googleapis.people
Module googleapis.people
API
Definitions
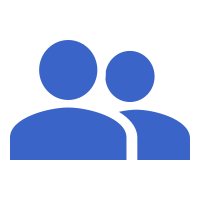
ballerinax/googleapis.people Ballerina library
Overview
Ballerina connector for Google People API connects the Google People API via Ballerina language with ease. It provides capability to perform operations related to contacts and contact groups in Google Contacts.
This module supports Google People API v1.0.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Google account
- Obtain tokens - Follow this link
Quickstart
To use the Google People API connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
Import the ballerinax/googleapis.people module into the Ballerina project.
import ballerinax/googleapis.people as people;
Step 2: Create a new connector instance
Enter the credentials in the Google People API client configuration, and create the Google People API client by passing the configuration.
people:ConnectionConfig googleContactConfig = { auth: { clientId: "<CLIENT_ID>", clientSecret: <CLIENT_SECRET>, refreshUrl: people:REFRESH_URL, refreshToken: <REFRESH_TOKEN> } }; people:Client googleContactClient = check new (googleContactConfig);
Step 3: Invoke connector operation
-
Create a contact via the
createContact
method by passing aPerson
record andFieldMask[]
as parameters.people:Person person = { emailAddresses: [], names: [{ familyName: "Hardy", givenName: "Jason", unstructuredName: "Jason Hardy" }] }; people:FieldMask[] personFields = [people:NAME, people:PHONE_NUMBER, people:EMAIL_ADDRESS]; people:PersonResponse createContact = check googleContactClient->createContact(person, personFields);
-
Use
bal run
command to compile and run the Ballerina program.
Clients
googleapis.people: Client
Ballerina Google People API connector provides the capability to access Google People API. This connector lets you to read and manage the authenticated user's contacts and contact groups.
Constructor
Initializes the connector. During initialization you can pass either http:BearerTokenConfig if you have a bearer token or http:OAuth2RefreshTokenGrantConfig if you have Oauth tokens. Create a Google account and obtain tokens following this guide. Configure the OAuth2 tokens to have the required permissions.
init (ConnectionConfig googleContactConfig)
- googleContactConfig ConnectionConfig - Configuration for the connector
listOtherContacts
function listOtherContacts(OtherContactFieldMask[] readMasks, ContactListOptions? options) returns stream<PersonResponse>|error
Fetch all from "Other Contacts".
Parameters
- readMasks OtherContactFieldMask[] - Restrict which fields on the person are returned
- options ContactListOptions? (default ()) - Record that contains options
Return Type
- stream<PersonResponse>|error - Stream of
PersonResponse
will have information specified byRead Masks
on success else anerror
createContact
function createContact(Person person, FieldMask[] personFields) returns PersonResponse|error
Create a contact.
Parameters
- person Person - Record of type of
CreatePerson
- personFields FieldMask[] - Restrict which fields on the person are returned
Return Type
- PersonResponse|error -
PersonResponse
will have information specified byPerson Fields
on success else anerror
getContact
function getContact(string resourceName, FieldMask[] personFields) returns PersonResponse|error
Fetch a contact.
Parameters
- resourceName string - Contact resource name
- personFields FieldMask[] - Restrict which fields on the person are returned
Return Type
- PersonResponse|error -
PersonResponse
will have information specified byPerson Fields
on success else anerror
searchContacts
function searchContacts(string query, FieldMask[] readMasks) returns PersonResponse[]|error
Search a contacts.
Parameters
- query string - String to be searched
- readMasks FieldMask[] - Restrict which fields on the person are returned
Return Type
- PersonResponse[]|error -
PersonResponse[]
will have information specified byPerson Fields
on success else anerror
updateContactPhoto
Update contact photo for a contact.
Parameters
- resourceName string - Contact resource name
- imagePath string - Path to image from root directory
Return Type
- error? - Nil on success, else an 'error'
deleteContactPhoto
Delete a contact photo.
