Module keap
API
Definitions
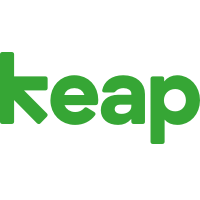
ballerinax/keap Ballerina library
Overview
This is a generated connector for Keap API v1.70.0.453309-hf-202203291431 OpenAPI specification. Keap is an all-in-one CRM, sales and marketing automation software that helps organize leads, land the job, get paid, and keep growing.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Keap account
- Obtain tokens by following this guide
Quickstart
To use the Keap connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/keap
module into the Ballerina project.
import ballerinax/keap;
Step 2: Create a new connector instance
Create a keap:ClientConfig
with the Bearer_Token
obtained, and initialize the connector with it.
keap:ClientConfig clientConfig = { auth: { token: <Bearer_Token> } }; keap:Client baseClient = check new Client(clientConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to list opportunities, using the connector.
Gets a list of opportunities.
public function main() returns error? { keap:OpportunityList response = check baseClient->listOpportunities(); log:printInfo(response.toString()); }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
keap: Client
This is a generated connector for Keap API v1.70.0.453309-hf-202203291431 OpenAPI specification. Keap is an all-in-one CRM, sales and marketing automation software that helps organize leads, land the job, get paid, and keep growing.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Keap account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.infusionsoft.com/crm/rest/" - URL of the target service
getAccountProfile
function getAccountProfile() returns AccountProfile|error
Retrieve account profile
Return Type
- AccountProfile|error - OK
updateAccountInfo
function updateAccountInfo(AccountProfile payload) returns AccountProfile|error
Updates an account profile
Parameters
- payload AccountProfile - accountInfo
Return Type
- AccountProfile|error - OK
listAffiliates
function listAffiliates(string? name, string? code, string? parentId, string? contactId, string? programId, string? status, string? 'order, string? orderDirection, int? offset, int? 'limit) returns AffiliateList|error
List Affiliates
Parameters
- name string? (default ()) - Optional affiliate name to query on
- code string? (default ()) - Optional affiliate parent ID to query on
- parentId string? (default ()) - Optional affiliate code to query on
- contactId string? (default ()) - Optional contact ID to query on
- programId string? (default ()) - Optional affiliate program ID to query on
- status string? (default ()) - Optional affiliate status to query on
- 'order string? (default ()) - Attribute to order items by
- orderDirection string? (default ()) - How to order the data i.e. ascending (A-Z) or descending (Z-A)
- offset int? (default ()) - Count to offset the returned results by
- 'limit int? (default ()) - Sets a beginning range of items to return
Return Type
- AffiliateList|error - OK
createAffiliate
function createAffiliate(CreateAffiliate payload) returns Affiliate|error
Create an affiliate
Parameters
- payload CreateAffiliate - affiliate
listCommissions
function listCommissions(string? since, string? until, string? 'order, string? orderDirection, int? 'limit, int? offset, int? affiliateId) returns AffiliateCommissionList|error
List Commissions
Parameters
- since string? (default ()) - Date to start searching from ex.
2017-01-01T22:17:59.039Z
- until string? (default ()) - Date to search to ex.
2017-01-01T22:17:59.039Z
- 'order string? (default ()) - Attribute to order items by
- orderDirection string? (default ()) - How to order the data i.e. ascending (A-Z) or descending (Z-A)
- 'limit int? (default ()) - Sets a total of items to return
- offset int? (default ()) - Sets a beginning range of items to return
- affiliateId int? (default ()) - Affiliate to retrieve commissions for
Return Type
retrieveAffiliateModel
function retrieveAffiliateModel() returns ObjectModel|error
Retrieve Affiliate Model
Return Type
- ObjectModel|error - OK
listPrograms
function listPrograms(int? affiliateId, string? 'order, string? orderDirection, int? offset, int? 'limit) returns AffiliateProgramList|error
List Commission Programs
Parameters
- affiliateId int? (default ()) - Id of the affiliate you would like programs for
- 'order string? (default ()) - Attribute to order items by
- orderDirection string? (default ()) - How to order the data i.e. ascending (A-Z) or descending (Z-A)
- offset int? (default ()) - Count to offset the returned results by
- 'limit int? (default ()) - Sets a beginning range of items to return
Return Type
listAffiliateRedirectLinks
function listAffiliateRedirectLinks(string? affiliateId, string? 'order, string? orderDirection, int? offset, int? 'limit) returns RedirectList|error
List Affiliate Redirects
Parameters
- affiliateId string? (default ()) - Affiliate ID to search by
- 'order string? (default ()) - Attribute to order items by
- orderDirection string? (default ()) - How to order the data i.e. ascending (A-Z) or descending (Z-A)
- offset int? (default ()) - Count to offset the returned results by
- 'limit int? (default ()) - Sets a beginning range of items to return
Return Type
- RedirectList|error - OK
listSummaries
function listSummaries(int[]? affiliateIds, string? 'order, string? orderDirection) returns AffiliateSummaryList|error
List affiliate summaries
Parameters
- affiliateIds int[]? (default ()) - Ids of the affiliates you would like a summary for
- 'order string? (default ()) - Attribute to order items by
- orderDirection string? (default ()) - How to order the data i.e. ascending (A-Z) or descending (Z-A)
Return Type
listAffiliateClawbacks
function listAffiliateClawbacks(string affiliateId, string? since, string? until, string? 'order, string? orderDirection, int? offset, int? 'limit) returns AffiliateClawbackList|error
List Affiliate clawbacks
Parameters
- affiliateId string - affiliateId
- since string? (default ()) - Optional inclusive start date
- until string? (default ()) - Optional inclusive end date
- 'order string? (default ()) - Attribute to order items by
- orderDirection string? (default ()) - How to order the data i.e. ascending (A-Z) or descending (Z-A)
- offset int? (default ()) - Count to offset the returned results by
- 'limit int? (default ()) - Sets a beginning range of items to return
Return Type
getAffiliate
Retrieve an affiliate
Parameters
- id int - id
listAppointments
function listAppointments(string? since, string? until, int? 'limit, int? offset, int? contactId) returns AppointmentList|error
List Appointments
Parameters
- since string? (default ()) - Date to start searching from ex.
2017-01-01T22:17:59.039Z
- until string? (default ()) - Date to search to ex.
2017-01-01T22:17:59.039Z
- 'limit int? (default ()) - Sets a total of items to return
- offset int? (default ()) - Sets a beginning range of items to return
- contactId int? (default ()) - Optionally find appointments with a contact
Return Type
- AppointmentList|error - OK
createAppointment
function createAppointment(Appointment payload) returns Appointment|error
Create an Appointment
Parameters
- payload Appointment - appointment
Return Type
- Appointment|error - Created
retrieveAppointmentModel
function retrieveAppointmentModel() returns ObjectModel|error
Retrieve Appointment Model
Return Type
- ObjectModel|error - OK
createAppointmentCustomField
function createAppointmentCustomField(CreateRestCustomField payload) returns CustomFieldMetaData|error
Create a Custom Field
Parameters
- payload CreateRestCustomField - customField
Return Type
- CustomFieldMetaData|error - Created
getAppointment
function getAppointment(int appointmentId) returns Appointment|error
Retrieve an Appointment
Parameters
- appointmentId int - appointmentId
Return Type
- Appointment|error - OK
updateAppointment
function updateAppointment(int appointmentId, Appointment payload) returns Appointment|error
Replace an Appointment
Return Type
- Appointment|error - OK
deleteAppointment
Delete an Appointment
Parameters
- appointmentId int - appointmentId
updatePropertiesOnAppointment
function updatePropertiesOnAppointment(int appointmentId, Appointment payload) returns Appointment|error
Update an Appointment
Return Type
- Appointment|error - OK
listCampaigns
function listCampaigns(int? 'limit, int? offset, string? searchText, string? 'order, string? orderDirection) returns CampaignList|error
List Campaigns
Parameters
- 'limit int? (default ()) - Sets a total of items to return
- offset int? (default ()) - Sets a beginning range of items to return
- searchText string? (default ()) - Optional text to search
- 'order string? (default ()) - Attribute to order items by
- orderDirection string? (default ()) - How to order the data i.e. ascending (A-Z) or descending (Z-A)
Return Type
- CampaignList|error - OK
createAchieveApiGoalEvent
function createAchieveApiGoalEvent(string integration, string callName, AchieveApiGoalEvent payload) returns GoalEventResultDTO[]|error
Achieve API Goal
Parameters
- integration string - integration
- callName string - callName
- payload AchieveApiGoalEvent - goalEvent
Return Type
- GoalEventResultDTO[]|error - Created
getCampaign
Retrieve a Campaign
Parameters
- campaignId int - campaignId
- optionalProperties string[]? (default ()) - Comma-delimited list of Campaign properties to include in the response. (The fields
goals
andsequences
aren't included, by default.)
addContactsToCampaignSequence
function addContactsToCampaignSequence(int campaignId, int sequenceId, SetOfIds payload) returns json|error
Add Multiple to Campaign Sequence
Return Type
- json|error - OK
addContactToCampaignSequence
function addContactToCampaignSequence(int campaignId, int sequenceId, int contactId) returns Response|error
Add to Campaign Sequence
removeContactFromCampaignSequence
function removeContactFromCampaignSequence(int campaignId, int sequenceId, int contactId) returns Response|error
Remove from Campaign Sequence
listCompanies
function listCompanies(int? 'limit, int? offset, string? companyName, string? 'order, string? orderDirection, string[]? optionalProperties) returns CompanyList|error
List Companies
Parameters
- 'limit int? (default ()) - Sets a total of items to return
- offset int? (default ()) - Sets a beginning range of items to return
- companyName string? (default ()) - Optional company name to query on
- 'order string? (default ()) - Attribute to order items by
- orderDirection string? (default ()) - How to order the data i.e. ascending (A-Z) or descending (Z-A)
- optionalProperties string[]? (default ()) - Comma-delimited list of Company properties to include in the response. (Fields such as
notes
,fax_number
andcustom_fields
aren't included, by default.)
