googleapis.mybusiness
Module googleapis.mybusiness
API
Definitions
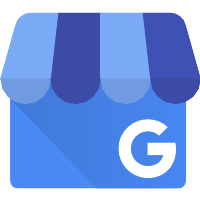
ballerinax/googleapis.mybusiness Ballerina library
Overview
This is a generated connector for Google My Business API v4.9 OpenAPI specification. The Google My Business API provides an interface for managing business location information on Google.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Google account
- Obtain tokens - Follow this link
Quickstart
To use the Google My Business connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/googleapis.mybusiness
module into the Ballerina project.
import ballerinax/googleapis.mybusiness;
Step 2: Create a new connector instance
Create a mybusiness:ClientConfig
with the OAuth2 tokens obtained, and initialize the connector with it.
mybusiness:ClientConfig clientConfig = { auth: { clientId: <CLIENT_ID>, clientSecret: <CLIENT_SECRET>, refreshUrl: <REFRESH_URL>, refreshToken: <REFRESH_TOKEN> } }; mybusiness:Client baseClient = check new Client(clientConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to return the paginated list of reviews for the specified location using the connector. This operation is only valid if the specified location is verified.
Returns the paginated list of reviews for the specified location.
public function main() { mybusiness:ListReviewsResponse|error response = baseClient->listAccountsLocationsReviews("<The name of the location to fetch reviews for>"); if (response is mybusiness:ListReviewsResponse) { log:printInfo(response.toString()); } else { log:printError(response.message()); } }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
googleapis.mybusiness: Client
This is a generated connector for Google My Business API v4.9 OpenAPI specification. The Google My Business API provides an interface for managing business location information on Google.
Constructor
Gets invoked to initialize the connector
.
The connector initialization doesn't require setting the API credentials.
Create a Google account and obtain tokens by following this guide.
Some operations may require passing the token as a parameter.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig {} - The configurations to be used when initializing the
connector
- serviceUrl string "https://mybusiness.googleapis.com/" - URL of the target service
listAccounts
function listAccounts(string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType, string? filter, string? name, int? pageSize, string? pageToken) returns ListAccountsResponse|error
Lists all of the accounts for the authenticated user. This includes all accounts that the user owns, as well as any accounts for which the user has management rights.
Parameters
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- filter string? (default ()) - A filter constraining the accounts to return. The response includes only entries that match the filter. If
filter
is empty, then no constraints are applied and all accounts (paginated) are retrieved for the requested account. For example, a request with the filtertype=USER_GROUP
will only return user groups.
- name string? (default ()) - The resource name of the account for which the list of directly accessible accounts is to be retrieved. This only makes sense for Organizations and User Groups. If empty, will return
ListAccounts
for the authenticated user.
- pageSize int? (default ()) - How many accounts to fetch per page. Default is 20, minimum is 2, and maximum page size is 20.
- pageToken string? (default ()) - If specified, the next page of accounts is retrieved. The
pageToken
is returned when a call toaccounts.list
returns more results than can fit into the requested page size.
Return Type
- ListAccountsResponse|error - Successful response
createAccounts
function createAccounts(Account payload, string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType, string? primaryOwner) returns Account|error
Creates an account with the specified name and type under the given parent. - Personal accounts and Organizations cannot be created. - User Groups cannot be created with a Personal account as primary owner. - Location Groups cannot be created with a primary owner of a Personal account if the Personal account is in an Organization. - Location Groups cannot own Location Groups.
Parameters
- payload Account - Account request
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- primaryOwner string? (default ()) - The resource name of the account which will be the primary owner of the account being created. It should be of the form
accounts/{account_id}/
.
listAttributes
function listAttributes(string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType, string? categoryId, string? country, string? languageCode, string? name, int? pageSize, string? pageToken) returns ListAttributeMetadataResponse|error
Returns the list of available attributes that would be available for a location with the given primary category and country.
Parameters
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- categoryId string? (default ()) - The primary category stable ID to find available attributes.
- country string? (default ()) - The ISO 3166-1 alpha-2 country code to find available attributes.
- languageCode string? (default ()) - The BCP 47 code of language to get attribute display names in. If this language is not available, they will be provided in English.
- name string? (default ()) - Resource name of the location to look up available attributes.
- pageSize int? (default ()) - How many attributes to include per page. Default is 200, minimum is 1.
- pageToken string? (default ()) - If specified, the next page of attribute metadata is retrieved. The
pageToken
is returned when a call toattributes.list
returns more results than can fit into the requested page size.
Return Type
- ListAttributeMetadataResponse|error - Successful response
listCategories
function listCategories(string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType, string? languageCode, int? pageSize, string? pageToken, string? regionCode, string? searchTerm, string? view) returns ListBusinessCategoriesResponse|error
Returns a list of business categories. Search will match the category name but not the category ID. Note: Search only matches the front of a category name (that is, 'food' may return 'Food Court' but not 'Fast Food Restaurant').
Parameters
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- languageCode string? (default ()) - The BCP 47 code of language. If the language is not available, it will default to English.
- pageSize int? (default ()) - How many categories to fetch per page. Default is 100, minimum is 1, and maximum page size is 100.
- pageToken string? (default ()) - If specified, the next page of categories will be fetched.
- regionCode string? (default ()) - The ISO 3166-1 alpha-2 country code.
- searchTerm string? (default ()) - Optional filter string from user.
- view string? (default ()) - Optional. Specifies which parts to the Category resource should be returned in the response.
Return Type
- ListBusinessCategoriesResponse|error - Successful response
batchgetCategories
function batchgetCategories(string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType, string[]? categoryIds, string? languageCode, string? regionCode, string? view) returns BatchGetBusinessCategoriesResponse|error
Returns a list of business categories for the provided language and GConcept ids.
Parameters
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- categoryIds string[]? (default ()) - Required. At least one name must be set. The GConcept ids the localized category names should be returned for.
- languageCode string? (default ()) - Required. The BCP 47 code of language that the category names should be returned in.
- regionCode string? (default ()) - Optional. The ISO 3166-1 alpha-2 country code used to infer non-standard language.
- view string? (default ()) - Required. Specifies which parts to the Category resource should be returned in the response.
Return Type
- BatchGetBusinessCategoriesResponse|error - Successful response
searchChains
function searchChains(string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType, string? chainDisplayName, int? resultCount) returns SearchChainsResponse|error
Searches the chain based on chain name.
Parameters
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- chainDisplayName string? (default ()) - Search for a chain by its name. Exact/partial/fuzzy/related queries are supported. Examples: "walmart", "wal-mart", "walmmmart", "沃尔玛"
- resultCount int? (default ()) - The maximum number of matched chains to return from this query. The default is 10. The maximum possible value is 500.
Return Type
- SearchChainsResponse|error - Successful response
searchGooglelocations
function searchGooglelocations(SearchGoogleLocationsRequest payload, string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType) returns SearchGoogleLocationsResponse|error
Search all of the possible locations that are a match to the specified request.
Parameters
- payload SearchGoogleLocationsRequest - SearchGoogleLocations request
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Return Type
- SearchGoogleLocationsResponse|error - Successful response
getChains
function getChains(string name, string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType) returns Chain|error
Gets the specified chain. Returns NOT_FOUND
if the chain does not exist.
Parameters
- name string - The chain's resource name, in the format
chains/{chain_place_id}
.
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
updatenotificationsAccounts
function updatenotificationsAccounts(string name, Notifications payload, string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType) returns Notifications|error
Sets the pubsub notification settings for the account informing My Business which topic to send pubsub notifications for: - New reviews for locations administered by the account. - Updated reviews for locations administered by the account. - New GoogleUpdates
for locations administered by the account. An account will only have one notification settings resource, and only one pubsub topic can be set.
Parameters
- name string - The notification settings resource name.
- payload Notifications - Notifications request
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Return Type
- Notifications|error - Successful response
deleteAccountsLocationsQuestions
function deleteAccountsLocationsQuestions(string name, string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType) returns Response|error
Deletes a specific question written by the current user.
Parameters
- name string - The name of the question to delete.
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
patchAccountsLocationsQuestions
function patchAccountsLocationsQuestions(string name, Question payload, string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType, string? updateMask) returns Question|error
Updates a specific question written by the current user.
Parameters
- name string - The name of the question to update.
- payload Question - Question request
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- updateMask string? (default ()) - The specific fields to update. If no mask is specified, then this is treated as a full update and all editable fields are set to the values passed in.
reportinsightsAccountsLocationsLocalposts
function reportinsightsAccountsLocationsLocalposts(string name, ReportLocalPostInsightsRequest payload, string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType) returns ReportLocalPostInsightsResponse|error
Returns insights for a set of local posts associated with a single listing. Which metrics and how they are reported are options specified in the request proto. Note: Insight reports are limited to 100 local_post_names
per call.
Parameters
- name string - Required. The name of the location for which to fetch insights.
- payload ReportLocalPostInsightsRequest - ReportLocalPostInsights request
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Return Type
- ReportLocalPostInsightsResponse|error - Successful response
batchgetAccountsLocations
function batchgetAccountsLocations(string name, BatchGetLocationsRequest payload, string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType) returns BatchGetLocationsResponse|error
Gets all of the specified locations in the given account.
Parameters
- name string - The name of the account from which to fetch locations.
- payload BatchGetLocationsRequest - BatchGetLocations request
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Return Type
- BatchGetLocationsResponse|error - Successful response
batchgetreviewsAccountsLocations
function batchgetreviewsAccountsLocations(string name, BatchGetReviewsRequest payload, string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType) returns BatchGetReviewsResponse|error
Returns the paginated list of reviews for all specified locations. This operation is only valid if the specified locations are verified. Note: Reviews are limited to a batch size of 200 location_names
per call.
Parameters
- name string - The name of the account from which to retrieve a list of reviews across multiple locations.
- payload BatchGetReviewsRequest - BatchGetReviews request
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Return Type
- BatchGetReviewsResponse|error - Successful response
reportinsightsAccountsLocations
function reportinsightsAccountsLocations(string name, ReportLocationInsightsRequest payload, string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType) returns ReportLocationInsightsResponse|error
Returns a report containing insights on one or more metrics by location. Note: Insight reports are limited to a batch size of 10 location_names
per call.
Parameters
- name string - The account resource name.
- payload ReportLocationInsightsRequest - ReportLocationInsights request
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Return Type
- ReportLocationInsightsResponse|error - Successful response
updatereplyAccountsLocationsReviews
function updatereplyAccountsLocationsReviews(string name, ReviewReply payload, string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType) returns ReviewReply|error
Updates the reply to the specified review. A reply is created if one does not exist. This operation is only valid if the specified location is verified.
Parameters
- name string - The name of the review to respond to.
- payload ReviewReply - ReviewReply request
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Return Type
- ReviewReply|error - Successful response
deletereplyAccountsLocationsReviews
function deletereplyAccountsLocationsReviews(string name, string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType) returns Response|error
Deletes the response to the specified review. This operation is only valid if the specified location is verified.
Parameters
- name string - The name of the review reply to delete.
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
acceptAccountsInvitations
function acceptAccountsInvitations(string name, AcceptInvitationRequest payload, string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType) returns Response|error
Accepts the specified invitation.
Parameters
- name string - The name of the invitation that is being accepted.
- payload AcceptInvitationRequest - AcceptInvitation request
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
associateAccountsLocations
function associateAccountsLocations(string name, AssociateLocationRequest payload, string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType) returns Response|error
Associates a location to a place ID. Any previous association is overwritten. This operation is only valid if the location is unverified. The association must be valid, that is, it appears in the list of FindMatchingLocations
.
Parameters
- name string - The resource name of the location to associate.
- payload AssociateLocationRequest - AssociateLocation request
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
clearassociationAccountsLocations
function clearassociationAccountsLocations(string name, ClearLocationAssociationRequest payload, string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType) returns Response|error
Clears an association between a location and its place ID. This operation is only valid if the location is unverified.
Parameters
- name string - The resource name of the location to disassociate.
- payload ClearLocationAssociationRequest - ClearLocationAssociation request
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
completeAccountsLocationsVerifications
function completeAccountsLocationsVerifications(string name, CompleteVerificationRequest payload, string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType) returns CompleteVerificationResponse|error
Completes a PENDING
verification. It is only necessary for non AUTO
verification methods. AUTO
verification request is instantly VERIFIED
upon creation.
Parameters
- name string - Resource name of the verification to complete.
- payload CompleteVerificationRequest - CompleteVerification request
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Return Type
- CompleteVerificationResponse|error - Successful response
declineAccountsInvitations
function declineAccountsInvitations(string name, DeclineInvitationRequest payload, string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType) returns Response|error
Declines the specified invitation.
Parameters
- name string - The name of the account invitation that is being declined.
- payload DeclineInvitationRequest - DeclineInvitation request
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
fetchverificationoptionsAccountsLocations
function fetchverificationoptionsAccountsLocations(string name, FetchVerificationOptionsRequest payload, string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType) returns FetchVerificationOptionsResponse|error
Reports all eligible verification options for a location in a specific language.
Parameters
- name string - Resource name of the location to verify.
- payload FetchVerificationOptionsRequest - FetchVerificationOptions request
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Return Type
- FetchVerificationOptionsResponse|error - Successful response
findmatchesAccountsLocations
function findmatchesAccountsLocations(string name, FindMatchingLocationsRequest payload, string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType) returns FindMatchingLocationsResponse|error
Finds all of the possible locations that are a match to the specified location. This operation is only valid if the location is unverified.
Parameters
- name string - The resource name of the location to find matches for.
- payload FindMatchingLocationsRequest - FindMatchingLocations request
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Return Type
- FindMatchingLocationsResponse|error - Successful response
generateaccountnumberAccounts
function generateaccountnumberAccounts(string name, GenerateAccountNumberRequest payload, string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType) returns Account|error
Generates an account number for this account. The account number is not provisioned when an account is created. Use this request to create an account number when it is required.
Parameters
- name string - The name of the account to generate an account number for.
- payload GenerateAccountNumberRequest - GenerateAccountNumber request
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
getgoogleupdatedAccountsLocationsLodging
function getgoogleupdatedAccountsLocationsLodging(string name, string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType, string? readMask) returns GetGoogleUpdatedLodgingResponse|error
Returns the Google updated Lodging of a specific location.
