netsuite
Module netsuite
API
Declarations
Definitions
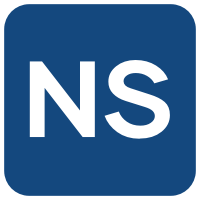
ballerinax/netsuite Ballerina library
Overview
NetSuite's SuiteTalk SOAP API provides the capability to access NetSuite operations related to different kinds of NetSuite records such as Account, Client, Transactions, Invoice, Classifications etc.
This module supports NetSuite WSDL 2020.2.0 version.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a NetSuite account
- Obtain tokens tokens
-
Go to NetSuite and sign in to your account.
-
If you have NetSuite permission to create an integration application, the following steps may be helpful. If not, proceed to step 3.
-
Enable the SuiteTalk Web service features of the account (Setup->Company->Enable Features).
-
Create an integration application (Setup->Integration->New), enable TBA code grant and scope, and obtain the following credentials:
- Client ID
- Client secret
-
-
If you have client ID, client secret from your administrator, obtain the credentials below by following the token based authorization in the NetSuite documentation.
- Access token
- Access token Secret
-
Obtain the SuitTalk Base URL that contains the account ID under the company URLs (Setup->Company->Company Information).
e.g., https://<ACCOUNT_ID>.suitetalk.api.netsuite.com
-
Quickstart
To use the NetSuite connector in your Ballerina application, update the .bal file as follows:
Step 1 - Import connector
- Create a Ballerina file and import the following Netsuite module.
import ballerinax/netsuite;
Step 2 - Create a new connector instance
- Create a NetSuite client by providing credentials. Initialize the connector by giving authentication details in the HTTP client configuration, which has built-in support for Token Based Authentication(TBA). The NetSuite connector can be initialized by the HTTP client configuration using the client ID, client secret, access token, and the access token secret. You can use the Ballerina Configuration variable to store your credentials.
public function main() returns error? { netsuite:NetsuiteConfiguration nsConfig = { accountId: "<accountId>", consumerId: "<consumerId>", consumerSecret: "<consumerSecret>", token: "<token>", tokenSecret: "<tokenSecret>", baseURL: "<webServiceURL>" }; netsuite:Client netsuiteClient = check new(nsConfig);
Step 3 - Invoke connector operation
- Invoke the connector operation using the client.
netsuite:RecordInputRef currency = { internalId: "1", 'type: "currency" }; netsuite:NewAccount account = { acctNumber: "1234567", acctName: "Ballerina NetSuite Demo Account", currency: currency }; netsuite:RecordAddResponse newAccount = check netsuiteClient->addNewAccount(account);
- Use
bal run
command to compile and run the Ballerina program.
Clients
netsuite: Client
NetSuite's SuiteTalk SOAP API provides capability to access NetSuite operations related different kind of NetSuite records such as Account, Client, Transactions, Invoice, Classifications etc.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
This connector supports NetSuite Token Based Authentication.
Follow this guide
to Obtain token for NetSuite connector configuration.
init (ConnectionConfig config, ClientConfiguration httpClientConfig)
- config ConnectionConfig - NetSuite connection configuration
- httpClientConfig ClientConfiguration {} - HTTP configuration
addNewVendor
function addNewVendor(NewVendor vendor) returns RecordAddResponse|error
Creates a new vendor record instance in NetSuite according to the given detail.
Parameters
- vendor NewVendor - Details of NetSuite record instance creation
Return Type
- RecordAddResponse|error - RecordAddResponse type record or else the relevant error
addNewVendorBill
function addNewVendorBill(NewVendorBill vendorBill) returns RecordAddResponse|error
Creates a new vendor bill record instance in NetSuite according to the given detail.
Parameters
- vendorBill NewVendorBill - Details of NetSuite record instance creation
Return Type
- RecordAddResponse|error - RecordAddResponse type record or else the relevant error
addNewCustomer
function addNewCustomer(NewCustomer customer) returns RecordAddResponse|error
Creates a new customer record instance in NetSuite according to the given detail.
Parameters
- customer NewCustomer - Details of NetSuite record instance creation
Return Type
- RecordAddResponse|error - RecordAddResponse type record or else the relevant error
addNewContact
function addNewContact(NewContact contact) returns RecordAddResponse|error
Creates a new contact record instance in NetSuite according to the given detail.
Parameters
- contact NewContact - Details of NetSuite record instance creation
Return Type
- RecordAddResponse|error - RecordAddResponse type record or else the relevant error
addNewInvoice
function addNewInvoice(NewInvoice invoice) returns RecordAddResponse|error
Creates an invoice record instance in NetSuite according to the given detail.
Parameters
- invoice NewInvoice - Invoice type record with detail
Return Type
- RecordAddResponse|error - RecordAddResponse type record otherwise the relevant error
addNewCurrency
function addNewCurrency(NewCurrency currency) returns RecordAddResponse|error
Creates a new currency record instance in NetSuite according to the given detail.
Parameters
- currency NewCurrency - Currency type record with detail
Return Type
- RecordAddResponse|error - RecordAddResponse type record otherwise the relevant error
addNewSalesOrder
function addNewSalesOrder(NewSalesOrder salesOrder) returns RecordAddResponse|error
Creates a new salesOrder record instance in NetSuite according to the given detail.
Parameters
- salesOrder NewSalesOrder - SalesOrder type record with detail
Return Type
- RecordAddResponse|error - RecordAddResponse type record otherwise the relevant error
addNewClassification
function addNewClassification(NewClassification classification) returns RecordAddResponse|error
Creates a new classification record instance in NetSuite according to the given detail.
Parameters
- classification NewClassification - Classification type record with detail
Return Type
- RecordAddResponse|error - RecordAddResponse type record otherwise the relevant error
addNewAccount
function addNewAccount(NewAccount account) returns RecordAddResponse|error
Creates an new account record instance in NetSuite according to the given detail.
Parameters
- account NewAccount - Account type record with detail
Return Type
- RecordAddResponse|error - RecordAddResponse type record otherwise the relevant error
deleteRecord
function deleteRecord(RecordDetail info) returns RecordDeletionResponse|error
Deletes a record instance from NetSuite according to the given detail if they are valid.
Parameters
- info RecordDetail - Details of NetSuite record instance to be deleted
Return Type
- RecordDeletionResponse|error - RecordDeletionResponse type record otherwise the relevant error
updateVendorRecord
function updateVendorRecord(Vendor vendor) returns RecordUpdateResponse|error
Updates a NetSuite vendor instance by internal ID.
Parameters
- vendor Vendor - Vendor record with details and internal ID
Return Type
- RecordUpdateResponse|error - RecordUpdateResponse type record otherwise the relevant error
updateVendorBillRecord
function updateVendorBillRecord(VendorBill vendorBill) returns RecordUpdateResponse|error
Updates a NetSuite vendor bill instance by internal ID.
Parameters
- vendorBill VendorBill - Vendor bill record with details and internal ID
Return Type
- RecordUpdateResponse|error - RecordUpdateResponse type record otherwise the relevant error
updateCustomerRecord
function updateCustomerRecord(Customer customer) returns RecordUpdateResponse|error
Updates a NetSuite customer instance by internal ID.
Parameters
- customer Customer - Customer record with details and internal ID
Return Type
- RecordUpdateResponse|error - RecordUpdateResponse type record otherwise the relevant error
updateContactRecord
function updateContactRecord(Contact contact) returns RecordUpdateResponse|error
Updates a NetSuite contact instance by internal ID.
Parameters
- contact Contact - Contact record with details and internal ID
Return Type
- RecordUpdateResponse|error - RecordUpdateResponse type record otherwise the relevant error
updateCurrencyRecord
function updateCurrencyRecord(Currency currency) returns RecordUpdateResponse|error
Updates a NetSuite currency instance by internal ID.
