listen.notes
Module listen.notes
Definitions
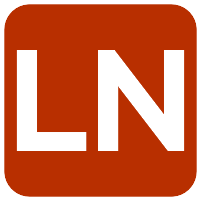
ballerinax/listen.notes Ballerina library
Overview
This is a generated connector for Listen Notes API v2.0 OpenAPI specification.
Simple & no-nonsense podcast search & directory API. Search all podcasts and episodes by people, places, or topics.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Listen Notes account.
- Obtain tokens by following this guide.
Quickstart
To use the Listen Notes connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/listen.notes
module into the Ballerina project.
import ballerinax/listen.notes;
Step 2: Create a new connector instance
Create a notes:ApiKeysConfig
with the <API_KEY>
obtained, and initialize the connector with it.
notes:ApiKeysConfig apiKeyConfig = { xListenapiKey: `<API_KEY>` }; notes:Client baseClient = check new Client(apiKeyConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example to search using the connector.
public function main() { string q = "game of thrones"; notes:SearchResponse|error response = baseClient->search(q); if (response is notes:SearchResponse) { log:printInfo(response.toString()); } else { log:printError(response.message()); } }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
listen.notes: Client
This is a generated connector for Listen Notes API v2.0 OpenAPI specification. Simple & no-nonsense podcast search & directory API. Search all podcasts and episodes by people, places, or topics.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Listen Notes account and obtain tokens by following this guide.
init (ApiKeysConfig apiKeyConfig, ConnectionConfig config, string serviceUrl)
- apiKeyConfig ApiKeysConfig - API keys for authorization
- config ConnectionConfig {} - The configurations to be used when initializing the
connector
- serviceUrl string "https://listen-api.listennotes.com/api/v2" - URL of the target service
search
function search(string q, int sortByDate, string 'type, int offset, int lenMin, int? lenMax, int? episodeCountMin, int? episodeCountMax, string? genreIds, int? publishedBefore, int publishedAfter, string onlyIn, string? language, string? region, string? ocid, string? ncid, int safeMode) returns SearchResponse|error
Full-text search
Parameters
- q string - Search term, e.g., person, place, topic... You can use double quotes to do verbatim match, e.g., "game of thrones". Otherwise, it's fuzzy search.
- sortByDate int (default 0) - Sort by date or not? If 0, then sort by relevance. If 1, then sort by date.
- 'type string (default "episode") - What type of contents do you want to search for?
- offset int (default 0) - Offset for search results, for pagination. You'll use next_offset from response for this parameter.
- lenMin int (default 0) - Minimum audio length in minutes. Applicable only when type parameter is episode.
- lenMax int? (default ()) - Maximum audio length in minutes. Applicable only when type parameter is episode.
- episodeCountMin int? (default ()) - Minimum number of episodes. Applicable only when type parameter is podcast.
- episodeCountMax int? (default ()) - Maximum number of episodes. Applicable only when type parameter is podcast.
- genreIds string? (default ()) - A comma-delimited string of a list of genre ids. If not specified, then all genres are included. You can find the id and the name of all genres from
GET /genres
. It works only when type is episode or podcast.
- publishedBefore int? (default ()) - Only show episodes/podcasts/curated lists published before this timestamp (in milliseconds). If published_before & published_after are used at the same time, published_before should be bigger than published_after.
- publishedAfter int (default 0) - Only show episodes/podcasts/curated lists published after this timestamp (in milliseconds). If published_before & published_after are used at the same time, published_before should be bigger than published_after.
- onlyIn string (default "title,description,author,audio") - A comma-delimited string to search only in specific fields. Allowed values are title, description, author, and audio. If not specified, then search every fields.
- language string? (default ()) - Limit search results to a specific language. If not specified, it'll be any language. You can get a list of supported languages from
GET /languages
. It works only when type is episode or podcast.
- region string? (default ()) - Limit search results to a specific region (e.g., us, gb, in...). If not specified, it'll be any region. You can get the supported country codes from
GET /regions
. It works only when type is episode or podcast.
- ocid string? (default ()) - A comma-delimited string of podcast ids (up to 5 podcasts) - you can get a podcast id from the podcast_id field in response. This parameter is to limit search results from only a few specific podcasts. It works only when type is episode.
- ncid string? (default ()) - A comma-delimited string of podcast ids (up to 5 podcasts) - you can get a podcast id from the podcast_id field in response. This parameter is to exclude search results of a few specific podcasts. It works only when type is episode.
- safeMode int (default 0) - Whether or not to exclude podcasts/episodes with explicit language. 1 is yes and 0 is no. It works only when type is episode or podcast.
Return Type
- SearchResponse|error - OK
typeahead
function typeahead(string q, int showPodcasts, int showGenres, int safeMode) returns TypeaheadResponse|error
Typeahead search
Parameters
- q string - Search term, e.g., person, place, topic... You can use double quotes to do verbatim match, e.g., "game of thrones". Otherwise, it's fuzzy search.
- showPodcasts int (default 0) - Autosuggest podcasts. This only searches podcast title and publisher and returns very limited info of 5 podcasts. 1 is yes, 0 is no. It's a bit slow to autosuggest podcasts, so we turn it off by default. If show_podcasts=1, you can also pass iTunes id (e.g., 474722933) to the q parameter to fetch podcast meta data.
- showGenres int (default 0) - Whether or not to autosuggest genres. 1 is yes, 0 is no.
- safeMode int (default 0) - Whether or not to exclude podcasts/episodes with explicit language. 1 is yes and 0 is no. It works only when show_podcasts is 1.
Return Type
- TypeaheadResponse|error - OK
getBestPodcasts
function getBestPodcasts(string? genreId, int? page, string region, string? publisherRegion, string? language, int safeMode) returns BestPodcastsResponse|error
Fetch a list of best podcasts by genre
Parameters
- genreId string? (default ()) - You can get the id from
GET /genres
. If not specified, it'll be the overall best podcasts, which can be considered as a special genre.