Return Type
- CompanyList|error - OK
createCompany
function createCompany(CreateOrPatchCompany payload) returns Company|error
Create a Company
Parameters
- payload CreateOrPatchCompany - company
retrieveCompanyModel
function retrieveCompanyModel() returns ObjectModel|error
Retrieve Company Model
Return Type
- ObjectModel|error - OK
getCompany
Retrieve a Company
Parameters
- companyId int - companyId
- optionalProperties string[]? (default ()) - Comma-delimited list of Company properties to include in the response. (Fields such as
notes
,fax_number
andcustom_fields
aren't included, by default.)
updateCompanies
function updateCompanies(int companyId, CreateOrPatchCompany payload) returns Company|error
Update a Company
updateCompany
function updateCompany(int companyId, CreateOrPatchCompany payload) returns Company|error
Update a Company
listContacts
function listContacts(int? 'limit, int? offset, string? email, string? givenName, string? familyName, string? 'order, string? orderDirection, string? since, string? until, string[]? optionalProperties) returns ContactList|error
List Contacts
Parameters
- 'limit int? (default ()) - Sets a total of items to return
- offset int? (default ()) - Sets a beginning range of items to return
- email string? (default ()) - Optional email address to query on
- givenName string? (default ()) - Optional first name or forename to query on
- familyName string? (default ()) - Optional last name or surname to query on
- 'order string? (default ()) - Attribute to order items by
- orderDirection string? (default ()) - How to order the data i.e. ascending (A-Z) or descending (Z-A)
- since string? (default ()) - Date to start searching from on LastUpdated ex.
2017-01-01T22:17:59.039Z
- until string? (default ()) - Date to search to on LastUpdated ex.
2017-01-01T22:17:59.039Z
- optionalProperties string[]? (default ()) - Comma-delimited list of Contact properties to include in the response. (Some fields such as
lead_source_id
,custom_fields
, andjob_title
aren't included, by default.)
Return Type
- ContactList|error - OK
createOrUpdateContact
function createOrUpdateContact(UpsertContact payload) returns FullContact|error
Create or Update a Contact
Parameters
- payload UpsertContact - contact
Return Type
- FullContact|error - OK
createContact
function createContact(CreateOrPatchContact payload) returns FullContact|error
Create a Contact
Parameters
- payload CreateOrPatchContact - contact
Return Type
- FullContact|error - Created
retrieveContactModel
function retrieveContactModel() returns ObjectModel|error
Retrieve Contact Model
Return Type
- ObjectModel|error - OK
createCustomField
function createCustomField(CreateRestCustomField payload) returns CustomFieldMetaData|error
Create a Custom Field
Parameters
- payload CreateRestCustomField - customField
Return Type
- CustomFieldMetaData|error - Created
deleteContact
Delete a Contact
Parameters
- contactId int - contactId
updatePropertiesOnContact
function updatePropertiesOnContact(int contactId, CreateOrPatchContact payload, string[]? updateMask) returns FullContact|error
Update a Contact
Parameters
- contactId int - contactId
- payload CreateOrPatchContact - contact
- updateMask string[]? (default ()) - An optional list of properties to be updated. If set, only the provided properties will be updated and others will be skipped.
Return Type
- FullContact|error - OK
listCreditCards
function listCreditCards(int contactId) returns ContactCreditCard[]|error
Retrieve Credit Cards
Parameters
- contactId int - contactId
Return Type
- ContactCreditCard[]|error - OK
createCreditCard
function createCreditCard(int contactId, CreditCard payload) returns CreditCardAdded|error
Create a Credit Card
Return Type
- CreditCardAdded|error - Created
listEmailsForContact
function listEmailsForContact(int contactId, int? 'limit, int? offset, string? email) returns EmailSentQueryResultList|error
List Emails
Parameters
- contactId int - contactId
- 'limit int? (default ()) - Sets a total of items to return
- offset int? (default ()) - Sets a beginning range of items to return
- email string? (default ()) - Optional email address to query on
Return Type
createEmailForContact
function createEmailForContact(int contactId, EmailSentCreate payload) returns EmailSentCreate|error
Create an Email Record
Parameters
- contactId int - contactId
- payload EmailSentCreate - Email records to persist, with content.
Return Type
- EmailSentCreate|error - Created
listAppliedTags
function listAppliedTags(int contactId, int? 'limit, int? offset) returns ContactTagList|error
List Applied Tags
Parameters
- contactId int - contactId
- 'limit int? (default ()) - Sets a total of items to return
- offset int? (default ()) - Sets a beginning range of items to return
Return Type
- ContactTagList|error - OK
applyTagsToContactId
function applyTagsToContactId(int contactId, TagId payload) returns EntryLongString[]|error
Apply Tags
Return Type
- EntryLongString[]|error - OK
removeAppliedTagsFromContact
Remove Applied Tags
removeTagsFromContact
Remove Applied Tag
replaceEmailAddress
function replaceEmailAddress(string email, UpdateEmailAddress payload) returns RestEmailAddress|error
Replace an Email Address
Return Type
- RestEmailAddress|error - OK
listEmails
function listEmails(int? 'limit, int? offset, int? contactId, string? email, string? sinceSentDate, string? untilSentDate, boolean ordered) returns EmailSentQueryResultList|error
List Emails
Parameters
- 'limit int? (default ()) - Sets a total of items to return
- offset int? (default ()) - Sets a beginning range of items to return
- contactId int? (default ()) - Optional Contact Id to find Emails for
- email string? (default ()) - Optional email address to query on
- sinceSentDate string? (default ()) - Optional date to query on, emails sent since the provided date, must be present if untilDate is provided
- untilSentDate string? (default ()) - Optional date to query on, email sent until the provided date
- ordered boolean (default true) - Optional boolean to turn off ORDER BY in SQL query
Return Type
createEmail
function createEmail(EmailSentCreate payload) returns EmailSentCreate|error
Create an Email Record
Parameters
- payload EmailSentCreate - Email records to persist, with content.
Return Type
- EmailSentCreate|error - Created
sendEmail
function sendEmail(EmailSendRequest payload) returns Response|error
Send an Email
Parameters
- payload EmailSendRequest - emailSendRequest
createEmails
function createEmails(EmailSentCreateList payload) returns EmailSentCreateList|error
Create a set of Email Records
Parameters
- payload EmailSentCreateList - Email records to persist, with content.
Return Type
- EmailSentCreateList|error - Created
deleteEmails
Un-sync a batch of Email Records
Parameters
- payload SetOfIds - emailIds
Return Type
- json|error - OK
getEmail
function getEmail(int id) returns EmailSentQueryResultWithContent|error
Retrieve an Email
Parameters
- id int - id
Return Type
updateEmail
function updateEmail(int id, EmailSentCreate payload) returns EmailSentCreate|error
Update an Email Record
Return Type
- EmailSentCreate|error - OK
Deprecated
deleteEmail
Delete an Email Record
Parameters
- id int - id
listFiles
function listFiles(int? 'limit, int? offset, string? viewable, string? permission, string? 'type, string? name, int? contactId) returns FileList|error
List Files
Parameters
- 'limit int? (default ()) - Sets a total of items to return
- offset int? (default ()) - Sets a beginning range of items to return
- viewable string? (default ()) - Include public or private files in response (PUBLIC or PRIVATE), defaults to BOTH.
- permission string? (default ()) - Filter based on the permission of files (USER or COMPANY), defaults to BOTH.
- 'type string? (default ()) - Filter based on the type of file.
- name string? (default ()) - Filter files based on name, with '*' preceding or following to indicate LIKE queries.