Parameters
- name string - Required. Google identifier for this location in the form:
accounts/{account_id}/locations/{location_id}/lodging
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- readMask string? (default ()) - Optional. The specific fields to return. If no mask is specified, then it returns the full Lodging (same as "*"). Repeated field items cannot be individually specified.
Return Type
- GetGoogleUpdatedLodgingResponse|error - Successful response
getgoogleupdatedAccountsLocations
function getgoogleupdatedAccountsLocations(string name, string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType) returns GoogleUpdatedLocation|error
Gets the Google-updated version of the specified location. Returns NOT_FOUND
if the location does not exist.
Parameters
- name string - The name of the location to fetch.
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Return Type
- GoogleUpdatedLocation|error - Successful response
listrecommendgooglelocationsAccounts
function listrecommendgooglelocationsAccounts(string name, string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType, int? pageSize, string? pageToken) returns ListRecommendedGoogleLocationsResponse|error
List all the GoogleLocations that have been recommended to the specified GMB account. Recommendations are provided for personal accounts and location groups only, requests for all other account types will result in an error. The recommendations for location groups are based on the locations in that group. The recommendations for personal accounts are based on all of the locations that the user has access to on Google My Business (which includes locations they can access through location groups), and is a superset of all recommendations generated for the user.
Parameters
- name string - Name of the account resource to fetch recommended Google locations for.
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- pageSize int? (default ()) - How many locations to fetch per page. Default is 25, minimum is 1, and maximum page size is 100.
- pageToken string? (default ()) - If specified, the next page of locations is retrieved.
Return Type
- ListRecommendedGoogleLocationsResponse|error - Successful response
reportGooglelocations
function reportGooglelocations(string name, ReportGoogleLocationRequest payload, string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType) returns Response|error
Report a GoogleLocation.
Parameters
- name string - Resource name of a [GoogleLocation], in the format
googleLocations/{googleLocationId}
.
- payload ReportGoogleLocationRequest - ReportGoogleLocation request
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
transferAccountsLocations
function transferAccountsLocations(string name, TransferLocationRequest payload, string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType) returns Location|error
Moves a location from an account that the user owns to another account that the same user administers. The user must be an owner of the account the location is currently associated with and must also be at least a manager of the destination account. Returns the Location with its new resource name.
Parameters
- name string - The name of the location to transfer.
- payload TransferLocationRequest - TransferLocation request
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
verifyAccountsLocations
function verifyAccountsLocations(string name, VerifyLocationRequest payload, string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType) returns VerifyLocationResponse|error
Starts the verification process for a location.
Parameters
- name string - Resource name of the location to verify.
- payload VerifyLocationRequest - VerifyLocation request
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Return Type
- VerifyLocationResponse|error - Successful response
listAccountsLocationsAdmins
function listAccountsLocationsAdmins(string parent, string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType) returns ListLocationAdminsResponse|error
Lists all of the admins for the specified location.
Parameters
- parent string - The name of the location to list admins of.
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Return Type
- ListLocationAdminsResponse|error - Successful response
createAccountsLocationsAdmins
function createAccountsLocationsAdmins(string parent, Admin payload, string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType) returns Admin|error
Invites the specified user to become an administrator for the specified location. The invitee must accept the invitation in order to be granted access to the location. See AcceptInvitation to programmatically accept an invitation.
Parameters
- parent string - The resource name of the location this admin is created for.
- payload Admin - Admin request
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
listAccountsLocationsQuestionsAnswers
function listAccountsLocationsQuestionsAnswers(string parent, string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType, string? orderBy, int? pageSize, string? pageToken) returns ListAnswersResponse|error
Returns the paginated list of answers for a specified question.
Parameters
- parent string - The name of the question to fetch answers for.
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- orderBy string? (default ()) - The order to return the answers. Valid options include 'update_time desc' and 'upvote_count desc', which will return the answers sorted descendingly by the requested field. The default sort order is 'update_time desc'.
- pageSize int? (default ()) - How many answers to fetch per page. The default and maximum
page_size
values are 10.
- pageToken string? (default ()) - If specified, the next page of answers is retrieved.
Return Type
- ListAnswersResponse|error - Successful response
deleteAccountsLocationsQuestionsAnswers
function deleteAccountsLocationsQuestionsAnswers(string parent, string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType) returns Response|error
Deletes the answer written by the current user to a question.
Parameters
- parent string - The name of the question to delete an answer for.
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
upsertAccountsLocationsQuestionsAnswers
function upsertAccountsLocationsQuestionsAnswers(string parent, UpsertAnswerRequest payload, string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType) returns Answer|error
Creates an answer or updates the existing answer written by the user for the specified question. A user can only create one answer per question.
Parameters
- parent string - The name of the question to write an answer for.
- payload UpsertAnswerRequest - UpsertAnswer request
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
listAccountsLocationsInsurancenetworks
function listAccountsLocationsInsurancenetworks(string parent, string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType, string? languageCode, int? pageSize, string? pageToken) returns ListInsuranceNetworksResponse|error
Returns a list of all insurance networks supported by Google.
Parameters
- parent string - Required. The name of the location whose insurance networks will be listed. The name is in the form: accounts/{account_id}/locations/{location_id}
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- languageCode string? (default ()) - Optional. The BCP 47 code for the language. If a language code is not provided, it defaults to English. Right now only 'en' is supported.
- pageSize int? (default ()) - How many insurance networks to return per page. The default value is 5000. Maximum page size is 10000.
- pageToken string? (default ()) - If specified, returns the next page of insurance networks.
Return Type
- ListInsuranceNetworksResponse|error - Successful response
listAccountsInvitations
function listAccountsInvitations(string parent, string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType, string? targetType) returns ListInvitationsResponse|error
Lists pending invitations for the specified account.
Parameters
- parent string - The name of the account from which the list of invitations is being retrieved.
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- targetType string? (default ()) - Specifies which target types should appear in the response.
Return Type
- ListInvitationsResponse|error - Successful response
listAccountsLocationsLocalposts
function listAccountsLocationsLocalposts(string parent, string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType, int? pageSize, string? pageToken) returns ListLocalPostsResponse|error
Returns a list of local posts associated with a location.
Parameters
- parent string - The name of the location whose local posts will be listed.
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- pageSize int? (default ()) - How many local posts to return per page. Default of 20. The minimum is 1, and maximum page size is 100.
- pageToken string? (default ()) - If specified, returns the next page of local posts.
Return Type
- ListLocalPostsResponse|error - Successful response
createAccountsLocationsLocalposts
function createAccountsLocationsLocalposts(string parent, LocalPost payload, string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType) returns LocalPost|error
Creates a new local post associated with the specified location, and returns it.
Parameters
- parent string - The name of the location in which to create this local post.
- payload LocalPost - LocalPost request
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
listAccountsLocations
function listAccountsLocations(string parent, string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType, string? filter, string? languageCode, string? orderBy, int? pageSize, string? pageToken) returns ListLocationsResponse|error
Lists the locations for the specified account.
Parameters
- parent string - The name of the account to fetch locations from. If the Account is of AccountType PERSONAL, only Locations that are directly owned by the Account are returned, otherwise it will return all accessible locations from the Account, either directly or indirectly.
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- filter string? (default ()) - A filter constraining the locations to return. The response includes only entries that match the filter. If
filter
is empty, then constraints are applied and all locations (paginated) are retrieved for the requested account. For more information about valid fields and example usage, see Work with Location Data Guide.
- languageCode string? (default ()) - The BCP 47 code of language to get display location properties in. If this language is not available, they will be provided in the language of the location. If neither is available, they will be provided in English. Deprecated. After August 15th, 2020, this field will no longer be applied. Instead, the language of the location will always be used.
- orderBy string? (default ()) - Sorting order for the request. Multiple fields should be comma-separated, following SQL syntax. The default sorting order is ascending. To specify descending order, a suffix " desc" should be added. Valid fields to order_by are location_name and store_code. For example: "location_name, store_code desc" or "location_name" or "store_code desc"
- pageSize int? (default ()) - How many locations to fetch per page. Default is 100, minimum is 1, and maximum page size is 100.
- pageToken string? (default ()) - If specified, it fetches the next
page
of locations. The page token is returned by previous calls toListLocations
when there were more locations than could fit in the requested page size.
Return Type
- ListLocationsResponse|error - Successful response
createAccountsLocations
function createAccountsLocations(string parent, Location payload, string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType, string? requestId, boolean? validateOnly) returns Location|error
Creates a new location owned by the specified account, and returns it.
Parameters
- parent string - The name of the account in which to create this location.
- payload Location - Location request
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- requestId string? (default ()) - A unique request ID for the server to detect duplicated requests. We recommend using UUIDs. Max length is 50 characters.
- validateOnly boolean? (default ()) - If true, the request is validated without actually creating the location.
listAccountsLocationsMedia
function listAccountsLocationsMedia(string parent, string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType, int? pageSize, string? pageToken) returns ListMediaItemsResponse|error
Returns a list of media items associated with a location.
Parameters
- parent string - The name of the location whose media items will be listed.
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- pageSize int? (default ()) - How many media items to return per page. The default value is 100, which is also the maximum supported number of media items able to be added to a location with the My Business API. Maximum page size is 2500.
- pageToken string? (default ()) - If specified, returns the next page of media items.
Return Type
- ListMediaItemsResponse|error - Successful response
createAccountsLocationsMedia
function createAccountsLocationsMedia(string parent, MediaItem payload, string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType) returns MediaItem|error
Creates a new media item for the location.
Parameters
- parent string - The resource name of the location where this media item will be created.
- payload MediaItem - MediaItem request
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
listAccountsLocationsMediaCustomers
function listAccountsLocationsMediaCustomers(string parent, string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType, int? pageSize, string? pageToken) returns ListCustomerMediaItemsResponse|error
Returns a list of media items associated with a location that have been contributed by customers.
Parameters
- parent string - The name of the location whose customer media items will be listed.
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- pageSize int? (default ()) - How many media items to return per page. The default value is 100, the maximum supported page size is 200.
- pageToken string? (default ()) - If specified, returns the next page of media items.
Return Type
- ListCustomerMediaItemsResponse|error - Successful response
startuploadAccountsLocationsMedia
function startuploadAccountsLocationsMedia(string parent, StartUploadMediaItemDataRequest payload, string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType) returns MediaItemDataRef|error
Generates a MediaItemDataRef
for media item uploading.
Parameters
- parent string - The resource name of the location this media item is to be added to.
- payload StartUploadMediaItemDataRequest - StartUploadMediaItemData request
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Return Type
- MediaItemDataRef|error - Successful response
listAccountsLocationsQuestions
function listAccountsLocationsQuestions(string parent, string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType, int? answersPerQuestion, string? filter, string? orderBy, int? pageSize, string? pageToken) returns ListQuestionsResponse|error
Returns the paginated list of questions and some of its answers for a specified location.
Parameters
- parent string - The name of the location to fetch questions for.
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- answersPerQuestion int? (default ()) - How many answers to fetch per question. The default and maximum
answers_per_question
values are 10.
- filter string? (default ()) - A filter constraining the questions to return. The only filter currently supported is "ignore_answered=true"
- orderBy string? (default ()) - The order to return the questions. Valid options include 'update_time desc' and 'upvote_count desc', which will return the questions sorted descendingly by the requested field. The default sort order is 'update_time desc'.
- pageSize int? (default ()) - How many questions to fetch per page. The default and maximum
page_size
values are 10.
- pageToken string? (default ()) - If specified, the next page of questions is retrieved.
Return Type
- ListQuestionsResponse|error - Successful response
createAccountsLocationsQuestions
function createAccountsLocationsQuestions(string parent, Question payload, string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType) returns Question|error
Adds a question for the specified location.
Parameters
- parent string - The name of the location to write a question for.
- payload Question - Question request
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
listAccountsLocationsReviews
function listAccountsLocationsReviews(string parent, string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType, string? orderBy, int? pageSize, string? pageToken) returns ListReviewsResponse|error
Returns the paginated list of reviews for the specified location. This operation is only valid if the specified location is verified.
Parameters
- parent string - The name of the location to fetch reviews for.
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- orderBy string? (default ()) - Specifies the field to sort reviews by. If unspecified, the order of reviews returned will default to
update_time desc
. Valid orders to sort by arerating
,rating desc
andupdate_time desc
.
- pageSize int? (default ()) - How many reviews to fetch per page. The maximum
page_size
is 50.
- pageToken string? (default ()) - If specified, it fetches the next page of reviews.
Return Type
- ListReviewsResponse|error - Successful response
listAccountsLocationsVerifications
function listAccountsLocationsVerifications(string parent, string? xgafv, string? accessToken, string? alt, string? callback, string? fields, string? 'key, string? oauthToken, string? quotaUser, string? uploadProtocol, string? uploadType, int? pageSize, string? pageToken) returns ListVerificationsResponse|error
List verifications of a location, ordered by create time.
Parameters
- parent string - Resource name of the location that verification requests belong to.
- xgafv string? (default ()) - V1 error format.
- accessToken string? (default ()) - OAuth access token.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauthToken string? (default ()) - OAuth 2.0 token for the current user.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- pageSize int? (default ()) - How many verification to include per page. If not set, return all.
- pageToken string? (default ()) - If specified, returns the next page of verifications.
Return Type
- ListVerificationsResponse|error - Successful response
Records
googleapis.mybusiness: AcceptInvitationRequest
Request message for AccessControl.AcceptInvitation.
googleapis.mybusiness: Accessibility
Physical adaptations made to the property in consideration of varying levels of human physical ability.
Fields
- mobilityAccessible boolean? - Mobility accessible. Throughout the property there are physical adaptations to ease the stay of a person in a wheelchair, such as auto-opening doors, wide elevators, wide bathrooms or ramps.
- mobilityAccessibleElevator boolean? - Mobility accessible elevator. A lift that transports people from one level to another and is built to accommodate a wheelchair-using passenger owing to the width of its doors and placement of call buttons.
- mobilityAccessibleElevatorException string? - Mobility accessible elevator exception.
- mobilityAccessibleException string? - Mobility accessible exception.
- mobilityAccessibleParking boolean? - Mobility accessible parking. The presence of a marked, designated area of prescribed size in which only registered, labeled vehicles transporting a person with physical challenges may park.
- mobilityAccessibleParkingException string? - Mobility accessible parking exception.