- page int? (default ()) - Page number of those podcasts in this genre.
- region string (default "us") - Filter best podcasts by country/region. Please note that podcasts that are "best" in a country/region may not be produced in that country/region. For example, a podcast from the US may be very popular in Canada. You can get the supported country codes (e.g., us, jp, gb...) from
GET /regions
. If not specified, you'll get "best podcasts" in United States.
- publisherRegion string? (default ()) - Filter best podcasts by the publisher's country/region. This is to narrow down the results to include "best podcasts" produced in a specific country/region. You can get the supported country codes (e.g., us, jp, gb...) from
GET /regions
. If not specified, you'll get "best podcasts" produced in any country/region. If you want to get a country/region's "best podcasts" that are also produced in that country/region, then you need to specify both region and publisher_region, e.g.,region=jp
andpublisher_region=jp
.
- language string? (default ()) - Filter best podcasts by language. You can get a list of supported languages (e.g., English, Chinese, Japanese...) from
GET /languages
. If not specified, you'll get "best podcasts" in any language.
- safeMode int (default 0) - Whether or not to exclude podcasts with explicit language. 1 is yes, and 0 is no.
Return Type
getPodcastById
function getPodcastById(string id, int? nextEpisodePubDate, string sort) returns PodcastFull|error
Fetch detailed meta data and episodes for a podcast by id
Parameters
- id string - Podcast id. You can get podcast id from using other endpoints, e.g.,
GET /search
,GET /best_podcasts
...
- nextEpisodePubDate int? (default ()) - For episodes pagination. It's the value of next_episode_pub_date from the response of last request. If not specified, just return latest 10 episodes or oldest 10 episodes, depending on the value of the sort parameter.
- sort string (default "recent_first") - How do you want to sort the episodes of this podcast?
Return Type
- PodcastFull|error - OK
deletePodcastById
function deletePodcastById(string id, string? reason) returns DeletePodcastResponse|error
Request to delete a podcast
Parameters
- id string - Podcast id. You can get podcast id from using other endpoints, e.g.,
GET /search
,GET /best_podcasts
...
- reason string? (default ()) - The reason why this podcast should be deleted, e.g., copyright violation, the podcaster wants to delete it... You can put "testing" here to indicate that you are testing this endpoint, so we will not actually delete the podcast.
Return Type
getEpisodeById
function getEpisodeById(string id, int showTranscript) returns EpisodeFull|error
Fetch detailed meta data for an episode by id
Parameters
- id string - id for a specific episode. You can get episode id from using other endpoints, e.g.,
GET /search
...
- showTranscript int (default 0) - To include the transcript of this episode or not? If it is 1, then include the transcript in the transcript field. The default value is 0 - we don't include transcript by default, because 1) it would make the response data very big, thus slow response time; 2) less than 1% of episodes have transcripts. The transcript field is available only in the PRO/ENTERPRISE plan.
Return Type
- EpisodeFull|error - OK
getEpisodesInBatch
function getEpisodesInBatch(GetEpisodesInBatchForm payload) returns GetEpisodesInBatchResponse|error
Batch fetch basic meta data for episodes
Parameters
- payload GetEpisodesInBatchForm - Get episodes batch payload
Return Type
getPodcastsInBatch
function getPodcastsInBatch(GetPodcastsInBatchForm payload) returns GetPodcastsInBatchResponse|error
Batch fetch basic meta data for podcasts
Parameters
- payload GetPodcastsInBatchForm - Get podcasts batch payload
Return Type
getCuratedPodcastById
function getCuratedPodcastById(string id) returns CuratedListFull|error
Fetch a curated list of podcasts by id
Parameters
- id string - id for a specific curated list of podcasts. You can get the id from the response of
GET /search?type=curated
orGET /curated_podcasts
.
Return Type
- CuratedListFull|error - OK
getGenres
function getGenres(int topLevelOnly) returns GetGenresResponse|error
Fetch a list of podcast genres
Parameters
- topLevelOnly int (default 0) - Just show top level genres? If 1, yes, just show top level genres. If 0, no, show all genres.
Return Type
- GetGenresResponse|error - OK
getRegions
function getRegions() returns GetRegionsResponse|error
Fetch a list of supported countries/regions for best podcasts
Return Type
- GetRegionsResponse|error - OK
getLanguages
function getLanguages() returns GetLanguagesResponse|error
Fetch a list of supported languages for podcasts
Return Type
justListen
function justListen() returns EpisodeSimple|error
Fetch a random podcast episode
Return Type
- EpisodeSimple|error - OK
getCuratedPodcasts
function getCuratedPodcasts(int page) returns GetCuratedPodcastsResponse|error
Fetch curated lists of podcasts
Parameters
- page int (default 1) - Page number of curated lists.
Return Type
getPodcastRecommendations
function getPodcastRecommendations(string id, int safeMode) returns GetPodcastRecommendationsResponse|error
Fetch recommendations for a podcast
Parameters
- id string - Podcast id.
- safeMode int (default 0) - Whether or not to exclude podcasts with explicit language. 1 is yes, and 0 is no.
Return Type
getEpisodeRecommendations
function getEpisodeRecommendations(string id, int safeMode) returns GetEpisodeRecommendationsResponse|error
Fetch recommendations for an episode
Parameters
- id string - Episode id.
- safeMode int (default 0) - Whether or not to exclude podcasts with explicit language. 1 is yes, and 0 is no.
Return Type
submitPodcast
function submitPodcast(SubmitPodcastForm payload) returns SubmitPodcastResponse|error
Submit a podcast to Listen Notes database
Parameters
- payload SubmitPodcastForm - Submit podcast payload
Return Type
getPlaylistById
function getPlaylistById(string id, string 'type, int lastTimestampMs, string sort) returns PlaylistResponse|error
Fetch a playlist's info and items (i.e., episodes or podcasts).