- contactId int? (default ()) - Filter based on Contact Id, if user has permission to see Contact files.
createFile
function createFile(FileUpload payload) returns FileInformation|error
Upload File
Parameters
- payload FileUpload - fileUpload
Return Type
- FileInformation|error - OK
getFile
function getFile(int fileId, string[]? optionalProperties) returns FileInformation|error
Retrieve File
Parameters
- fileId int - fileId
- optionalProperties string[]? (default ()) - Comma-delimited list of File properties to include in the response. (Some fields such as
file_data
aren't included, by default.)
Return Type
- FileInformation|error - OK
updateFile
function updateFile(int fileId, FileUpload payload) returns FileInformation|error
Replace File
Return Type
- FileInformation|error - OK
deleteFile
Delete File
Parameters
- fileId int - fileId
listStoredHookSubscriptions
List Stored Hook Subscriptions
createAHookSubscription
function createAHookSubscription(RestHookRequest payload) returns RestHook|error
Create a Hook Subscription
Parameters
- payload RestHookRequest - restHookRequest
listHookEventTypes
List Hook Event Types
retrieveAHookSubscription
Retrieve a Hook Subscription
Parameters
- 'key string - key
updateAHookSubscription
function updateAHookSubscription(string 'key, RestHookRequest payload) returns RestHook|error
Update a Hook Subscription
deleteAHookSubscription
Delete a Hook Subscription
Parameters
- 'key string - key
verifyAHookSubscriptionDelayed
Verify a Hook Subscription, Delayed
verifyAHookSubscription
Verify a Hook Subscription
Parameters
- 'key string - key
listCountries
function listCountries() returns CountriesByCode|error
List Countries
Return Type
- CountriesByCode|error - OK
listCountriesProvinces
function listCountriesProvinces(string countryCode) returns ProvincesByCode|error
List a Country's Provinces
Parameters
- countryCode string - countryCode
Return Type
- ProvincesByCode|error - OK
getMerchantAccounts
function getMerchantAccounts() returns RestMerchantAccountResponse|error
List Merchants
Return Type
listNotes
List Notes
Parameters
- 'limit int? (default ()) - Sets a total of items to return
- offset int? (default ()) - Sets a beginning range of items to return
- userId int? (default ()) - Filter based on the user id assigned to the note.
- contactId int? (default ()) - Filter based on the contact id assigned to the note.
createNote
function createNote(RequestNote payload) returns Note|error
Create a Note
Parameters
- payload RequestNote - createNote
retrieveNoteModel
function retrieveNoteModel() returns ObjectModel|error
Retrieve Note Model
Return Type
- ObjectModel|error - OK
createNoteCustomField
function createNoteCustomField(CreateRestCustomField payload) returns CustomFieldMetaData|error
Create a Custom Field
Parameters
- payload CreateRestCustomField - customField
Return Type
- CustomFieldMetaData|error - Created
getNote
Retrieve a Note
Parameters
- noteId int - noteId
deleteNote
Delete a Note
Parameters
- noteId int - noteId
updatePropertiesOnNote
function updatePropertiesOnNote(int noteId, UpdateNote payload) returns Note|error
Update a Note
getUserInfo
function getUserInfo() returns UserInfoDTO|error
Retrieve User Info
Return Type
- UserInfoDTO|error - OK
listOpportunities
function listOpportunities(int? 'limit, int? offset, int? userId, int? stageId, string? searchTerm, string? 'order) returns OpportunityList|error
List Opportunities
Parameters
- 'limit int? (default ()) - Sets a total of items to return
- offset int? (default ()) - Sets a beginning range of items to return
- userId int? (default ()) - Returns opportunities for the provided user id
- stageId int? (default ()) - Returns opportunities for the provided stage id
- searchTerm string? (default ()) - Returns opportunities that match any of the contact's
given_name
,family_name
,company_name
, andemail_addresses
(searchesEMAIL1
only) fields as well asopportunity_title
- 'order string? (default ()) - Attribute to order items by
Return Type
- OpportunityList|error - OK
updateOpportunity
function updateOpportunity(Opportunity payload) returns Opportunity|error
Replace an Opportunity
Parameters
- payload Opportunity - opportunity
Return Type
- Opportunity|error - OK
createOpportunity
function createOpportunity(Opportunity payload) returns Opportunity|error
Create an Opportunity
Parameters
- payload Opportunity - opportunity
Return Type
- Opportunity|error - OK
retrieveOpportunityModel
function retrieveOpportunityModel() returns ObjectModel|error
Retrieve Opportunity Model
Return Type
- ObjectModel|error - OK
getOpportunity
function getOpportunity(int opportunityId, string[]? optionalProperties) returns Opportunity|error
Retrieve an Opportunity
Parameters
- opportunityId int - opportunityId
- optionalProperties string[]? (default ()) - Comma-delimited list of Opportunity properties to include in the response. (Some fields such as
custom_fields
aren't included, by default.)
Return Type
- Opportunity|error - OK
updatePropertiesOnOpportunity
function updatePropertiesOnOpportunity(int opportunityId, Opportunity payload) returns Opportunity|error
Update an Opportunity
Return Type
- Opportunity|error - OK
listOpportunityStagePipelines
function listOpportunityStagePipelines() returns SalesPipeline[]|error
List Opportunity Stage Pipeline
Return Type
- SalesPipeline[]|error - OK
listOrders
function listOrders(string? since, string? until, int? 'limit, int? offset, boolean? paid, string? 'order, int? contactId, int? productId, string? contactName) returns OrderList|error
List Orders
Parameters
- since string? (default ()) - Date to start searching from ex.
2017-01-01T22:17:59.039Z
- until string? (default ()) - Date to search to ex.
2017-01-01T22:17:59.039Z
- 'limit int? (default ()) - Sets a total of items to return
- offset int? (default ()) - Sets a beginning range of items to return
- paid boolean? (default ()) - Sets paid status of items to return
- 'order string? (default ()) - Attribute to order items by
- contactId int? (default ()) - Returns orders for the provided contact id
- productId int? (default ()) - Returns orders containing the provided product id
- contactName string? (default ()) - Returns orders for the provided contact name
createOrder
function createOrder(CreateOrder payload) returns Order|error
Create an Order
Parameters
- payload CreateOrder - createOrder
retrieveOrderModel
function retrieveOrderModel() returns ObjectModel|error
Retrieve Custom Order Model
Return Type
- ObjectModel|error - OK
getOrder
function getOrder(int orderId) returns EcommerceReportingOrder|error
Retrieve an Order
Parameters
- orderId int - orderId
Return Type
deleteOrder
Delete an Order
Parameters
- orderId int - orderId
createOrderItemsOnOrder
function createOrderItemsOnOrder(int orderId, CreateOrderItem payload) returns OrderItem|error
Create an Order Item
deleteOrderOrderItem
Delete an Order Item
replacePaymentPlan
function replacePaymentPlan(int orderId, PaymentPlan payload) returns PaymentPlan|error
Replace an Order Pay Plan
Return Type
- PaymentPlan|error - OK
listOrderPayments
function listOrderPayments(int orderId) returns InvoicePayment[]|error
Retrieve Order Payments
Parameters
- orderId int - orderId
Return Type
- InvoicePayment[]|error - OK
createPaymentOnOrder
function createPaymentOnOrder(int orderId, CreatePayment payload) returns PaymentResult|error
Create a Payment
Return Type
- PaymentResult|error - Created
listTransactionsForOrder
function listTransactionsForOrder(int orderId, string? since, string? until, int? 'limit, int? offset, int? contactId) returns TransactionList|error
Retrieve Order Transactions
Parameters
- orderId int - orderId
- since string? (default ()) - Date to start searching from ex.
2017-01-01T22:17:59.039Z
- until string? (default ()) - Date to search to ex.