Parameters
- currency Currency - Currency record with details and internal ID
Return Type
- RecordUpdateResponse|error - RecordUpdateResponse type record otherwise the relevant error
updateInvoiceRecord
function updateInvoiceRecord(Invoice invoice) returns RecordUpdateResponse|error
Updates a NetSuite invoice instance by internal ID.
Parameters
- invoice Invoice - Invoice record with details and internalId
Return Type
- RecordUpdateResponse|error - RecordUpdateResponse type record otherwise the relevant error
updateSalesOrderRecord
function updateSalesOrderRecord(SalesOrder salesOrder) returns RecordUpdateResponse|error
Updates a NetSuite salesOrder instance by internal ID.
Parameters
- salesOrder SalesOrder - SalesOrder record with details and internalId
Return Type
- RecordUpdateResponse|error - RecordUpdateResponse type record otherwise the relevant error
updateClassificationRecord
function updateClassificationRecord(Classification classification) returns RecordUpdateResponse|error
Updates a NetSuite classification instance by internal ID.
Parameters
- classification Classification - Classification record with details and internalId
Return Type
- RecordUpdateResponse|error - RecordUpdateResponse type record otherwise the relevant error
updateAccountRecord
function updateAccountRecord(Account account) returns RecordUpdateResponse|error
Updates a NetSuite account instance by internal ID.
Parameters
- account Account - Account record with details and internal ID
Return Type
- RecordUpdateResponse|error - RecordUpdateResponse type record otherwise the relevant error
getAllCurrencyRecords
Retrieves all currency types instances from NetSuite.
Return Type
getSavedSearchIDs
function getSavedSearchIDs(string searchType) returns SavedSearchResponse|error
Retrieve a list of existing saved search IDs on a per-record-type basis.
Parameters
- searchType string - Netsuite saved search types
Return Type
- SavedSearchResponse|error - If success returns the list of saved search references otherwise the relevant error
performSavedSearchById
function performSavedSearchById(string savedSearchId, string advancedSearchType) returns stream<SearchResult, error?>|error
Perform a saved search search operation using the saved search ID.
Parameters
- savedSearchId string - Saved search ID (Internal ID)
- advancedSearchType string - Type of the saved search from the list given here: [CalendarEventSearchAdvanced, PhoneCallSearchAdvanced, FileSearchAdvanced, FolderSearchAdvanced, NoteSearchAdvanced, MessageSearchAdvanced, BinSearchAdvanced, ClassificationSearchAdvanced, DepartmentSearchAdvanced, LocationSearchAdvanced, SalesTaxItemSearchAdvanced, SubsidiarySearchAdvanced, EmployeeSearchAdvanced, CampaignSearchAdvanced, ContactSearchAdvanced, CustomerSearchAdvanced, PartnerSearchAdvanced, VendorSearchAdvanced, EntityGroupSearchAdvanced, JobSearchAdvanced, SiteCategorySearchAdvanced, SupportCaseSearchAdvanced, SolutionSearchAdvanced, TopicSearchAdvanced, IssueSearchAdvanced,CustomRecordSearchAdvanced, TimeBillSearchAdvanced, BudgetSearchAdvanced, AccountSearchAdvanced, AccountingTransactionSearchAdvanced, OpportunitySearchAdvanced, TransactionSearchAdvanced, TaskSearchAdvanced, ItemSearchAdvanced, GiftCertificateSearchAdvanced, PromotionCodeSearchAdvanced]
Return Type
- stream<SearchResult, error?>|error - Ballerina stream of json type otherwise the relevant error
searchCustomerRecords
function searchCustomerRecords(SearchElement[] searchElements) returns stream<SearchResult, error?>|error
Retrieves NetSuite client instances from NetSuite according to the given detail if they are valid.
Parameters
- searchElements SearchElement[] - Details of a NetSuite record to be retrieved from NetSuite
Return Type
- stream<SearchResult, error?>|error - Ballerina stream of customer type records otherwise the relevant error
searchTransactionRecords
function searchTransactionRecords(SearchElement[] searchElements) returns stream<SearchResult, error?>|error
Retrieves NetSuite transaction instances from NetSuite according to the given detail if they are valid.
Parameters
- searchElements SearchElement[] - Details of a NetSuite record to be retrieved from NetSuite
Return Type
- stream<SearchResult, error?>|error - Ballerina stream of transaction type records otherwise the relevant error
searchAccountRecords
function searchAccountRecords(SearchElement[] searchElements) returns stream<SearchResult, error?>|error
Retrieves NetSuite account record instances from NetSuite according to the given detail.
Parameters
- searchElements SearchElement[] - Details of a NetSuite record to be retrieved from NetSuite
Return Type
- stream<SearchResult, error?>|error - Ballerina stream of account type records otherwise the relevant error
searchContactRecords
function searchContactRecords(SearchElement[] searchElements) returns stream<SearchResult, error?>|error
Retrieves NetSuite contact record instances from NetSuite according to the given detail.
Parameters
- searchElements SearchElement[] - Details of a NetSuite record to be retrieved from NetSuite
Return Type
- stream<SearchResult, error?>|error - Ballerina stream of contact type records otherwise the relevant error
searchVendorRecords
function searchVendorRecords(SearchElement[] searchElements) returns stream<SearchResult, error?>|error
Retrieves NetSuite vendor record instances from NetSuite according to the given detail.
Parameters
- searchElements SearchElement[] - Details of a NetSuite record to be retrieved from NetSuite
Return Type
- stream<SearchResult, error?>|error - Ballerina stream of vendor type records otherwise the relevant error
getVendorRecord
function getVendorRecord(RecordInfo recordInfo) returns Vendor|error
Gets a vendor record from Netsuite by using internal ID.
Parameters
- recordInfo RecordInfo - Ballerina record for Netsuite record information
getVendorBillRecord
function getVendorBillRecord(RecordInfo recordInfo) returns VendorBill|error
Gets a vendorBill record from Netsuite by using internal ID.
Parameters
- recordInfo RecordInfo - Ballerina record for Netsuite record information
Return Type
- VendorBill|error - VendorBill type record otherwise the relevant error
getCustomerRecord
function getCustomerRecord(RecordInfo recordInfo) returns Customer|error
Gets a customer record from Netsuite by using internal ID.
Parameters
- recordInfo RecordInfo - Ballerina record for Netsuite record information
getContactRecord
function getContactRecord(RecordInfo recordInfo) returns Contact|error
Gets a contact record from Netsuite by using internal ID.
Parameters
- recordInfo RecordInfo - Ballerina record for Netsuite record information
getCurrencyRecord
function getCurrencyRecord(RecordInfo recordInfo) returns Currency|error
Gets a currency record from Netsuite by using internal ID.
Parameters
- recordInfo RecordInfo - Ballerina record for Netsuite record information
getClassificationRecord
function getClassificationRecord(RecordInfo recordInfo) returns Classification|error
Gets a currency record from Netsuite by using internal ID.
Parameters
- recordInfo RecordInfo - Ballerina record for Netsuite record information
Return Type
- Classification|error - Classification type record otherwise the relevant error
getInvoiceRecord
function getInvoiceRecord(RecordInfo recordInfo) returns Invoice|error
Gets a invoice record from Netsuite by using internal ID.
Parameters
- recordInfo RecordInfo - Ballerina record for Netsuite record information
getSalesOrderRecord
function getSalesOrderRecord(RecordInfo recordInfo) returns SalesOrder|error
Gets a salesOrder record from Netsuite by using internal ID.
Parameters
- recordInfo RecordInfo - Ballerina record for Netsuite record information
Return Type
- SalesOrder|error - SalesOrder type record otherwise the relevant error
getAccountRecord
function getAccountRecord(RecordInfo recordInfo) returns Account|error
Gets a account record from Netsuite by using internal Id.
