list-name one week prior to bestsellers-date
- offset int? (default ()) - Sets the starting point of the result set
- sortOrder string? (default ()) - Sets the sort order of the result set
Return Type
- InlineResponse200|error - Best seller list
getListsBestSellersHistoryJson
function getListsBestSellersHistoryJson(string? ageGroup, string? author, string? contributor, string? isbn, string? price, string? publisher, string? title) returns InlineResponse2001|error
Best Seller History List
Parameters
- ageGroup string? (default ()) - The target age group for the best seller.
- author string? (default ()) - The author of the best seller. The author field does not include additional contributors (see Data Structure for more details about the author and contributor fields). When searching the author field, you can specify any combination of first, middle and last names. When sort-by is set to author, the results will be sorted by author's first name.
- contributor string? (default ()) - The author of the best seller, as well as other contributors such as the illustrator (to search or sort by author name only, use author instead). When searching, you can specify any combination of first, middle and last names of any of the contributors. When sort-by is set to contributor, the results will be sorted by the first name of the first contributor listed.
- isbn string? (default ()) - International Standard Book Number, 10 or 13 digits A best seller may have both 10-digit and 13-digit ISBNs, and may have multiple ISBNs of each type. To search on multiple ISBNs, separate the ISBNs with semicolons (example: 9780446579933;0061374229).
- price string? (default ()) - The publisher's list price of the best seller, including decimal point
- publisher string? (default ()) - The standardized name of the publisher
- title string? (default ()) - The title of the best seller When searching, you can specify a portion of a title or a full title.
Return Type
- InlineResponse2001|error - Best seller history list
getListsNamesFormat
function getListsNamesFormat(string format) returns InlineResponse2002|error
Best Seller List Names
Parameters
- format string - Type format
Return Type
- InlineResponse2002|error - Best seller list names
getListsOverviewFormat
function getListsOverviewFormat(string format, string? publishedDate) returns InlineResponse2003|error
Best Seller List Overview
Parameters
- format string - Type format
- publishedDate string? (default ()) - The best-seller list publication date. YYYY-MM-DD You do not have to specify the exact date the list was published. The service will search forward (into the future) for the closest publication date to the date you specify. For example, a request for lists/overview/2013-05-22 will retrieve the list that was published on 05-26. If you do not include a published_date, the current week's best-sellers lists will be returned.
Return Type
- InlineResponse2003|error - Best seller list overview
getListsDateListJson
function getListsDateListJson(string date, string list, int? isbn, string? listName, string? publishedDate, string? bestsellersDate, int? weeksOnList, string? rank, int? rankLastWeek, int? offset, string? sortOrder) returns InlineResponse2004|error
Best Seller List by Date
Parameters
- date string - Date filter
- list string - Name of the Best Sellers List. You can get the full list from /lists/names.json
- isbn int? (default ()) - International Standard Book Number, 10 or 13 digits
- listName string? (default ()) - The name of the Times best-seller list. To get valid values, use a list names request. Be sure to replace spaces with hyphens (e.g., e-book-fiction or hardcover-fiction, not E-Book Fiction or Hardcover Fiction). (The parameter is not case sensitive.)
- publishedDate string? (default ()) - YYYY-MM-DD The date the best-seller list was published on NYTimes.com (compare bestsellers-date)
- bestsellersDate string? (default ()) - YYYY-MM-DD The week-ending date for the sales reflected on list-name. Times best-seller lists are compiled using available book sale data. The bestsellers-date may be significantly earlier than published-date. For additional information, see the explanation at the bottom of any best-seller list page on NYTimes.com (example: Hardcover Fiction, published Dec. 5 but reflecting sales to Nov. 29).
- weeksOnList int? (default ()) - The number of weeks that the best seller has been on list-name, as of bestsellers-date
- rank string? (default ()) - The rank of the best seller on list-name as of bestsellers-date
- rankLastWeek int? (default ()) - The rank of the best seller on list-name one week prior to bestsellers-date
- offset int? (default ()) - Sets the starting point of the result set
- sortOrder string? (default ()) - The default is ASC (ascending). The sort-order parameter is used with the sort-by parameter — for details, see each request type.
Return Type
- InlineResponse2004|error - Best seller list by date
getReviewsFormat
function getReviewsFormat(string format, int? isbn, string? title, string? author) returns InlineResponse2005|error
Reviews
Parameters
- format string - Type format
- isbn int? (default ()) - Searching by ISBN is the recommended method. You can enter 10- or 13-digit ISBNs.
- title string? (default ()) - You’ll need to enter the full title of the book. Spaces in the title will be converted into the characters %20.
- author string? (default ()) - You’ll need to enter the author’s first and last name, separated by a space. This space will be converted into the characters %20.
Return Type
- InlineResponse2005|error - Reviews
Records
nytimes.books: ApiKeysConfig
Provides API key configurations needed when communicating with a remote HTTP endpoint.