2017-01-01T22:17:59.039Z
- 'limit int? (default ()) - Sets a total of items to return
- offset int? (default ()) - Sets a beginning range of items to return
- contactId int? (default ()) - Returns transactions for the provided contact id
Return Type
- TransactionList|error - OK
listProducts
function listProducts(int? 'limit, int? offset, boolean? active) returns ProductList|error
List Products
Parameters
- 'limit int? (default ()) - Sets a total of items to return
- offset int? (default ()) - Sets a beginning range of items to return
- active boolean? (default ()) - Sets status of items to return
Return Type
- ProductList|error - OK
createProduct
function createProduct(CreateProduct payload) returns Product|error
Create a Product
Parameters
- payload CreateProduct - createProduct
listProductsFromSyncToken
function listProductsFromSyncToken(string? syncToken, int? 'limit, int? offset) returns ProductStatusList|error
Retrieve Synced Products
Parameters
- syncToken string? (default ()) - sync_token
- 'limit int? (default ()) - Sets a total of items to return
- offset int? (default ()) - Sets a beginning range of items to return
Return Type
- ProductStatusList|error - OK
Deprecated
retrieveProduct
Retrieve a Product
Parameters
- productId int - productId
deleteProduct
Delete a Product
Parameters
- productId int - productId
updateProduct
function updateProduct(int productId, CreateProduct payload) returns Product|error
Update a Product
createProductImage
function createProductImage(int productId, CreateProductImage payload) returns Response|error
Upload a product image
deleteProductImage
Delete a product image
Parameters
- productId int - productId
createProductSubscription
function createProductSubscription(int productId, CreateProductSubscription payload) returns ProductSubscription|error
Create a Product Subscription
Return Type
- ProductSubscription|error - Created
retrieveProductSubscription
function retrieveProductSubscription(int productId, int subscriptionId) returns ProductSubscription|error
Retrieve a Product Subscription
Return Type
- ProductSubscription|error - OK
deleteProductSubscription
Delete a Product Subscription
getConfiguration
function getConfiguration() returns RestApplicationConfiguration|error
Retrieve application configuration
Return Type
getApplicationEnabled
Retrieve application status
getContactOptionTypes
List Contact types
listSubscriptions
function listSubscriptions(int? 'limit, int? offset, int? contactId) returns SubscriptionList|error
List Subscriptions
Parameters
- 'limit int? (default ()) - Sets a total of items to return
- offset int? (default ()) - Sets a beginning range of items to return
- contactId int? (default ()) - Returns subscriptions for the provided contact id
Return Type
- SubscriptionList|error - OK
createSubscription
function createSubscription(CreateSubscription payload) returns Subscription|error
Create Subscription
Parameters
- payload CreateSubscription - createSubscription
Return Type
- Subscription|error - Created
retrieveSubscriptionModel
function retrieveSubscriptionModel() returns ObjectModel|error
Retrieve Subscription Model
Return Type
- ObjectModel|error - OK
listTags
List Tags
Parameters
- 'limit int? (default ()) - Sets a total of items to return
- offset int? (default ()) - Sets a beginning range of items to return
- category int? (default ()) - Category Id of tags to filter by
- name string? (default ()) - Filter for tags with a specific name
createTag
Create Tag
Parameters
- payload CreateTag - tag
createTagCategory
function createTagCategory(CreateTagCategory payload) returns TagCategory|error
Create Tag Category
Parameters
- payload CreateTagCategory - tagCategory
Return Type
- TagCategory|error - OK
getTag
Retrieve a Tag
Parameters
- id int - id
listCompaniesForTagId
function listCompaniesForTagId(int tagId, int? 'limit, int? offset) returns TaggedCompanyList|error
List Tagged Companies
Parameters
- tagId int - tagId
- 'limit int? (default ()) - Sets a total of items to return
- offset int? (default ()) - Sets a beginning range of items to return
Return Type
- TaggedCompanyList|error - OK
listContactsForTagId
function listContactsForTagId(int tagId, int? 'limit, int? offset) returns TaggedContactList|error
List Tagged Contacts
Parameters
- tagId int - tagId
- 'limit int? (default ()) - Sets a total of items to return
- offset int? (default ()) - Sets a beginning range of items to return
Return Type
- TaggedContactList|error - OK
applyTagToContactIds
function applyTagToContactIds(int tagId, SetOfIds payload) returns EntryLongString[]|error
Apply Tag to Contacts
Return Type
- EntryLongString[]|error - OK
removeTagFromContactIds
Remove Tag from Contacts
removeTagFromContactId
Remove Tag from Contact
listTasks
function listTasks(int? contactId, boolean? hasDueDate, int? userId, string? since, string? until, boolean? completed, int? 'limit, int? offset, string? 'order) returns TaskList|error
List Tasks
Parameters
- contactId int? (default ()) - contact_id
- hasDueDate boolean? (default ()) - has_due_date
- userId int? (default ()) - user_id
- since string? (default ()) - Date to start searching from ex.
2017-01-01T22:17:59.039Z
- until string? (default ()) - Date to search to ex.
2017-01-01T22:17:59.039Z
- completed boolean? (default ()) - Sets completed status of items to return
- 'limit int? (default ()) - Sets a total of items to return
- offset int? (default ()) - Sets a beginning range of items to return
- 'order string? (default ()) - Attribute to order items by
createTask
Create a Task
Parameters
- payload Task - task
retrieveTaskModel
function retrieveTaskModel() returns ObjectModel|error
Retrieve Task Model
Return Type
- ObjectModel|error - OK
createTaskCustomField
function createTaskCustomField(CreateRestCustomField payload) returns CustomFieldMetaData|error
Create a Custom Field
Parameters
- payload CreateRestCustomField - customField
Return Type
- CustomFieldMetaData|error - Created
listTasksForCurrentUser
function listTasksForCurrentUser(int? contactId, boolean? hasDueDate, int? userId, string? since, string? until, boolean? completed, int? 'limit, int? offset, string? 'order) returns TaskList|error
Search Tasks
Parameters
- contactId int? (default ()) - Returns tasks for the provided contact id
- hasDueDate boolean? (default ()) - Returns tasks that have an 'action date' when set to true
- userId int? (default ()) - Returns tasks for the provided user id
- since string? (default ()) - Date to start searching from ex.
2017-01-01T22:17:59.039Z
- until string? (default ()) - Date to search to ex.
2017-01-01T22:17:59.039Z
- completed boolean? (default ()) - Sets completed status of items to return
- 'limit int? (default ()) - Sets a total of items to return
- offset int? (default ()) - Sets a beginning range of items to return
- 'order string? (default ()) - Attribute to order items by
getTask
Retrieve a Task
Parameters
- taskId string - taskId
updateTask
Replace a Task
deleteTask
Delete a Task
Parameters
- taskId string - taskId
updatePropertiesOnTask
Update a Task
listTransactions
function listTransactions(string? since, string? until, int? 'limit, int? offset, int? contactId) returns TransactionList|error
List Transactions
Parameters
- since string? (default ()) - Date to start searching from ex.
2017-01-01T22:17:59.039Z
- until string? (default ()) - Date to search to ex.
2017-01-01T22:17:59.039Z
- 'limit int? (default ()) - Sets a total of items to return
- offset int? (default ()) - Sets a beginning range of items to return
- contactId int? (default ()) - Returns transactions for the provided contact id
Return Type
- TransactionList|error - OK
getTransaction
function getTransaction(int transactionId) returns EcommerceReportingTransaction|error
Retrieve a Transaction
Parameters
- transactionId int - transactionId
Return Type
listUsers
function listUsers(int? 'limit, int? offset, boolean? includeInactive, boolean? includePartners) returns Users|error
List Users
Parameters
- 'limit int? (default ()) - Sets a total of items to return
- offset int? (default ()) - Sets a beginning range of items to return
- includeInactive boolean? (default ()) - Include users that are Inactive in results, defaults to TRUE
- includePartners boolean? (default ()) - Include partner users in results, defaults to TRUE
createUser
function createUser(CreateUser payload) returns RestUser|error
Create a User
Parameters
- payload CreateUser - user
getUserSignature
Get User email signature
Parameters
- userId int - userId
Records
keap: AccountProfile
Fields
- address ContactAddress? -
- business_goals string[]? - The goals of this business, ie. Grow Business, Convert more leads
- business_primary_color string? -
- business_secondary_color string? -
- business_type string? - The type of business
- currency_code string? -
- email string? -
- language_tag string? -
- logo_url string? -
- name string? -
- phone string? -
- phone_ext string? -
- time_zone string? -
- website string? -
keap: AchieveApiGoalEvent
Fields
- contact_id int -
keap: Address
Fields
- country_code string? -
- line1 string? -
- line2 string? -
- locality string? -
- region string? -
- zip_code string? -
- zip_four string? -
keap: AddressInformation
Fields
- company string? -
- country_code string? -
- first_name string? -
- is_invoice_to_company boolean? -
- last_name string? -
- line1 string? -
- line2 string? -
- locality string? -
- middle_name string? -
- phone string? -
- region string? -
- zip_code string? -
- zip_four string? -
keap: Affiliate
Fields
- code string? -
- contact_id int? -
- id int? -