Parameters
- id string - Playlist id (always 11 characters, e.g., m1pe7z60bsw). You can get the podcast id from the url of a playlist, e.g., m1pe7z60bsw is the playlist id of listennotes.com/listen/podcasts-about-podcasting-m1pe7z60bsw
- 'type string (default "episode_list") - The type of this playlist, which should be either episode_list or podcast_list.
- lastTimestampMs int (default 0) - For playlist items pagination. It's the value of last_timestamp_ms from the response of last request. If it's 0 or not specified, just return the latest or the oldest 20 items, depending on the value of the sort parameter.
- sort string (default "recent_added_first") - How do you want to sort playlist items?
Return Type
- PlaylistResponse|error - OK
getPlaylists
function getPlaylists(string sort, int page) returns PlaylistsResponse|error
Fetch a list of your playlists.
Parameters
- sort string (default "recent_added_first") - How do you want to sort playlists?
- page int (default 1) - Page number of playlists.
Return Type
- PlaylistsResponse|error - OK
Records
listen.notes: ApiKeysConfig
Provides API key configurations needed when communicating with a remote HTTP endpoint.
Fields
- xListenapiKey string - Represents API Key
X-ListenAPI-Key
listen.notes: BestPodcastsResponse
Fields
- has_previous boolean -
- name string - This genre's name.
- listennotes_url BestPodcastsLNUrlField - Url of the list of best podcasts on ListenNotes.com.
- previous_page_number int -
- page_number int -
- has_next boolean -
- next_page_number int -
- parent_id int - The id of parent genre.
- id int - The id of this genre
- total int -
- podcasts PodcastSimple[] -
listen.notes: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
listen.notes: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
listen.notes: CuratedListFull
Fields
- id CuratedIdField? - Curated list id.
- description CuratedDescriptionField? - This curated list's description.
- source_url CuratedSourceUrlField? - Url of the source of this curated list.
- source_domain CuratedSourceDomainField? - The domain name of the source of this curated list.
- pub_date_ms CuratedPubDateMsField? - Published date of this curated list. In milliseconds.
- listennotes_url CuratedLNUrlField? - The url of this curated list on ListenNotes.com.
- title CuratedNameField? - Curated list name.
- total CuratedTotalPodcastsField? - The total number of podcasts in this curated list.
- podcasts PodcastSimple[]? - Complete meta data of all podcasts in this curated list.
listen.notes: CuratedListSearchResult
When type is curated.
Fields
- id CuratedIdField? - Curated list id.
- pub_date_ms CuratedPubDateMsField? - Published date of this curated list. In milliseconds.
- description_highlighted string? - Highlighted segment of this curated list's description
- description_original string? - Plain text of this curated list's description
- title_highlighted string? - Highlighted segment of this curated list's title
- title_original string? - Plain text of this curated list's title
- listennotes_url CuratedLNUrlField? - The url of this curated list on ListenNotes.com.
- source_url CuratedSourceUrlField? - Url of the source of this curated list.
- source_domain CuratedSourceDomainField? - The domain name of the source of this curated list.
- total CuratedTotalPodcastsField? - The total number of podcasts in this curated list.
- podcasts PodcastMinimum[]? - Up to 5 podcasts in this curated list.
listen.notes: CuratedListSimple
Fields
- id CuratedIdField? - Curated list id.
- description CuratedDescriptionField? - This curated list's description.
- source_url CuratedSourceUrlField? - Url of the source of this curated list.
- source_domain CuratedSourceDomainField? - The domain name of the source of this curated list.
- pub_date_ms CuratedPubDateMsField? - Published date of this curated list. In milliseconds.
- listennotes_url CuratedLNUrlField? - The url of this curated list on ListenNotes.com.
- title CuratedNameField? - Curated list name.
- total CuratedTotalPodcastsField? - The total number of podcasts in this curated list.
- podcasts PodcastMinimum[]? - Minimum meta data of up to 5 podcasts in this curated list.
listen.notes: CustomAudio
A custom audio in a playlist, which is a type of playlist item.
Fields
- title string? - Custom audio title.
- audio string? - Audio url, which can be played directly.
- audio_length_sec int? - Audio length in seconds.
- image string? - High resolution image url of this custom audio.
- thumbnail string? - Low resolution image url of this custom audio.
- pub_date_ms int? - Published date (in milliseconds) of this custom audio. For now, it's the same as added_at_ms of this playlist item.
listen.notes: DeletedItem
A deleted episode or podcast. An episode or a podcast could be deleted from our podcast database. Possible reasons: 1) Podcast producers sometimes delete their old episodes. 2) Copyright issues.
Fields
- id string? - Episode id or podcast id.
- title string? - Episode title or podcast title.
- status string? - The status of this episode or podcast. For now, the only possible value is deleted.
- 'error string? - Why this episode or podcast is deleted?
listen.notes: DeletePodcastResponse
Fields
- status string - The status of this podcast deletion request.
listen.notes: EpisodeFull
Fields
- maybe_audio_invalid MaybeAudioInvalidField? - Whether or not this episode's audio is invalid. Podcasters may delete the original audio.
- pub_date_ms EpisodePubDateMsField? - Published date for this episode. In millisecond.
- audio AudioField? - Audio url of this episode, which can be played directly.
- listennotes_edit_url EpisodeLNEditUrlField? - Edit url of this episode where you can update the audio url if you find the audio is broken.
- image EpisodeImageField? - Image url for this episode. If an episode doesn't have its own image, then this field would be the url of the podcast artwork image. If you are using PRO/ENTERPRISE plan, then it's a high resolution image (1400x1400). If you are using FREE plan, then it's the same as thumbnail, low resolution image (300x300).
