microsoft.teams
Module microsoft.teams
API
Declarations
Definitions
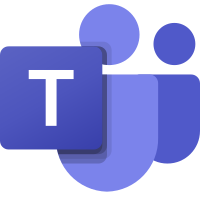
ballerinax/microsoft.teams Ballerina library
Overview
Ballerina connector for Microsoft Teams is connecting to MS Teams platform API in Microsoft Graph via Ballerina language. It provides capability to perform basic functionalities provided in MS Teams such as Sending messages, Viewing messages, Creating Teams, Channels and Chats, deleting and updating resources etc programmatically.
This module supports Microsoft Graph API v1.0
.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
-
Create an Azure account to register an application in the Azure portal
-
Obtain tokens
- Use this guide to register an application with the Microsoft identity platform
- The necessary scopes for this connector are shown below.
Permissions name Type Description Channel.Create Delegated Create channels Channel.Delete.All Delegated Delete channels Channel.ReadBasic.All Delegated Read the names and descriptions of channels ChannelMember.Read.All Delegated Read the members of channels ChannelMember.ReadWrite.All Delegated Add and remove members from channels ChannelMessage.Send Delegated Send channel messages ChannelSettings.ReadWrite.All Delegated Read and write the names, descriptions, and settings of channels Chat.Create Delegated Create chats Chat.ReadWrite Delegated Read and write user chat messages ChatMember.ReadWrite Delegated Add and remove members from chats ChatMessage.Send Delegated Send user chat messages Group.ReadWrite.All Delegated Read and write all groups Team.Create Delegated Create teams Team.ReadBasic.All Delegated Read the names and descriptions of teams TeamMember.ReadWrite.All Delegated Add and remove members from teams TeamSettings.ReadWrite.All Delegated Read and change teams' settings
Quickstart
To use the MS Teams connector in your Ballerina application, update the .bal file as follows:
Step 1 - Import connector
Import the ballerinax/microsoft.teams module into the Ballerina project.
import ballerinax/microsoft.teams;
Step 2 - Create a new connector instance
You can now make the connection configuration using the OAuth2 refresh token grant config.
teams:ConnectionConfig configuration = { auth: { refreshUrl: <REFRESH_URL>, refreshToken : <REFRESH_TOKEN>, clientId : <CLIENT_ID>, clientSecret : <CLIENT_SECRET> } }; teams:Client teamsClient = check new (configuration);
Step 3 - Invoke connector operation
- Create a team
teams:Team info = { displayName: "<TEAM_NAME>", description: "<TEAM_DESCRIPTION>" }; string|error newTeamId = teamsClient->createTeam(info); if (newTeamId is string) { log:printInfo("Team succesfully created " + newTeamId); log:printInfo("Success!"); } else { log:printError(newTeamId.message()); }
- Use
bal run
command to compile and run the Ballerina program
Clients
microsoft.teams: Client
This is connecting to the Microsoft Graph RESTful web API that enables you to access Microsoft Cloud service resources.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Microsoft 365 Work and School account
and obtain tokens following this guide. Configure the Access token to
have the required permission.
init (ConnectionConfig config)
- config ConnectionConfig - ConnectionConfig required to initialize the
Client
endpoint
createTeam
Creates a new team.
Parameters
- info Team - The information for creating team
createTeamFromGroup
Creates a new team from existing Azure AD group.
- In order to create a team, the group must have a least one owner.
Parameters
- groupId string - The Azure AD group ID
- info Team? (default ()) - The information for creating team
getTeam
Retrieves the properties and relationships of the specified team.
Parameters
- teamId string - The ID of the team
updateTeam
Updates the properties of the specified team.
Return Type
- error? -
error
if the operation fails or()
if nothing is to be returned
addMemberToTeam
function addMemberToTeam(string teamId, Member info) returns MemberData|error
Adds a new member to a team.
Return Type
- MemberData|error - A record
teams:Member
if success. Elseerror
deleteTeam
Deletes a group.
Parameters
- teamId string - The ID of the team
Return Type
- error? -
error
if the operation fails or()
if nothing is to be returned
getChannelsInTeam
function getChannelsInTeam(string teamId, string? queryParams) returns ChannelData[]|error
Retrieves the list of channels in a team.
