notion
Module notion
API
Definitions
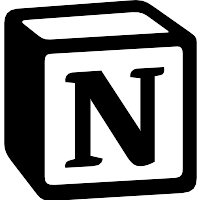
ballerinax/notion Ballerina library
Overview
This is a generated connector for Notion API v1 OpenAPI Specification.
Notion API provide capability to access all-in-one workspace where you can write, plan, collaborate and get organized. it has components such as notes, databases, kanban boards, wikis, calendars, and reminders. You can connect these components to take notes, add tasks, manage projects & more. Notion provides the building blocks and you can create your own layouts and toolkit to get work done. This ballerina connector allows you to connect Notion through its REST API.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Notion Account
- Obtaining tokens
- Obtain your token by creating an integration. Go to settings->integration. Follow this link for more information
Quickstart
To use the notion connector in your Ballerina application, update the .bal file as follows:
Step 1 - Import connector
First, import the ballerinax/notion module and others into the Ballerina project.
import ballerinax/notion;
Step 2 - Create a new connector instance
You can initialize the client as follows using ballerina configurable variables.
configurable http:BearerTokenConfig & readonly auth = ?; notion:ClientConfig clientConfig = { auth : auth }; notion:Client baseClient = check new Client(clientConfig);
Step 3 - Invoke connector operation
- Invoke connector operations using the client Get user detail by providing user ID
public function main() returns error? { notion:User response = check baseClient->retrieveUser(<UserID>); }
- Use
bal run
command to compile and run the Ballerina program.
Clients
notion: Client
This is a generated connector for Notion API v1 OpenAPI Specification. Notion API provides capability to access all-in-one workspace where you can write, plan, collaborate and get organized. it has components such as notes, databases, kanban boards, wikis, calendars, and reminders. You can connect these components to take notes, add tasks, manage projects & more. Notion provides the building blocks and you can create your own layouts and toolkit to get work done. This ballerina connector allows you to connect Notion through its REST API.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Notion account and obtain tokens following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.notion.com" - URL of the target service
retrieveBlockChildren
function retrieveBlockChildren(string id, string? pageSize, string notionVersion) returns BlockChildrenResponse|error
Retrieve block children
Parameters
- id string - block children ID
- pageSize string? (default ()) - Page size
- notionVersion string (default "2021-05-13") - API Version
Return Type
- BlockChildrenResponse|error - 200 Success - Retrieve block children
appendBlockChildren
function appendBlockChildren(string id, PageUpdateRequestBody payload, string notionVersion) returns ChildBlockContent|error
Append block children
Parameters
- id string - block children ID
- payload PageUpdateRequestBody -
- notionVersion string (default "2021-05-13") - API Version
Return Type
- ChildBlockContent|error - 200 Success - Append block children
listAllDatabases
function listAllDatabases(string? startCursor, int? pageSize, string notionVersion) returns DatabaseResponse|error
List all databases
Parameters
- startCursor string? (default ()) - If supplied, this endpoint will return a page of results starting after the cursor provided. If not supplied, this endpoint will return the first page of results.
- pageSize int? (default ()) - The number of items from the full list desired in the response. Maximum- 100
- notionVersion string (default "2021-05-13") - API Version
Return Type
- DatabaseResponse|error - 200 Success - List all databases
createDatabase
function createDatabase(DatabaseBodyParams payload, string notionVersion) returns DatabaseBodyParams|error
Create a database
Parameters
- payload DatabaseBodyParams - Page information
- notionVersion string (default "2021-05-13") - API Version
Return Type
- DatabaseBodyParams|error - 200 Success - Create a database
retrieveDatabase
Retrieve a database
queryDatabase
function queryDatabase(string id, DatabaseContent payload, string notionVersion) returns DatabaseResponse|error
Query a Database
Parameters
- id string - Database ID
- payload DatabaseContent -
- notionVersion string (default "2021-05-13") - API Version
Return Type
- DatabaseResponse|error - 200 Success - Query a Database (Single Filter)
createPage
function createPage(PageBodyParams payload, string notionVersion) returns PageResponse|error
Create a page
Parameters
- payload PageBodyParams - Page information
- notionVersion string (default "2021-05-13") - API Version
Return Type
- PageResponse|error - 200 Success - Create Page
retrievePage
function retrievePage(string id, string notionVersion) returns PageResponse|error
Retrieve a Page
Return Type
- PageResponse|error - 200 Success - Retrieve a Page
updatePageProperties
function updatePageProperties(string id, PageContent payload, string notionVersion) returns PageUpdatedProperties|error
Update Page properties
Parameters
- id string - Page ID
- payload PageContent -
- notionVersion string (default "2021-05-13") - API Version
Return Type
- PageUpdatedProperties|error - 200 Success - Update Page properties
listAllUsers
function listAllUsers(string? startCursor, int? pageSize, string notionVersion) returns PaginatedUsers|error
List all users
Parameters
- startCursor string? (default ()) - If supplied, this endpoint will return a page of results starting after the cursor provided. If not supplied, this endpoint will return the first page of results.
