microsoft.excel
Module microsoft.excel
API
Declarations
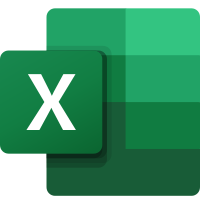
ballerinax/microsoft.excel Ballerina library
Overview
Ballerina connector for Microsoft Excel connects the Microsoft Graph Excel API via Ballerina. It provides the capability to perform CRUD (Create, Read, Update, and Delete) operations on Excel workbooks stored in a Microsoft OneDrive.
This module supports Microsoft Graph API v1.0 version and only allows to perform functions behalf of the currently logged in user.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Microsoft Office 365 account
- Obtain tokens
Follow the steps here
Quickstart
To use the Microsoft Excel connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
Import the ballerinax/microsoft.excel
module into the Ballerina project.
import ballerinax/microsoft.excel;
Step 2: Create a new connector instance
Create an excel:ConnectionConfig
with the OAuth2 tokens obtained and initialize the connector with it.
excel:ConnectionConfig configuration = { auth: { refreshUrl: <REFRESH_URL>, refreshToken : <REFRESH_TOKEN>, clientId : <CLIENT_ID>, clientSecret : <CLIENT_SECRET> } }; excel:Client excelClient = check new (configuration);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to add a worksheet using the connector.public function main() returns error? { excel:Worksheet response = check excelClient->addWorksheet("workbookIdOrPath", "sheetName"); }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
microsoft.excel: Client
Ballerina Microsoft Excel connector provides the capability to access Microsoft Graph Excel API It provides capability to perform perform CRUD (Create, Read, Update, and Delete) operations on Excel workbooks stored in Microsoft OneDrive. If you have more than one call to make within a certain period of time, Microsoft recommends to create a session and pass the session ID with each request. By default, this connector uses sessionless.
Constructor
Initializes the connector. During initialization you can pass either http:BearerTokenConfig if you have a bearer token or http:OAuth2RefreshTokenGrantConfig if you have OAuth tokens. Create a Microsoft account and obtain tokens following this guide
init (ConnectionConfig config)
- config ConnectionConfig -
createSession
Creates a session. Excel APIs supports two types of sessions Persistent session - All changes made to the workbook are persisted (saved). This is the most efficient and performant mode of operation. Non-persistent session - Changes made by the API are not saved to the source location. Instead, the Excel backend server keeps a temporary copy of the file that reflects the changes made during that particular API session. When the Excel session expires, the changes are lost. This mode is useful for apps that need to do analysis or obtain the results of a calculation or a chart image, but not affect the document state.
Parameters
- workbookIdOrPath string - Workbook ID or file path. Path should be with the
.xlsx
extension from root. If a workbook is in root, path will be<FILE_NAME>.xlsx
)
- persistChanges boolean (default true) - All changes made to the workbook are persisted or not?
addWorksheet
function addWorksheet(string workbookIdOrPath, string? worksheetName, string? sessionId) returns Worksheet|error
Adds a new worksheet to the workbook.
Parameters
- workbookIdOrPath string - Workbook ID or file path. Path should be with the
.xlsx
extension from root. If a workbook is in root, path will be<FILE_NAME>.xlsx
)
- worksheetName string? (default ()) - The name of the worksheet to be added. If specified, name should be unqiue. If not specified, Excel determines the name of the new worksheet
- sessionId string? (default ()) - Session ID
getWorksheet
function getWorksheet(string workbookIdOrPath, string worksheetNameOrId, string? sessionId) returns Worksheet|error
Retrieves the properties of a worksheet.
Parameters
- workbookIdOrPath string - Workbook ID or file path. Path should be with the
.xlsx
extension from root. If a workbook is in root, path will be<FILE_NAME>.xlsx
)
- worksheetNameOrId string - Worksheet name or ID
- sessionId string? (default ()) - Session ID
listWorksheets
function listWorksheets(string workbookIdOrPath, string? query, string? sessionId) returns Worksheet[]|error
Retrieves a list of worksheets.
