neutrino
Module neutrino
Definitions
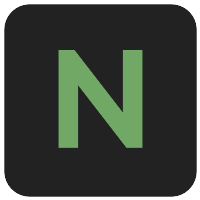
ballerinax/neutrino Ballerina library
Overview
This is a generated connector for Neutrino API v3.5.0 OpenAPI specification. The Neutrino API is a general-purpose API.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Neutrino account
- Obtain tokens by following this guide
Quickstart
To use the Neutrino connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/neutrino
module into the Ballerina project.
import ballerinax/neutrino;
Step 2: Create a new connector instance
Create a neutrino:ApiKeysConfig
with the User ID and API key obtained, and initialize the connector with it.
neutrino:ApiKeysConfig config = { userId: "<USER_ID>", apiKey: "<API_KEY>" } neutrino:Client baseClient = check new Client();
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to get location information about an IP address using the connector.
Get location information about an IP address
public function main() { neutrino:Body19 body19 = { 'output\-case: "kebab", ip: "162.209.104.195" }; neutrino:IPInfoResponse|error response = baseClient->getIPInfo(body19); if (response is neutrino:IPInfoResponse) { log:printInfo(response.toString()); } else { log:printError(response.message()); } }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
neutrino: Client
This is a generated connector for Neutrino API v3.5.0 OpenAPI specification. The Neutrino API is a general-purpose API.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Neutrino API account and obtain tokens by following this guide.
init (ApiKeysConfig apiKeyConfig, ConnectionConfig config, string serviceUrl)
- apiKeyConfig ApiKeysConfig - API keys for authorization
- config ConnectionConfig {} - The configurations to be used when initializing the
connector
- serviceUrl string "https://neutrinoapi.net/" - URL of the target service
verifySMS
function verifySMS(SmsverifyBody payload) returns SMSVerifyResponse|error
SMS Verify
Parameters
- payload SmsverifyBody - Request payload
Return Type
- SMSVerifyResponse|error - Response status
verifyPhone
function verifyPhone(PhoneverifyBody payload) returns PhoneVerifyResponse|error
Phone Verify
Parameters
- payload PhoneverifyBody - Request payload
Return Type
- PhoneVerifyResponse|error - Response status
lookupHLR
function lookupHLR(HlrlookupBody payload) returns HLRLookupResponse|error
HLR Lookup
Parameters
- payload HlrlookupBody - Request payload
Return Type
- HLRLookupResponse|error - Response status
sendSMS
function sendSMS(SmsmessageBody payload) returns SMSMessageResponse|error
SMS Message
Parameters
- payload SmsmessageBody - Request payload
Return Type
- SMSMessageResponse|error - Response status
verifySecurityCode
function verifySecurityCode(VerifysecuritycodeBody payload) returns VerifySecurityCodeResponse|error
Verify Security Code
Parameters
- payload VerifysecuritycodeBody - Request payload
Return Type
- VerifySecurityCodeResponse|error - Response status
playbackPhone
function playbackPhone(PhoneplaybackBody payload) returns PhonePlaybackResponse|error
Phone Playback
Parameters
- payload PhoneplaybackBody - Request payload
Return Type
- PhonePlaybackResponse|error - Response status
getUserAgentInfo
function getUserAgentInfo(UseragentinfoBody payload) returns UserAgentInfoResponse|error
User Agent Info
Parameters
- payload UseragentinfoBody - Request payload
Return Type
- UserAgentInfoResponse|error - Response status
validateEmail
function validateEmail(EmailvalidateBody payload) returns EmailValidateResponse|error
Email Validate
Parameters
- payload EmailvalidateBody - Request payload
Return Type
- EmailValidateResponse|error - Response status
validatePhone
function validatePhone(PhonevalidateBody payload) returns PhoneValidateResponse|error
Phone Validate
Parameters
- payload PhonevalidateBody - Request payload
Return Type
- PhoneValidateResponse|error - Response status
filterBadWord
function filterBadWord(BadwordfilterBody payload) returns BadWordFilterResponse|error
Bad Word Filter
Parameters
- payload BadwordfilterBody - Request payload
Return Type
- BadWordFilterResponse|error - Response status
hostReputation
function hostReputation(HostreputationBody payload) returns HostReputationResponse|error
Host Reputation
Parameters
- payload HostreputationBody - Request payload
Return Type
- HostReputationResponse|error - Response status
probeIP
function probeIP(IpprobeBody payload) returns IPProbeResponse|error
IP Probe
Parameters
- payload IpprobeBody - Request payload
Return Type
- IPProbeResponse|error - Response status
verifyEmail
function verifyEmail(EmailverifyBody payload) returns EmailVerifyResponse|error
Email Verify
Parameters
- payload EmailverifyBody - Request payload
Return Type
- EmailVerifyResponse|error - Response status
detectIPBlocklist
function detectIPBlocklist(IpblocklistBody payload) returns IPBlocklistResponse|error
IP Blocklist
Parameters
- payload IpblocklistBody - Request payload
Return Type
- IPBlocklistResponse|error - Response status
downloadIPBlocklist
function downloadIPBlocklist(IpblocklistdownloadBody payload) returns string|error
IP Blocklist Download
Parameters
- payload IpblocklistdownloadBody - Request payload
getIPInfo
function getIPInfo(IpinfoBody payload) returns IPInfoResponse|error
IP Info
Parameters
- payload IpinfoBody - Request payload
Return Type
- IPInfoResponse|error - Response status
reverseGeocode
function reverseGeocode(GeocodereverseBody payload) returns GeocodeReverseResponse|error
Geocode Reverse
Parameters
- payload GeocodereverseBody - Request payload
Return Type
- GeocodeReverseResponse|error - Response status
geocodeAddress
function geocodeAddress(GeocodeaddressBody payload) returns GeocodeAddressResponse|error
Geocode Address
Parameters
- payload GeocodeaddressBody - Request payload
Return Type
- GeocodeAddressResponse|error - Response status
convertCurrency