- mobilityAccessiblePool boolean? - Mobility accessible pool. A swimming pool equipped with a mechanical chair that can be lowered and raised for the purpose of moving physically challenged guests into and out of the pool. May be powered by electricity or water. Also known as pool lift.
- mobilityAccessiblePoolException string? - Mobility accessible pool exception.
googleapis.mybusiness: Account
An account is a container for your business's locations. If you are the only user who manages locations for your business, you can use your personal Google Account. To share management of locations with multiple users, [create a business account] (https://support.google.com/business/answer/6085339?ref_topic=6085325).
Fields
- accountName string? - The name of the account. Note: For an account with AccountType
PERSONAL
, this is the first and last name of the user account.
- accountNumber string? - Account reference number if provisioned.
- name string? - The resource name, in the format
accounts/{account_id}
.
- organizationInfo OrganizationInfo? - Additional Info stored for an organization.
- permissionLevel string? - Output only. Specifies the PermissionLevel the caller has for this account.
- role string? - Output only. Specifies the AccountRole the caller has for this account.
- state AccountState? - Indicates status of the account, such as whether the account has been verified by Google.
- 'type string? - Output only. Specifies the AccountType of this account.
googleapis.mybusiness: AccountState
Indicates status of the account, such as whether the account has been verified by Google.
Fields
- status string? - If verified, future locations that are created are automatically connected to Google Maps, and have Google+ pages created, without requiring moderation.
googleapis.mybusiness: Activities
Amenities and features related to leisure and play.
Fields
- beachAccess boolean? - Beach access. The hotel property is in close proximity to a beach and offers a way to get to that beach. This can include a route to the beach such as stairs down if hotel is on a bluff, or a short trail. Not the same as beachfront (with beach access, the hotel's proximity is close to but not right on the beach).
- beachAccessException string? - Beach access exception.
- beachFront boolean? - Breach front. The hotel property is physically located on the beach alongside an ocean, sea, gulf, or bay. It is not on a lake, river, stream, or pond. The hotel is not separated from the beach by a public road allowing vehicular, pedestrian, or bicycle traffic.
- beachFrontException string? - Beach front exception.
- bicycleRental boolean? - Bicycle rental. The hotel owns bicycles that it permits guests to borrow and use. Can be free or for a fee.
- bicycleRentalException string? - Bicycle rental exception.
- boutiqueStores boolean? - Boutique stores. There are stores selling clothing, jewelry, art and decor either on hotel premises or very close by. Does not refer to the hotel gift shop or convenience store.
- boutiqueStoresException string? - Boutique stores exception.
- casino boolean? - Casino. A space designated for gambling and gaming featuring croupier-run table and card games, as well as electronic slot machines. May be on hotel premises or located nearby.
- casinoException string? - Casino exception.
- freeBicycleRental boolean? - Free bicycle rental. The hotel owns bicycles that it permits guests to borrow and use for free.
- freeBicycleRentalException string? - Free bicycle rental exception.
- freeWatercraftRental boolean? - Free watercraft rental. The hotel owns watercraft that it permits guests to borrow and use for free.
- freeWatercraftRentalException string? - Free Watercraft rental exception.
- gameRoom boolean? - Game room. There is a room at the hotel containing electronic machines for play such as pinball, prize machines, driving simulators, and other items commonly found at a family fun center or arcade. May also include non-electronic games like pool, foosball, darts, and more. May or may not be designed for children. Also known as arcade, fun room, or family fun center.
- gameRoomException string? - Game room exception.
- golf boolean? - Golf. There is a golf course on hotel grounds or there is a nearby, independently run golf course that allows use by hotel guests. Can be free or for a fee.
- golfException string? - Golf exception.
- horsebackRiding boolean? - Horseback riding. The hotel has a horse barn onsite or an affiliation with a nearby barn to allow for guests to sit astride a horse and direct it to walk, trot, cantor, gallop and/or jump. Can be in a riding ring, on designated paths, or in the wilderness. May or may not involve instruction.
- horsebackRidingException string? - Horseback riding exception.
- nightclub boolean? - Nightclub. There is a room at the hotel with a bar, a dance floor, and seating where designated staffers play dance music. There may also be a designated area for the performance of live music, singing and comedy acts.
- nightclubException string? - Nightclub exception.
- privateBeach boolean? - Private beach. The beach which is in close proximity to the hotel is open only to guests.
- privateBeachException string? - Private beach exception.
- scuba boolean? - Scuba. The provision for guests to dive under naturally occurring water fitted with a self-contained underwater breathing apparatus (SCUBA) for the purpose of exploring underwater life. Apparatus consists of a tank providing oxygen to the diver through a mask. Requires certification of the diver and supervision. The hotel may have the activity at its own waterfront or have an affiliation with a nearby facility. Required equipment is most often supplied to guests. Can be free or for a fee. Not snorkeling. Not done in a swimming pool.
- scubaException string? - Scuba exception.
- snorkeling boolean? - Snorkeling. The provision for guests to participate in a recreational water activity in which swimmers wear a diving mask, a simple, shaped breathing tube and flippers/swim fins for the purpose of exploring below the surface of an ocean, gulf or lake. Does not usually require user certification or professional supervision. Equipment may or may not be available for rent or purchase. Not scuba diving.
- snorkelingException string? - Snorkeling exception.
- tennis boolean? - Tennis. The hotel has the requisite court(s) on site or has an affiliation with a nearby facility for the purpose of providing guests with the opportunity to play a two-sided court-based game in which players use a stringed racquet to hit a ball across a net to the side of the opposing player. The court can be indoors or outdoors. Instructors, racquets and balls may or may not be provided.
- tennisException string? - Tennis exception.
- waterSkiing boolean? - Water skiing. The provision of giving guests the opportunity to be pulled across naturally occurring water while standing on skis and holding a tow rope attached to a motorboat. Can occur on hotel premises or at a nearby waterfront. Most often performed in a lake or ocean.
- waterSkiingException string? - Water skiing exception.
- watercraftRental boolean? - Watercraft rental. The hotel owns water vessels that it permits guests to borrow and use. Can be free or for a fee. Watercraft may include boats, pedal boats, rowboats, sailboats, powerboats, canoes, kayaks, or personal watercraft (such as a Jet Ski).
- watercraftRentalException string? - Watercraft rental exception.
googleapis.mybusiness: AddressInput
Input for ADDRESS verification.
Fields
- mailerContactName string? - Contact name the mail should be sent to.
googleapis.mybusiness: AddressVerificationData
Display data for verifications through postcard.
Fields
- address PostalAddress? - Represents a postal address, e.g. for postal delivery or payments addresses. Given a postal address, a postal service can deliver items to a premise, P.O. Box or similar. It is not intended to model geographical locations (roads, towns, mountains). In typical usage an address would be created via user input or from importing existing data, depending on the type of process. Advice on address input / editing: - Use an i18n-ready address widget such as https://github.com/google/libaddressinput) - Users should not be presented with UI elements for input or editing of fields outside countries where that field is used. For more guidance on how to use this schema, please see: https://support.google.com/business/answer/6397478
- businessName string? - Merchant's business name.
googleapis.mybusiness: Admin
An administrator of an Account or a Location.
Fields
- adminName string? - The name of the admin. When making the initial invitation, this is the invitee's email address. On
GET
calls, the user's email address is returned if the invitation is still pending. Otherwise, it contains the user's first and last names.
- name string? - The resource name. For account admins, this is in the form:
accounts/{account_id}/admins/{admin_id}
For location admins, this is in the form:accounts/{account_id}/locations/{location_id}/admins/{admin_id}
- pendingInvitation boolean? - Output only. Indicates whether this admin has a pending invitation for the specified resource.
- role string? - Specifies the AdminRole that this admin uses with the specified Account or Location resource.
googleapis.mybusiness: AdWordsLocationExtensions
Additional information that is surfaced in AdWords.
Fields
- adPhone string? - An alternate phone number to display on AdWords location extensions instead of the location's primary phone number.
googleapis.mybusiness: Answer
Represents an answer to a question
Fields
- author Author? - Represents the author of a question or answer
- createTime string? - Output only. The timestamp for when the answer was written.
- name string? - Output only. The unique name for the answer accounts//locations//questions//answers/
- text string? - The text of the answer. It should contain at least one non-whitespace character. The maximum length is 4096 characters.
- updateTime string? - Output only. The timestamp for when the answer was last modified.
- upvoteCount int? - Output only. The number of upvotes for the answer.
googleapis.mybusiness: AssociateLocationRequest
Request message for Locations.AssociateLocationRequest.
Fields
- placeId string? - The association to establish. If not set, it indicates no match.
googleapis.mybusiness: Attribute
A location attribute. Attributes provide additional information about a location. The attributes that can be set on a location may vary based on the properties of that location (for example, category). Available attributes are determined by Google and may be added and removed without API changes.
Fields
- attributeId string? - The ID of the attribute. Attribute IDs are provided by Google.
- repeatedEnumValue RepeatedEnumAttributeValue? - Values for an attribute with a
value_type
of REPEATED_ENUM. This consists of two lists of value IDs: those that are set (true) and those that are unset (false). Values absent are considered unknown. At least one value must be specified.
- urlValues UrlAttributeValue[]? - When the attribute value type is URL, this field contains the value(s) for this attribute, and the other values fields must be empty.
- valueType string? - Output only. The type of value that this attribute contains. This should be used to determine how to interpret the value.
- values anydata[]? - The values for this attribute. The type of the values supplied must match that expected for that attribute; see AttributeValueType. This is a repeated field where multiple attribute values may be provided. Attribute types only support one value.
googleapis.mybusiness: AttributeMetadata
Metadata for an attribute. Contains display information for the attribute, including a localized name and a heading for grouping related attributes together.
Fields
- attributeId string? - The ID of the attribute.
- displayName string? - The localized display name for the attribute, if available; otherwise, the English display name.
- groupDisplayName string? - The localized display name of the group that contains this attribute, if available; otherwise, the English group name. Related attributes are collected into a group and should be displayed together under the heading given here.
- isDeprecated boolean? - If true, the attribute is deprecated and should no longer be used. If deprecated, updating this attribute will not result in an error, but updates will not be saved. At some point after being deprecated, the attribute will be removed entirely and it will become an error.
- isRepeatable boolean? - If true, the attribute supports multiple values. If false, only a single value should be provided.
- valueMetadata AttributeValueMetadata[]? - For some types of attributes (for example, enums), a list of supported values and corresponding display names for those values is provided.
- valueType string? - The value type for the attribute. Values set and retrieved should be expected to be of this type.
googleapis.mybusiness: AttributeValueMetadata
Metadata for supported attribute values.
Fields
- displayName string? - The display name for this value, localized where available; otherwise, in English. The value display name is intended to be used in context with the attribute display name. For example, for a "WiFi" enum attribute, this could contain "Paid" to represent paid Wi-Fi.
- value anydata? - The attribute value.
googleapis.mybusiness: Attribution
Attribution information for customer media items, such as the contributor's name and profile picture.
Fields
- profileName string? - The user name to attribute the media item to.
- profilePhotoUrl string? - URL of the attributed user's profile photo thumbnail.
- profileUrl string? - The URL of the attributed user's Google Maps profile page.
- takedownUrl string? - The URL of the takedown page, where the media item can be reported if it is inappropriate.
googleapis.mybusiness: Author
Represents the author of a question or answer
Fields
- displayName string? - The display name of the user
- profilePhotoUrl string? - The profile photo URL of the user.
- 'type string? - The type of user the author is.
googleapis.mybusiness: BasicMetricsRequest
A request for basic metric insights.
Fields
- metricRequests MetricRequest[]? - A collection of metrics to return values for including the options for how the data should be returned.
- timeRange TimeRange? - A range of time. Data will be pulled over the range as a half-open inverval (that is, [start_time, end_time)).
googleapis.mybusiness: BatchGetBusinessCategoriesResponse
Response message for BusinessCategories.BatchGetBusinessCategories.
Fields
- categories Category[]? - Categories that match the GConcept ids provided in the request. They will not come in the same order as category ids in the request.
googleapis.mybusiness: BatchGetLocationsRequest
Request message for Locations.BatchGetLocations.
Fields
- locationNames string[]? - A collection of locations to fetch, specified by their names.
googleapis.mybusiness: BatchGetLocationsResponse
Response message for Locations.BatchGetLocations.
Fields
- locations Location[]? - A collection of locations.
googleapis.mybusiness: BatchGetReviewsRequest
Request message for Reviews.BatchGetReviews.
Fields
- ignoreRatingOnlyReviews boolean? - Whether to ignore rating-only reviews.
- locationNames string[]? - A collection of locations to fetch reviews for, specified by their names.
- orderBy string? - Optional. Specifies the field to sort reviews by. If unspecified, the order of reviews returned will default to
update_time desc
. Valid orders to sort by arerating
,rating desc
andupdate_time desc
.rating
will return reviews in ascending order.update_time
(i.e. ascending order) is not supported.
- pageSize int? - How many reviews to fetch per page. The default value is 200.
- pageToken string? - If specified, it fetches the next page of reviews.
googleapis.mybusiness: BatchGetReviewsResponse
Response message for Reviews.BatchGetReviews.
Fields
- locationReviews LocationReview[]? - Reviews with location information.
- nextPageToken string? - If the number of reviews exceeded the requested page size, this field is populated with a token to fetch the next page of reviews on a subsequent calls. If there are no more reviews, this field will not be present in the response.
googleapis.mybusiness: Business
Features of the property of specific interest to the business traveler.
Fields
- businessCenter boolean? - Business center. A designated room at the hotel with one or more desks and equipped with guest-use computers, printers, fax machines and/or photocopiers. May or may not be open 24/7. May or may not require a key to access. Not a meeting room or conference room.
- businessCenterException string? - Business center exception.
- meetingRooms boolean? - Meeting rooms. Rooms at the hotel designated for business-related gatherings. Rooms are usually equipped with tables or desks, office chairs and audio/visual facilities to allow for presentations and conference calls. Also known as conference rooms.
- meetingRoomsCount int? - Meeting rooms count. The number of meeting rooms at the property.
- meetingRoomsCountException string? - Meeting rooms count exception.
- meetingRoomsException string? - Meeting rooms exception.
googleapis.mybusiness: BusinessHours
Represents the time periods that this location is open for business. Holds a collection of TimePeriod instances.
