namsor
Module namsor
API
Definitions
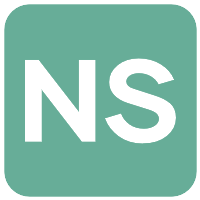
ballerinax/namsor Ballerina library
Overview
This is a generated connector for NamSor API v2 OpenAPI specification.
The NamSor API provides the capability to process personal names (gender, cultural origin or ethnicity) in all alphabets or languages.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a NamSor account
- Obtain tokens - Follow this link
Quickstart
To use the NamSor connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/namsor module into the Ballerina project.
import ballerinax/namsor;
Step 2: Create a new connector instance
Add the project configuration file by creating a Config.toml
file. Config file should have following configurations. Add the tokens obtained in the previous step to the Config.toml
file.
namsor:ApiKeysConfig apiKeyConfig = { xApiKey: "<API_KEY>" } namsor:Client baseClient = check new Client(apiKeyConfig, serviceUrl = "https://v2.namsor.com/NamSorAPIv2");
Step 3: Invoke connector operation
- You can get origin of the name by providing first name and last name as parameters.
namsor:FirstLastNameOriginedOut nameOrigin = check baseClient->origin("Jones", "Joe");
- Use
bal run
command to compile and run the Ballerina program.
Clients
namsor: Client
This is a generated connector for NamSor API v2 OpenAPI specification. The NamSor API provides the capability to process personal names (gender, cultural origin or ethnicity) in all alphabets or languages.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create an NamSor Account and obtain tokens following this guide.
init (ApiKeysConfig apiKeyConfig, ConnectionConfig config, string serviceUrl)
- apiKeyConfig ApiKeysConfig - API keys for authorization
- config ConnectionConfig {} - The configurations to be used when initializing the
connector
- serviceUrl string "https://v2.namsor.com/NamSorAPIv2" - URL of the target service
country
function country(string personalNameFull) returns PersonalNameGeoOut|error
[USES 10 UNITS PER NAME] Infer the likely country of residence of a personal full name, or one surname. Assumes names as they are in the country of residence OR the country of origin.
Parameters
- personalNameFull string - Personal full name
Return Type
- PersonalNameGeoOut|error - A origined name.
nameType
function nameType(string properNoun) returns ProperNounCategorizedOut|error
Infer the likely type of a proper noun (personal name, brand name, place name etc.)
Parameters
- properNoun string - The proper noun
Return Type
- ProperNounCategorizedOut|error - A typed name.
disable
Activate/deactivate an API Key.
Parameters
- 'source string - The API Key to set as enabled/disabled.
- disabled boolean - Disbled/Not disabled
origin
function origin(string firstName, string lastName) returns FirstLastNameOriginedOut|error
[USES 10 UNITS PER NAME] Infer the likely country of origin of a personal name. Assumes names as they are in the country of origin. For US, CA, AU, NZ and other melting-pots : use 'diaspora' instead.
Return Type
- FirstLastNameOriginedOut|error - A origined name.
softwareVersion
function softwareVersion() returns SoftwareVersionOut|error
Get the current software version
Return Type
- SoftwareVersionOut|error - The current software version
apiStatus
function apiStatus() returns APIClassifiersStatusOut|error
Prints the current status of the classifiers. A classifier name in apiStatus corresponds to a service name in apiServices.
Return Type
- APIClassifiersStatusOut|error - Available classifiers and status
availableServices
function availableServices() returns APIServicesOut|error
List of classification services and usage cost in Units per classification (default is 1=ONE Unit). Some API endpoints (ex. Corridor) combine multiple classifiers.
Return Type
- APIServicesOut|error - Available services
taxonomyClasses
function taxonomyClasses(string classifierName) returns APIClassifierTaxonomyOut|error
Print the taxonomy classes valid for the given classifier.
Parameters
- classifierName string - Name of the classifier
Return Type
- APIClassifierTaxonomyOut|error - Available plans
apiUsage
function apiUsage() returns APIPeriodUsageOut|error
Print current API usage.
Return Type
- APIPeriodUsageOut|error - Print current API usage.
apiUsageHistory
function apiUsageHistory() returns APIUsageHistoryOut|error
Print historical API usage.
Return Type
- APIUsageHistoryOut|error - Print historical API usage (NB. new output format form v2.0.15)
apiUsageHistoryAggregate
function apiUsageHistoryAggregate() returns APIUsageAggregatedOut|error
Print historical API usage (in an aggregated view, by service, by day/hour/min).
Return Type
- APIUsageAggregatedOut|error - Print historical API usage.
learnable
Activate/deactivate learning from a source.
Parameters
- 'source string - The API Key to set as learnable/non learnable.
- learnable boolean - Learnable/non learnable.
anonymize
Activate/deactivate anonymization for a source.
Parameters
- 'source string - The API Key to set as anonymized/non anonymized.
- anonymized boolean - Anonymized/non anonymized.
nameTypeGeo
function nameTypeGeo(string properNoun, string countryIso2) returns ProperNounCategorizedOut|error
Infer the likely type of a proper noun (personal name, brand name, place name etc.)
Return Type
- ProperNounCategorizedOut|error - A typed name.
nameTypeBatch
function nameTypeBatch(BatchNameIn payload) returns BatchProperNounCategorizedOut|error
Infer the likely common type of up to 100 proper nouns (personal name, brand name, place name etc.)
Parameters
- payload BatchNameIn - A list of proper names
Return Type
- BatchProperNounCategorizedOut|error - A list of commonTypeized names.
nameTypeGeoBatch
function nameTypeGeoBatch(BatchNameGeoIn payload) returns BatchProperNounCategorizedOut|error
Infer the likely common type of up to 100 proper nouns (personal name, brand name, place name etc.)
Parameters
- payload BatchNameGeoIn - A list of proper names
Return Type
- BatchProperNounCategorizedOut|error - A list of commonTypeized names.
corridor
function corridor(string countryIso2From, string firstNameFrom, string lastNameFrom, string countryIso2To, string firstNameTo, string lastNameTo) returns CorridorOut|error
[USES 20 UNITS PER NAME COUPLE] Infer several classifications for a cross border interaction between names (ex. remit, travel, intl com)
Parameters
- countryIso2From string - Start ISO2 country code to filter
- firstNameFrom string - Start first name to filter
- lastNameFrom string - Start last name to filter
- countryIso2To string - End ISO2 country code to filter
- firstNameTo string - End first name to filter
- lastNameTo string - End last name to filter
Return Type
- CorridorOut|error - Two classified names.
corridorBatch
function corridorBatch(BatchCorridorIn payload) returns BatchCorridorOut|error
[USES 20 UNITS PER NAME PAIR] Infer several classifications for up to 100 cross border interaction between names (ex. remit, travel, intl com)
Parameters
- payload BatchCorridorIn - A list of name pairs, with country code (nameFrom -> nameTo).