Parameters
- workbookIdOrPath string - Workbook ID or file path. Path should be with the
.xlsx
extension from root. If a workbook is in root, path will be<FILE_NAME>.xlsx
)
- query string? (default ()) - Query string that can control the amount of data returned in a response. String should start with
?
and followed by query parameters. Example:?$top=2&$count=true
. For more information about query parameters, refer https://docs.microsoft.com/en-us/graph/query-parameters
- sessionId string? (default ()) - Session ID
updateWorksheet
function updateWorksheet(string workbookIdOrPath, string worksheetNameOrId, Worksheet worksheet, string? sessionId) returns Worksheet|error
Update the properties of worksheet.
Parameters
- workbookIdOrPath string - Workbook ID or file path. Path should be with the
.xlsx
extension from root. If a workbook is in root, path will be<FILE_NAME>.xlsx
)
- worksheetNameOrId string - Worksheet name or ID
- worksheet Worksheet - 'Worksheet' record contains values for relevant fields that should be updated
- sessionId string? (default ()) - Session ID
getCell
function getCell(string workbookIdOrPath, string worksheetNameOrId, int row, int column, string? sessionId) returns Cell|error
Gets the range object containing the single cell based on row and column numbers.
Parameters
- workbookIdOrPath string - Workbook ID or file path. Path should be with the
.xlsx
extension from root. If a workbook is in root, path will be<FILE_NAME>.xlsx
)
- worksheetNameOrId string - Worksheet name or ID
- row int - number of the cell to be retrieved. Zero-indexed
- column int - Column number of the cell to be retrieved. Zero-indexed
- sessionId string? (default ()) - Session ID
deleteWorksheet
function deleteWorksheet(string workbookIdOrPath, string worksheetNameOrId, string? sessionId) returns error?
Deletes the worksheet from the workbook.
Parameters
- workbookIdOrPath string - Workbook ID or file path. Path should be with the
.xlsx
extension from root. If a workbook is in root, path will be<FILE_NAME>.xlsx
)
- worksheetNameOrId string - Worksheet name or ID
- sessionId string? (default ()) - Session ID
Return Type
- error? -
()
or else anerror
if failed
addTable
function addTable(string workbookIdOrPath, string worksheetNameOrId, string address, boolean? hasHeaders, string? sessionId) returns Table|error
Creates a new table.
Parameters
- workbookIdOrPath string - Workbook ID or file path. Path should be with the
.xlsx
extension from root. If a workbook is in root, path will be<FILE_NAME>.xlsx
)
- worksheetNameOrId string - Worksheet name or ID
- address string - Range address or name of the range object representing the data source
- hasHeaders boolean? (default ()) - Boolean value that indicates whether the data being imported has column labels. If the source does not contain headers (i.e,. when this property set to false), Excel will automatically generate header shifting the data down by one row
- sessionId string? (default ()) - Session ID
getTable
function getTable(string workbookIdOrPath, string worksheetNameOrId, string tableNameOrId, string? query, string? sessionId) returns Table|error
Retrieves the properties of table.
Parameters
- workbookIdOrPath string - Workbook ID or file path. Path should be with the
.xlsx
extension from root. If a worksbook is in root, path will be<FILE_NAME>.xlsx
)
- worksheetNameOrId string - Worksheet name or ID
- tableNameOrId string - Table name or ID
- query string? (default ()) - Query string that can control the amount of data returned in a response. String should start with
?
and followed by query parameters. Example:?$top=2&$count=true
. For more information about query parameters, refer https://docs.microsoft.com/en-us/graph/query-parameters
- sessionId string? (default ()) - Session ID
listTables
function listTables(string workbookIdOrPath, string? worksheetNameOrId, string? query, string? sessionId) returns Table[]|error
Retrieves a list of tables.
