microsoft.outlook.mail
Module microsoft.outlook.mail
API
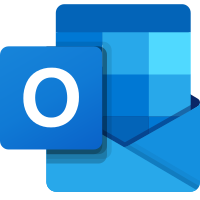
ballerinax/microsoft.outlook.mail Ballerina library
Overview
Ballerina connector for Microsoft Outlook mail provides access to the Microsoft Outlook mail service in Microsoft Graph v1.0 via the Ballerina language. It provides the capability to perform more useful functionalities provided in Microsoft outlook mail such as sending messages, listing messages, creating drafts, mail folders, deleting messages, updating messages, etc.
This module supports Microsoft Graph (Mail) API v1.0.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create Microsoft Outlook Account
- Obtaining tokens
- Follow this link and obtain the client ID, client secret, and refresh token.
Quickstart
To use the Outlook mail connector in your Ballerina application, update the .bal file as follows:
Step 1 - Import connector
Import the ballerinax/microsoft.outlook.mail
module into the Ballerina project.
import ballerinax/microsoft.outlook.mail;
Step 2: - Create a new connector instance
You can now make the connection configuration using the OAuth2 refresh token grant config.
mail:ConnectionConfig configuration = { auth: { refreshUrl: <REFRESH_URL>, refreshToken : <REFRESH_TOKEN>, clientId : <CLIENT_ID>, clientSecret : <CLIENT_SECRET> } }; mail:Client outlookClient = check new(configuration);
Step 3: Invoke connector operation
- Send a new message using the sendMessage remote operation
public function main() returns error? { mail:MessageContent messageContent = { message: { subject: "Ballerina Test Email", importance: "Low", body: { "contentType": "HTML", "content": "This is sent by sendMessage operation <b>Test</b>!" }, toRecipients: [ { emailAddress: { address: "<email address>", name: "<name>" } } ] }, saveToSentItems: true }; http:Response response = check oneDriveClient->sendMessage(messageContent); }
- Use
bal run
command to compile and run the Ballerina program.
Clients
microsoft.outlook.mail: Client
Microsoft Outlook mail API provides capability to access Outlook mail operations related to messages, attachments, drafts, mail folder, and child mail folders.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create an Microsoft Outlook Account and obtain tokens by following
this guide
init (ConnectionConfig config)
- config ConnectionConfig - Configuration for client connector
listMessages
function listMessages(string? folderId, string? optionalUriParameters) returns error|stream<Message, error?>
Gets the messages in the signed-in user's mailbox (including the Deleted Items and Clutter folders)
Parameters
- folderId string? (default ()) - The ID of the specific folder in the user's mailbox or the name of well-known folders (inbox, sentitems etc.)
- optionalUriParameters string? (default ()) - The optional query parameter string (https://docs.microsoft.com/en-us/graph/api/user-list-messages?view=graph-rest-1.0&tabs=http#optional-query-parameters)
Return Type
createMessage
function createMessage(DraftMessage message, string? folderId) returns Message|error
Creates a draft of a new message
Parameters
- message DraftMessage - The detail of the draft
- folderId string? (default ()) - The mail folder where the draft should be saved in
Return Type
getMessage
function getMessage(string messageId, string? folderId, string? optionalUriParameters, string bodyContentType) returns Message|error
Retrieves the properties and relationships of a message
Parameters
- messageId string - The ID of the message
- folderId string? (default ()) - The ID of the folder where the message is in
- optionalUriParameters string? (default ()) - The OData Query Parameters to help customize the response (https://docs.microsoft.com/en-us/graph/api/message-get?view=graph-rest-1.0&tabs=http#optional-query-parameters)
- bodyContentType string (default "html") - The format of the body and uniqueBody of the specified message (values : html (default), text)
Return Type
updateMessage
function updateMessage(string messageId, MessageUpdateContent message, string? folderId) returns Message|error
Updates the properties of a message
Parameters
- messageId string - The ID of the message
- message MessageUpdateContent - The message properties to be updated
- folderId string? (default ()) - The ID of the folder where the message is in
Return Type
deleteMessage
Deletes a message in the specified user's mailbox
Parameters
- messageId string - The ID of the message
- folderId string? (default ()) - The ID of the folder where the message is in
sendDraftMessage
Sends an existing draft message
Parameters
- messageId string - The ID of the message
copyMessage
function copyMessage(string messageId, string destinationFolderId, string? folderId) returns Response|error
Copies a message to a folder
Parameters
- messageId string - The ID of the message
- destinationFolderId string - The ID of the destination folder
- folderId string? (default ()) - The ID of the folder where the message is in
