mailchimp
Module mailchimp
API
Definitions
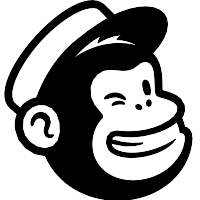
ballerinax/mailchimp Ballerina library
Overview
- Explain What does this module do*
This module supports
Obtaining tokens
Prerequisites
- If you don't have a Mailchimp account already, you’ll need to create one in order to use the API.
Obtaining tokens
- Navigate to the API Keys section of your Mailchimp account.
- If you already have an API key listed and you’d like to use it for your application, simply copy it. Otherwise, click Create a Key and give it a descriptive name that will remind you which application it’s used for.
Quickstart
API Root URL
The root url for the API is https://
There are a few ways to find your data center. It’s the first part of the URL you see in the API keys section of your account; if the URL is https://us6.mailchimp.com/account/api/, then the data center subdomain is us6
. It’s also appended to your API key in the form key-dc; if your API key is 0123456789abcdef0123456789abcde-us6
, then the data center subdomain is us6
.
Creating a Client
To use the connector, first you must create a mailchimp:Client
object. The samples for creating a Mailchimp client can be found below.
mailchimp:ClientConfig clientConfig = { auth: { username: "<username>", password: "<API_KEY>" } }; mailchimp:Client mailchimpClient = check new (clientConfig,"<API_Root_URL>");
Snippets
Health Check - Ping Operation
mailchimp:GetPingResponse getPingResponse = check mailchimpClient->getPing(); log:printInfo(getPingResponse?.health_status);
Get Campaigns
mailchimp:GetCampaignsResponse campaigns = check mailchimpClient->getCampaigns(); log:printInfo("Response: ", campaigns=campaigns);
Create a Campaign
mailchimp:PostCampaignsRequest campaignCreateRequest = { 'type: "regular" }; mailchimp:PostCampaignsResponse campaignCreateResponse = check mailchimpClient->postCampaigns(campaignCreateRequest); log:printInfo("Response: ", createdCampaign=campaignCreateResponse);
Clients
mailchimp: Client
This is a generated connector for Mailchimp Marketing API v3.0.52 OpenAPI Specification. The Mailchimp Marketing API allow to manage audiences, control automation workflows, sync email activity with your database and more.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Mailchimp account and navigate to the API Keys section.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://server.api.mailchimp.com/3.0" - URL of the target service
getRoot
List api root resources
Parameters
- fields string[]? (default ()) - A comma-separated list of fields to return. Reference parameters of sub-objects with dot notation.
- excludeFields string[]? (default ()) - A comma-separated list of fields to exclude. Reference parameters of sub-objects with dot notation.
getAuthorizedApps
function getAuthorizedApps(string[]? fields, string[]? excludeFields, int count, int offset) returns InlineResponse200|error
List authorized apps
Parameters
- fields string[]? (default ()) - A comma-separated list of fields to return. Reference parameters of sub-objects with dot notation.
- excludeFields string[]? (default ()) - A comma-separated list of fields to exclude. Reference parameters of sub-objects with dot notation.
- count int (default 10) - The number of records to return. Default value is 10. Maximum value is 1000
- offset int (default 0) - Used for pagination, this it the number of records from a collection to skip. Default value is 0.
Return Type
- InlineResponse200|error - An array of objects, each representing an authorized application.
getAuthorizedAppsId
function getAuthorizedAppsId(string appId, string[]? fields, string[]? excludeFields) returns InlineResponse2001|error
Get authorized app info
Parameters
- appId string - The unique id for the connected authorized application.
- fields string[]? (default ()) - A comma-separated list of fields to return. Reference parameters of sub-objects with dot notation.
- excludeFields string[]? (default ()) - A comma-separated list of fields to exclude. Reference parameters of sub-objects with dot notation.
Return Type
- InlineResponse2001|error - An authorized app.
getAutomations
function getAutomations(int count, int offset, string[]? fields, string[]? excludeFields, string? beforeCreateTime, string? sinceCreateTime, string? beforeStartTime, string? sinceStartTime, string? status) returns InlineResponse2002|error
List automations
Parameters
- count int (default 10) - The number of records to return. Default value is 10. Maximum value is 1000
- offset int (default 0) - Used for pagination, this it the number of records from a collection to skip. Default value is 0.
- fields string[]? (default ()) - A comma-separated list of fields to return. Reference parameters of sub-objects with dot notation.
- excludeFields string[]? (default ()) - A comma-separated list of fields to exclude. Reference parameters of sub-objects with dot notation.
- beforeCreateTime string? (default ()) - Restrict the response to automations created before this time. Uses the ISO 8601 time format: 2015-10-21T15:41:36+00:00.
- sinceCreateTime string? (default ()) - Restrict the response to automations created after this time. Uses the ISO 8601 time format: 2015-10-21T15:41:36+00:00.
- beforeStartTime string? (default ()) - Restrict the response to automations started before this time. Uses the ISO 8601 time format: 2015-10-21T15:41:36+00:00.
- sinceStartTime string? (default ()) - Restrict the response to automations started after this time. Uses the ISO 8601 time format: 2015-10-21T15:41:36+00:00.
- status string? (default ()) - Restrict the results to automations with the specified status.
Return Type
- InlineResponse2002|error - An array of objects, each representing an Automation workflow.
postAutomations
function postAutomations(AutomationWorkflow payload) returns AutomationWorkflow1|error
Add automation
Parameters
- payload AutomationWorkflow - Add automation input payload
Return Type
- AutomationWorkflow1|error - A summary of an individual Automation workflow's settings and content.
getAutomationsId
function getAutomationsId(string workflowId, string[]? fields, string[]? excludeFields) returns AutomationWorkflow1|error
Get automation info
Parameters
- workflowId string - The unique id for the Automation workflow.
- fields string[]? (default ()) - A comma-separated list of fields to return. Reference parameters of sub-objects with dot notation.
- excludeFields string[]? (default ()) - A comma-separated list of fields to exclude. Reference parameters of sub-objects with dot notation.
Return Type
- AutomationWorkflow1|error - A summary of an individual Automation workflow's settings and content.
postAutomationsIdActionsPauseAllEmails
Pause automation emails
Parameters
- workflowId string - The unique id for the Automation workflow.
postAutomationsIdActionsStartAllEmails
Start automation emails
Parameters
- workflowId string - The unique id for the Automation workflow.
archiveAutomations
Archive automation
Parameters
- workflowId string - The unique id for the Automation workflow.
getAutomationsIdEmails
function getAutomationsIdEmails(string workflowId) returns AutomationEmails|error
List automated emails
Parameters
- workflowId string - The unique id for the Automation workflow.
Return Type
- AutomationEmails|error - A summary of the emails in an Automation workflow.
getAutomationsIdEmailsId
function getAutomationsIdEmailsId(string workflowId, string workflowEmailId) returns AutomationWorkflowEmail|error
Get workflow email info
Parameters
- workflowId string - The unique id for the Automation workflow.
- workflowEmailId string - The unique id for the Automation workflow email.
Return Type
- AutomationWorkflowEmail|error - A summary of an individual Automation workflow email.
deleteAutomationsIdEmailsId
function deleteAutomationsIdEmailsId(string workflowId, string workflowEmailId) returns Response|error
Delete workflow email
Parameters
- workflowId string - The unique id for the Automation workflow.
- workflowEmailId string - The unique id for the Automation workflow email.
patchAutomationEmailWorkflowId
function patchAutomationEmailWorkflowId(string workflowId, string workflowEmailId, UpdateInformationAboutASpecificWorkflowEmail payload) returns AutomationWorkflowEmail|error
Update workflow email
Parameters
- workflowId string - The unique id for the Automation workflow.
- workflowEmailId string - The unique id for the Automation workflow email.
- payload UpdateInformationAboutASpecificWorkflowEmail - Update workflow email input payload
Return Type
- AutomationWorkflowEmail|error - A summary of an individual Automation workflow email.
getAutomationsIdEmailsIdQueue
function getAutomationsIdEmailsIdQueue(string workflowId, string workflowEmailId) returns InlineResponse2003|error
List automated email subscribers
Parameters
- workflowId string - The unique id for the Automation workflow.
- workflowEmailId string - The unique id for the Automation workflow email.
Return Type
- InlineResponse2003|error - An automation workflow
postAutomationsIdEmailsIdQueue
function postAutomationsIdEmailsIdQueue(string workflowId, string workflowEmailId, SubscriberInAutomationQueue payload) returns SubscriberInAutomationQueue1|error
Add subscriber to workflow email
Parameters
- workflowId string - The unique id for the Automation workflow.
- workflowEmailId string - The unique id for the Automation workflow email.
- payload SubscriberInAutomationQueue - Add subscriber to workflow email input payload
Return Type
- SubscriberInAutomationQueue1|error - Information about subscribers in an Automation email queue.
getAutomationsIdEmailsIdQueueId
function getAutomationsIdEmailsIdQueueId(string workflowId, string workflowEmailId, string subscriberHash) returns SubscriberInAutomationQueue1|error
Get automated email subscriber
Parameters
- workflowId string - The unique id for the Automation workflow.
- workflowEmailId string - The unique id for the Automation workflow email.
- subscriberHash string - The MD5 hash of the lowercase version of the list member's email address.
Return Type
- SubscriberInAutomationQueue1|error - Information about subscribers in an Automation email queue.
postAutomationsIdEmailsIdActionsPause
function postAutomationsIdEmailsIdActionsPause(string workflowId, string workflowEmailId) returns Response|error
Pause automated email
Parameters
- workflowId string - The unique id for the Automation workflow.
- workflowEmailId string - The unique id for the Automation workflow email.
postAutomationsIdEmailsIdActionsStart
function postAutomationsIdEmailsIdActionsStart(string workflowId, string workflowEmailId) returns Response|error
Start automated email
Parameters
- workflowId string - The unique id for the Automation workflow.
- workflowEmailId string - The unique id for the Automation workflow email.
getAutomationsIdRemovedSubscribers
function getAutomationsIdRemovedSubscribers(string workflowId) returns RemovedSubscribers|error
List subscribers removed from workflow
Parameters
- workflowId string - The unique id for the Automation workflow.
Return Type
- RemovedSubscribers|error - A summary of the subscribers who were removed from an Automation workflow.
postAutomationsIdRemovedSubscribers
function postAutomationsIdRemovedSubscribers(string workflowId, SubscriberInAutomationQueue2 payload) returns SubscriberRemovedFromAutomationWorkflow|error
Remove subscriber from workflow
Parameters
- workflowId string - The unique id for the Automation workflow.
- payload SubscriberInAutomationQueue2 - Remove subscriber from workflow input payload
Return Type
- SubscriberRemovedFromAutomationWorkflow|error - A summary of a subscriber removed from an Automation workflow.
getAutomationsIdRemovedSubscribersId
function getAutomationsIdRemovedSubscribersId(string workflowId, string subscriberHash) returns SubscriberRemovedFromAutomationWorkflow|error
Get subscriber removed from workflow
Parameters
- workflowId string - The unique id for the Automation workflow.
- subscriberHash string - The MD5 hash of the lowercase version of the list member's email address.
Return Type
- SubscriberRemovedFromAutomationWorkflow|error - A summary of a subscriber removed from an Automation workflow
getCampaigns
function getCampaigns(string[]? fields, string[]? excludeFields, int count, int offset, string? 'type, string? status, string? beforeSendTime, string? sinceSendTime, string? beforeCreateTime, string? sinceCreateTime, string? listId, string? folderId, string? memberId, string? sortField, string? sortDir) returns InlineResponse2004|error
List campaigns
Parameters
- fields string[]? (default ()) - A comma-separated list of fields to return. Reference parameters of sub-objects with dot notation.
- excludeFields string[]? (default ()) - A comma-separated list of fields to exclude. Reference parameters of sub-objects with dot notation.
- count int (default 10) - The number of records to return. Default value is 10. Maximum value is 1000
- offset int (default 0) - Used for pagination, this it the number of records from a collection to skip. Default value is 0.
- 'type string? (default ()) - The campaign type.
- status string? (default ()) - The status of the campaign.
- beforeSendTime string? (default ()) - Restrict the response to campaigns sent before the set time. Uses ISO 8601 time format: 2015-10-21T15:41:36+00:00.
- sinceSendTime string? (default ()) - Restrict the response to campaigns sent after the set time. Uses ISO 8601 time format: 2015-10-21T15:41:36+00:00.
- beforeCreateTime string? (default ()) - Restrict the response to campaigns created before the set time. Uses ISO 8601 time format: 2015-10-21T15:41:36+00:00.
- sinceCreateTime string? (default ()) - Restrict the response to campaigns created after the set time. Uses ISO 8601 time format: 2015-10-21T15:41:36+00:00.
- listId string? (default ()) - The unique id for the list.
- folderId string? (default ()) - The unique folder id.
- memberId string? (default ()) - Retrieve campaigns sent to a particular list member. Member ID is The MD5 hash of the lowercase version of the list member’s email address.
- sortField string? (default ()) - Returns files sorted by the specified field.
- sortDir string? (default ()) - Determines the order direction for sorted results.
Return Type
- InlineResponse2004|error - An array of campaigns
postCampaigns
Add campaign
Parameters
- payload Campaign - Add campaign input payload
getCampaignsId
function getCampaignsId(string campaignId, string[]? fields, string[]? excludeFields) returns Campaign1|error
Get campaign info
Parameters
- campaignId string - The unique id for the campaign.
- fields string[]? (default ()) - A comma-separated list of fields to return. Reference parameters of sub-objects with dot notation.
- excludeFields string[]? (default ()) - A comma-separated list of fields to exclude. Reference parameters of sub-objects with dot notation.
deleteCampaignsId
Delete campaign
Parameters
- campaignId string - The unique id for the campaign.
patchCampaignsId
Update campaign settings
Parameters
- campaignId string - The unique id for the campaign.
- payload Campaign2 - Update campaign input payload
postCampaignsIdActionsCancelSend
Cancel campaign
Parameters
- campaignId string - The unique id for the campaign.
postCampaignsIdActionsReplicate
Replicate campaign
Parameters
- campaignId string - The unique id for the campaign.
postCampaignsIdActionsSend
Send campaign
Parameters
- campaignId string - The unique id for the campaign.
postCampaignsIdActionsSchedule
function postCampaignsIdActionsSchedule(string campaignId, ActionsScheduleBody payload) returns Response|error
Schedule campaign
Parameters
- campaignId string - The unique id for the campaign.
- payload ActionsScheduleBody - Schedule campaign input payload
postCampaignsIdActionsUnschedule
Unschedule campaign
Parameters
- campaignId string - The unique id for the campaign.
postCampaignsIdActionsTest
function postCampaignsIdActionsTest(string campaignId, ActionsTestBody payload) returns Response|error
Send test email
Parameters
- campaignId string - The unique id for the campaign.
- payload ActionsTestBody - Send test email input payload
postCampaignsIdActionsPause
Pause rss campaign
Parameters
- campaignId string - The unique id for the campaign.
postCampaignsIdActionsResume
Resume rss campaign
Parameters
- campaignId string - The unique id for the campaign.
postCampaignsIdActionsCreateResend
Resend campaign
Parameters
- campaignId string - The unique id for the campaign.
getCampaignsIdContent
function getCampaignsIdContent(string campaignId, string[]? fields, string[]? excludeFields) returns CampaignContent|error
Get campaign content
Parameters
- campaignId string - The unique id for the campaign.
- fields string[]? (default ()) - A comma-separated list of fields to return. Reference parameters of sub-objects with dot notation.
- excludeFields string[]? (default ()) - A comma-separated list of fields to exclude. Reference parameters of sub-objects with dot notation.
Return Type
- CampaignContent|error - The HTML and plain-text content for a campaign
putCampaignsIdContent
function putCampaignsIdContent(string campaignId, CampaignContent1 payload) returns CampaignContent|error
Set campaign content
Parameters
- campaignId string - The unique id for the campaign.
- payload CampaignContent1 - Set campaign content input payload
Return Type
- CampaignContent|error - The HTML and plain-text content for a campaign
getCampaignsIdFeedback
function getCampaignsIdFeedback(string campaignId, string[]? fields, string[]? excludeFields) returns CampaignReports|error
List campaign feedback
Parameters
- campaignId string - The unique id for the campaign.
- fields string[]? (default ()) - A comma-separated list of fields to return. Reference parameters of sub-objects with dot notation.
- excludeFields string[]? (default ()) - A comma-separated list of fields to exclude. Reference parameters of sub-objects with dot notation.
Return Type
- CampaignReports|error - A summary of the comment feedback for a specific campaign
postCampaignsIdFeedback
function postCampaignsIdFeedback(string campaignId, CampaignFeedback payload) returns CampaignFeedback1|error
Add campaign feedback
Parameters
- campaignId string - The unique id for the campaign.
- payload CampaignFeedback - Add campaign feedback input payload
Return Type
- CampaignFeedback1|error - A specific feedback message from a specific campaign
getCampaignsIdFeedbackId
function getCampaignsIdFeedbackId(string campaignId, string feedbackId, string[]? fields, string[]? excludeFields) returns CampaignFeedback1|error
Get campaign feedback message
Parameters
- campaignId string - The unique id for the campaign.
- feedbackId string - The unique id for the feedback message.
- fields string[]? (default ()) - A comma-separated list of fields to return. Reference parameters of sub-objects with dot notation.
- excludeFields string[]? (default ()) - A comma-separated list of fields to exclude. Reference parameters of sub-objects with dot notation.
Return Type
- CampaignFeedback1|error - A specific feedback message from a specific campaign
deleteCampaignsIdFeedbackId
Delete campaign feedback message
Parameters
- campaignId string - The unique id for the campaign.
- feedbackId string - The unique id for the feedback message.
patchCampaignsIdFeedbackId
function patchCampaignsIdFeedbackId(string campaignId, string feedbackId, CampaignFeedback2 payload) returns CampaignFeedback1|error
Update campaign feedback message
Parameters
- campaignId string - The unique id for the campaign.
- feedbackId string - The unique id for the feedback message.
- payload CampaignFeedback2 - Update campaign feedback messages input payload
Return Type
- CampaignFeedback1|error - A specific feedback message from a specific campaign
getCampaignsIdSendChecklist
function getCampaignsIdSendChecklist(string campaignId, string[]? fields, string[]? excludeFields) returns SendChecklist|error
Get campaign send checklist
Parameters
- campaignId string - The unique id for the campaign.
- fields string[]? (default ()) - A comma-separated list of fields to return. Reference parameters of sub-objects with dot notation.
- excludeFields string[]? (default ()) - A comma-separated list of fields to exclude. Reference parameters of sub-objects with dot notation.
Return Type
- SendChecklist|error - The send checklist for the campaign
getLists
function getLists(string[]? fields, string[]? excludeFields, int count, int offset, string? beforeDateCreated, string? sinceDateCreated, string? beforeCampaignLastSent, string? sinceCampaignLastSent, string? email, string? sortField, string? sortDir, boolean? hasEcommerceStore) returns SubscriberLists|error
Get lists info
Parameters
- fields string[]? (default ()) - A comma-separated list of fields to return. Reference parameters of sub-objects with dot notation.
- excludeFields string[]? (default ()) - A comma-separated list of fields to exclude. Reference parameters of sub-objects with dot notation.
- count int (default 10) - The number of records to return. Default value is 10. Maximum value is 1000
- offset int (default 0) - Used for pagination, this it the number of records from a collection to skip. Default value is 0.
- beforeDateCreated string? (default ()) - Restrict response to lists created before the set date. Uses ISO 8601 time format: 2015-10-21T15:41:36+00:00.
- sinceDateCreated string? (default ()) - Restrict results to lists created after the set date. Uses ISO 8601 time format: 2015-10-21T15:41:36+00:00.
- beforeCampaignLastSent string? (default ()) - Restrict results to lists created before the last campaign send date. Uses ISO 8601 time format: 2015-10-21T15:41:36+00:00.
- sinceCampaignLastSent string? (default ()) - Restrict results to lists created after the last campaign send date. Uses ISO 8601 time format: 2015-10-21T15:41:36+00:00.
- email string? (default ()) - Restrict results to lists that include a specific subscriber's email address.
- sortField string? (default ()) - Returns files sorted by the specified field.
- sortDir string? (default ()) - Determines the order direction for sorted results.
- hasEcommerceStore boolean? (default ()) - Restrict results to lists that contain an active, connected, undeleted ecommerce store.