Return Type
- BatchCorridorOut|error - A list of classified name pairs.
gender
function gender(string firstName, string lastName) returns FirstLastNameGenderedOut|error
Infer the likely gender of a name.
Return Type
- FirstLastNameGenderedOut|error - A genderized name.
genderGeo
function genderGeo(string firstName, string lastName, string countryIso2) returns FirstLastNameGenderedOut|error
Infer the likely gender of a name, given a local context (ISO2 country code).
Parameters
- firstName string - First name
- lastName string - Last name
- countryIso2 string - ISO2 country code
Return Type
- FirstLastNameGenderedOut|error - A genderized name.
genderGeoBatch
function genderGeoBatch(BatchFirstLastNameGeoIn payload) returns BatchFirstLastNameGenderedOut|error
Infer the likely gender of up to 100 names, each given a local context (ISO2 country code).
Parameters
- payload BatchFirstLastNameGeoIn - A list of names, with country code.
Return Type
- BatchFirstLastNameGenderedOut|error - A list of genderized names.
genderBatch
function genderBatch(BatchFirstLastNameIn payload) returns BatchFirstLastNameGenderedOut|error
Infer the likely gender of up to 100 names, detecting automatically the cultural context.
Parameters
- payload BatchFirstLastNameIn - A list of personal names
Return Type
- BatchFirstLastNameGenderedOut|error - A list of genderized names.
genderFullGeo
function genderFullGeo(string fullName, string countryIso2) returns PersonalNameGenderedOut|error
Infer the likely gender of a full name, given a local context (ISO2 country code).
Return Type
- PersonalNameGenderedOut|error - A genderized name.
genderFull
function genderFull(string fullName) returns PersonalNameGenderedOut|error
Infer the likely gender of a full name, ex. John H. Smith
Parameters
- fullName string - Full name
Return Type
- PersonalNameGenderedOut|error - A genderized name.
genderFullBatch
function genderFullBatch(BatchPersonalNameIn payload) returns BatchPersonalNameGenderedOut|error
Infer the likely gender of up to 100 full names, detecting automatically the cultural context.
Parameters
- payload BatchPersonalNameIn - A list of personal names
Return Type
- BatchPersonalNameGenderedOut|error - A list of genderized names.
genderFullGeoBatch
function genderFullGeoBatch(BatchPersonalNameGeoIn payload) returns BatchPersonalNameGenderedOut|error
Infer the likely gender of up to 100 full names, with a given cultural context (country ISO2 code).
Parameters
- payload BatchPersonalNameGeoIn - A list of personal names, with a country ISO2 code
Return Type
- BatchPersonalNameGenderedOut|error - A list of genderized names.
originBatch
function originBatch(BatchFirstLastNameIn payload) returns BatchFirstLastNameOriginedOut|error
[USES 10 UNITS PER NAME] Infer the likely country of origin of up to 100 names, detecting automatically the cultural context.
Parameters
- payload BatchFirstLastNameIn - A list of personal names
Return Type
- BatchFirstLastNameOriginedOut|error - A list of genderized names.
countryBatch
function countryBatch(BatchPersonalNameIn payload) returns BatchPersonalNameGeoOut|error
[USES 10 UNITS PER NAME] Infer the likely country of residence of up to 100 personal full names, or surnames. Assumes names as they are in the country of residence OR the country of origin.
Parameters
- payload BatchPersonalNameIn - A list of personal names
Return Type
- BatchPersonalNameGeoOut|error - A list of genderized names.
usRaceEthnicity
function usRaceEthnicity(string firstName, string lastName) returns FirstLastNameUSRaceEthnicityOut|error
[USES 10 UNITS PER NAME] Infer a US resident's likely race/ethnicity according to US Census taxonomy W_NL (white, non latino), HL (hispano latino), A (asian, non latino), B_NL (black, non latino). Optionally add header X-OPTION-USRACEETHNICITY-TAXONOMY: USRACEETHNICITY-6CLASSES for two additional classes, AI_AN (American Indian or Alaskan Native) and PI (Pacific Islander).
Return Type
- FirstLastNameUSRaceEthnicityOut|error - a US resident's likely race/ethnicity : W_NL (white, non latino), HL (hispano latino), A (asian, non latino), B_NL (black, non latino), AI_AN (American Indian or Alaskan Native*) and PI (Pacific Islander*). *optionally
usRaceEthnicityZIP5
function usRaceEthnicityZIP5(string firstName, string lastName, string zip5Code) returns FirstLastNameUSRaceEthnicityOut|error
[USES 10 UNITS PER NAME] Infer a US resident's likely race/ethnicity according to US Census taxonomy, using (optional) ZIP5 code info. Output is W_NL (white, non latino), HL (hispano latino), A (asian, non latino), B_NL (black, non latino). Optionally add header X-OPTION-USRACEETHNICITY-TAXONOMY: USRACEETHNICITY-6CLASSES for two additional classes, AI_AN (American Indian or Alaskan Native) and PI (Pacific Islander).
Parameters
- firstName string - First name
- lastName string - Last name
- zip5Code string - 5-digit ZIP Code
Return Type
- FirstLastNameUSRaceEthnicityOut|error - a US resident's likely race/ethnicity : W_NL (white, non latino), HL (hispano latino), A (asian, non latino), B_NL (black, non latino), AI_AN (American Indian or Alaskan Native*) and PI (Pacific Islander*). *optionally
usRaceEthnicityBatch
function usRaceEthnicityBatch(BatchFirstLastNameGeoIn payload) returns BatchFirstLastNameUSRaceEthnicityOut|error
[USES 10 UNITS PER NAME] Infer up-to 100 US resident's likely race/ethnicity according to US Census taxonomy. Output is W_NL (white, non latino), HL (hispano latino), A (asian, non latino), B_NL (black, non latino). Optionally add header X-OPTION-USRACEETHNICITY-TAXONOMY: USRACEETHNICITY-6CLASSES for two additional classes, AI_AN (American Indian or Alaskan Native) and PI (Pacific Islander).