forwardMessage
function forwardMessage(string messageId, string comment, string[] addressList, string? folderId) returns Response|error
Forwards a message
Parameters
- messageId string - The ID of the message
- comment string - The comment of the forwarding message
- addressList string[] - The receivers' email list
- folderId string? (default ()) - The ID of the folder where the message is in
Return Type
sendMessage
function sendMessage(MessageContent messageContent) returns Response|error
Sends a message
Parameters
- messageContent MessageContent - The message content and properties
listAttachment
function listAttachment(string messageId, string? folderId, string[]? childFolderIds) returns stream<FileAttachment, error?>|error
Retrieves a list of attachments
Parameters
- messageId string - The ID of the message
- folderId string? (default ()) - The ID of the folder
- childFolderIds string[]? (default ()) - The IDs of the childFolders respectively
Return Type
- stream<FileAttachment, error?>|error - If success returns a ballerina stream of file attachment records otherwise the relevant error
createMailFolder
function createMailFolder(string displayName, boolean? isHidden) returns MailFolder|error
Creates a new mail folder in the root folder of the user's mailbox
Parameters
- displayName string - The display name of the mail folder
- isHidden boolean? (default ()) - Indicates whether the folder should be hidden or not
Return Type
- MailFolder|error - If success returns the created mail folder detail otherwise the relevant error
createChildMailFolder
function createChildMailFolder(string parentFolderId, string displayName, boolean? isHidden) returns MailFolder|error
Creates a new child mailFolder
Parameters
- parentFolderId string - The ID of the parent folder
- displayName string - The display name of the child mail folder
- isHidden boolean? (default ()) - Indicates whether the child folder should be hidden or not
Return Type
- MailFolder|error - If success returns the created mail folder detail otherwise the relevant error
getMailFolder
function getMailFolder(string mailFolderId) returns MailFolder|error
Retrieves the details of a message folder
Parameters
- mailFolderId string - The ID of the mail folder
Return Type
- MailFolder|error - If success returns the requested mail folder details as a mail folder record otherwise the relevant error
deleteMailFolder
Deletes the specified mail folder or search Mail folder
Parameters
- mailFolderId string - The ID of the folder by its well-known folder name, if one exists ( Eg: inbox, sentitems etc. https://docs.microsoft.com/en-us/graph/api/resources/mailfolder?view=graph-rest-1.0)
addFileAttachment
function addFileAttachment(string messageId, FileAttachment attachment, string? folderId, string[]? childFolderIds) returns FileAttachment|error
Adds an attachment that's smaller than 3MB to a message
Parameters
- messageId string - The ID of the message
- attachment FileAttachment - The File attachment detail
- folderId string? (default ()) - The ID of the folder where the message is saved in
- childFolderIds string[]? (default ()) - The IDs of the child folders
Return Type
- FileAttachment|error - If success returns the added file attachment details as a record otherwise the relevant error
listMailFolders
function listMailFolders(boolean? includeHiddenFolders) returns stream<MailFolder, error?>|error
Gets the mail folder collection directly under the root folder
Parameters
- includeHiddenFolders boolean? (default ()) - Indicates whether the hidden folder should be included in the collection or not
Return Type
- stream<MailFolder, error?>|error - If success returns a ballerina stream of mail folder records otherwise the relevant error
listChildMailFolders
function listChildMailFolders(string parentFolderId, boolean? includeHiddenFolders) returns stream<MailFolder, error?>|error
Gets the folder collection under the specified folder.
Parameters
- parentFolderId string - The ID of the parent folder
- includeHiddenFolders boolean? (default ()) - Indicates whether the hidden folder should be included in the collection or not
Return Type
- stream<MailFolder, error?>|error - If success returns a ballerina stream of mail folder records otherwise the relevant error
createMailSearchFolder
function createMailSearchFolder(string parentFolderId, MailSearchFolder mailSearchFolder) returns MailSearchFolder|error
Creates a new mailSearchFolder in the specified user's mailbox
Parameters
- parentFolderId string - The ID of the parent folder
- mailSearchFolder MailSearchFolder - The details of the mail search folder
Return Type
- MailSearchFolder|error - If success returns the newly created mail search folder details as a MailSearchFolder record otherwise the relevant error
addLargeFileAttachments
function addLargeFileAttachments(string messageId, string attachmentName, stream<Block, error?>|string|byte[] file, int? fileSize) returns error?