function convertCurrency(ConvertBody payload) returns ConvertResponse|error
Currency Convert
Parameters
- payload ConvertBody - Request payload
Return Type
- ConvertResponse|error - Response status
lookupBIN
function lookupBIN(BinlookupBody payload) returns BINLookupResponse|error
BIN Lookup
Parameters
- payload BinlookupBody - Request payload
Return Type
- BINLookupResponse|error - Response status
downloadBINList
function downloadBINList(BinlistdownloadBody payload) returns string|error
BIN List Download
Parameters
- payload BinlistdownloadBody - Request payload
getURLInfo
function getURLInfo(UrlinfoBody payload) returns URLInfoResponse|error
URL Info
Parameters
- payload UrlinfoBody - Request payload
Return Type
- URLInfoResponse|error - Response status
cleanHTML
function cleanHTML(HtmlcleanBody payload) returns string|error
HTML Clean
Parameters
- payload HtmlcleanBody - Request payload
browserBot
function browserBot(BrowserbotBody payload) returns BrowserBotResponse|error
Browser Bot
Parameters
- payload BrowserbotBody - Request payload
Return Type
- BrowserBotResponse|error - Response status
Records
neutrino: APIError
Fields
- apiError int - API error code. If set and > 0 then an API error has occurred your request could not be completed
- apiErrorMsg string - API error message
neutrino: ApiKeysConfig
Provides API key configurations needed when communicating with a remote HTTP endpoint.
Fields
- userId string - Represents API Key
user-id
- apiKey string - Represent API Key
api-key
neutrino: BadwordfilterBody
Fields
- 'output\-case string - The output case style
- content string - The content to scan. This can be either a URL to load from, a file upload or an HTML content string
- 'censor\-character string? - The character to use to censor out the bad words found
- catalog string(default "strict") - Which catalog of bad words to use, we currently maintain two bad word catalogs: <br> <ul> <li>strict - the largest database of bad words which includes profanity, obscenity, sexual, rude, cuss, dirty, swear and objectionable words and phrases. This catalog is suitable for environments of all ages including educational or children's content</li> <li>obscene - like the strict catalog but does not include any mild profanities, idiomatic phrases or words which are considered formal terminology. This catalog is suitable for adult environments where certain types of bad words are considered OK</li> </ul>
neutrino: BadWordFilterResponse
Fields
- badWordsList string[] - An array of the bad words found
- badWordsTotal int - Total number of bad words detected
- censoredContent string - The censored content (only set if censor-character has been set)
- isBad boolean - Does the text contain bad words
neutrino: BinlistdownloadBody
Fields
- 'include\-iso3 boolean? - Include ISO 3-letter country codes and ISO 3-letter currency codes in the data. These will be added to columns 10 and 11 respectively
- 'include\-8digit boolean? - Include 8-digit and higher BIN codes. Use this option if you want to download BINs with more than 6-digits
neutrino: BinlookupBody
Fields
- 'output\-case string - The output case style
- 'bin\-number string - The BIN or IIN number. This is the first 6, 8 or 10 digits of a card number, use 8 (or more) digits for the highest level of accuracy
- 'customer\-ip string? - Pass in the customers IP address and we will return some extra information about them
neutrino: BINLookupResponse
Fields
- country string - The full country name of the issuer
- ipCity string - The city of the customers IP (if detectable)
- ipMatchesBin boolean - True if the customers IP country matches the BIN country
- cardType string - The card type, will always be one of: DEBIT, CREDIT, CHARGE CARD
- cardCategory string - The card category. There are many different card categories the most common card categories are: CLASSIC, BUSINESS, CORPORATE, PLATINUM, PREPAID
- ipCountryCode string - The ISO 2-letter country code of the customers IP
- ipCountry string - The country of the customers IP
- issuer string - The card issuer
- ipBlocklisted boolean - True if the customers IP is listed on one of our blocklists, see the <a href="http://www.neutrinoapi.com/api/ip-blocklist/">IP Blocklist API</a>
- valid boolean - Is this a valid BIN or IIN number
- ipBlocklists string[] - An array of strings indicating which blocklists this IP is listed on
- issuerWebsite string - The card issuers website
- countryCode string - The ISO 2-letter country code of the issuer
- ipRegion string - The region of the customers IP (if detectable)
- cardBrand string - The card brand (e.g. Visa or Mastercard)
- issuerPhone string - The card issuers phone number
- countryCode3 string - The ISO 3-letter country code of the issuer
- currencyCode string - ISO 4217 currency code associated with the country of the issuer
- ipCountryCode3 string - The ISO 3-letter country code of the customers IP
- isCommercial boolean - Is this a commercial/business use card
- isPrepaid boolean - Is this a prepaid or prepaid reloadable card
neutrino: Blacklist
Fields
- isListed boolean - True if the host is currently black-listed
- listHost string - The hostname of the DNSBL
- listRating int - The list rating [1-3] with 1 being the best rating and 3 the lowest rating
- listName string - The name of the DNSBL
- txtRecord string - The TXT record returned for this listing (only set if listed)
- returnCode string - The specific return code for this listing (only set if listed)
- responseTime int - The DNSBL server response time in milliseconds
neutrino: BrowserbotBody
Fields
- 'output\-case string - The output case style
- url string - The URL to load
- timeout int(default 30) - Timeout in seconds. Give up if still trying to load the page after this number of seconds
- delay int(default 3) - Delay in seconds to wait before capturing any page data, executing selectors or JavaScript