Parameters
- payload BatchFirstLastNameGeoIn - A list of personal names
Return Type
- BatchFirstLastNameUSRaceEthnicityOut|error - A list of US resident's likely race/ethnicity. W_NL (white, non latino), HL (hispano latino), A (asian, non latino), B_NL (black, non latino), AI_AN (American Indian or Alaskan Native*) and PI (Pacific Islander*). *optionally
usZipRaceEthnicityBatch
function usZipRaceEthnicityBatch(BatchFirstLastNameGeoZippedIn payload) returns BatchFirstLastNameUSRaceEthnicityOut|error
[USES 10 UNITS PER NAME] Infer up-to 100 US resident's likely race/ethnicity according to US Census taxonomy, with (optional) ZIP code. Output is W_NL (white, non latino), HL (hispano latino), A (asian, non latino), B_NL (black, non latino). Optionally add header X-OPTION-USRACEETHNICITY-TAXONOMY: USRACEETHNICITY-6CLASSES for two additional classes, AI_AN (American Indian or Alaskan Native) and PI (Pacific Islander).
Parameters
- payload BatchFirstLastNameGeoZippedIn - A list of personal names
Return Type
- BatchFirstLastNameUSRaceEthnicityOut|error - A list of US resident's likely race/ethnicity. W_NL (white, non latino), HL (hispano latino), A (asian, non latino), B_NL (black, non latino), AI_AN (American Indian or Alaskan Native*) and PI (Pacific Islander*). *optionally
diaspora
function diaspora(string countryIso2, string firstName, string lastName) returns FirstLastNameDiasporaedOut|error
[USES 20 UNITS PER NAME] Infer the likely ethnicity/diaspora of a personal name, given a country of residence ISO2 code (ex. US, CA, AU, NZ etc.)
Parameters
- countryIso2 string - ISO2 country code
- firstName string - First name
- lastName string - Last name
Return Type
- FirstLastNameDiasporaedOut|error - A diaspora / ethnicity for given name and geography.
diasporaBatch
function diasporaBatch(BatchFirstLastNameGeoIn payload) returns BatchFirstLastNameDiasporaedOut|error
[USES 20 UNITS PER NAME] Infer the likely ethnicity/diaspora of up to 100 personal names, given a country of residence ISO2 code (ex. US, CA, AU, NZ etc.)
Parameters
- payload BatchFirstLastNameGeoIn - A list of personal names
Return Type
- BatchFirstLastNameDiasporaedOut|error - A list of diaspora / ethnicity given a name and residency.
parseNameGeo
function parseNameGeo(string nameFull, string countryIso2) returns PersonalNameParsedOut|error
Infer the likely first/last name structure of a name, ex. John Smith or SMITH, John or SMITH; John. For better accuracy, provide a geographic context.
Return Type
- PersonalNameParsedOut|error - A origined name.
parseNameBatch
function parseNameBatch(BatchPersonalNameIn payload) returns BatchPersonalNameParsedOut|error
Infer the likely first/last name structure of a name, ex. John Smith or SMITH, John or SMITH; John.
Parameters
- payload BatchPersonalNameIn - A list of personal names
Return Type
- BatchPersonalNameParsedOut|error - A list of parsed names.
parseNameGeoBatch
function parseNameGeoBatch(BatchPersonalNameGeoIn payload) returns BatchPersonalNameParsedOut|error
Infer the likely first/last name structure of a name, ex. John Smith or SMITH, John or SMITH; John. Giving a local context improves precision.
Parameters
- payload BatchPersonalNameGeoIn - A list of personal names
Return Type
- BatchPersonalNameParsedOut|error - A list of parsed names.
parseChineseName
function parseChineseName(string chineseName) returns PersonalNameParsedOut|error
Infer the likely first/last name structure of a name, ex. 王晓明 -> 王(surname) 晓明(given name)
Parameters
- chineseName string - Chinese name
Return Type
- PersonalNameParsedOut|error - A origined name.
parseChineseNameBatch
function parseChineseNameBatch(BatchPersonalNameIn payload) returns BatchPersonalNameParsedOut|error
Infer the likely first/last name structure of a name, ex. 王晓明 -> 王(surname) 晓明(given name).
Parameters
- payload BatchPersonalNameIn - A list of personal names
Return Type
- BatchPersonalNameParsedOut|error - A list of parsed names.
pinyinChineseName
function pinyinChineseName(string chineseName) returns PersonalNameParsedOut|error
Romanize the Chinese name to Pinyin, ex. 王晓明 -> Wang (surname) Xiaoming (given name)
Parameters
- chineseName string - Chinese name
Return Type
- PersonalNameParsedOut|error - A pinyin name.
pinyinChineseNameBatch
function pinyinChineseNameBatch(BatchPersonalNameIn payload) returns BatchPersonalNameParsedOut|error
Romanize a list of Chinese name to Pinyin, ex. 王晓明 -> Wang (surname) Xiaoming (given name).
Parameters
- payload BatchPersonalNameIn - A list of Chinese names
Return Type
- BatchPersonalNameParsedOut|error - A list of Pinyin names.
chineseNameMatch
function chineseNameMatch(string chineseSurnameLatin, string chineseGivenNameLatin, string chineseName) returns NameMatchedOut|error
Return a score for matching Chinese name ex. 王晓明 with a romanized name ex. Wang Xiaoming
Parameters
- chineseSurnameLatin string - Chinese sur name in latin
- chineseGivenNameLatin string - Chinese given name in latin
- chineseName string - Chinese name
Return Type
- NameMatchedOut|error - A romanized name.
chineseNameMatchBatch
function chineseNameMatchBatch(BatchMatchPersonalFirstLastNameIn payload) returns BatchNameMatchedOut|error
Identify Chinese name candidates, based on the romanized name (firstName = chineseGivenName; lastName=chineseSurname), ex. Wang Xiaoming
Parameters
- payload BatchMatchPersonalFirstLastNameIn - A list of personal Chinese names in LATIN, firstName = chineseGivenName; lastName=chineseSurname
Return Type
- BatchNameMatchedOut|error - A list of genderized names.
genderChineseNamePinyin
function genderChineseNamePinyin(string chineseSurnameLatin, string chineseGivenNameLatin) returns FirstLastNameGenderedOut|error
Infer the likely gender of a Chinese name in LATIN (Pinyin).
Parameters
- chineseSurnameLatin string - Chinese sur name in latin
- chineseGivenNameLatin string - Chinese given name in latin
Return Type
- FirstLastNameGenderedOut|error - A genderized name.
genderChineseNamePinyinBatch
function genderChineseNamePinyinBatch(BatchFirstLastNameIn payload) returns BatchFirstLastNameGenderedOut|error
Infer the likely gender of up to 100 Chinese names in LATIN (Pinyin).
Parameters
- payload BatchFirstLastNameIn - A list of names, with country code.