Parameters
- workbookIdOrPath string - Workbook ID or file path. Path should be with the
.xlsx
extension from root. If a worksbook is in root, path will be<FILE_NAME>.xlsx
)
- worksheetNameOrId string? (default ()) - Worksheet name or ID
- query string? (default ()) - Query string that can control the amount of data returned in a response. String should start with
?
and followed by query parameters. Example:?$top=2&$count=true
. For more information about query parameters, refer https://docs.microsoft.com/en-us/graph/query-parameters
- sessionId string? (default ()) - Session ID
updateTable
function updateTable(string workbookIdOrPath, string worksheetNameOrId, string tableNameOrId, Table 'table, string? sessionId) returns Table|error
Updates the properties of a table.
Parameters
- workbookIdOrPath string - Workbook ID or file path. Path should be with the
.xlsx
extension from root. If a worksbook is in root, path will be<FILE_NAME>.xlsx
)
- worksheetNameOrId string - Worksheet name or ID
- tableNameOrId string - Table name or ID
- 'table Table -
Table
record contains the values for relevant fields that should be updated
- sessionId string? (default ()) - Session ID
createRow
function createRow(string workbookIdOrPath, string worksheetNameOrId, string tableNameOrId, json values, int? index, string? sessionId) returns Row|error
Adds rows to the end of the table. Note that the API can accept multiple rows data using this operation. Adding one row at a time could lead to performance degradation. The recommended approach would be to batch the rows together in a single call rather than doing single row insertion. For best results, collect the rows to be inserted on the application side and perform single rows add operation.
Parameters
- workbookIdOrPath string - Workbook ID or file path. Path should be with the
.xlsx
extension from root. If a worksbook is in root, path will be<FILE_NAME>.xlsx
)
- worksheetNameOrId string - Worksheet name or ID
- tableNameOrId string - Table name or ID
- values json - A 2-dimensional array of unformatted values of the table rows (boolean or string or number).
- index int? (default ()) - Specifies the relative position of the new row. If null, the addition happens at the end. Any rows below the inserted row are shifted downwards. Zero-indexed
- sessionId string? (default ()) - Session ID
listRows
function listRows(string workbookIdOrPath, string worksheetNameOrId, string tableNameOrId, string? query, string? sessionId) returns Row[]|error
Retrieves a list of table rows.
Parameters
- workbookIdOrPath string - Workbook ID or file path. Path should be with the
.xlsx
extension from root. If a worksbook is in root, path will be<FILE_NAME>.xlsx
)
- worksheetNameOrId string - Worksheet name or ID
- tableNameOrId string - Table name or ID
- query string? (default ()) - Query string that can control the amount of data returned in a response. String should start with
?
and followed by query parameters. Example:?$top=2&$count=true
. For more information about query parameters, refer https://docs.microsoft.com/en-us/graph/query-parameters
- sessionId string? (default ()) - Session ID
updateRow
function updateRow(string workbookIdOrPath, string worksheetNameOrId, string tableNameOrId, int index, json[] values, string? sessionId) returns Row|error
Updates the properties of a row.
Parameters
- workbookIdOrPath string - Workbook ID or file path. Path should be with the
.xlsx
extension from root. If a workbook is in root, path will be<FILE_NAME>.xlsx
)
- worksheetNameOrId string - Worksheet name or ID
- tableNameOrId string - Table name or ID
- index int - The index number of the row within the rows collection of the table
- values json[] - A 2-dimensional array of unformatted values of the table rows (boolean or string or number). Provide values for relevant fields that should be updated. Existing properties that are not included in the request will maintain their previous values or be recalculated based on changes to other property values. For best performance you shouldn't include existing values that haven't changed
- sessionId string? (default ()) - Session ID
deleteRow
function deleteRow(string workbookIdOrPath, string worksheetNameOrId, string tableNameOrId, int index, string? sessionId) returns error?
Deletes the row from the table.
