microsoft.outlook.calendar
Module microsoft.outlook.calendar
API
Declarations
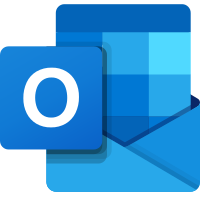
ballerinax/microsoft.outlook.calendar Ballerina library
Overview
Ballerina connector for Microsoft Outlook Calendar is connecting the Calendar API in Microsoft Graph via Ballerina language easily. It provides the capability to perform CRUD (Create, Read, Update, and Delete) operations on Event
& Calendar
stored in Microsoft OneDrive.
This module supports the Microsoft Outlook Calendar API version 1.0.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Microsoft Outlook Account
- Obtain tokens
- Follow this link client ID, client secret, and refresh token.
Quickstart
To use the outlook calendar connector in your Ballerina application, update the .bal file as follows:
Step 1: Import MS Outlook Calendar Package
import the ballerinax/microsoft.outlook.calendar module into the Ballerina project.
import ballerinax/microsoft.outlook.calendar;
Step 2: Configure the connection to an existing Azure AD app
You can now make the connection configuration using the OAuth2 refresh token grant config.
calendar:ConnectionConfig configuration = { auth: { refreshUrl: <REFRESH_URL>, refreshToken : <REFRESH_TOKEN>, clientId : <CLIENT_ID>, clientSecret : <CLIENT_SECRET> } }; calendar:Client calendarClient = check new(configuration);
Step 3: Invoke connector operation
- Now you can use the operations available within the connector. Note that they are in the form of remote operations. Following is an example on how to create a calendar using the connector.
public function main() returns error? { calendar:EventMetadata eventMetadata = { subject: "Test-Subject", body : { content: "Test-Body" }, 'start: { dateTime: "2021-07-16T12:00:00", timeZone: calendar:TIMEZONE_LK }, end: { dateTime: "2021-07-16T14:00:00", timeZone: calendar:TIMEZONE_LK }, location:{ displayName:"Harry's Bar" }, attendees: [{ emailAddress: { address:"samanthab@contoso.onmicrosoft.com", name: "Samantha Booth" }, 'type: calendar:ATTENDEE_TYPE_REQUIRED, status: { response : calendar:RESPONSE_NOT_RESPONDED } }], allowNewTimeProposals: true }; calendar:Event|error generatedEvent = calendarClient->createEvent(eventMetadata); log:printInfo(generatedEvent.toString()); }
- Use
bal run
command to compile and run the Ballerina program.
Clients
microsoft.outlook.calendar: Client
Ballerina Microsoft Outlook Calendar connector provides the capability to access MS Outlook Calendar API. This connector provides the capability to easily create appointments & events, organize meetings, manage user's calendar etc.
Constructor
Initializes the connector. During initialization you can pass either http:BearerTokenConfig if you have a bearer token or http:OAuth2RefreshTokenGrantConfig if you have Oauth tokens. Create a Microsoft account and obtain tokens following this guide.
init (ConnectionConfig config)
- config ConnectionConfig - Configuration for the connector
getEvent
function getEvent(string eventId, TimeZone? timeZone, ContentType? contentType, string? queryParams) returns Event|error
############################################################################# Operations on a Event resource The Event resource is the top-level object representing a event in outlook. ############################################################################# Get event by proving the ID Get the properties and relationships of the specified event object. API doc : https://docs.microsoft.com/en-us/graph/api/event-get
Parameters
- eventId string - ID of an event. Read-only.
- timeZone TimeZone? (default ()) - Preferred Time Zone of the start and end time. (Default :
UTC
)
- contentType ContentType? (default ()) - Preferred Content-Type of the body. Values :
text
ortext
(Default :html
)
- queryParams string? (default ()) - Optional query parameters. This method support OData query parameters to customize the response.
It should be an array of type
string
in the format<QUERY_PARAMETER_NAME>=<PARAMETER_VALUE>
Note: For more information about query parameters, refer here: https://docs.microsoft.com/en-us/graph/query-parameters
listEvents
function listEvents(TimeZone? timeZone, ContentType? contentType, string? queryParams) returns stream<Event, error?>|error
Get list of events Get the properties and relationships of all event objects as a array. API doc : https://docs.microsoft.com/en-us/graph/api/user-list-events
Parameters
- timeZone TimeZone? (default ()) - Preferred Time Zone of the start and end time. (Default :
UTC
)
- contentType ContentType? (default ()) - Preferred Content-Type of the body. Values :
text
ortext
(Default :html
)
- queryParams string? (default ()) - Query parameters. This method support OData query parameters to customize the response.