Return Type
- BatchFirstLastNameGenderedOut|error - A list of genderized names.
genderChineseName
function genderChineseName(string chineseName) returns PersonalNameGenderedOut|error
Infer the likely gender of a Chinese full name ex. 王晓明
Parameters
- chineseName string - Chinese name
Return Type
- PersonalNameGenderedOut|error - A genderized name.
genderChineseNameBatch
function genderChineseNameBatch(BatchPersonalNameIn payload) returns BatchPersonalNameGenderedOut|error
Infer the likely gender of up to 100 full names ex. 王晓明
Parameters
- payload BatchPersonalNameIn - A list of personal names, with a country ISO2 code
Return Type
- BatchPersonalNameGenderedOut|error - A list of genderized names.
chineseNameCandidates
function chineseNameCandidates(string chineseSurnameLatin, string chineseGivenNameLatin) returns NameMatchCandidatesOut|error
Identify Chinese name candidates, based on the romanized name ex. Wang Xiaoming
Parameters
- chineseSurnameLatin string - Chinese sur name in latin
- chineseGivenNameLatin string - Chinese given name in latin
Return Type
- NameMatchCandidatesOut|error - A romanized name.
chineseNameCandidatesBatch
function chineseNameCandidatesBatch(BatchFirstLastNameIn payload) returns BatchNameMatchCandidatesOut|error
Identify Chinese name candidates, based on the romanized name (firstName = chineseGivenName; lastName=chineseSurname), ex. Wang Xiaoming
Parameters
- payload BatchFirstLastNameIn - A list of personal Chinese names in LATIN, firstName = chineseGivenName; lastName=chineseSurname
Return Type
- BatchNameMatchCandidatesOut|error - A list of genderized names.
chineseNameGenderCandidates
function chineseNameGenderCandidates(string chineseSurnameLatin, string chineseGivenNameLatin, string knownGender) returns NameMatchCandidatesOut|error
Identify Chinese name candidates, based on the romanized name ex. Wang Xiaoming - having a known gender ('male' or 'female')
Parameters
- chineseSurnameLatin string - Chinese sur name in latin
- chineseGivenNameLatin string - Chinese given name in latin
- knownGender string - Known gender
Return Type
- NameMatchCandidatesOut|error - A romanized name.
chineseNameCandidatesGenderBatch
function chineseNameCandidatesGenderBatch(BatchFirstLastNameGenderIn payload) returns BatchNameMatchCandidatesOut|error
Identify Chinese name candidates, based on the romanized name (firstName = chineseGivenName; lastName=chineseSurname) ex. Wang Xiaoming.
Parameters
- payload BatchFirstLastNameGenderIn - A list of personal Chinese names in LATIN, firstName = chineseGivenName; lastName=chineseSurname
Return Type
- BatchNameMatchCandidatesOut|error - A list of genderized names.
parseJapaneseName
function parseJapaneseName(string japaneseName) returns PersonalNameParsedOut|error
Infer the likely first/last name structure of a name, ex. 山本 早苗 or Yamamoto Sanae
Parameters
- japaneseName string - Japanese name
Return Type
- PersonalNameParsedOut|error - A origined name.
parseJapaneseNameBatch
function parseJapaneseNameBatch(BatchPersonalNameIn payload) returns BatchPersonalNameParsedOut|error
Infer the likely first/last name structure of a name, ex. 山本 早苗 or Yamamoto Sanae
Parameters
- payload BatchPersonalNameIn - A list of personal names
Return Type
- BatchPersonalNameParsedOut|error - A list of parsed names.
japaneseNameKanjiCandidates
function japaneseNameKanjiCandidates(string japaneseSurnameLatin, string japaneseGivenNameLatin, string knownGender) returns NameMatchCandidatesOut|error
Identify japanese name candidates in KANJI, based on the romanized name ex. Yamamoto Sanae - and a known gender.
Parameters
- japaneseSurnameLatin string - Japanese sur name in latin
- japaneseGivenNameLatin string - Japanese given name in latin
- knownGender string - Known gender
Return Type
- NameMatchCandidatesOut|error - A romanized name.
japanesenamekanjicandidates1
function japanesenamekanjicandidates1(string japaneseSurnameLatin, string japaneseGivenNameLatin) returns NameMatchCandidatesOut|error
Identify japanese name candidates in KANJI, based on the romanized name ex. Yamamoto Sanae
Parameters
- japaneseSurnameLatin string - Japanese sur name in latin
- japaneseGivenNameLatin string - Japanese given name in latin
Return Type
- NameMatchCandidatesOut|error - A romanized name.
japaneseNameLatinCandidates
function japaneseNameLatinCandidates(string japaneseSurnameKanji, string japaneseGivenNameKanji) returns NameMatchCandidatesOut|error
Romanize japanese name, based on the name in Kanji.
Parameters
- japaneseSurnameKanji string - Japanese sur name in kanji
- japaneseGivenNameKanji string - Japanese given name in kanji
Return Type
- NameMatchCandidatesOut|error - A romanized name.
japaneseNameKanjiCandidatesBatch
function japaneseNameKanjiCandidatesBatch(BatchFirstLastNameIn payload) returns BatchNameMatchCandidatesOut|error
Identify japanese name candidates in KANJI, based on the romanized name (firstName = japaneseGivenName; lastName=japaneseSurname), ex. Yamamoto Sanae
Parameters
- payload BatchFirstLastNameIn - A list of personal japanese names in LATIN, firstName = japaneseGivenName; lastName=japaneseSurname
Return Type
- BatchNameMatchCandidatesOut|error - A list of genderized names.
japaneseNameGenderKanjiCandidatesBatch
function japaneseNameGenderKanjiCandidatesBatch(BatchFirstLastNameGenderIn payload) returns BatchNameMatchCandidatesOut|error
Identify japanese name candidates in KANJI, based on the romanized name (firstName = japaneseGivenName; lastName=japaneseSurname) with KNOWN gender, ex. Yamamoto Sanae
Parameters
- payload BatchFirstLastNameGenderIn - A list of personal japanese names in LATIN, firstName = japaneseGivenName; lastName=japaneseSurname and known gender
Return Type
- BatchNameMatchCandidatesOut|error - A list of genderized names.
japaneseNameLatinCandidatesBatch
function japaneseNameLatinCandidatesBatch(BatchFirstLastNameIn payload) returns BatchNameMatchCandidatesOut|error
Romanize japanese names, based on the name in KANJI
Parameters
- payload BatchFirstLastNameIn - A list of personal japanese names in KANJI, firstName = japaneseGivenName; lastName=japaneseSurname
Return Type
- BatchNameMatchCandidatesOut|error - A list of genderized names.
japaneseNameMatch
function japaneseNameMatch(string japaneseSurnameLatin, string japaneseGivenNameLatin, string japaneseName) returns NameMatchedOut|error
Return a score for matching Japanese name in KANJI ex. 山本 早苗 with a romanized name ex. Yamamoto Sanae
Parameters
- japaneseSurnameLatin string - Japanese sur name in latin
- japaneseGivenNameLatin string - Japanese given name in latin
- japaneseName string - Japanese name
Return Type
- NameMatchedOut|error - A romanized name.