- name string? -
- notify_on_lead boolean? -
- notify_on_sale boolean? -
- parent_id int? -
- status string? -
- track_leads_for int? -
keap: AffiliateClawbackList
Fields
- clawbacks RestAffiliateClawback[]? -
- count int? -
- next string? -
- previous string? -
keap: AffiliateCommission
Fields
- amount_earned float? -
- contact_first_name string? -
- contact_id int? -
- contact_last_name string? -
- date_earned string? -
- description string? -
- invoice_id int? -
- product_name string? -
- sales_affiliate_id int? -
- sold_by_first_name string? -
- sold_by_last_name string? -
keap: AffiliateCommissionList
Fields
- commissions AffiliateCommission[]? -
- count int? -
- next string? -
- previous string? -
keap: AffiliateList
Fields
- affiliates Affiliate[]? -
- count int? -
- next string? -
- previous string? -
keap: AffiliatePaymentList
Fields
- count int? -
- next string? -
- payments RestAffiliatePayment[]? -
- previous string? -
keap: AffiliateProgram
Fields
- affiliate_id int? -
- id int? -
- name string? -
- notes string? -
- priority int? -
keap: AffiliateProgramList
Fields
- count int? -
- next string? -
- previous string? -
- programs AffiliateProgram[]? -
keap: AffiliateRedirect
Fields
- affiliate_id int? -
- id int? -
- local_url_code string? -
- name string? -
- program_ids int[]? -
- redirect_url string? -
keap: AffiliateSummary
Fields
- affiliate_id int? -
- amount_earned decimal? -
- balance decimal? -
- clawbacks decimal? -
keap: AffiliateSummaryList
Fields
- count int? -
- summaries AffiliateSummary[]? -
keap: Appointment
Fields
- contact_id int? - Required for pop-up reminders
- description string? -
- end_date string -
- location string? -
- remind_time int? - Value in minutes before start_date to show pop-up reminder. Acceptable values are [5,10,15,30,60,120,240,480,1440,2880]
- start_date string -
- title string -
- user int? - Required only for pop-up reminders
keap: AppointmentList
Fields
- appointments Appointment[]? -
- count int? -
- next string? -
- previous string? -
- sync_token string? -
keap: BasicCompany
Fields
- company_name string? -
- email string? -
- id int? -
keap: BasicContact
Fields
- email string? -
- first_name string? -
- id int? -
- last_name string? -
keap: Campaign
Fields
- active_contact_count int? -
- completed_contact_count int? -
- created_by_global_id int? -
- date_created string? -
- error_message string? -
- goals Goal[]? -
- id int? -
- locked boolean? -
- name string? -
- published_date string? -
- published_status boolean? -
- published_time_zone string? -
- sequences Sequence[]? -
- time_zone string? -
keap: CampaignLandingPageNodeDTO
Fields
- formId string? -
- id string? -
- landingPageId string? -
- leadBucketIds string[]? -
- name string? -
- properties record {}? -
- ready boolean? -
- 'type string? -
keap: CampaignList
Fields
- campaigns Campaign[]? -
- count int? -
- next string? -
- previous string? -
keap: CampaignNodeDTO
Fields
- id string? -
- name string? -
- properties record {}? -
- ready boolean? -
- 'type string? -
keap: CategoryReference
Fields
- id int - The id for the tag category
keap: CheckListItemDetails
Fields
- description string? -
- done_date string? -
- id int? -
- instance_id int? -
- item_order int? -
- required boolean? -
keap: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
keap: Company
Fields
- address CompanyAddress? -
- company_name string? -
- custom_fields CustomFieldValue[]? -
- email_address string? -
- email_opted_in boolean? -
- email_status string? -
- fax_number CompanyFaxNumber? -
- id int? -
- notes string? -
- phone_number CompanyPhoneNumber? -
- website string? -
keap: CompanyAddress
Fields
- country_code string? -
- line1 string? -
- line2 string? -
- locality string? -
- region string? -
- zip_code string? -
- zip_four string? -
keap: CompanyFaxNumber
Fields
- number string? -
- 'type string? -
keap: CompanyList
Fields
- companies Company[]? -
- count int? -
- next string? -
- previous string? -
keap: CompanyPhoneNumber
Fields
- extension string? -
- number string? -
- 'type string? -
keap: CompanyReference
Fields
- company_name string? -
- id int? -
keap: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
keap: ContactAddress
Fields
- country_code string? -
- 'field string -
- line1 string? -
- line2 string? -
- locality string? -
- postal_code string? - Field used to store postal codes containing a combination of letters and numbers ex. 'EC1A', 'S1 2HE', '75000'
- region string? -
- zip_code string? - Mainly used in the United States, this is typically numeric. ex. '85001', '90002' Note: this is to be used instead of 'postal_code', not in addition to.
- zip_four string? - Last four of a full zip code ex. '8244', '4320'. This field is supplemental to the zip_code field, otherwise will be ignored.
keap: ContactCreditCard
Fields
- card_number string? -
- card_type string? -
- id int? -
- validation_status string? -
keap: ContactList
Fields
- contacts RestPartialContact[]? -
- count int? -
- next string? -
- previous string? -
keap: ContactOrigin
Fields
- date string -
- ip_address string -
keap: ContactTag
Fields
- date_applied string? -
- tag SimpleTag? -
keap: ContactTagList
Fields
- count int? -
- next string? -
- previous string? -
- tags ContactTag[]? -
keap: CountriesByCode
Fields
- countries record {} - A key-value pair of country code and country name.
keap: CreateAffiliate
Fields
- code string -
- contact_id int -
- name string? -
- notify_on_lead boolean? -
- notify_on_sale boolean? -
- parent_id int? -
- password string -
- status string? -
- track_leads_for int? -
keap: CreateContactOrigin
Fields
- ip_address string -
keap: CreateOrder
Fields
- contact_id int -
- lead_affiliate_id int? -
- order_date string -
- order_items CreateOrderItem[] -
- order_title string -
- order_type string -
- promo_codes string[]? - Uses multiple strings as promo codes. The corresponding discount will be applied to the order.
- sales_affiliate_id int? -
- shipping_address AddressInformation? -
keap: CreateOrderItem
Fields
- description string? -
- price string? - Overridable price of the product, if not specified, the default will be used. Must be greater than or equal to 0.
- product_id int - The id of the product to be added to the order.
- quantity int - Quantity must be greater than or equal to 1
keap: CreateOrPatchCompany
Fields
- address CompanyAddress? -
- company_name string -
- custom_fields CustomFieldValue[]? -
- email_address string? -
- fax_number CompanyFaxNumber? -
- notes string? -
- opt_in_reason string? -
- phone_number CompanyPhoneNumber? -
- website string? -
keap: CreateOrPatchContact
Fields
- addresses ContactAddress[]? -
- anniversary string? -
- birthday string? -
- company RequestCompanyReference? -
- contact_type string? -
- custom_fields CustomFieldValue[]? -
- email_addresses EmailAddress[]? -
- family_name string? -
- fax_numbers FaxNumber[]? -
- given_name string? -
- job_title string? -
- lead_source_id int? -
- middle_name string? -
- opt_in_reason string? -
- origin CreateContactOrigin? -
- owner_id int? -
- phone_numbers PhoneNumber[]? -
- preferred_locale string? -
- preferred_name string? -
- prefix string? -
- social_accounts SocialAccount[]? -
- source_type string? -
- spouse_name string? -
- suffix string? -
- time_zone string? -
- website string? -
keap: CreatePayment
Fields
- apply_to_commissions boolean? -
- charge_now boolean? -
- credit_card_id int? -
- date string? - Used when charge_now:false or inserting historical data. ex
2017-01-01T22:17:59.039Z
- notes string? -
- payment_amount string? -
- payment_gateway_id string? -
- payment_method_type string? -
keap: CreateProduct
Fields
- active boolean? -
- product_desc string? -
- product_name string -
- product_price decimal? -
- product_short_desc string? -
- sku string? -
- subscription_only boolean? -
keap: CreateProductImage
Fields
- checksum string? - SHA256 checksum of image in Hex.
- file_data string - The image data, base64 encoded.
- file_name string - The name of the file with extension, must match file data. Acceptable file types: [.png, .gif, .jpg, .jpeg].
keap: CreateProductSubscription
Fields
- active boolean? -
- cycle_type string -
- frequency int? -
- number_of_cycles int? -
- plan_price decimal -
- subscription_plan_index int? -
keap: CreateRestCustomField
Fields
- field_type string -
- group_id int? - An optional tab group to place the field under in the interface. If not specified, will default to the 'Custom Fields' tab.
- label string -
- options CreateRestCustomFieldOption[]? -
- user_group_id int? - An optional user group to choose from when selecting values for User or UserListBox fields.
keap: CreateRestCustomFieldOption
Fields
- label string? -
- options CreateRestCustomFieldOption[]? -
keap: CreateSubscription
Fields
- allow_duplicate boolean? - If true, it will disable the check to see if there is already an identical subscription for the contact. Default is false.
- allow_tax boolean? - Only works if the product the product subscription is for is taxable. Default is false.
- auto_charge boolean? - Defaults to true.
- billing_amount decimal? - Must be 0 or greater. Default is the price in the product subscription.
- contact_id int -
- credit_card_id int? - Default is the contact's most recently used card, if auto charge is true. Default is 0 otherwise.
- first_bill_date string? - The first day the subscription will bill, in EST. Must not be in the past. Default is today.
- payment_gateway_id int? - Default is the app's default merchant.
- quantity int? - Must be greater than 0. Default is 1.