- thumbnail EpisodeThumbnailField? - Thumbnail image (300x300) url for this episode. If an episode doesn't have its own image, then this field would be the url of the podcast artwork thumbnail image.
- description EpisodeDescriptionField? - Html of this episode's full description
- title EpisodeNameField? - Episode name.
- explicit_content ExplicitField? - Whether this podcast contains explicit language.
- listennotes_url EpisodeLNUrlField? - The url of this episode on ListenNotes.com.
- audio_length_sec AudioLengthSecField? - Audio length of this episode. In seconds.
- id EpisodeIdField? - Episode id.
- podcast PodcastSimple? -
- link LinkField? - Web link of this episode.
- transcript TranscriptField? - The transcript of this episode, in plain text (with the newline character \n). If there's not transcript, it is null. This field is available only in the PRO/ENTERPRISE plan.
listen.notes: EpisodeMinimum
Fields
- maybe_audio_invalid MaybeAudioInvalidField? - Whether or not this episode's audio is invalid. Podcasters may delete the original audio.
- pub_date_ms EpisodePubDateMsField? - Published date for this episode. In millisecond.
- audio AudioField? - Audio url of this episode, which can be played directly.
- listennotes_edit_url EpisodeLNEditUrlField? - Edit url of this episode where you can update the audio url if you find the audio is broken.
- image ImageField? - Image url for this podcast's artwork. If you are using PRO/ENTERPRISE plan, then it's a high resolution image (1400x1400). If you are using FREE plan, then it's the same as thumbnail, low resolution image (300x300).
- thumbnail ThumbnailField? - Thumbnail image url for this podcast's artwork (300x300).
- description EpisodeDescriptionField? - Html of this episode's full description
- title EpisodeNameField? - Episode name.
- explicit_content ExplicitField? - Whether this podcast contains explicit language.
- listennotes_url EpisodeLNUrlField? - The url of this episode on ListenNotes.com.
- audio_length_sec AudioLengthSecField? - Audio length of this episode. In seconds.
- id EpisodeIdField? - Episode id.
- link LinkField? - Web link of this episode.
listen.notes: EpisodeSearchResult
When type is episode.
Fields
- audio AudioField? - Audio url of this episode, which can be played directly.
- audio_length_sec AudioLengthSecField? - Audio length of this episode. In seconds.
- rss RssField? - RSS url of this podcast. This field is available only in the PRO/ENTERPRISE plan.
- description_highlighted string? - Highlighted segment of this episode's description
- description_original string? - Plain text of this episode's description
- title_highlighted string? - Highlighted segment of this episode's title
- title_original string? - Plain text of this episode' title
- transcripts_highlighted string[]? - Up to 2 highlighted segments of the audio transcript of this episode.
- image EpisodeImageField? - Image url for this episode. If an episode doesn't have its own image, then this field would be the url of the podcast artwork image. If you are using PRO/ENTERPRISE plan, then it's a high resolution image (1400x1400). If you are using FREE plan, then it's the same as thumbnail, low resolution image (300x300).
- thumbnail EpisodeThumbnailField? - Thumbnail image (300x300) url for this episode. If an episode doesn't have its own image, then this field would be the url of the podcast artwork thumbnail image.
- itunes_id ITunesIdField? - iTunes id for this podcast.
- pub_date_ms EpisodePubDateMsField? - Published date for this episode. In millisecond.
- id EpisodeIdField? - Episode id.
- listennotes_url EpisodeLNUrlField? - The url of this episode on ListenNotes.com.
- explicit_content ExplicitField? - Whether this podcast contains explicit language.
- link LinkField? - Web link of this episode.
- podcast EpisodesearchresultPodcast? - The podcast that this episode belongs to.
listen.notes: EpisodesearchresultPodcast
The podcast that this episode belongs to.
Fields
- id PodcastIdField? - Podcast id.
- genre_ids GenreIdsField? - Array of genre ids.
- listennotes_url PodcastLNUrlField? - The url of this podcast on ListenNotes.com.
- title_highlighted PodcastTitleHighlightedField? - Highlighted segment of podcast name.
- title_original PodcastTitleOriginalField? - Plain text of podcast name.
- publisher_highlighted PublisherHighlightedField? - Highlighted segment of this podcast's publisher name.
- publisher_original PublisherOriginalField? - Plain text of this podcast's publisher name.
- image ImageField? - Image url for this podcast's artwork. If you are using PRO/ENTERPRISE plan, then it's a high resolution image (1400x1400). If you are using FREE plan, then it's the same as thumbnail, low resolution image (300x300).
- thumbnail ThumbnailField? - Thumbnail image url for this podcast's artwork (300x300).
- listen_score ListenScoreField? - The estimated popularity score of a podcast compared to all other rss-based public podcasts in the world on a scale from 0 to 100. If the score is not available, it'll be null. Learn more at listennotes.com/listen-score
- listen_score_global_rank ListenScoreGlobalRankField? - The estimated popularity ranking of a podcast compared to all other rss-based public podcasts in the world. For example, if the value is 0.5%, then this podcast is one of the top 0.5% most popular shows out of all podcasts globally, ranked by Listen Score. If the ranking is not available, it'll be null. Learn more at listennotes.com/listen-score
listen.notes: EpisodeSimple
Fields
- maybe_audio_invalid MaybeAudioInvalidField? - Whether or not this episode's audio is invalid. Podcasters may delete the original audio.
- pub_date_ms EpisodePubDateMsField? - Published date for this episode. In millisecond.
- audio AudioField? - Audio url of this episode, which can be played directly.
- listennotes_edit_url EpisodeLNEditUrlField? - Edit url of this episode where you can update the audio url if you find the audio is broken.
