hubspot.crm.contact
Module hubspot.crm.contact
API
Definitions
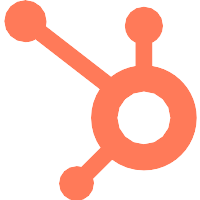
ballerinax/hubspot.crm.contact Ballerina library
Overview
This is a generated connector from HubSpot OpenAPI specification.
This API provides access to collections of CRM objects, which return a map of property names to values. Each object type has its own set of default properties, which can be found by exploring the CRM Object Properties API.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a HubSpot developer account
- Obtain tokens
- Use this guide to obtain the credentials which are needed to create the <CLIENT_ID> and <CLIENT_SECRET>
Quickstart
To use the HubSpot CRM Contacts connector in your Ballerina application, update the .bal file as follows:
Step 1 - Import connector
First, import the ballerinax/hubspot.crm.contact module into the Ballerina project.
import ballerinax/hubspot.crm.contact;
Step 2 - Create a new connector instance
You can now make the connection configuration using the access token.
contact:ClientConfig clientConfig = { auth : { token: <ACCESS_TOKEN> } }; contact:Client baseClient = check new Client(clientConfig);
Step 3 - Invoke connector operation
- Create a contact instance
contact:SimplePublicObjectInput contact = { properties : { "city": "Cambridge", "domain": "biglytics.net", "industry": "Technology", "name": "Biglytics", "phone": "(877) 929-0687", "state": "Massachusetts" } }; contact:SimplePublicObject|error bEvent = baseClient->create(contact); if (bEvent is contact:SimplePublicObject) { log:printInfo("Created the contact" + bEvent.toString()); } else { log:printError(msg = bEvent.message()); }
- List contacts
contact:SimplePublicObjectWithAssociationsArray|error bEvent = baseClient->getPage(); if (bEvent is contact:SimplePublicObjectWithAssociationsArray) { log:printInfo("Contact list" + bEvent.toString()); } else { log:printError(msg = bEvent.message()); }
- Use
bal run
command to compile and run the Ballerina program
Clients
hubspot.crm.contact: Client
This is a generated connector from HubSpot OpenAPI specification. This API provides access to collections of CRM objects, which return a map of property names to values. Each object type has its own set of default properties, which can be found by exploring the CRM Object Properties API.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials. Create a HubSpot account and obtain OAuth tokens following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.hubapi.com" - URL of the target service
getPage
function getPage(int 'limit, string? after, string[]? properties, string[]? associations, boolean archived) returns CollectionResponseSimplePublicObjectWithAssociationsForwardPaging|error
List
Parameters
- 'limit int (default 10) - The maximum number of results to display per page.
- after string? (default ()) - The paging cursor token of the last successfully read resource will be returned as the
paging.next.after
JSON property of a paged response containing more results.
- properties string[]? (default ()) - A comma separated list of the properties to be returned in the response. If any of the specified properties are not present on the requested object(s), they will be ignored.
- associations string[]? (default ()) - A comma separated list of object types to retrieve associated IDs for. If any of the specified associations do not exist, they will be ignored.
- archived boolean (default false) - Whether to return only results that have been archived.
Return Type
- CollectionResponseSimplePublicObjectWithAssociationsForwardPaging|error - successful operation
create
function create(SimplePublicObjectInput payload) returns SimplePublicObject|error
Create
Parameters
- payload SimplePublicObjectInput - Contact data
Return Type
- SimplePublicObject|error - successful operation
batchArchive
function batchArchive(SimplePublicObjectIdArray payload) returns Response|error
Archive a batch of contacts by ID
Parameters
- payload SimplePublicObjectIdArray - Record containing an array of contact IDs
batchCreate
function batchCreate(SimplePublicObjectInputArray payload) returns BatchResponseSimplePublicObject|BatchResponseSimplePublicObjectWithErrors|error
Create a batch of contacts
Parameters
- payload SimplePublicObjectInputArray - Record with an array of contact data
Return Type
- BatchResponseSimplePublicObject|BatchResponseSimplePublicObjectWithErrors|error - successful operation
batchRead
function batchRead(SimplePublicObjectIdReadArray payload, boolean archived) returns BatchResponseSimplePublicObject|BatchResponseSimplePublicObjectWithErrors|error
Read a batch of contacts by internal ID, or unique property values
Parameters
- payload SimplePublicObjectIdReadArray - Object which contains array of internal IDs of contacts
- archived boolean (default false) - Whether to return only results that have been archived.