- sale_affiliate_id int? -
- subscription_plan_id int? - Id of the product subscription.
keap: CreateTag
Fields
- category CategoryReference? -
- description string? - The tag description
- name string - The tag name
keap: CreateTagCategory
Fields
- description string? - The category description
- name string - The category name
keap: CreateUser
Fields
- admin boolean? -
- email string -
- given_name string -
- partner boolean? -
keap: CreditCard
Fields
- address ContactAddress? -
- card_number string? -
- card_type string? -
- email_address string? -
- expiration_month string? -
- expiration_year string? -
- maestro_issue_number string? -
- maestro_start_date_month string? -
- maestro_start_date_year string? -
- name_on_card string? -
- verification_code string? -
keap: CreditCardAdded
Fields
- address ContactAddress? -
- card_type string? -
- contact_id int? -
- email_address string? -
- expiration_month string? -
- expiration_year string? -
- id int? -
- maestro_issue_number string? -
- maestro_start_date_month string? -
- maestro_start_date_year string? -
- name_on_card string? -
- validation_message string? -
- validation_status string? -
keap: CustomFieldMetaData
Fields
- field_name string? -
- field_type string? -
- id int? -
- label string? -
- options CustomFieldOption[]? -
- record_type string? -
keap: CustomFieldOption
Fields
- id string? -
- label string? -
- options CustomFieldOption[]? -
keap: CustomFieldValue
Fields
- content record {}? -
- id int? -
keap: EcommerceReportingOrder
Fields
- allow_payment boolean? -
- allow_paypal boolean? -
- contact EcommerceReportingOrderContactDetails? -
- creation_date string? -
- id int? -
- lead_affiliate_id int? -
- modification_date string? -
- notes string? -
- order_date string? -
- order_items EcommerceReportingOrderItem[]? -
- payment_plan EcommerceReportingPaymentPlan? -
- recurring boolean? -
- refund_total decimal? -
- sales_affiliate_id int? -
- shipping_information EcommerceReportingShippingInformation? -
- source_type string? -
- status string? -
- terms string? -
- title string? -
- total decimal? -
- total_due decimal? -
- total_paid decimal? -
keap: EcommerceReportingOrderContactDetails
Fields
- company_name string? -
- email string? -
- first_name string? -
- id int? -
- job_title string? -
- last_name string? -
keap: EcommerceReportingOrderItem
Fields
- cost decimal? -
- description string? -
- discount decimal? -
- id int? -
- jobRecurringId int? -
- name string? -
- notes string? -
- price decimal? -
- product EcommerceReportingSimpleProduct? -
- quantity int? -
- specialAmount decimal? -
- specialId int? -
- specialPctOrAmt int? -
- subscriptionPlan SubscriptionPlan? -
- 'type string? -
keap: EcommerceReportingPaymentGateway
Fields
- merchant_account_id int? -
- use_default boolean? -
keap: EcommerceReportingPaymentPlan
Fields
- auto_charge boolean? -
- credit_card_id int? -
- days_between_payments int? -
- initial_payment_amount decimal? -
- initial_payment_date string? -
- number_of_payments int? -
- payment_gateway EcommerceReportingPaymentGateway? -
- plan_start_date string? -
keap: EcommerceReportingShippingInformation
Fields
- city string? -
- company string? -
- country string? -
- first_name string? -
- id int? -
- invoiceToCompany boolean? -
- last_name string? -
- middle_name string? -
- phone string? -
- state string? -
- street1 string? -
- street2 string? -
- zip string? -
keap: EcommerceReportingSimpleProduct
Fields
- description string? -
- id int? -
- name string? -
- shippable boolean? -
- sku string? -
- taxable boolean? -
keap: EcommerceReportingTransaction
Fields
- amount decimal? -
- collection_method string? -
- contact_id int? -
- currency string? -
- errors string? -
- gateway string? -
- gateway_account_name string? -
- id int? -
- order_ids string? -
- orders EcommerceReportingOrder[]? -
- paymentDate string? -
- status string? -
- test boolean? -
- transaction_date string? -
- 'type string? -
keap: EmailAddress
Fields
- email string? -
- 'field string -
keap: EmailSendRequest
Fields
- address_field string? - Email field of each Contact record to address the email to, such as 'Email', 'EmailAddress2', 'EmailAddress3' or '_CustomFieldName', defaulting to the contact's primary email
- attachments EmailSendRequestAttachment[]? - Attachments to be sent with each copy of the email, maximum of 10 with size of 1MB each
- contacts int[] - An array of Contact Ids to receive the email
- html_content string? - The HTML-formatted content of the email, encoded in Base64
- plain_content string? - The plain-text content of the email, encoded in Base64
- subject string - The subject line of the email
- user_id int - The infusionsoft user to send the email on behalf of
keap: EmailSendRequestAttachment
Fields
- file_data string? - The content of the attachment, encoded in Base64
- file_name string? - The filename of the attached file, including extension
keap: EmailSentCreate
Fields
- clicked_date string? -
- contact_id int? -
- headers string? -
- html_content string? - Base64 encoded HTML
- id int? -
- opened_date string? -
- original_provider string? - Provider that sent the email case insensitive, must be in list [GOOGLE, INFUSIONSOFT]. If omitted gets mapped to UNKNOWN.
- original_provider_id string? - Provider id that sent the email, must be unique when combined with provider. If omitted a UUID without dashes is autogenerated for the record.
- plain_content string? - Base64 encoded text
- provider_source_id string? - The email address of the synced email account that generated this message.
- received_date string? -
- sent_date string? -
- sent_from_address string? -
- sent_from_reply_address string? -
- sent_to_address string -
- sent_to_bcc_addresses string? -
- sent_to_cc_addresses string? -
- subject string? -
keap: EmailSentCreateError
Fields
- email EmailSentCreate? -
- error_message string? -
keap: EmailSentCreateList
Fields
- emails EmailSentCreate[]? -
- errors EmailSentCreateError[]? -
keap: EmailSentQueryResult
Fields
- clicked_date string? -
- contact_id int? -
- headers string? -
- id int? -
- opened_date string? -
- original_provider string? -
- original_provider_id string? -
- received_date string? -
- sent_date string? -
- sent_from_address string? -
- sent_from_reply_address string? -
- sent_to_address string? -
- sent_to_bcc_addresses string? -
- sent_to_cc_addresses string? -
- subject string? -
keap: EmailSentQueryResultList
Fields
- count int? -
- emails EmailSentQueryResult[]? -
- next string? -
- previous string? -
keap: EmailSentQueryResultWithContent
Fields
- clicked_date string? -
- contact_id int? -
- headers string? -
- html_content string? - Base64 encoded HTML
- id int? -
- opened_date string? -
- original_provider string? -
- original_provider_id string? -
- plain_content string? - Base64 encoded plain text
- received_date string? -
- sent_date string? -
- sent_from_address string? -
- sent_from_reply_address string? -
- sent_to_address string? -
- sent_to_bcc_addresses string? -
- sent_to_cc_addresses string? -
- subject string? -
keap: EntryLongString
Fields
- 'key string? -
keap: Error
Fields
- cause Throwable? -
- localizedMessage string? -
- message string? -
- stackTrace StackTraceElement[]? -
- suppressed Throwable[]? -
keap: FaxNumber
Fields
- 'field string -
- number string? -
- 'type string? -
keap: FileDescriptor
Fields
- category string? -
- contact_id int? -
- created_by int? -
- date_created string? -
- download_url string? -
- file_box_type string? -
- file_name string? -
- file_size int? -
- id int -
- last_updated string? -
- 'public boolean? -
- remote_file_key string? -
keap: FileInformation
Fields
- file_data string? -
- file_descriptor FileDescriptor? -
keap: FileList
Fields
- count int? -
- files FileDescriptor[]? -
- next string? -
- previous string? -
keap: FileUpload
Fields
- file_name string -
- 'public boolean? -
- file_data string -
- contact_id int -
- is_public boolean -
- file_association string -
keap: FullContact
Fields
- ScoreValue string? -
- addresses ContactAddress[]? -
- anniversary string? -
- birthday string? -
- company CompanyReference? -
- company_name string? -
- contact_type string? -
- custom_fields CustomFieldValue[]? -
- date_created string? -
- email_addresses EmailAddress[]? -
- email_opted_in boolean? -
- email_status string? -
- family_name string? -
- fax_numbers FaxNumber[]? -
- given_name string? -
- id int? -
- job_title string? -
- last_updated string? -
- lead_source_id int? -
- middle_name string? -
- opt_in_reason string? -
- origin ContactOrigin? -
- owner_id int? -
- phone_numbers PhoneNumber[]? -
- preferred_locale string? -
- preferred_name string? -
- prefix string? -
- relationships Relationship[]? -
- social_accounts SocialAccount[]? -
- source_type string? -
- spouse_name string? -
- suffix string? -
- tag_ids int[]? -
- time_zone string? -
- website string? -
keap: Goal
Fields
- historical_contact_counts HistoricalCounts? -
- id int? -
- name string? -
- next_sequence_ids int[]? -
- previous_sequence_ids int[]? -
- 'type string? -
keap: GoalEventResultDTO
Fields
- campaign_id int? -
- goal_id int? -
- message string? -
- success boolean? -
keap: HistoricalCounts
Fields
- '24\_hours int? -
- '30\_days int? -
keap: InvoicePayment
Fields
- amount decimal? -
- id int? -
- invoice_id int? -
- last_updated string? -
- note string? -
- pay_date string? -
- pay_status string? -
- payment_id int? -
- refund_invoice_payment_id int? -
- skip_commission boolean? -
keap: Item
Fields
- id int? -
- name string? -
- next_item_id int? -
- previous_item_id int? -
- 'type string? -
keap: Merchant
Fields
- account_name string? -
- id int? -
- is_test boolean? -
- 'type string? -
keap: Note
Fields
- body string? -
- contact_id int? -
- date_created string? -
- id int? -
- last_updated string? -
- last_updated_by NoteUser? -
- title string? -
- 'type string? -
- user_id int? -
keap: NoteList
Fields
- count int? -
- next string? -
- notes Note[]? -
- previous string? -
keap: NoteUser
Fields
- family_name string? -
- given_name string? -
- user_id int? -
keap: OAuth2RefreshTokenGrantConfig
OAuth2 Refresh Token Grant Configs
Fields
- Fields Included from *OAuth2RefreshTokenGrantConfig
- refreshUrl string(default "https://api.infusionsoft.com/token") - Refresh URL
keap: ObjectModel
Fields
- custom_fields CustomFieldMetaData[]? -
- optional_properties string[]? - These fields are not transmitted by default on this model, but can be requested by specifying them in a comma-separated list in the optional_properties query parameter.
