mitto.sms
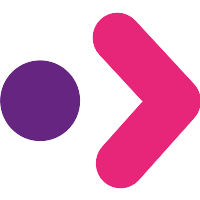
ballerinax/mitto.sms Ballerina library
Overview
This is a generated connector for Mitto SMS and Bulk SMS APIs v1 OpenAPI specification. Send individual SMS reliably and securely, or send them in bulk. You can choose from different servers to test with based on where you want to send a message to.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Mitto account
- Obtain tokens by following this guide
Quickstart
To use the Neutrino connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/mitto.sms
module into the Ballerina project.
import ballerinax/mitto.sms;
Step 2: Create a new connector instance
Create a sms:ApiKeysConfig
with the API key obtained, and initialize the connector with it.
sms:ApiKeysConfig config = { xMittoApiKey: "<API_KEY>" } sms:Client baseClient = check new Client(config);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to send an sms using the connector.
Send an sms
public function main() { sms:Body body = { 'from: "MITTO SMS", to: "15555552795", text: "Hello, World!", test: true }; sms:Sms response = check baseClient->sendSms(body); log:printInfo(response.toString()); }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
mitto.sms: Client
This is a generated connector for Mitto SMS and Bulk SMS APIs v1 OpenAPI specification. Send individual SMS reliably and securely, or send them in bulk. You can choose from different servers to test with based on where you want to send a message to.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Mitto account and obtain tokens by following this guide.
init (ApiKeysConfig apiKeyConfig, ConnectionConfig config, string serviceUrl)
- apiKeyConfig ApiKeysConfig - API keys for authorization
- config ConnectionConfig {} - The configurations to be used when initializing the
connector
- serviceUrl string "https://rest.mittoapi.net" - URL of the target service
sendSms
Send an SMS
Parameters
- payload SmsBody - Request payload
trackConversions
function trackConversions(SmsConvertedBody payload) returns Response|error
Track Conversions
Parameters
- payload SmsConvertedBody - Request payload
sendBulkSms
function sendBulkSms(SmsbulkBody payload) returns Smsbulk|error
Send SMS in Bulk
Parameters
- payload SmsbulkBody - Request payload
getUsageByCountry
function getUsageByCountry(UsageBycountryBody payload) returns Usage|error
Usage by Country
Parameters
- payload UsageBycountryBody - Request payload
Records
mitto.sms: ApiKeysConfig
Provides API key configurations needed when communicating with a remote HTTP endpoint.
Fields
- xMittoApiKey string - Represents API Key
X-Mitto-API-Key
mitto.sms: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
mitto.sms: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
mitto.sms: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
mitto.sms: Sms
Fields
- id string? - ID string of the SMS message. When the length of the body text of the message exceeds the limit, then several concatenated are send to the receiver and in that case only id of the first message is returned. Not returned when making a test call or when error occurs.
- timestamp string? - Timestamp of the SMS message in ISO 8601 format. Example - 2019-04-13T17:51:06.3091182Z For a description of the format, refer to Date and Time Formats page of the W3 Consortium.
- responseCode int? - Text describing the responseCode. For details, refer to Status Codes.
- textLength int? - The length of the message you sent. If there is an error, or if the message is empty, this will be 0.
- responseText string? - Text describing the responseCode. For details, refer to Status Codes.
- test boolean? - When "test" is set to true, it indicates you are making a test API call. (No actual SMS is sent.) When not present, or when "test" is set to false or not included, an actual message is sent.
mitto.sms: SmsBody
Fields
- test boolean? - When set to true, the API call is in test mode and no actual SMS is delivered. The response returns “test”: true. For test calls, there is no id parameter in the response. When set to false, you make the API call in production mode. The system delivers an SMS, and returns the id parameter in the response. If you do not use this parameter , by default you make the API call in production mode.
- 'from string - Free-form text with which the sender identifies themselves to the recipient. This is what the recipient will see as from whom the message is. Can be a phone number, or the name of the company or the service. NOTE - what you provide here may not be shown to the customer depending on how carriers choose to handle your message.
- to string - The number to which the message is sent. Numbers are specified in E.164 format.
- text string - The body of the SMS message. If the message contains characters outside the range of the GSM Standard and Extended tables, then you need to set the character encoding to Unicode (type=Unicode as a query parameter). For details, refer to Long SMSs and Changing the Default Encoding of the Message.
- 'type string? - Character set in which the message body will be encoded. If not specified, the default encoding (GSM) is used. For Unicode, set type Unicode. For Binary, set type to be Byte. For automatic detection set type to auto.