japaneseNameMatchFeedbackLoop
function japaneseNameMatchFeedbackLoop(string japaneseSurnameLatin, string japaneseGivenNameLatin, string japaneseName) returns FeedbackLoopOut|error
[CREDITS 1 UNIT] Feedback loop to better perform matching Japanese name in KANJI ex. 山本 早苗 with a romanized name ex. Yamamoto Sanae
Parameters
- japaneseSurnameLatin string - Japanese sur name in latin
- japaneseGivenNameLatin string - Japanese given name in latin
- japaneseName string - Japanese name
Return Type
- FeedbackLoopOut|error - A romanized name.
japaneseNameMatchBatch
function japaneseNameMatchBatch(BatchMatchPersonalFirstLastNameIn payload) returns BatchNameMatchedOut|error
Return a score for matching a list of Japanese names in KANJI ex. 山本 早苗 with romanized names ex. Yamamoto Sanae
Parameters
- payload BatchMatchPersonalFirstLastNameIn - A list of personal Japanese names in LATIN, firstName = japaneseGivenName; lastName=japaneseSurname
Return Type
- BatchNameMatchedOut|error - A list of matched names.
genderJapaneseNamePinyin
function genderJapaneseNamePinyin(string japaneseSurname, string japaneseGivenName) returns FirstLastNameGenderedOut|error
Infer the likely gender of a Japanese name in LATIN (Pinyin).
Return Type
- FirstLastNameGenderedOut|error - A genderized name.
genderJapaneseNamePinyinBatch
function genderJapaneseNamePinyinBatch(BatchFirstLastNameIn payload) returns BatchFirstLastNameGenderedOut|error
Infer the likely gender of up to 100 Japanese names in LATIN (Pinyin).
Parameters
- payload BatchFirstLastNameIn - A list of names, with country code.
Return Type
- BatchFirstLastNameGenderedOut|error - A list of genderized names.
genderJapaneseNameFull
function genderJapaneseNameFull(string japaneseName) returns PersonalNameGenderedOut|error
Infer the likely gender of a Japanese full name ex. 王晓明
Parameters
- japaneseName string - Japanese name
Return Type
- PersonalNameGenderedOut|error - A genderized name.
genderJapaneseNameFullBatch
function genderJapaneseNameFullBatch(BatchPersonalNameIn payload) returns BatchPersonalNameGenderedOut|error
Infer the likely gender of up to 100 full names
Parameters
- payload BatchPersonalNameIn - A list of personal names
Return Type
- BatchPersonalNameGenderedOut|error - A list of genderized names.
phoneCode
function phoneCode(string firstName, string lastName, string phoneNumber) returns FirstLastNamePhoneCodedOut|error
[USES 11 UNITS PER NAME] Infer the likely country and phone prefix, given a personal name and formatted / unformatted phone number.
Return Type
- FirstLastNamePhoneCodedOut|error - A name with country and phone code.
phoneCodeGeo
function phoneCodeGeo(string firstName, string lastName, string phoneNumber, string countryIso2) returns FirstLastNamePhoneCodedOut|error
[USES 11 UNITS PER NAME] Infer the likely phone prefix, given a personal name and formatted / unformatted phone number, with a local context (ISO2 country of residence).
Parameters
- firstName string - First name
- lastName string - Last name
- phoneNumber string - Phone number
- countryIso2 string - ISO2 country code
Return Type
- FirstLastNamePhoneCodedOut|error - A name with country and phone code.
phoneCodeGeoFeedbackLoop
function phoneCodeGeoFeedbackLoop(string firstName, string lastName, string phoneNumber, string phoneNumberE164, string countryIso2) returns FirstLastNamePhoneCodedOut|error
[CREDITS 1 UNIT] Feedback loop to better infer the likely phone prefix, given a personal name and formatted / unformatted phone number, with a local context (ISO2 country of residence).
Parameters
- firstName string - First name
- lastName string - Last name
- phoneNumber string - Phone number
- phoneNumberE164 string - Phone number in E164 standard
- countryIso2 string - ISO2 country code
Return Type
- FirstLastNamePhoneCodedOut|error - A name with country and phone code.
phoneCodeBatch
function phoneCodeBatch(BatchFirstLastNamePhoneNumberIn payload) returns BatchFirstLastNamePhoneCodedOut|error
[USES 11 UNITS PER NAME] Infer the likely country and phone prefix, of up to 100 personal names, detecting automatically the local context given a name and formatted / unformatted phone number.
Parameters
- payload BatchFirstLastNamePhoneNumberIn - A list of personal names
Return Type
- BatchFirstLastNamePhoneCodedOut|error - A list of genderized names.
phoneCodeGeoBatch
function phoneCodeGeoBatch(BatchFirstLastNamePhoneNumberGeoIn payload) returns BatchFirstLastNamePhoneCodedOut|error
[USES 11 UNITS PER NAME] Infer the likely country and phone prefix, of up to 100 personal names, with a local context (ISO2 country of residence).
Parameters
- payload BatchFirstLastNamePhoneNumberGeoIn - A list of personal names
Return Type
- BatchFirstLastNamePhoneCodedOut|error - A list of genderized names.
parseName
function parseName(string nameFull) returns PersonalNameParsedOut|error
Infer the likely first/last name structure of a name, ex. John Smith or SMITH, John or SMITH; John.
Parameters
- nameFull string - Full name to infer
Return Type
- PersonalNameParsedOut|error - A origined name.
Records
namsor: APIBillingPeriodUsageOut
The current billing period.
Fields
- apiKey string? - User API Key.
- subscriptionStarted int? - Datetime when the user subscribed to the plan.
- periodStarted int? - Datetime when the the plan's current period started.
- periodEnded int? - Datetime when the the plan's current period endend.
- stripeCurrentPeriodEnd int? - Datetime when the the plan's current period endend (in Stripe). Internal and Stripe periodicity should ~coincide.
- stripeCurrentPeriodStart int? - Datetime when the the plan's current period started (in Stripe). Internal and Stripe periodicity should ~coincide.
- billingStatus string? - Current period billing status ex OPEN.
- usage int? - Current period usage in units (NB some API endpoints use more than one unit).
- softLimit int? - Current period soft limit (reaching the limit sends an email notification).
- hardLimit int? - Current period hard limit (reaching the limit sends an email notification and blocks the API Key).
namsor: APIClassifierOut
The list of classifiers and versions.