Parameters
- recordInfo RecordInfo - Ballerina record for Netsuite record information
getNetSuiteServerTime
Returns the NetSuite server time in GMT, regardless of a user's time zone.
makeCustomRequest
Provides ability to send a SOAP based request with custom xml body elements(Child elements of Body element). You can use this function to access any other operation or record operation that the current connector doesn't support yet. Eg: urn:getServerTime/
Constants
Enums
netsuite: AccountType
Members
netsuite: AdvancedSearchTypes
Members
netsuite: BasicSearchOperator
Members
netsuite: ConsolidatedRate
Members
netsuite: Country
Members
netsuite: GlobalSubscriptionStatusType
Members
netsuite: RecordCoreType
Members
netsuite: RecordGetAllType
Members
netsuite: RecordSaveSearchType
Members
netsuite: SalesOrderStatus
Members
netsuite: SearchType
Members
netsuite: TransactionType
Members
Records
netsuite: Account
NetSuite Account type record
Fields
- internalId string - Internal ID
- externalId string? - External ID
- acctNumber string? - Account Number
- acctName string? - Account Name
- legalName string? - Legal Name of the Account
- currency RecordRef? - Account currency
- acctType _unbilledReceivable|_statistical|_otherIncome|_otherExpense|_otherCurrentLiability|_otherCurrentAsset|_otherAsset|_nonPosting|_longTermLiability|_income|_fixedAsset|_expense|_equity|_deferredRevenue|_deferredExpense|_creditCard|_costOfGoodsSold|_bank|_accountsReceivable|_accountsPayable|string - Account type
- unitsType RecordRef - Units type
- unit RecordRef - Displays the base unit assigned to the unitsType
- includeChildren boolean - checks for children
- exchangeRate string - Exchange rate
- generalRate string - General Rate of the Account
- cashFlowRate _historical|_current|_average|string - The type of exchange rate that is used to translate foreign currency amounts for this account
- billableExpensesAcct RecordRef - Track Billable Expenses in
- deferralAcct RecordRef - Deferral Account
- description string - Account description
- curDocNum decimal - Next Check Number
- isInactive boolean - Checks whether active or not
- department RecordRef - Netsuite Department
- class RecordRef - Class of the Account
- location RecordRef - Restrict to Location
- inventory boolean - If TRUE, the account balance is included in the Inventory KPI
- eliminate boolean - Eliminate Intercompany Transactions
- openingBalance decimal - Opening Balance of the account
- revalue boolean - Revalue Open Balance for Foreign Currency Transactions
- subsidiary Subsidiary - A subsidiary of the account
- anydata... -
netsuite: AccountingBookDetail
NetSuite AccountingBookDetail type record
Fields
- accountingBook RecordRef? - Accounting Book
- currency RecordRef? - The currency is used
- exchangeRate decimal? - The exchange rate
netsuite: AccountingBookDetailList
NetSuite AccountingBookDetailList type record
Fields
- replaceAll boolean(default true) - Whether to replace the current values
- accountingBookDetail AccountingBookDetail[] - An array of accounting book details
netsuite: Address
Represents an address record in NetSuite
Fields
- internalId string? - Netsuite Internal ID
- attention string? - Field Description
- addressee string? - addressee of this record
- addrPhone string? - Address phone
- addr1 string? - Address Part01
- addr2 string? - Address Part02
- addr3 string? - Address Part03
- city string? - City of the Address
- state string? - State of the Address
- zip string? - Zip code the area
- addrText string? - Address stress
- override boolean? - override the existing address
netsuite: BooleanCustomFieldRef
Represents boolean number custom Field Type in NetSuite UI
Fields
- internalId string - References a unique instance of a custom field type
- scriptId string? - Script Id
- value boolean - boolean value
netsuite: Category
NetSuite category type record
Fields
- Fields Included from *RecordRef
netsuite: Classification
Netsuite Classification type record
Fields
- internalId string - Internal ID
- name string? - Name of the classification
- parent RecordRef? - References parent classifications
- includeChildren boolean? - Checks for child classifications
- name string - Name of the classification
- includeChildren boolean - Checks for child classifications
- isInactive boolean - shows whether classification is active or not
- subsidiaryList RecordRef - Subsidiary List
- externalId string - external ID
- anydata... -
netsuite: CommonSearchResult
Fields
- pageIndex int -
- totalPages int -
- searchId string -
- totalRecords int -
- pageSize int -
netsuite: ConnectionConfig
Configuration record for NetSuite.
Fields
- accountId string - NetSuite Account ID
- consumerId string - Netsuite Integration App consumer ID
- consumerSecret string - Netsuite Integration application consumer secret
- token string - Netsuite user role access token
- tokenSecret string - Netsuite user role access secret
- baseURL string - Netsuite SuiteTalk URLs for SOAP web services (Available at Setup->Company->Company Information->Company URLs)
netsuite: Contact
Netsuite Contact type record
Fields
- internalId string - internal ID of the record
- salutation string? - The contact's salutation
- firstName string? - First name of the contact
- middleName string - Middle name of the contact
- lastName string - Last name of the contact
- entityId string - Entity ID
- title string - Contact's title at his or her company
- phone string - Main phone number
- fax string - Fax number
- email string - Email address
- defaultAddress string - The default billing address
- isPrivate boolean - If true the contact can only be viewed by the user that entered the contact record.(default: false)
- isInactive boolean - If true, this contact is not displayed on the Contacts list in the UI.(default: false)
- altEmail string - An alternate email address for this contact
- officePhone string - Office phone number
- homePhone string - Home phone number
- mobilePhone string - Mobile phone number
- supervisorPhone string - SuperVisor phone number
- assistantPhone string - Assistant Phone number
- comments string - Any other information
- image string - References an existing file
- billPay boolean - BillPay value
- dateCreated string - The date of record creation
- lastModifiedDate string - The date of the last modification
- addressBookList ContactAddressBook[] - List of Address Books
- SubscriptionsList Subscription[] - List of subscriptions
- assistant RecordRef - References to an existing contact
- supervisor RecordRef - References an existing contact
- contactSource RecordRef - The way how this contact came to do business with the company
- company RecordRef - The company this contact works for
- customForm RecordRef - NetSuite custom form record reference
- anydata... -
- subsidiary RecordRef? - The subsidiary to associate with this contact
netsuite: ContactAddressBook
NeSuite contactAddressBook type record
Fields
- defaultShipping boolean? - default shipping address
- defaultBilling boolean? - default billing address
- label string? - contactBookLabel
- addressBookAddress Address[]? - AddressBook address
- internalId string? - InternalID of the record
netsuite: Currency
Netsuite Currency type record
Fields
- internalId string - Internal ID of the currency record
- name string? - Name of the record
- symbol string? - Symbol of the record
- exchangeRate decimal? - The exchange rate of the currency
- displaySymbol string - Display symbol of the currency type
- currencyPrecision string - Precision symbol of the currency type
- isInactive boolean - Shows whether the currency type is active or not
- isBaseCurrency boolean - Shows whether the currency type is a NetSuite base currency or not
- anydata... -
netsuite: Customer
Netsuite Customer type record
Fields
- internalId string - Internal ID of the customer record
- salutation string - The title of this person
- firstName string - First name
- middleName string - Middle name
- lastName string - Last name
- phone string - Main phone number
- fax string - Fax phone number
- email string - Email Address
- defaultAddress string - Default Address
- title string - The job title
- homePhone string - Home phone number
- mobilePhone string - Field Description
- accountNumber string - Field Description
- entityId string - Entity ID
- isInactive boolean - This field is false by default, shows whether active or not
- isPerson boolean - This is set to True which specifies the type