Parameters
- workbookIdOrPath string - Workbook ID or file path. Path should be with the
.xlsx
extension from root. If a workbook is in root, path will be<FILE_NAME>.xlsx
)
- worksheetNameOrId string - Worksheet name or ID
- tableNameOrId string - Table name or ID
- index int - Row index
- sessionId string? (default ()) - Session ID
Return Type
- error? -
()
or else anerror
if failed
createColumn
function createColumn(string workbookIdOrPath, string worksheetNameOrId, string tableNameOrId, json values, int? index, string? sessionId) returns Column|error
Creates a new table column.
Parameters
- workbookIdOrPath string - Workbook ID or file path. Path should be with the
.xlsx
extension from root. If a worksbook is in root, path will be<FILE_NAME>.xlsx
)
- worksheetNameOrId string - Worksheet name or ID
- tableNameOrId string - Table name or ID
- values json - A 2-dimensional array of unformatted values of the table columns (boolean or string or number).
- index int? (default ()) - The index number of the column within the columns collection of the table
- sessionId string? (default ()) - Session ID
listColumns
function listColumns(string workbookIdOrPath, string worksheetNameOrId, string tableNameOrId, string? query, string? sessionId) returns Column[]|error
Retrieves a list of table columns.
Parameters
- workbookIdOrPath string - Workbook ID or file path. Path should be with the
.xlsx
extension from root. If a worksbook is in root, path will be<FILE_NAME>.xlsx
)
- worksheetNameOrId string - Worksheet name or ID
- tableNameOrId string - Table name or ID
- query string? (default ()) - Query string that can control the amount of data returned in a response. String should start with
?
and followed by query parameters. Example:?$top=2&$count=true
. For more information about query parameters, refer https://docs.microsoft.com/en-us/graph/query-parameters
- sessionId string? (default ()) - Session ID
updateColumn
function updateColumn(string workbookIdOrPath, string worksheetNameOrId, string tableNameOrId, int index, json[]? values, string? name, string? sessionId) returns Column|error
Updates the properties of a column.
Parameters
- workbookIdOrPath string - Workbook ID or file path. Path should be with the
.xlsx
extension from root. If a workbook is in root, path will be<FILE_NAME>.xlsx
)
- worksheetNameOrId string - Worksheet name or ID
- tableNameOrId string - Table name or ID
- index int - The index number of the column within the columns collection of the table
- values json[]? (default ()) - A 2-dimensional array of unformatted values of the table rows (boolean or string or number). Provide values for relevant fields that should be updated. Existing properties that are not included in the request will maintain their previous values or be recalculated based on changes to other property values. For best performance you shouldn't include existing values that haven't changed
- name string? (default ()) - The name of the table column
- sessionId string? (default ()) - Session ID
deleteColumn
function deleteColumn(string workbookIdOrPath, string worksheetNameOrId, string tableNameOrId, int index, string? sessionId) returns error?
Deletes a column from the table.
Parameters
- workbookIdOrPath string - Workbook ID or file path. Path should be with the
.xlsx
extension from root. If a worksbook is in root, path will be<FILE_NAME>.xlsx
)
- worksheetNameOrId string - Worksheet name or ID
- tableNameOrId string - Table name or ID
- index int - The index number of the column within the columns collection of the table
- sessionId string? (default ()) - Session ID
Return Type
- error? -
()
or else anerror
if failed
deleteTable
function deleteTable(string workbookIdOrPath, string worksheetNameOrId, string tableNameOrId, string? sessionId) returns error?
Deletes a table.
Parameters
- workbookIdOrPath string - Workbook ID or file path. Path should be with the
.xlsx
extension from root. If a worksbook is in root, path will be<FILE_NAME>.xlsx
)
- worksheetNameOrId string - Worksheet name or ID
- tableNameOrId string - Table name or ID
- sessionId string? (default ()) - Session ID
Return Type
- error? -
()
or else anerror
if failed
addChart
function addChart(string workbookIdOrPath, string worksheetNameOrId, string 'type, json sourceData, SeriesBy? seriesBy, string? sessionId) returns Chart|error
Creates a new chart.