Parameters
- teamId string - The ID of the team
Return Type
- ChannelData[]|error - An array of
teams:Channel
if success. Elseerror
createChannel
function createChannel(string teamId, Channel info) returns ChannelData|error
Creates a new channel.
Return Type
- ChannelData|error - A record
teams:Channel
if success. Elseerror
getChannel
function getChannel(string teamId, string channelId, string? queryParams) returns ChannelData|error
Gets a channel.
Return Type
- ChannelData|error - A record
teams:Channel
if success. Elseerror
updateChannel
Updates a channel.
Parameters
- teamId string - The ID of the team
- channelId string - The ID of the channel
- info Channel - The information to update the channel
Return Type
- error? -
error
if the operation fails or()
if nothing is to be returned
addMemberToChannel
function addMemberToChannel(string teamId, string channelId, string userId, string role) returns MemberData|error
Adds a new member to channel.
Parameters
- teamId string - The ID of the team
- channelId string - The ID of the channel
- userId string - The ID of the user to add to the channel
- role string - The role for the user. Must be
owner
or empty.
Return Type
- MemberData|error - A record
teams:MemberData
if success. Elseerror
listChannelMembers
function listChannelMembers(string teamId, string channelId) returns MemberData[]|error
Retrieves a list of members from a channel.
Return Type
- MemberData[]|error - An array of
teams:Member
if success. Elseerror
deleteChannelMember
Deletes a member from a channel.
Parameters
- teamId string - The ID of the team
- channelId string - The ID of the channel
- membershipId string - Membership ID of the member
Return Type
- error? -
error
if the operation fails or()
if nothing is to be returned
sendChannelMessage
function sendChannelMessage(string teamId, string channelId, Message body) returns MessageData|error
Sends a new message in the specified channel.
Parameters
- teamId string - The ID of the team
- channelId string - The ID of the channel
- body Message - The information to send in the message
Return Type
- MessageData|error - A record
teams:Message
if success. Elseerror
sendReplyMessage
function sendReplyMessage(string teamId, string channelId, string messageId, Message reply) returns MessageData|error
Sends a reply message to a message in a specified channel.
Parameters
- teamId string - The ID of the team
- channelId string - The ID of the channel
- messageId string - The ID of the message
- reply Message - The information to send in the reply
Return Type
- MessageData|error - A record
teams:Message
if success. Elseerror
deleteChannel
Deletes a group.
Return Type
- error? -
error
if the operation fails or()
if nothing is to be returned
createChat
Creates a new chat.
Parameters
- info Chat - The information for the new chat
getChat
Retrieves a single chat (without its messages).
Parameters
- chatId string - The ID of the chat
updateChat
Updates the properties of a chat object.
listChatMembers
function listChatMembers(string chatId) returns MemberData[]|error
Lists all conversation members in a chat.
Parameters
- chatId string - The ID of the chat
Return Type
- MemberData[]|error - An array of
teams:MemberData
if success. Elseerror
addMemberToChat
function addMemberToChat(string chatId, Member data) returns MemberData|error
Adds a new member to a chat.
Return Type
- MemberData|error - A record
teams:MemberData
if success. Elseerror
removeMemberFromChat
Removes a member from a chat.
Return Type
- error? -
error
if the operation fails or()
if nothing is to be returned
sendChatMessage
function sendChatMessage(string chatId, Message body) returns MessageData|error
Sends a new message in the specified chat.
Return Type
- MessageData|error - A record
teams:Message
if success. Elseerror
getChatMessage
function getChatMessage(string chatId, string messageId) returns MessageData[]|error
Retrieves a single message or a message reply in a chat.
Return Type
- MessageData[]|error - An array of
teams:Message
if success. Elseerror
Enums
microsoft.teams: ChannelMemberhipType
The type of the channel.
Members
microsoft.teams: ChatType
Specifies the type of chat.
Members
microsoft.teams: GiphyContentRating
Giphy content rating.
Members
microsoft.teams: MessageContentType
The type of the content.