- category RecordRef - References a value in a user defined list at Setup
- addressbookList CustomerAddressbook[] - Field Description
- currencyList CustomerCurrency[] - Field Description
- anydata... -
- companyName string? - Company name
- subsidiary RecordRef? - Selects the subsidiary to associate with this entity or job
netsuite: CustomerAddressbook
Netsuite CustomerAddressbook type record
Fields
- Fields Included from *ContactAddressBook
- isResidential boolean - Whether addressBook is residential or not
netsuite: CustomerCurrency
NetSuite CustomerCurrency type record
Fields
- currency RecordRef? - The NetSuite currency
- balance decimal? - balance of the customerCurrency
- consolBalance decimal? - Consolidated balance
- depositBalance decimal? - Deposit Balance
- consolDepositBalance decimal? - Consolidated Deposit
- overdueBalance decimal? - OverDue Balance
- consolOverdueBalance decimal? - Consolidated overdue balance
- unbilledOrders decimal? - Unbilled orders
- consolUnbilledOrders decimal? - Consolidated unbilled orders
- overrideCurrencyFormat boolean? - checks whether override the currency format
netsuite: CustomFieldList
Represents NetSuite CustomFieldList type record
Fields
- customFields CustomField[] - An array of customFields
netsuite: DoubleCustomFieldRef
Represents decimal number custom Field Type in NetSuite UI
Fields
- internalId string - References a unique instance of a custom field type
- scriptId string? - Script Id
- value decimal - decimal value
netsuite: Installment
NetSuite Installment type record
Fields
- amount string? - The amount
- dueDate string? - The due date
- amountDue decimal? - The due amount
- seqNum int? - Sequence number
- status string? - The status
netsuite: InventoryAssignment
NetSuite InventoryAssignment type record
Fields
- internalId string? - The internal ID of the inventory assignment
- issueInventoryNumber RecordRef? - The issue inventory number
- receiptInventoryNumber string? - The receipt inventory number
- binNumber RecordRef? - Bin number
- toBinNumber RecordRef? - To Bin number
- quantity decimal? - The quantity
- expirationDate string? - The expiration date
- quantityAvailable decimal? - The available quantity
- inventoryStatus RecordRef? - The inventory status
- toInventoryStatus RecordRef? - To inventory status
netsuite: InventoryAssignmentList
NetSuite InventoryAssignmentList type record
Fields
- inventoryAssignment InventoryAssignment[] - An array of inventory assignments
- customForm RecordRef - Reference to a custom form
netsuite: Invoice
Represents a NetSuite Invoice record
Fields
- internalId string - The internalId of the invoice
- entity RecordRef? - The customer of the invoice
- itemList Item[]? - The item list for the invoice
- invoiceId string? - The ID of the invoice
- Fields Included from *InvoiceCommon
netsuite: InvoiceCommon
Represents a NetSuite Invoice record
Fields
- recognizedRevenue decimal? - Recognized Revenue: cumulative amount of revenue recognized for this transaction
- discountTotal decimal? - The amount discounted on this invoice
- deferredRevenue decimal? - The amount of revenue deferred on this transaction
- total decimal? - The total of line items, tax and shipping costs
- email string? - References an email for the invoice
- createdDate string? - Created date of the invoice
- lastModifiedDate string? - The last modified Date of the invoice
- status string? - The status of the Invoice
- classification Classification? - The classification of the invoice
- currency RecordRef? - The currency of the invoice
- 'class RecordRef? - The class of the invoice
- department RecordRef? - A department to associate with this invoice
- subsidiary RecordRef? - The subsidiary of the invoice
netsuite: Item
NetSuite general Item record
Fields
- subscription RecordRef? - Subscription of salesOrderItem
- item RecordRef - References an item type record
- quantityAvailable decimal? - The available quantity
- quantityOnHand decimal? - Sets the quantity on hand for this item
- quantity decimal? - Quantity of the item
- units RecordRef? - Number of item units
- description string? - Item description
- price RecordRef? - Price of the item
- rate string? - Defines the rate for this item.
- amount decimal - Amount of the item
- isTaxable boolean? - Shows whether item is taxable
- location RecordRef? - Locations for details
netsuite: LandedCostData
NetSuite LandedCostData type record
Fields
- costCategory RecordRef? - The cost category
- amount decimal? - The amount
netsuite: LandedCostDataList
NetSuite LandedCostDataList type record
Fields
- landedCostData LandedCostData[] - An array of landed cost data
netsuite: LandedCostSummary
NetSuite LandedCostSummary type record
Fields
- category RecordRef? - The category of the landed cost summary
- amount decimal? - The amount
- 'source string? - The source of the landed cost summary
- 'transaction RecordRef? - Reference to landed cost summary
netsuite: ListOrRecordRef
Represents an array of type RecordRef
Fields
- recordName string - name of the record
- internalId string - internal Id of the record ref
netsuite: LongCustomFieldRef
Represents integer custom Field Type in NetSuite UI
Fields
- internalId string - References a unique instance of a custom field type
- scriptId string? - Script Id
- value int - Integer value
netsuite: MultiSelectCustomFieldRef
Represents Multiple Select custom Field Type in NetSuite UI
Fields
- internalId string - References a unique instance of a custom field type
- scriptId string? - Script Id
- value ListOrRecordRef[] - An array of lists/records or documents
netsuite: NewAccount
NetSuite Account type record
Fields
- acctNumber string - Account Number
- acctName string - Account Name
- currency RecordInputRef? - Account Currency Detail(Type: netsuite:CURRENCY)
- acctType _unbilledReceivable|_statistical|_otherIncome|_otherExpense|_otherCurrentLiability|_otherCurrentAsset|_otherAsset|_nonPosting|_longTermLiability|_income|_fixedAsset|_expense|_equity|_deferredRevenue|_deferredExpense|_creditCard|_costOfGoodsSold|_bank|_accountsReceivable|_accountsPayable|string - Account type
- unitsType RecordRef - Units type
- unit RecordRef - Displays the base unit assigned to the unitsType
- includeChildren boolean - checks for children
- exchangeRate string - Exchange rate
- generalRate string - General Rate of the Account
- cashFlowRate _historical|_current|_average|string - The type of exchange rate that is used to translate foreign currency amounts for this account
- billableExpensesAcct RecordRef - Track Billable Expenses in
- deferralAcct RecordRef - Deferral Account
- description string - Account description
- curDocNum decimal - Next Check Number
- isInactive boolean - Checks whether active or not
- department RecordRef - Netsuite Department
- class RecordRef - Class of the Account
- location RecordRef - Restrict to Location
- inventory boolean - If TRUE, the account balance is included in the Inventory KPI
- eliminate boolean - Eliminate Intercompany Transactions
- openingBalance decimal - Opening Balance of the account
- revalue boolean - Revalue Open Balance for Foreign Currency Transactions
- subsidiary Subsidiary - A subsidiary of the account
- anydata... -
netsuite: NewClassification
Netsuite Classification type record
Fields
- name string - Name of the classification
- parent RecordInputRef? - References parent classifications
- name string - Name of the classification
- includeChildren boolean - Checks for child classifications
- isInactive boolean - shows whether classification is active or not
- subsidiaryList RecordRef - Subsidiary List
- externalId string - external ID
- anydata... -
netsuite: NewContact
Netsuite Contact type record
Fields
- firstName string - First name of the contact
- subsidiary RecordInputRef - The subsidiary to associate with this contact
- middleName string - Middle name of the contact
- lastName string - Last name of the contact
- entityId string - Entity ID
- title string - Contact's title at his or her company
- phone string - Main phone number
- fax string - Fax number
- email string - Email address
- defaultAddress string - The default billing address
- isPrivate boolean - If true the contact can only be viewed by the user that entered the contact record.(default: false)
- isInactive boolean - If true, this contact is not displayed on the Contacts list in the UI.(default: false)