Return Type
- SubscriberLists|error - A collection of subscriber lists for this account. Lists contain subscribers who have opted-in to receive correspondence from you or your organization
postLists
function postLists(SubscriberList payload) returns SubscriberList1|error
Add list
Parameters
- payload SubscriberList - Add list input payload
Return Type
- SubscriberList1|error - Information about a specific list.
getListsId
function getListsId(string listId, string[]? fields, string[]? excludeFields, boolean? includeTotalContacts) returns SubscriberList1|error
Get list info
Parameters
- listId string - The unique ID for the list.
- fields string[]? (default ()) - A comma-separated list of fields to return. Reference parameters of sub-objects with dot notation.
- excludeFields string[]? (default ()) - A comma-separated list of fields to exclude. Reference parameters of sub-objects with dot notation.
- includeTotalContacts boolean? (default ()) - Return the total_contacts field in the stats response, which contains an approximate contact count for the given list.
Return Type
- SubscriberList1|error - Information about a specific list
postListsId
function postListsId(string listId, MembersToSubscribeunsubscribeTofromAListInBatch payload, boolean? skipMergeValidation, boolean? skipDuplicateCheck) returns BatchUpdateListMembers|error
Batch subscribe or unsubscribe
Parameters
- listId string - The unique ID for the list.
- payload MembersToSubscribeunsubscribeTofromAListInBatch - Batch subscribe or unsubscribe input payload
- skipMergeValidation boolean? (default ()) - If skip_merge_validation is true, member data will be accepted without merge field values, even if the merge field is usually required. This defaults to false.
- skipDuplicateCheck boolean? (default ()) - If skip_duplicate_check is true, we will ignore duplicates sent in the request when using the batch sub/unsub on the lists endpoint. The status of the first appearance in the request will be saved. This defaults to false.
Return Type
- BatchUpdateListMembers|error - Batch update list members
deleteListsId
Delete list
Parameters
- listId string - The unique ID for the list.
patchListsId
function patchListsId(string listId, SubscriberList2 payload) returns SubscriberList1|error
Update lists
Parameters
- listId string - The unique ID for the list.
- payload SubscriberList2 - Update the settings for a list input payload
Return Type
- SubscriberList1|error - Information about a specific list
getListsIdAbuseReports
function getListsIdAbuseReports(string listId, string[]? fields, string[]? excludeFields, int count, int offset) returns AbuseComplaints|error
List abuse reports
Parameters
- listId string - The unique ID for the list.
- fields string[]? (default ()) - A comma-separated list of fields to return. Reference parameters of sub-objects with dot notation.
- excludeFields string[]? (default ()) - A comma-separated list of fields to exclude. Reference parameters of sub-objects with dot notation.
- count int (default 10) - The number of records to return. Default value is 10. Maximum value is 1000
- offset int (default 0) - Used for pagination, this it the number of records from a collection to skip. Default value is 0.
Return Type
- AbuseComplaints|error - A collection of abuse complaints for a specific list. An abuse complaint occurs when your recipient clicks to 'report spam' in their email program
getListsIdAbuseReportsId
function getListsIdAbuseReportsId(string listId, string reportId, string[]? fields, string[]? excludeFields, int count, int offset) returns AbuseComplaint|error
Get abuse report
Parameters
- listId string - The unique ID for the list.
- reportId string - The id for the abuse report.
- fields string[]? (default ()) - A comma-separated list of fields to return. Reference parameters of sub-objects with dot notation.
- excludeFields string[]? (default ()) - A comma-separated list of fields to exclude. Reference parameters of sub-objects with dot notation.
- count int (default 10) - The number of records to return. Default value is 10. Maximum value is 1000
- offset int (default 0) - Used for pagination, this it the number of records from a collection to skip. Default value is 0.
Return Type
- AbuseComplaint|error - Details of abuse complaints for a specific list. An abuse complaint occurs when your recipient clicks to 'report spam' in their email program
getListsIdActivity
function getListsIdActivity(string listId, string[]? fields, string[]? excludeFields) returns ListActivity|error
List recent activity
Parameters
- listId string - The unique ID for the list.
- fields string[]? (default ()) - A comma-separated list of fields to return. Reference parameters of sub-objects with dot notation.
- excludeFields string[]? (default ()) - A comma-separated list of fields to exclude. Reference parameters of sub-objects with dot notation.
Return Type
- ListActivity|error - Up to the previous 180 days of daily detailed aggregated activity stats for a specific list. Does not include AutoResponder or Automation activity
getListsIdClients
function getListsIdClients(string listId, string[]? fields, string[]? excludeFields) returns EmailClients|error
List top email clients
Parameters
- listId string - The unique ID for the list.
- fields string[]? (default ()) - A comma-separated list of fields to return. Reference parameters of sub-objects with dot notation.
- excludeFields string[]? (default ()) - A comma-separated list of fields to exclude. Reference parameters of sub-objects with dot notation.
Return Type
- EmailClients|error - The top email clients based on user-agent strings
getListsIdGrowthHistory
function getListsIdGrowthHistory(string listId, string[]? fields, string[]? excludeFields, int count, int offset, string? sortField, string? sortDir) returns GrowthHistory|error
List growth history data
Parameters
- listId string - The unique ID for the list.
- fields string[]? (default ()) - A comma-separated list of fields to return. Reference parameters of sub-objects with dot notation.
- excludeFields string[]? (default ()) - A comma-separated list of fields to exclude. Reference parameters of sub-objects with dot notation.
- count int (default 10) - The number of records to return. Default value is 10. Maximum value is 1000
- offset int (default 0) - Used for pagination, this it the number of records from a collection to skip. Default value is 0.
- sortField string? (default ()) - Returns files sorted by the specified field.
- sortDir string? (default ()) - Determines the order direction for sorted results.
Return Type
- GrowthHistory|error - A month-by-month summary of a specific list's growth activity
getListsIdGrowthHistoryId
function getListsIdGrowthHistoryId(string listId, string month, string[]? fields, string[]? excludeFields) returns GrowthHistory1|error
Get growth history by month
Parameters
- listId string - The unique ID for the list.
- month string - A specific month of list growth history.
- fields string[]? (default ()) - A comma-separated list of fields to return. Reference parameters of sub-objects with dot notation.
- excludeFields string[]? (default ()) - A comma-separated list of fields to exclude. Reference parameters of sub-objects with dot notation.
Return Type
- GrowthHistory1|error - A summary of a specific list's growth activity for a specific month and year
getListsIdInterestCategories
function getListsIdInterestCategories(string listId, string[]? fields, string[]? excludeFields, int count, int offset, string? 'type) returns InterestGroupings|error
List interest categories
Parameters
- listId string - The unique ID for the list.
- fields string[]? (default ()) - A comma-separated list of fields to return. Reference parameters of sub-objects with dot notation.
- excludeFields string[]? (default ()) - A comma-separated list of fields to exclude. Reference parameters of sub-objects with dot notation.
- count int (default 10) - The number of records to return. Default value is 10. Maximum value is 1000
- offset int (default 0) - Used for pagination, this it the number of records from a collection to skip. Default value is 0.
- 'type string? (default ()) - Restrict results a type of interest group
Return Type
- InterestGroupings|error - Information about this list's interest categories
postListsIdInterestCategories
function postListsIdInterestCategories(string listId, InterestCategory payload) returns InterestCategory1|error
Add interest category
Parameters
- listId string - The unique ID for the list.
- payload InterestCategory - Add interest category input payload
Return Type
- InterestCategory1|error - Interest categories organize interests, which are used to group subscribers based on their preferences. These correspond to Group Titles the application
getListsIdInterestCategoriesId
function getListsIdInterestCategoriesId(string listId, string interestCategoryId, string[]? fields, string[]? excludeFields) returns InterestCategory1|error
Get interest category info
Parameters
- listId string - The unique ID for the list.
- interestCategoryId string - The unique ID for the interest category.
- fields string[]? (default ()) - A comma-separated list of fields to return. Reference parameters of sub-objects with dot notation.
- excludeFields string[]? (default ()) - A comma-separated list of fields to exclude. Reference parameters of sub-objects with dot notation.
Return Type
- InterestCategory1|error - Interest categories organize interests, which are used to group subscribers based on their preferences. These correspond to Group Titles the application
deleteListsIdInterestCategoriesId
function deleteListsIdInterestCategoriesId(string listId, string interestCategoryId) returns Response|error
Delete interest category
Parameters
- listId string - The unique ID for the list.
- interestCategoryId string - The unique ID for the interest category.
patchListsIdInterestCategoriesId
function patchListsIdInterestCategoriesId(string listId, string interestCategoryId, InterestCategory2 payload) returns InterestCategory1|error
Update interest category
Parameters
- listId string - The unique ID for the list.
- interestCategoryId string - The unique ID for the interest category.
- payload InterestCategory2 - Update interest category input payload
Return Type
- InterestCategory1|error - Interest categories organize interests, which are used to group subscribers based on their preferences. These correspond to Group Titles the application
getListsIdInterestCategoriesIdInterests
function getListsIdInterestCategoriesIdInterests(string listId, string interestCategoryId, string[]? fields, string[]? excludeFields, int count, int offset) returns Interests|error
List interests in category
Parameters
- listId string - The unique ID for the list.
- interestCategoryId string - The unique ID for the interest category.
- fields string[]? (default ()) - A comma-separated list of fields to return. Reference parameters of sub-objects with dot notation.
- excludeFields string[]? (default ()) - A comma-separated list of fields to exclude. Reference parameters of sub-objects with dot notation.
- count int (default 10) - The number of records to return. Default value is 10. Maximum value is 1000
- offset int (default 0) - Used for pagination, this it the number of records from a collection to skip. Default value is 0.
postListsIdInterestCategoriesIdInterests
function postListsIdInterestCategoriesIdInterests(string listId, string interestCategoryId, Interest payload) returns Interest1|error
Add interest in category
Parameters
- listId string - The unique ID for the list.
- interestCategoryId string - The unique ID for the interest category.
- payload Interest - Add interest in category input payload
Return Type
getListsIdInterestCategoriesIdInterestsId
function getListsIdInterestCategoriesIdInterestsId(string listId, string interestCategoryId, string interestId, string[]? fields, string[]? excludeFields) returns Interest1|error
Get interest in category
Parameters
- listId string - The unique ID for the list.
- interestCategoryId string - The unique ID for the interest category.
- interestId string - The specific interest or 'group name'.
- fields string[]? (default ()) - A comma-separated list of fields to return. Reference parameters of sub-objects with dot notation.
- excludeFields string[]? (default ()) - A comma-separated list of fields to exclude. Reference parameters of sub-objects with dot notation.
Return Type
deleteListsIdInterestCategoriesIdInterestsId
function deleteListsIdInterestCategoriesIdInterestsId(string listId, string interestCategoryId, string interestId) returns Response|error
Delete interest in category
Parameters
- listId string - The unique ID for the list.
- interestCategoryId string - The unique ID for the interest category.
- interestId string - The specific interest or 'group name'.
patchListsIdInterestCategoriesIdInterestsId
function patchListsIdInterestCategoriesIdInterestsId(string listId, string interestCategoryId, string interestId, Interest2 payload) returns Interest1|error
Update interest in category
Parameters
- listId string - The unique ID for the list.
- interestCategoryId string - The unique ID for the interest category.
- interestId string - The specific interest or 'group name'.
- payload Interest2 - Update interest in category input payload
Return Type
previewASegment
function previewASegment(string listId, string[]? fields, string[]? excludeFields, int count, int offset, string? 'type, string? sinceCreatedAt, string? beforeCreatedAt, boolean? includeCleaned, boolean? includeTransactional, boolean? includeUnsubscribed, string? sinceUpdatedAt, string? beforeUpdatedAt) returns CollectionOfSegments|error
List segments
Parameters
- listId string - The unique ID for the list.
- fields string[]? (default ()) - A comma-separated list of fields to return. Reference parameters of sub-objects with dot notation.
- excludeFields string[]? (default ()) - A comma-separated list of fields to exclude. Reference parameters of sub-objects with dot notation.
- count int (default 10) - The number of records to return. Default value is 10. Maximum value is 1000
- offset int (default 0) - Used for pagination, this it the number of records from a collection to skip. Default value is 0.
- 'type string? (default ()) - Limit results based on segment type.
- sinceCreatedAt string? (default ()) - Restrict results to segments created after the set time. Uses ISO 8601 time format: 2015-10-21T15:41:36+00:00.
- beforeCreatedAt string? (default ()) - Restrict results to segments created before the set time. Uses ISO 8601 time format: 2015-10-21T15:41:36+00:00.
- includeCleaned boolean? (default ()) - Include cleaned members in response
- includeTransactional boolean? (default ()) - Include transactional members in response
- includeUnsubscribed boolean? (default ()) - Include unsubscribed members in response
- sinceUpdatedAt string? (default ()) - Restrict results to segments update after the set time. Uses ISO 8601 time format: 2015-10-21T15:41:36+00:00.
- beforeUpdatedAt string? (default ()) - Restrict results to segments update before the set time. Uses ISO 8601 time format: 2015-10-21T15:41:36+00:00.
Return Type
- CollectionOfSegments|error - A list of available segments
postListsIdSegments
Add segment
getListsIdSegmentsId
function getListsIdSegmentsId(string listId, string segmentId, string[]? fields, string[]? excludeFields, boolean? includeCleaned, boolean? includeTransactional, boolean? includeUnsubscribed) returns List4|error
Get segment info
Parameters
- listId string - The unique ID for the list.
- segmentId string - The unique id for the segment.
- fields string[]? (default ()) - A comma-separated list of fields to return. Reference parameters of sub-objects with dot notation.
- excludeFields string[]? (default ()) - A comma-separated list of fields to exclude. Reference parameters of sub-objects with dot notation.
- includeCleaned boolean? (default ()) - Include cleaned members in response
- includeTransactional boolean? (default ()) - Include transactional members in response
- includeUnsubscribed boolean? (default ()) - Include unsubscribed members in response
postListsIdSegmentsId
function postListsIdSegmentsId(string listId, string segmentId, MembersToAddremoveTofromAStaticSegment payload) returns BatchAddremoveListMembersTofromStaticSegment|error
Batch add or remove members
Parameters
- listId string - The unique ID for the list.
- segmentId string - The unique id for the segment.
- payload MembersToAddremoveTofromAStaticSegment - Batch add or remove members input payload
Return Type
- BatchAddremoveListMembersTofromStaticSegment|error - Batch add/remove List members to/from static segment
deleteListsIdSegmentsId
Delete segment
Parameters
- listId string - The unique ID for the list.
- segmentId string - The unique id for the segment.
patchListsIdSegmentsId
Update segment
Parameters
- listId string - The unique ID for the list.
- segmentId string - The unique id for the segment.
- payload List5 - Update segment input payload
getListsIdSegmentsIdMembers
function getListsIdSegmentsIdMembers(string listId, string segmentId, string[]? fields, string[]? excludeFields, int count, int offset, boolean? includeCleaned, boolean? includeTransactional, boolean? includeUnsubscribed) returns SegmentMembers|error
List members in segment
Parameters
- listId string - The unique ID for the list.
- segmentId string - The unique id for the segment.
- fields string[]? (default ()) - A comma-separated list of fields to return. Reference parameters of sub-objects with dot notation.
- excludeFields string[]? (default ()) - A comma-separated list of fields to exclude. Reference parameters of sub-objects with dot notation.
- count int (default 10) - The number of records to return. Default value is 10. Maximum value is 1000
- offset int (default 0) - Used for pagination, this it the number of records from a collection to skip. Default value is 0.
- includeCleaned boolean? (default ()) - Include cleaned members in response
- includeTransactional boolean? (default ()) - Include transactional members in response
- includeUnsubscribed boolean? (default ()) - Include unsubscribed members in response
Return Type
- SegmentMembers|error - View members in a specific list segment
postListsIdSegmentsIdMembers
function postListsIdSegmentsIdMembers(string listId, string segmentId, SegmentIdMembersBody payload) returns ListMembers|error
Add member to segment
Parameters
- listId string - The unique ID for the list.
- segmentId string - The unique id for the segment.
- payload SegmentIdMembersBody - Add member to segment input payload
Return Type
- ListMembers|error - Individuals who are currently or have been previously subscribed to this list, including members who have bounced or unsubscribed.
deleteListsIdSegmentsIdMembersId
function deleteListsIdSegmentsIdMembersId(string listId, string segmentId, string subscriberHash) returns Response|error
Remove list member from segment
Parameters
- listId string - The unique ID for the list.
- segmentId string - The unique id for the segment.
- subscriberHash string - The MD5 hash of the lowercase version of the list member's email address.
searchTagsByName
function searchTagsByName(string listId, string? name) returns TagSearchResults|error
Search for tags on a list by name.
Parameters
- listId string - The unique ID for the list.
- name string? (default ()) - The search query used to filter tags. The search query will be compared to each tag as a prefix, so all tags that have a name starting with this field will be returned.
Return Type
- TagSearchResults|error - A list of tags matching the input query
getListsIdMembers
function getListsIdMembers(string listId, string[]? fields, string[]? excludeFields, int count, int offset, string? emailType, string? status, string? sinceTimestampOpt, string? beforeTimestampOpt, string? sinceLastChanged, string? beforeLastChanged, string? uniqueEmailId, boolean? vipOnly, string? interestCategoryId, string? interestIds, string? interestMatch, string? sortField, string? sortDir, boolean? sinceLastCampaign, string? unsubscribedSince) returns ListMembers1|error
List members info
Parameters
- listId string - The unique ID for the list.
- fields string[]? (default ()) - A comma-separated list of fields to return. Reference parameters of sub-objects with dot notation.
- excludeFields string[]? (default ()) - A comma-separated list of fields to exclude. Reference parameters of sub-objects with dot notation.
- count int (default 10) - The number of records to return. Default value is 10. Maximum value is 1000
- offset int (default 0) - Used for pagination, this it the number of records from a collection to skip. Default value is 0.
- emailType string? (default ()) - The email type.
- status string? (default ()) - The subscriber's status.
- sinceTimestampOpt string? (default ()) - Restrict results to subscribers who opted-in after the set timeframe. Uses ISO 8601 time format: 2015-10-21T15:41:36+00:00.
- beforeTimestampOpt string? (default ()) - Restrict results to subscribers who opted-in before the set timeframe. Uses ISO 8601 time format: 2015-10-21T15:41:36+00:00.
- sinceLastChanged string? (default ()) - Restrict results to subscribers whose information changed after the set timeframe. Uses ISO 8601 time format: 2015-10-21T15:41:36+00:00.
- beforeLastChanged string? (default ()) - Restrict results to subscribers whose information changed before the set timeframe. Uses ISO 8601 time format: 2015-10-21T15:41:36+00:00.
- uniqueEmailId string? (default ()) - A unique identifier for the email address across all Mailchimp lists.
- vipOnly boolean? (default ()) - A filter to return only the list's VIP members. Passing
true
will restrict results to VIP list members, passingfalse
will return all list members.
- interestCategoryId string? (default ()) - The unique id for the interest category.
- interestIds string? (default ()) - Used to filter list members by interests. Must be accompanied by interest_category_id and interest_match. The value must be a comma separated list of interest ids present for any supplied interest categories.
- interestMatch string? (default ()) - Used to filter list members by interests. Must be accompanied by interest_category_id and interest_ids. "any" will match a member with any of the interest supplied, "all" will only match members with every interest supplied, and "none" will match members without any of the interest supplied.
- sortField string? (default ()) - Returns files sorted by the specified field.
- sortDir string? (default ()) - Determines the order direction for sorted results.
- sinceLastCampaign boolean? (default ()) - Filter subscribers by those subscribed/unsubscribed/pending/cleaned since last email campaign send. Member status is required to use this filter.
- unsubscribedSince string? (default ()) - Filter subscribers by those unsubscribed since a specific date. Using any status other than unsubscribed with this filter will result in an error.
Return Type
- ListMembers1|error - Manage members of a specific Mailchimp list, including currently subscribed, unsubscribed, and bounced members
postListsIdMembers
function postListsIdMembers(string listId, AddListMembers1 payload, boolean? skipMergeValidation) returns ListMembers2|error
Add member to list
Parameters
- listId string - The unique ID for the list.
- payload AddListMembers1 - Add members to list input payload
- skipMergeValidation boolean? (default ()) - If skip_merge_validation is true, member data will be accepted without merge field values, even if the merge field is usually required. This defaults to false.