Parameters
- resourceName string - Contact resource name
Return Type
- error? - Nil on success, else an 'error'
getBatchContacts
function getBatchContacts(string[] resourceNames, FieldMask[] personFields) returns PersonResponse[]|error
Get Batch contacts.
Parameters
- resourceNames string[] - String array of contact resource names
- personFields FieldMask[] - Restrict which fields on the person are returned
Return Type
- PersonResponse[]|error -
PersonResponse[]
will have information specified byPerson Fields
on success, else anerror
updateContact
function updateContact(string resourceName, Person person, FieldMask[] updatePersonFields, FieldMask[]? personFields) returns PersonResponse|error
Update a contact.
Parameters
- resourceName string - Contact resource name
- person Person - Person/Contact details
- updatePersonFields FieldMask[] - Restrict which fields on the person are returned
- personFields FieldMask[]? (default ()) - Restrict which fields on the person are returned
Return Type
- PersonResponse|error -
PersonResponse
will have information specified byPerson Fields
on success else anerror
deleteContact
Delete a Contact.
Parameters
- resourceName string - Contact resource name
Return Type
- error? - Nil on success, else an
error
listContacts
function listContacts(FieldMask[] personFields, ContactListOptions? options) returns stream<PersonResponse>|error
Get Peoples
Parameters
- personFields FieldMask[] - Restrict which fields on the person are returned
- options ContactListOptions? (default ()) - Record that contains options
Return Type
- stream<PersonResponse>|error -
stream<PersonResponse>
will have information specified byPerson Fields
on success or else anerror
createContactGroup
function createContactGroup(string contactGroupName) returns ContactGroup|error
Create a ContactGroup
.
Parameters
- contactGroupName string - Name of the
ContactGroup
to be created
Return Type
- ContactGroup|error -
ContactGroup
on success else anerror
getBatchContactGroup
function getBatchContactGroup(string[] resourceNames) returns ContactGroup[]|error
Get Batch contact groups.
Parameters
- resourceNames string[] - An array of strings with names of
Contact Groups
Return Type
- ContactGroup[]|error -
ContactGroup[]
on success else anerror
listContactGroup
function listContactGroup() returns ContactGroup[]|error
Fetch ContactGroups
of authenticated user.
Return Type
- ContactGroup[]|error -
ContactGroup[]
on success else anerror
getContactGroup
function getContactGroup(string resourceName, int maxMembers) returns ContactGroup|error
Fetch a ContactGroup
.
Parameters
- resourceName string - Name of the
ContactGroup
to be created
- maxMembers int - maximum number of members returned in contact group
Return Type
- ContactGroup|error -
ContactGroup
on success else anerror
updateContactGroup
function updateContactGroup(string resourceName, string updateName) returns ContactGroup|error
Update a ContactGroup
.
Parameters
- resourceName string - Name of the
ContactGroup
to be created
- updateName string - Name to be updated
Return Type
- ContactGroup|error -
ContactGroup
on success else anerror
deleteContactGroup
Delete a Contact Group.
Parameters
- resourceName string - Contact Group resource name
Return Type
- error? - Nil on success, else an
error
modifyContactGroup
function modifyContactGroup(string contactGroupResourceName, string[]? resourceNameToAdd, string[]? resourceNameToRemove) returns error?
Modify a contacts in Contact Group.
Parameters
- contactGroupResourceName string - Contact Group resource name
- resourceNameToAdd string[]? (default ()) - Contact resource name to add
- resourceNameToRemove string[]? (default ()) - Contact resource name to remove
Return Type
- error? - Nil on success, else an
error
Constants
googleapis.people: REFRESH_URL
Holds the value for URL of refresh token end point
Enums
googleapis.people: AgeRange
Define an age range.
Members
googleapis.people: ContentType
Define biography type of content.
Members
googleapis.people: FieldMask
Define a Fields returned when specified.
Members
googleapis.people: GroupType
Define an contact group type.