It should be an array of type
string
in the format<QUERY_PARAMETER_NAME>=<PARAMETER_VALUE>
Note: For more information about query parameters, refer here: https://docs.microsoft.com/en-us/graph/query-parameters
addQuickEvent
Quick add event This create a event with minimum necessary inputs. API doc : https://docs.microsoft.com/en-us/graph/api/user-post-events
Parameters
- subject string - Subject of the event
- description string? (default ()) - Description of the event
- calendarId string? (default ()) - Calendar ID of the calendar that you want to create the event. If not, Default will be used.
createEvent
function createEvent(EventMetadata eventMetadata, string? calendarId) returns Event|error
Create an event This create a new event with Event object as parameters. API doc : https://docs.microsoft.com/en-us/graph/api/user-post-events
Parameters
- eventMetadata EventMetadata - Metadata related to Event that we are passing on input.
- calendarId string? (default ()) - Calendar ID of the calendar that you want to create the event.
updateEvent
function updateEvent(string eventId, EventMetadata eventMetadata, string? calendarId) returns Event|error
Update an event This updates the properties of a Event object. API doc : https://docs.microsoft.com/en-us/graph/api/event-update
Parameters
- eventId string - ID of an event.
- eventMetadata EventMetadata - Metadata related to Event that we are passing on input.
- calendarId string? (default ()) - Calendar ID of the calendar that you want to update the event.
deleteEvent
Delete an event This removes the specified event from the containing calendar. API doc : https://docs.microsoft.com/en-us/graph/api/event-delete
Parameters
- eventId string - ID of an event.
Return Type
- error? -
error
if failed.
createCalendar
function createCalendar(CalendarMetadata calendarMetadata) returns Calendar|error
################################################################################## Operations on a Calendar resource The Calendar resource is the top-level object representing a calendar in outlook. ################################################################################# Create a calendar This create a new calendar for the user. API doc : https://docs.microsoft.com/en-us/graph/api/user-post-calendars
Parameters
- calendarMetadata CalendarMetadata - Metadata related to calendar that we are passing on input.
deleteCalendar
Delete a calendar This removes the specified calendar. API doc : https://docs.microsoft.com/en-us/graph/api/calendar-delete
Parameters
- calendarId string - ID of a calendar.
Return Type
- error? -
error
if failed.
getCalendar
Get a calendar by proving the ID Get the properties and relationships of a calendar object. API doc : https://docs.microsoft.com/en-us/graph/api/calendar-get
Parameters
- calendarId string - ID of an event. Read-only.
- queryParams string? (default ()) - Optional query parameters. This method support OData query parameters to customize the response.
It should be an array of type
string
in the format<QUERY_PARAMETER_NAME>=<PARAMETER_VALUE>
Note: For more information about query parameters, refer here: https://docs.microsoft.com/en-us/graph/query-parameters
updateCalendar
function updateCalendar(string calendarId, string? name, CalendarColor? color, boolean? isDefaultCalendar) returns Calendar|error
Update a calender This updates the properties of a calendar object. API doc : https://docs.microsoft.com/en-us/graph/api/calendar-update
Parameters
- calendarId string - ID of an calendar.
- name string? (default ()) - Name of the calendar.
- color CalendarColor? (default ()) - Color of the calendar.
- isDefaultCalendar boolean? (default ()) - Default calendar.
listCalendars
Get list of calendars. Get all the user's calendars. API doc : https://docs.microsoft.com/en-us/graph/api/user-list-calendars
Parameters
- queryParams string? (default ()) - Optional query parameters. This method support OData query parameters to customize the response.
