icons8
Module icons8
API
Definitions
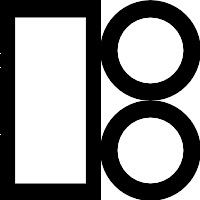
ballerinax/icons8 Ballerina library
Overview
This is a generated connector for Icons8 API v3.0 OpenAPI specification.
The Icons8 API provides the capability to search and obtain icons.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create an Icons8 account.
- Obtain tokens - Follow this guide.
Quickstart
To use the Icons8 connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/icons8 module into the Ballerina project.
import ballerinax/icons8;
Step 2: Create a new connector instance
You can now make the connection configuration using the Icons8 API keys config.
icons8:ApiKeysConfig & readonly apiKeyConfig = ?; icons8:Client iconsClient = check new (apiKeyConfig);
Step 3: Invoke connector operation
Following is code demonstrates how to get icons created after a date using ballerinax/icons8
connector.
public function main() returns error? { icons8:TotalsResponse response = check iconsClient->getTotals("2014-12-31"); }
Clients
icons8: Client
This is a generated connector for Icons8 API v3.0 OpenAPI specification.
The Icons8 API provides the capability to search and obtain icons.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create an Icons8 account and obtain tokens by following this guide.
init (ApiKeysConfig apiKeyConfig, ConnectionConfig config, string serviceUrl)
- apiKeyConfig ApiKeysConfig - API keys for authorization
- config ConnectionConfig {} - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.icons8.com" - URL of the target service
getCategories
function getCategories(string platform, string language) returns Categories|error
Categories
Parameters
- platform string - the platform that we are searching icons for
- language string - the language code to get localized result
Return Type
- Categories|error - OK
getByCategory
function getByCategory(string category, string subcategory, decimal amount, decimal offset, string platform, string language) returns Icon|error
By Category
Parameters
- category string - the code of category
- subcategory string - the code of subcategory
- amount decimal - the maximum number of icons which you'd like to receive
- offset decimal - the offset from the first received result
- platform string - the style of the icons
- language string - the language code to get localized result
getLatest
function getLatest(decimal amount, decimal offset, string term, string platform, string language) returns LatestIcons|error
Latest
Parameters
- amount decimal - the maximum number of icons which you'd like to receive
- offset decimal - the offset from the first received result
- term string - Term
- platform string - the style of the icons
- language string - the language code to get localized result
Return Type
- LatestIcons|error - OK
getByKeyword
function getByKeyword(string term, decimal amount, boolean exactAmount, decimal offset, string platform, string language) returns Category|error
By Keyword v3
Parameters
- term string - the name or tag of the icon or any other phrase. e.g. use "@home" to find icons with the tag "home" and "=home" to find icons with the name "home"
- amount decimal - the maximum number of icons which you'd like to receive (will be multiplied by platforms count, if you didn't specify the platform and didn't set the 'exact_amount' parameter)
- exactAmount boolean - set it to 'true' if you'd like to receive the exact amount of icons, not multiplied by platforms count
- offset decimal - the offset from the first received result
- platform string - the style of the icons
- language string - the language code to get localized result
getTotals
function getTotals(string since) returns TotalsResponse|error
Totals
Parameters
- since string - the optional date to calculate the total number of icons that were created after it. It should be in format "four year digits - dash - two month number digits - dash - two day number digits. For example 2014-12-31 means "31th of December, 2014".
Return Type
- TotalsResponse|error - OK
postCollection
function postCollection(json payload) returns CreatedItem|error
From a Collection
Parameters
- payload json - Item to be added
Return Type
- CreatedItem|error - OK
postIcons
function postIcons(json payload) returns CreatedItem|error
From Separate Icons
Parameters
- payload json - Item to be added
Return Type
- CreatedItem|error - OK
Records
icons8: ApiKeysConfig
Provides API key configurations needed when communicating with a remote HTTP endpoint.
Fields
- token string - Represents API Key
token
icons8: Auth
Authorization
Fields
- hash string - Authorization hash
icons8: AuthArgument
Fields
- hash string - Authorization hash
icons8: Categories
Fields
- parameters CategoriesParameters? -
- result CategoriesResult? -
icons8: CategoriesParameters
Fields
- language string? -
- platform string? -
icons8: CategoriesResult
Fields
- categories anydata[]? -
icons8: Category
Fields
- parameters CategoryParameters? -
- result CategoryResult? -
icons8: CategoryParameters
Fields
- amount decimal? -
- category string? -
- language string? -
- offset string? -
- platform string? -
- subcategory string? -
icons8: CategoryResult
Fields
- category anydata[]? -
icons8: CategoryV3Item
Fields
- category_code string? -
- category_name string? -
- subcategory anydata[]? -
icons8: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
icons8: CollectionWebFontTaskArguments
Fields
- arguments CollectionwebfonttaskargumentsArguments? -
icons8: CollectionwebfonttaskargumentsArguments
Fields
- collection string - collection identifier
- css_prefix string? - prefix for CSS rules, used to generate LESS/SCSS
- css_rules_case string? - glyph names conversion rule
- css_selector string? - template for glyph selector; placeholder {{glyph}} will be replaced with glyph names
- font_name string - name of iconic webfont
icons8: CompactCategoryV3Item
Fields
- api_code string -
- name string -
icons8: CompactIconV3Item
Fields
- category string? -
- common_icon_id decimal - identifier for group of the same icons in different styles; i.e. id of all "home" icons
- created string - date (ISO8601 format) of icon was published
- features Compacticonv3itemFeatures - internal service attribute; internal information about icon features; private; depend on the rights of the current user
- id decimal - unique icon identifier
- name string - icon name
- platform_code string - icon style
- share Compacticonv3itemShare? - internal service attribute; some links to spread the world
- subcategory Compacticonv3itemSubcategory? -
- svg string - plain SVG content of icon
- url string? - internal service attribute; the meaning of this attribute is "Identifier of thread at Disquss"
- vector Compacticonv3itemVector? - URLs to icon sources in different vector formats; private; depend on the current user and could be opened in user's browser only
icons8: Compacticonv3itemFeatures
internal service attribute; internal information about icon features; private; depend on the rights of the current user
Fields
- bitmap decimal - "Could user use images in bitmap / raster formats in any size ?"