Creates an upload session that allows an the client to iteratively upload a file that's lager than 3 MB and lesser than 150MB
Parameters
- messageId string - The ID of the message
- attachmentName string - The name of the attachment in the message
- fileSize int? (default ()) - Size of the file is mandatory for io:Block stream uploading
Return Type
- error? - If success returns null otherwise the relevant error
deleteAttachment
function deleteAttachment(string messageId, string attachmentID, string? folderId, string[]? childFolderIds) returns error?
Deletes an attachment
Parameters
- messageId string - Message ID
- attachmentID string - Attachment ID
- folderId string? (default ()) - Folder ID where the file is saved in
- childFolderIds string[]? (default ()) - Child folder IDs
Return Type
- error? - If success returns null otherwise the relevant error
Records
microsoft.outlook.mail: AttachmentItemContent
Represents elated content to a message in the form of an attachment
Fields
- attachmentType string - The attachment type
- contentType string? - The MIME type
- isInline boolean? - true if the attachment is an inline attachment; otherwise, false
- name string - The attachment's file name
- lastModifiedDateTime string? - The Timestamp type represents date and time information using ISO 8601 format and is always in UTC time. For example, midnight UTC on Jan 1, 2014 is 2014-01-01T00:00:00Z
- size int - The length of the attachment in bytes
microsoft.outlook.mail: ConnectionConfig
Represents configuration parameters to create Microsoft Outlook Mail client.
Fields
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig - Configurations related to client authentication
- httpVersion string(default "1.1") - The HTTP version understood by the client
- http1Settings ClientHttp1Settings(default {}) - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings(default {}) - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- followRedirects FollowRedirects?(default ()) - Configurations associated with Redirection
- poolConfig PoolConfiguration?(default ()) - Configurations associated with request pooling
- cache CacheConfig(default {}) - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig?(default ()) - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig?(default ()) - Configurations associated with retrying
- cookieConfig CookieConfig?(default ()) - Configurations associated with cookies
- responseLimits ResponseLimitConfigs(default {}) - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket?(default ()) - SSL/TLS-related options
microsoft.outlook.mail: CookieConfig
Client configuration for cookies.
Fields
- enabled boolean(default false) - User agents provide users with a mechanism for disabling or enabling cookies
- maxCookiesPerDomain int(default 50) - Maximum number of cookies per domain, which is 50
- maxTotalCookieCount int(default 3000) - Maximum number of total cookies allowed to be stored in cookie store, which is 3000
- blockThirdPartyCookies boolean(default true) - User can block cookies from third party responses and refuse to send cookies for third party requests, if needed
microsoft.outlook.mail: DraftMessage
Represents detail of a draft
Fields
- subject string? - The subject of the message
- toRecipients Recipient[]? - The To: recipients for the message
- ccRecipients Recipient[]? - The Cc: recipients for the message
- bccRecipients Recipient[]? - The Bcc: recipients for the message
- replyTo Recipient[]? - The email addresses to use when replying
- importance string? - The importance of the message. The possible values are: low, normal, and high
- body ItemBody? - The body of the message. It can be in HTML or text format
- parentFolderId string? - The ID of the parent folder of the message is saved in
- categories string[]? - The categories associated with the message
- inferenceClassification string? - The classification of the message for the user, based on inferred relevance or importance, or on an explicit override. The possible values are: focused or other.
- isDeliveryReceiptRequested boolean? - Indicates whether a read receipt is requested for the message
- isReadReceiptRequested boolean? - Indicates whether a read receipt is requested for the message
microsoft.outlook.mail: EmailAddress
The name and email address of a contact or message recipient
Fields
- address string - The email address of the person or entity
- name string? - The display name of the person or entity
microsoft.outlook.mail: FileAttachment
A file (such as a text file or Word document) attached to a message
Fields
- name string - The name representing the text that is displayed below the icon representing the embedded attachment. This does not need to be the actual file name.
- contentType string - The content type of the attachment
- contentBytes string - The base64-encoded contents of the file
- contentId string? - The ID of the attachment in the Exchange store
- isInline boolean? - Set to true if this is an inline attachment
- lastModifiedDateTime string? - The date and time when the attachment was last modified
- size int? - The size in bytes of the attachment
- id string? - The attachment ID
microsoft.outlook.mail: Flag
Allows setting a flag in an item for the user to follow up on later
Fields
- completedDateTime string? - The date and time that the follow-up was finished
- dueDateTime string? - The date and time that the follow up is to be finished. Note: To set the due date, you must also specify the startDateTime; otherwise, you will get a 400 Bad Request response.
