livestorm
Module livestorm
API
Definitions
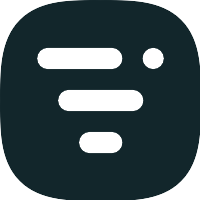
ballerinax/livestorm Ballerina library
Overview
This is a generated connector for Livestorm API v1 OpenAPI specification.
Livestorm is a browser based online web conferencing software used to share real-time live streams. It can be used to power remote live meetings, product demos, sales webinars, online lessons, onboarding sessions, more.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create Livestorm Account
- Obtaining tokens
- Log into Livestorm Account
- Click the
Account settings
- Navigate to
Integration
and choosePublic API
- Click
Generate a token
to create a token
Quickstart
Step 1: Import connector
Import the ballerinax/livestorm module into the Ballerina project.
import ballerinax/livestorm;
Step 2: Create a new connector instance
livestorm:ApiKeysConfig config = { authorization : "<your apiKey>" }; livestorm:Client myclient = check new livestorm:Client(config, {}, "https://api.livestorm.co/v1");
Step 3: Invoke connector operation
- You can get list of events created with
listEvents
method.livestorm:InlineResponse200 result = check myclient->listEvents();
- Use
bal run
command to compile and run the Ballerina program.
Clients
livestorm: Client
This is a generated connector for Livestorm API v1 OpenAPI specification. Client endpoint for Livestorm Public API. Livestorm is a browser based online web conferencing software used to share real-time live streams. It can be used to power remote live meetings, product demos, sales webinars, online lessons, onboarding sessions, more. In addition, all request and response bodies, including errors, are encoded in JSON format.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create an Livestorm Account and obtain tokens following this guide.
init (ApiKeysConfig apiKeyConfig, ConnectionConfig config, string serviceUrl)
- apiKeyConfig ApiKeysConfig - API keys for authorization
- config ConnectionConfig {} - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.livestorm.co/v1" - URL of the target service
listEvents
function listEvents(string? pageNumber, string? pageSize, string? filterTitle, string? filterEveryoneCanSpeak) returns InlineResponse200|error
List Events
Parameters
- pageNumber string? (default ()) - Page index to be returned
- pageSize string? (default ()) - Number of record to be returned by page
- filterTitle string? (default ()) - Filter events by title
- filterEveryoneCanSpeak string? (default ()) - Filter events by everyone can speak
Return Type
- InlineResponse200|error - Fetch List
createEvent
function createEvent(byte[] payload) returns InlineResponse201|error
Create a new event
Parameters
- payload byte[] - Request payload to create event
Return Type
- InlineResponse201|error - Create event response
getEventByID
function getEventByID(string id) returns InlineResponse2001|error
Retrieve an Event
Parameters
- id string - Event ID
Return Type
- InlineResponse2001|error - Get detail
listSessionByEvent
function listSessionByEvent(string id, string? pageNumber, string? pageSize, string? filterStatus, string? filterDateFrom, string? filterDateTo, string[]? include) returns InlineResponse2002|error
List Sessions for an Event
Parameters
- id string - Event ID
- pageNumber string? (default ()) - Page index to be returned
- pageSize string? (default ()) - Number of record to be returned by page
- filterStatus string? (default ()) - Filter Sessions by status : 'upcoming', 'live', 'on_demand', 'past', 'past_not_started', 'canceled', 'draft'
- filterDateFrom string? (default ()) - Filter Sessions which ‘estimated_started_at’ attribute starts from the given date (expressed as a Unix timestamp or an ISO 8601 date).
- filterDateTo string? (default ()) - Filter Sessions which ‘estimated_started_at’ attribute ends with the given date (expressed as a Unix timestamp or an ISO 8601 date).