- selector string? - Extract content from the page DOM using this selector. Commonly known as a CSS selector, you can find a good reference <a href="https://www.w3schools.com/cssref/css_selectors.asp">here</a>
- exec string[](default []) - Execute JavaScript on the page, each array element should contain a valid JavaScript statement. If a statement returns any kind of value it will be returned in the 'exec-results' response. You can also use the following special user interaction functions: <br> <br> <div> sleep(seconds); Just wait/sleep for the specified number of seconds. <br>click('selector'); Click on the first element matching the given selector. <br>focus('selector'); Focus on the first element matching the given selector. <br>keys('characters'); Send the specified keyboard characters. Use click() or focus() first to send keys to a specific element. <br>enter(); Send the Enter key. <br>tab(); Send the Tab key. <br> </div>
- 'user\-agent string? - Override the browsers default user-agent string with this one
- 'ignore\-certificate\-errors boolean(default false) - Ignore any TLS/SSL certificate errors and load the page anyway
neutrino: BrowserBotResponse
Fields
- url string - The page URL
- content string - The complete raw, decompressed and decoded page content. Usually will be either HTML, JSON or XML
- mimeType string - The document MIME type
- title string - The document title
- isError boolean - True if an error has occurred loading the page. Check the 'error-message' field for details
- isTimeout boolean - True if a timeout occurred while loading the page. You can set the timeout with the request parameter 'timeout'
- errorMessage string - Contains the error message if an error has occurred ('is-error' will be true)
- httpStatusCode int - The HTTP status code the URL returned
- httpStatusMessage string - The HTTP status message the URL returned
- isHttpOk boolean - True if the HTTP status is OK (200)
- isHttpRedirect boolean - True if the URL responded with an HTTP redirect
- httpRedirectUrl string - The redirected URL if the URL responded with an HTTP redirect
- serverIp string - The HTTP servers IP address
- loadTime decimal - The number of seconds taken to load the page (from initial request until DOM ready)
- responseHeaders record {} - Map containing all the HTTP response headers the URL responded with
- isSecure boolean - True if the page is secured using TLS/SSL
- securityDetails record {} - Map containing details of the TLS/SSL setup
- elements string[] - Array containing all the elements matching the supplied selector. <br>Each element object will contain the text content, HTML content and all current element attributes
- execResults string[] - If you executed any JavaScript this array holds the results as objects
- languageCode string - The ISO 2-letter language code of the page. Extracted from either the HTML document or via HTTP headers
neutrino: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
neutrino: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
neutrino: ConvertBody
Fields
- 'output\-case string - The output case style
- 'from\-value string - The value to convert from (e.g. 10.95)
- 'from\-type string - The type of the value to convert from (e.g. USD)
- 'to\-type string - The type to convert to (e.g. EUR)
neutrino: ConvertResponse
Fields
- valid boolean - True if the coversion was successful and produced a valid result
- result string - The result of the conversion in string format
- fromValue string - The value being converted from
- toType string - The type being converted to
- fromType string - The type of the value being converted from
- resultFloat decimal - The result of the conversion as a floating-point number
neutrino: EmailvalidateBody
Fields
- 'output\-case string - The output case style
- email string - An email address
- 'fix\-typos boolean(default false) - Automatically attempt to fix typos in the address
neutrino: EmailValidateResponse
Fields
- valid boolean - Is this a valid email
- syntaxError boolean - True if this address has a syntax error
- domain string - The email domain
- domainError boolean - True if this address has a domain error (e.g. no valid mail server records)
- isFreemail boolean - True if this address is a free-mail address
- email string - The email address. If you have used the fix-typos option then this will be the fixed address
- isDisposable boolean - True if this address is a disposable, temporary or darknet related email address
- typosFixed boolean - True if typos have been fixed
- isPersonal boolean - True if this address belongs to a person. False if this is a role based address, e.g. admin@, help@, office@, etc.
- provider string - The email service provider domain
neutrino: EmailverifyBody
Fields
- 'output\-case string - The output case style
- email string - An email address
- 'fix\-typos boolean(default false) - Automatically attempt to fix typos in the address
neutrino: EmailVerifyResponse
Fields
- valid boolean - Is this a valid email address (syntax and domain is valid)
- verified boolean - True if this address has passed SMTP verification. Check the smtp-status and smtp-response fields for specific verification details
- email string - The email address. If you have used the fix-typos option then this will be the fixed address
- typosFixed boolean - True if typos have been fixed
- syntaxError boolean - True if this address has a syntax error
- domainError boolean - True if this address has a domain error (e.g. no valid mail server records)
- domain string - The email domain
- provider string - The email service provider domain
- isFreemail boolean - True if this address is a free-mail address
- isDisposable boolean - True if this address is a disposable, temporary or darknet related email address
- isPersonal boolean - True if this address is for a person. False if this is a role based address, e.g. admin@, help@, office@, etc.
