hubspot.crm.pipeline
Module hubspot.crm.pipeline
API
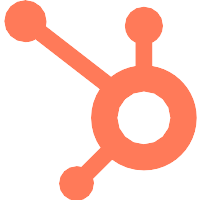
ballerinax/hubspot.crm.pipeline Ballerina library
Overview
This is a generated connector from HubSpot OpenAPI specification.
This API provides access to pipelines. Pipelines represent distinct stages in a workflow, like closing a deal or servicing a support ticket. These endpoints provide access to read and modify pipelines in HubSpot. They support deals
and tickets
object types.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a HubSpot developer account
- Obtain tokens
- Use this guide to obtain the credentials which are needed to create the <CLIENT_ID> and <CLIENT_SECRET>
Quickstart
To use the HubSpot CRM Pipelines connector in your Ballerina application, update the .bal file as follows:
Step 1 - Import connector
First, import the ballerinax/hubspot.crm.pipeline module into the Ballerina project.
import ballerinax/hubspot.crm.pipeline;
Step 2 - Create a new connector instance
You can now make the connection configuration using the access token.
pipeline:ClientConfig clientConfig = { auth : { token: <ACCESS_TOKEN> } }; pipeline:Client baseClient = check new Client(clientConfig);
Step 3 - Invoke connector operation
- Create a pipeline instance
pipeline:PipelineInput event = { stages: [ { label: "In progress", displayOrder: 0, metadata: { "ticketState": "OPEN", "probability": 0.5 } } ], label: "My replaced pipeline", displayOrder: 0 }; pipeline:PipelineInput|error bEvent = baseClient->create("deals", event); if (bEvent is pipeline:PipelineInput) { log:printInfo("Created the pipeline" + bEvent.toString()); } else { log:printError(msg = bEvent.toString()); }
- List pipelines
pipeline:CollectionResponsePipeline|error bEvent = baseClient->getAll("deals"); if (bEvent is pipeline:CollectionResponsePipeline) { log:printInfo("Pipeline list" + bEvent.toString()); } else { log:printError(msg = bEvent.message()); }
- Use
bal run
command to compile and run the Ballerina program
Clients
hubspot.crm.pipeline: Client
This is a generated connector from HubSpot OpenAPI specification.
Pipelines represent distinct stages in a workflow, like closing a deal or servicing a support ticket. These endpoints provide access to read and modify pipelines in HubSpot. Pipelines support deals
and tickets
object types.
When calling endpoints that take pipelineId as a parameter, that ID must correspond to an existing, un-archived pipeline. Otherwise the request will fail with a 404 Not Found
response.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a HubSpot account and obtain OAuth tokens following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.hubapi.com" - URL of the target service
getAll
function getAll(string objectType, boolean archived) returns CollectionResponsePipeline|error
Retrieve all pipelines
Parameters
- objectType string - CRM object type
- archived boolean (default false) - Whether to return only results that have been archived.
Return Type
- CollectionResponsePipeline|error - successful operation
create
function create(string objectType, PipelineInput payload) returns Pipeline|error
Create a pipeline
getById
Return a pipeline by ID
Parameters
- objectType string - CRM object type
- pipelineId string - Pipeline ID
- archived boolean (default false) - Whether to return only results that have been archived.
replace
function replace(string objectType, string pipelineId, PipelineInput payload) returns Pipeline|error
Replace a pipeline
Parameters
- objectType string - CRM object type
- pipelineId string - Pipeline ID
- payload PipelineInput - Pipeline input
archive
Archive a pipeline
update
function update(string objectType, string pipelineId, PipelinePatchInput payload, boolean archived) returns Pipeline|error
Update a pipeline
Parameters
- objectType string - CRM object type
- pipelineId string - Pipeline ID
- payload PipelinePatchInput - Attributes to update in pipeline
- archived boolean (default false) - Whether to return only results that have been archived.
stageGetall
function stageGetall(string objectType, string pipelineId, boolean archived) returns CollectionResponsePipelineStage|error
Return all stages of a pipeline
Parameters
- objectType string - CRM object type
- pipelineId string - Pipeline ID
- archived boolean (default false) - Whether to return only results that have been archived.