- flagStatus string - The date and time that the follow up is to be finished. Note: To set the due date, you must also specify the startDateTime; otherwise, you will get a 400 Bad Request response.
- startDateTime string? - The date and time that the follow-up is to begin
microsoft.outlook.mail: ForwardParamsList
Represents the list of forward Recipients and the comment
Fields
- comment string - The comment of the forward message
- toRecipients Recipient[] - The recipient list
microsoft.outlook.mail: InternetMessageHeader
A key-value pair that represents an Internet message header, as defined by RFC5322
Fields
- name string - Represents the key in a key-value pair
- value string - The value in a key-value pair
microsoft.outlook.mail: ItemBody
Represents properties of the body of an item, such as a message, event or group post
Fields
- content string - The content of the item
- contentType string - The type of the content. Possible values are text and html
microsoft.outlook.mail: LargeFileAttachment
Represents a large size file attachment
Fields
- AttachmentItem AttachmentItemContent - Detail of the attachment
microsoft.outlook.mail: MailFolder
A mail folder in a user's mailbox, such as Inbox and Drafts. Mail folders can contain messages, other Outlook items, and child mail folders. Outlook creates certain folders for users by default. Instead of using the corresponding folder id value, for convenience, you can use the well-known folder names from the table below when accessing these folders. For example, you can get the Drafts folder using its well-known name with the following query.
Fields
- childFolderCount int? - The number of immediate child mailFolders in the current mailFolder
- displayName string - The mailFolder's display name
- id string? - The mailFolder's unique identifier
- isHidden boolean? - Indicates whether the mailFolder is hidden. This property can be set only when creating the folder
- parentFolderId string? - The unique identifier for the mailFolder's parent mailFolder
- totalItemCount int? - The number of items in the mailFolder
- unreadItemCount int? - The number of items in the mailFolder marked as unread
- childFolders MailFolder[]? - The collection of child folders in the mailFolder
- messages Message[]? - The collection of messages in the mailFolder
- sizeInBytes int? - Size of the content in bytes
microsoft.outlook.mail: MailSearchFolder
A mailSearchFolder is a virtual folder in the user's mailbox that contains all the email items matching specified search criteria.
Fields
- displayName string - Display name of the folder
- isSupported boolean? - Indicates whether a search folder is editable using REST APIs
- includeNestedFolders boolean? - Indicates how the mailbox folder hierarchy should be traversed in the search. true means that a deep search should be done to include child folders in the hierarchy of each folder explicitly specified in sourceFolderIds. false means a shallow search of only each of the folders explicitly specified in sourceFolderIds.
- sourceFolderIds string[]? - The mailbox folders that should be mined
- filterQuery string? - The OData query to filter the messages
- id string? - The ID of the mail search folder
microsoft.outlook.mail: Message
A message in a mailFolder
Fields
- 'from Recipient? - The owner of the mailbox from which the message is sent
- ccRecipients Recipient[]? - Field Description
- bccRecipients Recipient[]? - The Bcc: recipients for the message
- replyTo Recipient[]? - The email addresses to use when replying
- subject string? - The subject of the message
- body ItemBody? - The body of the message. It can be in HTML or text format
- bodyPreview string? - The body of the message. It can be in HTML or text format
- categories string[]? - The categories associated with the message
- changeKey string? - The version of the message
- conversationId string? - The ID of the conversation the email belongs to
- conversationIndex string? - Indicates the position of the message within the conversation
- createdDateTime string? - The date and time the message was created. The date and time information uses ISO 8601 format and is always in UTC time. For example, midnight UTC on Jan 1, 2014 is 2014-01-01T00:00:00Z.
- hasAttachments boolean? - Indicates whether the message has attachments
- importance string? - The importance of the message. The possible values are: low, normal, and high
- inferenceClassification string? - he classification of the message for the user, based on inferred relevance or importance, or on an explicit override. The possible values are: focused or other.