- smtpStatus string - The SMTP verification status for the address: <br> <ul> <li>ok - SMTP verification was successful, this is a real address that can receive mail</li> <li>invalid - this is not a valid email address (has either a domain or syntax error)</li> <li>absent - this address is not registered with the email service provider</li> <li>unresponsive - the mail server(s) for this address timed-out or refused to open an SMTP connection</li> <li>unknown - sorry, we could not reliably determine the real status of this address (this address may or may not exist)</li> </ul>
- smtpResponse string - The raw SMTP response message received during verification
- isCatchAll boolean - True if this email domain has a catch-all policy (it will accept mail for any username)
- isDeferred boolean - True if the mail server responded with a temporary failure (either a 4xx response code or unresponsive server). You can retry this address later, we recommend waiting at least 15 minutes before retrying
neutrino: GeocodeaddressBody
Fields
- 'output\-case string - The output case style
- address string? - The full address, partial address or name of a place to try and locate. Comma separated address components are preferred.
- 'house\-number string? - The house/building number to locate
- street string? - The street/road name to locate
- city string? - The city/town name to locate
- county string? - The county/region name to locate
- state string? - The state name to locate
- 'postal\-code string? - The postal code to locate
- 'country\-code string? - Limit result to this country (the default is no country bias)
- 'language\-code string(default "en") - The language to display results in, available languages are: <ul> <li>de, en, es, fr, it, pt, ru, zh</li> </ul>
- 'fuzzy\-search boolean(default false) - If no matches are found for the given address, start performing a recursive fuzzy search until a geolocation is found. This option is recommended for processing user input or implementing auto-complete. We use a combination of approximate string matching and data cleansing to find possible location matches
neutrino: GeocodeAddressResponse
Fields
- found int - The number of possible matching locations found
- locations Location[] - Array of matching location objects
neutrino: GeocodereverseBody
Fields
- 'output\-case string - The output case style
- latitude string - The location latitude in decimal degrees format
- longitude string - The location longitude in decimal degrees format
- 'language\-code string(default "en") - The language to display results in, available languages are: <ul> <li>de, en, es, fr, it, pt, ru</li> </ul>
- zoom string(default "address") - The zoom level to respond with: <br> <ul> <li>address - the most precise address available</li> <li>street - the street level</li> <li>city - the city level</li> <li>state - the state level</li> <li>country - the country level</li> </ul>
neutrino: GeocodeReverseResponse
Fields
- country string - The country of the location
- found boolean - True if these coordinates map to a real location
- address string - The fully formatted address
- city string - The city of the location
- countryCode string - The ISO 2-letter country code of the location
- postalCode string - The postal code for the location
- state string - The state of the location
- addressComponents record {} - The components which make up the address such as road, city, state, etc
- countryCode3 string - The ISO 3-letter country code of the location
- currencyCode string - ISO 4217 currency code associated with the country
- locationType string - The detected location type ordered roughly from most to least precise, possible values are: <br> <ul> <li>address - indicates a precise street address</li> <li>street - accurate to the street level but may not point to the exact location of the house/building number</li> <li>city - accurate to the city level, this includes villages, towns, suburbs, etc</li> <li>postal-code - indicates a postal code area (no house or street information present)</li> <li>railway - location is part of a rail network such as a station or railway track</li> <li>natural - indicates a natural feature, for example a mountain peak or a waterway</li> <li>island - location is an island or archipelago</li> <li>administrative - indicates an administrative boundary such as a country, state or province</li> </ul>
- locationTags string[] - Array of strings containing any location tags associated with the address. Tags are additional pieces of metadata about a specific location, there are thousands of different tags. Some examples of tags: shop, office, cafe, bank, pub
- latitude decimal - The location latitude
- longitude decimal - The location longitude
- timezone record {} - Map containing timezone details for the location
neutrino: HlrlookupBody
Fields
- 'output\-case string - The output case style
- number string - A phone number
- 'country\-code string? - ISO 2-letter country code, assume numbers are based in this country. <br>If not set numbers are assumed to be in international format (with or without the leading + sign)
neutrino: HLRLookupResponse
Fields
- numberValid boolean - True if this a valid phone number
- internationalCallingCode int - The numbers international calling code
- mnc string - The mobile MNC number (Mobile Network Code)
- numberType string - The number type, possible values are: <br> <ul> <li>mobile</li> <li>fixed-line</li> <li>premium-rate</li> <li>toll-free</li> <li>voip</li> <li>unknown</li> </ul>
- hlrValid boolean - Was the HLR lookup successful. If true then this is a working and registered cell-phone or mobile device (SMS and phone calls will be delivered)
- hlrStatus string - The HLR lookup status, possible values are: <br> <ul> <li>ok - the HLR lookup was successful and the device is connected</li> <li>absent - the number was once registered but the device has been switched off or out of network range for some time</li> <li>unknown - the number is not known by the mobile network</li> <li>invalid - the number is not a valid mobile MSISDN number</li> <li>fixed-line - the number is a registered fixed-line not mobile</li> <li>voip - the number has been detected as a VOIP line</li> <li>failed - the HLR lookup has failed, we could not determine the real status of this number</li> </ul>