Fields
- periods TimePeriod[]? - A collection of times that this location is open for business. Each period represents a range of hours when the location is open during the week.
googleapis.mybusiness: CallToAction
An action that is performed when the user clicks through the post
Fields
- actionType string? - The type of action that will be performed.
- url string? - The URL the user will be directed to upon clicking. This field should be left unset for Call CTA.
googleapis.mybusiness: CaloriesFact
This message denotes calories information with an upper bound and lower bound range. Lower amount must be specified. Both lower and upper amounts are non-negative numbers.
Fields
- lowerAmount int? - Required. Lower amount of calories
- unit string? - Required. Unit of the given calories information.
- upperAmount int? - Optional. Upper amount of calories
googleapis.mybusiness: Category
A category describing what this business is (not what it does). For a list of valid category IDs, and the mappings to their human-readable names, see categories.list.
Fields
- categoryId string? - @OutputOnly. A stable ID (provided by Google) for this category. The
category_id
must be specified when modifying the category (when creating or updating a location).
- displayName string? - @OutputOnly. The human-readable name of the category. This is set when reading the location. When modifying the location,
category_id
must be set.
- moreHoursTypes MoreHoursType[]? - Output only. More hours types that are available for this business category.
- serviceTypes ServiceType[]? - @OutputOnly. A list of all the service types that are available for this business category.
googleapis.mybusiness: Chain
A chain is a brand that your business's locations can be affiliated with.
Fields
- chainNames ChainName[]? - Names of the chain.
- locationCount int? - Number of locations that are part of this chain.
- name string? - The chain's resource name, in the format
chains/{chain_place_id}
.
- websites ChainUrl[]? - Websites of the chain.
googleapis.mybusiness: ChainName
Name to be used when displaying the chain.
Fields
- displayName string? - The display name for this chain.
- languageCode string? - The BCP 47 code of language of the name.
googleapis.mybusiness: ChainUrl
Url to be used when displaying the chain.
Fields
- url string? - The url for this chain.
googleapis.mybusiness: ClearLocationAssociationRequest
Request message for Locations.ClearLocationAssociationRequest.
googleapis.mybusiness: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
googleapis.mybusiness: CompleteVerificationRequest
Request message for Verifications.CompleteVerificationAction.
Fields
- pin string? - PIN code received by the merchant to complete the verification.
googleapis.mybusiness: CompleteVerificationResponse
Response message for Verifications.CompleteVerificationAction.
Fields
- verification Verification? - A verification represents a verification attempt on a location.
googleapis.mybusiness: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
googleapis.mybusiness: Connectivity
The ways in which the property provides guests with the ability to access the internet.
Fields
- freeWifi boolean? - Free wifi. The hotel offers guests wifi for free.
- freeWifiException string? - Free wifi exception.
- publicAreaWifiAvailable boolean? - Public area wifi available. Guests have the ability to wirelessly connect to the internet in the areas of the hotel accessible to anyone. Can be free or for a fee.
- publicAreaWifiAvailableException string? - Public area wifi available exception.
- publicInternetTerminal boolean? - Public internet terminal. An area of the hotel supplied with computers and designated for the purpose of providing guests with the ability to access the internet.
- publicInternetTerminalException string? - Public internet terminal exception.
- wifiAvailable boolean? - Wifi available. The hotel provides the ability for guests to wirelessly connect to the internet. Can be in the public areas of the hotel and/or in the guest rooms. Can be free or for a fee.
- wifiAvailableException string? - Wifi available exception.
googleapis.mybusiness: Date
Represents a whole or partial calendar date, such as a birthday. The time of day and time zone are either specified elsewhere or are insignificant. The date is relative to the Gregorian Calendar. This can represent one of the following: * A full date, with non-zero year, month, and day values * A month and day value, with a zero year, such as an anniversary * A year on its own, with zero month and day values * A year and month value, with a zero day, such as a credit card expiration date Related types are google.type.TimeOfDay and google.protobuf.Timestamp
.
Fields
- day int? - Day of a month. Must be from 1 to 31 and valid for the year and month, or 0 to specify a year by itself or a year and month where the day isn't significant.
- month int? - Month of a year. Must be from 1 to 12, or 0 to specify a year without a month and day.
- year int? - Year of the date. Must be from 1 to 9999, or 0 to specify a date without a year.
googleapis.mybusiness: DeclineInvitationRequest
Request message for AccessControl.DeclineInvitation.
googleapis.mybusiness: DimensionalMetricValue
A value for a single metric with a given time dimension.
Fields
- metricOption string? - The option that requested this dimensional value.
- timeDimension TimeDimension? - The dimension for which data is divided over.
- value string? - The value. If no value is set, then the requested data is missing.
googleapis.mybusiness: Dimensions
Dimensions of the media item.
Fields
- heightPixels int? - Height of the media item, in pixels.
- widthPixels int? - Width of the media item, in pixels.
googleapis.mybusiness: DrivingDirectionMetricsRequest
A request for driving direction insights.
Fields
- languageCode string? - The BCP 47 code for the language. If a language code is not provided, it defaults to English.
- numDays string? - The number of days to aggregate data for. Results returned will be available data over the last number of requested days. Valid values are 7, 30, and 90.
googleapis.mybusiness: Duplicate
Information about the location that this location duplicates.
Fields
- access string? - Indicates whether the user has access to the location it duplicates.
- locationName string? - The resource name of the location that this duplicates. Only populated if the authenticated user has access rights to that location and that location is not deleted.
- placeId string? - The place ID of the location that this duplicates.
googleapis.mybusiness: EmailInput
Input for EMAIL verification.
Fields
- emailAddress string? - Email address where the PIN should be sent to. An email address is accepted only if it is one of the addresses provided by FetchVerificationOptions. If the EmailVerificationData has is_user_name_editable set to true, the client may specify a different user name (local-part) but must match the domain name.
googleapis.mybusiness: EmailVerificationData
Display data for verifications through email.
Fields
- domainName string? - Domain name in the email address. e.g. "gmail.com" in foo@gmail.com
- isUserNameEditable boolean? - Whether client is allowed to provide a different user name.
- userName string? - User name in the email address. e.g. "foo" in foo@gmail.com
googleapis.mybusiness: Empty
A generic empty message that you can re-use to avoid defining duplicated empty messages in your APIs. A typical example is to use it as the request or the response type of an API method. For instance: service Foo { rpc Bar(google.protobuf.Empty) returns (google.protobuf.Empty); } The JSON representation for Empty
is empty JSON object {}
.
googleapis.mybusiness: EnhancedCleaning
Enhanced cleaning measures implemented by the hotel during COVID-19.
Fields
- commercialGradeDisinfectantCleaning boolean? - Commercial-grade disinfectant used to clean the property.
- commercialGradeDisinfectantCleaningException string? - Commercial grade disinfectant cleaning exception.
- commonAreasEnhancedCleaning boolean? - Enhanced cleaning of common areas.
- commonAreasEnhancedCleaningException string? - Common areas enhanced cleaning exception.
- employeesTrainedCleaningProcedures boolean? - Employees trained in COVID-19 cleaning procedures.
- employeesTrainedCleaningProceduresException string? - Employees trained cleaning procedures exception.
- employeesTrainedThoroughHandWashing boolean? - Employees trained in thorough hand-washing.
- employeesTrainedThoroughHandWashingException string? - Employees trained thorough hand washing exception.
- employeesWearProtectiveEquipment boolean? - Employees wear masks, face shields, and/or gloves.
- employeesWearProtectiveEquipmentException string? - Employees wear protective equipment exception.
- guestRoomsEnhancedCleaning boolean? - Enhanced cleaning of guest rooms.
- guestRoomsEnhancedCleaningException string? - Guest rooms enhanced cleaning exception.
googleapis.mybusiness: Families
Services and amenities for families and young guests.
Fields
- babysitting boolean? - Babysitting. Child care that is offered by hotel staffers or coordinated by hotel staffers with local child care professionals. Can be free or for a fee.
- babysittingException string? - Babysitting exception.
- kidsActivities boolean? - Kids activities. Recreational options such as sports, films, crafts and games designed for the enjoyment of children and offered at the hotel. May or may not be supervised. May or may not be at a designated time or place. Cab be free or for a fee.
- kidsActivitiesException string? - Kids activities exception.
- kidsClub boolean? - Kids club. An organized program of group activities held at the hotel and designed for the enjoyment of children. Facilitated by hotel staff (or staff procured by the hotel) in an area(s) designated for the purpose of entertaining children without their parents. May include games, outings, water sports, team sports, arts and crafts, and films. Usually has set hours. Can be free or for a fee. Also known as Kids Camp or Kids program.
- kidsClubException string? - Kids club exception.
googleapis.mybusiness: FetchVerificationOptionsRequest
Request message for Verifications.FetchVerificationOptions.
Fields
- context ServiceBusinessContext? - Additional data for service business verification.
- languageCode string? - The BCP 47 language code representing the language that is to be used for the verification process. Available options vary by language.
googleapis.mybusiness: FetchVerificationOptionsResponse
Response message for Verifications.FetchVerificationOptions.
Fields
- options VerificationOption[]? - The available verification options.
googleapis.mybusiness: FindMatchingLocationsRequest
Request message for Locations.FindMatchingLocations.
Fields
- languageCode string? - The preferred language for the matching location (in BCP-47 format).
- maxCacheDuration string? - Deprecated. This field is ignored for all requests.
- numResults int? - The number of matches to return. The default value is 3, with a maximum of 10. Note that latency may increase if more are requested. There is no pagination.
googleapis.mybusiness: FindMatchingLocationsResponse
Response message for Locations.FindMatchingLocations.
Fields
- matchTime string? - When the matching algorithm was last executed for this location.
- matchedLocations MatchedLocation[]? - A collection of locations that are potential matches to the specified location, listed in order from best to least match. If there is an exact match, it will be in the first position.
googleapis.mybusiness: FollowersMetadata
Follower metadata for a location.
Fields
- count string? - Total number of followers for the location.
- name string? - The resource name for this. accounts/{account_id}/locations/{location_id}/followers/metadata
googleapis.mybusiness: FoodAndDrink
Meals, snacks, and beverages available at the property.
Fields
- bar boolean? - Bar. A designated room, lounge or area of an on-site restaurant with seating at a counter behind which a hotel staffer takes the guest's order and provides the requested alcoholic drink. Can be indoors or outdoors. Also known as Pub.
- barException string? - Bar exception.
- breakfastAvailable boolean? - Breakfast available. The morning meal is offered to all guests. Can be free or for a fee.
- breakfastAvailableException string? - Breakfast available exception.
- breakfastBuffet boolean? - Breakfast buffet. Breakfast meal service where guests serve themselves from a variety of dishes/foods that are put out on a table.
- breakfastBuffetException string? - Breakfast buffet exception.
- buffet boolean? - Buffet. A type of meal where guests serve themselves from a variety of dishes/foods that are put out on a table. Includes lunch and/or dinner meals. A breakfast-only buffet is not sufficient.
- buffetException string? - Buffet exception.
- dinnerBuffet boolean? - Dinner buffet. Dinner meal service where guests serve themselves from a variety of dishes/foods that are put out on a table.
- dinnerBuffetException string? - Dinner buffet exception.
- freeBreakfast boolean? - Free breakfast. Breakfast is offered for free to all guests. Does not apply if limited to certain room packages.
- freeBreakfastException string? - Free breakfast exception.
- restaurant boolean? - Restaurant. A business onsite at the hotel that is open to the public as well as guests, and offers meals and beverages to consume at tables or counters. May or may not include table service. Also known as cafe, buffet, eatery. A "breakfast room" where the hotel serves breakfast only to guests (not the general public) does not count as a restaurant.
- restaurantException string? - Restaurant exception.
- restaurantsCount int? - Restaurants count. The number of restaurants at the hotel.
- restaurantsCountException string? - Restaurants count exception.
- roomService boolean? - Room service. A hotel staffer delivers meals prepared onsite to a guest's room as per their request. May or may not be available during specific hours. Services should be available to all guests (not based on rate/room booked/reward program, etc).
- roomServiceException string? - Room service exception.
- tableService boolean? - Table service. A restaurant in which a staff member is assigned to a guest's table to take their order, deliver and clear away food, and deliver the bill, if applicable. Also known as sit-down restaurant.
- tableServiceException string? - Table service exception.
- twentyFourHourRoomService boolean? - 24hr room service. Room service is available 24 hours a day.
- twentyFourHourRoomServiceException string? - 24hr room service exception.
- vendingMachine boolean? - Vending machine. A glass-fronted mechanized cabinet displaying and dispensing snacks and beverages for purchase by coins, paper money and/or credit cards.
- vendingMachineException string? - Vending machine exception.
googleapis.mybusiness: FoodMenu
Menu of a business that serves food dishes.
Fields
- cuisines string[]? - Optional. Cuisine information for the food menu. It is highly recommended to provide this field.
- labels MenuLabel[]? - Required. Language-tagged labels for the menu. E.g. "menu", "lunch special". Display names should be 140 characters or less, with descriptions 1,000 characters or less. At least one set of labels is required.
- sections FoodMenuSection[]? - Required. Sections of the menu.
- sourceUrl string? - Optional. Source URL of menu if there is a webpage to go to.
googleapis.mybusiness: FoodMenuItem
Item of a Section. It can be the dish itself, or can contain multiple FoodMenuItemOption.
Fields
- attributes FoodMenuItemAttributes? - Attributes of a food item/dish.
- labels MenuLabel[]? - Required. Language tagged labels for this menu item. Display names should be 140 characters or less, with descriptions 1,000 characters or less. At least one set of labels is required.
- options FoodMenuItemOption[]? - Optional. This is for an item that comes in multiple different options, and users are required to make choices. E.g. "regular" vs. "large" pizza. When options are specified, labels and attributes at item level will automatically become the first option's labels and attributes. Clients only need to specify other additional food options in this field.
googleapis.mybusiness: FoodMenuItemAttributes
Attributes of a food item/dish.
Fields
- allergen string[]? - Optional. Allergens associated with the food dish. It is highly recommended to provide this field.
- dietaryRestriction string[]? - Optional. Dietary information of the food dish. It is highly recommended to provide this field.
- ingredients Ingredient[]? - Optional. Ingredients of the food dish option.