Return Type
- BatchResponseSimplePublicObject|BatchResponseSimplePublicObjectWithErrors|error - successful operation
batchUpdate
function batchUpdate(SimplePublicObjectArray payload) returns BatchResponseSimplePublicObject|BatchResponseSimplePublicObjectWithErrors|error
Update a batch of contacts
Parameters
- payload SimplePublicObjectArray - Record with array of updated contact data
Return Type
- BatchResponseSimplePublicObject|BatchResponseSimplePublicObjectWithErrors|error - successful operation
search
function search(PublicObjectSearchRequest payload) returns CollectionResponseWithTotalSimplePublicObjectForwardPaging|error
Filter, Sort, and Search CRM Objects
Parameters
- payload PublicObjectSearchRequest - Contact search request
Return Type
- CollectionResponseWithTotalSimplePublicObjectForwardPaging|error - successful operation
getObjectById
function getObjectById(string contactId, string[]? properties, string[]? associations, boolean archived, string? idProperty) returns SimplePublicObjectWithAssociations|error
Read
Parameters
- contactId string - Contact ID
- properties string[]? (default ()) - A comma separated list of the properties to be returned in the response. If any of the specified properties are not present on the requested object(s), they will be ignored.
- associations string[]? (default ()) - A comma separated list of object types to retrieve associated IDs for. If any of the specified associations do not exist, they will be ignored.
- archived boolean (default false) - Whether to return only results that have been archived.
- idProperty string? (default ()) - The name of a property whose values are unique for this object type
Return Type
- SimplePublicObjectWithAssociations|error - successful operation
archive
Archive
Parameters
- contactId string - Contact ID
update
function update(string contactId, SimplePublicObjectInput payload, string? idProperty) returns SimplePublicObject|error
Update
Parameters
- contactId string - Contact ID
- payload SimplePublicObjectInput - Attributes to update in contact
- idProperty string? (default ()) - The name of a property whose values are unique for this object type
Return Type
- SimplePublicObject|error - successful operation
associationsGetAll
function associationsGetAll(string contactId, string toObjectType, string? after, int 'limit) returns CollectionResponseAssociatedIdForwardPaging|error
List associations of a contact by type
Parameters
- contactId string - Contact ID
- toObjectType string - Object type to associate with
- after string? (default ()) - The paging cursor token of the last successfully read resource will be returned as the
paging.next.after
JSON property of a paged response containing more results.
- 'limit int (default 500) - The maximum number of results to display per page.
Return Type
- CollectionResponseAssociatedIdForwardPaging|error - successful operation
associationsCreate
function associationsCreate(string contactId, string toObjectType, string toObjectId, string associationType) returns SimplePublicObjectWithAssociations|error
Associate a contact with another object
Parameters
- contactId string - Contact ID
- toObjectType string - Object type to associate with
- toObjectId string - Object ID to associate
- associationType string - Type of the association
Return Type
- SimplePublicObjectWithAssociations|error - successful operation
associationsArchive
function associationsArchive(string contactId, string toObjectType, string toObjectId, string associationType) returns Response|error
Remove an association between two contacts
Records
hubspot.crm.contact: AssociatedId
Fields
- id string -
- 'type string -
hubspot.crm.contact: BatchResponseSimplePublicObject
Fields
- status string -
- results SimplePublicObject[] -
- requestedAt string? -
- startedAt string -
- completedAt string -
- links record {}? -
hubspot.crm.contact: BatchResponseSimplePublicObjectWithErrors
Fields
- status string -
- results SimplePublicObject[] -
- numErrors int? -
- errors StandardError[]? -
- requestedAt string? -
- startedAt string -
- completedAt string -
- links record {}? -
hubspot.crm.contact: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
hubspot.crm.contact: CollectionResponseAssociatedId