keap: Opportunity
Fields
- affiliate_id int? -
- contact OpportunityContact -
- custom_fields CustomFieldValue[]? -
- date_created string? -
- estimated_close_date string? -
- id int? -
- include_in_forecast int? -
- last_updated string? -
- next_action_date string? -
- next_action_notes string? -
- opportunity_notes string? -
- opportunity_title string -
- projected_revenue_high decimal? -
- projected_revenue_low decimal? -
- stage SimpleOpportunityStage -
- user SimpleUser? -
keap: OpportunityContact
Fields
- company_name string? -
- email string? -
- first_name string? -
- id int -
- job_title string? -
- last_name string? -
- phone_number string? -
keap: OpportunityList
Fields
- count int? -
- next string? -
- opportunities Opportunity[]? -
- previous string? -
keap: Order
Fields
- contact EcommerceReportingOrderContactDetails? -
- creation_date string? -
- id int? -
- lead_affiliate_id int? -
- modification_date string? -
- notes string? -
- order_date string? -
- order_items OrderItem[]? -
- payment_plan PaymentPlan? -
- refund_total decimal? -
- sales_affiliate_id int? -
- shipping_information AddressInformation? -
- status string? -
- title string? -
- total decimal? -
- total_due decimal? -
- total_paid decimal? -
keap: OrderItem
Fields
- billingCycle int? -
- cost decimal? -
- description string? -
- discount decimal? -
- frequency int? -
- id int? -
- jobRecurringId int? -
- name string? -
- notes string? -
- numberOfPayments int? -
- orderItemTaxes OrderItemTax[]? -
- price decimal? -
- product Product? -
- quantity int? -
- recurringBilling boolean? -
- specialAmount decimal? -
- specialId int? -
- specialPctOrAmt int? -
- 'type string? -
keap: OrderItemTax
Fields
- amount decimal? -
- id int? -
- name string? -
- orderItemId int? -
- rate decimal? -
- taxTemplateId int? -
keap: OrderList
Fields
- count int? -
- next string? -
- orders EcommerceReportingOrder[]? -
- previous string? -
keap: PaymentGateway
Fields
- merchant_account_id int? -
- use_default boolean? -
keap: PaymentPlan
Fields
- auto_charge boolean? -
- credit_card_id int? -
- days_between_payments int -
- initial_payment_amount decimal? -
- initial_payment_date string? -
- number_of_payments int -
- payment_gateway PaymentGateway? -
- plan_start_date string -
keap: PaymentResult
Fields
- id int? -
- invoice_id int? -
- payment_amount decimal? -
- payment_status string? -
- transaction_id int? -
keap: PhoneNumber
Fields
- extension string? -
- 'field string -
- number string? -
- 'type string? -
keap: Product
Fields
- id int? -
- product_desc string? -
- product_name string? -
- product_options ProductOption[]? -
- product_price decimal? -
- product_short_desc string? -
- sku string? -
- status int? -
- subscription_only boolean? -
- subscription_plans SubscriptionPlan[]? -
- url string? -
keap: ProductList
Fields
- count int? -
- next string? -
- previous string? -
- products Product[]? -
- sync_token string? -
keap: ProductOption
Fields
- allow_spaces boolean? -
- can_contain_character boolean? -
- can_contain_number boolean? -
- can_end_with_character boolean? -
- can_end_with_number boolean? -
- can_start_with_character boolean? -
- can_start_with_number boolean? -
- display_index int? -
- id int? -
- label string? -
- max_chars int? -
- min_chars int? -
- name string? -
- required boolean? -
- text_message string? -
- 'type string? -
- values ProductOptionValue[]? -
keap: ProductOptionValue
Fields
- id int? -
- index int? -
- is_default boolean? -
- label string? -
- price_adjustment decimal? -
- sku string? -
keap: ProductStatus
Fields
- product Product? -
- status string? -
keap: ProductStatusList
Fields
- count int? -
- next string? -
- previous string? -
- product_statuses ProductStatus[]? -
- sync_token string? -
keap: ProductSubscription
Fields
- active boolean? -
- cycle_type string -
- frequency int? -
- id int -
- number_of_cycles int? -
- plan_price decimal -
- subscription_plan_index int? -
- subscription_plan_name string? -
- url string? -
keap: ProvincesByCode
Fields
- provinces record {} - A key-value pair of province code and province name.
keap: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
keap: RedirectList
Fields
- count int? -
- next string? -
- previous string? -
- redirects AffiliateRedirect[]? -
keap: Relationship
Fields
- id int? -
- linked_contact_id int? -
- relationship_type_id int? -
keap: RequestCompanyReference
Fields
- id int? -
keap: RequestNote
Fields
- body string? -
- contact_id int -
- title string? -
- 'type string? -
- user_id int? -
keap: RestAffiliateClawback
Fields
- amount decimal? -
- contact_id int? -
- date_earned string? -
- description string? -
- family_name string? -
- given_name string? -
- invoice_id int? -
- product_name string? -
- sale_affiliate_id int? -
- sold_by_family_name string? -
- sold_by_given_name string? -
- subscription_plan_name string? -
keap: RestAffiliatePayment
Fields
- amount decimal? -
- date string? -
- id int? -
- notes string? -
- 'type string? -
keap: RestApplicationConfiguration
Fields
- affiliate RestApplicationConfigurationModuleAffiliate? -
- application RestApplicationConfigurationModuleApplication? -
- appointment RestApplicationConfigurationModuleAppointment? -
- contact RestApplicationConfigurationModuleContact? -
- ecommerce RestApplicationConfigurationModuleECommerce? -
- fulfillment RestApplicationConfigurationModuleFulfillment? -
- invoice RestApplicationConfigurationModuleInvoice? -
- opportunity RestApplicationConfigurationModuleOpportunity? -
- template RestApplicationConfigurationModuleTemplate? -
keap: RestApplicationConfigurationModuleAffiliate
Fields
- choose_affiliate boolean? -
- commission RestApplicationConfigurationModuleAffiliateCommission? -
- custom_affiliate_url string? -
- display_affiliate_ip_address boolean? -
- do_not_notify_affiliate boolean? -
- skip_pay_if_unused boolean? -
- use_referral_history_if_no_tracking_cookie boolean? -
keap: RestApplicationConfigurationModuleAffiliateCommission
Fields
- calculation_type string? -
- levels int? -
- participant_types string? -
keap: RestApplicationConfigurationModuleApplication
Fields
- default_view_locale string? -
- features_enabled RestApplicationConfigurationModuleApplicationFeaturesEnabled? -
- time_zone string? -
keap: RestApplicationConfigurationModuleApplicationCompany
Fields
- caller_id_number string? -
- city string? -
- country string? -
- email string? -
- name string? -
- phone string? -
- phone_ext string? -
- state string? -
- street_address_1 string? -
- street_address_2 string? -
- web_logo_url string? -
- website string? -
- zip string? -
keap: RestApplicationConfigurationModuleApplicationFeaturesEnabled
Fields
- marketing boolean? -
keap: RestApplicationConfigurationModuleAppointment
Fields
- appointment_types string? -
- share_opportunity_with_related_user boolean? -
keap: RestApplicationConfigurationModuleContact
Fields
- address_labels RestApplicationConfigurationModuleContactAddressLabels? -
- contact_types string? -
- default_new_contact_form string? -
- disable_contact_edit_in_client_login boolean? -
- fax_types string? -
- phone_types string? -
- suffix_types string? -
- title_types string? -
keap: RestApplicationConfigurationModuleContactAddressLabels
Fields
- line_1 string? -
- line_2 string? -
- line_3 string? -
keap: RestApplicationConfigurationModuleECommerce
Fields
- credit_card_types string? -
- currency string? -
- default_charge_max_retry_attempts int? -
- default_country string? -
- default_merchant string? -
- default_number_of_days_between_charge_attempts int? -
- default_tax string? -
- default_to_auto_charge boolean? -
- merchant_account_max_retry_attempts int? -
- payment_types string? -
- promo_codes string? -
- track_cost_per_unit boolean? -
- track_inventory boolean? -
keap: RestApplicationConfigurationModuleEMail
Fields
- append_contact_key_to_links boolean? -
- default_opt_in_link string? -
