Note: There is a newer version (2.3.1) of this package available. Click here to view docs for the latest version.
Module hubspot.events
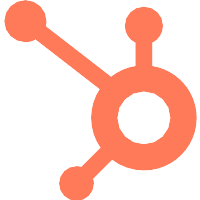
ballerinax/hubspot.events Ballerina library
2.3.0
Overview
This is a generated connector from HubSpot OpenAPI specification.
These APIs allow accessing CRM object events.
Prerequisites
- Create a HubSpot developer account
- Obtain tokens
- Use this guide to obtain the credentials which are needed to create the <CLIENT_ID> and <CLIENT_SECRET>
Quickstart
To use the HubSpot Events connector in your Ballerina application, update the .bal file as follows:
Step 1 - Import connector
First, import the ballerinax/hubspot.event module into the Ballerina project.
import ballerinax/hubspot.event;
Step 2 - Create a new connector instance
You can now make the connection configuration using the access token.
event:ClientConfig clientConfig = { auth : { token: <ACCESS_TOKEN> } }; event:Client baseClient = check new Client(clientConfig);
Step 3 - Invoke connector operation
- List Events
event:CollectionResponseExternalUnifiedEvent|error eventList = baseClient->listEvents("contact",<OBJECT_ID>); if (eventList is event:CollectionResponseExternalUnifiedEvent) { log:printInfo("Event List " + eventList.toString()); } else { log:printInfo(msg = eventList.message()); }
- Use
bal run
command to compile and run the Ballerina program