instagram.bussiness
Module instagram.bussiness
API
Definitions
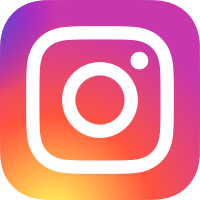
ballerinax/instagram.bussiness Ballerina library
Overview
This is a generated connector for Instagram Graph API v12.0 OpenAPI specification.
Instagram is a free photo-sharing application that enables its users to take photos, apply filters, and share them on social networks.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Instagram account.
- Obtain tokens by following this guide.
Quickstart
To use the Instagram connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/instagram.bussiness
module into the Ballerina project.
import ballerinax/instagram.bussiness;
Step 2: Create a new connector instance
Initialize the connector.
bussiness:BearerTokenConfig apiKeyConfig = { token = "<ACCESS_TOKEN>" }; bussiness:Client baseClient = check new Client(apiKeyConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example to get fields of a image, video, or album using the connector.
public function main() { string[] fields = ["id","username"]; string mediaId = "2684065555656187876_50003825255"; bussiness:MediaFieldsObject|error result = baseClient->getMediaInfo(mediaId, fields); if (result is bussiness:MediaFieldsObject) { log:printInfo(result.toString()); } else { log:printInfo(result.toString()); } }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
instagram.bussiness: Client
This is a generated connector for Instagram Graph API v12.0 OpenAPI specification. Instagram is a free photo-sharing application that enables its users to take photos, apply filters, and share them on social networks. The Instagram Graph API is a collection of Facebook Graph API endpoints that allow apps to access data in Instagram Professional accounts (both Business and Creator accounts).
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create an Instagram account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://graph.instagram.com/" - URL of the target service
getMediaInfo
function getMediaInfo(string igMediaId, string[]? fields) returns MediaFieldsObject|error
Retrieves information about a media object.
Parameters
- igMediaId string - ID of the image, video, or album.
- fields string[]? (default ()) - A comma-separated list of Fields you want returned.
Return Type
- MediaFieldsObject|error - Success
getMediaComments
Getting Comments on a Media Object.
Parameters
- igMediaId string - ID of the image, video, or album.
createComment
Creating a Comment on a Media Object
Parameters
- igMediaId string - ID of the image, video, or album.
- message string - The text to be included in the comment.
getMediaInsights
function getMediaInsights(string igMediaId, string[]? metric) returns MediaMetrics|error
Get insights data on an IG Media object.
Parameters
- igMediaId string - ID of the image, video, or album.
- metric string[]? (default ()) - A comma-separated list of Metrics you want returned.
Return Type
- MediaMetrics|error - Success
getUserData
function getUserData(string igUserId, string username, string fieldset) returns BussinessDiscoveryData|error
Returns data about another Instagram Business or Creator IG User.
Perform this request on the Instagram Business or Creator IG User who is making the query, and identify the targeted business with the username parameter.
Parameters
- igUserId string - ID of an IG User who calls the function.
- username string - The username of the Instagram Business or Creator IG User you want to get data about.
- fieldset string - A comma-separated list of Fields you want returned.
Return Type
- BussinessDiscoveryData|error - Success
getUserInsights
function getUserInsights(string igUserId, string period, int since, int until, string[]? metric) returns UserMetrics|error
Returns insights on an IG User.
Parameters
- igUserId string - ID of an IG User who calls the function.
- period string - A Period that is compatible with the metrics you are requesting.
- since int - Used in conjunction with {until} to define a Range. If you omit since and until, the API defaults to a 2 day range - yesterday through today.
- until int - Used in conjunction with {since} to define a Range. If you omit since and until, the API defaults to a 2 day range - yesterday through today.
- metric string[]? (default ()) - A comma-separated list of Metrics you want returned. If requesting multiple metrics, they must all have the same compatible Period.
Return Type
- UserMetrics|error - Success
getUserMedia
Gets all IG Media objects on an IG User.
Parameters
- igUserId string - ID of an IG User who calls the function.
getHashtagFields
function getHashtagFields(string igHashtagId, string[]? fields) returns HashtagResponse|error
Returns Fields and Edges on an IG Hashtag.
Parameters
- igHashtagId string - The ID of the IG Hashtag.
- fields string[]? (default ()) - A comma-separated list of Fields and Edges you want returned. If omitted, default fields will be returned.
Return Type
- HashtagResponse|error - Success
getRecentHashtagMedia
function getRecentHashtagMedia(string igHashtagId, string userId, string[]? fields) returns Media|error
Returns a list of the most recently published photo and video IG Media objects published with a specific hashtag.
Parameters
- igHashtagId string - The ID of the IG Hashtag.
- userId string - The ID of the IG User performing the query.
- fields string[]? (default ()) - A comma-separated list of Fields you want returned.
searchHashtag
Returns the ID of an IG Hashtag.
Records
instagram.bussiness: BussinessDiscoveryData
Bussiness discover data object.
Fields
- business_discovery BussinessdiscoverydataBusinessDiscovery? - Information about the Instagram Business account.
instagram.bussiness: BussinessdiscoverydataBusinessDiscovery
Information about the Instagram Business account.