- portedNetwork string - The ported to network/carrier name (only set if the number has been ported)
- imsi string - The mobile IMSI number (International Mobile Subscriber Identity)
- mcc string - The mobile MCC number (Mobile Country Code)
- internationalNumber string - The number represented in full international format
- localNumber string - The number represented in local dialing format
- countryCode string - The number location as an ISO 2-letter country code
- isPorted boolean - Has this number been ported to another network
- msin string - The mobile MSIN number (Mobile Subscription Identification Number)
- location string - The number location. Could be a city, region or country depending on the type of number
- originNetwork string - The origin network/carrier name
- isMobile boolean - True if this is a mobile number (only true with 100% certainty, if the number type is unknown this value will be false)
- isRoaming boolean - Is this number currently roaming from its origin country
- country string - The phone number country
- countryCode3 string - The number location as an ISO 3-letter country code
- currencyCode string - ISO 4217 currency code associated with the country
- roamingCountryCode string - If the number is currently roaming, the ISO 2-letter country code of the roaming in country
- msc string - The mobile MSC number (Mobile Switching Center)
- currentNetwork string - The currently used network/carrier name
neutrino: HostreputationBody
Fields
- 'output\-case string - The output case style
- host string - An IP address, domain name, FQDN or URL. <br>If you supply a domain/URL it will be checked against the URI DNSBL lists
- 'list\-rating int(default 3) - Only check lists with this rating or better
neutrino: HostReputationResponse
Fields
- isListed boolean - Is this host blacklisted
- lists Blacklist[] - Array of objects for each DNSBL checked
- listCount int - The number of DNSBLs the host is listed on
- host string - The IP address or host name
neutrino: HtmlcleanBody
Fields
- content string - The HTML content. This can be either a URL to load from, a file upload or an HTML content string
- 'output\-type string - The level of sanitization, possible values are: <br> <b>plain-text</b>: reduce the content to plain text only (no HTML tags at all) <br> <br> <b>simple-text</b>: allow only very basic text formatting tags like b, em, i, strong, u <br> <br> <b>basic-html</b>: allow advanced text formatting and hyper links <br> <br> <b>basic-html-with-images</b>: same as basic html but also allows image tags <br> <br> <b>advanced-html</b>: same as basic html with images but also allows many more common HTML tags like table, ul, dl, pre <br>
neutrino: IpblocklistBody
Fields
- 'output\-case string - The output case style
- ip string - An IPv4 or IPv6 address
- 'vpn\-lookup boolean(default false) - Include public VPN provider IP addresses. <br> <b>NOTE</b>: For more advanced VPN detection including the ability to identify private and stealth VPNs use the <a href="https://www.neutrinoapi.com/api/ip-probe/">IP Probe API</a>
neutrino: IpblocklistdownloadBody
Fields
- format string? - The data format. Can be either CSV or TXT
- 'include\-vpn boolean? - Include public VPN provider IP addresses, this option is only available for Tier 3 or higher accounts. <br> <b>WARNING</b>: This option will add at least an additional 8 million IP addresses to the download
neutrino: IPBlocklistResponse
Fields
- ip string - The IP address
- isBot boolean - IP is hosting a malicious bot or is part of a botnet. Includes brute-force crackers
- isExploitBot boolean - IP is hosting an exploit finding bot or is running exploit scanning software
- isMalware boolean - IP is involved in distributing or is running malware
- isSpider boolean - IP is running a hostile web spider / web crawler
- isDshield boolean - IP has been flagged as an attack source on DShield (dshield.org)
- listCount int - The number of blocklists the IP is listed on
- isProxy boolean - IP has been detected as an anonymous web proxy or anonymous HTTP proxy
- isHijacked boolean - IP is part of a hijacked netblock or a netblock controlled by a criminal organization
- isTor boolean - IP is a Tor node or running a Tor related service
- isSpyware boolean - IP is involved in distributing or is running spyware
- isSpamBot boolean - IP address is hosting a spam bot, comment spamming or any other spamming type software
- isListed boolean - Is this IP on a blocklist
- isVpn boolean - IP belongs to a public VPN provider (only set if the 'vpn-lookup' option is enabled)
- lastSeen int - The last time this IP was seen on a blocklist (in Unix time or 0 if not listed recently)
- blocklists string[] - An array of strings indicating which blocklists this IP is listed on (empty if not listed)
- sensors string[] - An array of objects containing details on which sensors were used to detect this IP
neutrino: IpinfoBody
Fields
- 'output\-case string - The output case style
- ip string - IPv4 or IPv6 address
- 'reverse\-lookup boolean(default false) - Do a reverse DNS (PTR) lookup. This option can add extra delay to the request so only use it if you need it
neutrino: IPInfoResponse
Fields
- valid boolean - True if this is a valid IPv4 or IPv6 address
- country string - Full country name
- hostname string - The IPs full hostname (only set if reverse-lookup has been used)
- city string - Name of the city (if detectable)
- 'country\-code string - ISO 2-letter country code
- latitude decimal - Location latitude
- region string - Name of the region (if detectable)
- longitude decimal - Location longitude
- 'continent\-code string - ISO 2-letter continent code
- ip string - The IP address
- 'country\-code3 string - ISO 3-letter country code
