microsoft.onenote
Module microsoft.onenote
API
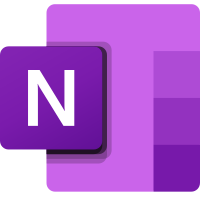
ballerinax/microsoft.onenote Ballerina library
Overview
Ballerina connector for Microsoft OneNote connects the Microsoft OneNote API through Ballerina. It provides the capability to perform CRUD operations on OneNote notebooks, sections and pages.
This module supports Microsoft Graph API v1.0 version and only allows to perform functions behalf of the currently logged in user.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Microsoft Office365 account
- Obtain tokens - Follow this link
Quickstart
To use the MS OneNote connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/microsoft.onenote
module as shown below.
import ballerinax/microsoft.onenote;
Step 2: Create a new connector instance
Create a microsoft.onenote:ConnectionConfig
with the OAuth2 tokens obtained and initialize the connector with it.
microsoft.onenote:ConnectionConfig configuration = { auth: { refreshUrl: <REFRESH_URL>, refreshToken : <REFRESH_TOKEN>, clientId : <CLIENT_ID>, clientSecret : <CLIENT_SECRET> } }; microsoft.onenote:Client oneNoteClient = check new(configuration);
Step 3: Invoke connector operation
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to create a notebook using the connector.
public function main() returns error? { Notebook notebook = check oneNoteClient->createNotebook("test"); }
Clients
microsoft.onenote: Client
Ballerina Microsoft OneNote connector provides the capability to access Microsoft Graph OneNote API It provides capability to perform perform CRUD (Create, Read, Update, and Delete) operations on [OneNote] (https://docs.microsoft.com/en-us/graph/api/resources/onenote-api-overview?view=graph-rest-1.0).
Constructor
Initializes the connector. During initialization you can pass either http:BearerTokenConfig if you have a bearer token or http:OAuth2RefreshTokenGrantConfig if you have OAuth tokens. Create a Microsoft account and obtain tokens following this guide
init (ConnectionConfig config)
- config ConnectionConfig - Configuration required to initialize the client
createNotebook
Creates a notebook.
Parameters
- noteBookName string - Display name for notebook
listNotebooks
Lists notebooks.
Parameters
- oDataQuery string? (default ()) - Optional. OData Query. Example: "$top=2&$count=true". For details, refer https://docs.microsoft.com/en-us/graph/query-parameters#odata-system-query-options
getNotebook
Gets a notebook.
Parameters
- notebookId string - Notebook ID
getRecentNotebooks
function getRecentNotebooks() returns RecentNotebook[]|error
Lists recent notebooks.
Return Type
- RecentNotebook[]|error - RecentNotebook[] if successful or else an error
listSections
Lists sections.
Parameters
- notebookId string - Notebook ID
- oDataQuery string? (default ()) - Optional. OData Query. Example: "$top=2&$count=true". For details, refer https://docs.microsoft.com/en-us/graph/query-parameters#odata-system-query-options
getSection
Gets a section.
Parameters
- sectionId string - Section ID
createSection
Creates a section.
listSectionGroups
function listSectionGroups(string notebookId, string? oDataQuery) returns SectionGroup[]|error
Lists section groups.
Parameters
- notebookId string - Notebook ID
- oDataQuery string? (default ()) - Optional. OData Query. Example: "$top=2&$count=true". For details, refer https://docs.microsoft.com/en-us/graph/query-parameters#odata-system-query-options
Return Type
- SectionGroup[]|error - SectionGroup[] if successful or else an error
createSectionGroup
function createSectionGroup(string notebookId, string sectionGroupName) returns SectionGroup|error
Creates a section group.
Return Type
- SectionGroup|error - Section if successful or else an error
getSectionGroup
function getSectionGroup(string sectionGroupId) returns SectionGroup|error
Gets a section group.
Parameters
- sectionGroupId string - Section group ID
Return Type
- SectionGroup|error - Section if successful or else an error
createSectionInSectionGroup
function createSectionInSectionGroup(string sectionGroupId, string sectionName) returns Section|error
Creates a section in a section group.
listPages
Lists pages of a section.
Parameters
- sectionId string - Section ID
- oDataQuery string? (default ()) - Optional. OData Query. Example: "$top=2&$count=true". For details, refer https://docs.microsoft.com/en-us/graph/query-parameters#odata-system-query-options
getPage
Gets a page.