Return Type
- ListMembers2|error - Individuals who are currently or have been previously subscribed to this list, including members who have bounced or unsubscribed
getListsIdMembersId
function getListsIdMembersId(string listId, string subscriberHash, string[]? fields, string[]? excludeFields) returns ListMembers2|error
Get member info
Parameters
- listId string - The unique ID for the list.
- subscriberHash string - The MD5 hash of the lowercase version of the list member's email address.
- fields string[]? (default ()) - A comma-separated list of fields to return. Reference parameters of sub-objects with dot notation.
- excludeFields string[]? (default ()) - A comma-separated list of fields to exclude. Reference parameters of sub-objects with dot notation.
Return Type
- ListMembers2|error - Individuals who are currently or have been previously subscribed to this list, including members who have bounced or unsubscribed
putListsIdMembersId
function putListsIdMembersId(string listId, string subscriberHash, AddListMembers2 payload, boolean? skipMergeValidation) returns ListMembers2|error
Add or update list member
Parameters
- listId string - The unique ID for the list.
- subscriberHash string - The MD5 hash of the lowercase version of the list member's email address.
- payload AddListMembers2 - Add or update list member input payload
- skipMergeValidation boolean? (default ()) - If skip_merge_validation is true, member data will be accepted without merge field values, even if the merge field is usually required. This defaults to false.
Return Type
- ListMembers2|error - Individuals who are currently or have been previously subscribed to this list, including members who have bounced or unsubscribed
deleteListsIdMembersId
Archive list member
Parameters
- listId string - The unique ID for the list.
- subscriberHash string - The MD5 hash of the lowercase version of the list member's email address.
patchListsIdMembersId
function patchListsIdMembersId(string listId, string subscriberHash, AddListMembers3 payload, boolean? skipMergeValidation) returns ListMembers2|error
Update list member
Parameters
- listId string - The unique ID for the list.
- subscriberHash string - The MD5 hash of the lowercase version of the list member's email address.
- payload AddListMembers3 - Update list member input payload
- skipMergeValidation boolean? (default ()) - If skip_merge_validation is true, member data will be accepted without merge field values, even if the merge field is usually required. This defaults to false.
Return Type
- ListMembers2|error - Individuals who are currently or have been previously subscribed to this list, including members who have bounced or unsubscribed
getListsIdMembersIdActivity
function getListsIdMembersIdActivity(string listId, string subscriberHash, string[]? fields, string[]? excludeFields, string[]? action) returns MemberActivityEvents|error
View recent activity 50
Parameters
- listId string - The unique ID for the list.
- subscriberHash string - The MD5 hash of the lowercase version of the list member's email address.
- fields string[]? (default ()) - A comma-separated list of fields to return. Reference parameters of sub-objects with dot notation.
- excludeFields string[]? (default ()) - A comma-separated list of fields to exclude. Reference parameters of sub-objects with dot notation.
- action string[]? (default ()) - A comma seperated list of actions to return.
Return Type
- MemberActivityEvents|error - The last 50 member events for a list
getListsIdMembersIdActivityFeed
function getListsIdMembersIdActivityFeed(string listId, string subscriberHash, string[]? fields, string[]? excludeFields, int count, int offset, string[]? activityFilters) returns MemberActivityEvents1|error
View recent activity
Parameters
- listId string - The unique ID for the list.
- subscriberHash string - The MD5 hash of the lowercase version of the list member's email address.
- fields string[]? (default ()) - A comma-separated list of fields to return. Reference parameters of sub-objects with dot notation.
- excludeFields string[]? (default ()) - A comma-separated list of fields to exclude. Reference parameters of sub-objects with dot notation.
- count int (default 10) - The number of records to return. Default value is 10. Maximum value is 1000
- offset int (default 0) - Used for pagination, this it the number of records from a collection to skip. Default value is 0.
- activityFilters string[]? (default ()) - A comma-separated list of activity filters that correspond to a set of activity types, e.g "?activity_filters=open,bounce,click".
Return Type
- MemberActivityEvents1|error - The member activity events for a given member
getListMemberTags
function getListMemberTags(string listId, string subscriberHash, string[]? fields, string[]? excludeFields, int count, int offset) returns CollectionOfTags|error
List member tags
Parameters
- listId string - The unique ID for the list.
- subscriberHash string - The MD5 hash of the lowercase version of the list member's email address.
- fields string[]? (default ()) - A comma-separated list of fields to return. Reference parameters of sub-objects with dot notation.
- excludeFields string[]? (default ()) - A comma-separated list of fields to exclude. Reference parameters of sub-objects with dot notation.
- count int (default 10) - The number of records to return. Default value is 10. Maximum value is 1000
- offset int (default 0) - Used for pagination, this it the number of records from a collection to skip. Default value is 0.
Return Type
- CollectionOfTags|error - A list of tags assigned to a list member
postListMemberTags
function postListMemberTags(string listId, string subscriberHash, MemberTags payload) returns Response|error
Add or remove member tags
Parameters
- listId string - The unique ID for the list.
- subscriberHash string - The MD5 hash of the lowercase version of the list member's email address.
- payload MemberTags - Add or remove member tags input payload
getListsIdMembersIdEvents
function getListsIdMembersIdEvents(string listId, string subscriberHash, int count, int offset, string[]? fields, string[]? excludeFields) returns CollectionOfEvents|error
List member events
Parameters
- listId string - The unique ID for the list.
- subscriberHash string - The MD5 hash of the lowercase version of the list member's email address. This endpoint also accepts email addresses.
- count int (default 10) - The number of records to return. Default value is 10. Maximum value is 1000
- offset int (default 0) - Used for pagination, this it the number of records from a collection to skip. Default value is 0.
- fields string[]? (default ()) - A comma-separated list of fields to return. Reference parameters of sub-objects with dot notation.
- excludeFields string[]? (default ()) - A comma-separated list of fields to exclude. Reference parameters of sub-objects with dot notation.
Return Type
- CollectionOfEvents|error - A collection of events for a given contact
postListMemberEvents
function postListMemberEvents(string listId, string subscriberHash, Events payload) returns Response|error
Add event
Parameters
- listId string - The unique ID for the list.
- subscriberHash string - The MD5 hash of the lowercase version of the list member's email address. This endpoint also accepts email addresses.
- payload Events - Add event input payload
getListsIdMembersIdGoals
function getListsIdMembersIdGoals(string listId, string subscriberHash, string[]? fields, string[]? excludeFields) returns CollectionOfMemberActivityEvents|error
List member goal events
Parameters
- listId string - The unique ID for the list.
- subscriberHash string - The MD5 hash of the lowercase version of the list member's email address.
- fields string[]? (default ()) - A comma-separated list of fields to return. Reference parameters of sub-objects with dot notation.
- excludeFields string[]? (default ()) - A comma-separated list of fields to exclude. Reference parameters of sub-objects with dot notation.
Return Type
- CollectionOfMemberActivityEvents|error - The last 50 Goal events for a member on a specific list
getListsIdMembersIdNotes
function getListsIdMembersIdNotes(string listId, string subscriberHash, string? sortField, string? sortDir, string[]? fields, string[]? excludeFields, int count, int offset) returns CollectionOfNotes|error
List recent member notes
Parameters
- listId string - The unique ID for the list.
- subscriberHash string - The MD5 hash of the lowercase version of the list member's email address.
- sortField string? (default ()) - Returns notes sorted by the specified field.
- sortDir string? (default ()) - Determines the order direction for sorted results.
- fields string[]? (default ()) - A comma-separated list of fields to return. Reference parameters of sub-objects with dot notation.
- excludeFields string[]? (default ()) - A comma-separated list of fields to exclude. Reference parameters of sub-objects with dot notation.
- count int (default 10) - The number of records to return. Default value is 10. Maximum value is 1000
- offset int (default 0) - Used for pagination, this it the number of records from a collection to skip. Default value is 0.
Return Type
- CollectionOfNotes|error - The last 10 notes for a specific list member, based on date created
postListsIdMembersIdNotes
function postListsIdMembersIdNotes(string listId, string subscriberHash, MemberNotes payload) returns MemberNotes1|error
Add member note
Parameters
- listId string - The unique ID for the list.
- subscriberHash string - The MD5 hash of the lowercase version of the list member's email address.
- payload MemberNotes - Add member note input payload
Return Type
- MemberNotes1|error - A specific note for a specific member
getListsIdMembersIdNotesId
function getListsIdMembersIdNotesId(string listId, string subscriberHash, string noteId, string[]? fields, string[]? excludeFields) returns MemberNotes1|error
Get member note
Parameters
- listId string - The unique ID for the list.
- subscriberHash string - The MD5 hash of the lowercase version of the list member's email address.
- noteId string - The id for the note.
- fields string[]? (default ()) - A comma-separated list of fields to return. Reference parameters of sub-objects with dot notation.
- excludeFields string[]? (default ()) - A comma-separated list of fields to exclude. Reference parameters of sub-objects with dot notation.
Return Type
- MemberNotes1|error - A specific note for a specific member
deleteListsIdMembersIdNotesId
function deleteListsIdMembersIdNotesId(string listId, string subscriberHash, string noteId) returns Response|error
Delete note
Parameters
- listId string - The unique ID for the list.
- subscriberHash string - The MD5 hash of the lowercase version of the list member's email address.
- noteId string - The id for the note.
patchListsIdMembersIdNotesId
function patchListsIdMembersIdNotesId(string listId, string subscriberHash, string noteId, MemberNotes2 payload) returns MemberNotes1|error
Update note
Parameters
- listId string - The unique ID for the list.
- subscriberHash string - The MD5 hash of the lowercase version of the list member's email address.
- noteId string - The id for the note.
- payload MemberNotes2 - Update note input payload
Return Type
- MemberNotes1|error - A specific note for a specific member
postListsIdMembersHashActionsDeletePermanent
function postListsIdMembersHashActionsDeletePermanent(string listId, string subscriberHash) returns Response|error
Delete list member
Parameters
- listId string - The unique ID for the list.
- subscriberHash string - The MD5 hash of the lowercase version of the list member's email address.
getListsIdMergeFields
function getListsIdMergeFields(string listId, string[]? fields, string[]? excludeFields, int count, int offset, string? 'type, boolean? required) returns CollectionOfMergeFields|error
List merge fields
Parameters
- listId string - The unique ID for the list.
- fields string[]? (default ()) - A comma-separated list of fields to return. Reference parameters of sub-objects with dot notation.
- excludeFields string[]? (default ()) - A comma-separated list of fields to exclude. Reference parameters of sub-objects with dot notation.
- count int (default 10) - The number of records to return. Default value is 10. Maximum value is 1000
- offset int (default 0) - Used for pagination, this it the number of records from a collection to skip. Default value is 0.
- 'type string? (default ()) - The merge field type.
- required boolean? (default ()) - The boolean value if the merge field is required.
Return Type
- CollectionOfMergeFields|error - The merge field (formerly merge vars) for a list. These correspond to merge fields in Mailchimp's lists and subscriber profiles
postListsIdMergeFields
function postListsIdMergeFields(string listId, MergeField payload) returns MergeField1|error
Add merge field
Parameters
- listId string - The unique ID for the list.
- payload MergeField - Add merge field input payload
Return Type
- MergeField1|error - A merge field (formerly merge vars) for a specific list. These correspond to merge fields in Mailchimp's lists and subscriber profiles
getListsIdMergeFieldsId
function getListsIdMergeFieldsId(string listId, string mergeId, string[]? excludeFields, string[]? fields) returns MergeField1|error
Get merge field
Parameters
- listId string - The unique ID for the list.
- mergeId string - The id for the merge field.
- excludeFields string[]? (default ()) - A comma-separated list of fields to exclude. Reference parameters of sub-objects with dot notation.
- fields string[]? (default ()) - A comma-separated list of fields to return. Reference parameters of sub-objects with dot notation.
Return Type
- MergeField1|error - A merge field (formerly merge vars) for a specific list. These correspond to merge fields in Mailchimp's lists and subscriber profiles
deleteListsIdMergeFieldsId
Delete merge field
patchListsIdMergeFieldsId
function patchListsIdMergeFieldsId(string listId, string mergeId, MergeField2 payload) returns MergeField1|error
Update merge field
Parameters
- listId string - The unique ID for the list.
- mergeId string - The id for the merge field.
- payload MergeField2 - Update merge field input payload
Return Type
- MergeField1|error - A merge field (formerly merge vars) for a specific list. These correspond to merge fields in Mailchimp's lists and subscriber profiles
getListsIdWebhooks
function getListsIdWebhooks(string listId) returns ListWebhooks|error
List webhooks
Parameters
- listId string - The unique ID for the list.
Return Type
- ListWebhooks|error - Manage webhooks for a specific list
postListsIdWebhooks
function postListsIdWebhooks(string listId, AddWebhook payload) returns ListWebhooks1|error
Add webhook
Return Type
- ListWebhooks1|error - Webhook configured for the given list
getListsIdWebhooksId
function getListsIdWebhooksId(string listId, string webhookId) returns ListWebhooks1|error
Get webhook info
Return Type
- ListWebhooks1|error - Webhook configured for the given list
deleteListsIdWebhooksId
Delete webhook
patchListsIdWebhooksId
function patchListsIdWebhooksId(string listId, string webhookId, AddWebhook1 payload) returns ListWebhooks1|error
Update webhook
Parameters
- listId string - The unique ID for the list.
- webhookId string - The webhook's id.
- payload AddWebhook1 - Update webhook input payload
Return Type
- ListWebhooks1|error - Webhook configured for the given list
getListsIdSignupForms
function getListsIdSignupForms(string listId) returns ListSignupForms|error
List signup forms
Parameters
- listId string - The unique ID for the list.
Return Type
- ListSignupForms|error - List Signup Forms.
postListsIdSignupForms
function postListsIdSignupForms(string listId, SignupForm payload) returns SignupForm1|error
Customize signup form
Parameters
- listId string - The unique ID for the list.
- payload SignupForm - Customize signup form input payload
Return Type
- SignupForm1|error - List Signup Forms.
getListsIdLocations
function getListsIdLocations(string listId, string[]? fields, string[]? excludeFields) returns ListLocations|error
List locations
Parameters
- listId string - The unique ID for the list.
- fields string[]? (default ()) - A comma-separated list of fields to return. Reference parameters of sub-objects with dot notation.
- excludeFields string[]? (default ()) - A comma-separated list of fields to exclude. Reference parameters of sub-objects with dot notation.
Return Type
- ListLocations|error - A summary of List's locations
getSearchMembers
function getSearchMembers(string query, string[]? fields, string[]? excludeFields, string? listId) returns Members|error
Search members
Parameters
- query string - The search query used to filter results. Query should be a valid email, or a string representing a contact's first or last name.
- fields string[]? (default ()) - A comma-separated list of fields to return. Reference parameters of sub-objects with dot notation.
- excludeFields string[]? (default ()) - A comma-separated list of fields to exclude. Reference parameters of sub-objects with dot notation.
- listId string? (default ()) - The unique id for the list.
getVerifiedDomain
function getVerifiedDomain(string domainName) returns VerifiedDomains|error
Get domain info
Parameters
- domainName string - The domain name.
Return Type
- VerifiedDomains|error - The domains on the account
deleteVerifiedDomain
Delete domain
Parameters
- domainName string - The domain name.
verifyDomain
function verifyDomain(string domainName, ActionsVerifyBody payload) returns VerifiedDomains|error
Verify domain
Parameters
- domainName string - The domain name.
- payload ActionsVerifyBody - Verify domain input payload
Return Type
- VerifiedDomains|error - The domain being verified for sending.
getVerifiedDomains
function getVerifiedDomains() returns VerifiedDomains1|error
List sending domains
Return Type
- VerifiedDomains1|error - The domains on the account.
createVerifiedDomain
function createVerifiedDomain(VerifiedDomains2 payload) returns VerifiedDomains|error
Add domain to account
Parameters
- payload VerifiedDomains2 - Add domain to account input payload
Return Type
- VerifiedDomains|error - The newly-created domain.
Records
mailchimp: AbTestingOptions
A/B Testing options for a campaign.
Fields
- split_test string? - The type of AB split to run.
- pick_winner string? - How we should evaluate a winner. Based on 'opens', 'clicks', or 'manual'.
- wait_units string? - How unit of time for measuring the winner ('hours' or 'days'). This cannot be changed after a campaign is sent.
- wait_time int? - The amount of time to wait before picking a winner. This cannot be changed after a campaign is sent.
- split_size int? - The size of the split groups. Campaigns split based on 'schedule' are forced to have a 50/50 split. Valid split integers are between 1-50.
- from_name_a string? - For campaigns split on 'From Name', the name for Group A.
- from_name_b string? - For campaigns split on 'From Name', the name for Group B.
- reply_email_a string? - For campaigns split on 'From Name', the reply-to address for Group A.
- reply_email_b string? - For campaigns split on 'From Name', the reply-to address for Group B.
- subject_a string? - For campaigns split on 'Subject Line', the subject line for Group A.
- subject_b string? - For campaigns split on 'Subject Line', the subject line for Group B.
- send_time_a string? - The send time for Group A.
- send_time_b string? - The send time for Group B.
- send_time_winner string? - The send time for the winning version.
mailchimp: AbTestOptions
The settings specific to A/B test campaigns.
Fields
- winner_criteria string - The combination that performs the best. This may be determined automatically by click rate, open rate, or total revenue -- or you may choose manually based on the reporting data you find the most valuable. For Multivariate Campaigns testing send_time, winner_criteria is ignored. For Multivariate Campaigns with 'manual' as the winner_criteria, the winner must be chosen in the Mailchimp web application.
- wait_time int? - The number of minutes to wait before choosing the winning campaign. The value of wait_time must be greater than 0 and in whole hours, specified in minutes.
- test_size int? - The percentage of recipients to send the test combinations to, must be a value between 10 and 100.
- subject_lines string[]? - The possible subject lines to test. If no subject lines are provided, settings.subject_line will be used.
- send_times string[]? - The possible send times to test. The times provided should be in the format YYYY-MM-DD HH:MM:SS. If send_times are provided to test, the test_size will be set to 100% and winner_criteria will be ignored.
- from_names string[]? - The possible from names. The number of from_names provided must match the number of reply_to_addresses. If no from_names are provided, settings.from_name will be used.
- reply_to_addresses string[]? - The possible reply-to addresses. The number of reply_to_addresses provided must match the number of from_names. If no reply_to_addresses are provided, settings.reply_to will be used.
mailchimp: AbTestOptions1
The settings specific to A/B test campaigns.
Fields
- winning_combination_id string? - ID for the winning combination.
- winning_campaign_id string? - ID of the campaign that was sent to the remaining recipients based on the winning combination.
- winner_criteria string? - The combination that performs the best. This may be determined automatically by click rate, open rate, or total revenue -- or you may choose manually based on the reporting data you find the most valuable. For Multivariate Campaigns testing send_time, winner_criteria is ignored. For Multivariate Campaigns with 'manual' as the winner_criteria, the winner must be chosen in the Mailchimp web application.
- wait_time int? - The number of minutes to wait before choosing the winning campaign. The value of wait_time must be greater than 0 and in whole hours, specified in minutes.
- test_size int? - The percentage of recipients to send the test combinations to, must be a value between 10 and 100.
- subject_lines string[]? - The possible subject lines to test. If no subject lines are provided, settings.subject_line will be used.
- send_times string[]? - The possible send times to test. The times provided should be in the format YYYY-MM-DD HH:MM:SS. If send_times are provided to test, the test_size will be set to 100% and winner_criteria will be ignored.
- from_names string[]? - The possible from names. The number of from_names provided must match the number of reply_to_addresses. If no from_names are provided, settings.from_name will be used.
- reply_to_addresses string[]? - The possible reply-to addresses. The number of reply_to_addresses provided must match the number of from_names. If no reply_to_addresses are provided, settings.reply_to will be used.
- contents string[]? - Descriptions of possible email contents. To set campaign contents, make a PUT request to /campaigns/{campaign_id}/content with the field 'variate_contents'.
- combinations AbTestOptions1Combinations[]? - Combinations of possible variables used to build emails.
mailchimp: AbTestOptions1Combinations
Fields
- id string? - Unique ID for the combination.
- subject_line int? - The index of
variate_settings.subject_lines
used.
- send_time int? - The index of
variate_settings.send_times
used.
- from_name int? - The index of
variate_settings.from_names
used.
- reply_to int? - The index of
variate_settings.reply_to_addresses
used.
- content_description int? - The index of
variate_settings.contents
used.