- nolink decimal - "Could user use images without any link to Icons8 ?"
- vector decimal - "Could user use images in vector formats ?"
icons8: Compacticonv3itemShare
internal service attribute; some links to spread the world
Fields
- png anydata[]? - some raster images for share the icon
- url string? - short URL to use in twitter
icons8: Compacticonv3itemSubcategory
Fields
- api_code string -
- name string -
icons8: Compacticonv3itemVector
URLs to icon sources in different vector formats; private; depend on the current user and could be opened in user's browser only
Fields
- eps string? - absolute URL to file in EPS format
- pdf string? - absolute URL to file in PDF format
- 'svg\-editable string? - absolute URL to original SVG file
- 'svg\-simplified string? - absolute URL to compressed and simplified SVG file
icons8: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
icons8: CreatedItem
Fields
- messages anydata[]? -
- result CreateditemResult? -
icons8: CreateditemResult
Fields
- description string? - human readable description
- id string - task identifier
- results CreateditemResultResults? -
- status string - task status
- 'type string - task type
icons8: CreateditemResultResults
Fields
- zip string? -
icons8: Icon
Fields
- parameters IconParameters? -
- result IconResult? -
icons8: IconParameters
Fields
- amount decimal? -
- language string? -
- offset string? -
- platform string? -
- term string? -
icons8: IconResult
Fields
- search anydata[]? -
icons8: IconV3Item
Fields
- Fields Included from *CompactIconV3Item
- category string
- common_icon_id decimal
- created string
- features Compacticonv3itemFeatures
- id decimal
- name string
- platform_code string
- share Compacticonv3itemShare
- subcategory Compacticonv3itemSubcategory
- svg string
- url string
- vector Compacticonv3itemVector
- anydata...
- category string? -
- common_icon_id decimal - identifier for group of the same icons in different styles; i.e. id of all "home" icons
- created string - date (ISO8601 format) of icon was published
- features Compacticonv3itemFeatures - internal service attribute; internal information about icon features; private; depend on the rights of the current user
- id decimal - unique icon identifier
- name string - icon name
- platform_code string - icon style
- share Compacticonv3itemShare? - internal service attribute; some links to spread the world
- subcategory Compacticonv3itemSubcategory? -
- svg string - plain SVG content of icon
- url string? - internal service attribute; the meaning of this attribute is "Identifier of thread at Disquss"
- vector Compacticonv3itemVector? - URLs to icon sources in different vector formats; private; depend on the current user and could be opened in user's browser only
icons8: LatestIcons
Fields
- parameters IconParameters? -
- result LatesticonsResult? -
icons8: LatesticonsResult
Fields
- latest anydata[]? -
icons8: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
icons8: Task
Task
Fields
- arguments TaskArguments? - Task arguments
icons8: TaskArguments
Task arguments
Fields
- collection string - collection identifier
- css_prefix string(default "icons8") - prefix for CSS rules, used to generate LESS/SCSS
- css_rules_case string(default "lowercase") - glyph names conversion rule
- css_selector string(default ".icons8-{{glyph}}") - template for glyph selector; placeholder {{glyph}} will be replaced with glyph names
- font_name string - name of iconic webfont
icons8: TaskError
Fields
- code string - error code
- description string - human readable error message
icons8: TaskResult
Fields
- description string? - human readable description
- id string - task identifier
- results TaskresultResults? -
- status string - task status
- 'type string - task type
icons8: TaskresultResults
Fields
- zip string? - URL to webfont files in ZIP format
icons8: TotalsResponse
Fields
- parameters TotalsresponseParameters? -
- result TotalsresponseResult? -
icons8: TotalsresponseParameters
Fields
- since string? -
icons8: TotalsresponseResult
Fields
- total anydata[]? -
icons8: TotalV3Item
Fields
- api_code string - icons style code; in other places it named
plarform
orplatform_api_code
- name string -
- total decimal -
Anydata types
icons8: Meta
Meta
icons8: Search
Search
icons8: WebFonts
WebFonts
Import
import ballerinax/icons8;
Metadata
Released date: about 2 years ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 5
Current verison: 5
Weekly downloads
Keywords
Content & Files/Images & Design
Cost/Freemium
Contributors
Dependencies