- mediaKeys string[]? - Optional. The media keys of the media associated with the dish. Only photo media is supported. When there are multiple photos associated, the first photo is considered as the preferred photo.
- nutritionFacts NutritionFacts? - This message represents nutrition facts for a food dish.
- portionSize PortionSize? - Serving portion size of a food dish.
- preparationMethods string[]? - Optional. Methods on how the food dish option is prepared.
- price Money? - Represents an amount of money with its currency type.
- servesNumPeople int? - Optional. Number of people can be served by this food dish option.
- spiciness string? - Optional. Spiciness level of the food dish.
googleapis.mybusiness: FoodMenuItemOption
Option of an Item. It requires an explicit user selection.
Fields
- attributes FoodMenuItemAttributes? - Attributes of a food item/dish.
- labels MenuLabel[]? - Required. Language tagged labels for this menu item option. E.g.: "beef pad thai", "veggie pad thai", "small pizza", "large pizza". Display names should be 140 characters or less, with descriptions 1,000 characters or less. At least one set of labels is required.
googleapis.mybusiness: FoodMenus
Menus of a business that serve food dishes.
Fields
- menus FoodMenu[]? - Optional. A collection of food menus.
- name string? - Required. Google identifier for this location in the form:
accounts/{account_id}/locations/{location_id}/foodMenus
googleapis.mybusiness: FoodMenuSection
Section of a menu. It can contain multiple items/dishes.
Fields
- items FoodMenuItem[]? - Required. Items of the section. Each Section must have at least an item.
- labels MenuLabel[]? - Required. Language tagged labels for this menu section. Display names should be 140 characters or less, with descriptions 1,000 characters or less. At least one set of labels is required.
googleapis.mybusiness: FreeFormServiceItem
Represents a free-form service offered by the merchant. These are services that are not exposed as part of our structure service data. The merchant manually enters the names for of such services via a geomerchant surface.
Fields
- categoryId string? - Required. The
category_id
andservice_type_id
should match the possible combinations provided in theCategory
message.
- label Label? - Label to be used when displaying the price list, section, or item.
googleapis.mybusiness: GenerateAccountNumberRequest
Request message for Accounts.GenerateAccountNumber.
googleapis.mybusiness: GetGoogleUpdatedLodgingResponse
Response message for LodgingService.GetGoogleUpdatedLodging
Fields
- diffMask string? - Required. The fields in the Lodging that have been updated by Google. Repeated field items are not individually specified.
- lodging Lodging? - Lodging of a location that provides accomodations.
googleapis.mybusiness: GoogleLocation
Represents a Location that is present on Google. This can be a location that has been claimed by the user, someone else, or could be unclaimed.
Fields
- location Location? - A location. See the [help center article] (https://support.google.com/business/answer/3038177) for a detailed description of these fields, or the category endpoint for a list of valid business categories.
- name string? - Resource name of this GoogleLocation, in the format
googleLocations/{googleLocationId}
.
- requestAdminRightsUrl string? - A URL that will redirect the user to the request admin rights UI. This field is only present if the location has already been claimed by any user, including the current user.
googleapis.mybusiness: GoogleUpdatedLocation
Represents a location that was modified by Google.
Fields
- diffMask string? - The fields that Google updated.
- location Location? - A location. See the [help center article] (https://support.google.com/business/answer/3038177) for a detailed description of these fields, or the category endpoint for a list of valid business categories.
googleapis.mybusiness: GuestUnitFeatures
Features and available amenities in the guest unit.
Fields
- bungalowOrVilla boolean? - Bungalow or villa. An independent structure that is part of a hotel or resort that is rented to one party for a vacation stay. The hotel or resort may be completely comprised of bungalows or villas, or they may be one of several guestroom options. Guests in the bungalows or villas most often have the same, if not more, amenities and services offered to guests in other guestroom types.
- bungalowOrVillaException string? - Bungalow or villa exception.
- connectingUnitAvailable boolean? - Connecting unit available. A guestroom type that features access to an adjacent guestroom for the purpose of booking both rooms. Most often used by families who need more than one room to accommodate the number of people in their group.
- connectingUnitAvailableException string? - Connecting unit available exception.
- executiveFloor boolean? - Executive floor. A floor of the hotel where the guestrooms are only bookable by members of the hotel's frequent guest membership program. Benefits of this room class include access to a designated lounge which may or may not feature free breakfast, cocktails or other perks specific to members of the program.
- executiveFloorException string? - Executive floor exception.
- maxAdultOccupantsCount int? - Max adult occupants count. The total number of adult guests allowed to stay overnight in the guestroom.
- maxAdultOccupantsCountException string? - Max adult occupants count exception.
- maxChildOccupantsCount int? - Max child occupants count. The total number of children allowed to stay overnight in the room.
- maxChildOccupantsCountException string? - Max child occupants count exception.
- maxOccupantsCount int? - Max occupants count. The total number of guests allowed to stay overnight in the guestroom.
- maxOccupantsCountException string? - Max occupants count exception.
- privateHome boolean? - Private home. A privately owned home (house, townhouse, apartment, cabin, bungalow etc) that may or not serve as the owner's residence, but is rented out in its entirety or by the room(s) to paying guest(s) for vacation stays. Not for lease-based, long-term residency.
- privateHomeException string? - Private home exception.
- suite boolean? - Suite. A guestroom category that implies both a bedroom area and a separate living area. There may or may not be full walls and doors separating the two areas, but regardless, they are very distinct. Does not mean a couch or chair in a bedroom.
- suiteException string? - Suite exception.
- tier string? - Tier. Classification of the unit based on available features/amenities. A non-standard tier is only permitted if at least one other unit type falls under the standard tier.
- tierException string? - Tier exception.
- totalLivingAreas LivingArea? - An individual room, such as kitchen, bathroom, bedroom, within a bookable guest unit.
- views ViewsFromUnit? - Views available from the guest unit itself.
googleapis.mybusiness: GuestUnitType
A specific type of unit primarily defined by its features.
Fields
- codes string[]? - Required. Unit or room code identifiers for a single GuestUnitType. Each code must be unique within a Lodging instance.
- features GuestUnitFeatures? - Features and available amenities in the guest unit.
- label string? - Required. Short, English label or name of the GuestUnitType. Target <50 chars.
googleapis.mybusiness: HealthAndSafety
Health and safety measures implemented by the hotel during COVID-19.
Fields
- enhancedCleaning EnhancedCleaning? - Enhanced cleaning measures implemented by the hotel during COVID-19.
- increasedFoodSafety IncreasedFoodSafety? - Increased food safety measures implemented by the hotel during COVID-19.
- minimizedContact MinimizedContact? - Minimized contact measures implemented by the hotel during COVID-19.
- personalProtection PersonalProtection? - Personal protection measures implemented by the hotel during COVID-19.
- physicalDistancing PhysicalDistancing? - Physical distancing measures implemented by the hotel during COVID-19.
googleapis.mybusiness: HealthProviderAttributes
The Health provider attributes linked with this location.
Fields
- insuranceNetworks InsuranceNetwork[]? - Optional. A list of insurance networks accpected by this location.
- name string? - Required. Google identifier for this location in the form:
accounts/{account_id}/locations/{location_id}/healthProviderAttributes
googleapis.mybusiness: Housekeeping
Conveniences provided in guest units to facilitate an easier, more comfortable stay.
Fields
- dailyHousekeeping boolean? - Daily housekeeping. Guest units are cleaned by hotel staff daily during guest's stay.
- dailyHousekeepingException string? - Daily housekeeping exception.
- housekeepingAvailable boolean? - Housekeeping available. Guest units are cleaned by hotel staff during guest's stay. Schedule may vary from daily, weekly, or specific days of the week.
- housekeepingAvailableException string? - Housekeeping available exception.
- turndownService boolean? - Turndown service. Hotel staff enters guest units to prepare the bed for sleep use. May or may not include some light housekeeping. May or may not include an evening snack or candy. Also known as evening service.
- turndownServiceException string? - Turndown service exception.
googleapis.mybusiness: IncreasedFoodSafety
Increased food safety measures implemented by the hotel during COVID-19.
Fields
- diningAreasAdditionalSanitation boolean? - Additional sanitation in dining areas.
- diningAreasAdditionalSanitationException string? - Dining areas additional sanitation exception.
- disposableFlatware boolean? - Disposable flatware.
- disposableFlatwareException string? - Disposable flatware exception.
- foodPreparationAndServingAdditionalSafety boolean? - Additional safety measures during food prep and serving.
- foodPreparationAndServingAdditionalSafetyException string? - Food preparation and serving additional safety exception.
- individualPackagedMeals boolean? - Individually-packaged meals.
- individualPackagedMealsException string? - Individual packaged meals exception.
- singleUseFoodMenus boolean? - Single-use menus.
- singleUseFoodMenusException string? - Single use food menus exception.
googleapis.mybusiness: Ingredient
This message denotes an ingredient information of a food dish.
Fields
- labels MenuLabel[]? - Required. Labels to describe ingredient. Display names should be 140 characters or less, with descriptions 1,000 characters or less. At least one set of labels is required.
googleapis.mybusiness: InsuranceNetwork
A single insurance network. Next id: 5
Fields
- networkId string? - Required. The id of this insurance network generated by Google.
- networkNames record {}? - Output only. A map of network display names in requested languages where the language is the key and localized display name is the value. The display name in English is set by default.
- payerNames record {}? - Output only. A map of payer display names in requested languages where the language is the key and localized display name is the value. The display name in English is set by default.
- state string? - Output only. The state of this insurance network.
googleapis.mybusiness: Invitation
Output only. Represents a pending invitation.
Fields
- name string? - The resource name for the invitation.
- role string? - The invited role on the account.
- targetAccount Account? - An account is a container for your business's locations. If you are the only user who manages locations for your business, you can use your personal Google Account. To share management of locations with multiple users, [create a business account] (https://support.google.com/business/answer/6085339?ref_topic=6085325).
- targetLocation TargetLocation? - Represents a target location for a pending invitation.
googleapis.mybusiness: Item
A single list item. Each variation of an item in the price list should have its own Item with its own price data.
Fields
- itemId string? - Required. ID for the item. Price list, section, and item IDs cannot be duplicated within this Location.
- labels Label[]? - Required. Language-tagged labels for the item. We recommend that item names be 140 characters or less, and descriptions 250 characters or less. At least one set of labels is required.
- price Money? - Represents an amount of money with its currency type.
googleapis.mybusiness: Label
Label to be used when displaying the price list, section, or item.
Fields
- description string? - Optional. Description of the price list, section, or item.
- displayName string? - Required. Display name for the price list, section, or item.
- languageCode string? - Optional. The BCP-47 language code that these strings apply for. Only one set of labels may be set per language.
googleapis.mybusiness: LanguagesSpoken
Languages spoken by at least one staff member.
Fields
- arabicSpoken boolean? - Arabic. At least one staff member speaks Arabic.
- arabicSpokenException string? - Arabic exception.
- cantoneseSpoken boolean? - Cantonese. At least one staff member speaks Cantonese.
- cantoneseSpokenException string? - Cantonese exception.
- dutchSpoken boolean? - Dutch. At least one staff member speaks Dutch.
- dutchSpokenException string? - Dutch exception.
- englishSpoken boolean? - English. At least one staff member speaks English.
- englishSpokenException string? - English exception.
- filipinoSpoken boolean? - Filipino. At least one staff member speaks Filipino.
- filipinoSpokenException string? - Filipino exception.
- frenchSpoken boolean? - French. At least one staff member speaks French.
- frenchSpokenException string? - French exception.
- germanSpoken boolean? - German. At least one staff member speaks German.
- germanSpokenException string? - German exception.
- hindiSpoken boolean? - Hindi. At least one staff member speaks Hindi.
- hindiSpokenException string? - Hindi exception.
- indonesianSpoken boolean? - Indonesian. At least one staff member speaks Indonesian.
- indonesianSpokenException string? - Indonesian exception.
- italianSpoken boolean? - Italian. At least one staff member speaks Italian.
- italianSpokenException string? - Italian exception.
- japaneseSpoken boolean? - Japanese. At least one staff member speaks Japanese.
- japaneseSpokenException string? - Japanese exception.
- koreanSpoken boolean? - Korean. At least one staff member speaks Korean.
- koreanSpokenException string? - Korean exception.
- mandarinSpoken boolean? - Mandarin. At least one staff member speaks Mandarin.
- mandarinSpokenException string? - Mandarin exception.
- portugueseSpoken boolean? - Portuguese. At least one staff member speaks Portuguese.
- portugueseSpokenException string? - Portuguese exception.
- russianSpoken boolean? - Russian. At least one staff member speaks Russian.
- russianSpokenException string? - Russian exception.
- spanishSpoken boolean? - Spanish. At least one staff member speaks Spanish.
- spanishSpokenException string? - Spanish exception.
- vietnameseSpoken boolean? - Vietnamese. At least one staff member speaks Vietnamese.
- vietnameseSpokenException string? - Vietnamese exception.
googleapis.mybusiness: LatLng
An object that represents a latitude/longitude pair. This is expressed as a pair of doubles to represent degrees latitude and degrees longitude. Unless specified otherwise, this must conform to the WGS84 standard. Values must be within normalized ranges.
Fields
- latitude decimal? - The latitude in degrees. It must be in the range [-90.0, +90.0].
- longitude decimal? - The longitude in degrees. It must be in the range [-180.0, +180.0].
googleapis.mybusiness: ListAccountAdminsResponse
Response message for AccessControl.ListAccountAdmins.
Fields
- admins Admin[]? - A collection of Admin instances.
googleapis.mybusiness: ListAccountsResponse
Response message for Accounts.ListAccounts.
Fields
- accounts Account[]? - A collection of accounts to which the user has access. The personal account of the user doing the query will always be the first item of the result, unless it is filtered out.
- nextPageToken string? - If the number of accounts exceeds the requested page size, this field is populated with a token to fetch the next page of accounts on a subsequent call to
accounts.list
. If there are no more accounts, this field is not present in the response.
googleapis.mybusiness: ListAnswersResponse
Response message for QuestionsAndAnswers.ListAnswers
Fields
- answers Answer[]? - The requested answers.
- nextPageToken string? - If the number of answers exceeds the requested max page size, this field is populated with a token to fetch the next page of answers on a subsequent call. If there are no more answers, this field is not present in the response.