Members
googleapis.people: OtherContactFieldMask
Define a OtherContacts Fields returned when specified.
Members
Records
googleapis.people: Address
Define a Address.
Fields
- metadata FieldMetaData? - Metadata about Address
- formattedValue string? - Unstructured value of address
- 'type string? -
- formattedType string? - Type of address formatted
- poBox string? - Post box Number of address
- streetAddress string? - Street address
- extendedAddress string? - Extended address of address
- city string? - City of address
- region string? - Region of address
- postalCode string? - Postal code of address
- country string? - Country of address
- countryCode string? - Country code of address
googleapis.people: AgeRangeType
Define an Age Range.
Fields
- metadata FieldMetaData? - Metadata about Age Range
- ageRange AgeRange? - Age range
googleapis.people: BatchGetResponse
Define a BatchGetResponse.
Fields
- responses json[] - Array of PersonResponse
googleapis.people: Biography
Define an Biography.
Fields
- metadata FieldMetaData? - Metadata about Biography
- value string? - Age range value
- contentType ContentType? - Age range
googleapis.people: Birthday
Define an Birthday.
Fields
- metadata FieldMetaData? - Metadata about Birthday
- text string? - String representing user's birthday
- date Date? - Date of BirthDay
googleapis.people: BraggingRights
Define an BraggingRights.
Fields
- metadata FieldMetaData? - Metadata about BraggingRights
- value string? - Age range
googleapis.people: CalendarUrl
Define a Calendar Url.
Fields
- metadata FieldMetaData? - Metadata about Calendar Url
- url string? - Calendar URL
- 'type string? -
- formattedType string? - Type of calendar URL formatted
googleapis.people: ClientData
Define an Arbitrary client data that is populated by clients.
Fields
- metadata FieldMetaData? - Metadata about client data
- key string? - Client specified key of client data
- value string? - Client specified value of client data
googleapis.people: ConnectionConfig
Object for Google People API configuration.
Fields
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig - Configurations related to client authentication
- httpVersion string(default "1.1") - The HTTP version understood by the client
- http1Settings ClientHttp1Settings(default {}) - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings(default {}) - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- followRedirects FollowRedirects?(default ()) - Configurations associated with Redirection
- poolConfig PoolConfiguration?(default ()) - Configurations associated with request pooling
- cache CacheConfig(default {}) - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig?(default ()) - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig?(default ()) - Configurations associated with retrying
- cookieConfig CookieConfig?(default ()) - Configurations associated with cookies
- responseLimits ResponseLimitConfigs(default {}) - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket?(default ()) - SSL/TLS-related options
googleapis.people: ConnectionsResponse
Define a people connection response.
Fields
- connections PersonResponse[] - Array of PersonResponse of authenticated user
- nextPageToken string? - Next page token
- nextSyncToken string? - Next sync token
- totalPeople int? - Total contacts
- totalItems int? - Total pages
googleapis.people: ConnectionsStreamResponse
Define a Stream response of a Connection/Person.
Fields
- nextSyncToken string? - Next sync token
- totalPeople int? - Total contacts
- totalItems int? - Total pages
googleapis.people: ContactGroup
Define an ContactGroup.
Fields
- resourceName string - Resource name
- etag string? - Entity tag of resource
- metadata ContactGroupMetadata? - Metadata about contact group
- groupType GroupType? - Contact group type
- name string? - Contact group name set by group owner or a system
- formattedName string? - Name in formatted
- memberResourceNames string[]? - List of contact person names that are members of contact group
- memberCount int? - Total number of contacts
- clientData GroupClientData[]? - Contact group's client data
googleapis.people: ContactGroupBatch
Define a contact group batch result.
Fields
- responses json[] - Array of contact group responses
googleapis.people: ContactGroupList
Define a Contact Group List.
Fields
- contactGroups ContactGroup[] - Array of Contact Group
- totalItems int - Total contacts
- nextSyncToken string - Next sync token
googleapis.people: ContactGroupListResponse
Define a OtherContact List Response.