Fields
- classifierName string? - The classifier name
- serving boolean? - True if the classifier is serving requests (has reached minimal learning, is not shutting down)
- learning boolean? - True if the classifier is learning
- shuttingDown boolean? - True if the classifier is shutting down
- probabilityCalibrated boolean? - True if the classifier has finished the initial learning and calibrated probabilities (meanwhile, during initial learning, probabilities will be equal to -1)
namsor: APIClassifiersStatusOut
Fields
- softwareVersion SoftwareVersionOut? - The software version.
- classifiers APIClassifierOut[]? - The list of classifiers and versions.
namsor: APIClassifierTaxonomyOut
Fields
- classifierName string? - Name of the classifier as per apiStatus (corresponds also to the name of the service in apiServices)
- taxonomyClasses string[]? - The taxonomy classes this classifier classifies to
namsor: APICounterV2Out
Detailed usage as reported by the deduplicating API counter.
Fields
- apiKey APIKeyOut? - The API Key.
- apiService string? - The apiService corresponds to the classifier name.
- createdDateTime int? - The create datetime of the counter.
- totalUsage int? - The usage of the counter.
- lastFlushedDateTime int? - The flush datetime of the counter.
- lastUsedDateTime int? - The last usage datetime of the counter.
- serviceFeaturesUsage record {}? - Usage of special features, such as Chinese, Japanese.
namsor: APIKeyOut
The API Key.
Fields
- apiKey string? - The user API Key.
- userId string? - The user identifier.
- admin boolean? - The API Key has admin role.
- vetted boolean? - The API Key is vetted (assumed truthful) for machine learning.
- learnable boolean? - The API Key is learnable (without assuming truthfulness) for machine learning. Set learnable=false and anonymized=true for highest privacy (ie. to forget data as it's processed).
- anonymized boolean? - The API Key is anonymized (using SHA-252 digest for logging). Set learnable=false and anonymized=true for highest privacy (ie. to forget data as it's processed).
- partner boolean? - The API Key has partner role.
- striped boolean? - The API Key is associated to a valid Stripe account.
- corporate boolean? - The API Key has role corporate (ex SWIFT payments instead of Stripe payments).
- disabled boolean? - The API Key is temporarily or permanently disabled.
namsor: ApiKeysConfig
Provides API key configurations needed when communicating with a remote HTTP endpoint.
Fields
- xApiKey string - All requests on the NamSor API needs to include an API key. The API key can be provided as part of the query string or as a request header. The name of the API key needs to be
X-API-KEY
namsor: APIPeriodUsageOut
Fields
- subscription APIPlanSubscriptionOut? - The API Plan governing the subscription.
- billingPeriod APIBillingPeriodUsageOut? - The current billing period.
- overageExclTax decimal? - Overage amount including any tax.
- overageInclTax decimal? - Overage amount including tax (if applicable).
- overageCurrency string? - Currency of the overage amount.
- overageQuantity int? - Quantity above monthly quota of the current subscritpion, in units.
namsor: APIPlanSubscriptionOut
The API Plan governing the subscription.
Fields
- apiKey string? - User API Key.
- planStarted int? - Datetime when the user subscribed to the current plan.
- priorPlanStarted int? - Datetime when the user subscribed to the prior plan.
- planEnded int? - Datetime when the user ended the plan.
- taxRate decimal? - Applicable tax rate for the plan.
- planName string? - Current plan name.
- planBaseFeesKey string? - Current plan key (as in Stripe product).
- planStatus string? - Plan status.
- planQuota int? - Current plan quota in quantity of units (NB: some API use several units per name).
- priceUSD decimal? - Current plan monthly price expressed in USD (includes a free quota).
- priceOverageUSD decimal? - Current plan price for overages expressed in USD (extra price per unit above the free quota).
- price decimal? - Current plan price for overages expressed in Currency (extra price per unit above the free quota).
- priceOverage decimal? - Current plan price for overages expressed in Currency (extra price per unit above the free quota).
- currency string? - Current plan Currency for prices.
- currencyFactor decimal? - For USD, GBP, EUR - the factor is 1.
- stripeCustomerId string? - Stripe customer identifier.
- stripeStatus string? - Stripe status ex active.
- stripeSubscription string? - Stripe subscription identifier.
- userId string? - Internal user identifier.
namsor: APIServiceOut
List of API Services
Fields
- serviceName string? - A service name corresponds to classifier name in apiStatus (ex. personalname_gender or personalfullname_gender)
- serviceGroup string? - Groups together classifiers providing a similar service (ex. gender groups personalname_gender and personalfullname_gender)
- costInUnits int? - Indicates how many units per call this service costs (ex. the number of units per name)
namsor: APIServicesOut
Fields
- apiServices APIServiceOut[]? - List of API Services
namsor: APIUsageAggregatedOut
Fields
- timeUnit string? - Time unit is DAY, WEEK or MONTH depending on prior usage
- periodStart int? - Start datetime of the reporting period
- periodEnd int? - End datetime of the reporting period
- totalUsage int? - Total usage in the current period
- historyTruncated boolean? - If the history was truncaded due to data limit
- data int[]? - Data points : usage per DAY, WEEK or MONTH and per apiService
- colHeaders string[]? - apiServices as column headers
- rowHeaders string[]? - dates as row headers
namsor: APIUsageHistoryOut
Fields
- detailedUsage APICounterV2Out[]? - Detailed usage as reported by the deduplicating API counter.
namsor: BatchCorridorIn
Fields
- corridorFromTo CorridorIn[]? -
namsor: BatchCorridorOut
Fields
- corridorFromTo CorridorOut[]? - Classified corridors
namsor: BatchFirstLastNameDiasporaedOut
Represents the output of inferring the LIKELY ethnicity from a personal name, given an country of residence.
Fields
- personalNames FirstLastNameDiasporaedOut[]? - Classified diaspora names
namsor: BatchFirstLastNameGenderedOut
Represents the output of inferring the LIKELY gender from a list of personal names.
Fields
- personalNames FirstLastNameGenderedOut[]? - Classified genderized names
namsor: BatchFirstLastNameGenderIn
Fields
- personalNames FirstLastNameGenderIn[]? -
namsor: BatchFirstLastNameGeoIn
Fields
- personalNames FirstLastNameGeoIn[]? -
namsor: BatchFirstLastNameGeoZippedIn
Fields
- personalNames FirstLastNameGeoZippedIn[]? -
namsor: BatchFirstLastNameIn
Fields
- personalNames FirstLastNameIn[]? -
namsor: BatchFirstLastNameOriginedOut
Represents the output of inferring the LIKELY origin from a list of personal names.