It should be an array of type
string
in the format<QUERY_PARAMETER_NAME>=<PARAMETER_VALUE>
Note: For more information about query parameters, refer here: https://docs.microsoft.com/en-us/graph/query-parameters
Enums
microsoft.outlook.calendar: AttendeeType
Members
microsoft.outlook.calendar: CalendarColor
Members
microsoft.outlook.calendar: ContentType
Members
microsoft.outlook.calendar: EventType
Members
microsoft.outlook.calendar: Importance
Members
microsoft.outlook.calendar: LocationType
Members
microsoft.outlook.calendar: OnlineMeetingProviderType
Members
microsoft.outlook.calendar: PhoneType
Members
microsoft.outlook.calendar: RecurrencePattern
Describes the frequency by which a recurring event repeats.
You can specify the recurrence pattern of a recurring event in one of 6 ways depending on your scenario. For each pattern type, specify the amount of time between occurrences. The actual occurrences of the recurring event always follow this pattern falling within the date range that you specify for the event. A recurring event is always defined by its recurrencePattern(how frequently the event repeats), and its recurrenceRange (over how long the event repeats).
API Doc : https://docs.microsoft.com/en-us/graph/api/resources/recurrencepattern?view=graph-rest-1.0
Members
microsoft.outlook.calendar: RecurrenceRangeType
Members
microsoft.outlook.calendar: ResponseType
Members
microsoft.outlook.calendar: Sensitivity
Members
microsoft.outlook.calendar: ShowAs
Members
microsoft.outlook.calendar: TimeZone
Members
Records
microsoft.outlook.calendar: Attendee
Fields
- emailAddress EmailAddress? -
- proposedNewTime TimeSlot? -
- status ResponseStatus? -
- 'type string? -
microsoft.outlook.calendar: Calendar
Get the properties and relationships of the specified calendar object. Calendar Resource
Fields
- Fields Included from *GeneratedCalendarData
- Fields Included from *CalendarMetadata
- name string
- isDefaultCalendar boolean|()
- allowedOnlineMeetingProviders OnlineMeetingProviderType[]
- canEdit boolean
- canShare boolean
- isRemovable boolean
- isTallyingResponses boolean
- canViewPrivateItems boolean
- color "maxColor"|"lightRed"|"lightBrown"|"lightPink"|"lightTeal"|"lightYellow"|"lightGray"|"lightOrange"|"lightGreen"|"lightBlue"|"auto"|()
- defaultOnlineMeetingProvider OnlineMeetingProviderType
- owner EmailAddress
- anydata...
microsoft.outlook.calendar: CalendarMetadata
Fields
- name string? -
- isDefaultCalendar boolean? -
- allowedOnlineMeetingProviders OnlineMeetingProviderType[]? -
- canEdit boolean? -
- canShare boolean? -
- isRemovable boolean? -
- isTallyingResponses boolean? -
- canViewPrivateItems boolean? -
- color CalendarColor? -
- defaultOnlineMeetingProvider OnlineMeetingProviderType? -
- owner EmailAddress? -
microsoft.outlook.calendar: ConnectionConfig
Client configuration details.
Fields
- Fields Included from *ConnectionConfig
- auth AuthConfig
- httpVersion HttpVersion
- http1Settings ClientHttp1Settings
- http2Settings ClientHttp2Settings
- timeout decimal
- forwarded string
- poolConfig PoolConfiguration
- cache CacheConfig
- compression Compression
- circuitBreaker CircuitBreakerConfig
- retryConfig RetryConfig
- responseLimits ResponseLimitConfigs
- secureSocket ClientSecureSocket
- proxy ProxyConfig
- validation boolean
- anydata...
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig - Configurations related to client authentication
microsoft.outlook.calendar: DateTimeTimeZone
Fields
- dateTime string? -
- timeZone string? -
microsoft.outlook.calendar: EmailAddress
Fields
- address string? -
- name string? -
microsoft.outlook.calendar: Event
Get the properties and relationships of the specified event object. Event Resource
Fields
- Fields Included from *GeneratedEventData
- Fields Included from *EventMetadata
- subject string|()
- body ItemBody
- bodyPreview string
- categories string[]
- originalStartTimeZone string
- originalEndTimeZone string
- start DateTimeTimeZone|()
- end DateTimeTimeZone|()
- location Location
- locations Location[]
- attendees Attendee[]
- recurrence PatternedRecurrence|()
- allowNewTimeProposals boolean
- hasAttachments boolean
- hideAttendees boolean
- isAllDay boolean
- isOnlineMeeting boolean
- isReminderOn boolean
- importance Importance
- reminderMinutesBeforeStart int
- responseRequested boolean
- responseStatus ResponseStatus
- sensitivity Sensitivity
- showAs ShowAs
- onlineMeetingProvider OnlineMeetingProviderType
- anydata...