- pageSize int? (default ()) - The number of items from the full list desired in the response. Maximum- 100
- notionVersion string (default "2021-05-13") - API Version
Return Type
- PaginatedUsers|error - 200 Success - List all users
retrieveUser
Retrieve a user
searchPages
Searches all pages and child pages
Parameters
- notionVersion string (default "2021-05-13") - API Version
Return Type
- json|error - 200 Success - List all user
Records
notion: Annotations
Style information which applies to the whole rich text object
Fields
- bold boolean? - Whether the text is bolded
- italic boolean? - Whether the text is italic
- strikethrough boolean? - Whether the text is struck through
- underline boolean? - Whether the text is underlined
- code boolean? - Whether the text is "code style"
- color string? - Color of the text. Possible values are- "default", "gray", "brown", "orange", "yellow", "green", "blue", "purple", "pink", "red", "gray_background", "brown_background", "orange_background", "yellow_background", "green_background", "blue_background", "purple_background", "pink_background", "red_background"
notion: BlockChildrenResponse
The response for block children
Fields
- has_more boolean? - Indicates whether it has more objects or not
- next_cursor anydata? - Incidates the position of the response list
- 'object string? - The response object
- results ResultDetails[]? - The array of result details
notion: BlockContent
Block content
Fields
- text BlockcontentText[]? - block objects
notion: BlockcontentText
Fields
- text BlockContent[]? - block objects
- children BlockContent[]? - Any nested children blocks
- checked boolean? - Whether the to_do is checked or not
- content string? - Content of a block element
notion: ChildBlockContent
Child block details
Fields
- child_page ChildblockcontentChildPage? - Child page
- created_time string? - The created date/time
- has_children boolean? - Indicates whether it has children blocks
- id string? - ID of the block
- last_edited_time string? - The last edited date/time
- 'object string? - Always "block" for block types
- 'type string? - Type of the block. Possible values include "paragraph", "heading_1", "heading_2", "heading_3", "bulleted_list_item" etc.
notion: ChildblockcontentChildPage
Child page
Fields
- title string? - Title of the page
notion: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
notion: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
notion: Database
The database object
Fields
- created_time string? - The created date/time
- id string? - Database ID
- last_edited_time string? - The last edited date/time
- 'object string? - Object type "database"
- properties ObjectProperties? - Object Properties
- title DatabaseTitle[]? - Title of the database
notion: DatabaseAnnotations
The annotation
Fields
- bold boolean? - Indicates whether the title is bold or not
- code boolean? - Indicates whether the title is code or not
- color string? - Indicates whether the title is color or not
- italic boolean? - Indicates whether the title is italic or not
- strikethrough boolean? - Indicates whether the title is strikethrough or not
- underline boolean? - Indicates whether the title is underline or not
notion: DatabaseBodyParams
DatabaseDetails
Fields
- properties DatabaseProperties? - Property schema of database. The keys are the names of properties as they appear in Notion and the values are property schema objects
- parent DatabaseParent? - Parent Page Detail
- title DatabaseTitle[]? - Property schema of database
notion: DatabaseContent
Database content
Fields
- filter DatabasecontentFilter? - Filter detail
- sorts DatabasecontentSorts[]? - Sorting details
notion: DatabasecontentFilter
Filter detail
Fields
- or DatabasecontentFilterOr[]? - The filter set
notion: DatabasecontentFilterOr
The filter item
Fields
- property string? - The filter property
- 'select DatabasecontentFilterSelect? - The select details
notion: DatabasecontentFilterSelect
The select details
Fields
- 'equals string? - The equals values
notion: DatabasecontentSorts
Sort items
Fields
- direction string? - The sort direction
- property string? - The sort property
notion: DatabaseParent
Parent Page Detail
Fields
- page_id string? - Database ID
notion: DatabaseProperties
Property schema of database. The keys are the names of properties as they appear in Notion and the values are property schema objects
Fields
- title string? - Each database must have exactly one database property schema object of type "title". This database property controls the title that appears at the top of the page when the page is opened.