Return Type
- CollectionResponsePipelineStage|error - successful operation
stageCreate
function stageCreate(string objectType, string pipelineId, PipelineStageInput payload) returns PipelineStage|error
Create a pipeline stage
Parameters
- objectType string - CRM object type
- pipelineId string - Pipeline ID
- payload PipelineStageInput - Pipeline stage input
Return Type
- PipelineStage|error - successful operation
stageGetbyid
function stageGetbyid(string objectType, string pipelineId, string stageId, boolean archived) returns PipelineStage|error
Return a pipeline stage by ID
Parameters
- objectType string - CRM object type
- pipelineId string - Pipeline ID
- stageId string - Stage ID
- archived boolean (default false) - Whether to return only results that have been archived.
Return Type
- PipelineStage|error - successful operation
stageReplace
function stageReplace(string objectType, string pipelineId, string stageId, PipelineStageInput payload) returns PipelineStage|error
Replace a pipeline stage
Parameters
- objectType string - CRM object type
- pipelineId string - Pipeline ID
- stageId string - Stage ID
- payload PipelineStageInput - Pipeline stage input
Return Type
- PipelineStage|error - successful operation
stageArchive
Archive a pipeline stage
Parameters
- objectType string - CRM object type
- pipelineId string - Pipeline ID
- stageId string - Stage ID
stageUpdate
function stageUpdate(string objectType, string pipelineId, string stageId, PipelineStagePatchInput payload, boolean archived) returns PipelineStage|error
Update a pipeline stage
Parameters
- objectType string - CRM object type
- pipelineId string - Pipeline ID
- stageId string - Stage ID
- payload PipelineStagePatchInput - Pipeline stage patch input
- archived boolean (default false) - Whether to return only results that have been archived.
Return Type
- PipelineStage|error - successful operation
Records
hubspot.crm.pipeline: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
hubspot.crm.pipeline: CollectionResponsePipeline
Fields
- results Pipeline[] -
- paging Paging? -
hubspot.crm.pipeline: CollectionResponsePipelineStage
Fields
- results PipelineStage[] -
- paging Paging? -
hubspot.crm.pipeline: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
hubspot.crm.pipeline: Error
Fields
- message string - A human readable message describing the error along with remediation steps where appropriate
- correlationId string - A unique identifier for the request. Include this value with any error reports or support tickets
- category string - The error category
- subCategory string? - A specific category that contains more specific detail about the error
- errors ErrorDetail[]? - further information about the error
- context record {}? - Context about the error condition
- links record {}? - A map of link names to associated URIs containing documentation about the error or recommended remediation steps
hubspot.crm.pipeline: ErrorDetail
Fields
- message string - A human readable message describing the error along with remediation steps where appropriate
- 'in string? - The name of the field or parameter in which the error was found.
- code string? - The status code associated with the error detail
- subCategory string? - A specific category that contains more specific detail about the error
- context record {}? - Context about the error condition
hubspot.crm.pipeline: NextPage
Fields
- after string -
- link string? -
hubspot.crm.pipeline: OAuth2RefreshTokenGrantConfig
OAuth2 Refresh Token Grant Configs
Fields
- Fields Included from *OAuth2RefreshTokenGrantConfig
- refreshUrl string(default "https://api.hubapi.com/oauth/v1/token") - Refresh URL
hubspot.crm.pipeline: Paging
Fields
- next NextPage? -
hubspot.crm.pipeline: Pipeline
A pipeline definition.
Fields
- stages PipelineStage[] - The stages associated with the pipeline. They can be retrieved and updated via the pipeline stages endpoints.
- createdAt string - The date the pipeline was created. The default pipelines will have createdAt = 0.
- archivedAt string? - The date the pipeline was archived.
archivedAt
will only be present if the pipeline is archived.
- updatedAt string - The date the pipeline was last updated.
- archived boolean - Whether the pipeline is archived.
- label string - A unique label used to organize pipelines in HubSpot's UI
- displayOrder int - The order for displaying this pipeline. If two pipelines have a matching
displayOrder
, they will be sorted alphabetically by label.
- id string - A unique identifier generated by HubSpot that can be used to retrieve and update the pipeline.
hubspot.crm.pipeline: PipelineInput
An input used to create or replace a pipeline's definition.