microsoft.outlook.calendar: EventMetadata
Metadata related to Event
resource
Fields
- subject string? - The text of the event's subject line
- body ItemBody? - The body of the message associated with the event. It can be in HTML or text format
- bodyPreview string? - The preview of the message associated with the event. It is in text format
- categories string[]? - The categories associated with the event
- originalStartTimeZone string? - The start time zone that was set when the event was created A value of tzone://Microsoft/Custom indicates that a legacy custom time zone was set in desktop Outlook
- originalEndTimeZone string? - The end time zone that was set when the event was created A value of tzone://Microsoft/Custom indicates that a legacy custom time zone was set in desktop Outlook
- 'start DateTimeTimeZone? - The date, time, and time zone that the event starts. By default, the start time is in UTC
- end DateTimeTimeZone? - The date, time, and time zone that the event ends. By default, the end time is in UTC
- location Location? - The location of the event
- locations Location[]? - The locations where the event is held or attended from The location and locations properties always correspond with each other If you update the location property, any prior locations in the locations collection would be removed and replaced by the new location value
- attendees Attendee[]? - The collection of attendees for the event
- recurrence PatternedRecurrence? - The recurrence pattern for the event
- allowNewTimeProposals boolean? - True if the meeting organizer allows invitees to propose a new time when responding, false otherwise. Optional. Default is true
- hasAttachments boolean? - Set to true if the event has attachments
- hideAttendees boolean? - When set to true, each attendee only sees themselves in the meeting request & meeting Tracking list. Default is false
- isAllDay boolean? - Set to true if the event lasts all day
- isOnlineMeeting boolean? - True if this event has online meeting information, false otherwise. Default is false. Optional
- isReminderOn boolean? - Set to true if an alert is set to remind the user of the event
- importance Importance? - The importance of the event. The possible values are: low, normal, high
- reminderMinutesBeforeStart int? - The number of minutes before the event start time that the reminder alert occurs
- responseRequested boolean? - Default is true, which represents the organizer would like an invitee to send a response to the event
- responseStatus ResponseStatus? - Indicates the type of response sent in response to an event message
- sensitivity Sensitivity? - The possible values are: normal, personal, private, confidential
- showAs ShowAs? - The status to show. The possible values are: free, tentative, busy, oof, workingElsewhere, unknown
- onlineMeetingProvider OnlineMeetingProviderType? - Represents the online meeting service provider The possible values are teamsForBusiness, skypeForBusiness, and skypeForConsumer. Optional
microsoft.outlook.calendar: GeneratedCalendarData
Fields
- idreadonly string -
- hexColorreadonly string? -
- changeKeyreadonly string? -
microsoft.outlook.calendar: GeneratedEventData
Generated Event
Data.
Fields
- idreadonly string - Unique ID for event. Read-only
- changeKey string? - Identifies the version of the event object. Every time the event is changed, ChangeKey changes as well This allows Exchange to apply changes to the correct version of the object
- createdDateTime string? - The Timestamp type represents date and time information using ISO 8601 format and is always in UTC time. For example, midnight UTC on Jan 1, 2014 is 2014-01-01T00:00:00Z
- occurrenceId string? - Unique ID for occurrence
- transactionId string? - A custom identifier specified by a client app for the server to avoid redundant POST operations in case of client retries to create the same event. This is useful when low network connectivity causes the client to time out before receiving a response from the server for the client's prior create-event request. After you set transactionId when creating an event, you cannot change transactionId in a subsequent update This property is only returned in a response payload if an app has set it. Optional.
- seriesMasterId string? - The ID for the recurring series master item, if this event is part of a recurring series
- onlineMeetingUrlreadonly string? - A URL for an online meeting The property is set only when an organizer specifies an event as an online meeting such as a Skype meeting. Read-only
- iCalUId string? - A unique identifier for an event across calendars. This ID is different for each occurrence in a recurring series. Read-only
- onlineMeeting OnlineMeetingInfo? - Details for an attendee to join the meeting online. Read-only.