- recipients int? - The number of recipients for this combination.
mailchimp: AbTestOptions2
The settings specific to A/B test campaigns.
Fields
- winning_combination_id string? - ID for the winning combination.
- winning_campaign_id string? - ID of the campaign that was sent to the remaining recipients based on the winning combination.
- winner_criteria string - The combination that performs the best. This may be determined automatically by click rate, open rate, or total revenue -- or you may choose manually based on the reporting data you find the most valuable. For Multivariate Campaigns testing send_time, winner_criteria is ignored. For Multivariate Campaigns with 'manual' as the winner_criteria, the winner must be chosen in the Mailchimp web application.
- wait_time int? - The number of minutes to wait before choosing the winning campaign. The value of wait_time must be greater than 0 and in whole hours, specified in minutes.
- test_size int? - The percentage of recipients to send the test combinations to, must be a value between 10 and 100.
- subject_lines string[]? - The possible subject lines to test. If no subject lines are provided, settings.subject_line will be used.
- send_times string[]? - The possible send times to test. The times provided should be in the format YYYY-MM-DD HH:MM:SS. If send_times are provided to test, the test_size will be set to 100% and winner_criteria will be ignored.
- from_names string[]? - The possible from names. The number of from_names provided must match the number of reply_to_addresses. If no from_names are provided, settings.from_name will be used.
- reply_to_addresses string[]? - The possible reply-to addresses. The number of reply_to_addresses provided must match the number of from_names. If no reply_to_addresses are provided, settings.reply_to will be used.
- contents string[]? - Descriptions of possible email contents. To set campaign contents, make a PUT request to /campaigns/{campaign_id}/content with the field 'variate_contents'.
- combinations AbTestOptions1Combinations[]? - Combinations of possible variables used to build emails.
mailchimp: AbuseComplaint
Details of abuse complaints for a specific list. An abuse complaint occurs when your recipient clicks to 'report spam' in their email program.
Fields
- id int? - The id for the abuse report
- campaign_id string? - The campaign id for the abuse report
- list_id string? - The list id for the abuse report.
- email_id string? - The MD5 hash of the lowercase version of the list member's email address.
- email_address string? - Email address for a subscriber.
- merge_fields record {}? - An individual merge var and value for a member.
- vip boolean? - VIP status for subscriber.
- date string? - Date for the abuse report
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: AbuseComplaint1
Details of abuse complaints for a specific list. An abuse complaint occurs when your recipient clicks to 'report spam' in their email program.
Fields
- id int? - The id for the abuse report
- campaign_id string? - The campaign id for the abuse report
- list_id string? - The list id for the abuse report.
- email_id string? - The MD5 hash of the lowercase version of the list member's email address.
- email_address string? - Email address for a subscriber.
- merge_fields record {}? - An individual merge var and value for a member.
- vip boolean? - VIP status for subscriber.
- date string? - Date for the abuse report
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: AbuseComplaints
A collection of abuse complaints for a specific list. An abuse complaint occurs when your recipient clicks to 'report spam' in their email program.
Fields
- abuse_reports AbuseComplaint1[]? - An array of objects, each representing an abuse report resource.
- list_id string? - The list id for the abuse report.
- total_items int? - The total number of items matching the query regardless of pagination.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: AccountContact
Information about the account contact.
Fields
- company string? - The company name for the account.
- addr1 string? - The street address for the account contact.
- addr2 string? - The street address for the account contact.
- city string? - The city for the account contact.
- state string? - The state for the account contact.
- zip string? - The zip code for the account contact.
- country string? - The country for the account contact.
mailchimp: ActionsScheduleBody
Fields
- schedule_time string - The UTC date and time to schedule the campaign for delivery in ISO 8601 format. Campaigns may only be scheduled to send on the quarter-hour (:00, :15, :30, :45).
- timewarp boolean? - Choose whether the campaign should use Timewarp when sending. Campaigns scheduled with Timewarp are localized based on the recipients' time zones. For example, a Timewarp campaign with a
schedule_time
of 13:00 will be sent to each recipient at 1:00pm in their local time. Cannot be set totrue
for campaigns using Batch Delivery.
- batch_delivery BatchDelivery? - Choose whether the campaign should use Batch Delivery. Cannot be set to
true
for campaigns using Timewarp.
mailchimp: ActionsTestBody
Fields
- test_emails string[] - An array of email addresses to send the test email to.
- send_type string - Choose the type of test email to send.
mailchimp: ActionsVerifyBody
Submit a response to the verification challenge and verify a domain for sending.
Fields
- code string - The code that was sent to the email address provided when adding a new domain to verify.
mailchimp: AddListMembers
Individuals who are currently or have been previously subscribed to this list, including members who have bounced or unsubscribed.
Fields
- email_address string? - Email address for a subscriber.
- email_type string? - Type of email this member asked to get ('html' or 'text').
- status string? - Subscriber's current status.
- merge_fields record {}? - An individual merge var and value for a member.
- interests record {}? - The key of this object's properties is the ID of the interest in question.
- language string? - If set/detected, the subscriber's language.
- vip boolean? - VIP status for subscriber.
- location Location? - Subscriber location information.
- ip_signup string? - IP address the subscriber signed up from.
- timestamp_signup string? - The date and time the subscriber signed up for the list in ISO 8601 format.
- ip_opt string? - The IP address the subscriber used to confirm their opt-in status.
- timestamp_opt string? - The date and time the subscribe confirmed their opt-in status in ISO 8601 format.
mailchimp: AddListMembers1
Individuals who are currently or have been previously subscribed to this list, including members who have bounced or unsubscribed.
Fields
- email_address string - Email address for a subscriber.
- email_type string? - Type of email this member asked to get ('html' or 'text').
- status string - Subscriber's current status.
- merge_fields record {}? - An individual merge var and value for a member.
- interests record {}? - The key of this object's properties is the ID of the interest in question.
- language string? - If set/detected, the subscriber's language.
- vip boolean? - VIP status for subscriber.
- location Location? - Subscriber location information.
- marketing_permissions MarketingPermission[]? - The marketing permissions for the subscriber.
- ip_signup string? - IP address the subscriber signed up from.
- timestamp_signup string? - The date and time the subscriber signed up for the list in ISO 8601 format.
- ip_opt string? - The IP address the subscriber used to confirm their opt-in status.
- timestamp_opt string? - The date and time the subscribe confirmed their opt-in status in ISO 8601 format.
- tags string[]? - The tags that are associated with a member.
mailchimp: AddListMembers2
Individuals who are currently or have been previously subscribed to this list, including members who have bounced or unsubscribed.
Fields
- email_address string - Email address for a subscriber. This value is required only if the email address is not already present on the list.
- status_if_new string - Subscriber's status. This value is required only if the email address is not already present on the list.
- email_type string? - Type of email this member asked to get ('html' or 'text').
- status string? - Subscriber's current status.
- merge_fields record {}? - An individual merge var and value for a member.
- interests record {}? - The key of this object's properties is the ID of the interest in question.
- language string? - If set/detected, the subscriber's language.
- vip boolean? - VIP status for subscriber.
- location Location? - Subscriber location information.
- marketing_permissions MarketingPermission[]? - The marketing permissions for the subscriber.
- ip_signup string? - IP address the subscriber signed up from.
- timestamp_signup string? - The date and time the subscriber signed up for the list in ISO 8601 format.
- ip_opt string? - The IP address the subscriber used to confirm their opt-in status.
- timestamp_opt string? - The date and time the subscriber confirmed their opt-in status in ISO 8601 format.
mailchimp: AddListMembers3
Individuals who are currently or have been previously subscribed to this list, including members who have bounced or unsubscribed.
Fields
- email_address string? - Email address for a subscriber.
- email_type string? - Type of email this member asked to get ('html' or 'text').
- status string? - Subscriber's current status.
- merge_fields record {}? - An individual merge var and value for a member.
- interests record {}? - The key of this object's properties is the ID of the interest in question.
- language string? - If set/detected, the subscriber's language.
- vip boolean? - VIP status for subscriber.
- location Location? - Subscriber location information.
- marketing_permissions MarketingPermission[]? - The marketing permissions for the subscriber.
- ip_signup string? - IP address the subscriber signed up from.
- timestamp_signup string? - The date and time the subscriber signed up for the list in ISO 8601 format.
- ip_opt string? - The IP address the subscriber used to confirm their opt-in status.
- timestamp_opt string? - The date and time the subscriber confirmed their opt-in status in ISO 8601 format.
mailchimp: AddWebhook
Configure a webhook for the given list.
Fields
- url string? - A valid URL for the Webhook.
- events Events1? - The events that can trigger the webhook and whether they are enabled.
- sources Sources? - The possible sources of any events that can trigger the webhook and whether they are enabled.
mailchimp: AddWebhook1
Configure a webhook for the given list.
Fields
- url string? - A valid URL for the Webhook.
- events Events1? - The events that can trigger the webhook and whether they are enabled.
- sources Sources? - The possible sources of any events that can trigger the webhook and whether they are enabled.
mailchimp: AnOptionForSignupFormStyles
An option for Signup Form Styles.
Fields
- property string? - A string that identifies the property.
- value string? - A string that identifies value of the property.
mailchimp: ApiRoot
The API root resource links to all other resources available in the API.
Fields
- account_id string? - The Mailchimp account ID.
- login_id string? - The ID associated with the user who owns this API key. If you can login to multiple accounts, this ID will be the same for each account.
- account_name string? - The name of the account.
- email string? - The account email address.
- first_name string? - The first name tied to the account.
- last_name string? - The last name tied to the account.
- username string? - The username tied to the account.
- avatar_url string? - URL of the avatar for the user.
- member_since string? - The date and time that the account was created in ISO 8601 format.
- pricing_plan_type string? - The type of pricing plan the account is on.
- first_payment string? - Date of first payment for monthly plans.
- account_timezone string? - The timezone currently set for the account.
- account_industry string? - The user-specified industry associated with the account.
- contact AccountContact? - Information about the account contact.
- pro_enabled boolean? - Legacy - whether the account includes Mailchimp Pro.
- last_login string? - The date and time of the last login for this account in ISO 8601 format.
- total_subscribers int? - The total number of subscribers across all lists in the account.
- industry_stats IndustryStats? - The average campaign statistics for all campaigns in the account's specified industry.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: AutomationCampaignSettings
The settings for the Automation workflow.
Fields
- from_name string? - The 'from' name for the Automation (not an email address).
- reply_to string? - The reply-to email address for the Automation.
mailchimp: AutomationCampaignSettings1
The settings for the Automation workflow.
Fields
- title string? - The title of the Automation.
- from_name string? - The 'from' name for the Automation (not an email address).
- reply_to string? - The reply-to email address for the Automation.
- use_conversation boolean? - Whether to use Mailchimp Conversation feature to manage replies
- to_name string? - The Automation's custom 'To' name, typically the first name merge field.
- authenticate boolean? - Whether Mailchimp authenticated the Automation. Defaults to
true
.
- auto_footer boolean? - Whether to automatically append Mailchimp's default footer to the Automation.
- inline_css boolean? - Whether to automatically inline the CSS included with the Automation content.
mailchimp: AutomationDelay
The delay settings for an automation email.
Fields
- amount int? - The delay amount for an automation email.
- 'type string? - The type of delay for an automation email.
- direction string? - Whether the delay settings describe before or after the delay action of an automation email.
- action string - The action that triggers the delay of an automation emails.
mailchimp: AutomationDelay1
The delay settings for an Automation email.
Fields
- amount int? - The delay amount for an Automation email.
- 'type string? - The type of delay for an Automation email.
- direction string? - Whether the delay settings describe before or after the delay action of an Automation email.
- action string? - The action that triggers the delay of an Automation email.
- action_description string? - The user-friendly description of the action that triggers an Automation email.
- full_description string? - The user-friendly description of the delay and trigger action settings for an Automation email.
mailchimp: AutomationEmails
A summary of the emails in an Automation workflow.
Fields
- emails AutomationWorkflowEmail1[]? - An array of objects, each representing an email in an Automation workflow.
- total_items int? - The total number of items matching the query regardless of pagination.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: AutomationTrackingOptions
The tracking options for the Automation.
Fields
- opens boolean? - Whether to track opens. Defaults to
true
.
- html_clicks boolean? - Whether to track clicks in the HTML version of the Automation. Defaults to
true
.
- text_clicks boolean? - Whether to track clicks in the plain-text version of the Automation. Defaults to
true
.
- goal_tracking boolean? - Deprecated
- ecomm360 boolean? - Whether to enable e-commerce tracking.
- google_analytics string? - The custom slug for Google Analytics tracking (max of 50 bytes).
- salesforce SalesforceCrmTracking1? - Deprecated
- capsule CapsuleCrmTracking1? - Deprecated
mailchimp: AutomationTrigger
Trigger settings for the Automation.
Fields
- workflow_type string - The type of Automation workflow. Currently only supports 'abandonedCart'.
mailchimp: AutomationTrigger1
Available triggers for Automation workflows.
Fields
- workflow_type string - The type of Automation workflow.
- workflow_title string? - The title of the workflow type.
- runtime AutomationWorkflowRuntimeSettings? - A workflow's runtime settings for an Automation.
- workflow_emails_count int? - The number of emails in the Automation workflow.
mailchimp: AutomationWorkflow
A summary of an individual Automation workflow's settings and content.
Fields
- recipients List - List settings for the Automation.
- settings AutomationCampaignSettings? - The settings for the Automation workflow.
- trigger_settings AutomationTrigger - Trigger settings for the Automation.
mailchimp: AutomationWorkflow1
A summary of an individual Automation workflow's settings and content.
Fields
- id string? - A string that identifies the Automation.
- create_time string? - The date and time the Automation was created in ISO 8601 format.
- start_time string? - The date and time the Automation was started in ISO 8601 format.
- status string? - The current status of the Automation.
- emails_sent int? - The total number of emails sent for the Automation.
- recipients List6? - List settings for the Automation.
- settings AutomationCampaignSettings1? - The settings for the Automation workflow.
- tracking AutomationTrackingOptions? - The tracking options for the Automation.
- trigger_settings AutomationTrigger1? - Available triggers for Automation workflows.
- report_summary CampaignReportSummary? - A summary of opens and clicks for sent campaigns.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: AutomationWorkflow2
A summary of an individual Automation workflow's settings and content.
Fields
- id string? - A string that identifies the Automation.
- create_time string? - The date and time the Automation was created in ISO 8601 format.
- start_time string? - The date and time the Automation was started in ISO 8601 format.
- status string? - The current status of the Automation.
- emails_sent int? - The total number of emails sent for the Automation.
- recipients List6? - List settings for the Automation.
- settings AutomationCampaignSettings1? - The settings for the Automation workflow.
- tracking AutomationTrackingOptions? - The tracking options for the Automation.
- trigger_settings AutomationTrigger1? - Available triggers for Automation workflows.
- report_summary CampaignReportSummary? - A summary of opens and clicks for sent campaigns.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: AutomationWorkflowEmail
A summary of an individual Automation workflow email.
Fields
- id string? - A string that uniquely identifies the Automation email.
- web_id int? - The ID used in the Mailchimp web application. View this automation in your Mailchimp account at
https://{dc}.admin.mailchimp.com/campaigns/show/?id={web_id}
.
- workflow_id string? - A string that uniquely identifies an Automation workflow.
- position int? - The position of an Automation email in a workflow.
- delay AutomationDelay1? - The delay settings for an Automation email.
- create_time string? - The date and time the campaign was created in ISO 8601 format.
- start_time string? - The date and time the campaign was started in ISO 8601 format.
- archive_url string? - The link to the campaign's archive version in ISO 8601 format.
- status string? - The current status of the campaign.
- emails_sent int? - The total number of emails sent for this campaign.
- send_time string? - The date and time a campaign was sent in ISO 8601 format
- content_type string? - How the campaign's content is put together ('template', 'drag_and_drop', 'html', 'url').
- needs_block_refresh boolean? - Determines if the automation email needs its blocks refreshed by opening the web-based campaign editor.
- has_logo_merge_tag boolean? - Determines if the campaign contains the |BRAND:LOGO| merge tag.
- recipients List10? - List settings for the campaign.
- settings CampaignSettings5? - Settings for the campaign including the email subject, from name, and from email address.
- tracking CampaignTrackingOptions1? - The tracking options for a campaign.
- social_card CampaignSocialCard? - The preview for the campaign, rendered by social networks like Facebook and Twitter. Learn more.
- trigger_settings AutomationTrigger1? - Available triggers for Automation workflows.
- report_summary CampaignReportSummary3? - For sent campaigns, a summary of opens and clicks.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: AutomationWorkflowEmail1
A summary of an individual Automation workflow email.
Fields
- id string? - A string that uniquely identifies the Automation email.
- web_id int? - The ID used in the Mailchimp web application. View this automation in your Mailchimp account at
https://{dc}.admin.mailchimp.com/campaigns/show/?id={web_id}
.
- workflow_id string? - A string that uniquely identifies an Automation workflow.
- position int? - The position of an Automation email in a workflow.
- delay AutomationDelay1? - The delay settings for an Automation email.
- create_time string? - The date and time the campaign was created in ISO 8601 format.
- start_time string? - The date and time the campaign was started in ISO 8601 format.
- archive_url string? - The link to the campaign's archive version in ISO 8601 format.
- status string? - The current status of the campaign.
- emails_sent int? - The total number of emails sent for this campaign.
- send_time string? - The date and time a campaign was sent in ISO 8601 format
- content_type string? - How the campaign's content is put together ('template', 'drag_and_drop', 'html', 'url').
- needs_block_refresh boolean? - Determines if the automation email needs its blocks refreshed by opening the web-based campaign editor.
- has_logo_merge_tag boolean? - Determines if the campaign contains the |BRAND:LOGO| merge tag.
- recipients List10? - List settings for the campaign.
- settings CampaignSettings5? - Settings for the campaign including the email subject, from name, and from email address.
- tracking CampaignTrackingOptions1? - The tracking options for a campaign.
- social_card CampaignSocialCard? - The preview for the campaign, rendered by social networks like Facebook and Twitter. Learn more.
- trigger_settings AutomationTrigger1? - Available triggers for Automation workflows.
- report_summary CampaignReportSummary3? - For sent campaigns, a summary of opens and clicks.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: AutomationWorkflowRuntimeSettings
A workflow's runtime settings for an Automation.
Fields
- days string[]? - The days an Automation workflow can send.
- hours Hours? - The hours an Automation workflow can send.
mailchimp: BatchAddremoveListMembersTofromStaticSegment
Batch add/remove List members to/from static segment
Fields
- members_added ListMembers5[]? - An array of objects, each representing a new member that was added to the static segment.
- members_removed ListMembers5[]? - An array of objects, each representing an existing list member that got deleted from the static segment.
- errors BatchAddremoveListMembersTofromStaticSegmentErrors[]? - An array of objects, each representing an array of email addresses that could not be added to the segment or removed and an error message providing more details.
- total_added int? - The total number of items matching the query, irrespective of pagination.
- total_removed int? - The total number of items matching the query, irrespective of pagination.
- error_count int? - The total number of items matching the query, irrespective of pagination.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: BatchAddremoveListMembersTofromStaticSegmentErrors
Fields
- email_addresses string[]? - Email addresses added to the static segment or removed
- 'error string? - The error message indicating why the email addresses could not be added or updated.
mailchimp: BatchDelivery
Choose whether the campaign should use Batch Delivery. Cannot be set to true
for campaigns using Timewarp.
Fields
- batch_delay int - The delay, in minutes, between batches.
- batch_count int - The number of batches for the campaign send.
mailchimp: BatchUpdateListMembers
Batch update list members.
Fields
- new_members ListMembers5[]? - An array of objects, each representing a new member that was added to the list.
- updated_members ListMembers5[]? - An array of objects, each representing an existing list member whose subscription status was updated.
- errors BatchUpdateListMembersErrors[]? - An array of objects, each representing an email address that could not be added to the list or updated and an error message providing more details.
- total_created int? - The total number of items matching the query, irrespective of pagination.
- total_updated int? - The total number of items matching the query, irrespective of pagination.
- error_count int? - The total number of items matching the query, irrespective of pagination.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: BatchUpdateListMembersErrors
Fields
- email_address string? - The email address that could not be added or updated.
- 'error string? - The error message indicating why the email address could not be added or updated.
- error_code string? - A unique code that identifies this specifc error.
mailchimp: Campaign
A summary of an individual campaign's settings and content.
Fields
- recipients List1? - List settings for the campaign.