Fields
- apiKey string - All requests on the New York Times Books API needs to include an API key. The API key can be provided as part of the query string or as a request header. The name of the API key needs to be
api-key
.
nytimes.books: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
nytimes.books: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
nytimes.books: InlineResponse200
Fields
- copyright string? -
- last_modified string? -
- num_results int? -
- results InlineResponse200Results[]? -
- status string? -
nytimes.books: InlineResponse2001
Fields
- copyright string? -
- num_results int? -
- results InlineResponse2001Results[]? -
- status string? -
nytimes.books: InlineResponse2001Isbns
Fields
- isbn10 string? -
- isbn13 string? -
nytimes.books: InlineResponse2001RanksHistory
Fields
- asterisk int? -
- bestsellers_date string? -
- dagger int? -
- display_name string? -
- list_name string? -
- primary_isbn10 string? -
- primary_isbn13 string? -
- published_date string? -
- rank int? -
- ranks_last_week anydata? -
- weeks_on_list int? -
nytimes.books: InlineResponse2001Results
Fields
- age_group string? -
- author string? -
- contributor string? -
- contributor_note string? -
- description string? -
- isbns InlineResponse2001Isbns[]? -
- price int? -
- publisher string? -
- ranks_history InlineResponse2001RanksHistory[]? -
- reviews InlineResponse2001Reviews[]? -
- title string? -
nytimes.books: InlineResponse2001Reviews
Fields
- article_chapter_link string? -
- book_review_link string? -
- first_chapter_link string? -
- sunday_review_link string? -
nytimes.books: InlineResponse2002
Fields
- copyright string? -
- num_results int? -
- results InlineResponse2002Results[]? -
- status string? -
nytimes.books: InlineResponse2002Results
Fields
- display_name string? -
- list_name string? -
- list_name_encoded string? -
- newest_published_date string? -
- oldest_published_date string? -
- updated string? -
nytimes.books: InlineResponse2003
Fields
- copyright string? -
- num_results int? -
- results InlineResponse2003Results? -
- status string? -
nytimes.books: InlineResponse2003Results
Fields
- bestsellers_date string? -
- lists InlineResponse2003ResultsLists[]? -
- published_date string? -
nytimes.books: InlineResponse2003ResultsBooks
Fields
- age_group string? -
- author string? -
- contributor string? -
- contributor_note string? -
- created_date string? -
- description string? -
- price int? -
- primary_isbn10 string? -
- primary_isbn13 string? -
- publisher string? -
- rank int? -
- title string? -
- updated_date string? -
nytimes.books: InlineResponse2003ResultsLists
Fields
- books InlineResponse2003ResultsBooks[]? -
- display_name string? -
- list_id int? -
- list_image string? -
- list_name string? -
- updated string? -
nytimes.books: InlineResponse2004
Fields
- copyright string? -
- last_modified string? -
- num_results int? -
- results InlineResponse2004Results? -
- status string? -
nytimes.books: InlineResponse2004Results
Fields
- bestsellers_date string? -
- books InlineResponse2004ResultsBooks[]? -
- corrections record {}[]? -
- display_name string? -
- list_name string? -
- normal_list_ends_at int? -
- published_date string? -
- updated string? -
nytimes.books: InlineResponse2004ResultsBooks
Fields
- age_group string? -
- amazon_product_url string? -
- article_chapter_link string? -
- asterisk int? -
- author string? -
- book_image string? -
- book_review_link string? -
- contributor string? -
- contributor_note string? -
- dagger int? -
- description string? -
- first_chapter_link string? -
- isbns InlineResponse2001Isbns[]? -
- price int? -
- primary_isbn10 string? -
- primary_isbn13 string? -
- publisher string? -
- rank int? -
- rank_last_week int? -
- sunday_review_link string? -
- title string? -
- weeks_on_list int? -
nytimes.books: InlineResponse2005
Fields
- copyright string? -
- num_results int? -
- results InlineResponse2005Results[]? -
- status string? -
nytimes.books: InlineResponse2005Results
Fields
- book_author string? -
- book_title string? -
- byline string? -
- isbn13 string[]? -
- publication_dt string? -
- summary string? -
- url string? -
nytimes.books: InlineResponse200BookDetails
Fields
- age_group string? -
- author string? -
- contributor string? -
- contributor_note string? -
- description string? -
- price int? -
- primary_isbn10 string? -
- primary_isbn13 string? -
- publisher string? -
- title string? -
nytimes.books: InlineResponse200Results
Fields
- amazon_product_url string? -
- asterisk int? -
- bestsellers_date string? -
- book_details InlineResponse200BookDetails[]? -
- dagger int? -
- display_name string? -
- isbns InlineResponse2001Isbns[]? -
- list_name string? -
- published_date string? -
- rank int? -
- rank_last_week int? -
- reviews InlineResponse2001Reviews[]? -
- weeks_on_list int? -
nytimes.books: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
Import
import ballerinax/nytimes.books;
Metadata
Released date: almost 2 years ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 7
Current verison: 7
Weekly downloads
Keywords
Lifestyle & Entertainment/News & Lifestyle
Cost/Free
Contributors
Dependencies