newsapi
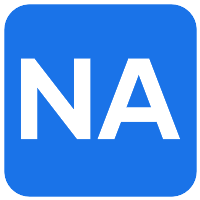
ballerinax/newsapi Ballerina library
Overview
This is a generated connector for News API v2.0.0 OpenAPI specification.
News API used to fetch news(articles, headlines and sources) from news sources and blogs across the web.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create News API Account
- Obtaining tokens
- Log into News API Account
- Get API key by clicking to
Get API Key
Quickstart
Step 1: Import connector
Import the ballerinax/newsapi module into the Ballerina project.
import ballerinax/newsapi;
Step 2: Create a new connector instance
newsapi:ApiKeysConfig config = { apiKey : "<your apiKey>" }; newsapi:Client myclient = check new newsapi:Client(config, {}, "https://newsapi.org/v2");
Step 3: Invoke connector operation
- You can get top headlines as follows with
listTopHeadlines
method by passing country as a parameter.newsapi:WSNewsTopHeadlineResponse result = check myclient->listTopHeadlines(country="us");
- Use
bal run
command to compile and run the Ballerina program.
Clients
newsapi: Client
This is a generated connector for News API v2.0.0 OpenAPI specification. News API used to fetch news(articles, headlines and sources) from news sources and blogs across the web.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create an News API Account and obtain tokens following this guide.
init (ApiKeysConfig apiKeyConfig, ConnectionConfig config, string serviceUrl)
- apiKeyConfig ApiKeysConfig - API keys for authorization
- config ConnectionConfig {} - The configurations to be used when initializing the
connector
- serviceUrl string "https://newsapi.org/v2" - URL of the target service
listSources
function listSources(string? language, string? country, string? category) returns WSNewsSourcesResponse|error
Returns a list of news sources or blogs
Parameters
- language string? (default ()) - Find sources that display news in a specific language. Possible options: ar de en es fr he it nl no pt ru se ud zh. Default: all languages
- country string? (default ()) - Find sources that display news in a specific country. Default: all countries
- category string? (default ()) - Find sources that display news of this category. Default: all categories
Return Type
- WSNewsSourcesResponse|error - Record containing list of news sources
listTopHeadlines
function listTopHeadlines(string? q, string? sources, string? country, string? category, int? page, int pageSize) returns WSNewsTopHeadlineResponse|error
Returns a list of top headlines
Parameters
- q string? (default ()) - Keywords or a phrase to search for
- sources string? (default ()) - Comma-seperated string of source identifiers for news sources or blogs
- country string? (default ()) - Find sources that display news in a specific country. Default: all countries
- category string? (default ()) - Find sources that display news of this category. Default: all categories
- page int? (default ()) - Use this to page through results if total results found is greater than page size
- pageSize int (default 20) - Number of results to return per page (request). 20 is default, 100 is maximum
Return Type
- WSNewsTopHeadlineResponse|error - Record containing list of top headlines
listArticles
function listArticles(int page, int pageSize, string? q, string? qInTitle, string? sources, string? domains, string? excludeDomains, string? 'from, string? to, string? language, string? sortBy) returns WSNewsTopHeadlineResponse|error
Returns list of articles
Parameters
- page int - Use this to page through results
- pageSize int - Number of results to return per page. 20 is default, 100 is maximum
- q string? (default ()) - Keywords or phrases to search for in article title and body. Complete value for query must be URL-encoded
- qInTitle string? (default ()) - Keywords or phrases to search for in article title only. Complete value for qInTitle must be URL-encoded
- sources string? (default ()) - Comma-seperated string of source identifiers (maximum 20) for news sources or blogs
- domains string? (default ()) - Comma-seperated string of domains (eg bbc.co.uk, techcrunch.com, engadget.com) to restrict search
- excludeDomains string? (default ()) - Comma-seperated string of domains (eg bbc.co.uk, techcrunch.com, engadget.com) to remove from results
- 'from string? (default ()) - Date and optional time for oldest article allowed. This should be in ISO 8601 format (e.g. 2021-02-15 or 2021-02-15T19:07:40) Default: oldest according to pricing plan
- to string? (default ()) - Date and optional time for newest article allowed. This should be in ISO 8601 format (e.g. 2021-02-15 or 2021-02-15T19:07:40) Default: newest according to pricing plan
- language string? (default ()) - Find sources that display news in a specific language. Possible options: ar de en es fr he it nl no pt ru se ud zh .Default: all languages
- sortBy string? (default ()) - Order to sort articles in. Possible options: relevancy, popularity, publishedAt
Return Type
- WSNewsTopHeadlineResponse|error - Record containing list of articles
Records
newsapi: ApiKeysConfig
Provides API key configurations needed when communicating with a remote HTTP endpoint.
Fields
- apiKey string - All requests on the News API needs to include an API key. The API key can be provided as part of the query string or as a request header. The name of the API key needs to be
apiKey
newsapi: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
newsapi: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
newsapi: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
newsapi: WSNewsArticle
Record representing a news article
Fields
- 'source WSNewsSource? - Record representing source of a news articles
- author string? - Author of article
- title string? - Headline or title of article
- description string? - Description or snippet from article
- url string? - Direct URL to article
- urlToImage string? - URL to a relevant image for article
- publishedAt string? - Date and time that article was published, in UTC (+000)
- content string? - Unformatted content of article, where available. This is truncated to 200 chars
newsapi: WSNewsErrorResponse
News API error response
Fields
- status string? - If request was successful or not. Options: ok, error. In case of ok, below two properties will not be present
- code string? - Short code identifying type of error returned
- message string? - Fuller description of error, usually including how to fix it
newsapi: WSNewsSource
Record representing source of a news articles
Fields
- id string? - Identifier of news source. You can use this with our other endpoints
- name string? - Name of news source
- description string? - Description of news source
- url string? - URL of homepage
- category string? - Find sources that display news of this category. Default: all categories
- language string? - Find sources that display news in a specific language. Possible options: ar de en es fr he it nl no pt ru se ud zh .Default: all languages
- country string? - Find sources that display news in a specific country. Default: all countries
newsapi: WSNewsSourcesResponse
Record representing list sources
Fields
- status string? - If request was successful or not. Options: ok, error. In case of ok, below two properties will not be present
- sources WSNewsSource[]? - Results of request
newsapi: WSNewsTopHeadlineResponse
Record representing top headlines
Fields
- status string? - If request was successful or not. Options: ok, error. In case of error a code and message property will be populated
- totalResults int? - Total number of results available for request. Only a limited number are shown at a time though, so use page parameter in requests to page through them
- articles WSNewsArticle[]? - Results of request
Import
import ballerinax/newsapi;
Metadata
Released date: over 1 year ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 2013
Current verison: 307
Weekly downloads
Keywords
Lifestyle & Entertainment/News & Lifestyle
Cost/Freemium
Contributors
Dependencies