- 'currency\-code string - ISO 4217 currency code associated with the country
- 'host\-domain string - The IPs host domain (only set if reverse-lookup has been used)
- timezone record {} - Map containing timezone details for the location
- 'is\-v6 boolean - True if this is a IPv6 address. False if IPv4
- 'is\-v4\-mapped boolean - True if this is a <a href="https://en.wikipedia.org/wiki/IPv6#IPv4-mapped_IPv6_addresses">IPv4 mapped IPv6 address</a>
- 'is\-bogon boolean - True if this is a bogon IP address such as a private network, local network or reserved address
neutrino: IpprobeBody
Fields
- 'output\-case string - The output case style
- ip string - IPv4 or IPv6 address
neutrino: IPProbeResponse
Fields
- valid boolean - True if this is a valid IPv4 or IPv6 address
- country string - Full country name
- providerType string - The detected provider type, possible values are: <br> <ul> <li>isp - IP belongs to an internet service provider. This includes both mobile, home and business internet providers</li> <li>hosting - IP belongs to a hosting company. This includes website hosting, cloud computing platforms and colocation facilities</li> <li>vpn - IP belongs to a VPN provider</li> <li>proxy - IP belongs to a proxy service. This includes HTTP/SOCKS proxies and browser based proxies</li> <li>university - IP belongs to a university/college/campus</li> <li>government - IP belongs to a government department. This includes military facilities</li> <li>commercial - IP belongs to a commercial entity such as a corporate headquarters or company office</li> <li>unknown - could not identify the provider type</li> </ul>
- countryCode string - ISO 2-letter country code
- hostname string - The IPs full hostname (PTR)
- providerDomain string - The domain name of the provider
- city string - Full city name (if detectable)
- providerWebsite string - The website URL for the provider
- ip string - The IP address
- region string - Full region name (if detectable)
- providerDescription string - A description of the provider (usually extracted from the providers website)
- continentCode string - ISO 2-letter continent code
- isHosting boolean - True if this IP belongs to a hosting company. Note that this can still be true even if the provider type is VPN/proxy, this occurs in the case that the IP is detected as both types
- isIsp boolean - True if this IP belongs to an internet service provider. Note that this can still be true even if the provider type is VPN/proxy, this occurs in the case that the IP is detected as both types
- countryCode3 string - ISO 3-letter country code
- currencyCode string - ISO 4217 currency code associated with the country
- isVpn boolean - True if this IP ia a VPN
- isProxy boolean - True if this IP ia a proxy
- asn string - The autonomous system (AS) number
- asCidr string - The autonomous system (AS) CIDR range
- asCountryCode string - The autonomous system (AS) ISO 2-letter country code
- asCountryCode3 string - The autonomous system (AS) ISO 3-letter country code
- asDomains string[] - Array of all the domains associated with the autonomous system (AS)
- asDescription string - The autonomous system (AS) description / company name
- asAge int - The age of the autonomous system (AS) in number of years since registration
- hostDomain string - The IPs host domain
- vpnDomain string - The domain of the VPN provider (may be empty if the VPN domain is not detectable)
- isV6 boolean - True if this is a IPv6 address. False if IPv4
- isV4Mapped boolean - True if this is a <a href="https://en.wikipedia.org/wiki/IPv6#IPv4-mapped_IPv6_addresses">IPv4 mapped IPv6 address</a>
- isBogon boolean - True if this is a bogon IP address such as a private network, local network or reserved address
neutrino: Location
Fields
- country string - The country of the location
- address string - The fully formatted address
- city string - The city of the location
- countryCode string - The ISO 2-letter country code of the location
- countryCode3 string - The ISO 3-letter country code of the location
- latitude decimal - The location latitude
- postalCode string - The postal code for the location
- longitude decimal - The location longitude
- state string - The state of the location
- addressComponents record {} - The components which make up the address such as road, city, state, etc
- currencyCode string - ISO 4217 currency code associated with the country
- locationType string - The detected location type ordered roughly from most to least precise, possible values are: <br> <ul> <li>address - indicates a precise street address</li> <li>street - accurate to the street level but may not point to the exact location of the house/building number</li> <li>city - accurate to the city level, this includes villages, towns, suburbs, etc</li> <li>postal-code - indicates a postal code area (no house or street information present)</li> <li>railway - location is part of a rail network such as a station or railway track</li> <li>natural - indicates a natural feature, for example a mountain peak or a waterway</li> <li>island - location is an island or archipelago</li> <li>administrative - indicates an administrative boundary such as a country, state or province</li> </ul>
- locationTags string[] - Array of strings containing any location tags associated with the address. Tags are additional pieces of metadata about a specific location, there are thousands of different tags. Some examples of tags: shop, office, cafe, bank, pub
- timezone record {} - Map containing timezone details for the location
neutrino: PhoneplaybackBody
Fields
- 'output\-case string - The output case style
- number string - The phone number to call. Must be in valid international format
- 'audio\-url string - A URL to a valid audio file. Accepted audio formats are: <ul> <li>MP3</li> <li>WAV</li> <li>OGG</li> </ul>You can use the following MP3 URL for testing: <br>https://www.neutrinoapi.com/test-files/test1.mp3