Parameters
- workbookIdOrPath string - Workbook ID or file path. Path should be with the
.xlsx
extension from root. If a worksbook is in root, path will be<FILE_NAME>.xlsx
)
- worksheetNameOrId string - Worksheet name or ID
- 'type string -
- sourceData json - The Range object corresponding to the source data
- seriesBy SeriesBy? (default ()) - Specifies the way columns or rows are used as data series on the chart
- sessionId string? (default ()) - Session ID
getChart
function getChart(string workbookIdOrPath, string worksheetNameOrId, string chartName, string? query, string? sessionId) returns Chart|error
Retrieves the properties of a chart.
Parameters
- workbookIdOrPath string - Workbook ID or file path. Path should be with the
.xlsx
extension from root. If a worksbook is in root, path will be<FILE_NAME>.xlsx
)
- worksheetNameOrId string - Worksheet name or ID
- chartName string - Chart name
- query string? (default ()) - Query string that can control the amount of data returned in a response. String should start with
?
and followed by query parameters. Example:?$top=2&$count=true
. For more information about query parameters, refer https://docs.microsoft.com/en-us/graph/query-parameters
- sessionId string? (default ()) - Session ID
listCharts
function listCharts(string workbookIdOrPath, string worksheetNameOrId, string? query, string? sessionId) returns Chart[]|error
Retrieve a list of charts.
Parameters
- workbookIdOrPath string - Workbook ID or file path. Path should be with the
.xlsx
extension from root. If a worksbook is in root, path will be<FILE_NAME>.xlsx
)
- worksheetNameOrId string - Worksheet name or ID
- query string? (default ()) - Query string that can control the amount of data returned in a response. String should start with
?
and followed by query parameters. Example:?$top=2&$count=true
. For more information about query parameters, refer https://docs.microsoft.com/en-us/graph/query-parameters
- sessionId string? (default ()) - Session ID
updateChart
function updateChart(string workbookIdOrPath, string worksheetNameOrId, string chartName, Chart chart, string? sessionId) returns Chart|error
Updates the properties of chart.
Parameters
- workbookIdOrPath string - Workbook ID or file path. Path should be with the
.xlsx
extension from root. If a worksbook is in root, path will be<FILE_NAME>.xlsx
)
- worksheetNameOrId string - Worksheet name or ID
- chartName string - Chart name
- chart Chart - 'Chart' record contains values for relevant fields that should be updated
- sessionId string? (default ()) - Session ID
getChartImage
function getChartImage(string workbookIdOrPath, string worksheetNameOrId, string chartName, int? width, int? height, FittingMode? fittingMode, string? sessionId) returns string|error
Renders the chart as a base64-encoded image by scaling the chart to fit the specified dimensions.
Parameters
- workbookIdOrPath string - Workbook ID or file path. Path should be with the
.xlsx
extension from root. If a worksbook is in root, path will be<FILE_NAME>.xlsx
)
- worksheetNameOrId string - Worksheet name or ID
- chartName string - Chart name
- width int? (default ()) - The desired width of the resulting image
- height int? (default ()) - The desired height of the resulting image.
- fittingMode FittingMode? (default ()) - The method used to scale the chart to the specified dimensions (if both height and width are set)
- sessionId string? (default ()) - Session ID
resetChartData
function resetChartData(string workbookIdOrPath, string worksheetNameOrId, string chartName, json sourceData, SeriesBy? seriesBy, string? sessionId) returns error?
Resets the source data for the chart.
Parameters
- workbookIdOrPath string - Workbook ID or file path. Path should be with the
.xlsx
extension from root. If a worksbook is in root, path will be<FILE_NAME>.xlsx
)
- worksheetNameOrId string - Worksheet name or ID
- chartName string - Chart name
- sourceData json - The Range object corresponding to the source data
- seriesBy SeriesBy? (default ()) - Specifies the way columns or rows are used as data series on the chart
- sessionId string? (default ()) - Session ID
Return Type
- error? -
()
or else anerror
if failed
setChartPosition
function setChartPosition(string workbookIdOrPath, string worksheetNameOrId, string chartName, string startCell, string? endCell, string? sessionId) returns error?