- include string[]? (default ()) - Include Related Data
Return Type
- InlineResponse2002|error - Fetch List
createEventSession
function createEventSession(string id, byte[] payload) returns InlineResponse2011|error
Create a new event session
Return Type
- InlineResponse2011|error - Create event session response
listSessionPeople
function listSessionPeople(string id, string? pageNumber, string? pageSize, string? filterRole, boolean? filterAttended, string? filterEmail, string[]? include) returns InlineResponse2003|error
List People for a Session
Parameters
- id string - Session ID
- pageNumber string? (default ()) - Page index to be returned
- pageSize string? (default ()) - Number of record to be returned by page
- filterRole string? (default ()) - Filter People by role : 'participant', 'team_member'
- filterAttended boolean? (default ()) - Filter People that attend or not the Session
- filterEmail string? (default ()) - Filter People by their email (exact match)
- include string[]? (default ()) - Include Related Data
Return Type
- InlineResponse2003|error - Fetch List
registerPeopleForSession
function registerPeopleForSession(string id, byte[] payload) returns InlineResponse2012|error
Register a participant to a Session
Return Type
- InlineResponse2012|error - Register participant response
Records
livestorm: ApiKeysConfig
Provides API key configurations needed when communicating with a remote HTTP endpoint.
Fields
- authorization string - All requests on the Livestorm API needs to include an API key. The API key can be provided as part of the query string or as a request header. The name of the API key needs to be
Authorization
.
livestorm: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
livestorm: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
livestorm: Event
Event response
Fields
- id string? - Event ID
- 'type string? - Event type
- attributes EventAttribute? - Event attributes
livestorm: EventAttribute
Event attributes
Fields
- title string? - Event title
- slug string? - Event slug
- registration_link string? - Event title
- estimated_duration int? - Estimated duration
- registration_page_enabled boolean? - Registration page enabled or not
- everyone_can_speak boolean? - Everyone can speack or not
- description string? - Description about event
- language string? - Event language
- published_at int? - Updated timestamp
- created_at int? - Created timestamp
- updated_at int? - Updated timestamp
- owner Owner? - Owner details
- sessions_count int? - Number of sessions
- fields Field[]? - Fields available
livestorm: EventData
Event post data
Fields
- 'type string? - Event type
- attributes EventRequestAttribute? - Event request attributes
- relationships EventRelationship? - Event releationship
livestorm: EventRelationship
Event releationship
Fields
- sessions Session[]? - Event session
livestorm: EventRequestAttribute
Event request attributes
Fields
- copy_from_event_id string? - Event ID to copy
- owner_id string? - Owner ID
- title string? - Event title
- slug string? - Event slug
livestorm: EventsBody
PosteventsRequest
Fields
- data EventData? - Event post data
livestorm: Field
Event fields
Fields
- id string? - Event field ID
- 'type string? - Type of fields
- 'order int? - Order of fields
- required boolean? - Required or not
livestorm: IdPeopleBody
RegisterPeopleRequest
Fields
- data PeopleData? - Add People data
livestorm: IdSessionsBody
PostSessionRequest
Fields
- data SessionData? - Add Session data
livestorm: InlineResponse200
Fields
- data Event[] - Event Data
- meta Meta? - Metadata
livestorm: InlineResponse2001
Get an event by ID response
Fields
- data Event? - Event response
livestorm: InlineResponse2002
Fields
- data InlineResponse2002Data[] - Data
- meta Meta? - Metadata
livestorm: InlineResponse2002Attributes
Attributes
Fields
- event_type_id string? -
- status string? -
- timezone string? -
- room_link string? -
- attendees_count int? -
- duration int? -
- estimated_started_at int? -
- started_at int? -
- ended_at int? -
- canceled_at int? -
- created_at int? -
- updated_at int? -
- registrants_count int? -
livestorm: InlineResponse2002Data
Fields
- id string? - Event ID
- 'type string? - Event type