Fields
- contactGroups ContactGroup[] - Array of contact groups
- totalItems string? - Total contact groups
- nextPageToken string? - Next page token
- nextSyncToken string? - Next sync token
googleapis.people: ContactGroupMetadata
Define an ContactGroupMetadata.
Fields
- updateTime string? - Time of update
- deleted boolean? - Whether deleted is true
googleapis.people: ContactGroupResponse
Define an ContactGroupResponse.
Fields
- requestedResourceName string - Resource requested
- status json - Status of resource
- contactGroup json - Detail of contactGroup
googleapis.people: ContactListOptions
Define a Stream response of a Connection/Person.
Fields
- pageToken string?(default ()) - Page token
- requestSyncToken boolean?(default ()) - Whether request is needed
- syncToken string?(default ()) - Sync token
googleapis.people: ContactsTriggerResponse
Define a people connection trigger response.
Fields
- nextPageToken string? - Next page token
- nextSyncToken string - Next sync token
- totalPeople int? - Total contacts
- totalItems int? - Total pages
googleapis.people: CoverPhoto
Define an Cover photo.
Fields
- metadata FieldMetaData? - Metadata about cover photo
- url string? - URL of cover photo
- 'default boolean? -
googleapis.people: Date
Define a Date entity.
Fields
- year int? - Year of date
- month int? - Month of date
- day int? - Day of date
googleapis.people: EmailAddress
Define an Email Address.
Fields
- metadata FieldMetaData? - Metadata about Email Address
- value string? - Value of email address
- 'type string? -
- formattedType string? - Type of email address formatted
- displayName string? - Display name of email
googleapis.people: Event
Define an Event.
Fields
- metadata FieldMetaData? - Metadata about Event
- date Date? - Date of event
- 'type string? -
- formattedType string? - Type of event formatted
googleapis.people: ExternalId
Define an External entity.
Fields
- metadata FieldMetaData? - Metadata about External entity
- value string? - Value of external ID
- 'type string? -
- formattedType string? - Type of External entity formatted
googleapis.people: FieldMetaData
Define an FieldMetaData.
Fields
- primary boolean? - True if field is primary field
- verified boolean? - True if field is verified
- 'sources MetaDataSource? -
googleapis.people: FileAs
Define a name that should be used to sort person in a list.
Fields
- metadata FieldMetaData? - Metadata about file-as
- value string? - Value of file-as
googleapis.people: Gender
Define an Gender.
Fields
- metadata FieldMetaData? - Metadata about Gender
- value string? - Gender for person
- formattedValue string? - Type of Gender formatted
- addressMeAs string? - Type that should be used to address person
googleapis.people: GroupClientData
Define a Contact Group Client Data.
Fields
- key string? - Client specified key of client data.
- value string? - Client specified value of client data
googleapis.people: ImClient
Define an Instant Messaging client.
Fields
- metadata FieldMetaData? - Metadata about Instant Messaging client
- username string? - Username used in Instant Messaging client
- 'type string? -
- formattedType string? - Type that should be used to address person
- protocol string? - Protocol of Instant Messaging client
- formattedProtocol string? - Protocol of Instant Messaging client formatted
googleapis.people: Interest
Define an Interest.
Fields
- metadata FieldMetaData? - Metadata about Interest
- value string? - Name of Interest
googleapis.people: Locale
Define an Locale.
Fields
- metadata FieldMetaData? - Metadata about locale
- value string? - IETF BCP 47 language tag representing locale
googleapis.people: Location
Define an Location.
Fields
- metadata FieldMetaData? - Metadata about Location
- value string? - Value of location
- 'type string? -
- current string? - Whether location is current location
- buildingId string? - Building identifier
- floor string? - Floor name identifier
- floorSection string? - Floor section in a floor
- deskCode string? - Desk location.
googleapis.people: Membership
Define a Membership.