Positions the chart relative to cells on the worksheet.
Parameters
- workbookIdOrPath string - Workbook ID or file path. Path should be with the
.xlsx
extension from root. If a worksbook is in root, path will be<FILE_NAME>.xlsx
)
- worksheetNameOrId string - Worksheet name or ID
- chartName string - Chart name
- startCell string - The start cell. This is where the chart will be moved to. The start cell is the top-left or top-right cell, depending on the user's right-to-left display settings.
- endCell string? (default ()) - The end cell. If specified, the chart's width and height will be set to fully cover up this cell/range
- sessionId string? (default ()) - Session ID
Return Type
- error? -
()
or else anerror
if failed
deleteChart
function deleteChart(string workbookIdOrPath, string worksheetNameOrId, string chartName, string? sessionId) returns error?
Deletes a chart.
Parameters
- workbookIdOrPath string - Workbook ID or file path. Path should be with the
.xlsx
extension from root. If a worksbook is in root, path will be<FILE_NAME>.xlsx
)
- worksheetNameOrId string - Worksheet name or ID
- chartName string - Chart name
- sessionId string? (default ()) - Session ID
Return Type
- error? -
()
or else anerror
if failed
getWorkbookApplication
function getWorkbookApplication(string workbookIdOrPath, string? sessionId) returns WorkbookApplication|error
Retrieves the properties of a workbookApplication.
Parameters
- workbookIdOrPath string - Workbook ID or file path. Path should be with the
.xlsx
extension from root. If a worksbook is in root, path will be<FILE_NAME>.xlsx
)
- sessionId string? (default ()) - Session ID
Return Type
- WorkbookApplication|error -
WorkbookApplication
or else anerror
if failed
calculateWorkbookApplication
function calculateWorkbookApplication(string workbookIdOrPath, CalculationType 'type, string? sessionId) returns error?
Recalculates all currently opened workbooks in Excel.
Parameters
- workbookIdOrPath string - Workbook ID or file path. Path should be with the
.xlsx
extension from root. If a worksbook is in root, path will be<FILE_NAME>.xlsx
)
- 'type CalculationType - Specifies the calculation type to use
- sessionId string? (default ()) - Session ID
Return Type
- error? -
()
or else anerror
if failed
Enums
microsoft.excel: CalculationType
Specifies the calculation type to use in the workbook.
Members
microsoft.excel: FittingMode
Specifies the options used to scale the chart to the specified dimensions.
Members
microsoft.excel: SeriesBy
Specifies the way columns or rows are used as data series on the chart.
Members
microsoft.excel: Visibility
Specifies Visibility options in the worksheet.
Members
Records
microsoft.excel: Cell
Represents cell properties.
Fields
- address string - Represents the range reference in A1-style. Address value will contain the Sheet reference (e.g. Sheet1!A1:B4)
- addressLocal string - Represents cell reference in the language of the user
- columnIndex int - Represents the column number of the first cell in the range. Zero-indexed
- formulas json - Represents the formula in A1-style notation
- formulasLocal json - Represents the formula in A1-style notation, in the user's language and number-formatting locale. For example, the English "=SUM(A1, 1.5)" formula would become "=SUMME(A1; 1,5)" in German
- formulasR1C1 json - Represents the formula in R1C1-style notation
- hidden boolean - Represents if cell is hidden
- numberFormat json - Excel's number format code for the given cell
- rowIndex int - Returns the row number of the first cell in the range. Zero-indexed
- text json - Text values of the specified range. The Text value will not depend on the cell width. The # sign substitution that happens in Excel UI will not affect the text value returned by the API
- valueTypes json - Represents the type of data of each cell. The data returned could be of type string, number, or a boolean. Cell that contain an error will return the error string
- values json[] - Raw value of the specified cell
microsoft.excel: Chart
Chart object in a workbook.