- image EpisodeImageField? - Image url for this episode. If an episode doesn't have its own image, then this field would be the url of the podcast artwork image. If you are using PRO/ENTERPRISE plan, then it's a high resolution image (1400x1400). If you are using FREE plan, then it's the same as thumbnail, low resolution image (300x300).
- thumbnail EpisodeThumbnailField? - Thumbnail image (300x300) url for this episode. If an episode doesn't have its own image, then this field would be the url of the podcast artwork thumbnail image.
- description EpisodeDescriptionField? - Html of this episode's full description
- title EpisodeNameField? - Episode name.
- explicit_content ExplicitField? - Whether this podcast contains explicit language.
- listennotes_url EpisodeLNUrlField? - The url of this episode on ListenNotes.com.
- audio_length_sec AudioLengthSecField? - Audio length of this episode. In seconds.
- id EpisodeIdField? - Episode id.
- link LinkField? - Web link of this episode.
- podcast PodcastMinimum? -
listen.notes: Genre
Fields
- id int? - Genre id
- name string? - Genre name.
- parent_id int? - Parent genre id.
listen.notes: GetCuratedPodcastsResponse
Fields
- has_previous boolean -
- previous_page_number int -
- page_number int -
- next_page_number int -
- has_next boolean -
- total int -
- curated_lists CuratedListSimple[] -
listen.notes: GetEpisodeRecommendationsResponse
Fields
- recommendations EpisodeSimple[] -
listen.notes: GetEpisodesInBatchForm
Fields
- ids string - Comma-separated list of episode ids.
listen.notes: GetEpisodesInBatchResponse
Fields
- episodes EpisodeSimple[] -
listen.notes: GetGenresResponse
Fields
- genres Genre[] -
listen.notes: GetLanguagesResponse
Fields
- languages string[] -
listen.notes: GetPodcastRecommendationsResponse
Fields
- recommendations PodcastSimple[] -
listen.notes: GetPodcastsInBatchForm
Fields
- ids string? - Comma-separated list of podcast ids.
- rsses string? - Comma-separated rss urls.
- itunes_ids string? - Comma-separated itunes ids.
- show_latest_episodes int? - Whether or not to fetch up to 10 latest episodes from these podcasts, sorted by pub_date. 1 is yes, and 0 is no.
- next_episode_pub_date int? - For latest episodes pagination. It's the value of next_episode_pub_date from the response of last request. If not specified, just return latest 10 episodes.
listen.notes: GetPodcastsInBatchResponse
Fields
- podcasts PodcastSimple[] -
- latest_episodes EpisodeSimple[]? - Up to 10 latest episodes from these podcasts, sorted by pub_date. This field shows up only when show_latest_episodes is 1.
listen.notes: GetRegionsResponse
Fields
- regions record {} -
listen.notes: PlaylistItem
An item in a playlist
Fields
- id int? - Playlist item id.
- 'type string? - The type of this playlist item. If a playlist is episode_list, then an item could be either episode or custom_audio. If it's podcast_list, then an item can only be podcast.
- notes string? - Notes for this item.
- added_at_ms int? - Timestamp (in milliseconds) when this item is added.
- data EpisodeSimple|PodcastSimple|CustomAudio|DeletedItem? - Playlist item data
listen.notes: PlaylistResponse
Fields
- id PlaylistIdField? - A 11-character playlist id.
- name PlaylistNameField? - Playlist name.
- description PlaylistDescriptionField? - Playlist description.
- image PlaylistImageField? - High resolution image url of the playlist.
- thumbnail PlaylistThumbnailField? - Low resolution image url of the playlist.
- listennotes_url PlaylistListennotesUrlField? - The url of this playlist on ListenNotes.com.
- visibility PlaylistVisibilityField? - Visibility of this playlist.
- last_timestamp_ms PlaylistLastTimestampMsField? - Passed to the last_timestamp_ms parameter of
GET /playlists/{id}
to paginate through items of that playlist.
- total int? - Total number of items in this playlist.
- 'type string? - The type of this playlist, which should be either episode_list or podcast_list.
- items PlaylistItem[]? - A list of playlist items.
listen.notes: PlaylistsResponse
Fields
- previous_page_number int? -
- page_number int? -
- has_next boolean? -
- has_previous boolean? -
- next_page_number int? -
- total int? -
- playlists PlaylistsresponsePlaylists[]? -
listen.notes: PlaylistsresponsePlaylists
A playlist
Fields
- episode_count int? - The number of episodes (including custom audio) in this playlist.
- podcast_count int? - The number of podcasts in this playlist.
- id PlaylistIdField? - A 11-character playlist id.
- name PlaylistNameField? - Playlist name.
- description PlaylistDescriptionField? - Playlist description.
- image PlaylistImageField? - High resolution image url of the playlist.
- thumbnail PlaylistThumbnailField? - Low resolution image url of the playlist.
- listennotes_url PlaylistListennotesUrlField? - The url of this playlist on ListenNotes.com.
- visibility PlaylistVisibilityField? - Visibility of this playlist.
- last_timestamp_ms PlaylistLastTimestampMsField? - Passed to the last_timestamp_ms parameter of
GET /playlists/{id}
to paginate through items of that playlist.
listen.notes: PodcastExtraField
Fields
- youtube_url string? - YouTube url affiliated with this podcast
- facebook_handle string? - Facebook username affiliated with this podcast
- instagram_handle string? - Instagram username affiliated with this podcast
- twitter_handle string? - Twitter username affiliated with this podcast
- wechat_handle string? - WeChat username affiliated with this podcast
- patreon_handle string? - Patreon username affiliated with this podcast
- google_url string? - Google Podcasts url for this podcast
- linkedin_url string? - LinkedIn url affiliated with this podcast
- spotify_url string? - Spotify url for this podcast
- url1 string? - Url affiliated with this podcast
- url2 string? - Url affiliated with this podcast
- url3 string? - Url affiliated with this podcast
listen.notes: PodcastFull
Fields
- episodes EpisodeMinimum[]? -
- is_claimed IsClaimedField? - Whether this podcast is claimed by its producer on ListenNotes.com.