- altEmail string - An alternate email address for this contact
- officePhone string - Office phone number
- homePhone string - Home phone number
- mobilePhone string - Mobile phone number
- supervisorPhone string - SuperVisor phone number
- assistantPhone string - Assistant Phone number
- comments string - Any other information
- image string - References an existing file
- billPay boolean - BillPay value
- dateCreated string - The date of record creation
- lastModifiedDate string - The date of the last modification
- addressBookList ContactAddressBook[] - List of Address Books
- SubscriptionsList Subscription[] - List of subscriptions
- assistant RecordRef - References to an existing contact
- supervisor RecordRef - References an existing contact
- contactSource RecordRef - The way how this contact came to do business with the company
- company RecordRef - The company this contact works for
- customForm RecordRef - NetSuite custom form record reference
- anydata... -
netsuite: NewCurrency
Netsuite Currency type record
Fields
- name string - Name of the record
- symbol string - Symbol of the record
- exchangeRate decimal - The exchange rate of the currency
- displaySymbol string - Display symbol of the currency type
- currencyPrecision string - Precision symbol of the currency type
- isInactive boolean - Shows whether the currency type is active or not
- isBaseCurrency boolean - Shows whether the currency type is a NetSuite base currency or not
- anydata... -
netsuite: NewCustomer
Netsuite Customer type record
Fields
- companyName string - Company name
- subsidiary RecordInputRef - Selects the subsidiary to associate with this entity or job
- salutation string - The title of this person
- firstName string - First name
- middleName string - Middle name
- lastName string - Last name
- phone string - Main phone number
- fax string - Fax phone number
- email string - Email Address
- defaultAddress string - Default Address
- title string - The job title
- homePhone string - Home phone number
- mobilePhone string - Field Description
- accountNumber string - Field Description
- entityId string - Entity ID
- isInactive boolean - This field is false by default, shows whether active or not
- isPerson boolean - This is set to True which specifies the type
- category RecordRef - References a value in a user defined list at Setup
- addressbookList CustomerAddressbook[] - Field Description
- currencyList CustomerCurrency[] - Field Description
- anydata... -
netsuite: NewInvoice
Represents a NetSuite Invoice record
Fields
- entity RecordInputRef - The customer of the invoice
- itemList Item[] - The item list for the invoice
- Fields Included from *InvoiceCommon
netsuite: NewSalesOrder
Netsuite Sales Order type record
Fields
- entity RecordInputRef - The customer of the sales Order
- itemList Item[] - The list of items
- Fields Included from *SalesOrderCommon
- internalId string
- createdDate string
- tranDate string
- tranId string
- orderStatus _undefined|_closed|_fullyBilled|_pendingBilling|_pendingBillingPartFulfilled|_partiallyFulfilled|_cancelled|_pendingFulfillment|_pendingApproval|string
- nextBill string
- startDate string
- endDate string
- memo string
- excludeCommission boolean
- totalCostEstimate decimal|()
- estGrossProfit decimal|()
- estGrossProfitPercent decimal|()
- exchangeRate decimal|()
- currencyName string
- isTaxable boolean
- email string
- shipDate string
- subTotal decimal|()
- discountTotal decimal|()
- total decimal|()
- balance decimal|()
- status string
- billingAddress Address
- shippingAddress Address
- subsidiary RecordRef
- customForm RecordRef
- currency RecordRef
- drAccount RecordRef
- fxAccount RecordRef
- opportunity RecordRef
- salesRep RecordRef
- partner RecordRef
- salesGroup RecordRef
- leadSource RecordRef
- entityTaxRegNum RecordRef
- createdFrom RecordRef
- anydata...
netsuite: NewVendor
NetSuite Vendor type record
Fields
- companyName string - Company Name
- subsidiary RecordRef - Refers the subsidiary
- Fields Included from *VendorCommon
- customForm RecordRef
- externalId string
- entityId string
- altName string
- isPerson boolean
- phoneticName string
- salutation string
- firstName string
- middleName string
- lastName string
- phone string
- fax string
- email string
- url string
- defaultAddress string
- isInactive boolean
- lastModifiedDate string
- dateCreated string
- category RecordRef
- title string
- printOnCheckAs string
- altPhone string
- homePhone string
- mobilePhone string
- altEmail string
- comments string
- image RecordRef
- representingSubsidiary RecordRef
- accountNumber string
- legalName string
- vatRegNumber string
- expenseAccount RecordRef
- payablesAccount RecordRef
- terms RecordRef
- incoterm RecordRef
- creditLimit decimal|()
- balancePrimary decimal|()
- openingBalance decimal|()
- openingBalanceDate string
- openingBalanceAccount RecordRef
- balance decimal|()
- unbilledOrdersPrimary decimal|()
- bcn string
- unbilledOrders decimal|()
- currency RecordRef
- is1099Eligible boolean
- isJobResourceVend boolean
- laborCost decimal|()
- purchaseOrderQuantity decimal|()
- purchaseOrderAmount decimal|()
- purchaseOrderQuantityDiff decimal|()
- receiptQuantity decimal|()
- receiptAmount decimal|()
- receiptQuantityDiff decimal|()
- workCalendar RecordRef
- taxIdNum string
- taxItem RecordRef
- giveAccess boolean
- sendEmail boolean
- billPay boolean
- isAccountant boolean
- password string
- password2 string
- requirePwdChange boolean
- eligibleForCommission boolean
- emailTransactions boolean
- printTransactions boolean
- faxTransactions boolean
- defaultTaxReg RecordRef
- predictedDays int|()
- predConfidence decimal|()
- customFieldList CustomFieldList
- anydata...
netsuite: NewVendorBill
NetSuite VendorBill type record
Fields
- subsidiary RecordRef? - The subsidiary reference
- entity RecordRef - Vendor of the Bill
- Fields Included from *VendorBillCommon
- createdDate string
- lastModifiedDate string
- nexus RecordRef
- subsidiaryTaxRegNum RecordRef
- taxRegOverride boolean
- taxDetailsOverride boolean
- customForm RecordRef
- billAddressList RecordRef
- account RecordRef
- approvalStatus RecordRef
- nextApprover RecordRef
- vatRegNum string
- postingPeriod RecordRef
- tranDate string
- currencyName string
- billingAddress Address
- exchangeRate decimal|()
- entityTaxRegNum RecordRef
- taxPointDate string
- terms RecordRef
- dueDate string
- discountDate string
- tranId string
- userTotal decimal|()
- discountAmount decimal|()
- taxTotal decimal|()
- paymentHold boolean
- memo string
- tax2Total decimal|()
- creditLimit decimal|()
- availableVendorCredit decimal|()
- currency RecordRef
- class RecordRef
- department RecordRef
- location RecordRef
- status string
- landedCostMethod string
- landedCostPerLine boolean
- transactionNumber string
- overrideInstallments boolean
- expenseList VendorBillExpenseList
- accountingBookDetailList AccountingBookDetailList
- itemList VendorBillItemList
- installmentList Installment[]
- landedCostsList PurchLandedCostList
- purchaseOrderList RecordRef[]
- taxDetailsList TaxDetailsList
- customFieldList CustomFieldList
- anydata...
netsuite: PurchLandedCostList
Represents PurchLandedCostList type record
Fields
- replaceAll boolean(default true) - Whether to replace current values
- landedCosts LandedCostSummary[] - The landed costs
netsuite: RecordAddResponse
Ballerina record for netsuite record creation response
Fields
- isSuccess boolean - Boolean for checking submission NetSuite failures
- afterSubmitFailed boolean? - Boolean for checking After submission NetSuite failures
- internalId string - NetSuite record ID
- recordType string - Netsuite record type
- warning string? - Netsuite warnings
netsuite: RecordBaseRef
Represents record reference base to NetSuite Records
Fields
- internalId string - NetSuite Internal ID
- name string? - Name of the Record
- externalId string? - NetSuite external ID
netsuite: RecordDeletionResponse
Ballerina record for Netsuite record deletion response
Fields
- Fields Included from *RecordAddResponse
netsuite: RecordDetail
Ballerina record for Netsuite record delete response
Fields
- Fields Included from *RecordInfo
- deletionReasonId string? - Reason ID for deletion
- deletionReasonMemo string? - NetSuite Reason memo for deletion
netsuite: RecordInfo
Ballerina record for Netsuite record delete response
Fields
- recordType string - NetSuite Record type Eg: "currency","invoice", netsuite:INVOICE etc.