- organizer Recipient? - The organizer of the event
- originalStart string? - The Timestamp type represents date and time information using ISO 8601 format and is always in UTC time. For example, midnight UTC on Jan 1, 2014 is 2014-01-01T00:00:00Z
- 'typereadonly EventType? - The event type. The possible values are: singleInstance, occurrence, exception, seriesMaster. Read-only
- webLinkreadonly string? - The URL to open the event in Outlook on the web.Outlook on the web opens the event in the browser if you are signed in to your mailbox. Otherwise, Outlook on the web prompts you to sign in. This URL cannot be accessed from within an iFrame
microsoft.outlook.calendar: ItemBody
Fields
- contentType string? -
- content string? -
microsoft.outlook.calendar: Location
Fields
- address PhysicalAddress? -
- coordinates OutlookGeoCoordinates? -
- displayName string? -
- locationEmailAddress string? -
- locationUri string? -
- locationType LocationType? -
microsoft.outlook.calendar: OnlineMeetingInfo
Details for an attendee to join the meeting online. Read-only.
Fields
- conferenceId string? - The ID of the conference
- joinUrl string? - The external link that launches the online meeting. This is a URL that clients will launch into a browser and will redirect the user to join the meeting
- phones Phone? - All of the phone numbers associated with this conference
- quickDial string? - All of the phone numbers associated with this conference
- tollFreeNumbers string[]? - The toll free numbers that can be used to join the conference
- tollNumber string? - The toll number that can be used to join the conference
microsoft.outlook.calendar: OutlookGeoCoordinates
Fields
- accuracy float? -
- altitude float? -
- altitudeAccuracy float? -
- latitude float? -
- longitude float? -
microsoft.outlook.calendar: PatternedRecurrence
The recurrence pattern and range.
Fields
- pattern RecurrencePattern? - The frequency of an event. Do not specify for a one-time access review
- range RecurrenceRange? - The duration of an event
microsoft.outlook.calendar: Phone
Fields
- number string? -
- 'type PhoneType? -
microsoft.outlook.calendar: PhysicalAddress
Fields
- city string? -
- countryOrRegion string? -
- postalCode string? -
- state string? -
- street string? -
microsoft.outlook.calendar: Recipient
Represents information about a user in the sending or receiving end of an event, message or group post.
Fields
- emailAddress EmailAddress? - The recipient's email address
microsoft.outlook.calendar: RecurrenceRange
Describes a date range over which a recurring event repeats.
You can specify the date range for a recurring event in one of 3 ways depending on your scenario While you must always specify a startDate value for the date range, you can specify a recurring event that ends by a specific date, or that doesn't end, or that ends after a specific number of occurrences.
Note that the actual occurrences within the date range always follow the recurrence pattern that you specify for the recurring event. A recurring event is always defined by its recurrencePattern (how frequently the event repeats), and its recurrenceRange (for how long the event repeats).
Fields
- endDate string? - The date to stop applying the recurrence pattern Depending on the recurrence pattern of the event, the last occurrence of the meeting may not be this date. Required if type is endDate
- numberOfOccurrences int? - The number of times to repeat the event. Required and must be positive if type is numbered.
- recurrenceTimeZone string? - Time zone for the startDate and endDate properties Optional. If not specified, the time zone of the event is used
- startDate string? - The date to start applying the recurrence pattern. The first occurrence of the meeting may be this date or later, depending on the recurrence pattern of the event. Must be the same value as the start property of the recurring event. Required
- 'type RecurrenceRangeType? - The recurrence range. The possible values are: endDate, noEnd, numbered. Required
microsoft.outlook.calendar: ResponseStatus
Fields
- response ResponseType? -
- time string? -
microsoft.outlook.calendar: TimeSlot
Fields
- 'start DateTimeTimeZone? -
- end DateTimeTimeZone? -
Import
import ballerinax/microsoft.outlook.calendar;
Metadata
Released date: over 1 year ago
Version: 2.4.0
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 3
Current verison: 1
Weekly downloads
Keywords
Productivity/Calendars
Cost/Free
Vendor/Microsoft
Contributors