- totalSize int? - The total number of answers posted for this question across all pages.
googleapis.mybusiness: ListAttributeMetadataResponse
Response message for Locations.ListAttributeMetadata.
Fields
- attributes AttributeMetadata[]? - A collection of attribute metadata for the available attributes.
- nextPageToken string? - If the number of attributes exceeded the requested page size, this field will be populated with a token to fetch the next page of attributes on a subsequent call to
attributes.list
. If there are no more attributes, this field will not be present in the response.
googleapis.mybusiness: ListBusinessCategoriesResponse
Fields
- categories Category[]? - The categories. Categories are BASIC view. They don't contain any ServiceType information.
- nextPageToken string? - If the number of categories exceeded the requested page size, this field will be populated with a token to fetch the next page of categories on a subsequent call to
ListBusinessCategories
.
- totalCategoryCount int? - The total number of categories for the request parameters.
googleapis.mybusiness: ListCustomerMediaItemsResponse
Response message for Media.ListCustomerMediaItems.
Fields
- mediaItems MediaItem[]? - The returned list of media items.
- nextPageToken string? - If there are more media items than the requested page size, then this field is populated with a token to fetch the next page of media items on a subsequent call to ListCustomerMediaItems.
- totalMediaItemCount int? - The total number of media items for this location, irrespective of pagination. This number is approximate, particularly when there are multiple pages of results.
googleapis.mybusiness: ListInsuranceNetworksResponse
Response message for InsuranceNetworkService.ListInsuranceNetworks
Fields
- networks InsuranceNetwork[]? - A list of insurance networks that are supported by Google.
- nextPageToken string? - If there are more insurance networks than the requested page size, then this field is populated with a token to fetch the next page of insurance networks on a subsequent call to ListInsuranceNetworks.
googleapis.mybusiness: ListInvitationsResponse
Response message for AccessControl.ListInvitations.
Fields
- invitations Invitation[]? - A collection of location invitations that are pending for the account. The number of invitations listed here cannot exceed 1000.
googleapis.mybusiness: ListLocalPostsResponse
Response message for ListLocalPosts
Fields
- localPosts LocalPost[]? - The returned list of local posts.
- nextPageToken string? - If there are more local posts than the requested page size, then this field is populated with a token to fetch the next page of local posts on a subsequent call to
ListLocalPosts
.
googleapis.mybusiness: ListLocationAdminsResponse
Response message for AccessControl.ListLocationAdmins.
Fields
- admins Admin[]? - A collection of Admin instances.
googleapis.mybusiness: ListLocationsResponse
Response message for Locations.ListLocations.
Fields
- locations Location[]? - The locations.
- nextPageToken string? - If the number of locations exceeded the requested page size, this field is populated with a token to fetch the next page of locations on a subsequent call to
ListLocations
. If there are no more locations, this field is not present in the response.
- totalSize int? - The approximate number of Locations in the list irrespective of pagination.
googleapis.mybusiness: ListMediaItemsResponse
Response message for Media.ListMediaItems.
Fields
- mediaItems MediaItem[]? - The returned list of media items.
- nextPageToken string? - If there are more media items than the requested page size, then this field is populated with a token to fetch the next page of media items on a subsequent call to ListMediaItems.
- totalMediaItemCount int? - The total number of media items for this location, irrespective of pagination.
googleapis.mybusiness: ListQuestionsResponse
Response message for QuestionsAndAnswers.ListQuestions
Fields
- nextPageToken string? - If the number of questions exceeds the requested max page size, this field is populated with a token to fetch the next page of questions on a subsequent call. If there are no more questions, this field is not present in the response.
- questions Question[]? - The requested questions,
- totalSize int? - The total number of questions posted for this location across all pages.
googleapis.mybusiness: ListRecommendedGoogleLocationsResponse
Response message for GoogleLocations.ListRecommendedGoogleLocations. It also contains some locations that have been claimed by other GMB users since the last time they were recommended to this GMB account.
Fields
- googleLocations GoogleLocation[]? - The locations recommended to a GMB account. Each of these represents a GoogleLocation that is present on Maps. The locations are sorted in decreasing order of relevance to the GMB account.
- nextPageToken string? - During pagination, if there are more locations available to be fetched in the next page, this field is populated with a token to fetch the next page of locations in a subsequent call. If there are no more locations to be fetched, this field is not present in the response.
- totalSize int? - The total number of recommended locations for this GMB account, irrespective of pagination.
googleapis.mybusiness: ListReviewsResponse
Response message for Reviews.ListReviews.
Fields
- averageRating decimal? - The average star rating of all reviews for this location on a scale of 1 to 5, where 5 is the highest rating.
- nextPageToken string? - If the number of reviews exceeded the requested page size, this field is populated with a token to fetch the next page of reviews on a subsequent call to ListReviews. If there are no more reviews, this field is not present in the response.
- reviews Review[]? - The reviews.
- totalReviewCount int? - The total number of reviews for this location.
googleapis.mybusiness: ListVerificationsResponse
Response message for Verifications.ListVerifications.
Fields
- nextPageToken string? - If the number of verifications exceeded the requested page size, this field will be populated with a token to fetch the next page of verification on a subsequent call. If there are no more attributes, this field will not be present in the response.
- verifications Verification[]? - List of the verifications.
googleapis.mybusiness: LivingArea
An individual room, such as kitchen, bathroom, bedroom, within a bookable guest unit.
Fields
- accessibility LivingAreaAccessibility? - Accessibility features of the living area.
- eating LivingAreaEating? - Information about eating features in the living area.
- features LivingAreaFeatures? - Features in the living area.
- layout LivingAreaLayout? - Information about the layout of the living area.
- sleeping LivingAreaSleeping? - Information about sleeping features in the living area.
googleapis.mybusiness: LivingAreaAccessibility
Accessibility features of the living area.
Fields
- adaCompliantUnit boolean? - ADA compliant unit. A guestroom designed to accommodate the physical challenges of a guest with mobility and/or auditory and/or visual issues, as determined by legislative policy. Usually features enlarged doorways, roll-in showers with seats, bathroom grab bars, and communication equipment for the hearing and sight challenged.
- adaCompliantUnitException string? - ADA compliant unit exception.
- hearingAccessibleDoorbell boolean? - Hearing-accessible doorbell. A visual indicator(s) of a knock or ring at the door.
- hearingAccessibleDoorbellException string? - Hearing-accessible doorbell exception.
- hearingAccessibleFireAlarm boolean? - Hearing-accessible fire alarm. A device that gives warning of a fire through flashing lights.
- hearingAccessibleFireAlarmException string? - Hearing-accessible fire alarm exception.
- hearingAccessibleUnit boolean? - Hearing-accessible unit. A guestroom designed to accommodate the physical challenges of a guest with auditory issues.
- hearingAccessibleUnitException string? - Hearing-accessible unit exception.
- mobilityAccessibleBathtub boolean? - Mobility-accessible bathtub. A bathtub that accomodates the physically challenged with additional railings or hand grips, a transfer seat or lift, and/or a door to enable walking into the tub.
- mobilityAccessibleBathtubException string? - Mobility-accessible bathtub exception.
- mobilityAccessibleShower boolean? - Mobility-accessible shower. A shower with an enlarged door or access point to accommodate a wheelchair or a waterproof seat for the physically challenged.
- mobilityAccessibleShowerException string? - Mobility-accessible shower exception.
- mobilityAccessibleToilet boolean? - Mobility-accessible toilet. A toilet with a higher seat, grab bars, and/or a larger area around it to accommodate the physically challenged.
- mobilityAccessibleToiletException string? - Mobility-accessible toilet exception.
- mobilityAccessibleUnit boolean? - Mobility-accessible unit. A guestroom designed to accommodate the physical challenges of a guest with mobility and/or auditory and/or visual issues. Usually features enlarged doorways, roll-in showers with seats, bathroom grab bars, and communication equipment for the hearing and sight challenged.
- mobilityAccessibleUnitException string? - Mobility-accessible unit exception.
googleapis.mybusiness: LivingAreaEating
Information about eating features in the living area.
Fields
- coffeeMaker boolean? - Coffee maker. An electric appliance that brews coffee by heating and forcing water through ground coffee.
- coffeeMakerException string? - Coffee maker exception.
- cookware boolean? - Cookware. Kitchen pots, pans and utensils used in connection with the preparation of food.
- cookwareException string? - Cookware exception.
- dishwasher boolean? - Dishwasher. A counter-height electrical cabinet containing racks for dirty dishware, cookware and cutlery, and a dispenser for soap built into the pull-down door. The cabinet is attached to the plumbing system to facilitate the automatic cleaning of its contents.
- dishwasherException string? - Dishwasher exception.
- indoorGrill boolean? - Indoor grill. Metal grates built into an indoor cooktop on which food is cooked over an open flame or electric heat source.
- indoorGrillException string? - Indoor grill exception.
- kettle boolean? - Kettle. A covered container with a handle and a spout used for boiling water.
- kettleException string? - Kettle exception.
- kitchenAvailable boolean? - Kitchen available. An area of the guestroom designated for the preparation and storage of food via the presence of a refrigerator, cook top, oven and sink, as well as cutlery, dishes and cookware. Usually includes small appliances such a coffee maker and a microwave. May or may not include an automatic dishwasher.
- kitchenAvailableException string? - Kitchen available exception.
- microwave boolean? - Microwave. An electric oven that quickly cooks and heats food by microwave energy. Smaller than a standing or wall mounted oven. Usually placed on a kitchen counter, a shelf or tabletop or mounted above a cooktop.
- microwaveException string? - Microwave exception.
- minibar boolean? - Minibar. A small refrigerated cabinet in the guestroom containing bottles/cans of soft drinks, mini bottles of alcohol, and snacks. The items are most commonly available for a fee.
- minibarException string? - Minibar exception.
- outdoorGrill boolean? - Outdoor grill. Metal grates on which food is cooked over an open flame or electric heat source. Part of an outdoor apparatus that supports the grates. Also known as barbecue grill or barbecue.
- outdoorGrillException string? - Outdoor grill exception.
- oven boolean? - Oven. A temperature controlled, heated metal cabinet powered by gas or electricity in which food is placed for the purpose of cooking or reheating.
- ovenException string? - Oven exception.
- refrigerator boolean? - Refrigerator. A large, climate-controlled electrical cabinet with vertical doors. Built for the purpose of chilling and storing perishable foods.
- refrigeratorException string? - Refrigerator exception.
- sink boolean? - Sink. A basin with a faucet attached to a water source and used for the purpose of washing and rinsing.
- sinkException string? - Sink exception.
- snackbar boolean? - Snackbar. A small cabinet in the guestroom containing snacks. The items are most commonly available for a fee.
- snackbarException string? - Snackbar exception.
- stove boolean? - Stove. A kitchen appliance powered by gas or electricity for the purpose of creating a flame or hot surface on which pots of food can be cooked. Also known as cooktop or hob.
- stoveException string? - Stove exception.
- teaStation boolean? - Tea station. A small area with the supplies needed to heat water and make tea.
- teaStationException string? - Tea station exception.
- toaster boolean? - Toaster. A small, temperature controlled electric appliance with rectangular slots at the top that are lined with heated coils for the purpose of browning slices of bread products.
- toasterException string? - Toaster exception.
googleapis.mybusiness: LivingAreaFeatures
Features in the living area.
Fields
- airConditioning boolean? - Air conditioning. An electrical machine used to cool the temperature of the guestroom.
- airConditioningException string? - Air conditioning exception.
- bathtub boolean? - Bathtub. A fixed plumbing feature set on the floor and consisting of a large container that accommodates the body of an adult for the purpose of seated bathing. Includes knobs or fixtures to control the temperature of the water, a faucet through which the water flows, and a drain that can be closed for filling and opened for draining.
- bathtubException string? - Bathtub exception.
- bidet boolean? - Bidet. A plumbing fixture attached to a toilet or a low, fixed sink designed for the purpose of washing after toilet use.
- bidetException string? - Bidet exception.
- dryer boolean? - Dryer. An electrical machine designed to dry clothing.
- dryerException string? - Dryer exception.
- electronicRoomKey boolean? - Electronic room key. A card coded by the check-in computer that is read by the lock on the hotel guestroom door to allow for entry.
- electronicRoomKeyException string? - Electronic room key exception.
- fireplace boolean? - Fireplace. A framed opening (aka hearth) at the base of a chimney in which logs or an electrical fire feature are burned to provide a relaxing ambiance or to heat the room. Often made of bricks or stone.
- fireplaceException string? - Fireplace exception.
- hairdryer boolean? - Hairdryer. A handheld electric appliance that blows temperature-controlled air for the purpose of drying wet hair. Can be mounted to a bathroom wall or a freestanding device stored in the guestroom's bathroom or closet.
- hairdryerException string? - Hairdryer exception.
- heating boolean? - Heating. An electrical machine used to warm the temperature of the guestroom.
- heatingException string? - Heating exception.
- inunitSafe boolean? - In-unit safe. A strong fireproof cabinet with a programmable lock, used for the protected storage of valuables in a guestroom. Often built into a closet.
- inunitSafeException string? - In-unit safe exception.
- inunitWifiAvailable boolean? - In-unit Wifi available. Guests can wirelessly connect to the Internet in the guestroom. Can be free or for a fee.
- inunitWifiAvailableException string? - In-unit Wifi available exception.
- ironingEquipment boolean? - Ironing equipment. A device, usually with a flat metal base, that is heated to smooth, finish, or press clothes and a flat, padded, cloth-covered surface on which the clothes are worked.
- ironingEquipmentException string? - Ironing equipment exception.
- payPerViewMovies boolean? - Pay per view movies. Televisions with channels that offer films that can be viewed for a fee, and have an interface to allow the viewer to accept the terms and approve payment.
- payPerViewMoviesException string? - Pay per view movies exception.
- privateBathroom boolean? - Private bathroom. A bathroom designated for the express use of the guests staying in a specific guestroom.
- privateBathroomException string? - Private bathroom exception.
- shower boolean? - Shower. A fixed plumbing fixture for standing bathing that features a tall spray spout or faucet through which water flows, a knob or knobs that control the water's temperature, and a drain in the floor.
- showerException string? - Shower exception.
- toilet boolean? - Toilet. A fixed bathroom feature connected to a sewer or septic system and consisting of a water-flushed bowl with a seat, as well as a device that elicites the water-flushing action. Used for the process and disposal of human waste.