Fields
- personalNames FirstLastNameOriginedOut[]? - Classified origined names
namsor: BatchFirstLastNamePhoneCodedOut
Represents the output of inferring the LIKELY country and phone code of personal names+phones.
Fields
- personalNamesWithPhoneNumbers FirstLastNamePhoneCodedOut[]? - Classified phone-coded names
namsor: BatchFirstLastNamePhoneNumberGeoIn
Fields
- personalNamesWithPhoneNumbers FirstLastNamePhoneNumberGeoIn[]? -
namsor: BatchFirstLastNamePhoneNumberIn
Fields
- personalNamesWithPhoneNumbers FirstLastNamePhoneNumberIn[]? -
namsor: BatchFirstLastNameUSRaceEthnicityOut
Represents the output of inferring the LIKELY US 'race/ethnicity' from a personal name, given US country of residence and (optionally) a ZIP5 code.
Fields
- personalNames FirstLastNameUSRaceEthnicityOut[]? - Classified US 'race'/ethnicized names
namsor: BatchMatchPersonalFirstLastNameIn
Fields
- personalNames MatchPersonalFirstLastNameIn[]? -
namsor: BatchNameGeoIn
Fields
- properNouns NameGeoIn[]? -
namsor: BatchNameIn
Fields
- properNouns NameIn[]? -
namsor: BatchNameMatchCandidatesOut
Fields
- namesAndMatchCandidates NameMatchCandidatesOut[]? - Classified matched names
namsor: BatchNameMatchedOut
Fields
- matchedNames NameMatchedOut[]? - Classified matched names
namsor: BatchPersonalNameGenderedOut
Fields
- personalNames PersonalNameGenderedOut[]? - Classified genderized names
namsor: BatchPersonalNameGeoIn
Fields
- personalNames PersonalNameGeoIn[]? -
namsor: BatchPersonalNameGeoOut
Fields
- personalNames PersonalNameGeoOut[]? - Classified geo names
namsor: BatchPersonalNameIn
Fields
- personalNames PersonalNameIn[]? -
namsor: BatchPersonalNameParsedOut
Fields
- personalNames PersonalNameParsedOut[]? - Classified parsed names
namsor: BatchProperNounCategorizedOut
Represents the output of inferring the common type (anthroponym, toponym, brand, etc.) from a list of proper names.
Fields
- properNouns ProperNounCategorizedOut[]? - Classified typed proper names
namsor: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
namsor: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
namsor: CorridorIn
Represent any transnational interaction between names (ex. remittance, communication, cross-border investment, airline travel
Fields
- id string? - Id
- firstLastNameGeoFrom FirstLastNameGeoIn? - First name related to transnational interaction
- firstLastNameGeoTo FirstLastNameGeoIn? - First name related to transnational interaction
namsor: CorridorOut
Represent multiple classifications for corridor sender and receiver (gender, country, origin, diaspora)
Fields
- id string? - Id
- FirstLastNameGenderedOut FirstLastNameGenderedOut? - Represents the output of inferring the LIKELY gender from a personal name.
- FirstLastNameOriginedOut FirstLastNameOriginedOut? - Represents the output of inferring the LIKELY country of Origin from a personal name.
- FirstLastNameDiasporaedOut FirstLastNameDiasporaedOut? - Represents the output of inferring the LIKELY ethnicity from a personal name, given an country of residence.
- script string? - Script
namsor: FeedbackLoopOut
Fields
- feedbackCredits int? - Number of units recredited as per feedback loop successful classification
namsor: FirstLastNameDiasporaedOut
Represents the output of inferring the LIKELY ethnicity from a personal name, given an country of residence.
Fields
- script string? - Script
- id string? - Id
- firstName string? - The first name (also known as given name)
- lastName string? - The last name (also known as family name, or surname)
- score decimal? - Compatibility to NamSor_v1 Diaspora score value. Higher score is better, but score is not normalized. Use calibratedProbability if available.
- ethnicityAlt string? - The second best alternative ethnicity
- ethnicity string? - The most likely ethnicity
- lifted boolean? - Indicates if the output ethnicity is based on machine learning only, or further lifted as a known fact by a country-specific rule. Let us know if you believe ethnicity is incorrect on a specific case where lifted is true.
- countryIso2 string? - From input data, the countryIso2 of geographic context (US,CA etc.)
- ethnicitiesTop string[]? - List most likely ethnicities (top 10)
namsor: FirstLastNameGenderedOut
Represents the output of inferring the LIKELY gender from a personal name.
Fields
- script string? - Script
- id string? - Id
- firstName string? - The first name (also known as given name)
- lastName string? - The last name (also known as family name, or surname)
- likelyGender string? - Most likely gender
- genderScale decimal? - Compatibility to NamSor_v1 Gender Scale M[-1..U..+1]F value
- score decimal? - Compatibility to NamSor_v1 Gender score value. Higher score is better, but score is not normalized. Use calibratedProbability if available.
- probabilityCalibrated decimal? - The calibrated probability for inferred gender to have been guessed correctly.
namsor: FirstLastNameGenderIn
Fields
- id string? -
- firstName string? -
- lastName string? -
- gender string? - The known gender of the name
namsor: FirstLastNameGeoIn
First name related to transnational interaction
Fields
- id string? - Id
- firstName string? - First name
- lastName string? - Last name
- countryIso2 string? - ISO2 country code
namsor: FirstLastNameGeoZippedIn
Fields
- id string? -
- firstName string? -
- lastName string? -
- countryIso2 string? -
- zipCode string? -
namsor: FirstLastNameIn
Fields
- id string? -
- firstName string? -
- lastName string? -
namsor: FirstLastNameOriginedOut
Represents the output of inferring the LIKELY country of Origin from a personal name.
Fields
- script string? - Script
- id string? - Id
- firstName string? - The first name (also known as given name)
- lastName string? - The last name (also known as family name, or surname)
- countryOrigin string? - Most likely country of Origin
- countryOriginAlt string? - Second best alternative : country of Origin
- countriesOriginTop string[]? - List countries of Origin (top 10)
- score decimal? - Compatibility to NamSor_v1 Origin score value. Higher score is better, but score is not normalized. Use calibratedProbability if available.
- regionOrigin string? - Most likely region of Origin (based on countryOrigin ISO2 code)
- topRegionOrigin string? - Most likely top region of Origin (based on countryOrigin ISO2 code)
- subRegionOrigin string? - Most likely sub region of Origin (based on countryOrigin ISO2 code)
- probabilityCalibrated decimal? - The calibrated probability for countryOrigin to have been guessed correctly.
- probabilityAltCalibrated decimal? - The calibrated probability for countryOrigin OR countryOriginAlt to have been guessed correctly.
namsor: FirstLastNameOut
First / last name structure corresponding to the most likely parsing.