- settings CampaignSettings1? - The settings for your campaign, including subject, from name, reply-to address, and more.
- variate_settings AbTestOptions? - The settings specific to A/B test campaigns.
- tracking CampaignTrackingOptions? - The tracking options for a campaign.
- rss_opts RssOptions? - RSS options, specific to an RSS campaign.
- social_card CampaignSocialCard? - The preview for the campaign, rendered by social networks like Facebook and Twitter. Learn more.
- content_type string? - How the campaign's content is put together. The old drag and drop editor uses 'template' while the new editor uses 'multichannel'. Defaults to template.
mailchimp: Campaign1
A summary of an individual campaign's settings and content.
Fields
- id string? - A string that uniquely identifies this campaign.
- web_id int? - The ID used in the Mailchimp web application. View this campaign in your Mailchimp account at
https://{dc}.admin.mailchimp.com/campaigns/show/?id={web_id}
.
- parent_campaign_id string? - If this campaign is the child of another campaign, this identifies the parent campaign. For Example, for RSS or Automation children.
- create_time string? - The date and time the campaign was created in ISO 8601 format.
- archive_url string? - The link to the campaign's archive version in ISO 8601 format.
- long_archive_url string? - The original link to the campaign's archive version.
- status string? - The current status of the campaign.
- emails_sent int? - The total number of emails sent for this campaign.
- send_time string? - The date and time a campaign was sent.
- content_type string? - How the campaign's content is put together.
- needs_block_refresh boolean? - Determines if the campaign needs its blocks refreshed by opening the web-based campaign editor. Deprecated and will always return false.
- resendable boolean? - Determines if the campaign qualifies to be resent to non-openers.
- recipients List7? - List settings for the campaign.
- settings CampaignSettings3? - The settings for your campaign, including subject, from name, reply-to address, and more.
- variate_settings AbTestOptions1? - The settings specific to A/B test campaigns.
- tracking CampaignTrackingOptions? - The tracking options for a campaign.
- rss_opts RssOptions2? - RSS options for a campaign.
- ab_split_opts AbTestingOptions? - A/B Testing options for a campaign.
- social_card CampaignSocialCard? - The preview for the campaign, rendered by social networks like Facebook and Twitter. Learn more.
- report_summary CampaignReportSummary1? - For sent campaigns, a summary of opens, clicks, and e-commerce data.
- delivery_status CampaignDeliveryStatus? - Updates on campaigns in the process of sending.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: Campaign2
A summary of an individual campaign's settings and content.
Fields
- recipients List2? - List settings for the campaign.
- settings CampaignSettings2 - The settings for your campaign, including subject, from name, reply-to address, and more.
- variate_settings AbTestOptions? - The settings specific to A/B test campaigns.
- tracking CampaignTrackingOptions? - The tracking options for a campaign.
- rss_opts RssOptions1? - RSS options for a campaign.
- social_card CampaignSocialCard? - The preview for the campaign, rendered by social networks like Facebook and Twitter. Learn more.
mailchimp: Campaign3
A summary of an individual campaign's settings and content.
Fields
- id string? - A string that uniquely identifies this campaign.
- web_id int? - The ID used in the Mailchimp web application. View this campaign in your Mailchimp account at
https://{dc}.admin.mailchimp.com/campaigns/show/?id={web_id}
.
- parent_campaign_id string? - If this campaign is the child of another campaign, this identifies the parent campaign. For Example, for RSS or Automation children.
- create_time string? - The date and time the campaign was created in ISO 8601 format.
- archive_url string? - The link to the campaign's archive version.
- long_archive_url string? - The original link to the campaign's archive version.
- status string? - The current status of the campaign.
- emails_sent int? - The total number of emails sent for this campaign.
- send_time string? - The date and time a campaign was sent in ISO 8601 format.
- content_type string? - How the campaign's content is put together ('template', 'drag_and_drop', 'html', 'url').
- needs_block_refresh boolean? - Determines if the campaign needs its blocks refreshed by opening the web-based campaign editor. Deprecated and will always return false.
- resendable boolean? - Determines if the campaign qualifies to be resent to non-openers.
- recipients List9? - List settings for the campaign.
- settings CampaignSettings4? - The settings for your campaign, including subject, from name, reply-to address, and more.
- variate_settings AbTestOptions2? - The settings specific to A/B test campaigns.
- tracking CampaignTrackingOptions? - The tracking options for a campaign.
- rss_opts RssOptions3? - RSS options for a campaign.
- ab_split_opts AbTestingOptions? - A/B Testing options for a campaign.
- social_card CampaignSocialCard? - The preview for the campaign, rendered by social networks like Facebook and Twitter. Learn more.
- report_summary CampaignReportSummary2? - For sent campaigns, a summary of opens and clicks.
- delivery_status CampaignDeliveryStatus? - Updates on campaigns in the process of sending.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: Campaign4
A summary of an individual campaign's settings and content.
Fields
- id string? - A string that uniquely identifies this campaign.
- web_id int? - The ID used in the Mailchimp web application. View this campaign in your Mailchimp account at
https://{dc}.admin.mailchimp.com/campaigns/show/?id={web_id}
.
- parent_campaign_id string? - If this campaign is the child of another campaign, this identifies the parent campaign. For Example, for RSS or Automation children.
- create_time string? - The date and time the campaign was created in ISO 8601 format.
- archive_url string? - The link to the campaign's archive version in ISO 8601 format.
- long_archive_url string? - The original link to the campaign's archive version.
- status string? - The current status of the campaign.
- emails_sent int? - The total number of emails sent for this campaign.
- send_time string? - The date and time a campaign was sent.
- content_type string? - How the campaign's content is put together.
- needs_block_refresh boolean? - Determines if the campaign needs its blocks refreshed by opening the web-based campaign editor. Deprecated and will always return false.
- resendable boolean? - Determines if the campaign qualifies to be resent to non-openers.
- recipients List7? - List settings for the campaign.
- settings CampaignSettings3? - The settings for your campaign, including subject, from name, reply-to address, and more.
- variate_settings AbTestOptions1? - The settings specific to A/B test campaigns.
- tracking CampaignTrackingOptions? - The tracking options for a campaign.
- rss_opts RssOptions2? - RSS options for a campaign.
- ab_split_opts AbTestingOptions? - A/B Testing options for a campaign.
- social_card CampaignSocialCard? - The preview for the campaign, rendered by social networks like Facebook and Twitter. Learn more.
- report_summary CampaignReportSummary1? - For sent campaigns, a summary of opens, clicks, and e-commerce data.
- delivery_status CampaignDeliveryStatus? - Updates on campaigns in the process of sending.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: CampaignContent
The HTML and plain-text content for a campaign.
Fields
- variate_contents CampaignContentVariateContents[]? - Content options for multivariate campaigns.
- plain_text string? - The plain-text portion of the campaign. If left unspecified, we'll generate this automatically.
- html string? - The raw HTML for the campaign.
- archive_html string? - The Archive HTML for the campaign.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: CampaignContent1
The HTML and plain-text content for a campaign
Fields
- plain_text string? - The plain-text portion of the campaign. If left unspecified, we'll generate this automatically.
- html string? - The raw HTML for the campaign.
- url string? - When importing a campaign, the URL where the HTML lives.
- template TemplateContent? - Use this template to generate the HTML content of the campaign
- archive UploadArchive? - Available when uploading an archive to create campaign content. The archive should include all campaign content and images. Learn more.
- variate_contents CampaignscampaignIdcontentVariateContents[]? - Content options for Multivariate Campaigns. Each content option must provide HTML content and may optionally provide plain text. For campaigns not testing content, only one object should be provided.
mailchimp: CampaignContentVariateContents
Fields
- content_label string? - Label used to identify the content option.
- plain_text string? - The plain-text portion of the campaign. If left unspecified, we'll generate this automatically.
- html string? - The raw HTML for the campaign.
mailchimp: CampaignDefaults
Default values for campaigns created for this list.
Fields
- from_name string - The default from name for campaigns sent to this list.
- from_email string - The default from email for campaigns sent to this list.
- subject string - The default subject line for campaigns sent to this list.
- language string - The default language for this lists's forms.
mailchimp: CampaignDefaults1
Default values for campaigns created for this list.
Fields
- from_name string? - The default from name for campaigns sent to this list.
- from_email string? - The default from email for campaigns sent to this list.
- subject string? - The default subject line for campaigns sent to this list.
- language string? - The default language for this lists's forms.
mailchimp: CampaignDeliveryStatus
Updates on campaigns in the process of sending.
Fields
- enabled boolean? - Whether Campaign Delivery Status is enabled for this account and campaign.
- can_cancel boolean? - Whether a campaign send can be canceled.
- status string? - The current state of a campaign delivery.
- emails_sent int? - The total number of emails confirmed sent for this campaign so far.
- emails_canceled int? - The total number of emails canceled for this campaign.
mailchimp: CampaignFeedback
A specific feedback message from a specific campaign.
Fields
- block_id int? - The block id for the editable block that the feedback addresses.
- message string - The content of the feedback.
- is_complete boolean? - The status of feedback.
mailchimp: CampaignFeedback1
A specific feedback message from a specific campaign.
Fields
- feedback_id int? - The individual id for the feedback item.
- parent_id int? - If a reply, the id of the parent feedback item.
- block_id int? - The block id for the editable block that the feedback addresses.
- message string? - The content of the feedback.
- is_complete boolean? - The status of feedback.
- created_by string? - The login name of the user who created the feedback.
- created_at string? - The date and time the feedback item was created in ISO 8601 format.
- updated_at string? - The date and time the feedback was last updated in ISO 8601 format.
- 'source string? - The source of the feedback.
- campaign_id string? - The unique id for the campaign.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: CampaignFeedback2
A specific feedback message from a specific campaign.
Fields
- block_id int? - The block id for the editable block that the feedback addresses.
- message string? - The content of the feedback.
- is_complete boolean? - The status of feedback.
mailchimp: CampaignFeedback3
A specific feedback message from a specific campaign.
Fields
- feedback_id int? - The individual id for the feedback item.
- parent_id int? - If a reply, the id of the parent feedback item.
- block_id int? - The block id for the editable block that the feedback addresses.
- message string - The content of the feedback.
- is_complete boolean? - The status of feedback.
- created_by string? - The login name of the user who created the feedback.
- created_at string? - The date and time the feedback item was created in ISO 8601 format.
- updated_at string? - The date and time the feedback was last updated in ISO 8601 format.
- 'source string? - The source of the feedback.
- campaign_id string? - The unique id for the campaign.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: CampaignReports
A summary of the comment feedback for a specific campaign.
Fields
- feedback CampaignFeedback3[]? - A collection of feedback items for a campaign.
- campaign_id string? - The unique id for the campaign.
- total_items int? - The total number of items matching the query regardless of pagination.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: CampaignReportSummary
A summary of opens and clicks for sent campaigns.
Fields
- opens int? - The total number of opens for a campaign.
- unique_opens int? - The number of unique opens.
- open_rate decimal? - The number of unique opens divided by the total number of successful deliveries.
- clicks int? - The total number of clicks for an campaign.
- subscriber_clicks int? - The number of unique clicks.
- click_rate decimal? - The number of unique clicks, divided by the total number of successful deliveries.
mailchimp: CampaignReportSummary1
For sent campaigns, a summary of opens, clicks, and e-commerce data.
Fields
- opens int? - The total number of opens for a campaign.
- unique_opens int? - The number of unique opens.
- open_rate decimal? - The number of unique opens divided by the total number of successful deliveries.
- clicks int? - The total number of clicks for an campaign.
- subscriber_clicks int? - The number of unique clicks.
- click_rate decimal? - The number of unique clicks divided by the total number of successful deliveries.
- ecommerce EcommerceReport? - E-Commerce stats for a campaign.
mailchimp: CampaignReportSummary2
For sent campaigns, a summary of opens and clicks.
Fields
- opens int? - The total number of opens for a campaign.
- unique_opens int? - The number of unique opens.
- open_rate decimal? - The number of unique opens divided by the total number of successful deliveries.
- clicks int? - The total number of clicks for an campaign.
- subscriber_clicks int? - The number of unique clicks.
- click_rate decimal? - The number of unique clicks divided by the total number of successful deliveries.
- ecommerce EcommerceReport? - E-Commerce stats for a campaign.
mailchimp: CampaignReportSummary3
For sent campaigns, a summary of opens and clicks.
Fields
- opens int? - The total number of opens for a campaign.
- unique_opens int? - The number of unique opens.
- open_rate decimal? - The number of unique opens divided by the total number of successful deliveries.
- clicks int? - The total number of clicks for an campaign.
- subscriber_clicks int? - The number of unique clicks.
- click_rate decimal? - The number of unique clicks divided by the total number of successful deliveries.
mailchimp: CampaignscampaignIdcontentVariateContents
Fields
- content_label string - The label used to identify the content option.
- plain_text string? - The plain-text portion of the campaign. If left unspecified, we'll generate this automatically.
- html string? - The raw HTML for the campaign.
- url string? - When importing a campaign, the URL for the HTML.
- template TemplateContent1? - Use this template to generate the HTML content for the campaign.
- archive UploadArchive? - Available when uploading an archive to create campaign content. The archive should include all campaign content and images. Learn more.
mailchimp: CampaignSettings
Settings for the campaign including the email subject, from name, and from email address.
Fields
- subject_line string? - The subject line for the campaign.
- preview_text string? - The preview text for the campaign.
- title string? - The title of the Automation.
- from_name string? - The 'from' name for the Automation (not an email address).
- reply_to string? - The reply-to email address for the Automation.
mailchimp: CampaignSettings1
The settings for your campaign, including subject, from name, reply-to address, and more.
Fields
- subject_line string? - The subject line for the campaign.
- preview_text string? - The preview text for the campaign.
- title string? - The title of the campaign.
- from_name string? - The 'from' name on the campaign (not an email address).
- reply_to string? - The reply-to email address for the campaign. Note: while this field is not required for campaign creation, it is required for sending.
- use_conversation boolean? - Use Mailchimp Conversation feature to manage out-of-office replies.
- to_name string? - The campaign's custom 'To' name. Typically the first name merge field.
- folder_id string? - If the campaign is listed in a folder, the id for that folder.
- authenticate boolean? - Whether Mailchimp authenticated the campaign. Defaults to
true
.
- auto_footer boolean? - Automatically append Mailchimp's default footer to the campaign.
- inline_css boolean? - Automatically inline the CSS included with the campaign content.
- auto_tweet boolean? - Automatically tweet a link to the campaign archive page when the campaign is sent.
- fb_comments boolean? - Allows Facebook comments on the campaign (also force-enables the Campaign Archive toolbar). Defaults to
true
.
- template_id int? - The id of the template to use.
mailchimp: CampaignSettings2
The settings for your campaign, including subject, from name, reply-to address, and more.
Fields
- subject_line string - The subject line for the campaign.
- preview_text string? - The preview text for the campaign.
- title string? - The title of the campaign.
- from_name string - The 'from' name on the campaign (not an email address).
- reply_to string - The reply-to email address for the campaign.
- use_conversation boolean? - Use Mailchimp Conversation feature to manage out-of-office replies.
- to_name string? - The campaign's custom 'To' name. Typically the first name merge field.
- folder_id string? - If the campaign is listed in a folder, the id for that folder.
- authenticate boolean? - Whether Mailchimp authenticated the campaign. Defaults to
true
.
- auto_footer boolean? - Automatically append Mailchimp's default footer to the campaign.
- inline_css boolean? - Automatically inline the CSS included with the campaign content.
- auto_tweet boolean? - Automatically tweet a link to the campaign archive page when the campaign is sent.
- fb_comments boolean? - Allows Facebook comments on the campaign (also force-enables the Campaign Archive toolbar). Defaults to
true
.
- template_id int? - The id of the template to use.
mailchimp: CampaignSettings3
The settings for your campaign, including subject, from name, reply-to address, and more.
Fields
- subject_line string? - The subject line for the campaign.
- preview_text string? - The preview text for the campaign.
- title string? - The title of the campaign.
- from_name string? - The 'from' name on the campaign (not an email address).
- reply_to string? - The reply-to email address for the campaign.
- use_conversation boolean? - Use Mailchimp Conversation feature to manage out-of-office replies.
- to_name string? - The campaign's custom 'To' name. Typically the first name merge field.
- folder_id string? - If the campaign is listed in a folder, the id for that folder.
- authenticate boolean? - Whether Mailchimp authenticated the campaign. Defaults to
true
.
- auto_footer boolean? - Automatically append Mailchimp's default footer to the campaign.
- inline_css boolean? - Automatically inline the CSS included with the campaign content.
- auto_tweet boolean? - Automatically tweet a link to the campaign archive page when the campaign is sent.
- fb_comments boolean? - Allows Facebook comments on the campaign (also force-enables the Campaign Archive toolbar). Defaults to
true
.
- template_id int? - The id for the template used in this campaign.
- drag_and_drop boolean? - Whether the campaign uses the drag-and-drop editor.
mailchimp: CampaignSettings4
The settings for your campaign, including subject, from name, reply-to address, and more.
Fields
- subject_line string? - The subject line for the campaign.
- preview_text string? - The preview text for the campaign.
- title string? - The title of the campaign.
- from_name string? - The 'from' name on the campaign (not an email address).
- reply_to string? - The reply-to email address for the campaign.
- use_conversation boolean? - Use Mailchimp Conversation feature to manage replies.
- to_name string? - The campaign's custom 'To' name. Typically the first name merge field.
- folder_id string? - If the campaign is listed in a folder, the id for that folder.
- authenticate boolean? - Whether Mailchimp authenticated the campaign. Defaults to
true
.
- auto_footer boolean? - Automatically append Mailchimp's default footer to the campaign.
- inline_css boolean? - Automatically inline the CSS included with the campaign content.
- auto_tweet boolean? - Automatically tweet a link to the campaign archive page when the campaign is sent.
- fb_comments boolean? - Allows Facebook comments on the campaign (also force-enables the Campaign Archive toolbar). Defaults to
true
.
- template_id int? - The id for the template used in this campaign.
- drag_and_drop boolean? - Whether the campaign uses the drag-and-drop editor.
mailchimp: CampaignSettings5
Settings for the campaign including the email subject, from name, and from email address.
Fields
- subject_line string? - The subject line for the campaign.
- preview_text string? - The preview text for the campaign.
- title string? - The title of the campaign.
- from_name string? - The 'from' name on the campaign (not an email address).
- reply_to string? - The reply-to email address for the campaign.
- authenticate boolean? - Whether Mailchimp authenticated the campaign. Defaults to
true
.
- auto_footer boolean? - Automatically append Mailchimp's default footer to the campaign.
- inline_css boolean? - Automatically inline the CSS included with the campaign content.
- auto_tweet boolean? - Automatically tweet a link to the campaign archive page when the campaign is sent.
- fb_comments boolean? - Allows Facebook comments on the campaign (also force-enables the Campaign Archive toolbar). Defaults to
true
.
- template_id int? - The id for the template used in this campaign.
- drag_and_drop boolean? - Whether the campaign uses the drag-and-drop editor.
mailchimp: CampaignSocialCard
The preview for the campaign, rendered by social networks like Facebook and Twitter. Learn more.
Fields
- image_url string? - The url for the header image for the card.
- description string? - A short summary of the campaign to display.
- title string? - The title for the card. Typically the subject line of the campaign.
mailchimp: CampaignTrackingOptions
The tracking options for a campaign.
Fields
- opens boolean? - Whether to track opens. Defaults to
true
. Cannot be set to false for variate campaigns.
- html_clicks boolean? - Whether to track clicks in the HTML version of the campaign. Defaults to
true
. Cannot be set to false for variate campaigns.
- text_clicks boolean? - Whether to track clicks in the plain-text version of the campaign. Defaults to
true
. Cannot be set to false for variate campaigns.
- goal_tracking boolean? - Deprecated
- ecomm360 boolean? - Whether to enable e-commerce tracking.
- google_analytics string? - The custom slug for Google Analytics tracking (max of 50 bytes).
- salesforce SalesforceCrmTracking? - Deprecated
- capsule CapsuleCrmTracking? - Deprecated
mailchimp: CampaignTrackingOptions1
The tracking options for a campaign.
Fields
- opens boolean? - Whether to track opens. Defaults to
true
.
- html_clicks boolean? - Whether to track clicks in the HTML version of the campaign. Defaults to
true
.
- text_clicks boolean? - Whether to track clicks in the plain-text version of the campaign. Defaults to
true
.