Members
microsoft.teams: MessageImportance
The importance of the chat message.
Members
microsoft.teams: ReactionType
The reaction type.
Members
microsoft.teams: Role
The role of a user.
Members
microsoft.teams: TeamSpecialization
Indicates whether the team is intended for a particular use case.
Members
microsoft.teams: TeamVisibility
Describes the visibility of a team.
Members
Records
microsoft.teams: Channel
A channel in a Microsoft Team.
Fields
- displayName string - Channel name as it will appear to the user in Microsoft Teams
- Fields Included from *ChannelBaseData
- description string|()
- isFavoriteByDefault boolean|()
- membershipType ChannelMemberhipType
microsoft.teams: ChannelBaseData
Generic information for a channel.
Fields
- description string? - Optional textual description for the channel
- isFavoriteByDefault boolean? - Indicates whether the channel should automatically be marked 'favorite' for all members of the team
- membershipType ChannelMemberhipType? - The type of the channel
microsoft.teams: ChannelData
All information about a channel.
Fields
- id string? - The channel ID
- displayName string? - Channel name as it will appear to the user in Microsoft Teams
- email string? - The email address for sending messages to the channel
- webUrl string? - A hyperlink that will go to the channel in Microsoft Teams
- createdDateTime string? - Timestamp at which the channel was created
- Fields Included from *ChannelBaseData
- description string|()
- isFavoriteByDefault boolean|()
- membershipType ChannelMemberhipType
microsoft.teams: ChannelIdentity
Basic identification information about a channel in Microsoft Teams.
Fields
- teamId string - The identity of the channel in which the message was posted
- channelId string - The identity of the team in which the message was posted
microsoft.teams: Chat
A chat which is a collection of chatMessages between one or more participants.
Participants can be users or apps.
Fields
- chatType ChatType - Specifies the type of chat
- members Member[] - List of conversation members that should be added. Every single user, including the user initiating the create request, who will participate in the chat must be specified in this list.
- topic string? - Subject or topic for the chat. (Only available for group chats)
microsoft.teams: ChatData
Readonly data for a chat.
Fields
- id string? - Chat ID
- topic string? - Subject or topic for the chat. (Only available for group chats)
- chatType ChatType? - Specifies the type of chat
- createdDateTime string? - Date and time at which the chat was created
- lastUpdatedDateTime string? - Date and time at which the chat was renamed or list of members were last changed
- members Member[]? - The list of members in the conversation
microsoft.teams: ConnectionConfig
Client configuration details.
Fields
- Fields Included from *ConnectionConfig
- auth AuthConfig
- httpVersion HttpVersion
- http1Settings ClientHttp1Settings
- http2Settings ClientHttp2Settings
- timeout decimal
- forwarded string
- poolConfig PoolConfiguration
- cache CacheConfig
- compression Compression
- circuitBreaker CircuitBreakerConfig
- retryConfig RetryConfig
- responseLimits ResponseLimitConfigs
- secureSocket ClientSecureSocket
- proxy ProxyConfig
- validation boolean
- anydata...
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig - Configurations related to client authentication
microsoft.teams: Identity
An identity of a user, device, or application.
Fields
- id string - Unique identifier for the identity
- displayName string? - The identity's display name
microsoft.teams: IdentitySet
A set of identities associated with various events for an item.
Fields
- application Identity? - The application associated with this action
- device Identity? - The device associated with this action
- user Identity - The user associated with this action
microsoft.teams: ItemBody
Properties of the body of an item, such as a message, event or group post.
Fields
- content string - The content of the item
- contentType MessageContentType? - The type of the content. Possible values are
text
andhtml
microsoft.teams: Member
A user in a team, a channel, or a chat.
Fields
- roles Role[] - The roles for the user
- Fields Included from *MemberBaseData
microsoft.teams: MemberBaseData
Generic data for a user in a team, a channel, or a chat.
Fields
- visibleHistoryStartDateTime string? - The timestamp denoting how far back a conversation's history is shared with the conversation member
- email string? - The email address of the user
- displayName string? - The display name of the user
- userId string? - The guid of the user
microsoft.teams: MemberData
All information about a user in a team, a channel, or a chat.