Fields
- stages PipelineStageInput[] - Pipeline stage inputs used to create the new or replacement pipeline.
- label string - A unique label used to organize pipelines in HubSpot's UI
- displayOrder int - The order for displaying this pipeline. If two pipelines have a matching
displayOrder
, they will be sorted alphabetically by label.
hubspot.crm.pipeline: PipelinePatchInput
An input used to update some properties on a pipeline definition.
Fields
- label string? - A unique label used to organize pipelines in HubSpot's UI
- displayOrder int? - The order for displaying this pipeline. If two pipelines have a matching
displayOrder
, they will be sorted alphabetically by label.
- archived boolean? - Whether the pipeline is archived. This property should only be provided when restoring an archived pipeline. If it's provided in any other call, the request will fail and a
400 Bad Request
will be returned.
hubspot.crm.pipeline: PipelineStage
A pipeline stage definition.
Fields
- createdAt string - The date the pipeline stage was created. The stages on default pipelines will have createdAt = 0.
- archivedAt string? - The date the pipeline was archived.
archivedAt
will only be present if the pipeline is archived.
- updatedAt string - The date the pipeline stage was last updated.
- archived boolean - Whether the pipeline is archived.
- label string - A label used to organize pipeline stages in HubSpot's UI. Each pipeline stage's label must be unique within that pipeline.
- displayOrder int - The order for displaying this pipeline stage. If two pipeline stages have a matching
displayOrder
, they will be sorted alphabetically by label.
- metadata record {} - A JSON object containing properties that are not present on all object pipelines.
For
deals
pipelines, theprobability
field is required ({ "probability": 0.5 }
), and represents the likelihood a deal will close. Possible values are between 0.0 and 1.0 in increments of 0.1. Fortickets
pipelines, theticketState
field is optional ({ "ticketState": "OPEN" }
), and represents whether the ticket remains open or has been closed by a member of your Support team. Possible values areOPEN
orCLOSED
.
- id string - A unique identifier generated by HubSpot that can be used to retrieve and update the pipeline stage.
hubspot.crm.pipeline: PipelineStageInput
An input used to create or replace a pipeline stage's definition.
Fields
- label string - A label used to organize pipeline stages in HubSpot's UI. Each pipeline stage's label must be unique within that pipeline.
- displayOrder int - The order for displaying this pipeline stage. If two pipeline stages have a matching
displayOrder
, they will be sorted alphabetically by label.
- metadata record {} - A JSON object containing properties that are not present on all object pipelines.
For
deals
pipelines, theprobability
field is required ({ "probability": 0.5 }
), and represents the likelihood a deal will close. Possible values are between 0.0 and 1.0 in increments of 0.1. Fortickets
pipelines, theticketState
field is optional ({ "ticketState": "OPEN" }
), and represents whether the ticket remains open or has been closed by a member of your Support team. Possible values areOPEN
orCLOSED
.
hubspot.crm.pipeline: PipelineStagePatchInput
An input used to update some properties on a pipeline definition.
Fields
- label string? - A label used to organize pipeline stages in HubSpot's UI. Each pipeline stage's label must be unique within that pipeline.
- archived boolean? - Whether the pipeline is archived.
- displayOrder int? - The order for displaying this pipeline stage. If two pipeline stages have a matching
displayOrder
, they will be sorted alphabetically by label.
- metadata record {} - A JSON object containing properties that are not present on all object pipelines.
For
deals
pipelines, theprobability
field is required ({ "probability": 0.5 }
), and represents the likelihood a deal will close. Possible values are between 0.0 and 1.0 in increments of 0.1. Fortickets
pipelines, theticketState
field is optional ({ "ticketState": "OPEN" }
), and represents whether the ticket remains open or has been closed by a member of your Support team. Possible values areOPEN
orCLOSED
.
hubspot.crm.pipeline: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
Import
import ballerinax/hubspot.crm.pipeline;
Metadata
Released date: about 1 year ago
Version: 2.3.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 0
Current verison: 0
Weekly downloads
Keywords
Sales & CRM/Customer Relationship Management
Cost/Freemium
Contributors
Dependencies