- goal_tracking boolean? - Deprecated
- ecomm360 boolean? - Whether to enable e-commerce tracking.
- google_analytics string? - The custom slug for Google Analytics tracking (max of 50 bytes).
- clicktale string? - The custom slug for Click Tale tracking (max of 50 bytes).
- salesforce SalesforceCrmTracking1? - Deprecated
- capsule CapsuleCrmTracking2? - Deprecated
mailchimp: CapsuleCrmTracking
Deprecated
Fields
- notes boolean? - Update contact notes for a campaign based on subscriber email addresses.
mailchimp: CapsuleCrmTracking1
Deprecated
Fields
- notes boolean? - Update contact notes for a campaign based on a subscriber's email addresses.
mailchimp: CapsuleCrmTracking2
Deprecated
Fields
- notes boolean? - Update contact notes for a campaign based on a subscriber's email address.
mailchimp: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
mailchimp: CollectionAuthorization
Do particular authorization constraints around this collection limit creation of new instances?
Fields
- may_create boolean - May the user create additional instances of this resource?
- max_instances int - How many total instances of this resource are allowed? This is independent of any filter conditions applied to the query. As a special case, -1 indicates unlimited.
- current_total_instances int? - How many total instances of this resource are already in use? This is independent of any filter conditions applied to the query. Value may be larger than max_instances. As a special case, -1 is returned when access is unlimited.
mailchimp: CollectionOfContentForListSignupForms
Collection of Content for List Signup Forms.
Fields
- section string? - The content section name.
- value string? - The content section text.
mailchimp: CollectionOfElementStyleForListSignupForms
Collection of Element style for List Signup Forms.
Fields
- selector string? - A string that identifies the element selector.
- options AnOptionForSignupFormStyles[]? - A collection of options for a selector.
mailchimp: CollectionOfEvents
A collection of events for a given contact
Fields
- events Event[]? - An array of objects, each representing an event.
- total_items int? - The total number of items matching the query regardless of pagination.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: CollectionOfMemberActivityEvents
The last 50 Goal events for a member on a specific list.
Fields
- goals Goal[]? - The last 50 Goal events triggered by a member.
- list_id string? - The list id.
- email_id string? - The MD5 hash of the lowercase version of the list member's email address.
- total_items int? - The total number of items matching the query regardless of pagination.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: CollectionOfMergeFields
The merge field (formerly merge vars) for a list. These correspond to merge fields in Mailchimp's lists and subscriber profiles.
Fields
- merge_fields MergeField3[]? - An array of objects, each representing a merge field resource.
- list_id string? - The list id.
- total_items int? - The total number of items matching the query regardless of pagination.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: CollectionOfNotes
The last 10 notes for a specific list member, based on date created.
Fields
- notes MemberNotes3[]? - An array of objects, each representing a note resource.
- email_id string? - The MD5 hash of the lowercase version of the list member's email address.
- list_id string? - The list id.
- total_items int? - The total number of items matching the query regardless of pagination.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: CollectionOfSegments
A list of available segments.
Fields
- segments List8[]? - An array of objects, each representing a list segment.
- list_id string? - The list id.
- total_items int? - The total number of items matching the query regardless of pagination.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: CollectionOfTags
A list of tags assigned to a list member.
Fields
- tags CollectionOfTagsTags[]? - A list of tags assigned to the list member.
- total_items int? - The total number of items matching the query regardless of pagination.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: CollectionOfTagsTags
Fields
- id int? - The unique id for the tag.
- name string? - The name of the tag.
- date_added string? - The date and time the tag was added to the list member in ISO 8601 format.
mailchimp: Conditions
The conditions of the segment. Static and fuzzy segments don't have conditions.
Fields
- 'match string? - Match type.
- conditions record {}[]? - Segment match conditions. There are multiple possible types, see the condition types documentation.
mailchimp: Conditions1
The conditions of the segment. Static and fuzzy segments don't have conditions.
Fields
- 'match string? - Match type.
- conditions record {}[]? - An array of segment conditions.
mailchimp: Conditions2
The conditions of the segment. Static segments (tags) and fuzzy segments don't have conditions.
Fields
- 'match string? - Match type.
- conditions record {}[]? - Segment match conditions. There are multiple possible types, see the condition types documentation.
mailchimp: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth CredentialsConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
mailchimp: DailyListActivity
One day's worth of list activity. Doesn't include Automation activity.
Fields
- day string? - The date for the activity summary.
- emails_sent int? - The total number of emails sent on the date for the activity summary.
- unique_opens int? - The number of unique opens.
- recipient_clicks int? - The number of clicks.
- hard_bounce int? - The number of hard bounces.
- soft_bounce int? - The number of soft bounces
- subs int? - The number of subscribes.
- unsubs int? - The number of unsubscribes.
- other_adds int? - The number of subscribers who may have been added outside of the double opt-in process, such as imports or API activity.
- other_removes int? - The number of subscribers who may have been removed outside of unsubscribing or reporting an email as spam (for example, deleted subscribers).
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: DailySendingDays
The days of the week to send a daily RSS Campaign.
Fields
- sunday boolean? - Sends the daily RSS Campaign on Sundays.
- monday boolean? - Sends the daily RSS Campaign on Mondays.
- tuesday boolean? - Sends the daily RSS Campaign on Tuesdays.
- wednesday boolean? - Sends the daily RSS Campaign on Wednesdays.
- thursday boolean? - Sends the daily RSS Campaign on Thursdays.
- friday boolean? - Sends the daily RSS Campaign on Fridays.
- saturday boolean? - Sends the daily RSS Campaign on Saturdays.
mailchimp: EcommerceReport
E-Commerce stats for a campaign.
Fields
- total_orders int? - The total orders for a campaign.
- total_spent decimal? - The total spent for a campaign. Calculated as the sum of all order totals with no deductions.
- total_revenue decimal? - The total revenue for a campaign. Calculated as the sum of all order totals minus shipping and tax totals.
mailchimp: EcommerceStats
Ecommerce stats for the list member if the list is attached to a store.
Fields
- total_revenue decimal? - The total revenue the list member has brought in.
- number_of_orders decimal? - The total number of orders placed by the list member.
- currency_code string? - The three-letter ISO 4217 code for the currency that the store accepts.
mailchimp: EmailClient
The email client.
Fields
- 'client string? - The name of the email client.
- members int? - The number of subscribed members who used this email client.
mailchimp: EmailClients
The top email clients based on user-agent strings.
Fields
- clients EmailClient[]? - An array of top email clients.
- list_id string? - The list id.
- total_items int? - The total number of items matching the query regardless of pagination.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: Event
A specific event for a contact.
Fields
- occurred_at string? - The date and time the event occurred in ISO 8601 format.
- name string? - The name for this type of event ('purchased', 'visited', etc). Must be 2-30 characters in length
- properties record {}? - An optional list of properties
mailchimp: Events
A new event for a specific list member
Fields
- name string - The name for this type of event ('purchased', 'visited', etc). Must be 2-30 characters in length
- properties record {}? - An optional list of properties
- is_syncing boolean? - Events created with the is_syncing value set to
true
will not trigger automations.
- occurred_at string? - The date and time the event occurred in ISO 8601 format.
mailchimp: Events1
The events that can trigger the webhook and whether they are enabled.
Fields
- subscribe boolean? - Whether the webhook is triggered when a list subscriber is added.
- unsubscribe boolean? - Whether the webhook is triggered when a list member unsubscribes.
- profile boolean? - Whether the webhook is triggered when a subscriber's profile is updated.
- cleaned boolean? - Whether the webhook is triggered when a subscriber's email address is cleaned from the list.
- upemail boolean? - Whether the webhook is triggered when a subscriber's email address is changed.
- campaign boolean? - Whether the webhook is triggered when a campaign is sent or cancelled.
mailchimp: Events2
The events that can trigger the webhook and whether they are enabled.
Fields
- subscribe boolean? - Whether the webhook is triggered when a list subscriber is added.
- unsubscribe boolean? - Whether the webhook is triggered when a list member unsubscribes.
- profile boolean? - Whether the webhook is triggered when a subscriber's profile is updated.
- cleaned boolean? - Whether the webhook is triggered when a subscriber's email address is cleaned from the list.
- upemail boolean? - Whether the webhook is triggered when a subscriber's email address is changed.
- campaign boolean? - Whether the webhook is triggered when a campaign is sent or cancelled.
mailchimp: ExactMatches
Exact matches of the provided search query.
Fields
- members ListMembers3[]? - An array of objects, each representing a specific list member.
- total_items int? - The total number of items matching the query regardless of pagination.
mailchimp: Goal
A single instance of a goal activity.
Fields
- goal_id int? - The id for a Goal event.
- event string? - The name/type of Goal event triggered.
- last_visited_at string? - The date and time the user last triggered the Goal event in ISO 8601 format.
- data string? - Any extra data passed with the Goal event.
mailchimp: GrowthHistory
A month-by-month summary of a specific list's growth activity.
Fields
- history GrowthHistory2[]? - An array of objects, each representing a monthly growth report for a list.
- list_id string? - The list id.
- total_items int? - The total number of items matching the query regardless of pagination.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: GrowthHistory1
A summary of a specific list's growth activity for a specific month and year.
Fields
- list_id string? - The list id for the growth activity report.
- month string? - The month that the growth history is describing.
- existing int? - (deprecated)
- imports int? - (deprecated)
- optins int? - (deprecated)
- subscribed int? - Total subscribed members on the list at the end of the month.
- unsubscribed int? - Newly unsubscribed members on the list for a specific month.
- reconfirm int? - Newly reconfirmed members on the list for a specific month.
- cleaned int? - Newly cleaned (hard-bounced) members on the list for a specific month.
- pending int? - Pending members on the list for a specific month.
- deleted int? - Newly deleted members on the list for a specific month.
- 'transactional int? - Subscribers that have been sent transactional emails via Mandrill.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: GrowthHistory2
A summary of a specific list's growth activity for a specific month and year.
Fields
- list_id string? - The list id for the growth activity report.
- month string? - The month that the growth history is describing.
- existing int? - (deprecated)
- imports int? - (deprecated)
- optins int? - (deprecated)
- subscribed int? - Total subscribed members on the list at the end of the month.
- unsubscribed int? - Newly unsubscribed members on the list for a specific month.
- reconfirm int? - Newly reconfirmed members on the list for a specific month.
- cleaned int? - Newly cleaned (hard-bounced) members on the list for a specific month.
- pending int? - Pending members on the list for a specific month.
- deleted int? - Newly deleted members on the list for a specific month.
- 'transactional int? - Subscribers that have been sent transactional emails via Mandrill.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: Hours
The hours an Automation workflow can send.
Fields
- 'type string - When to send the Automation email.
mailchimp: IndustryStats
The average campaign statistics for all campaigns in the account's specified industry.
Fields
- open_rate decimal? - The average unique open rate for all campaigns in the account's specified industry.
- bounce_rate decimal? - The average bounce rate for all campaigns in the account's specified industry.
- click_rate decimal? - The average unique click rate for all campaigns in the account's specified industry.
mailchimp: InlineResponse200
An array of objects, each representing an authorized application.
Fields
- apps InlineResponse200Apps[]? - An array of objects, each representing an authorized application.
- total_items int? - The total number of items matching the query regardless of pagination.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: InlineResponse2001
An authorized app.
Fields
- id int? - The ID for the application.
- name string? - The name of the application.
- description string? - A short description of the application.
- users string[]? - An array of usernames for users who have linked the app.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: InlineResponse2002
An array of objects, each representing an Automation workflow.
Fields
- automations AutomationWorkflow2[]? - An array of objects, each representing an Automation workflow.
- total_items int? - The total number of items matching the query regardless of pagination.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: InlineResponse2003
An automation workflow
Fields
- workflow_id string? - A string that uniquely identifies an Automation workflow.
- email_id string? - A string that uniquely identifies an email in an Automation workflow.
- queue SubscriberInAutomationQueue3[]? - An array of objects, each representing a subscriber queue for an email in an Automation workflow.
- total_items int? - The total number of items matching the query regardless of pagination.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: InlineResponse2004
An array of campaigns.
Fields
- campaigns Campaign4[]? - An array of campaigns.
- total_items int? - The total number of items matching the query regardless of pagination.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: InlineResponse200Apps
An authorized app.
Fields
- id int? - The ID for the application.
- name string? - The name of the application.
- description string? - A short description of the application.
- users string[]? - An array of usernames for users who have linked the app.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: Interest
Assign subscribers to interests to group them together. Interests are referred to as 'group names' in the Mailchimp application.
Fields
- name string - The name of the interest. This can be shown publicly on a subscription form.
- display_order int? - The display order for interests.
mailchimp: Interest1
Assign subscribers to interests to group them together. Interests are referred to as 'group names' in the Mailchimp application.
Fields
- category_id string? - The id for the interest category.
- list_id string? - The ID for the list that this interest belongs to.
- id string? - The ID for the interest.
- name string? - The name of the interest. This can be shown publicly on a subscription form.
- subscriber_count string? - The number of subscribers associated with this interest.
- display_order int? - The display order for interests.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: Interest2
Assign subscribers to interests to group them together. Interests are referred to as 'group names' in the Mailchimp application.
Fields
- name string - The name of the interest. This can be shown publicly on a subscription form.
- display_order int? - The display order for interests.
mailchimp: Interest3
Assign subscribers to interests to group them together. Interests are referred to as 'group names' in the Mailchimp application.
Fields
- category_id string? - The id for the interest category.
- list_id string? - The ID for the list that this interest belongs to.
- id string? - The ID for the interest.
- name string? - The name of the interest. This can be shown publicly on a subscription form.
- subscriber_count string? - The number of subscribers associated with this interest.
- display_order int? - The display order for interests.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: InterestCategory
Interest categories organize interests, which are used to group subscribers based on their preferences. These correspond to Group Titles the application.
Fields
- title string - The text description of this category. This field appears on signup forms and is often phrased as a question.
- display_order int? - The order that the categories are displayed in the list. Lower numbers display first.
- 'type string - Determines how this category’s interests appear on signup forms.
mailchimp: InterestCategory1
Interest categories organize interests, which are used to group subscribers based on their preferences. These correspond to Group Titles the application.
Fields
- list_id string? - The unique list id for the category.
- id string? - The id for the interest category.
- title string? - The text description of this category. This field appears on signup forms and is often phrased as a question.
- display_order int? - The order that the categories are displayed in the list. Lower numbers display first.
- 'type string? - Determines how this category’s interests appear on signup forms.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: InterestCategory2
Interest categories organize interests, which are used to group subscribers based on their preferences. These correspond to Group Titles the application.
Fields
- title string - The text description of this category. This field appears on signup forms and is often phrased as a question.
- display_order int? - The order that the categories are displayed in the list. Lower numbers display first.
- 'type string - Determines how this category’s interests appear on signup forms.
mailchimp: InterestCategory3
Interest categories organize interests, which are used to group subscribers based on their preferences. These correspond to Group Titles the application.
Fields
- list_id string? - The unique list id for the category.
- id string? - The id for the interest category.
- title string? - The text description of this category. This field appears on signup forms and is often phrased as a question.
- display_order int? - The order that the categories are displayed in the list. Lower numbers display first.
- 'type string? - Determines how this category’s interests appear on signup forms.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: InterestGroupings
Information about this list's interest categories.
Fields
- list_id string? - The ID for the list that this category belongs to.
- categories InterestCategory3[]? - This array contains individual interest categories.
- total_items int? - The total number of items matching the query regardless of pagination.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: Interests
A list of this category's interests
Fields
- interests Interest3[]? - An array of this category's interests
- list_id string? - The unique list id that the interests belong to.
- category_id string? - The id for the interest category.
- total_items int? - The total number of items matching the query regardless of pagination.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: List
List settings for the Automation.
Fields
- list_id string? - The id of the List.
- store_id string? - The id of the store.
mailchimp: List1
List settings for the campaign.
Fields
- list_id string - The unique list id.
- segment_opts SegmentOptions? - An object representing all segmentation options. This object should contain a
saved_segment_id
to use an existing segment, or you can create a new segment by including bothmatch
andconditions
options.
mailchimp: List10
List settings for the campaign.
Fields
- list_id string? - The unique list id.
- list_is_active boolean? - The status of the list used, namely if it's deleted or disabled.
- list_name string? - The name of the list.
- recipient_count int? - Count of the recipients on the associated list. Formatted as an integer.
- segment_opts SegmentOptions1? - An object representing all segmentation options. This object should contain a
saved_segment_id
to use an existing segment, or you can create a new segment by including bothmatch
andconditions
options.
mailchimp: List2
List settings for the campaign.
Fields
- list_id string - The unique list id.
- segment_opts SegmentOptions1? - An object representing all segmentation options. This object should contain a
saved_segment_id
to use an existing segment, or you can create a new segment by including bothmatch
andconditions
options.
mailchimp: List3
Information about a specific list segment.
Fields
- name string - The name of the segment.
- static_segment string[]? - An array of emails to be used for a static segment. Any emails provided that are not present on the list will be ignored. Passing an empty array will create a static segment without any subscribers. This field cannot be provided with the options field.
- options Conditions? - The conditions of the segment. Static and fuzzy segments don't have conditions.
mailchimp: List4
Information about a specific segment.
Fields
- id int? - The unique id for the segment.
- name string? - The name of the segment.
- member_count int? - The number of active subscribers currently included in the segment.
- created_at string? - The date and time the segment was created in ISO 8601 format.
- updated_at string? - The date and time the segment was last updated in ISO 8601 format.
- options Conditions2? - The conditions of the segment. Static segments (tags) and fuzzy segments don't have conditions.
- list_id string? - The list id.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: List5
Information about a specific list segment.
Fields
- name string - The name of the segment.
- static_segment string[]? - An array of emails to be used for a static segment. Any emails provided that are not present on the list will be ignored. Passing an empty array for an existing static segment will reset that segment and remove all members. This field cannot be provided with the
options
field.
- options Conditions1? - The conditions of the segment. Static and fuzzy segments don't have conditions.
mailchimp: List6
List settings for the Automation.
Fields
- list_id string? - The unique list id.
- list_is_active boolean? - The status of the list used, namely if it's deleted or disabled.
- list_name string? - List Name.
- segment_opts SegmentOptions2? - An object representing all segmentation options.
- store_id string? - The id of the store.
mailchimp: List7
List settings for the campaign.
Fields
- list_id string? - The unique list id.
- list_is_active boolean? - The status of the list used, namely if it's deleted or disabled.
- list_name string? - The name of the list.
- recipient_count int? - Count of the recipients on the associated list. Formatted as an integer.
- segment_opts SegmentOptions1? - An object representing all segmentation options. This object should contain a
saved_segment_id
to use an existing segment, or you can create a new segment by including bothmatch
andconditions
options.
mailchimp: List8
Information about a specific segment.
Fields
- id int? - The unique id for the segment.
- name string? - The name of the segment.
- member_count int? - The number of active subscribers currently included in the segment.
- created_at string? - The date and time the segment was created in ISO 8601 format.
- updated_at string? - The date and time the segment was last updated in ISO 8601 format.
- options Conditions2? - The conditions of the segment. Static segments (tags) and fuzzy segments don't have conditions.
- list_id string? - The list id.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: List9
List settings for the campaign.
Fields
- list_id string - The unique list id.
- list_name string? - The name of the list.
- recipient_count int? - Count of the recipients on the associated list. Formatted as an integer.
- segment_opts SegmentOptions1? - An object representing all segmentation options. This object should contain a
saved_segment_id
to use an existing segment, or you can create a new segment by including bothmatch
andconditions
options.
mailchimp: ListActivity
Up to the previous 180 days of daily detailed aggregated activity stats for a specific list. Does not include AutoResponder or Automation activity.
Fields
- activity DailyListActivity[]? - Recent list activity.
- list_id string? - The unique id for the list.
- total_items int? - The total number of items matching the query regardless of pagination.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: ListContact
Contact information displayed in campaign footers to comply with international spam laws.
Fields
- company string - The company name for the list.
- address1 string - The street address for the list contact.
- address2 string? - The street address for the list contact.
- city string - The city for the list contact.
- state string? - The state for the list contact.
- zip string? - The postal or zip code for the list contact.
- country string - A two-character ISO3166 country code. Defaults to US if invalid.
- phone string? - The phone number for the list contact.
mailchimp: ListContact1
Contact information displayed in campaign footers to comply with international spam laws.
Fields
- company string - The company name for the list.
- address1 string - The street address for the list contact.
- address2 string? - The street address for the list contact.