- recordInternalId string - Internal ID of the Netsuite record
netsuite: RecordInputRef
References to NetSuite Records for Input operations
Fields
- Fields Included from *RecordBaseRef
- 'type string - Type of the Record Eg: "currency" or netsuite:CURRENCY
netsuite: RecordList
Ballerina record for storing search results
Fields
- records RecordRef[] - Array of record references
netsuite: RecordRef
References to NetSuite Records
Fields
- Fields Included from *RecordBaseRef
- 'type string - Type of the Record
netsuite: RecordUpdateResponse
Ballerina record for Netsuite record update response
Fields
- Fields Included from *RecordAddResponse
netsuite: SalesOrder
Netsuite Sales Order type record
Fields
- internalId string - Internal ID of the SalesOrder record
- entity RecordRef? - The customer of the sales Order
- itemList Item[]? - The list of items
- Fields Included from *SalesOrderCommon
- internalId string
- createdDate string
- tranDate string
- tranId string
- orderStatus _undefined|_closed|_fullyBilled|_pendingBilling|_pendingBillingPartFulfilled|_partiallyFulfilled|_cancelled|_pendingFulfillment|_pendingApproval|string
- nextBill string
- startDate string
- endDate string
- memo string
- excludeCommission boolean
- totalCostEstimate decimal|()
- estGrossProfit decimal|()
- estGrossProfitPercent decimal|()
- exchangeRate decimal|()
- currencyName string
- isTaxable boolean
- email string
- shipDate string
- subTotal decimal|()
- discountTotal decimal|()
- total decimal|()
- balance decimal|()
- status string
- billingAddress Address
- shippingAddress Address
- subsidiary RecordRef
- customForm RecordRef
- currency RecordRef
- drAccount RecordRef
- fxAccount RecordRef
- opportunity RecordRef
- salesRep RecordRef
- partner RecordRef
- salesGroup RecordRef
- leadSource RecordRef
- entityTaxRegNum RecordRef
- createdFrom RecordRef
- anydata...
netsuite: SalesOrderCommon
Netsuite Sales Order type record
Fields
- internalId string? - InternalId of the salesOrder record in Netsuite
- createdDate string? - created date of the salesOrder record in Netsuite
- tranDate string? - The posting date of this sales order
- tranId string? - Sales Order number
- orderStatus SalesOrderStatus|string? - status of sales orders
- nextBill string? - Date of the next bill
- startDate string? - The date for the first invoice to be created
- endDate string? - The end date of the order
- memo string? - A memo to describe this sales order
- excludeCommission boolean? - Option to exclude this transaction
- totalCostEstimate decimal? - Estimated Cost
- estGrossProfit decimal? - Estimated Gross Profit
- estGrossProfitPercent decimal? - Estimated Gross Profit Margin
- exchangeRate decimal? - The currency's exchange rate
- currencyName string? - Name of the currency
- isTaxable boolean? - A check mark in this box if this order is taxable
- email string? - The email address
- shipDate string? - Type or pick a shipping date for this order
- subTotal decimal? - Total before any discounts, shipping cost, handling cost or tax
- discountTotal decimal? - NetSuite enters the amount discounted on this sales order
- total decimal? - The total of line items, tax and shipping costs
- balance decimal? - The balance owed by this customer
- status string? - Status of the sales Order
- billingAddress Address? - The billing address
- shippingAddress Address? - The shipping address
- subsidiary RecordRef? - Subsidiary of the Sales Order
- customForm RecordRef? - References the customized sales order form
- currency RecordRef? - The currency of the sales Order
- drAccount RecordRef? - Deferred revenue reclassification account
- fxAccount RecordRef? - Foreign currency adjustment revenue account
- opportunity RecordRef? - References an Netsuite Opportunity
- salesRep RecordRef? - The sales representative associated with the company on the customer record
- partner RecordRef? - A partner to associate with this transaction
- salesGroup RecordRef? - A sales team to associate with this transaction
- leadSource RecordRef? - The lead source associated with this transaction
- entityTaxRegNum RecordRef? - The customer's tax registration number associated with this sales order
- createdFrom RecordRef? - The opportunity or estimate used to create this sales order
netsuite: SavedSearchReference
Saved search reference
Fields
- internalId string - Internal Id of the saved search record
- scriptId string - ScriptId of the saved search
- name string - Name of the Saved search
netsuite: SavedSearchResponse
Netsuite saveSearch list response record
Fields
- status boolean - Boolean for checking submission NetSuite failures
- totalReferences int - The total number of records for this search. Depending on the pageSize value, some or all the records may be returned in this response
- recordRefList SavedSearchReference[] - Netsuite record reference list
netsuite: SearchElement
Ballerina records for search operation
Fields
- fieldName string - Name of the search field (Eg: name, type)
- operator string|BasicSearchOperator - Searching operator (optional for boolean searches)
- searchType SearchType - Netsuite search field type
- value1 string(default EMPTY_STRING) - Primary search value
- value2 string? - Secondary search value
- multiValues string[]? - An array of strings for multi values in case there are more values excepts primary and secondary
- multiSelectValues RecordRef[]? - An array of record references for Search Multi Select Field.
netsuite: SearchResult
Represents search results
Fields
- commonSearchResult CommonSearchResult - Description on search results
netsuite: SelectCustomFieldRef
Represents list/record, document custom Field Type in NetSuite UI
Fields
- internalId string - References a unique instance of a custom field type
- scriptId string? - Script Id
- value ListOrRecordRef - The list/record or document
netsuite: StringOrDateCustomFieldRef
Represents free-form text, text area, phone number, e-mail address, hyperlink, rich text custom Field Type, date and time in NetSuite UI
Fields
- internalId string - References a unique instance of a custom field type
- scriptId string? - Script Id
- value string - Custom field value
netsuite: Subscription
Netsuite Subscription type record
Fields
- subscribed boolean - Subscription ID
- subscription RecordRef - Subscription Detail
- lastModifiedDate string - Last modified date of the subscription
netsuite: Subsidiary
Netsuite Subsidiary type record
Fields
- name string? - Name of the subsidiary
- country string? - Country of the subsidiary
- email string? - Email of the subsidiary
- isElimination boolean? - The elimination status of the subsidiary
- isInactive boolean? - Shows whether subsidiary is active or not
- legalName string? - Legal name of the subsidiary
- url string? - URL for the subsidiary
netsuite: TaxDetails
Represents tax details
Fields
- taxDetailsReference string? - Tax details reference
- lineType string? - Type of the line
- lineName string? - Name of the line
- netAmount decimal? - Net amount
- grossAmount decimal? - Gross amount
- taxType RecordRef? - Type of the tax
- taxCode RecordRef? - Tax code
- taxBasis decimal? - Tax basis
- taxRate decimal? - Tax rates
- taxAmount decimal? - Tax amount
- calcDetail string? - Calculation details
netsuite: TaxDetailsList
Represents TaxDetailsList type record
Fields
- replaceAll boolean(default true) - Whether to replace current values
- taxDetails TaxDetails[] - Array of tax detail records
netsuite: Vendor
NetSuite Vendor type record
Fields
- internalId string - Internal ID
- companyName string? - The Company name
- subsidiary RecordRef? - Refers subsidiary
- Fields Included from *VendorCommon
- customForm RecordRef
- externalId string
- entityId string
- altName string
- isPerson boolean
- phoneticName string
- salutation string
- firstName string
- middleName string
- lastName string
- phone string
- fax string
- email string
- url string
- defaultAddress string
- isInactive boolean
- lastModifiedDate string
- dateCreated string
- category RecordRef
- title string
- printOnCheckAs string
- altPhone string
- homePhone string
- mobilePhone string
- altEmail string
- comments string
- image RecordRef
- representingSubsidiary RecordRef
- accountNumber string
- legalName string
- vatRegNumber string
- expenseAccount RecordRef
- payablesAccount RecordRef
- terms RecordRef
- incoterm RecordRef
- creditLimit decimal|()
- balancePrimary decimal|()
- openingBalance decimal|()
- openingBalanceDate string
- openingBalanceAccount RecordRef
- balance decimal|()
- unbilledOrdersPrimary decimal|()
- bcn string
- unbilledOrders decimal|()
- currency RecordRef
- is1099Eligible boolean
- isJobResourceVend boolean
- laborCost decimal|()
- purchaseOrderQuantity decimal|()
- purchaseOrderAmount decimal|()
- purchaseOrderQuantityDiff decimal|()
- receiptQuantity decimal|()
- receiptAmount decimal|()
- receiptQuantityDiff decimal|()
- workCalendar RecordRef
- taxIdNum string
- taxItem RecordRef
- giveAccess boolean
- sendEmail boolean
- billPay boolean
- isAccountant boolean
- password string
- password2 string
- requirePwdChange boolean
- eligibleForCommission boolean
- emailTransactions boolean
- printTransactions boolean
- faxTransactions boolean
- defaultTaxReg RecordRef
- predictedDays int|()
- predConfidence decimal|()
- customFieldList CustomFieldList
- anydata...