- rich_text string? - Text database property schema objects
- number DatabasepropertiesNumber? - Number database property schema object
- 'select SelectOptions? - Select option
- multi_select SelectOptions[]? - Multi-select database property schema object
- date string? - Date database property schema
- people string? - People database property schema
- files string? - File database property schema object
- checkbox string? - Checkbox database property schema object
- url string? - URL database property schema object
- email string? - Email database property schema object
- phone_number string? - Phone number database property schema object
- last_edited_time string? - Last edited time database property schema object
- last_edited_by string? - Last edited by database property schema object
- created_time string? - Created time database property schema object
- created_by string? - Created by database property schema object
notion: DatabasepropertiesNumber
Number database property schema object
Fields
- format string? - How the number is displayed in Notion. Potential values include- number, number_with_commas, percent, dollar, euro, pound, yen, ruble, rupee, won, yuan
notion: DatabaseResponse
Database response content
Fields
- has_more boolean? - Indicates whether has more objects
- next_cursor anydata? - The next position of the result
- 'object string? - Object Type "list"
- results DatabaseresponseResults[]? - Database results
notion: DatabaseresponseParent
The parent database
Fields
- database_id string? - Database ID
- 'type string? - The type of object
notion: DatabaseresponseResults
The databases
Fields
- archived boolean? - Indicates whether the database is archived or not
- created_time string? - Indicates whether the database is archived or not
- id string? - The ID of the database
- last_edited_time string? - The last edited time
- 'object string? - The object type "page"
- parent DatabaseresponseParent? - The parent database
- properties ObjectProperties? - Object Properties
notion: DatabaseText
The text detail
Fields
- content string? - The text content
- link anydata? - The link to the text
notion: DatabaseTitle
The item sets
Fields
- annotations DatabaseAnnotations? - The annotation
- href anydata? - HyperLink
- plain_text string? - Plain text
- text DatabaseText? - The text detail
- 'type string? - The type of the object
notion: Filtering
When supplied, filters the results based on the provided criteria
Fields
- value string? - The value of the property to filter the results by and Possible values for object type include page or database
- property string? - The name of the property to filter by.
notion: ObjectProperties
Object Properties
Fields
- Author ObjectpropertiesAuthor? - Author of the database
- Link ObjectpropertiesLink? - Related links
- Name ObjectpropertiesName? - The name of the object
- Publisher ObjectpropertiesPublisher? - Publisher Detail
- 'Publishing\/Release\ Date ObjectpropertiesPublishingreleaseDate? - Publishing/Releasing Date
- Read ObjectpropertiesRead? - Read details
- Status ObjectpropertiesStatus? - The status ID
- Summary ObjectpropertiesSummary? - The summary of the database
- Type ObjectpropertiesType? - The database type details
notion: ObjectpropertiesAuthor
Author of the database
Fields
- id string? - Author ID
- multi_select ObjectpropertiesAuthorMultiSelect? - multi_select values
- 'type string? - The type of the object
notion: ObjectpropertiesAuthorMultiSelect
multi_select values
Fields
- options ObjectpropertiesAuthorMultiSelectOptions[]? - The multi_select option
notion: ObjectpropertiesAuthorMultiSelectOptions
The option items
Fields
- color string? - Color of the database
- id string? - ID of the database
- name string? - Name of the Database
notion: ObjectpropertiesLink
Related links
Fields
- id string? - Link ID
- 'type string? - Link type
- url record {}? - URL
notion: ObjectpropertiesName
The name of the object
Fields
- id string? - Name ID
- title record {}? - tilte
- 'type string? - title Type
notion: ObjectpropertiesPublisher
Publisher Detail
Fields
- id string? - Publisher ID
- 'select ObjectpropertiesPublisherSelect? - Select details of publisher
- 'type string? - The type of the object
notion: ObjectpropertiesPublisherSelect
Select details of publisher
Fields
- options ObjectpropertiesPublisherSelectOptions[]? - The set of options
notion: ObjectpropertiesPublisherSelectOptions
The set of items in options
Fields
- color string? - The color
- id string? - The ID of the option
- name string? - The name of the option
notion: ObjectpropertiesPublishingreleaseDate
Publishing/Releasing Date
Fields
- date record {}? - The date of publishing/release
- id string? - ID of date
- 'type string? - Type of the object
notion: ObjectpropertiesRead
Read details
Fields
- checkbox record {}? - checkbox details
- id string? - ID of the checkbox
- 'type string? - The object type
notion: ObjectpropertiesStatus
The status ID
Fields
- id string? - The ID of the status
- 'select ObjectpropertiesPublisherSelect? - Select details of publisher
- 'type string? - The object type
notion: ObjectpropertiesSummary
The summary of the database
Fields
- id string? - The ID of the summary
- text record {}? - The summary content
- 'type string? - The type of the object
notion: ObjectpropertiesType
The database type details
Fields
- id string? - The type ID
- 'select ObjectpropertiesPublisherSelect? - Select details of publisher