Fields
- metadata FieldMetaData? - Metadata about Membership
- contactGroupMembership json? - Group membership
- domainMembership string? - Domain membership
googleapis.people: MetaDataSource
Define an MetaDataSource.
Fields
- 'type string? -
- id string? - Identifier within source
googleapis.people: MiscKeyword
Define a miscellaneous keyword.
Fields
- metadata FieldMetaData? - Metadata about miscellaneous keyword
- value string? - Value of miscellaneous keyword
- 'type string? -
- formattedType string? - Type of miscellaneous keyword formatted
googleapis.people: Name
Define a Name.
Fields
- metadata FieldMetaData? - Metadata about Name
- displayName string? - Display name formatted
- displayNameLastFirst string? - Display name with last name
- unstructuredName string? - Free form name value
- familyName string? - Family name
- givenName string? - Given name
- middleName string? - Middle name
- honorificPrefix string? - Honorific prefixes, such as Mrs. or Dr
- honorificSuffix string? - Honorific suffixes, such as Jr.
- phoneticFullName string? - Full name spelled as it sounds
- phoneticFamilyName string? - Family name spelled as it sounds
- phoneticGivenName string? - Given name spelled as it sounds
- phoneticMiddleName string? - Middle name spelled as it sounds
- phoneticHonorificPrefix string? - Honorific prefixes spelled as they sound
- phoneticHonorificSuffix string? - Honorific suffixes spelled as they sound
googleapis.people: Nickname
Define an Nickname.
Fields
- metadata FieldMetaData? - Metadata about Nickname
- value string? - Value of nick name
- 'type string? -
googleapis.people: Occupation
Define an Occupation.
Fields
- metadata FieldMetaData? - Metadata about Occupation
- value string? - Value of occupation
googleapis.people: Organization
Define an Organization.
Fields
- metadata FieldMetaData? - Metadata about organization
- 'type string? -
- formattedType string? - Type organization formatted
- startDate Date? - Start date in organization
- endDate Date? - End date in organization
- current string? - Whether it is current organization
- name string? - Name of organization
- phoneticName string? - Phonetic name of organization
- department string? - Department at organization
- title string? - Job title at organization
- jobDescription string? - Job description in organization
- symbol string? - Symbol of organization.
- domain string? - Domain name of organization
- location string? - Location of organization
googleapis.people: OtherContactList
Define a OtherContactList.
Fields
- otherContacts PersonResponse[] - Other contacts of type Person
googleapis.people: OtherContactListResponse
Define a OtherContact List Response.
Fields
- otherContacts PersonResponse[] - Array of Person of in Other contacts
- nextPageToken string? - Next page token
- nextSyncToken string? - Next sync token
googleapis.people: Person
Define a Create Person Payload.
Fields
- addresses Address[]? - Person's addresses
- biographies Biography[]? - Person's biographies
- birthdays Birthday[]? - Person's birthdays
- braggingRights BraggingRights[]? - Person's bragging rights
- calendarUrls CalendarUrl[]? - Person's calendar URLs
- clientData ClientData[]? - Person's client data
- emailAddresses EmailAddress[]? - Person's email addresses
- events Event[]? - Person's events
- externalIds ExternalId[]? - Person's external IDs
- fileAses FileAs[]? - Person's file-ases
- genders Gender[]? - Person's genders
- imClients ImClient[]? - Person's instant messaging clients
- interests Interest[]? - Person's interests
- locales Locale[]? - Person's locale preferences
- locations Location[]? - Person's locations
- memberships Membership[]? - Person's group memberships
- miscKeywords MiscKeyword[]? - Person's miscellaneous keywords
- names Name[]? - Person's names
- nicknames Nickname[]? - Person's nicknames
- occupations Occupation[]? - Person's occupations
- organizations Organization[]? - Person's organizations
- phoneNumbers PhoneNumber[]? - Person's phoneNumbers
- relations Relation[]? - Person's relations
- sipAddresses SipAddress[]? - Person's sipAddresses
- skills Skill[]? - Person's skills
- urls Url[]? - Person's URLs
- userDefined UserDefined[]? - user defined data
googleapis.people: PersonMetadata
Define a Person's meta data.