Fields
- id string? - Unique ID of the user
- roles Role[]? - The roles for the user
- tenantId string? - TenantId which the Azure AD user belongs to
- Fields Included from *MemberBaseData
microsoft.teams: Message
An individual chat message within a channel or chat.
Fields
- body ItemBody - Plaintext/HTML representation of the content of the chat message
- Fields Included from *MessageBaseData
- subject string|()
- importance MessageImportance
- mentions MessageMention[]
microsoft.teams: MessageBaseData
Generic information for an individual chat message within a channel or chat.
Fields
- subject string? - The subject of the chat message, in plaintext
- importance MessageImportance? - The importance of the chat message. The possible values are:
normal
,high
,urgent
- mentions MessageMention[]? - List of entities mentioned in the chat message. Currently supports user, bot, team, channel.
microsoft.teams: MessageData
All information for an individual chat message within a channel or chat.
Fields
- id string? - Message ID
- body ItemBody? - Plaintext/HTML representation of the content of the chat message
- etag string? - Version number of the chat message
- replyToId string? - Id of the parent chat message or root chat message of the thread
- lastModifiedDateTime string? - Timestamp when the chat message is created (initial setting) or modified, including when a reaction is added or removed.
- createdDateTime string? - Timestamp of when the chat message was created
- lastEditedDateTime string? - Timestamp when edits to the chat message were made. Triggers an "Edited" flag in the Teams UI. If no edits are made the value is null.
- deletedDateTime string? - Timestamp at which the chat message was deleted, or null if not deleted
- chatId string? - If the message was sent in a chat, represents the identity of the chat
- webUrl string? - Link to the message in Microsoft Teams
- policyViolation MessagePolicyViolation? - Defines the properties of a policy violation set by a data loss prevention (DLP) application
- channelIdentity ChannelIdentity? - If the message was sent in a channel, represents identity of the channel
- messageType string? - The type of chat message
- summary string? - Summary text of the chat message that could be used for push notifications and summary views or fall back views
- locale string? - Locale of the chat message set by the client. Always set to en-us.
- 'from IdentitySet? - Details of the sender of the chat message
- reactions MessageReaction[]? - Collection Reactions for this chat message (for example, Like)
- Fields Included from *MessageBaseData
- subject string|()
- importance MessageImportance
- mentions MessageMention[]
microsoft.teams: MessageMention
A mention in a chatMessage entity.
The mention can be to a user, team, bot, or channel.
Fields
- id Unsigned32? - Index of an entity being mentioned in the specified Message
- mentionText string - String used to represent the mention. For example, a user's display name, a team name
- mentioned IdentitySet - The entity (user, application, team, or channel) that was mentioned
microsoft.teams: MessagePolicyViolation
A policy violation on a chat message.
Fields
- dlpAction string - The action taken by the DLP provider on the message with sensitive content
- justificationText string - Justification text provided by the sender of the message when overriding a policy violation
- policyTip PolicyTip - Information to display to the message sender about why the message was flagged as a violation
- userAction string - Indicates the action taken by the user on a message blocked by the DLP provider
- verdictDetails string - Indicates what actions the sender may take in response to the policy violation
microsoft.teams: MessageReaction
A reaction to a chatMessage entity.
Fields
- createdDateTime string - The Timestamp type represents date and time information using ISO 8601 format and is always in UTC
time. eg:
midnight UTC on Jan 1, 2014 is 2014-01-01T00:00:00Z
- reactionType ReactionType - Supported values are
like
,angry
,sad
,laugh
,heart
,surprised
- user IdentitySet - The user who reacted to the message
microsoft.teams: PolicyTip
The properties of a policy tip on a MessagePolicyViolation
object.
Policy tips provide the sender with
information about the policy violation.
Fields
- complianceUrl string - The URL a user can visit to read about the data loss prevention policies for the organization
- generalText string - Explanatory text shown to the sender of the message
- matchedConditionDescriptions string[] - The list of improper data in the message that was detected by the data loss prevention app
microsoft.teams: Team
Generic information about a team.