- toiletException string? - Toilet exception.
- tv boolean? - TV. A television is available in the guestroom.
- tvCasting boolean? - TV casting. A television equipped with a device through which the video entertainment accessed on a personal computer, phone or tablet can be wirelessly delivered to and viewed on the guestroom's television.
- tvCastingException string? - TV exception.
- tvException string? - TV exception.
- tvStreaming boolean? - TV streaming. Televisions that embed a range of web-based apps to allow for watching media from those apps.
- tvStreamingException string? - TV streaming exception.
- universalPowerAdapters boolean? - Universal power adapters. A power supply for electronic devices which plugs into a wall for the purpose of converting AC to a single DC voltage. Also know as AC adapter or charger.
- universalPowerAdaptersException string? - Universal power adapters exception.
- washer boolean? - Washer. An electrical machine connected to a running water source designed to launder clothing.
- washerException string? - Washer exception.
googleapis.mybusiness: LivingAreaLayout
Information about the layout of the living area.
Fields
- balcony boolean? - Balcony. An outdoor platform attached to a building and surrounded by a short wall, fence or other safety railing. The balcony is accessed through a door in a guestroom or suite and is for use by the guest staying in that room. May or may not include seating or outdoor furniture. Is not located on the ground floor. Also lanai.
- balconyException string? - Balcony exception.
- livingAreaSqMeters float? - Living area sq meters. The measurement in meters of the area of a guestroom's living space.
- livingAreaSqMetersException string? - Living area sq meters exception.
- loft boolean? - Loft. A three-walled upper area accessed by stairs or a ladder that overlooks the lower area of a room.
- loftException string? - Loft exception.
- nonSmoking boolean? - Non smoking. A guestroom in which the smoking of cigarettes, cigars and pipes is prohibited.
- nonSmokingException string? - Non smoking exception.
- patio boolean? - Patio. A paved, outdoor area with seating attached to and accessed through a ground-floor guestroom for use by the occupants of the guestroom.
- patioException string? - Patio exception.
- stairs boolean? - Stairs. There are steps leading from one level or story to another in the unit.
- stairsException string? - Stairs exception.
googleapis.mybusiness: LivingAreaSleeping
Information about sleeping features in the living area.
Fields
- bedsCount int? - Beds count. The number of permanent beds present in a guestroom. Does not include rollaway beds, cribs or sofabeds.
- bedsCountException string? - Beds count exception.
- bunkBedsCount int? - Bunk beds count. The number of furniture pieces in which one framed mattress is fixed directly above another by means of a physical frame. This allows one person(s) to sleep in the bottom bunk and one person(s) to sleep in the top bunk. Also known as double decker bed.
- bunkBedsCountException string? - Bunk beds count exception.
- cribsCount int? - Cribs count. The number of small beds for an infant or toddler that the guestroom can obtain. The bed is surrounded by a high railing to prevent the child from falling or climbing out of the bed
- cribsCountException string? - Cribs count exception.
- doubleBedsCount int? - Double beds count. The number of medium beds measuring 53"W x 75"L (135cm x 191cm). Also known as full size bed.
- doubleBedsCountException string? - Double beds count exception.
- featherPillows boolean? - Feather pillows. The option for guests to obtain bed pillows that are stuffed with the feathers and down of ducks or geese.
- featherPillowsException string? - Feather pillows exception.
- hypoallergenicBedding boolean? - Hypoallergenic bedding. Bedding such as linens, pillows, mattress covers and/or mattresses that are made of materials known to be resistant to allergens such as mold, dust and dander.
- hypoallergenicBeddingException string? - Hypoallergenic bedding exception.
- kingBedsCount int? - King beds count. The number of large beds measuring 76"W x 80"L (193cm x 102cm). Most often meant to accompany two people. Includes California king and super king.
- kingBedsCountException string? - King beds count exception.
- memoryFoamPillows boolean? - Memory foam pillows. The option for guests to obtain bed pillows that are stuffed with a man-made foam that responds to body heat by conforming to the body closely, and then recovers its shape when the pillow cools down.
- memoryFoamPillowsException string? - Memory foam pillows exception.
- otherBedsCount int? - Other beds count. The number of beds that are not standard mattress and boxspring setups such as Japanese tatami mats, trundle beds, air mattresses and cots.
- otherBedsCountException string? - Other beds count exception.
- queenBedsCount int? - Queen beds count. The number of medium-large beds measuring 60"W x 80"L (152cm x 102cm).
- queenBedsCountException string? - Queen beds count exception.
- rollAwayBedsCount int? - Roll away beds count. The number of mattresses on wheeled frames that can be folded in half and rolled away for easy storage that the guestroom can obtain upon request.
- rollAwayBedsCountException string? - Roll away beds count exception.
- singleOrTwinBedsCount int? - Single or twin count beds. The number of smaller beds measuring 38"W x 75"L (97cm x 191cm) that can accommodate one adult.
- singleOrTwinBedsCountException string? - Single or twin beds count exception.
- sofaBedsCount int? - Sofa beds count. The number of specially designed sofas that can be made to serve as a bed by lowering its hinged upholstered back to horizontal position or by pulling out a concealed mattress.
- sofaBedsCountException string? - Sofa beds count exception.
- syntheticPillows boolean? - Synthetic pillows. The option for guests to obtain bed pillows stuffed with polyester material crafted to reproduce the feel of a pillow stuffed with down and feathers.
- syntheticPillowsException string? - Synthetic pillows exception.
googleapis.mybusiness: LocalPost
Represents a local post for a location.
Fields
- alertType string? - The type of alert the post is created for. This field is only applicable for posts of topic_type Alert, and behaves as a sub-type of Alerts.
- callToAction CallToAction? - An action that is performed when the user clicks through the post
- createTime string? - Output only. Time of the creation of the post.
- event LocalPostEvent? - All the information pertaining to an event featured in a local post.
- languageCode string? - The language of the local post.
- media MediaItem[]? - The media associated with the post. source_url is the only supported data field for a LocalPost MediaItem.
- name string? - Output only. Google identifier for this local post in the form:
accounts/{account_id}/locations/{location_id}/localPosts/{local_post_id}
- offer LocalPostOffer? - Specific fields for offer posts.
- searchUrl string? - Output only. The link to the local post in Google search. This link can be used to share the post via social media, email, text, etc.
- state string? - Output only. The state of the post, indicating what part of its lifecycle it is in.
- summary string? - Description/body of the local post.
- topicType string? - Required. The topic type of the post: standard, event, offer, or alert.
- updateTime string? - Output only. Time of the last modification of the post made by the user.
googleapis.mybusiness: LocalPostEvent
All the information pertaining to an event featured in a local post.
Fields
- schedule TimeInterval? - An interval of time, inclusive. It must contain all fields to be valid.
- title string? - Name of the event.
googleapis.mybusiness: LocalPostMetrics
All the metrics requested for a Local Post.
Fields
- localPostName string? - Local post name
- metricValues MetricValue[]? - A list of values for the requested metrics.
googleapis.mybusiness: LocalPostOffer
Specific fields for offer posts.
Fields
- couponCode string? - Optional. Offer code that is usable in store or online.
- redeemOnlineUrl string? - Optional. Online link to redeem offer.
- termsConditions string? - Optional. Offer terms and conditions.
googleapis.mybusiness: Location
A location. See the [help center article] (https://support.google.com/business/answer/3038177) for a detailed description of these fields, or the category endpoint for a list of valid business categories.
Fields
- adWordsLocationExtensions AdWordsLocationExtensions? - Additional information that is surfaced in AdWords.
- additionalCategories Category[]? - Additional categories to describe your business. Categories help your customers find accurate, specific results for services they're interested in. To keep your business information accurate and live, make sure that you use as few categories as possible to describe your overall core business. Choose categories that are as specific as possible, but representative of your main business.
- additionalPhones string[]? - Up to two phone numbers (mobile or landline, no fax) at which your business can be called, in addition to your primary phone number.
- address PostalAddress? - Represents a postal address, e.g. for postal delivery or payments addresses. Given a postal address, a postal service can deliver items to a premise, P.O. Box or similar. It is not intended to model geographical locations (roads, towns, mountains). In typical usage an address would be created via user input or from importing existing data, depending on the type of process. Advice on address input / editing: - Use an i18n-ready address widget such as https://github.com/google/libaddressinput) - Users should not be presented with UI elements for input or editing of fields outside countries where that field is used. For more guidance on how to use this schema, please see: https://support.google.com/business/answer/6397478
- attributes Attribute[]? - Attributes for this location.
- labels string[]? - A collection of free-form strings to allow you to tag your business. These labels are NOT user facing; only you can see them. Limited to 255 characters (per label).
- languageCode string? - The language of the location. Set during creation and not updateable.
- latlng LatLng? - An object that represents a latitude/longitude pair. This is expressed as a pair of doubles to represent degrees latitude and degrees longitude. Unless specified otherwise, this must conform to the WGS84 standard. Values must be within normalized ranges.
- locationKey LocationKey? - Alternate/surrogate key references for a location.
- locationName string? - Location name should reflect your business's real-world name, as used consistently on your storefront, website, and stationery, and as known to customers. Any additional information, when relevant, can be included in other fields of the resource (for example,
Address
,Categories
). Don't add unnecessary information to your name (for example, prefer "Google" over "Google Inc. - Mountain View Corporate Headquarters"). Don't include marketing taglines, store codes, special characters, hours or closed/open status, phone numbers, website URLs, service/product information, location/address or directions, or containment information (for example, "Chase ATM in Duane Reade").
- locationState LocationState? - Contains a set of booleans that reflect the state of a Location.
- metadata Metadata? - Additional non-user-editable information about the location.
- moreHours MoreHours[]? - More hours for a business's different departments or specific customers.
- name string? - Google identifier for this location in the form:
accounts/{account_id}/locations/{location_id}
In the context of matches, this field will not be populated.
- openInfo OpenInfo? - Information related to the opening state of the business.
- priceLists PriceList[]? - Price list information for this location.
- primaryCategory Category? - A category describing what this business is (not what it does). For a list of valid category IDs, and the mappings to their human-readable names, see categories.list.
- primaryPhone string? - A phone number that connects to your individual business location as directly as possible. Use a local phone number instead of a central, call center helpline number whenever possible.
- profile Profile? - All information pertaining to the location's profile.
- regularHours BusinessHours? - Represents the time periods that this location is open for business. Holds a collection of TimePeriod instances.
- relationshipData RelationshipData? - Information of all parent and children locations related to this one.
- serviceArea ServiceAreaBusiness? - Service area businesses provide their service at the customer's location (for example, a locksmith or plumber).
- specialHours SpecialHours? - Represents a set of time periods when a location's operational hours differ from its normal business hours.
- storeCode string? - External identifier for this location, which must be unique inside a given account. This is a means of associating the location with your own records.
- websiteUrl string? - A URL for this business. If possible, use a URL that represents this individual business location instead of a generic website/URL that represents all locations, or the brand.
googleapis.mybusiness: LocationAssociation
How the media item is associated with its location.
Fields
- category string? - The category that this location photo belongs to.
- priceListItemId string? - The ID of a price list item that this location photo is associated with.
googleapis.mybusiness: LocationDrivingDirectionMetrics
A location indexed with the regions that people usually come from. This is captured by counting how many driving-direction requests to this location are from each region.
Fields
- locationName string? - The location resource name this metric value belongs to.
- timeZone string? - Time zone (IANA timezone IDs, for example, 'Europe/London') of the location.
- topDirectionSources TopDirectionSources[]? - Driving-direction requests by source region. By convention, these are sorted by count with at most 10 results.
googleapis.mybusiness: LocationKey
Alternate/surrogate key references for a location.
Fields
- explicitNoPlaceId boolean? - Output only. A value of true indicates that an unset place ID is deliberate, which is different from no association being made yet.
- placeId string? - If this location has been verified and is connected to/appears on Google Maps, this field is populated with the place ID for the location. This ID can be used in various Places APIs. If this location is unverified, this field may be populated if the location has been associated with a place that appears on Google Maps. This field can be set during Create calls, but not for Update. The additional
explicit_no_place_id
bool qualifies whether an unset place ID is deliberate or not.
- plusPageId string? - Output only. If this location has a Google+ page associated with it, this is populated with the Google+ page ID for this location.
- requestId string? - Output only. The
request_id
used to create this location. May be empty if this location was created outside of the GMB API or Google My Business Locations.
googleapis.mybusiness: LocationMetrics
A series of Metrics and BreakdownMetrics associated with a Location over some time range.
Fields
- locationName string? - The location resource name these values belong to.
- metricValues MetricValue[]? - A list of values for the requested metrics.
- timeZone string? - IANA timezone for the location.
googleapis.mybusiness: LocationReview
Represents a review with location information.
Fields
- name string? - Location resource name.
- review Review? - Output only. Represents a review for a location.
googleapis.mybusiness: LocationState
Contains a set of booleans that reflect the state of a Location.
Fields
- canDelete boolean? - Output only. Indicates whether the location can be deleted using the Google My Business API.
- canHaveFoodMenus boolean? - Output only. Indicates if the listing is eligible for food menu.
- canModifyServiceList boolean? - Output only. Indicates if the listing can modify a ServiceList.
- canOperateHealthData boolean? - Output only. Indicates whether the location can operate on Health data.
- canOperateLodgingData boolean? - Output only. Indicates whether the location can operate on Lodging data.
- canUpdate boolean? - Output only. Indicates whether the location can be updated.
- hasPendingEdits boolean? - Output only. Indicates whether any of this Location's properties are in the edit pending state.
- hasPendingVerification boolean? - Output only. Indicates whether the location has pending verification requests.
- isDisabled boolean? - Output only. Indicates whether the location is disabled.
- isDisconnected boolean? - Output only. Indicates whether the location is disconnected from a place on Google Maps.
- isDuplicate boolean? - Output only. Indicates whether the location is a duplicate of another location.
- isGoogleUpdated boolean? - Output only. Indicates whether the place ID associated with this location has updates.
- isLocalPostApiDisabled boolean? - Output only. Indicates whether accounts.locations.localPosts is disabled for this location.
- isPendingReview boolean? - Output only. Indicates whether the review of the location is pending.
- isPublished boolean? - Output only. Indicates whether the location is published.
- isSuspended boolean? - Output only. Indicates whether the location is suspended. Suspended locations are not visible to end users in Google products. If you believe this was a mistake, see the [help center article] (https://support.google.com/business/answer/4569145).