Fields
- 'sources json[]? -
- previousResourceNames string[]? - Previous resource name if changed
- linkedPeopleResourceNames string[]? - Resource names of linked contacts
- deleted boolean? - Show status whether deleted
- objectType string? - Type of person object
googleapis.people: PersonResponse
Define a Person.
Fields
- resourceName string - String of resource name
- etag string? - ETag of resource
- metadata PersonMetadata? - Metadata about person
- ageRanges AgeRangeType[]? - Person's age ranges
- coverPhotos CoverPhoto[]? - Person's cover photos
- photos Photo[]? - Person's photos
- Fields Included from *Person
- addresses Address[]
- biographies Biography[]
- birthdays Birthday[]
- braggingRights BraggingRights[]
- calendarUrls CalendarUrl[]
- clientData ClientData[]
- emailAddresses EmailAddress[]
- events Event[]
- externalIds ExternalId[]
- fileAses FileAs[]
- genders Gender[]
- imClients ImClient[]
- interests Interest[]
- locales Locale[]
- locations Location[]
- memberships Membership[]
- miscKeywords MiscKeyword[]
- names Name[]
- nicknames Nickname[]
- occupations Occupation[]
- organizations Organization[]
- phoneNumbers PhoneNumber[]
- relations Relation[]
- sipAddresses SipAddress[]
- skills Skill[]
- urls Url[]
- userDefined UserDefined[]
- anydata...
googleapis.people: PhoneNumber
Define a PhoneNumber.
Fields
- metadata FieldMetaData? - Metadata about PhoneNumber
- value string? - Phone number
- canonicalForm string? - Canonicalized ITU-T E.164 form of phone number
- 'type string? -
- formattedType string? - Phone number formatted
googleapis.people: Photo
Define a Photo.
Fields
- metadata FieldMetaData? - Metadata about Photo
- url string? - URL to Photo
- 'default boolean? -
googleapis.people: Relation
Define a Relation.
Fields
- metadata FieldMetaData? - Metadata about relation
- person string? - Name of relation
- 'type string? -
- formattedType string? - Type of relation formatted
googleapis.people: SearchResponse
Define a SearchResponse.
Fields
- results json[] - Array of results
googleapis.people: SearchResult
Define a SearchResult.
Fields
- person PersonResponse - Type of PersonResponse
googleapis.people: SipAddress
Define a SIP address.
Fields
- metadata FieldMetaData? - Metadata about SIP address
- value string? - Name of SIP address
- 'type string? -
- formattedType string? - Type of SIP address formatted
googleapis.people: Skill
Define a Skill.
Fields
- metadata FieldMetaData? - Metadata about Skill
- value string? - Name of skill
googleapis.people: SyncConnectionsResponse
Define a sync people connection response.
Fields
- connections PersonResponse[]? - Array of person of authenticated user
- nextPageToken string? - Next page token
- nextSyncToken string - Next sync token
- totalPeople int? - Total contacts
- totalItems int? - Total pages
googleapis.people: Url
Define an Url.
Fields
- metadata FieldMetaData? - Metadata about Url
- value string? - Name of url
- 'type string? -
- formattedType string? - Type of url formatted
googleapis.people: UserDefined
Define a Arbitrary user data.
Fields
- metadata FieldMetaData? - Metadata about user defined data
- key string? - User specified key of user defined data
- value string? - User specified value of user defined data
Import
import ballerinax/googleapis.people;
Metadata
Released date: over 3 years ago
Version: 2.2.0
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.0.0
GraalVM compatible: Yes
Pull count
Total: 2150
Current verison: 1513
Weekly downloads
Keywords
Sales & CRM/Contact Management
Cost/Free
Vendor/Google
Contributors