Fields
- id string & readonly? - Chart ID
- height float? - The height, in points, of the chart object
- left float? - The distance, in points, from the left side of the chart to the worksheet origin
- name string? - The name of a chart
- top float? - The distance, in points, from the top edge of the object to the top of row 1 (on a worksheet) or the top of the chart area (on a chart)
- width float? - The width, in points, of the chart object
microsoft.excel: Column
Represents column properties.
Fields
- id string - A unique key that identifies the column within the table. This property should be interpreted as an opaque string value and should not be parsed to any other type
- name string? - The name of the table column
- index int - The index number of the column within the columns collection of the table
- values json[] - Raw values of the specified range. The data returned could be of type string, number, or a boolean. Cell that contain an error will return the error string
microsoft.excel: ConnectionConfig
Client configuration details.
Fields
- Fields Included from *ConnectionConfig
- auth AuthConfig
- httpVersion HttpVersion
- http1Settings ClientHttp1Settings
- http2Settings ClientHttp2Settings
- timeout decimal
- forwarded string
- poolConfig PoolConfiguration
- cache CacheConfig
- compression Compression
- circuitBreaker CircuitBreakerConfig
- retryConfig RetryConfig
- responseLimits ResponseLimitConfigs
- secureSocket ClientSecureSocket
- proxy ProxyConfig
- validation boolean
- anydata...
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig - Configurations related to client authentication
microsoft.excel: Row
Represents row properties.
Fields
- index int - Returns the index number of the row within the rows collection of the table. Zero-indexed
- values json[] - Represents the raw values of the specified range. The data returned could be of type string, number, or a boolean. Cell that contain an error will return the error strings
microsoft.excel: Table
Represents an Excel table.
Fields
- id string & readonly? - Returns a value that uniquely identifies the table in a given workbook. The value of the identifier remains the same even when the table is renamed. This property should be interpreted as an opaque string value and should not be parsed to any other type
- name string? - Name of the table
- showHeaders boolean? - Indicates whether the header row is visible or not. This value can be set to show or remove the header row
- showTotals boolean? - Indicates whether the total row is visible or not. This value can be set to show or remove the total row.
- style string? - Constant value that represents the Table style. The possible values are: TableStyleLight1 thru TableStyleLight21, TableStyleMedium1 thru TableStyleMedium28, TableStyleStyleDark1 thru TableStyleStyleDark11. A custom user-defined style present in the workbook can also be specified.
- highlightFirstColumn boolean? - Indicates whether the first column contains special formatting
- highlightLastColumn boolean? - Indicates whether the last column contains special formatting
- showBandedColumns boolean? - Indicates whether the columns show banded formatting in which odd columns are highlighted differently from even ones to make reading the table easier
- showBandedRows boolean? - Indicates whether the rows show banded formatting in which odd rows are highlighted differently from even ones to make reading the table easier
- showFilterButton boolean? - Indicates whether the filter buttons are visible at the top of each column header. Setting this is only allowed if the table contains a header row
- legacyId string & readonly? - Legacy Id used in older Excle clients. The value of the identifier remains the same even when the table is renamed. This property should be interpreted as an opaque string value and should not be parsed to any other type
microsoft.excel: WorkbookApplication
Represents the Excel application that manages the workbook.
Fields
- calculationMode string - Returns the calculation mode used in the workbook
microsoft.excel: Worksheet
Represents worksheet properties.
Fields
- id string & readonly? - Returns a value that uniquely identifies the worksheet in a given workbook. The value of the identifier remains the same even when the worksheet is renamed or moved
- position int? - The zero-based position of the worksheet within the workbook
- name string? - Worksheet name
- visibility Visibility? - The visibility of the worksheet
Import
import ballerinax/microsoft.excel;
Metadata
Released date: over 1 year ago
Version: 2.4.0
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 1932
Current verison: 252
Weekly downloads
Keywords
Productivity/Spreadsheets
Cost/Free
Vendor/Microsoft
Contributors