- 'type PodcastTypeField? - The type of this podcast - episodic or serial.
- explicit_content ExplicitField? - Whether this podcast contains explicit language.
- website WebsiteField? - Website url of this podcast.
- total_episodes TotalEpisodesField? - Total number of episodes in this podcast.
- earliest_pub_date_ms EarliestPubDateMsField? - The published date of the oldest episode of this podcast. In milliseconds
- rss RssField? - RSS url of this podcast. This field is available only in the PRO/ENTERPRISE plan.
- latest_pub_date_ms LatestPubDateMsField? - The published date of the latest episode of this podcast. In milliseconds
- title PodcastNameField? - Podcast name.
- language LanguageField? - The language of this podcast. You can get all supported languages from
GET /languages
.
- description PodcastDescriptionField? - Html of this episode's full description
- email EmailField? - The email of this podcast's producer. This field is available only in the PRO/ENTERPRISE plan.
- image ImageField? - Image url for this podcast's artwork. If you are using PRO/ENTERPRISE plan, then it's a high resolution image (1400x1400). If you are using FREE plan, then it's the same as thumbnail, low resolution image (300x300).
- thumbnail ThumbnailField? - Thumbnail image url for this podcast's artwork (300x300).
- listennotes_url PodcastLNUrlField? - The url of this podcast on ListenNotes.com.
- id PodcastIdField? - Podcast id.
- country CountryField? - The country where this podcast is produced.
- publisher PublisherField? - Podcast publisher name.
- itunes_id ITunesIdField? - iTunes id for this podcast.
- looking_for PodcastLookingForField? -
- extra PodcastExtraField? -
- genre_ids GenreIdsField? - Array of genre ids.
- next_episode_pub_date NextEpisodePubDateField? - Passed to the next_episode_pub_date parameter of
GET /podcasts/{id}
to paginate through episodes of that podcast.
- listen_score ListenScoreField? - The estimated popularity score of a podcast compared to all other rss-based public podcasts in the world on a scale from 0 to 100. If the score is not available, it'll be null. Learn more at listennotes.com/listen-score
- listen_score_global_rank ListenScoreGlobalRankField? - The estimated popularity ranking of a podcast compared to all other rss-based public podcasts in the world. For example, if the value is 0.5%, then this podcast is one of the top 0.5% most popular shows out of all podcasts globally, ranked by Listen Score. If the ranking is not available, it'll be null. Learn more at listennotes.com/listen-score
listen.notes: PodcastLookingForField
Fields
- cohosts boolean? - Whether this podcast is looking for cohosts.
- cross_promotion boolean? - Whether this podcast is looking for cross promotion opportunities with other podcasts.
- sponsors boolean? - Whether this podcast is looking for sponsors.
- guests boolean? - Whether this podcast is looking for guests.
listen.notes: PodcastMinimum
Fields
- image ImageField? - Image url for this podcast's artwork. If you are using PRO/ENTERPRISE plan, then it's a high resolution image (1400x1400). If you are using FREE plan, then it's the same as thumbnail, low resolution image (300x300).
- thumbnail ThumbnailField? - Thumbnail image url for this podcast's artwork (300x300).
- title PodcastNameField? - Podcast name.
- listennotes_url PodcastLNUrlField? - The url of this podcast on ListenNotes.com.
- id PodcastIdField? - Podcast id.
- publisher PublisherField? - Podcast publisher name.
- listen_score ListenScoreField? - The estimated popularity score of a podcast compared to all other rss-based public podcasts in the world on a scale from 0 to 100. If the score is not available, it'll be null. Learn more at listennotes.com/listen-score
- listen_score_global_rank ListenScoreGlobalRankField? - The estimated popularity ranking of a podcast compared to all other rss-based public podcasts in the world. For example, if the value is 0.5%, then this podcast is one of the top 0.5% most popular shows out of all podcasts globally, ranked by Listen Score. If the ranking is not available, it'll be null. Learn more at listennotes.com/listen-score
listen.notes: PodcastSearchResult
When type is podcast.
Fields
- rss RssField? - RSS url of this podcast. This field is available only in the PRO/ENTERPRISE plan.
- description_highlighted string? - Highlighted segment of podcast description
- description_original string? - Plain text of podcast description
- title_highlighted PodcastTitleHighlightedField? - Highlighted segment of podcast name.
- title_original PodcastTitleOriginalField? - Plain text of podcast name.
- publisher_highlighted PublisherHighlightedField? - Highlighted segment of this podcast's publisher name.
- publisher_original PublisherOriginalField? - Plain text of this podcast's publisher name.
- image ImageField? - Image url for this podcast's artwork. If you are using PRO/ENTERPRISE plan, then it's a high resolution image (1400x1400). If you are using FREE plan, then it's the same as thumbnail, low resolution image (300x300).
- thumbnail ThumbnailField? - Thumbnail image url for this podcast's artwork (300x300).
- itunes_id ITunesIdField? - iTunes id for this podcast.
- latest_pub_date_ms LatestPubDateMsField? - The published date of the latest episode of this podcast. In milliseconds
- earliest_pub_date_ms EarliestPubDateMsField? - The published date of the oldest episode of this podcast. In milliseconds
- id PodcastIdField? - Podcast id.
- genre_ids GenreIdsField? - Array of genre ids.
- listennotes_url PodcastLNUrlField? - The url of this podcast on ListenNotes.com.
- total_episodes TotalEpisodesField? - Total number of episodes in this podcast.
- email EmailField? - The email of this podcast's producer. This field is available only in the PRO/ENTERPRISE plan.
- explicit_content ExplicitField? - Whether this podcast contains explicit language.