- 'type string? - The object type
notion: PageBodyParams
PageDetail
Fields
- properties PageProperties? - Page properties
- parent PageParent? - Parent Page Detail
- children PageChildren? - Page content for the new page
notion: PagechildrenInner
Page content for the new pag
Fields
- 'object string? - Always "block"
- 'type string? - Type of block
- paragraph BlockContent? - Block content
- heading_1 BlockContent? - Block content
- heading_2 BlockContent? - Block content
- heading_3 BlockContent? - Block content
- bulleted_list_item BlockContent? - Block content
- numbered_list_item BlockContent? - Block content
- to_do BlockContent? - Block content
- child_page BlockContent? - Block content
notion: PageContent
Page content
Fields
- properties PagecontentProperties? - Page properties
notion: PagecontentProperties
Page properties
Fields
- Status PagecontentPropertiesStatus? - Status properties
notion: PagecontentPropertiesStatus
Status properties
Fields
- 'select PagecontentPropertiesStatusSelect? - Select properties
notion: PagecontentPropertiesStatusSelect
Select properties
Fields
- name string? - page content name
notion: PageParent
Parent Page Detail
Fields
- database_id string? - Database ID
notion: PageProperties
Page properties
Fields
- name string? - The ID of the property
- 'type string? - Type of the property
notion: PageResponse
Page Details
Fields
- archived boolean? - Indicates whether it is archived or not
- created_time string? - The created date/time
- id string? - ID of the page
- last_edited_time string? - The last edited date/time
- 'object string? - The object type "page"
- parent PageresponseParent? - Parent Page
- properties ObjectProperties? - Object Properties
notion: PageresponseParent
Parent Page
Fields
- database_id string? - Database ID
- 'type string? - The type of the object
notion: PageUpdatedProperties
The page content after update
Fields
- archived boolean? - Indicates whether it is archived or not
- created_time string? - The created date/time
- id string? - The ID of the Page
- last_edited_time string? - The last edited date/time
- 'object string? - The object type "page"
- properties ObjectProperties? - Object Properties
notion: PageUpdateRequestBody
The request body for page update
Fields
- children PageupdaterequestbodyChildren[]? - Children pages
notion: PageupdaterequestbodyChildren
page items
Fields
- heading_2 PageupdaterequestbodyHeading2? - Page heading
- 'object string? - The page object "block"
- 'type string? - The type of the object
notion: PageupdaterequestbodyHeading2
Page heading
Fields
- text PageupdaterequestbodyHeading2Text1[]? - Page text
notion: PageupdaterequestbodyHeading2Text
Text value
Fields
- content string? - Text content
notion: PageupdaterequestbodyHeading2Text1
Page text items
Fields
- text PageupdaterequestbodyHeading2Text? - Text value
- 'type string? - The type of the object
notion: PaginatedDatabases
The response body for users list
Fields
- results Database[]? - Array of Users
- next_cursor string? - Next curser position of the user list
- has_more boolean? - Indicates whether there are more user records or not
notion: PaginatedUsers
The response body for users list
Fields
- results User[]? - Array of Users
- next_cursor string? - Next curser position of the user list
- has_more boolean? - Indicates whether there are more user records or not
notion: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
notion: ResultDetails
Result Details
Fields
- created_time string? - The created date/time
- has_children boolean? - Indicates whether it has child object or not
- id string? - Object ID
- last_edited_time string? - The last date/time
- 'object string? - Object Category
- 'type string? - object type
- unsupported record {}? - Unsupported
notion: SearchBody
Search request body parameters
Fields
- query string? - When supplied, limits which pages are returned by comparing the query to the page title
- sort Sorting? - When supplied, sorts the results based on the provided criteria
- filter Filtering? - When supplied, filters the results based on the provided criteria
- start_cursor string? - If supplied, this endpoint will return a page of results starting after the cursor provided or else this endpoint will return the first page of results
- page_size int? - The number of items from the full list desired in the response. Maximum- 100
notion: SelectOptions
Select option
Fields
- name string? - Name of the option as it appears in Notion
- color string? - Color of the option. Possible values include- default, gray, brown, orange, yellow, green, blue, purple, pink, red
notion: Sorting
When supplied, sorts the results based on the provided criteria
Fields
- direction string? - The direction to sort. Possible values include ascending and descending
- timestamp string? - The name of the timestamp to sort against. Possible values include last_edited_time
notion: User
User detail
Fields
- avatar_url anydata? - avatar URL
- id string? - User ID
- name string? - Name of the User
- 'object string? - The object type User
- person UserPerson? - The contact detail
- 'type string? - The object type
notion: UserPerson
The contact detail
Fields
- email string? - Email address
Import
import ballerinax/notion;
Metadata
Released date: about 2 years ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 10
Current verison: 7
Weekly downloads
Keywords
Productivity/Project Management
Cost/Freemium
Contributors
Dependencies