- default_opt_out_link string? -
- hide_emails_to_and_from_domains string? -
- whitelisted_domains string? -
keap: RestApplicationConfigurationModuleForms
Fields
- spam_filters_enabled boolean? -
keap: RestApplicationConfigurationModuleFulfillment
Fields
- default_message_fields string? -
- default_message_to string? -
keap: RestApplicationConfigurationModuleInvoice
Fields
- tax_label string? -
keap: RestApplicationConfigurationModuleNote
Fields
- appointment_types string? -
- share_opportunity_with_related_user boolean? -
keap: RestApplicationConfigurationModuleOpportunity
Fields
- default_stage string? -
keap: RestApplicationConfigurationModuleOpportunityStates
Fields
keap: RestApplicationConfigurationModuleOpportunityStatesActive
Fields
- stages string? -
keap: RestApplicationConfigurationModuleOpportunityStatesLoss
Fields
- reasons string? -
- stage string? -
keap: RestApplicationConfigurationModuleOpportunityStatesWin
Fields
- reasons string? -
- stage string? -
keap: RestApplicationConfigurationModuleTask
Fields
- appointment_types string? -
- share_opportunity_with_related_user boolean? -
keap: RestApplicationConfigurationModuleTemplate
Fields
- default_country_code int? -
- default_user_id int? -
keap: RestEmailAddress
Fields
- email string -
- opted_in boolean -
- status string -
keap: RestHook
Fields
- eventKey string? -
- hookUrl string? -
- 'key string? -
- status string? -
keap: RestHookRequest
Fields
- eventKey string? -
- hookUrl string? -
keap: RestMerchantAccountResponse
Fields
- default_merchant_account int? -
- merchant_accounts Merchant[]? -
keap: RestPartialContact
Fields
- ScoreValue string? -
- addresses ContactAddress[]? -
- anniversary string? -
- birthday string? -
- company CompanyReference? -
- company_name string? -
- contact_type string? -
- custom_fields CustomFieldValue[]? -
- date_created string? -
- email_addresses EmailAddress[]? -
- email_opted_in boolean? -
- email_status string? -
- family_name string? -
- fax_numbers FaxNumber[]? -
- given_name string? -
- id int? -
- job_title string? -
- last_updated string? -
- lead_source_id int? -
- middle_name string? -
- owner_id int? -
- phone_numbers PhoneNumber[]? -
- preferred_locale string? -
- preferred_name string? -
- prefix string? -
- social_accounts SocialAccount[]? -
- source_type string? -
- spouse_name string? -
- suffix string? -
- time_zone string? -
- website string? -
keap: RestUser
Fields
- address Address? -
- company_name string? -
- created_by int? -
- date_created string? -
- email_address string? -
- family_name string? -
- fax_numbers FaxNumber[]? -
- given_name string? -
- global_user_id int? -
- id int -
- infusionsoft_id string? -
- job_title string? -
- last_updated string? -
- last_updated_by int? -
- middle_name string? -
- partner boolean? -
- phone_numbers PhoneNumber[]? -
- preferred_name string? -
- status string? -
- time_zone string? -
- website string? -
keap: SalesPipeline
Fields
- end_stage boolean? -
- is_default boolean? -
- stage_count int? -
- stage_id int? -
- stage_name string? -
- stage_order int? -
keap: Sequence
Fields
- active_contact_count int? -
- active_contact_count_completed int? -
- historical_contact_count HistoricalCounts? -
- id int? -
- name string? -
- paths SequencePath[]? -
keap: SequencePath
Fields
- id int? -
- items Item[]? -
keap: SetOfIds
Fields
- ids int[] -
keap: Setting
Fields
- value string? -
keap: SimpleOpportunityStage
Fields
- details StageDetails? -
- id int -
- name string? -
- reasons string[]? -
keap: SimpleTag
Fields
- category string? -
- id int? -
- name string? -
keap: SimpleUser
Fields
- first_name string? -
- id int? -
- last_name string? -
keap: SocialAccount
Fields
- name string? -
- 'type string? -
keap: StackTraceElement
Fields
- className string? -
- fileName string? -
- lineNumber int? -
- methodName string? -
- nativeMethod boolean? -
keap: StageDetails
Fields
- check_list_items CheckListItemDetails[]? -
- probability int? -
- stage_order int? -
- target_num_days int? -
keap: Subscription
Fields
- active boolean? -
- allow_tax boolean? -
- auto_charge boolean? -
- billing_amount decimal? -
- billing_cycle string? -
- billing_frequency int? -
- contact_id int? -
- credit_card_id int? -
- end_date string? -
- id int? -
- next_bill_date string? -
- payment_gateway_id int? -
- product_id int? -
- quantity int? -
- sale_affiliate_id int? -
- start_date string? -
- subscription_plan_id int? -
- use_default_payment_gateway boolean? -
keap: SubscriptionList
Fields
- count int? -
- next string? -
- previous string? -
- subscriptions Subscription[]? -
keap: SubscriptionPlan
Fields
- active boolean? -
- cycle int? -
- frequency int? -
- id int? -
- number_of_cycles int? -
- plan_price decimal? -
- subscription_plan_index int? -
- subscription_plan_name string? -
- url string? -
keap: Tag
Fields
- category TagCategory? -
- description string? - The tag description
- id int - The id for the tag
- name string - The tag name
keap: TagCategory
Fields
- description string? - The category description
- id int - The id for the tag category
- name string - The category name
keap: TaggedCompany
Fields
- company BasicCompany? -
- date_applied string? -
keap: TaggedCompanyList
Fields
- companies TaggedCompany[]? -
- count int? -
- next string? -
- previous string? -
keap: TaggedContact
Fields
- contact BasicContact? -
- date_applied string? -
keap: TaggedContactList
Fields
- contacts TaggedContact[]? -
- count int? -
- next string? -
- previous string? -
keap: TagId
Fields
- tagIds int[]? -
keap: Tags
Fields
- count int? -
- next string? -
- previous string? -
- tags Tag[]? -
keap: Task
Fields
- completed boolean? -
- completion_date string? -
- contact BasicContact? -
- creation_date string? -
- description string? -
- due_date string? -
- modification_date string? -
- priority int? -
- remind_time int? - Value in minutes before start_date to show pop-up reminder. Acceptable values are [5,10,15,30,60,120,240,480,1440,2880]
- title string? -
- 'type string? -
- url string? -
- user_id int? -
keap: TaskList
Fields
- count int? -
- next string? -
- previous string? -
- sync_token string? -
- tasks Task[]? -
keap: Throwable
Fields
- cause Throwable? -
- localizedMessage string? -
- message string? -
- stackTrace StackTraceElement[]? -
- suppressed Throwable[]? -
keap: TransactionList
Fields
- count int? -
- next string? -
- previous string? -
- transactions EcommerceReportingTransaction[]? -
keap: UpdateEmailAddress
Fields
- opted_in boolean -
- reason string -
keap: UpdateNote
Fields
- body string? -
- contact_id int? -
- title string? -
- 'type string? -
- user_id int? -
keap: UpsertContact
Fields
- addresses ContactAddress[]? -
- anniversary string? -
- birthday string? -
- company RequestCompanyReference? -
- contact_type string? -
- custom_fields CustomFieldValue[]? -
- duplicate_option string? -
- email_addresses EmailAddress[]? -
- family_name string? -
- fax_numbers FaxNumber[]? -
- given_name string? -
- job_title string? -
- lead_source_id int? -
- middle_name string? -
- opt_in_reason string? -
- origin CreateContactOrigin? -
- owner_id int? -
- phone_numbers PhoneNumber[]? -
- preferred_locale string? -
- preferred_name string? -
- prefix string? -
- social_accounts SocialAccount[]? -
- source_type string? -
- spouse_name string? -
- suffix string? -
- time_zone string? -
- website string? -
keap: UserInfoDTO
Fields
- email string -
- family_name string -
- given_name string -
- global_user_id int -
- infusionsoft_id string -
- middle_name string? -
- preferred_name string? -
- sub string -
keap: Users
Fields
- count int? -
- next string? -
- previous string? -
- users RestUser[]? -
Import
import ballerinax/keap;
Metadata
Released date: almost 2 years ago
Version: 1.3.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 2
Current verison: 2
Weekly downloads
Keywords
Sales & CRM/Customer Relationship Management
Cost/Paid
Contributors
Dependencies