netsuite: VendorBill
NetSuite VendorBill type record
Fields
- internalId string - Internal Id of the Bill
- subsidiary RecordRef? - The subsidiary of the bill
- entity RecordRef? - The vendor of the bill
- Fields Included from *VendorBillCommon
- createdDate string
- lastModifiedDate string
- nexus RecordRef
- subsidiaryTaxRegNum RecordRef
- taxRegOverride boolean
- taxDetailsOverride boolean
- customForm RecordRef
- billAddressList RecordRef
- account RecordRef
- approvalStatus RecordRef
- nextApprover RecordRef
- vatRegNum string
- postingPeriod RecordRef
- tranDate string
- currencyName string
- billingAddress Address
- exchangeRate decimal|()
- entityTaxRegNum RecordRef
- taxPointDate string
- terms RecordRef
- dueDate string
- discountDate string
- tranId string
- userTotal decimal|()
- discountAmount decimal|()
- taxTotal decimal|()
- paymentHold boolean
- memo string
- tax2Total decimal|()
- creditLimit decimal|()
- availableVendorCredit decimal|()
- currency RecordRef
- class RecordRef
- department RecordRef
- location RecordRef
- status string
- landedCostMethod string
- landedCostPerLine boolean
- transactionNumber string
- overrideInstallments boolean
- expenseList VendorBillExpenseList
- accountingBookDetailList AccountingBookDetailList
- itemList VendorBillItemList
- installmentList Installment[]
- landedCostsList PurchLandedCostList
- purchaseOrderList RecordRef[]
- taxDetailsList TaxDetailsList
- customFieldList CustomFieldList
- anydata...
netsuite: VendorBillCommon
NetSuite VendorBill type record
Fields
- createdDate string? - The created date of the record
- lastModifiedDate string? - The last modified date of the record
- nexus RecordRef? - This field shows the nexus of the transaction. NetSuite automatically populates this field based on the nexus lookup logic. You can override the transaction nexus and tax registration number that NetSuite automatically selects by checking the Nexus Override box. When you select a different nexus in the dropdown list, the corresponding tax registration number is automatically selected in the Subsidiary Tax Reg. Number field
- subsidiaryTaxRegNum RecordRef? - This field shows the tax registration number of the transaction nexus. NetSuite automatically populates this field based on the nexus lookup logic
- taxRegOverride boolean? - Overrides the values in the Nexus and Subsidiary Tax Reg. Number fields
- taxDetailsOverride boolean? - Override the tax information on the Tax Details subtab of the transaction
- customForm RecordRef? - The appropriate standard or custom form to use.
- billAddressList RecordRef? - The billing address displayed in the Vendor field
- account RecordRef? - The Accounts Payable account that will be affected by this transaction
- approvalStatus RecordRef? - The approval status of this bill
- nextApprover RecordRef? - The next person set to approve this bill via approval routing
- vatRegNum string? - The vat Registration Number
- postingPeriod RecordRef? - Required on Add when the Accounting Periods feature is enabled
- tranDate string? - Required on Add. Defaults to the current date
- currencyName string? - A read only field that defaults to the currency associated with the customer referenced by the entity field
- billingAddress Address? - The billing address of the bill
- exchangeRate decimal? - Required on Add. Defaults to the rate associated with the Vendor set in the entity field.
- entityTaxRegNum RecordRef? - The tax registration number for this entity displays here
- taxPointDate string? - Tax point date is a legal requirement in many countries
- terms RecordRef? - NetSuite multiplies the tax rate by the taxable total of line items and enters it here
- dueDate string? - Sets the due date for the bill. Defaults to the current date.
- discountDate string? - A read-only field that returns the last day you can pay this bill in order to receive a discount
- tranId string? - Sets the number to identify this transaction, such as the vendor's invoice number.
- userTotal decimal? - A read-only field that returns the total amount of the transaction
- discountAmount decimal? - A read-only field that returns the amount discounted on this bill
- taxTotal decimal? - NetSuite multiplies the tax rate by the taxable total of line items and enters it here
- paymentHold boolean? - The Payment Hold box to apply a payment hold on a disputed bill
- memo string? - A memo that will appear on such reports as the 2-line Accounts Payable Register
- tax2Total decimal? - NetSuite multiplies the tax rate by the taxable total of line items and enters it here
- creditLimit decimal? - This is a read-only field that returns the credit limit set for the vendor
- availableVendorCredit decimal? - From the web services perspective, this field is available only with the get, getList, and asyncGetList operations
- currency RecordRef? - The transaction currency for this bill is shown here
- 'class RecordRef? - The class that applies to this item
- department RecordRef? - References a value in a user-defined list at Setup > Company > Classifications > Departments
- location RecordRef? - A location to associate with this line item
- status string? - VendorBill status
- landedCostMethod string? - Available values are: _quantity _value _weight
- landedCostPerLine boolean? - Check this box to enter a landed cost per line item
- transactionNumber string? - By default, transaction lists display only the Number field in searches and reporting
- overrideInstallments boolean? - This box to override the default calculated installment amounts and enter custom ones
- expenseList VendorBillExpenseList? - The expense list
- accountingBookDetailList AccountingBookDetailList? - The accounting book list
- itemList VendorBillItemList? - The item list
- installmentList Installment[]? - The installment list
- landedCostsList PurchLandedCostList? - The landed costs list
- purchaseOrderList RecordRef[]? - The purchase order list
- taxDetailsList TaxDetailsList? - The tax details list
- customFieldList CustomFieldList? - The custom field list
netsuite: VendorBillExpense
NetSuite VendorBillExpense type record
Fields
- orderDoc int? - Netsuite expenses orderDoc value
- orderLine int? - The order line
- line int - The line number of Netsuite list
- category RecordRef? - The expense category
- account RecordRef? - The Accounts Payable account that will be affected by this transaction
- amount decimal - The expense amount
- taxAmount decimal? - The tax amount
- tax1Amt decimal? - The tax amount
- memo string? - A memo that will appear on such reports as the 2-line Accounts Payable Register
- grossAmt decimal? - The gross amount
- taxDetailsReference string? - Tax details reference
- department RecordRef? - The department that applies to this item
- 'class RecordRef? - The class that applies to this item
- location RecordRef? - The location of the item
- customer RecordRef? - The Customer of the expense
- isBillable boolean? - Whether this is billable or not
- projectTask RecordRef? - The project task
- taxCode RecordRef? - Tax code
- taxRate1 decimal? - Tax rate 1
- taxRate2 decimal? - Tax rate 2
- amortizationSched RecordRef? - Amortization schedule
- amortizStartDate string? - Amortization start date
- amortizationEndDate string? - Amortization end date
- amortizationResidual string? - Amortization residual
- customFieldList CustomFieldList? - Custom field list
netsuite: VendorBillExpenseList
NetSuite VendorBillExpenseList type record
Fields
- replaceAll boolean(default true) - Whether to replace current values
- expenses VendorBillExpense[] - An array of expenses
netsuite: VendorBillItem
NetSuite VendorBillItem type record
Fields
- item RecordRef? - The vendorBill item
- vendorName string? - Name of the vendor
- line int? - The line number
- orderDoc int? - The order doc
- orderLine int? - The order line
- quantity decimal? - The quantity
- unit RecordRef? - The unit
- inventoryDetail InventoryAssignmentList? - The inventory details
- description string? - Description on the item
- serialNumbers string? - Serial num
- binNumbers string? - The bin numbers
- expirationDate string? - The expiration date
- taxCode RecordRef? - Tax code
- taxRate1 decimal? - Tax rate1
- taxRate2 decimal? - Tax rate2
- grossAmt decimal? - Gross amount
- tax1Amt decimal? - Tax amount
- rate string? - The vendorBillItem rate value
- amount decimal? - The amount