Fields
- results AssociatedId[] -
- paging Paging? -
hubspot.crm.contact: CollectionResponseAssociatedIdForwardPaging
Fields
- results AssociatedId[] -
- paging ForwardPaging? -
hubspot.crm.contact: CollectionResponseSimplePublicObjectWithAssociationsForwardPaging
Fields
- results SimplePublicObjectWithAssociations[] -
- paging ForwardPaging? -
hubspot.crm.contact: CollectionResponseWithTotalSimplePublicObjectForwardPaging
Fields
- total int -
- results SimplePublicObject[] -
- paging ForwardPaging? -
hubspot.crm.contact: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
hubspot.crm.contact: Error
Fields
- message string - A human readable message describing the error along with remediation steps where appropriate
- correlationId string - A unique identifier for the request. Include this value with any error reports or support tickets
- category string - The error category
- subCategory string? - A specific category that contains more specific detail about the error
- errors ErrorDetail[]? - further information about the error
- context record {}? - Context about the error condition
- links record {}? - A map of link names to associated URIs containing documentation about the error or recommended remediation steps
hubspot.crm.contact: ErrorCategory
Fields
- httpStatus string -
- name string -
hubspot.crm.contact: ErrorDetail
Fields
- message string - A human readable message describing the error along with remediation steps where appropriate
- 'in string? - The name of the field or parameter in which the error was found.
- code string? - The status code associated with the error detail
- subCategory string? - A specific category that contains more specific detail about the error
- context record {}? - Context about the error condition
hubspot.crm.contact: Filter
Fields
- value string? -
- propertyName string -
- operator string -
hubspot.crm.contact: FilterGroup
Fields
- filters Filter[] -
hubspot.crm.contact: ForwardPaging
Fields
- next NextPage? -
hubspot.crm.contact: NextPage
Fields
- after string -
- link string? -
hubspot.crm.contact: OAuth2RefreshTokenGrantConfig
OAuth2 Refresh Token Grant Configs
Fields
- Fields Included from *OAuth2RefreshTokenGrantConfig
- refreshUrl string(default "https://api.hubapi.com/oauth/v1/token") - Refresh URL
hubspot.crm.contact: Paging
Fields
- next NextPage? -
- prev PreviousPage? -
hubspot.crm.contact: PreviousPage
Fields
- before string -
- link string? -
hubspot.crm.contact: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
hubspot.crm.contact: PublicObjectSearchRequest
Fields
- filterGroups FilterGroup[] -
- sorts string[] -
- query string? -
- properties string[] -
- 'limit int -
- after int -
hubspot.crm.contact: SimplePublicObject
Fields
- id string -
- properties record {} -
- createdAt string -
- updatedAt string -
- archived boolean? -
- archivedAt string? -
hubspot.crm.contact: SimplePublicObjectArray
Fields
- inputs SimplePublicObjectBatchInput[] -
hubspot.crm.contact: SimplePublicObjectBatchInput
Fields
- properties record {} -
- id string -
hubspot.crm.contact: SimplePublicObjectId
Fields
- id string -
hubspot.crm.contact: SimplePublicObjectIdArray
Fields
- inputs SimplePublicObjectId[] -
hubspot.crm.contact: SimplePublicObjectIdReadArray
Fields
- properties string[] -
- idProperty string? -
- inputs SimplePublicObjectId[] -
hubspot.crm.contact: SimplePublicObjectInput
Fields
- properties record {} -
hubspot.crm.contact: SimplePublicObjectInputArray
Fields
- inputs SimplePublicObjectInput[] -
hubspot.crm.contact: SimplePublicObjectWithAssociations
Fields
- id string -
- properties record {} -
- createdAt string -
- updatedAt string -
- archived boolean? -
- archivedAt string? -
- associations record {}? -
hubspot.crm.contact: StandardError
Fields
- status string -
- id string? -
- category ErrorCategory -
- subCategory record {}? -
- message string -
- errors ErrorDetail[] -
- context record {} -
- links record {} -
Import
import ballerinax/hubspot.crm.contact;
Metadata
Released date: almost 2 years ago
Version: 2.3.0
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 2862
Current verison: 24
Weekly downloads
Keywords
Sales & CRM/Contact Management
Cost/Freemium
Contributors
Dependencies