- 'limit int(default 3) - Limit the total number of calls allowed to the supplied phone number, if the limit is reached error code 14 will be returned (0 means no limit)
- 'limit\-ttl int(default 1) - Set the TTL in number of days that the 'limit' option will remember a phone number (the default is 1 day and the maximum is 365 days)
neutrino: PhonePlaybackResponse
Fields
- calling boolean - True if the call is being made now
- numberValid boolean - True if this a valid phone number
neutrino: PhonevalidateBody
Fields
- 'output\-case string - The output case style
- number string - A phone number. This can be in international format (E.164) or local format. If passing local format you must also set either the 'country-code' OR 'ip' options as well
- 'country\-code string? - ISO 2-letter country code, assume numbers are based in this country. If not set numbers are assumed to be in international format (with or without the leading + sign)
- ip string? - Pass in a users IP address and we will assume numbers are based in the country of the IP address
neutrino: PhoneValidateResponse
Fields
- valid boolean - Is this a valid phone number
- internationalCallingCode int - The international calling code
- countryCode string - The phone number country as an ISO 2-letter country code
- location string - The phone number location. Could be the city, region or country depending on the type of number
- isMobile boolean - True if this is a mobile number. If the number type is unknown this value will be false
- 'type string - The number type based on the number prefix. <br>Possible values are: <br> <ul> <li>mobile</li> <li>fixed-line</li> <li>premium-rate</li> <li>toll-free</li> <li>voip</li> <li>unknown (use HLR lookup)</li> </ul>
- internationalNumber string - The number represented in full international format (E.164)
- localNumber string - The number represented in local dialing format
- country string - The phone number country
- countryCode3 string - The phone number country as an ISO 3-letter country code
- currencyCode string - ISO 4217 currency code associated with the country
- prefixNetwork string - The network/carrier who owns the prefix (this only works for some countries, use HLR lookup for global network detection)
neutrino: PhoneverifyBody
Fields
- 'output\-case string - The output case style
- number string - The phone number to send the verification code to
- 'code\-length int(default 6) - The number of digits to use in the security code (between 4 and 12)
- 'security\-code int? - Pass in your own security code. This is useful if you have implemented TOTP or similar 2FA methods. If not set then we will generate a secure random code
- 'playback\-delay int(default 800) - The delay in milliseconds between the playback of each security code
- 'country\-code string? - ISO 2-letter country code, assume numbers are based in this country. <br>If not set numbers are assumed to be in international format (with or without the leading + sign)
- 'language\-code string(default "en") - The language to playback the verification code in, available languages are: <ul> <li>de - German</li> <li>en - English</li> <li>es - Spanish</li> <li>fr - French</li> <li>it - Italian</li> <li>pt - Portuguese</li> <li>ru - Russian</li> </ul>
- 'limit int(default 3) - Limit the total number of calls allowed to the supplied phone number, if the limit is reached error code 14 will be returned (0 means no limit)
- 'limit\-ttl int(default 1) - Set the TTL in number of days that the 'limit' option will remember a phone number (the default is 1 day and the maximum is 365 days)
neutrino: PhoneVerifyResponse
Fields
- numberValid boolean - True if this a valid phone number
- calling boolean - True if the call is being made now
- securityCode string - The security code generated, you can save this code to perform your own verification or you can use the <a href="https://www.neutrinoapi.com/api/verify-security-code/">Verify Security Code API</a>
neutrino: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
neutrino: SmsmessageBody
Fields
- 'output\-case string - The output case style
- number string - The phone number to send a message to
- message string - The SMS message to send. Messages are truncated to a maximum of 150 characters for ASCII content OR 70 characters for UTF content
- 'country\-code string? - ISO 2-letter country code, assume numbers are based in this country. <br>If not set numbers are assumed to be in international format (with or without the leading + sign)
- 'limit int(default 0) - Limit the total number of SMS allowed to the supplied phone number, if the limit is reached error code 14 will be returned (the default is no limit)
- 'limit\-ttl int(default 1) - Set the TTL in number of days that the 'limit' option will remember a phone number (the default is 1 day and the maximum is 365 days)
neutrino: SMSMessageResponse
Fields
- numberValid boolean - True if this a valid phone number
- sent boolean - True if the SMS has been sent
neutrino: SmsverifyBody
Fields
- 'output\-case string - The output case style
- number string - The phone number to send a verification code to
- 'code\-length int(default 5) - The number of digits to use in the security code (must be between 4 and 12)
- 'security\-code int? - Pass in your own security code. This is useful if you have implemented TOTP or similar 2FA methods. If not set then we will generate a secure random code
- 'country\-code string? - ISO 2-letter country code, assume numbers are based in this country. <br>If not set numbers are assumed to be in international format (with or without the leading + sign)
- 'language\-code string(default "en") - The language to send the verification code in, available languages are: <ul> <li>de - German</li> <li>en - English</li> <li>es - Spanish</li> <li>fr - French</li> <li>it - Italian</li> <li>pt - Portuguese</li> <li>ru - Russian</li> </ul>
- 'limit int(default 0) - Limit the total number of SMS allowed to the supplied phone number, if the limit is reached error code 14 will be returned (the default is no limit)
