medium
Module medium
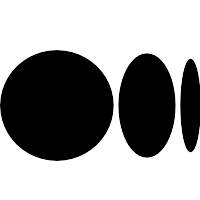
ballerinax/medium Ballerina library
Overview
This is a generated connector for Medium API v1 OpenAPI Specification.
Medium’s Publishing API provides capability to access the Medium network, create your content on Medium from anywhere you write, and expand your audience and your influence.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Medium account
- Obtain tokens
- Follow this link to obtain tokens
Quickstart
To use the Medium connector in your Ballerina application, update the .bal file as follows: Add steps to create a simple sample
Step 1 - Import connector
import ballerinax/medium;
Step 2 - Create a new connector instance
configurable http:BearerTokenConfig & readonly auth = ?; ClientConfig clientConfig = {auth : auth}; Client baseClient = check new Client(clientConfig);
Step 3 - Invoke connector operation
- Invoking connector operations using the client.
medium:UserResponse response = check baseClient->getUserDetail();
- Use
bal run
command to compile and run the Ballerina program.
Clients
medium: Client
This is a generated connector for Medium API v1 OpenAPI Specification. Medium’s Publishing API provides capability to access the Medium network, create your content on Medium from anywhere you write, and expand your audience and your influence.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Medium account and obtain tokens following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.medium.com/v1" - URL of the target service
getUserDetail
function getUserDetail() returns UserResponse|error
Get the authenticated user’s details
Return Type
- UserResponse|error - If success returns details of the user who has granted permission to the application otherwise the relevant error
getPublicationList
function getPublicationList(string userId) returns PublicationResponse|error
List the user’s publications
Parameters
- userId string - A unique identifier for the user.
Return Type
- PublicationResponse|error - If success returns a list of publications that the user is subscribed to, writes to, or edits otherwise the relevant error
getContributorList
function getContributorList(string publicationId) returns ContributorResponse|error
List contributors for a given publication
Parameters
- publicationId string - A unique identifier for the publication.
Return Type
- ContributorResponse|error - If success returns a list of contributors
createUserPost
function createUserPost(string authorId, Post payload) returns PostResponse|error
Creates a post on the authenticated user’s profile
Parameters
- authorId string - authorId is the user id of the authenticated user.
- payload Post - Creates a post for user.
Return Type
- PostResponse|error - If success returns a Post record that includes the newly created post detail otherwise the relevant error
Records
medium: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
medium: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
medium: Contributor
Contributor
Fields
- publicationId string? - An ID for the publication. This can be lifted from response of publications above
- userId string? - A user ID of the contributor.
- role string? - Role of the user identified by userId in the publication identified by
publicationId
. editor or writer
medium: ContributorResponse
list of contributors for a given publication
Fields
- data Contributor[]? -
medium: OAuth2RefreshTokenGrantConfig
OAuth2 Refresh Token Grant Configs
Fields
- Fields Included from *OAuth2RefreshTokenGrantConfig
- refreshUrl string(default "https://medium.com/v1/tokens") - Refresh URL
medium: Post
Fields
- title string - The title of the post. Note that this title is used for SEO and when rendering the post as a listing, but will not appear in the actual post—for that, the title must be specified in the content field as well. Titles longer than 100 characters will be ignored. In that case, a title will be synthesized from the first content in the post when it is published.
- contentFormat string - The format of the "content" field. There are two valid values, "html", and "markdown"
- content string - The body of the post, in a valid, semantic, HTML fragment, or Markdown. Further markups may be supported in the future. For a full list of accepted HTML tags, see here. If you want your title to appear on the post page, you must also include it as part of the post content.
- tags string[]? - Tags to classify the post. Only the first three will be used. Tags longer than 25 characters will be ignored.
- canonicalUrl string? - The original home of this content, if it was originally published elsewhere.
- publishStatus string(default "public") - The status of the post. Valid values are
public
,draft
, orunlisted
. The default ispublic
.
- license string(default "all-rights-reserved") - The license of the post. Valid values are
all-rights-reserved
,cc-40-by
,cc-40-by-sa
,cc-40-by-nd
,cc-40-by-nc
,cc-40-by-nc-nd
,cc-40-by-nc-sa
,cc-40-zero
,public-domain
. The default isall-rights-reserved
.
medium: PostDetails
Details of Post
Fields
- id string? - A unique identifier for the post.
- title string? - The post’s title
- authorId string? - The userId of the post’s author
- tags string[]? - The post’s tags
- url string? - The URL of the post on Medium
- canonicalUrl string? - The canonical URL of the post. If canonicalUrl was not specified in the creation of the post, this field will not be present.
- publishStatus string? - The publish status of the post.
- publishedAt int? - The post’s published date. If created as a draft, this field will not be present.
- license string? - The license of the post.
- licenseUrl string? - The URL to the license of the post.
medium: PostResponse
Container object for post info
Fields
- data PostDetails? - Details of Post
medium: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
medium: Publication
Publications provide a way for authors to work collaboratively within a common narrative framework, brand or point of view.
Fields
- id string? - A unique identifier for the publication.
- name string? - The publication’s name on Medium.
- description string? - Short description of the publication
- url string? - The URL to the publication’s homepage
- imageUrl string? - The URL to the publication’s image/logo
medium: PublicationResponse
Container object for publication list.
Fields
- data Publication[]? -
medium: User
Represents a user
Fields
- id string? - A unique identifier for the user.
- username string? - The user’s username on Medium.
- name string? - The user’s name on Medium.
- url string? - The URL to the user’s profile on Medium
- imageUrl string? - The URL to the user’s avatar on Medium
medium: UserResponse
Container object for user info
Fields
- data User? - Represents a user
Import
import ballerinax/medium;
Metadata
Released date: about 2 years ago
Version: 1.5.0
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 78
Current verison: 0
Weekly downloads
Keywords
Content & Files/Blogs
Cost/Freemium
Contributors
Dependencies