- website WebsiteField? - Website url of this podcast.
- listen_score ListenScoreField? - The estimated popularity score of a podcast compared to all other rss-based public podcasts in the world on a scale from 0 to 100. If the score is not available, it'll be null. Learn more at listennotes.com/listen-score
- listen_score_global_rank ListenScoreGlobalRankField? - The estimated popularity ranking of a podcast compared to all other rss-based public podcasts in the world. For example, if the value is 0.5%, then this podcast is one of the top 0.5% most popular shows out of all podcasts globally, ranked by Listen Score. If the ranking is not available, it'll be null. Learn more at listennotes.com/listen-score
listen.notes: PodcastSimple
Fields
- is_claimed IsClaimedField? - Whether this podcast is claimed by its producer on ListenNotes.com.
- 'type PodcastTypeField? - The type of this podcast - episodic or serial.
- explicit_content ExplicitField? - Whether this podcast contains explicit language.
- website WebsiteField? - Website url of this podcast.
- total_episodes TotalEpisodesField? - Total number of episodes in this podcast.
- earliest_pub_date_ms EarliestPubDateMsField? - The published date of the oldest episode of this podcast. In milliseconds
- rss RssField? - RSS url of this podcast. This field is available only in the PRO/ENTERPRISE plan.
- latest_pub_date_ms LatestPubDateMsField? - The published date of the latest episode of this podcast. In milliseconds
- title PodcastNameField? - Podcast name.
- language LanguageField? - The language of this podcast. You can get all supported languages from
GET /languages
.
- description PodcastDescriptionField? - Html of this episode's full description
- email EmailField? - The email of this podcast's producer. This field is available only in the PRO/ENTERPRISE plan.
- image ImageField? - Image url for this podcast's artwork. If you are using PRO/ENTERPRISE plan, then it's a high resolution image (1400x1400). If you are using FREE plan, then it's the same as thumbnail, low resolution image (300x300).
- thumbnail ThumbnailField? - Thumbnail image url for this podcast's artwork (300x300).
- listennotes_url PodcastLNUrlField? - The url of this podcast on ListenNotes.com.
- id PodcastIdField? - Podcast id.
- country CountryField? - The country where this podcast is produced.
- publisher PublisherField? - Podcast publisher name.
- itunes_id ITunesIdField? - iTunes id for this podcast.
- looking_for PodcastLookingForField? -
- extra PodcastExtraField? -
- genre_ids GenreIdsField? - Array of genre ids.
- listen_score ListenScoreField? - The estimated popularity score of a podcast compared to all other rss-based public podcasts in the world on a scale from 0 to 100. If the score is not available, it'll be null. Learn more at listennotes.com/listen-score
- listen_score_global_rank ListenScoreGlobalRankField? - The estimated popularity ranking of a podcast compared to all other rss-based public podcasts in the world. For example, if the value is 0.5%, then this podcast is one of the top 0.5% most popular shows out of all podcasts globally, ranked by Listen Score. If the ranking is not available, it'll be null. Learn more at listennotes.com/listen-score
listen.notes: PodcastTypeaheadResult
Fields
- publisher_highlighted PublisherHighlightedField? - Highlighted segment of this podcast's publisher name.
- publisher_original PublisherOriginalField? - Plain text of this podcast's publisher name.
- explicit_content ExplicitField? - Whether this podcast contains explicit language.
- image ImageField? - Image url for this podcast's artwork. If you are using PRO/ENTERPRISE plan, then it's a high resolution image (1400x1400). If you are using FREE plan, then it's the same as thumbnail, low resolution image (300x300).
- thumbnail ThumbnailField? - Thumbnail image url for this podcast's artwork (300x300).
- title_highlighted PodcastTitleHighlightedField? - Highlighted segment of podcast name.
- title_original PodcastTitleOriginalField? - Plain text of podcast name.
- id PodcastIdField? - Podcast id.
listen.notes: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
listen.notes: SearchResponse
Fields
- next_offset int? - Pass this value to the offset parameter to do pagination of search results.
- took decimal? - The time it took to fetch these search results. In seconds.
- total int? - The total number of search results.
- count int? - The number of search results in this page.
- results (EpisodeSearchResult|PodcastSearchResult|CuratedListSearchResult)[]? - A list of search results.
listen.notes: SubmitPodcastForm
Fields
- rss string - A valid podcast rss url.
- email string? - A valid email address. If email is specified, then we'll notify this email address once the podcast is accepted.
listen.notes: SubmitPodcastResponse
Fields
- status string - The status of this submission.
- podcast PodcastMinimum -
listen.notes: TypeaheadResponse
Fields
- terms string[] - Search term suggestions.
- genres Genre[]? - Genre suggestions. It'll show up when the show_genres parameter is 1.
- podcasts PodcastTypeaheadResult[]? - Podcast suggestions. It'll show up when the show_podcasts parameter is 1.
String types
listen.notes: AudioField
AudioField
Audio url of this episode, which can be played directly.
listen.notes: BestPodcastsLNUrlField
BestPodcastsLNUrlField
Url of the list of best podcasts on ListenNotes.com.
listen.notes: CountryField
CountryField
The country where this podcast is produced.
listen.notes: CuratedDescriptionField
CuratedDescriptionField
This curated list's description.
listen.notes: CuratedIdField
CuratedIdField
Curated list id.
listen.notes: CuratedLNUrlField
CuratedLNUrlField
The url of this curated list on ListenNotes.com.
listen.notes: CuratedNameField
CuratedNameField
Curated list name.
listen.notes: CuratedSourceDomainField
CuratedSourceDomainField
The domain name of the source of this curated list.
listen.notes: CuratedSourceUrlField
CuratedSourceUrlField
Url of the source of this curated list.
listen.notes: EmailField
EmailField
The email of this podcast's producer. This field is available only in the PRO/ENTERPRISE plan.