- options CustomFieldList? - The options of the vendorBillItem
- department RecordRef? - The department of the item
- 'class RecordRef? - The class of the item
- location RecordRef? - The location of the item
- customer RecordRef? - The customer of the item
- landedCostCategory RecordRef? - The landed cost category
- isBillable boolean? - Whether item is billable or not
- billVarianceStatus string? - The bill variance status
- billreceiptsList RecordRef[]? - The bill receipt list
- amortizStartDate string? - Amortization start date
- amortizationEndDate string? - Amortization end date
- amortizationResidual string? - Amortization residual
- taxAmount decimal? - The tax amount
- taxDetailsReference string? - The Details reference
- landedCost LandedCostDataList? - The landed cost
- customFieldList CustomFieldList? - The custom field list
netsuite: VendorBillItemList
NetSuite VendorBillItemList type record
Fields
- replaceAll boolean(default true) - Whether to replace current values
- item VendorBillItem[] - An array of VendorBillItem records
netsuite: VendorCommon
NetSuite Vendor type record
Fields
- customForm RecordRef? - Refers the custom form
- externalId string? - The external ID
- entityId string? - The entity ID
- altName string? - This is the name of this person or company
- isPerson boolean? - By default, this is set to True which specifies the type of vendor record as Individual. If set to False, the vendor record is set as a Company type
- phoneticName string? - The furigana character you want to use to sort this record
- salutation string? - The salutation
- firstName string? - First name
- middleName string? - Middle name
- lastName string? - Last name
- phone string? - Phone number
- fax string? - Fax
- email string? - Email
- url string? - Only available when isPerson is set to FALSE
- defaultAddress string? - Read-only field that returns the default address for the vendor
- isInactive boolean? - This field is false by default
- lastModifiedDate string? - The last modified date
- dateCreated string? - The record created date
- category RecordRef? - References a value in a user-defined list at Lists > Accounting > Currencies. This value sets the currency that all transactions involving this vendor are conducted in.
- title string? - Job title
- printOnCheckAs string? - Sets the Pay to the Order of line of a check instead of what you entered in the Vendor field
- altPhone string? - Phone numbers can be entered in the following formats: 999-999-9999, 1-999-999-9999, (999) 999-9999, 1(999) 999-9999 or 999-999-9999 ext 9999
- homePhone string? - Home page
- mobilePhone string? - Mobile Phone
- altEmail string? - Email
- comments string? - Any other information you wish to track for this vendor
- image RecordRef? - References an image file that has already been uploaded to the account
- representingSubsidiary RecordRef? - Indicates that this entity is an intercompany vendor
- accountNumber string? - The account number for vendors to reference
- legalName string? - The legal name for this vendor for financial purposes
- vatRegNumber string? - For the UK edition only. Note that this field is not validated when submitted via Web services.
- expenseAccount RecordRef? - References an existing account to be used for goods and services you purchase from this vendor
- payablesAccount RecordRef? - The default payable account for this vendor record
- terms RecordRef? - References a value in a user-defined list at Setup > Accounting > Setup Tasks > Accounting Lists > Term and sets the standard discount terms for this Vendor's invoices
- incoterm RecordRef? - The standardized three-letter trade term to be used on transactions related to this vendor
- creditLimit decimal? - A credit limit for your purchases from the vendor
- balancePrimary decimal? - This is a read-only calculated field that returns the vendor's current accounts payable balance in the specified currency
- openingBalance decimal? - The opening balance of your account with this vendor
- openingBalanceDate string? - The date of the balance entered in the Opening Balance field
- openingBalanceAccount RecordRef? - The account this opening balance is applied to
- balance decimal? - This is a read-only calculated field that returns the vendor's current accounts payable balance
- unbilledOrdersPrimary decimal? - This field displays the total amount of orders that have been entered but not yet billed in the specified currency
- bcn string? - The 15-digit registration number that identifies this vendor as a client of the Canada Customs and Revenue Agency (CCRA)
- unbilledOrders decimal? - Tffhe total amount of orders that have been entered but not yet billed in the specified currency
- currency RecordRef? - References a value in a user-defined list at Lists > Accounting > Currencies. This value sets the currency that all transactions involving this vendor are conducted in
- is1099Eligible boolean? - If set to TRUE, this vendor is 1099 eligible
- isJobResourceVend boolean? - This vendor as a resource on tasks and jobs
- laborCost decimal? - The cost of labor for this vendor in order to be able to calculate profitability on jobs
- purchaseOrderQuantity decimal? - The tolerance limit for the discrepancy between the quantity on the vendor bill and purchase order
- purchaseOrderAmount decimal? - The tolerance limit for the discrepancy between the amount on the vendor bill and purchase order
- purchaseOrderQuantityDiff decimal? - The difference limit for the discrepancy between the quantity on the vendor bill and purchase order
- receiptQuantity decimal? - The tolerance limit for the discrepancy between the quantity on the vendor bill and item receipt
- receiptAmount decimal? - The tolerance limit for the discrepancy between the amount on the vendor bill and item receipt
- receiptQuantityDiff decimal? - The difference limit for the discrepancy between the quantity on the vendor bill and item receipt
- workCalendar RecordRef? - The work calendar for this vendor
- taxIdNum string? - The Vendor's Tax ID number
- taxItem RecordRef? - The default tax code you want applied to purchase orders and bills for this vendor
- giveAccess boolean? - Access to your NetSuite account for the vendor
- sendEmail boolean? - This field can only be set on ADD. When set to TRUE, an email notification is sent to the Vendor to inform them that they have been granted access to your account
- billPay boolean? - Allows to send this vendor payments online. Before you can use this feature, you must set up Online Bill Pay at Setup > Accounting > Online Bill Pay.
- isAccountant boolean? - If set to TRUE, this vendor has free access to the NetSuite account and the isAccountant field is set to FALSE for any vendor record previously defined as the Free Accountant
- password string? - The password assigned to allow this vendor access to NetSuite
- password2 string? - The password confirmation field
- requirePwdChange boolean? - If set to TRUE, the vendor is required to change the default password assign on the next login attempt
- eligibleForCommission boolean? - Indicates eligible for commission
- emailTransactions boolean? - Sets a preferred transaction delivery method for this vendor
- printTransactions boolean? - Sets a preferred transaction delivery method for this vendor
- faxTransactions boolean? - Sets a preferred transaction delivery method for this vendor
- defaultTaxReg RecordRef? - The default tax registration number for this entity
- predictedDays int? - How late or early you expect this vendor to provide the required material in number of days. To indicate days early, enter a negative number
- predConfidence decimal? - The confidence you have that this vendor will provide the required material expressed as a percentage
- customFieldList CustomFieldList? - Custom field list
Union types
netsuite: CustomField
CustomField
Represents a union type for custom field types.
netsuite: ExistingRecordType
ExistingRecordType
RecordType Connector supports for update operation for now.
netsuite: NewRecordType
NewRecordType
RecordType Connector supports for creation operation for now.
Import
import ballerinax/netsuite;
Metadata
Released date: almost 3 years ago
Version: 2.2.0
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.0.0
GraalVM compatible: Yes
Pull count
Total: 4800
Current verison: 1341
Weekly downloads
Keywords
Business Management/ERP
Cost/Paid
Contributors