- city string - The city for the list contact.
- state string - The state for the list contact.
- zip string - The postal or zip code for the list contact.
- country string - A two-character ISO3166 country code. Defaults to US if invalid.
- phone string? - The phone number for the list contact.
mailchimp: ListContact2
Contact information displayed in campaign footers to comply with international spam laws.
Fields
- company string? - The company name for the list.
- address1 string? - The street address for the list contact.
- address2 string? - The street address for the list contact.
- city string? - The city for the list contact.
- state string? - The state for the list contact.
- zip string? - The postal or zip code for the list contact.
- country string? - A two-character ISO3166 country code. Defaults to US if invalid.
- phone string? - The phone number for the list contact.
mailchimp: ListLocation
Fields
- country string? - The name of the country.
- cc string? - The ISO 3166 2 digit country code.
- percent decimal? - The percent of subscribers in the country.
- total int? - The total number of subscribers in the country.
mailchimp: ListLocations
A summary of List's locations.
Fields
- locations ListLocation[]? - An array of objects, each representing a list's top subscriber locations.
- list_id string? - The unique id for the list.
- total_items int? - The total number of items matching the query regardless of pagination.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: ListMembers
Individuals who are currently or have been previously subscribed to this list, including members who have bounced or unsubscribed.
Fields
- id string? - The MD5 hash of the lowercase version of the list member's email address.
- email_address string? - Email address for a subscriber.
- unique_email_id string? - An identifier for the address across all of Mailchimp.
- email_type string? - Type of email this member asked to get ('html' or 'text').
- status string? - Subscriber's current status.
- merge_fields record {}? - An individual merge var and value for a member.
- interests record {}? - The key of this object's properties is the ID of the interest in question.
- stats SubscriberStats1? - Open and click rates for this subscriber.
- ip_signup string? - IP address the subscriber signed up from.
- timestamp_signup string? - The date and time the subscriber signed up for the list in ISO 8601 format.
- ip_opt string? - The IP address the subscriber used to confirm their opt-in status.
- timestamp_opt string? - The date and time the subscriber confirmed their opt-in status in ISO 8601 format.
- member_rating int? - Star rating for this member, between 1 and 5.
- last_changed string? - The date and time the member's info was last changed in ISO 8601 format.
- language string? - If set/detected, the subscriber's language.
- vip boolean? - VIP status for subscriber.
- email_client string? - The list member's email client.
- location Location1? - Subscriber location information.
- last_note Notes? - The most recent Note added about this member.
- list_id string? - The list id.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: ListMembers1
Manage members of a specific Mailchimp list, including currently subscribed, unsubscribed, and bounced members.
Fields
- members ListMembers3[]? - An array of objects, each representing a specific list member.
- list_id string? - The list id.
- total_items int? - The total number of items matching the query regardless of pagination.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: ListMembers1Tags
Fields
- id int? - The tag id.
- name string? - The name of the tag
mailchimp: ListMembers2
Individuals who are currently or have been previously subscribed to this list, including members who have bounced or unsubscribed.
Fields
- id string? - The MD5 hash of the lowercase version of the list member's email address.
- email_address string? - Email address for a subscriber.
- unique_email_id string? - An identifier for the address across all of Mailchimp.
- full_name string? - The contact's full name.
- web_id int? - The ID used in the Mailchimp web application. View this member in your Mailchimp account at
https://{dc}.admin.mailchimp.com/lists/members/view?id={web_id}
.
- email_type string? - Type of email this member asked to get ('html' or 'text').
- status string? - Subscriber's current status.
- unsubscribe_reason string? - A subscriber's reason for unsubscribing.
- merge_fields record {}? - An individual merge var and value for a member.
- interests record {}? - The key of this object's properties is the ID of the interest in question.
- stats SubscriberStats? - Open and click rates for this subscriber.
- ip_signup string? - IP address the subscriber signed up from.
- timestamp_signup string? - The date and time the subscriber signed up for the list in ISO 8601 format.
- ip_opt string? - The IP address the subscriber used to confirm their opt-in status.
- timestamp_opt string? - The date and time the subscribe confirmed their opt-in status in ISO 8601 format.
- member_rating int? - Star rating for this member, between 1 and 5.
- last_changed string? - The date and time the member's info was last changed in ISO 8601 format.
- language string? - If set/detected, the subscriber's language.
- vip boolean? - VIP status for subscriber.
- email_client string? - The list member's email client.
- location Location1? - Subscriber location information.
- marketing_permissions MarketingPermission1[]? - The marketing permissions for the subscriber.
- last_note Notes? - The most recent Note added about this member.
- 'source string? - The source from which the subscriber was added to this list.
- tags_count int? - The number of tags applied to this member.
- tags ListMembers1Tags[]? - Returns up to 50 tags applied to this member. To retrieve all tags see Member Tags.
- list_id string? - The list id.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: ListMembers3
Individuals who are currently or have been previously subscribed to this list, including members who have bounced or unsubscribed.
Fields
- id string? - The MD5 hash of the lowercase version of the list member's email address.
- email_address string? - Email address for a subscriber.
- unique_email_id string? - An identifier for the address across all of Mailchimp.
- full_name string? - The contact's full name.
- web_id int? - The ID used in the Mailchimp web application. View this member in your Mailchimp account at
https://{dc}.admin.mailchimp.com/lists/members/view?id={web_id}
.
- email_type string? - Type of email this member asked to get ('html' or 'text').
- status string? - Subscriber's current status.
- unsubscribe_reason string? - A subscriber's reason for unsubscribing.
- merge_fields record {}? - An individual merge var and value for a member.
- interests record {}? - The key of this object's properties is the ID of the interest in question.
- stats SubscriberStats? - Open and click rates for this subscriber.
- ip_signup string? - IP address the subscriber signed up from.
- timestamp_signup string? - The date and time the subscriber signed up for the list in ISO 8601 format.
- ip_opt string? - The IP address the subscriber used to confirm their opt-in status.
- timestamp_opt string? - The date and time the subscribe confirmed their opt-in status in ISO 8601 format.
- member_rating int? - Star rating for this member, between 1 and 5.
- last_changed string? - The date and time the member's info was last changed in ISO 8601 format.
- language string? - If set/detected, the subscriber's language.
- vip boolean? - VIP status for subscriber.
- email_client string? - The list member's email client.
- location Location1? - Subscriber location information.
- marketing_permissions MarketingPermission1[]? - The marketing permissions for the subscriber.
- last_note Notes? - The most recent Note added about this member.
- 'source string? - The source from which the subscriber was added to this list.
- tags_count int? - The number of tags applied to this member.
- tags ListMembers1Tags[]? - Returns up to 50 tags applied to this member. To retrieve all tags see Member Tags.
- list_id string? - The list id.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: ListMembers4
Individuals who are currently or have been previously subscribed to this list, including members who have bounced or unsubscribed.
Fields
- id string? - The MD5 hash of the lowercase version of the list member's email address.
- email_address string? - Email address for a subscriber.
- unique_email_id string? - An identifier for the address across all of Mailchimp.
- email_type string? - Type of email this member asked to get ('html' or 'text').
- status string? - Subscriber's current status.
- merge_fields record {}? - An individual merge var and value for a member.
- interests record {}? - The key of this object's properties is the ID of the interest in question.
- stats SubscriberStats1? - Open and click rates for this subscriber.
- ip_signup string? - IP address the subscriber signed up from.
- timestamp_signup string? - The date and time the subscriber signed up for the list in ISO 8601 format.
- ip_opt string? - The IP address the subscriber used to confirm their opt-in status.
- timestamp_opt string? - The date and time the subscriber confirmed their opt-in status in ISO 8601 format.
- member_rating int? - Star rating for this member, between 1 and 5.
- last_changed string? - The date and time the member's info was last changed in ISO 8601 format.
- language string? - If set/detected, the subscriber's language.
- vip boolean? - VIP status for subscriber.
- email_client string? - The list member's email client.
- location Location1? - Subscriber location information.
- last_note Notes? - The most recent Note added about this member.
- list_id string? - The list id.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: ListMembers5
Individuals who are currently or have been previously subscribed to this list, including members who have bounced or unsubscribed.
Fields
- id string? - The MD5 hash of the lowercase version of the list member's email address.
- email_address string? - Email address for a subscriber.
- unique_email_id string? - An identifier for the address across all of Mailchimp.
- email_type string? - Type of email this member asked to get ('html' or 'text').
- status string? - Subscriber's current status.
- merge_fields record {}? - An individual merge var and value for a member.
- interests record {}? - The key of this object's properties is the ID of the interest in question.
- stats SubscriberStats1? - Open and click rates for this subscriber.
- ip_signup string? - IP address the subscriber signed up from.
- timestamp_signup string? - The date and time the subscriber signed up for the list in ISO 8601 format.
- ip_opt string? - The IP address the subscriber used to confirm their opt-in status.
- timestamp_opt string? - The date and time the subscriber confirmed their opt-in status in ISO 8601 format.
- member_rating int? - Star rating for this member, between 1 and 5.
- last_changed string? - The date and time the member's info was last changed in ISO 8601 format.
- language string? - If set/detected, the subscriber's language.
- vip boolean? - VIP status for subscriber.
- email_client string? - The list member's email client.
- location Location1? - Subscriber location information.
- last_note Notes? - The most recent Note added about this member.
- tags_count int? - The number of tags applied to this member.
- tags ListMembers1Tags[]? - The tags applied to this member.
- list_id string? - The list id.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: ListSignupForms
List Signup Forms.
Fields
- signup_forms SignupForm2[]? - List signup form.
- list_id string? - The list id.
- total_items int? - The total number of items matching the query regardless of pagination.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: ListWebhooks
Manage webhooks for a specific list.
Fields
- webhooks ListWebhooks2[]? - An array of objects, each representing a specific list member.
- list_id string? - The list id.
- total_items int? - The total number of items matching the query regardless of pagination.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: ListWebhooks1
Webhook configured for the given list.
Fields
- id string? - An string that uniquely identifies this webhook.
- url string? - A valid URL for the Webhook.
- events Events2? - The events that can trigger the webhook and whether they are enabled.
- sources Sources1? - The possible sources of any events that can trigger the webhook and whether they are enabled.
- list_id string? - The unique id for the list.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: ListWebhooks2
Webhook configured for the given list.
Fields
- id string? - An string that uniquely identifies this webhook.
- url string? - A valid URL for the Webhook.
- events Events2? - The events that can trigger the webhook and whether they are enabled.
- sources Sources1? - The possible sources of any events that can trigger the webhook and whether they are enabled.
- list_id string? - The unique id for the list.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: Location
Subscriber location information.
Fields
- latitude decimal? - The location latitude.
- longitude decimal? - The location longitude.
mailchimp: Location1
Subscriber location information.
Fields
- latitude decimal? - The location latitude.
- longitude decimal? - The location longitude.
- gmtoff int? - The time difference in hours from GMT.
- dstoff int? - The offset for timezones where daylight saving time is observed.
- country_code string? - The unique code for the location country.
- timezone string? - The timezone for the location.
mailchimp: MarketingPermission
A single marketing permission a subscriber has either opted-in to or opted-out of.
Fields
- marketing_permission_id string? - The id for the marketing permission on the list
- enabled boolean? - If the subscriber has opted-in to the marketing permission.
mailchimp: MarketingPermission1
A single marketing permission a subscriber has either opted-in to or opted-out of.
Fields
- marketing_permission_id string? - The id for the marketing permission on the list
- text string? - The text of the marketing permission.
- enabled boolean? - If the subscriber has opted-in to the marketing permission.
mailchimp: MemberActivity
Member activity events.
Fields
- action string? - The type of action recorded for the subscriber.
- timestamp string? - The date and time recorded for the action.
- url string? - For clicks, the URL the subscriber clicked on.
- 'type string? - The type of campaign that was sent.
- campaign_id string? - The web-based ID for the campaign.
- title string? - If set, the campaign's title.
- parent_campaign string? - The ID of the parent campaign.
mailchimp: MemberActivityEvents
The last 50 member events for a list.
Fields
- activity MemberActivity[]? - An array of objects, each representing a member event.
- email_id string? - The MD5 hash of the lowercase version of the list member's email address.
- list_id string? - The list id.
- total_items int? - The total number of items matching the query regardless of pagination.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: MemberActivityEvents1
The member activity events for a given member.
Fields
- activity record {}[]? - An array of objects, each representing a contact event. There are multiple possible types, see the activity schema documentation.
- email_id string? - The MD5 hash of the lowercase version of the list member's email address.
- list_id string? - The list id.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: MemberNotes
A specific note for a specific member.
Fields
- note string? - The content of the note. Note length is limited to 1,000 characters.
mailchimp: MemberNotes1
A specific note for a specific member.
Fields
- id int? - The note id.
- created_at string? - The date and time the note was created in ISO 8601 format.
- created_by string? - The author of the note.
- updated_at string? - The date and time the note was last updated in ISO 8601 format.
- note string? - The content of the note.
- list_id string? - The unique id for the list.
- email_id string? - The MD5 hash of the lowercase version of the list member's email address.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: MemberNotes2
A specific note for a specific member.
Fields
- note string? - The content of the note. Note length is limited to 1,000 characters.
mailchimp: MemberNotes3
A specific note for a specific member.
Fields
- id int? - The note id.
- created_at string? - The date and time the note was created in ISO 8601 format.
- created_by string? - The author of the note.
- updated_at string? - The date and time the note was last updated in ISO 8601 format.
- note string? - The content of the note.
- list_id string? - The unique id for the list.
- email_id string? - The MD5 hash of the lowercase version of the list member's email address.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: Members
Members found for given search term
Fields
- exact_matches ExactMatches? - Exact matches of the provided search query.
- full_search PartialMatches? - Partial matches of the provided search query.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: MembersToAddremoveTofromAStaticSegment
Members to add/remove to/from a static segment
Fields
- members_to_add string[]? - An array of emails to be used for a static segment. Any emails provided that are not present on the list will be ignored. A maximum of 500 members can be sent.
- members_to_remove string[]? - An array of emails to be used for a static segment. Any emails provided that are not present on the list will be ignored. A maximum of 500 members can be sent.
mailchimp: MembersToSubscribeunsubscribeTofromAListInBatch
Members to subscribe to or unsubscribe from a list.
Fields
- members AddListMembers[] - An array of objects, each representing an email address and the subscription status for a specific list. Up to 500 members may be added or updated with each API call.
- update_existing boolean? - Whether this batch operation will change existing members' subscription status.
mailchimp: MemberTag
Add or remove tags on a member by declaring a tag either active or inactive on a member.
Fields
- name string - The name of the tag.
- status string - The status for the tag on the member, pass in active to add a tag or inactive to remove it.
mailchimp: MemberTags
A list of tags assigned to a list member.
Fields
- tags MemberTag[] - A list of tags assigned to the list member.
- is_syncing boolean? - When is_syncing is true, automations based on the tags in the request will not fire
mailchimp: MergeField
A merge field (formerly merge vars) for a specific list. These correspond to merge fields in Mailchimp's lists and subscriber profiles.
Fields
- tag string? - The tag used in Mailchimp campaigns and for the /members endpoint.
- name string - The name of the merge field.
- 'type string - The type for the merge field.
- required boolean? - The boolean value if the merge field is required.
- default_value string? - The default value for the merge field if
null
.
- 'public boolean? - Whether the merge field is displayed on the signup form.
- display_order int? - The order that the merge field displays on the list signup form.
- options MergeFieldOptions? - Extra options for some merge field types.
- help_text string? - Extra text to help the subscriber fill out the form.
mailchimp: MergeField1
A merge field (formerly merge vars) for a specific list. These correspond to merge fields in Mailchimp's lists and subscriber profiles.
Fields
- merge_id int? - An unchanging id for the merge field.
- tag string? - The tag used in Mailchimp campaigns and for the /members endpoint.
- name string? - The name of the merge field.
- 'type string? - The type for the merge field.
- required boolean? - The boolean value if the merge field is required.
- default_value string? - The default value for the merge field if
null
.
- 'public boolean? - Whether the merge field is displayed on the signup form.
- display_order int? - The order that the merge field displays on the list signup form.
- options MergeFieldOptions2? - Extra options for some merge field types.
- help_text string? - Extra text to help the subscriber fill out the form.
- list_id string? - A string that identifies this merge field collections' list.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: MergeField2
A merge field (formerly merge vars) for a specific list. These correspond to merge fields in Mailchimp's lists and subscriber profiles.
Fields
- tag string? - The tag used in Mailchimp campaigns and for the /members endpoint.
- name string - The name of the merge field.
- required boolean? - The boolean value if the merge field is required.
- default_value string? - The default value for the merge field if
null
.
- 'public boolean? - Whether the merge field is displayed on the signup form.
- display_order int? - The order that the merge field displays on the list signup form.
- options MergeFieldOptions1? - Extra options for some merge field types.
- help_text string? - Extra text to help the subscriber fill out the form.
mailchimp: MergeField3
A merge field (formerly merge vars) for a specific list. These correspond to merge fields in Mailchimp's lists and subscriber profiles.
Fields
- merge_id int? - An unchanging id for the merge field.
- tag string? - The tag used in Mailchimp campaigns and for the /members endpoint.
- name string? - The name of the merge field.
- 'type string? - The type for the merge field.
- required boolean? - The boolean value if the merge field is required.
- default_value string? - The default value for the merge field if
null
.
- 'public boolean? - Whether the merge field is displayed on the signup form.
- display_order int? - The order that the merge field displays on the list signup form.
- options MergeFieldOptions2? - Extra options for some merge field types.
- help_text string? - Extra text to help the subscriber fill out the form.
- list_id string? - A string that identifies this merge field collections' list.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: MergeFieldOptions
Extra options for some merge field types.
Fields
- default_country int? - In an address field, the default country code if none supplied.
- phone_format string? - In a phone field, the phone number type: US or International.
- date_format string? - In a date or birthday field, the format of the date.
- choices string[]? - In a radio or dropdown non-group field, the available options for members to pick from.
- size int? - In a text field, the default length of the text field.
mailchimp: MergeFieldOptions1
Extra options for some merge field types.
Fields
- default_country int? - In an address field, the default country code if none supplied.
- phone_format string? - In a phone field, the phone number type: US or International.
- date_format string? - In a date or birthday field, the format of the date.
- choices string[]? - In a radio or dropdown non-group field, the available options for members to pick from.
mailchimp: MergeFieldOptions2
Extra options for some merge field types.
Fields
- default_country int? - In an address field, the default country code if none supplied.
- phone_format string? - In a phone field, the phone number type: US or International.
- date_format string? - In a date or birthday field, the format of the date.
- choices string[]? - In a radio or dropdown non-group field, the available options for members to pick from.
- size int? - In a text field, the default length of the text field.
mailchimp: Notes
The most recent Note added about this member.
Fields
- note_id int? - The note id.
- created_at string? - The date and time the note was created in ISO 8601 format.
- created_by string? - The author of the note.
- note string? - The content of the note.
mailchimp: PartialMatches
Partial matches of the provided search query.
Fields
- members ListMembers3[]? - An array of objects, each representing a specific list member.
- total_items int? - The total number of items matching the query regardless of pagination.
mailchimp: ProblemDetailDocument
An error generated by the Mailchimp API. Conforms to IETF draft 'draft-nottingham-http-problem-06'.
Fields
- 'type string - An absolute URI that identifies the problem type. When dereferenced, it should provide human-readable documentation for the problem type.
- title string - A short, human-readable summary of the problem type. It shouldn't change based on the occurrence of the problem, except for purposes of localization.
- status int - The HTTP status code (RFC2616, Section 6) generated by the origin server for this occurrence of the problem.
- detail string - A human-readable explanation specific to this occurrence of the problem. Learn more about errors.
- instance string - A string that identifies this specific occurrence of the problem. Please provide this ID when contacting support.
mailchimp: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
mailchimp: RemovedSubscribers
A summary of the subscribers who were removed from an Automation workflow.
Fields
- workflow_id string? - A string that uniquely identifies an Automation workflow.
- subscribers SubscriberRemovedFromAutomationWorkflow1[]? - An array of objects, each representing a subscriber who was removed from an Automation workflow.
- total_items int? - The total number of items matching the query regardless of pagination.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: ResourceLink
This object represents a link from the resource where it is found to another resource or action that may be performed.
Fields
- rel string? - As with an HTML 'rel' attribute, this describes the type of link.
- href string? - This property contains a fully-qualified URL that can be called to retrieve the linked resource or perform the linked action.