listen.notes: EpisodeDescriptionField
EpisodeDescriptionField
Html of this episode's full description
listen.notes: EpisodeIdField
EpisodeIdField
Episode id.
listen.notes: EpisodeImageField
EpisodeImageField
Image url for this episode. If an episode doesn't have its own image, then this field would be the url of the podcast artwork image. If you are using PRO/ENTERPRISE plan, then it's a high resolution image (1400x1400). If you are using FREE plan, then it's the same as thumbnail, low resolution image (300x300).
listen.notes: EpisodeLNEditUrlField
EpisodeLNEditUrlField
Edit url of this episode where you can update the audio url if you find the audio is broken.
listen.notes: EpisodeLNUrlField
EpisodeLNUrlField
The url of this episode on ListenNotes.com.
listen.notes: EpisodeNameField
EpisodeNameField
Episode name.
listen.notes: EpisodeThumbnailField
EpisodeThumbnailField
Thumbnail image (300x300) url for this episode. If an episode doesn't have its own image, then this field would be the url of the podcast artwork thumbnail image.
listen.notes: ImageField
ImageField
Image url for this podcast's artwork. If you are using PRO/ENTERPRISE plan, then it's a high resolution image (1400x1400). If you are using FREE plan, then it's the same as thumbnail, low resolution image (300x300).
listen.notes: LanguageField
LanguageField
The language of this podcast. You can get all supported languages from GET /languages
.
listen.notes: LinkField
LinkField
Web link of this episode.
listen.notes: ListenScoreGlobalRankField
ListenScoreGlobalRankField
The estimated popularity ranking of a podcast compared to all other rss-based public podcasts in the world. For example, if the value is 0.5%, then this podcast is one of the top 0.5% most popular shows out of all podcasts globally, ranked by Listen Score. If the ranking is not available, it'll be null. Learn more at listennotes.com/listen-score
listen.notes: PlaylistDescriptionField
PlaylistDescriptionField
Playlist description.
listen.notes: PlaylistIdField
PlaylistIdField
A 11-character playlist id.
listen.notes: PlaylistImageField
PlaylistImageField
High resolution image url of the playlist.
listen.notes: PlaylistListennotesUrlField
PlaylistListennotesUrlField
The url of this playlist on ListenNotes.com.
listen.notes: PlaylistNameField
PlaylistNameField
Playlist name.
listen.notes: PlaylistThumbnailField
PlaylistThumbnailField
Low resolution image url of the playlist.
listen.notes: PlaylistVisibilityField
PlaylistVisibilityField
Visibility of this playlist.
listen.notes: PodcastDescriptionField
PodcastDescriptionField
Html of this episode's full description
listen.notes: PodcastIdField
PodcastIdField
Podcast id.
listen.notes: PodcastLNUrlField
PodcastLNUrlField
The url of this podcast on ListenNotes.com.
listen.notes: PodcastNameField
PodcastNameField
Podcast name.
listen.notes: PodcastTitleHighlightedField
PodcastTitleHighlightedField
Highlighted segment of podcast name.
listen.notes: PodcastTitleOriginalField
PodcastTitleOriginalField
Plain text of podcast name.
listen.notes: PodcastTypeField
PodcastTypeField
The type of this podcast - episodic or serial.
listen.notes: PublisherField
PublisherField
Podcast publisher name.
listen.notes: PublisherHighlightedField
PublisherHighlightedField
Highlighted segment of this podcast's publisher name.
listen.notes: PublisherOriginalField
PublisherOriginalField
Plain text of this podcast's publisher name.
listen.notes: RssField
RssField
RSS url of this podcast. This field is available only in the PRO/ENTERPRISE plan.
listen.notes: ThumbnailField
ThumbnailField
Thumbnail image url for this podcast's artwork (300x300).
listen.notes: TranscriptField
TranscriptField
The transcript of this episode, in plain text (with the newline character \n). If there's not transcript, it is null. This field is available only in the PRO/ENTERPRISE plan.
listen.notes: WebsiteField
WebsiteField
Website url of this podcast.
Integer types
listen.notes: AudioLengthSecField
AudioLengthSecField
Audio length of this episode. In seconds.
listen.notes: CuratedPubDateMsField
CuratedPubDateMsField
Published date of this curated list. In milliseconds.
listen.notes: CuratedTotalPodcastsField
CuratedTotalPodcastsField
The total number of podcasts in this curated list.
listen.notes: EarliestPubDateMsField
EarliestPubDateMsField
The published date of the oldest episode of this podcast. In milliseconds
listen.notes: EpisodePubDateMsField
EpisodePubDateMsField
Published date for this episode. In millisecond.
listen.notes: ITunesIdField
ITunesIdField
iTunes id for this podcast.
listen.notes: LatestPubDateMsField
LatestPubDateMsField
The published date of the latest episode of this podcast. In milliseconds
listen.notes: ListenScoreField
ListenScoreField
The estimated popularity score of a podcast compared to all other rss-based public podcasts in the world on a scale from 0 to 100. If the score is not available, it'll be null. Learn more at listennotes.com/listen-score
listen.notes: NextEpisodePubDateField
NextEpisodePubDateField
Passed to the next_episode_pub_date parameter of GET /podcasts/{id}
to paginate through episodes of that podcast.
listen.notes: PlaylistLastTimestampMsField
PlaylistLastTimestampMsField
Passed to the last_timestamp_ms parameter of GET /playlists/{id}
to paginate through items of that playlist.
listen.notes: TotalEpisodesField
TotalEpisodesField
Total number of episodes in this podcast.
Import
import ballerinax/listen.notes;
Metadata
Released date: over 1 year ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 0
Current verison: 0
Weekly downloads
Keywords
Content & Files/Video & Audio
Cost/Freemium
Contributors
Dependencies