- attributes InlineResponse2002Attributes? - Attributes
livestorm: InlineResponse2003
Fields
- data InlineResponse2003Data[] - Data
- meta Meta? - Metadata
livestorm: InlineResponse2003Attributes
Attributes
Fields
- role string? -
- created_at int? -
- updated_at int? -
- timezone string? -
- first_name string? -
- last_name string? -
- email string? -
- avatar_link string? -
- registrant_detail InlineResponse2003AttributesRegistrantDetail? -
- messages_count int? -
- questions_count int? -
- votes_count int? -
- up_votes_count int? -
livestorm: InlineResponse2003AttributesRegistrantDetail
Fields
- event_id string? -
- created_at int? -
- updated_at int? -
livestorm: InlineResponse2003AttributesRegistrantDetailFields
Fields
- id string? -
- 'type string? -
- value string? -
- required boolean? -
livestorm: InlineResponse2003Data
Fields
- id string? - Event ID
- 'type string? - Event type
- attributes InlineResponse2003Attributes? - Attributes
livestorm: InlineResponse201
Create new event response
Fields
- data Event? - Event response
livestorm: InlineResponse2011
Create session response
Fields
- data Session? - Event session
livestorm: InlineResponse2012
Register participant response
Fields
- data People? - Event session
livestorm: Meta
Metadata
Fields
- record_count int? - Total record count
- page_count int? - Page count
- items_per_page int? - Items per page
livestorm: Owner
Owner details
Fields
- id string? - Owner ID
- 'type string? - Type
- attributes OwnerAttribute? - Owner attributes
livestorm: OwnerAttribute
Owner attributes
Fields
- role string? - Role of owner
- created_at int? - Created timestamp
- updated_at int? - Updated timestamp
- timezone string? - Timezone
- first_name string? - First name
- last_name string? - Last name
- email string? - Email
- avatar_link string? - Avatar link
livestorm: People
Event session
Fields
- 'type string? - People type
- id string? - People ID
- attributes PeopleAttribute? - People attribute
livestorm: PeopleAttribute
People attribute
Fields
- role string? - Role assigned to
- created_at int? - Created time
- updated_at int? - Updated time
- timezone string? - Time zone
- first_name string? - First name
- last_name string? - Last name
- email string? - Email address
- avatar_link string? - Avatar url link
livestorm: PeopleData
Add People data
Fields
- 'type string? - Event type
- attributes PeopleRequestAttribute? - People request attributes
livestorm: PeopleRequestAttribute
People request attributes
Fields
- referrer string? - Referrer detail
- utm_source string? - UTM tracking source
- utm_medium string? - UTM medium
- utm_term string? - UTM term
- utm_content string? - UTM content
- utm_campaign string? - UTM campaign
livestorm: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
livestorm: Session
Event session
Fields
- 'type string? - Session type
- id string? - Session ID
- attributes SessionAttribute? - Event session attribute
livestorm: SessionAttribute
Event session attribute
Fields
- event_type_id string? - Event type ID
- status string? - Session status
- timezone string? - Time zone
- room_link string? - Url link to room
- attendees_count string? - Number of Attendees
- duration string? - Session duration
- estimated_started_at string? - Estimated started time
- started_at string? - Started time
- ended_at string? - Ended time
- canceled_at string? - Canceled time
- created_at int? - Created time
- updated_at int? - Updated time
- registrants_count string? - Number of registrants
livestorm: SessionData
Add Session data
Fields
- 'type string? - Event type
- attributes SessionRequestAttribute? - Session request attributes
- relationships SessionRelationship? - Session releationship
livestorm: SessionRelationship
Session releationship
Fields
- people People[]? - People
livestorm: SessionRequestAttribute
Session request attributes
Fields
- estimated_started_at string? - Estimated started time
- timezone string? - Time zone
Import
import ballerinax/livestorm;
Metadata
Released date: over 1 year ago
Version: 2.6.0
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
Pull count
Total: 847
Current verison: 2
Weekly downloads
Keywords
Communication/Video Conferencing
Cost/Freemium
Contributors
Dependencies