Fields
- biography string? - The bio of the person.
- id string? - The unique identifier of the Instagram business account.
- ig_id string? - Instagram ID.
- followers_count int? - The followers count of the profile.
- follows_count int? - The follows count of the profile.
- media_count int? - The media count of the profile.
- name string? - The name of the person.
- profile_picture_url string? - The profile picture of the person.
- username string? - The username of the person.
- website string? - The website of the person.
- media Media? - An object containing the media data requested.
instagram.bussiness: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
instagram.bussiness: Comment
Comment information.
Fields
- id string? - ID of the comment.
- timestamp string? - Time when the comment is created.
- text string? - Comment content.
- hidden boolean? - The boolean that shows if the comment is hidden.
- like_count int? - The number of likes.
- media string? - Comment media.
- replies string? - Replies for comment.
- user string? - Comment author.
- username string? - The username for the user.
instagram.bussiness: CommentResponse
Comment response.
Fields
- id string? - ID of the comment.
instagram.bussiness: Comments
An object containing the media data requested.
Fields
- data Comment[]? - An array containing comments.
- paging Paging? - A cursor-paginated edge.
instagram.bussiness: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
instagram.bussiness: HashtagResponse
Hashtag response.
Fields
- id string? - The hashtag's ID (included by default). IDs are static and global.
- name string? - The hashtag's name, without the leading hash symbol.
instagram.bussiness: Hashtags
Fields
- data HashtagResponse[]? - An array containing hashtag IDs.
instagram.bussiness: Media
An object containing the media data requested.
Fields
- data MediaFieldsObject[]? - An array containing requested media fields.
- paging Paging? - A cursor-paginated edge.
instagram.bussiness: MediaFieldsObject
Media fields.
Fields
- id string? - The Media's ID.
- caption string? - The Media's caption text. Not returnable for Media in albums.
- media_type string? - The Media's type. Can be
IMAGE
,VIDEO
, orCAROUSEL_ALBUM
.
- media_url string? - Media URL. Will be omitted from responses if the media contains copyrighted material, or has been flagged for a copyright violation.
- permalink string? - The Media's permanent URL.
- thumbnail_url string? - The Media's thumbnail image URL. Only available on VIDEO Media.
- timestamp string? - ISO 8601 formatted creation date in UTC (default is UTC ±00:00)
- username string? - Username of user who created the media.
- owner string? - ID of Instagram user who created the media. Only returned if the app user making the query also created the media, otherwise username field will be returned instead.
- comments_count int? - Count of comments on the media. Excludes comments on album child media and the media's caption. Includes replies on comments.
- ig_id string? - Instagram media ID.
- is_comment_enabled boolean? - Indicates if comments are enabled or disabled. Excludes album children.
- like_count int? - Count of likes on the media. Excludes likes on album child media and likes on promoted posts created from the media. Includes replies on comments.
- media_product_type string? - Surface where the media is published. Can be AD, FEED, IGTV, or STORY. Will return FEED instead of IGTV if targeting an Instagram TV video created on or after October 5, 2021.
- shortcode int? - Shortcode to the media.
- video_title string? - Instagram TV media title. Will not be returned if targeting an Instagram TV video created on or after October 5, 2021.
instagram.bussiness: MediaMetricObject
Media metric object.
Fields
- name string? - Name
- period string? - Period
- values MediametricobjectValues? - Values
- title string? - Title
- description string? - Description
- id string? - ID
instagram.bussiness: MediametricobjectValues
Values
Fields
- value anydata[]? - Value
instagram.bussiness: MediaMetrics
Media metrics
Fields
- data MediaMetricObject[]? - Array of media metrics.
instagram.bussiness: OAuth2RefreshTokenGrantConfig
OAuth2 Refresh Token Grant Configs
Fields
- Fields Included from *OAuth2RefreshTokenGrantConfig
- refreshUrl string(default "https://api.instagram.com/oauth/access_token") - Refresh URL
instagram.bussiness: Paging
A cursor-paginated edge.
Fields
- cursors PagingCursors? - Pagination cursor.
- next anydata? - The Graph API endpoint that will return the next page of data. If not included, this is the last page of data. Due to how pagination works with visibility and privacy, it is possible that a page may be empty but contain a next paging link. Stop paging when the next link no longer appears.
- previous anydata? - The Graph API endpoint that will return the previous page of data. If not included, this is the first page of data.
instagram.bussiness: PagingCursors
Pagination cursor.
Fields
- before string? - This is the cursor that points to the start of the page of data that has been returned.
- after string? - This is the cursor that points to the end of the page of data that has been returned.
instagram.bussiness: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
instagram.bussiness: UserMetricObject
User metric object.
Fields
- name string? - Name
- period string? - Period
- values MediametricobjectValues? - Values
- title string? - Title
- description string? - Description
- id string? - ID
instagram.bussiness: UserMetrics
Array of user metrics
Fields
- data UserMetricObject[]? - Array of user metrics
Import
import ballerinax/instagram.bussiness;
Metadata
Released date: about 2 years ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 5
Current verison: 5
Weekly downloads
Keywords
Marketing/Social Media Accounts
Cost/Free
Contributors
Dependencies