- 'limit\-ttl int(default 1) - Set the TTL in number of days that the 'limit' option will remember a phone number (the default is 1 day and the maximum is 365 days)
neutrino: SMSVerifyResponse
Fields
- numberValid boolean - True if this a valid phone number
- securityCode string - The security code generated, you can save this code to perform your own verification or you can use the <a href="https://www.neutrinoapi.com/api/verify-security-code/">Verify Security Code API</a>
- sent boolean - True if the SMS has been sent
neutrino: Timezone
Map containing timezone details
Fields
- id string - The time zone ID as per the IANA time zone database (tzdata)
- name string - The full time zone name
- abbr string - The time zone abbreviation
- date string - The current date at the time zone (ISO 8601 format 'YYYY-MM-DD')
- time string - The current time at the time zone (ISO 8601 format 'hh:mm:ss.sss')
neutrino: UrlinfoBody
Fields
- 'output\-case string - The output case style
- url string - The URL to probe
- 'fetch\-content boolean(default false) - If this URL responds with html, text, json or xml then return the response. This option is useful if you want to perform further processing on the URL content (e.g. with the HTML Extract or HTML Clean APIs)
- 'ignore\-certificate\-errors boolean(default false) - Ignore any TLS/SSL certificate errors and load the URL anyway
- timeout int(default 60) - Timeout in seconds. Give up if still trying to load the URL after this number of seconds
- 'retry int(default 0) - If the request fails for any reason try again this many times
neutrino: URLInfoResponse
Fields
- httpStatusMessage int - The HTTP status message assoicated with the status code
- serverRegion string - The servers IP geo-location: full region name (if detectable)
- query record {} - A key-value map of the URL query paramaters
- serverName string - The name of the server software hosting this URL
- urlPort int - The URL port
- serverCountry string - The servers IP geo-location: full country name
- real boolean - Is this URL actually serving real content
- serverCity string - The servers IP geo-location: full city name (if detectable)
- urlPath string - The URL path
- url string - The fully qualified URL. This may be different to the URL requested if http-redirect is true
- valid boolean - Is this a valid well-formed URL
- serverHostname string - The servers hostname (PTR record)
- loadTime decimal - The time taken to load the URL content in seconds
- httpOk boolean - True if this URL responded with an HTTP OK (200) status
- contentSize int - The size of the URL content in bytes
- httpStatus int - The HTTP status code this URL responded with. An HTTP status of 0 indicates a network level issue
- serverCountryCode string - The servers IP geo-location: ISO 2-letter country code
- contentEncoding string - The encoding format the URL uses
- serverIp string - The IP address of the server hosting this URL
- urlProtocol string - The URL protocol, usually http or https
- contentType string - The content-type this URL serves
- httpRedirect boolean - True if this URL responded with an HTTP redirect
- content string - The actual content this URL responded with. Only set if the 'fetch-content' option was used
- isTimeout boolean - True if a timeout occurred while loading the URL. You can set the timeout with the request parameter 'timeout'
- title string - The document title
- languageCode string - The ISO 2-letter language code of the page. Extracted from either the HTML document or via HTTP headers
- isError boolean - True if an error occurred while loading the URL. This includes network errors, TLS errors and timeouts
neutrino: UseragentinfoBody
Fields
- 'output\-case string - The output case style
- 'user\-agent string - A user agent string
neutrino: UserAgentInfoResponse
Fields
- mobileScreenWidth int - The estimated mobile device screen width in CSS 'px'
- mobileBrand string - The mobile device brand
- mobileModel string - The mobile device model
- producer string - The producer or manufacturer of the user agent
- browserName string - The browser software name
- mobileScreenHeight int - The estimated mobile device screen height in CSS 'px'
- isMobile boolean - True if this is a mobile user agent
- 'type string - The user agent type, possible values are: <br> <ul> <li>desktop-browser</li> <li>mobile-browser</li> <li>email-client</li> <li>feed-reader</li> <li>software-library</li> <li>media-player (includes smart TVs)</li> <li>robot</li> <li>unknown</li> </ul>
- 'version string - The browser software version
- operatingSystem string - The full operating system name which may include the major version number or code name
- mobileBrowser string - The mobile device browser name (this is usually the same as the browser name)
- isAndroid boolean - True if this is an Android based mobile user agent
- isIos boolean - True if this is an iOS based mobile user agent
- operatingSystemFamily string - The operating system family name, this is the OS name without any version information
- operatingSystemVersion string - The operating system version number (if detectable)
- engine string - The browser engine name
- engineVersion string - The browser engine version (if detectable)
neutrino: VerifysecuritycodeBody
Fields
- 'output\-case string - The output case style
- 'security\-code string - The security code to verify
- 'limit\-by string? - If set then enable additional brute-force protection by limiting the number of attempts by the supplied value. This can be set to any unique identifier you would like to limit by, for example a hash of the users email, phone number or IP address. Requests to this API will be ignored after approximately 10 failed verification attempts
neutrino: VerifySecurityCodeResponse
Fields
- verified boolean - True if the code is valid
Import
import ballerinax/neutrino;
Metadata
Released date: over 1 year ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 1
Current verison: 1
Weekly downloads
Keywords
Website & App Building/App Builders
Cost/Freemium
Contributors
Dependencies