- internetMessageHeader InternetMessageHeader[]? - A collection of message headers defined by RFC5322
- internetMessageId string? - The message ID in the format specified by RFC2822
- isDeliveryReceiptRequested boolean? - Indicates whether a read receipt is requested for the message
- isDraft boolean? - Indicates whether the message is a draft
- isRead boolean? - Indicates whether the message has been read
- isReadReceiptRequested boolean? - Indicates whether a read receipt is requested for the message
- lastModifiedDateTime string? - The date and time the message was last changed. The date and time information uses ISO 8601 format and is always in UTC time. For example, midnight UTC on Jan 1, 2014 is 2014-01-01T00:00:00Z
- parentFolderId string? - The unique identifier for the message's parent mailFolder
- receivedDateTime string? - The date and time the message was received
- sender Recipient? - The account that is actually used to generate the message
- sentDateTime string? - The date and time the message was sent. The date and time information uses ISO 8601 format and is always in UTC time. For example, midnight UTC on Jan 1, 2014 is 2014-01-01T00:00:00Z
- toRecipients Recipient[]? - The To: recipients for the message
- uniqueBody ItemBody? - The part of the body of the message that is unique to the current message. uniqueBody is not returned by default but can be retrieved for a given message by use of the ?$select=uniqueBody query. It can be in HTML or text format
- webLink string? - The URL to open the message in Outlook on the web
- flag Flag? - The flag value that indicates the status, start date, due date, or completion date for the message
- id string? - Unique identifier for the message (note that this value may change if a message is moved or altered)
microsoft.outlook.mail: MessageContent
The properties of a message
Fields
- message MessageRequestBody - Message Details
- saveToSentItems boolean? - Indicates whether the message is saved in "sentitem" or not
microsoft.outlook.mail: MessageRequestBody
Represents the message detail to be sent
Fields
- subject string? - The subject of the message
- toRecipients Recipient[]? - The To: recipients for the message
- ccRecipients Recipient[]? - The Cc: recipients for the message
- bccRecipients Recipient[]? - The Bcc: recipients for the message
- body ItemBody? - The body of the message. It can be in HTML or text format
- categories string[]? - The categories associated with the message
- hasAttachments boolean? - Indicates whether the message has attachments
- importance string? - The importance of the message. The possible values are: low, normal, and high.
- inferenceClassification string? - The classification of the message for the user, based on inferred relevance or importance, or on an explicit override. The possible values are: focused or other.
- isDeliveryReceiptRequested boolean? - Indicates whether a read receipt is requested for the message.
- isReadReceiptRequested boolean? - Indicates whether a read receipt is requested for the message
- attachments FileAttachment[]? - The fileAttachment and itemAttachment attachments for the message
microsoft.outlook.mail: MessageUpdateContent
Represents a message to be updated
Fields
- bccRecipients Recipient[]? - The Bcc: recipients for the message
- ccRecipients Recipient[]? - The Cc: recipients for the message
- 'from Recipient? - The owner of the mailbox from which the message is sent
- body ItemBody? - The body of the message. It can be in HTML or text format. Find out about safe HTML in a message body
- subject string? - The subject of the message
- toRecipients Recipient[]? - The To: recipients for the message
- categories string[]? - The categories associated with the message
- importance string? - The importance of the message. The possible values are: low, normal, and high
- inferenceClassification string? - The classification of the message for the user, based on inferred relevance or importance, or on an explicit override. The possible values are: focused or other.
- changeKey string? - The version of the message
- conversationId string? - The ID of the conversation the email belongs to
- conversationIndex string? - Indicates the position of the message within the conversation
- createdDateTime string? - The date and time the message was created. The date and time information uses ISO 8601 format and is always in UTC time. For example, midnight UTC on Jan 1, 2014 is 2014-01-01T00:00:00Z.
- hasAttachments boolean? - Indicates whether the message has attachments
- internetMessageId string? - The message ID in the format specified by RFC2822
- isDeliveryReceiptRequested boolean? - Indicates whether a read receipt is requested for the message
- isReadReceiptRequested boolean? - Indicates whether a read receipt is requested for the message
- isRead boolean? - Indicates whether the message has been read
- replyTo Recipient[]? - The email addresses to use when replying
- sender Recipient? - The account that is actually used to generate the message
- flag Flag? - The flag value that indicates the status, start date, due date, or completion date for the message
microsoft.outlook.mail: Recipient
Represents a message recipient
Fields
- emailAddress EmailAddress - the email address of the recipient
microsoft.outlook.mail: UploadSession
Represents a detail of upload session
Fields
- expirationDateTime string - The date/time of session expires
- nextExpectedRanges string[] - The next range of bytes to be uploaded
- uploadUrl string? - The URL for the upload requests
Import
import ballerinax/microsoft.outlook.mail;
Metadata
Released date: over 2 years ago
Version: 2.2.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.0.3
GraalVM compatible: Yes
Pull count
Total: 1676
Current verison: 1089
Weekly downloads
Keywords
Communication/Email
Cost/Paid
Vendor/Microsoft
Contributors