Fields
- displayName string? - The name of the team
- description string? - An optional description for the team. Maximum length: 1024 characters.
- classification string? - Describes the data or business sensitivity of the team
- specialization TeamSpecialization? - Indicates whether the team is intended for a particular use case
- visibility TeamVisibility? - The visibility of the group and team
- funSettings TeamFunSettings? - Settings to configure use of Giphy, memes, and stickers in the team
- guestSettings TeamGuestSettings? - Settings to configure whether guests can create, update, or delete channels in the team
- memberSettings TeamMemberSettings? - Settings to configure whether members can perform certain actions, for example, create channels and add bots, in the team
- messagingSettings TeamMessagingSettings? - Settings to configure messaging and mentions in the team
- isArchived boolean? - represent whether this team is in read-only mode
microsoft.teams: TeamData
All the information about a team.
Fields
- Fields Included from *Team
- displayName string
- description string
- classification string|()
- specialization TeamSpecialization
- visibility TeamVisibility
- funSettings TeamFunSettings
- guestSettings TeamGuestSettings
- memberSettings TeamMemberSettings
- messagingSettings TeamMessagingSettings
- isArchived boolean
- id string? - ID of the team
- webUrl string? - A hyperlink that will go to the team in the Microsoft Teams client
- createdDateTime string? - Timestamp at which the team was created
- internalId string? - The internal ID of the team
- operations TeamsAsyncOperation[]? - The async operations that ran or are running on this team
microsoft.teams: TeamFunSettings
Settings to configure use of Giphy, memes, and stickers in the team.
Fields
- allowGiphy boolean? - If set to true, enables Giphy use
- giphyContentRating GiphyContentRating? - Giphy content rating. Possible values are:
moderate
,strict
- allowStickersAndMemes boolean? - If set to true, enables users to include stickers and memes
- allowCustomMemes boolean? - If set to true, enables users to include custom memes
microsoft.teams: TeamGuestSettings
Settings to configure whether guests can create, update, or delete channels in the team.
Fields
- allowCreateUpdateChannels boolean? - If set to true, guests can add and update channels
- allowDeleteChannels boolean? - If set to true, guests can delete channels
microsoft.teams: TeamMemberSettings
Settings to configure whether members can perform certain actions, for example, create channels and add bots, in the team.
Fields
- Fields Included from *TeamGuestSettings
- allowCreatePrivateChannels boolean? - If set to true, members can add and update private channels
- allowAddRemoveApps boolean? - f set to true, members can add and remove apps
- allowCreateUpdateRemoveTabs boolean? - If set to true, members can add, update, and remove tabs
- allowCreateUpdateRemoveConnectors boolean? - If set to true, members can add, update, and remove connectors
microsoft.teams: TeamMessagingSettings
Settings to configure messaging and mentions in the team.
Fields
- allowUserEditMessages boolean? - If set to true, users can edit their messages
- allowUserDeleteMessages boolean? - If set to true, users can delete their messages
- allowOwnerDeleteMessages boolean? - If set to true, owners can delete any message
- allowTeamMentions boolean? - If set to true, @team mentions are allowed
- allowChannelMentions boolean? - If set to true, @channel mentions are allowed
microsoft.teams: TeamsAsyncOperation
Information about an operation that transcends the lifetime of a single API request.
Fields
- id string - Unique operation ID
- operationType string - Denotes which type of operation is being described
- createdDateTime string - Time when the operation was created
- status string - Operation status
- lastActionDateTime string - Time when the async operation was last updated
- attemptsCount int - Number of times the operation was attempted before being marked successful or failed
- targetResourceId string - The ID of the object that's created or modified as result of this async operation, typically a team
- targetResourceLocation string - The location of the object that's created or modified as result of this async operation
Import
import ballerinax/microsoft.teams;
Metadata
Released date: about 2 years ago
Version: 2.4.0
License: Apache-2.0
Compatibility
Platform: java11
Ballerina version: 2201.4.1
Pull count
Total: 1978
Current verison: 249
Weekly downloads
Keywords
Communication/Team Chat
Cost/Paid
Vendor/Microsoft
Contributors