Fields
- script string? - Script
- id string? - Id
- firstName string? - The first name (also known as given name)
- lastName string? - The last name (also known as family name, or surname)
namsor: FirstLastNamePhoneCodedOut
Represents the output of inferring the LIKELY country and phone code from a personal name and phone number.
Fields
- script string? - Script
- id string? - Id
- firstName string? - The first name (also known as given name)
- lastName string? - The last name (also known as family name, or surname)
- internationalPhoneNumberVerified string? - The normalized phone number, verified using libphonenumber.
- phoneCountryIso2Verified string? - The phone ISO2 country code, verified using libphonenumber.
- phoneCountryCode int? - The phone country code of the phone number, verified using libphonenumber.
- phoneCountryCodeAlt int? - The best alternative phone country code of the phone number.
- phoneCountryIso2 string? - The likely country of the phone number.
- phoneCountryIso2Alt string? - The best alternative country of the phone number.
- originCountryIso2 string? - The likely country of origin of the name.
- originCountryIso2Alt string? - The best alternative country of origin of the name.
- phoneNumber string? - The input phone number.
- verified boolean? - Indicates if the phone number could be positively verified using libphonenumber.
- score decimal? - Higher score is better, but score is not normalized. Use calibratedProbability if available.
- countryIso2 string? - ISO2 country code
namsor: FirstLastNamePhoneNumberGeoIn
Fields
- id string? -
- firstName string? -
- lastName string? -
- phoneNumber string? -
- FirstLastNameOriginedOut FirstLastNameOriginedOut? - Represents the output of inferring the LIKELY country of Origin from a personal name.
- countryIso2 string? -
- countryIso2Alt string? -
namsor: FirstLastNamePhoneNumberIn
Fields
- id string? -
- firstName string? -
- lastName string? -
- phoneNumber string? -
- FirstLastNameOriginedOut FirstLastNameOriginedOut? - Represents the output of inferring the LIKELY country of Origin from a personal name.
namsor: FirstLastNameUSRaceEthnicityOut
Represents the output of inferring the LIKELY US 'race/ethnicity' from a personal name, given US country of residence and (optionally) a ZIP5 code.
Fields
- script string? - Script
- id string? - Id
- firstName string? - The first name (also known as given name)
- lastName string? - The last name (also known as family name, or surname)
- raceEthnicityAlt string? - Second most likely US 'race'/ethnicity
- raceEthnicity string? - Most likely US 'race'/ethnicity
- score decimal? - Higher score is better, but score is not normalized. Use calibratedProbability if available.
- raceEthnicitiesTop string[]? - List 'race'/ethnicities
- probabilityCalibrated decimal? - The calibrated probability for raceEthnicity to have been guessed correctly.
- probabilityAltCalibrated decimal? - The calibrated probability for raceEthnicity OR raceEthnicityAlt to have been guessed correctly.
namsor: MatchPersonalFirstLastNameIn
Fields
- id string? -
- name1 FirstLastNameIn? -
- name2 PersonalNameIn? -
namsor: NameGeoIn
Fields
- id string? -
- name string? -
- countryIso2 string? -
namsor: NameIn
Fields
- id string? -
- name string? -
namsor: NameMatchCandidateOut
The ordered list of name matching candidates
Fields
- candidateName string? - The name matching candidate name
- probability decimal? - The name matching estimated probability.
- predScoreGivenName decimal? - The given name prediction score.
- predScoreFamilyName decimal? - The family name prediction score.
namsor: NameMatchCandidatesOut
Classified matched names
Fields
- script string? - Script
- id string? - Id
- firstName string? - The first name (also known as given name)
- lastName string? - The last name (also known as family name, or surname)
- orderOption string? - The option for ordering
- matchCandidates NameMatchCandidateOut[]? - The ordered list of name matching candidates
namsor: NameMatchedOut
Classified matched names
Fields
- script string? - Script
- id string? - Id
- matchStatus string? - The name matching status.
- score decimal? - Score.
namsor: PersonalNameGenderedOut
Classified genderized names
Fields
- script string? - Script
- id string? - Id
- name string? - The input name
- likelyGender string? - Most likely gender
- genderScale decimal? - Compatibility to NamSor_v1 Gender Scale M[-1..U..+1]F value
- score decimal? - Compatibility to NamSor_v1 Gender score value. Higher score is better, but score is not normalized. Use calibratedProbability if available.
- probabilityCalibrated decimal? - The calibrated probability for inferred gender to have been guessed correctly.
namsor: PersonalNameGeoIn
Fields
- id string? -
- name string? -
- countryIso2 string? -
namsor: PersonalNameGeoOut
Classified geo names
Fields
- script string? - Script
- id string? - Id
- name string? - The input name.
- score decimal? - Higher score is better, but score is not normalized. Use calibratedProbability if available.
- country string? - Most likely country
- countryAlt string? - Second best alternative : country
- region string? - Most likely region (based on country ISO2 code)
- topRegion string? - Most likely top region (based on country ISO2 code)
- subRegion string? - Most likely sub region (based on country ISO2 code)
- countriesTop string[]? - List countries (top 10)
- probabilityCalibrated decimal? - The calibrated probability for country to have been guessed correctly.
- probabilityAltCalibrated decimal? - The calibrated probability for country OR countryAlt to have been guessed correctly.
namsor: PersonalNameIn
Fields
- id string? -
- name string? -
namsor: PersonalNameParsedOut
Fields
- script string? - Script
- id string? - Id
- name string? - The input name
- nameParserType string? - Name parsing is addressed as a classification problem, for example FN1LN1 means a first then last name order.
- nameParserTypeAlt string? - Second best alternative parsing. Name parsing is addressed as a classification problem, for example FN1LN1 means a first then last name order.
- firstLastName FirstLastNameOut? - First / last name structure corresponding to the most likely parsing.
- score decimal? - Higher score is better, but score is not normalized. Use calibratedProbability if available.
namsor: ProperNounCategorizedOut
Classified typed proper names
Fields
- script string? - Script
- id string? - Id
- name string? - The input name
- commonType string? - The most likely common name type
- commonTypeAlt string? - Best alternative for : The most likely common name type
- score decimal? - Higher score is better, but score is not normalized. Use calibratedProbability if available.
namsor: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
namsor: SoftwareVersionOut
The software version.
Fields
- softwareNameAndVersion string? - The software version
- softwareVersion int[]? - The software version major minor build
Import
import ballerinax/namsor;
Metadata
Released date: almost 2 years ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 2
Current verison: 2
Weekly downloads
Keywords
Lifestyle & Entertainment/News & Lifestyle
Cost/Freemium
Contributors