Parameters
- pageId string - Page ID
createPage
Creates a page.
createPageWithHTML
Creates a page with HTML content.
deletePage
Deletes a page.
Parameters
- pageId string - Page ID
Return Type
- error? -
()
if the page deletion is successful or else an error if failed
Records
microsoft.onenote: ConnectionConfig
Client configuration details.
Fields
- Fields Included from *ConnectionConfig
- auth AuthConfig
- httpVersion HttpVersion
- http1Settings ClientHttp1Settings
- http2Settings ClientHttp2Settings
- timeout decimal
- forwarded string
- poolConfig PoolConfiguration
- cache CacheConfig
- compression Compression
- circuitBreaker CircuitBreakerConfig
- retryConfig RetryConfig
- responseLimits ResponseLimitConfigs
- secureSocket ClientSecureSocket
- proxy ProxyConfig
- validation boolean
- anydata...
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig - Configurations related to client authentication
microsoft.onenote: Notebook
Represents a Notebook.
Fields
- id string - The unique identifier of the notebook
- displayName string - The name of the notebook.
- createdBy json - Identity of the user, device, and application which created the item
- createdDateTime string - The date and time when the notebook was created
- isDefault boolean - Indicates whether this is the user's default notebook
- isShared boolean - Indicates whether the notebook is shared. If true, the contents of the notebook can be seen by people other than the owner.
- lastModifiedBy json - Identity of the user, device, and application which created the item
- lastModifiedDateTime string - The date and time when the notebook was last modified
- links json - Links for opening the notebook. The oneNoteClientURL link opens the notebook in the OneNote native client if it's installed. The oneNoteWebURL link opens the notebook in OneNote on the web.
- sectionGroupsUrl string - The URL for the sectionGroups navigation property, which returns all the section groups in the notebook
- sectionsUrl string - The URL for the sections navigation property, which returns all the sections in the notebook
- self string - The endpoint where you can get details about the notebook
- userRole string - Possible values are: Owner, Contributor, Reader, None. Owner represents owner-level access to the notebook. Contributor represents read/write access to the notebook. Reader represents read-only access to the notebook.
microsoft.onenote: Page
Represents a Page.
Fields
- id string - The unique identifier of the page
- title string - The title of the page
- createdDateTime string - The date and time when the page was created
- lastModifiedDateTime string - The date and time when the page was last modified
- self string - The endpoint where you can get details about the page
- content byte[]? - The page's HTML content
- contentUrl string - The URL for the page's HTML content
- createdByAppId string? - The unique identifier of the application that created the page. Read-only
microsoft.onenote: RecentNotebook
Represents a RecentNotebook.
Fields
- displayName string - The name of the notebook
- lastAccessedTime string - The date and time when the notebook was last accessed
- links json - Links for opening the notebook
- sourceService string - Source service
microsoft.onenote: Section
Represents a Section.
Fields
- id string - The unique identifier of the section
- displayName string - The name of the section
- createdBy json - Identity of the user, device, and application which created the item
- createdDateTime string - The date and time when the section was created
- isDefault boolean - Indicates whether this is the user's default section
- lastModifiedBy json - Identity of the user, device, and application which created the item
- lastModifiedDateTime string - The date and time when the section was last modified
- pagesUrl string - The pages endpoint where you can get details for all the pages in the section
- self string - The endpoint where you can get details about the section
microsoft.onenote: SectionGroup
Represents a SectionGroup.
Fields
- id string - The unique identifier of the section group
- displayName string - The name of the section group
- createdBy json - Identity of the user, device, and application which created the item
- createdDateTime string - The date and time when the section group was created
- lastModifiedBy json - Identity of the user, device, and application which created the item
- lastModifiedDateTime string - The date and time when the section group was last modified
- sectionGroupsUrl string - The URL for the sectionGroups navigation property, which returns all the section groups in the section group
- sectionsUrl string - The URL for the sections navigation property, which returns all the sections in the section group
- self string - The endpoint where you can get details about the section group
Import
import ballerinax/microsoft.onenote;
Metadata
Released date: over 1 year ago
Version: 2.4.0
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 1
Current verison: 1
Weekly downloads
Keywords
Content & Files/Notes
Cost/Freemium
Vendor/Microsoft
Contributors