- reference string? - First metadata field for tagging the message. The metadata is returned in the delivery report by the callback. It can be any free-form text you consider appropriate. You can use a different reference string for each message or tag multiple messages with the same string and group them together in this way. For details, refer to Delivery Reports and Add Metadata to the Message.
- udh string? - Custom Hex-encoded User Data Header.
- validity int? - Validity period of the SMS message in minutes. When a message has not been delivered to the receiver at the first attempt, subsequent delivery attempts will be made until the validity period expires, after which the message is discarded as undeliverable. If the specified validity period is different from the validity period of the supplier, then the shorter period is enforced. When not specified, the validity of a message defaults to 2,880 minutes (48 hours).
- pid int? - Protocol identifier to use. Must be consistent with the udh parameter value. When not specified, defaults to 0.
- flash boolean? - When set to true it will send the message in Flash mode. Flash SMS is when the SMS appears directly on the main screen without user interaction, and is not automatically stored in the inbox.
- callback string? - When used, it overrides the URL address set on the account. For more details see the use case Replace Callback URL in API Call.
mitto.sms: Smsbulk
Fields
- responseCode int? -
- responseText string? -
- messages SmsbulkMessages? -
mitto.sms: SmsbulkBody
Fields
- test boolean? - When set to true, the API call is in test mode and no actual SMSes are delivered. The response returns “test”: true. For test calls, there is no id parameter in the response. When set to false, you make the API call in production mode. The system delivers an SMS, and returns the id parameter in the response. If you do not use this parameter , by default you make the API call in production mode.
- 'from string - Free-form text with which the sender identifies themselves to the recipient. This is what the recipient will see as from whom the message is. Can be a phone number, or the name of the company or the service. NOTE - what you provide here may not be shown to the customer depending on how carriers choose to handle your message.
- to string - A list of strings separated by commas. Each string is the number to which your message is sent. Numbers are specified in E.164 format. You can repeat the same phone number, and the message will be sent to the number as many times as the number is listed.
- text string - The body of the SMS message. If the message contains characters outside the range of the GSM Standard and Extended tables, then you need to set the character encoding to Unicode (type=Unicode as a query parameter). For details, refer to Long SMSs and Changing the Default Encoding of the Message.
- 'type string? - Character set in which the message body will be encoded. If not specified, the default encoding (GSM) is used. For Unicode, set type Unicode. For Binary, set type to be Byte. For automatic detection set type to auto.
- reference string? - First metadata field for tagging the message. The metadata is returned in the delivery report by the callback. It can be any free-form text you consider appropriate. You can use a different reference string for each message or tag multiple messages with the same string and group them together in this way.
- udh string? - Custom Hex-encoded User Data Header.
- validity int? - Validity period of the SMS message in minutes. When a message has not been delivered to the receiver at the first attempt, subsequent delivery attempts will be made until the validity period expires, after which the message is discarded as undeliverable. If the specified validity period is different from the validity period of the supplier, then the shorter period is enforced. When not specified, the validity of a message defaults to 2,880 minutes (48 hours).
- pid int? - Protocol identifier to use. Must be consistent with the udh parameter value. When not specified, defaults to 0.
- flash boolean? - When set to true it will send the message in Flash mode. Flash SMS is when the SMS appears directly on the main screen without user interaction, and is not automatically stored in the inbox.
- callback string? - When used, it overrides the URL address set on the account.
mitto.sms: SmsbulkMessages
Fields
- id string? - The unique identifier for the individual message sent to a phone number.
- timestamp string? -
mitto.sms: SmsConvertedBody
Fields
- messageId string? -
mitto.sms: Usage
Fields
- country string? - The country code expressed according to ISO 3166-1 Alpha-3 code format.
- messagesCount string? - Number of messages sent per country.
- cost string? - This is the account name tied to your API key. If unknown, please contact your account manager at Mitto for more information.
mitto.sms: UsageBycountryBody
Fields
- startDate string - The date you want to start retrieving usage retrieving usage information from. The date should be expressed according to ISO-8601 format YYYY-MM-DD or YYYYMMDD.
- endDate string - The date you want to stop retrieving usage information from. As with the start date, use ISO-8601 format YYYY-MM-DD or YYYYMMDD.
- accountName string - The account name tied to your API key. If unknown, please contact your Account Manager.
Import
import ballerinax/mitto.sms;
Metadata
Released date: almost 2 years ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 3
Current verison: 2
Weekly downloads
Keywords
Communication/Call & SMS
Cost/Paid
Contributors
Dependencies