- method string? - The HTTP method that should be used when accessing the URL defined in 'href'.
- targetSchema string? - For GETs, this is a URL representing the schema that the response should conform to.
- schema string? - For HTTP methods that can receive bodies (POST and PUT), this is a URL representing the schema that the body should conform to.
mailchimp: RssOptions
RSS options, specific to an RSS campaign.
Fields
- feed_url string - The URL for the RSS feed.
- frequency string - The frequency of the RSS Campaign.
- schedule SendingSchedule? - The schedule for sending the RSS Campaign.
- constrain_rss_img boolean? - Whether to add CSS to images in the RSS feed to constrain their width in campaigns.
mailchimp: RssOptions1
RSS options for a campaign.
Fields
- feed_url string - The URL for the RSS feed.
- frequency string - The frequency of the RSS Campaign.
- schedule SendingSchedule? - The schedule for sending the RSS Campaign.
- constrain_rss_img boolean? - Whether to add CSS to images in the RSS feed to constrain their width in campaigns.
mailchimp: RssOptions2
RSS options for a campaign.
Fields
- feed_url string? - The URL for the RSS feed.
- frequency string? - The frequency of the RSS Campaign.
- schedule SendingSchedule? - The schedule for sending the RSS Campaign.
- last_sent string? - The date the campaign was last sent.
- constrain_rss_img boolean? - Whether to add CSS to images in the RSS feed to constrain their width in campaigns.
mailchimp: RssOptions3
RSS options for a campaign.
Fields
- feed_url string - The URL for the RSS feed.
- frequency string - The frequency of the RSS Campaign.
- schedule SendingSchedule1? - The schedule for sending the RSS Campaign.
- last_sent string? - The date the campaign was last sent.
- constrain_rss_img boolean? - Whether to add CSS to images in the RSS feed to constrain their width in campaigns.
mailchimp: SalesforceCrmTracking
Deprecated
Fields
- campaign boolean? - Create a campaign in a connected Salesforce account.
- notes boolean? - Update contact notes for a campaign based on subscriber email addresses.
mailchimp: SalesforceCrmTracking1
Deprecated
Fields
- campaign boolean? - Create a campaign in a connected Salesforce account.
- notes boolean? - Update contact notes for a campaign based on a subscriber's email address.
mailchimp: SegmentIdMembersBody
Fields
- email_address string - Email address for a subscriber.
mailchimp: SegmentMembers
View members in a specific list segment.
Fields
- members ListMembers4[]? - An array of objects, each representing a specific list member.
- total_items int? - The total number of items matching the query regardless of pagination.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: SegmentOptions
An object representing all segmentation options. This object should contain a saved_segment_id
to use an existing segment, or you can create a new segment by including both match
and conditions
options.
Fields
- saved_segment_id int? - The id for an existing saved segment.
- 'match string? - Segment match type.
- conditions record {}[]? - Segment match conditions. There are multiple possible types, see the condition types documentation.
mailchimp: SegmentOptions1
An object representing all segmentation options. This object should contain a saved_segment_id
to use an existing segment, or you can create a new segment by including both match
and conditions
options.
Fields
- saved_segment_id int? - The id for an existing saved segment.
- prebuilt_segment_id string? - The prebuilt segment id, if a prebuilt segment has been designated for this campaign.
- 'match string? - Segment match type.
- conditions record {}[]? - Segment match conditions. There are multiple possible types, see the condition types documentation.
mailchimp: SegmentOptions2
An object representing all segmentation options.
Fields
- saved_segment_id int? - The id for an existing saved segment.
- 'match string? - Segment match type.
- conditions record {}[]? - Segment match conditions. There are multiple possible types, see the condition types documentation.
mailchimp: SendChecklist
The send checklist for the campaign.
Fields
- is_ready boolean? - Whether the campaign is ready to send.
- items SendChecklistItems[]? - A list of feedback items to review before sending your campaign.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: SendChecklistItems
Fields
- 'type string? - The item type.
- id int? - The ID for the specific item.
- heading string? - The heading for the specific item.
- details string? - Details about the specific feedback item.
mailchimp: SendingSchedule
The schedule for sending the RSS Campaign.
Fields
- hour int? - The hour to send the campaign in local time. Acceptable hours are 0-23. For example, '4' would be 4am in your account's default time zone.
- daily_send DailySendingDays? - The days of the week to send a daily RSS Campaign.
- weekly_send_day string? - The day of the week to send a weekly RSS Campaign.
- monthly_send_date decimal? - The day of the month to send a monthly RSS Campaign. Acceptable days are 0-31, where '0' is always the last day of a month. Months with fewer than the selected number of days will not have an RSS campaign sent out that day. For example, RSS Campaigns set to send on the 30th will not go out in February.
mailchimp: SendingSchedule1
The schedule for sending the RSS Campaign.
Fields
- hour int? - The hour to send the campaign in local time. Acceptable hours are 0-23. For example, '4' would be 4am in your account's default time zone.
- daily_send DailySendingDays? - The days of the week to send a daily RSS Campaign.
- weekly_send_day string? - The day of the week to send a weekly RSS Campaign.
- monthly_send_date decimal? - The day of the month to send a monthly RSS Campaign. Acceptable days are 0-31, where '0' is always the last day of a month. Months with fewer than the selected number of days will not have an RSS campaign sent out that day. For example, RSS Campaigns set to send on the 30th will not go out in February.
mailchimp: SignupForm
List signup form.
Fields
- header SignupFormHeaderOptions? - Options for customizing your signup form header.
- contents CollectionOfContentForListSignupForms[]? - The signup form body content.
- styles CollectionOfElementStyleForListSignupForms[]? - An array of objects, each representing an element style for the signup form.
mailchimp: SignupForm1
List signup form.
Fields
- header SignupFormHeaderOptions? - Options for customizing your signup form header.
- contents CollectionOfContentForListSignupForms[]? - The signup form body content.
- styles CollectionOfElementStyleForListSignupForms[]? - An array of objects, each representing an element style for the signup form.
- signup_form_url string? - Signup form URL.
- list_id string? - The signup form's list id.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: SignupForm2
List signup form.
Fields
- header SignupFormHeaderOptions? - Options for customizing your signup form header.
- contents CollectionOfContentForListSignupForms[]? - The signup form body content.
- styles CollectionOfElementStyleForListSignupForms[]? - An array of objects, each representing an element style for the signup form.
- signup_form_url string? - Signup form URL.
- list_id string? - The signup form's list id.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: SignupFormHeaderOptions
Options for customizing your signup form header.
Fields
- image_url string? - Header image URL.
- text string? - Header text.
- image_width string? - Image width, in pixels.
- image_height string? - Image height, in pixels.
- image_alt string? - Alt text for the image.
- image_link string? - The URL that the header image will link to.
- image_align string? - Image alignment.
- image_border_width string? - Image border width.
- image_border_style string? - Image border style.
- image_border_color string? - Image border color.
- image_target string? - Image link target.
mailchimp: Sources
The possible sources of any events that can trigger the webhook and whether they are enabled.
Fields
- user boolean? - Whether the webhook is triggered by subscriber-initiated actions.
- admin boolean? - Whether the webhook is triggered by admin-initiated actions in the web interface.
- api boolean? - Whether the webhook is triggered by actions initiated via the API.
mailchimp: Sources1
The possible sources of any events that can trigger the webhook and whether they are enabled.
Fields
- user boolean? - Whether the webhook is triggered by subscriber-initiated actions.
- admin boolean? - Whether the webhook is triggered by admin-initiated actions in the web interface.
- api boolean? - Whether the webhook is triggered by actions initiated via the API.
mailchimp: Statistics
Stats for the list. Many of these are cached for at least five minutes.
Fields
- member_count int? - The number of active members in the list.
- total_contacts int? - The number of contacts in the list, including subscribed, unsubscribed, pending, cleaned, deleted, transactional, and those that need to be reconfirmed. Requires include_total_contacts query parameter to be included.
- unsubscribe_count int? - The number of members who have unsubscribed from the list.
- cleaned_count int? - The number of members cleaned from the list.
- member_count_since_send int? - The number of active members in the list since the last campaign was sent.
- unsubscribe_count_since_send int? - The number of members who have unsubscribed since the last campaign was sent.
- cleaned_count_since_send int? - The number of members cleaned from the list since the last campaign was sent.
- campaign_count int? - The number of campaigns in any status that use this list.
- campaign_last_sent string? - The date and time the last campaign was sent to this list in ISO 8601 format. This is updated when a campaign is sent to 10 or more recipients.
- merge_field_count int? - The number of merge vars for this list (not EMAIL, which is required).
- avg_sub_rate decimal? - The average number of subscriptions per month for the list (not returned if we haven't calculated it yet).
- avg_unsub_rate decimal? - The average number of unsubscriptions per month for the list (not returned if we haven't calculated it yet).
- target_sub_rate decimal? - The target number of subscriptions per month for the list to keep it growing (not returned if we haven't calculated it yet).
- open_rate decimal? - The average open rate (a percentage represented as a number between 0 and 100) per campaign for the list (not returned if we haven't calculated it yet).
- click_rate decimal? - The average click rate (a percentage represented as a number between 0 and 100) per campaign for the list (not returned if we haven't calculated it yet).
- last_sub_date string? - The date and time of the last time someone subscribed to this list in ISO 8601 format.
- last_unsub_date string? - The date and time of the last time someone unsubscribed from this list in ISO 8601 format.
mailchimp: SubscriberInAutomationQueue
Information about subscribers in an Automation email queue.
Fields
- email_address string - The list member's email address.
mailchimp: SubscriberInAutomationQueue1
Information about subscribers in an Automation email queue.
Fields
- id string? - The MD5 hash of the lowercase version of the list member's email address.
- workflow_id string? - A string that uniquely identifies an Automation workflow.
- email_id string? - A string that uniquely identifies an email in an Automation workflow.
- list_id string? - A string that uniquely identifies a list.
- list_is_active boolean? - The status of the list used, namely if it's deleted or disabled.
- email_address string? - The list member's email address.
- next_send string? - The date and time of the next send for the workflow email in ISO 8601 format.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: SubscriberInAutomationQueue2
Information about subscribers in an Automation email queue.
Fields
- email_address string - The list member's email address.
mailchimp: SubscriberInAutomationQueue3
Information about subscribers in an Automation email queue.
Fields
- id string? - The MD5 hash of the lowercase version of the list member's email address.
- workflow_id string? - A string that uniquely identifies an Automation workflow.
- email_id string? - A string that uniquely identifies an email in an Automation workflow.
- list_id string? - A string that uniquely identifies a list.
- email_address string - The list member's email address.
- next_send string? - The date and time of the next send for the workflow email in ISO 8601 format.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: SubscriberList
Information about a specific list.
Fields
- name string - The name of the list.
- contact ListContact - Contact information displayed in campaign footers to comply with international spam laws.
- permission_reminder string - The permission reminder for the list.
- use_archive_bar boolean(default false) - Whether campaigns for this list use the Archive Bar in archives by default.
- campaign_defaults CampaignDefaults - Default values for campaigns created for this list.
- notify_on_subscribe string(default "false") - The email address to send subscribe notifications to.
- notify_on_unsubscribe string(default "false") - The email address to send unsubscribe notifications to.
- email_type_option boolean - Whether the list supports multiple formats for emails. When set to
true
, subscribers can choose whether they want to receive HTML or plain-text emails. When set tofalse
, subscribers will receive HTML emails, with a plain-text alternative backup.
- double_optin boolean(default false) - Whether or not to require the subscriber to confirm subscription via email.
- marketing_permissions boolean(default false) - Whether or not the list has marketing permissions (eg. GDPR) enabled.
mailchimp: SubscriberList1
Information about a specific list.
Fields
- id string? - A string that uniquely identifies this list.
- web_id int? - The ID used in the Mailchimp web application. View this list in your Mailchimp account at
https://{dc}.admin.mailchimp.com/lists/members/?id={web_id}
.
- name string? - The name of the list.
- contact ListContact2? - Contact information displayed in campaign footers to comply with international spam laws.
- permission_reminder string? - The permission reminder for the list.
- use_archive_bar boolean? - Whether campaigns for this list use the Archive Bar in archives by default.
- campaign_defaults CampaignDefaults1? - Default values for campaigns created for this list.
- notify_on_subscribe string? - The email address to send subscribe notifications to.
- notify_on_unsubscribe string? - The email address to send unsubscribe notifications to.
- date_created string? - The date and time that this list was created in ISO 8601 format.
- list_rating int? - An auto-generated activity score for the list (0-5).
- email_type_option boolean? - Whether the list supports multiple formats for emails. When set to
true
, subscribers can choose whether they want to receive HTML or plain-text emails. When set tofalse
, subscribers will receive HTML emails, with a plain-text alternative backup.
- subscribe_url_short string? - Our url shortened version of this list's subscribe form.
- subscribe_url_long string? - The full version of this list's subscribe form (host will vary).
- beamer_address string? - The list's Email Beamer address.
- visibility string? - Legacy - visibility settings are no longer used
- double_optin boolean? - Whether or not to require the subscriber to confirm subscription via email.
- has_welcome boolean? - Whether or not this list has a welcome automation connected. Welcome Automations: welcomeSeries, singleWelcome, emailFollowup.
- marketing_permissions boolean? - Whether or not the list has marketing permissions (eg. GDPR) enabled.
- modules string[]? - Any list-specific modules installed for this list.
- stats Statistics? - Stats for the list. Many of these are cached for at least five minutes.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: SubscriberList2
Information about a specific list.
Fields
- name string - The name of the list.
- contact ListContact1 - Contact information displayed in campaign footers to comply with international spam laws.
- permission_reminder string - The permission reminder for the list.
- use_archive_bar boolean(default false) - Whether campaigns for this list use the Archive Bar in archives by default.
- campaign_defaults CampaignDefaults - Default values for campaigns created for this list.
- notify_on_subscribe string(default "false") - The email address to send subscribe notifications to.
- notify_on_unsubscribe string(default "false") - The email address to send unsubscribe notifications to.
- email_type_option boolean - Whether the list supports multiple formats for emails. When set to
true
, subscribers can choose whether they want to receive HTML or plain-text emails. When set tofalse
, subscribers will receive HTML emails, with a plain-text alternative backup.
- double_optin boolean(default false) - Whether or not to require the subscriber to confirm subscription via email.
- marketing_permissions boolean(default false) - Whether or not the list has marketing permissions (eg. GDPR) enabled.
mailchimp: SubscriberList3
Information about a specific list.
Fields
- id string? - A string that uniquely identifies this list.
- web_id int? - The ID used in the Mailchimp web application. View this list in your Mailchimp account at
https://{dc}.admin.mailchimp.com/lists/members/?id={web_id}
.
- name string? - The name of the list.
- contact ListContact2? - Contact information displayed in campaign footers to comply with international spam laws.
- permission_reminder string? - The permission reminder for the list.
- use_archive_bar boolean? - Whether campaigns for this list use the Archive Bar in archives by default.
- campaign_defaults CampaignDefaults1? - Default values for campaigns created for this list.
- notify_on_subscribe string? - The email address to send subscribe notifications to.
- notify_on_unsubscribe string? - The email address to send unsubscribe notifications to.
- date_created string? - The date and time that this list was created in ISO 8601 format.
- list_rating int? - An auto-generated activity score for the list (0-5).
- email_type_option boolean? - Whether the list supports multiple formats for emails. When set to
true
, subscribers can choose whether they want to receive HTML or plain-text emails. When set tofalse
, subscribers will receive HTML emails, with a plain-text alternative backup.
- subscribe_url_short string? - Our url shortened version of this list's subscribe form.
- subscribe_url_long string? - The full version of this list's subscribe form (host will vary).
- beamer_address string? - The list's Email Beamer address.
- visibility string? - Legacy - visibility settings are no longer used
- double_optin boolean? - Whether or not to require the subscriber to confirm subscription via email.
- has_welcome boolean? - Whether or not this list has a welcome automation connected. Welcome Automations: welcomeSeries, singleWelcome, emailFollowup.
- marketing_permissions boolean? - Whether or not the list has marketing permissions (eg. GDPR) enabled.
- modules string[]? - Any list-specific modules installed for this list.
- stats Statistics? - Stats for the list. Many of these are cached for at least five minutes.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: SubscriberLists
A collection of subscriber lists for this account. Lists contain subscribers who have opted-in to receive correspondence from you or your organization.
Fields
- lists SubscriberList3[] - An array of objects, each representing a list.
- total_items int? - The total number of items matching the query regardless of pagination.
- constraints CollectionAuthorization? - Do particular authorization constraints around this collection limit creation of new instances?
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: SubscriberRemovedFromAutomationWorkflow
A summary of a subscriber removed from an Automation workflow.
Fields
- id string? - The MD5 hash of the lowercase version of the list member's email address.
- workflow_id string? - A string that uniquely identifies an Automation workflow.
- list_id string? - A string that uniquely identifies a list.
- email_address string? - The list member's email address.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: SubscriberRemovedFromAutomationWorkflow1
A summary of a subscriber removed from an Automation workflow.
Fields
- id string? - The MD5 hash of the lowercase version of the list member's email address.
- workflow_id string? - A string that uniquely identifies an Automation workflow.
- list_id string? - A string that uniquely identifies a list.
- email_address string? - The list member's email address.
- _links ResourceLink[]? - A list of link types and descriptions for the API schema documents.
mailchimp: SubscriberStats
Open and click rates for this subscriber.
Fields
- avg_open_rate decimal? - A subscriber's average open rate.
- avg_click_rate decimal? - A subscriber's average clickthrough rate.
- ecommerce_data EcommerceStats? - Ecommerce stats for the list member if the list is attached to a store.
mailchimp: SubscriberStats1
Open and click rates for this subscriber.
Fields
- avg_open_rate decimal? - A subscriber's average open rate.
- avg_click_rate decimal? - A subscriber's average clickthrough rate.
mailchimp: TagSearchResults
A list of tags matching the input query.
Fields
- tags TagSearchResultsTags[]? - A list of matching tags.
- total_items int? - The total number of items matching the query regardless of pagination.
mailchimp: TagSearchResultsTags
Fields
- id int? - The unique id for the tag.
- name string? - The name of the tag.
mailchimp: TemplateContent
Use this template to generate the HTML content of the campaign
Fields
- id int - The id of the template to use.
- sections record {}? - Content for the sections of the template. Each key should be the unique mc:edit area name from the template.
mailchimp: TemplateContent1
Use this template to generate the HTML content for the campaign.
Fields
- id int - The id of the template to use.
- sections record {}? - Content for the sections of the template. Each key should be the unique mc:edit area name from the template.
mailchimp: UpdateInformationAboutASpecificWorkflowEmail
Update information about an individual Automation workflow email.
Fields
- settings CampaignSettings? - Settings for the campaign including the email subject, from name, and from email address.
- delay AutomationDelay? - The delay settings for an automation email.
mailchimp: UploadArchive
Available when uploading an archive to create campaign content. The archive should include all campaign content and images. Learn more.
Fields
- archive_content string - The base64-encoded representation of the archive file.
- archive_type string? - The type of encoded file. Defaults to zip.
mailchimp: VerifiedDomains
The verified domains currently on the account.
Fields
- domain string? - The name of this domain.
- verified boolean? - Whether the domain has been verified for sending.
- authenticated boolean? - Whether domain authentication is enabled for this domain.
- verification_email string? - The e-mail address receiving the two-factor challenge for this domain.
- verification_sent string? - The date/time that the two-factor challenge was sent to the verification email.
mailchimp: VerifiedDomains1
The verified domains currently on the account.
Fields
- domains VerifiedDomains3[]? - The domains on the account
- total_items int? - The total number of items matching the query regardless of pagination.
mailchimp: VerifiedDomains2
The verified domains currently on the account.
Fields
- verification_email string - The e-mail address at the domain you want to verify. This will receive a two-factor challenge to be used in the verify action.
mailchimp: VerifiedDomains3
The verified domains currently on the account.
Fields
- domain string? - The name of this domain.
- verified boolean? - Whether the domain has been verified for sending.
- authenticated boolean? - Whether domain authentication is enabled for this domain.
- verification_email string? - The e-mail address receiving the two-factor challenge for this domain.
- verification_sent string? - The date/time that the two-factor challenge was sent to the verification email.
Import
import ballerinax/mailchimp;
Metadata
Released date: almost 2 years ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 2528
Current verison: 303
Weekly downloads
Keywords
Marketing/Email Newsletters
Cost/Freemium
Contributors