- isVerified boolean? - Output only. Indicates whether the location is verified.
- needsReverification boolean? - Output only. Indicates whether the location requires reverification.
googleapis.mybusiness: Lodging
Lodging of a location that provides accomodations.
Fields
- accessibility Accessibility? - Physical adaptations made to the property in consideration of varying levels of human physical ability.
- activities Activities? - Amenities and features related to leisure and play.
- allUnits GuestUnitFeatures? - Features and available amenities in the guest unit.
- business Business? - Features of the property of specific interest to the business traveler.
- commonLivingArea LivingArea? - An individual room, such as kitchen, bathroom, bedroom, within a bookable guest unit.
- connectivity Connectivity? - The ways in which the property provides guests with the ability to access the internet.
- families Families? - Services and amenities for families and young guests.
- foodAndDrink FoodAndDrink? - Meals, snacks, and beverages available at the property.
- guestUnits GuestUnitType[]? - Individual GuestUnitTypes that are available in this Lodging.
- healthAndSafety HealthAndSafety? - Health and safety measures implemented by the hotel during COVID-19.
- housekeeping Housekeeping? - Conveniences provided in guest units to facilitate an easier, more comfortable stay.
- metadata LodgingMetadata? - Metadata for the Lodging.
- name string? - Required. Google identifier for this location in the form:
accounts/{account_id}/locations/{location_id}/lodging
- parking Parking? - Parking options at the property.
- pets Pets? - Policies regarding guest-owned animals.
- policies Policies? - Property rules that impact guests.
- pools Pools? - Swimming pool or recreational water facilities available at the hotel.
- property Property? - General factual information about the property's physical structure and important dates.
- services Services? - Conveniences or help provided by the property to facilitate an easier, more comfortable stay.
- someUnits GuestUnitFeatures? - Features and available amenities in the guest unit.
- transportation Transportation? - Vehicles or vehicular services facilitated or owned by the property.
- wellness Wellness? - Guest facilities at the property to promote or maintain health, beauty, and fitness.
googleapis.mybusiness: LodgingMetadata
Metadata for the Lodging.
Fields
- updateTime string? - Required. The latest time at which the Lodging data is asserted to be true in the real world. This is not necessarily the time at which the request is made.
googleapis.mybusiness: MatchedLocation
Represents a possible match to a location.
Fields
- isExactMatch boolean? - Is this an exact match?
- location Location? - A location. See the [help center article] (https://support.google.com/business/answer/3038177) for a detailed description of these fields, or the category endpoint for a list of valid business categories.
googleapis.mybusiness: MediaInsights
Insights and statistics for the media item.
Fields
- viewCount string? - Output only. The number of times the media item has been viewed.
googleapis.mybusiness: MediaItem
A single media item.
Fields
- attribution Attribution? - Attribution information for customer media items, such as the contributor's name and profile picture.
- createTime string? - Output only. Creation time of this media item.
- dataRef MediaItemDataRef? - Reference to the photo binary data of a
MediaItem
uploaded through the My Business API. Create a data ref using StartUploadMediaItemData, and use this ref when uploading bytes to [UpdateMedia] and subsequently calling CreateMediaItem.
- description string? - Description for this media item. Descriptions cannot be modified through the My Business API, but can be set when creating a new media item that is not a cover photo.
- dimensions Dimensions? - Dimensions of the media item.
- googleUrl string? - Output only. Google-hosted URL for this media item. This URL is not static since it may change over time. For video this will be a preview image with an overlaid play icon.
- insights MediaInsights? - Insights and statistics for the media item.
- locationAssociation LocationAssociation? - How the media item is associated with its location.
- mediaFormat string? - The format of this media item. Must be set when the media item is created, and is read-only on all other requests. Cannot be updated.
- name string? - The resource name for this media item.
accounts/{account_id}/locations/{location_id}/media/{media_key}
- sourceUrl string? - A publicly accessible URL where the media item can be retrieved from. When creating one of this or data_ref must be set to specify the source of the media item. If
source_url
was used when creating a media item, it will be populated with that source URL when the media item is retrieved. This field cannot be updated.
- thumbnailUrl string? - Output only. Where provided, the URL of a thumbnail image for this media item.
googleapis.mybusiness: MediaItemDataRef
Reference to the photo binary data of a MediaItem
uploaded through the My Business API. Create a data ref using StartUploadMediaItemData, and use this ref when uploading bytes to [UpdateMedia] and subsequently calling CreateMediaItem.
Fields
- resourceName string? - The unique ID for this media item's binary data. Used to upload the photo data with [UpdateMedia] and when creating a new media item from those bytes with CreateMediaItem. Example of uploading bytes:
curl -X POST -T{path_to_file} "http://mybusiness.googleapis.com/upload/v1/media/{resource_name}?upload_type=media"
For CreateMediaItem calls, set this as theMediaItem
data_ref
.
googleapis.mybusiness: MenuLabel
Label to be used when displaying the menu and its various sub-components.
Fields
- description string? - Optional. Supplementary information of the component.
- displayName string? - Required. Display name of the component.
- languageCode string? - Optional. The BCP 47 code of language. If the language is not available, it will default to English.
googleapis.mybusiness: Metadata
Additional non-user-editable information about the location.
Fields
- duplicate Duplicate? - Information about the location that this location duplicates.
- mapsUrl string? - A link to the location on Maps.
- newReviewUrl string? - A link to the page on Google Search where a customer can leave a review for the location.
googleapis.mybusiness: MetricRequest
A request to return values for one metric and the options for how those values should be returned.
Fields
- metric string? - The requested metric.
- options string[]? - How the values should appear when returned.
googleapis.mybusiness: MetricValue
A value for a single Metric from a starting time.
Fields
- dimensionalValues DimensionalMetricValue[]? - Dimensional values for this metric.
- metric string? - The metric for which the value applies.
- totalValue DimensionalMetricValue? - A value for a single metric with a given time dimension.
googleapis.mybusiness: MinimizedContact
Minimized contact measures implemented by the hotel during COVID-19.
Fields
- contactlessCheckinCheckout boolean? - No-contact check-in and check-out.
- contactlessCheckinCheckoutException string? - Contactless check-in check-out exception.
- digitalGuestRoomKeys boolean? - Keyless mobile entry to guest rooms.
- digitalGuestRoomKeysException string? - Digital guest room keys exception.
- housekeepingScheduledRequestOnly boolean? - Housekeeping scheduled by request only.
- housekeepingScheduledRequestOnlyException string? - Housekeeping scheduled request only exception.
- noHighTouchItemsCommonAreas boolean? - High-touch items, such as magazines, removed from common areas.
- noHighTouchItemsCommonAreasException string? - No high touch items common areas exception.
- noHighTouchItemsGuestRooms boolean? - High-touch items, such as decorative pillows, removed from guest rooms.
- noHighTouchItemsGuestRoomsException string? - No high touch items guest rooms exception.
- plasticKeycardsDisinfected boolean? - Plastic key cards are disinfected or discarded.
- plasticKeycardsDisinfectedException string? - Plastic keycards disinfected exception.
- roomBookingsBuffer boolean? - Buffer maintained between room bookings.
- roomBookingsBufferException string? - Room bookings buffer exception.
googleapis.mybusiness: Money
Represents an amount of money with its currency type.
Fields
- currencyCode string? - The three-letter currency code defined in ISO 4217.
- nanos int? - Number of nano (10^-9) units of the amount. The value must be between -999,999,999 and +999,999,999 inclusive. If
units
is positive,nanos
must be positive or zero. Ifunits
is zero,nanos
can be positive, zero, or negative. Ifunits
is negative,nanos
must be negative or zero. For example $-1.75 is represented asunits
=-1 andnanos
=-750,000,000.
- units string? - The whole units of the amount. For example if
currencyCode
is"USD"
, then 1 unit is one US dollar.
googleapis.mybusiness: MoreHours
The time periods during which a location is open for certain types of business.
Fields
- hoursTypeId string? - Required. Type of hours. Clients should call {#link businessCategories:BatchGet} to get supported hours types for categories of their locations.
- periods TimePeriod[]? - Required. A collection of times that this location is open. Each period represents a range of hours when the location is open during the week.
googleapis.mybusiness: MoreHoursType
More hours types that a business can offers, in addition to its regular hours.
Fields
- displayName string? - Output only. The human-readable English display name for the hours type.
- hoursTypeId string? - Output only. A stable ID provided by Google for this hours type.
- localizedDisplayName string? - Output only. The human-readable localized display name for the hours type.
googleapis.mybusiness: Notifications
A Google Cloud Pub/Sub topic where notifications can be published when a location is updated or has a new review. There will be only one notification settings resource per-account.
Fields
- name string? - Output only. The notifications resource name.
- notificationTypes string[]? - The types of notifications that will be sent to the Cloud Pub/Sub topic. At least one must be specified. To stop receiving notifications entirely, use DeleteNotifications.
- topicName string? - The Google Cloud Pub/Sub topic that will receive notifications when locations managed by this account are updated. If unset, no notifications will be posted. The account mybusiness-api-pubsub@system.gserviceaccount.com must have at least Publish permissions on the Cloud Pub/Sub topic.
googleapis.mybusiness: NutritionFact
This message denotes nutrition information with an upper bound and lower bound range and can be represented by mass unit. Lower amount must be specified. Both lower and upper amounts are non-negative numbers.
Fields
- lowerAmount decimal? - Required. Lower amount of nutrition
- unit string? - Required. Unit of the given nutrition information.
- upperAmount decimal? - Optional. Upper amount of nutrition
googleapis.mybusiness: NutritionFacts
This message represents nutrition facts for a food dish.
Fields
- calories CaloriesFact? - This message denotes calories information with an upper bound and lower bound range. Lower amount must be specified. Both lower and upper amounts are non-negative numbers.
- cholesterol NutritionFact? - This message denotes nutrition information with an upper bound and lower bound range and can be represented by mass unit. Lower amount must be specified. Both lower and upper amounts are non-negative numbers.
- protein NutritionFact? - This message denotes nutrition information with an upper bound and lower bound range and can be represented by mass unit. Lower amount must be specified. Both lower and upper amounts are non-negative numbers.
- sodium NutritionFact? - This message denotes nutrition information with an upper bound and lower bound range and can be represented by mass unit. Lower amount must be specified. Both lower and upper amounts are non-negative numbers.
- totalCarbohydrate NutritionFact? - This message denotes nutrition information with an upper bound and lower bound range and can be represented by mass unit. Lower amount must be specified. Both lower and upper amounts are non-negative numbers.
- totalFat NutritionFact? - This message denotes nutrition information with an upper bound and lower bound range and can be represented by mass unit. Lower amount must be specified. Both lower and upper amounts are non-negative numbers.
googleapis.mybusiness: OpenInfo
Information related to the opening state of the business.
Fields
- canReopen boolean? - Output only. Indicates whether this business is eligible for re-open.
- openingDate Date? - Represents a whole or partial calendar date, such as a birthday. The time of day and time zone are either specified elsewhere or are insignificant. The date is relative to the Gregorian Calendar. This can represent one of the following: * A full date, with non-zero year, month, and day values * A month and day value, with a zero year, such as an anniversary * A year on its own, with zero month and day values * A year and month value, with a zero day, such as a credit card expiration date Related types are google.type.TimeOfDay and
google.protobuf.Timestamp
.
- status string? - Indicates whether or not the Location is currently open for business. All locations are open by default, unless updated to be closed.
googleapis.mybusiness: OrganizationInfo
Additional Info stored for an organization.
Fields
- phoneNumber string? - The contact number for the organization.
- postalAddress PostalAddress? - Represents a postal address, e.g. for postal delivery or payments addresses. Given a postal address, a postal service can deliver items to a premise, P.O. Box or similar. It is not intended to model geographical locations (roads, towns, mountains). In typical usage an address would be created via user input or from importing existing data, depending on the type of process. Advice on address input / editing: - Use an i18n-ready address widget such as https://github.com/google/libaddressinput) - Users should not be presented with UI elements for input or editing of fields outside countries where that field is used. For more guidance on how to use this schema, please see: https://support.google.com/business/answer/6397478
- registeredDomain string? - The registered domain for the account.
googleapis.mybusiness: Parking
Parking options at the property.
Fields
- electricCarChargingStations boolean? - Electric car charging stations. Electric power stations, usually located outdoors, into which guests plug their electric cars to receive a charge.
- electricCarChargingStationsException string? - Electric car charging stations exception.
- freeParking boolean? - Free parking. The hotel allows the cars of guests to be parked for free. Parking facility may be an outdoor lot or an indoor garage, but must be onsite. Nearby parking does not apply. Parking may be performed by the guest or by hotel staff. Free parking must be available to all guests (limited conditions does not apply).
- freeParkingException string? - Free parking exception.
- freeSelfParking boolean? - Free self parking. Guests park their own cars for free. Parking facility may be an outdoor lot or an indoor garage, but must be onsite. Nearby parking does not apply.
- freeSelfParkingException string? - Free self parking exception.
- freeValetParking boolean? - Free valet parking. Hotel staff member parks the cars of guests. Parking with this service is free.
- freeValetParkingException string? - Free valet parking exception.
- parkingAvailable boolean? - Parking available. The hotel allows the cars of guests to be parked. Can be free or for a fee. Parking facility may be an outdoor lot or an indoor garage, but must be onsite. Nearby parking does not apply. Parking may be performed by the guest or by hotel staff.
- parkingAvailableException string? - Parking available exception.
- selfParkingAvailable boolean? - Self parking available. Guests park their own cars. Parking facility may be an outdoor lot or an indoor garage, but must be onsite. Nearby parking does not apply. Can be free or for a fee.
- selfParkingAvailableException string? - Self parking available exception.
- valetParkingAvailable boolean? - Valet parking available. Hotel staff member parks the cars of guests. Parking with this service can be free or for a fee.
- valetParkingAvailableException string? - Valet parking available exception.
googleapis.mybusiness: PaymentOptions
Forms of payment accepted at the property.
Fields
- cash boolean? - Cash. The hotel accepts payment by paper/coin currency.
- cashException string? - Cash exception.
- cheque boolean? - Cheque. The hotel accepts a printed document issued by the guest's bank in the guest's name as a form of payment.
- chequeException string? - Cheque exception.
- creditCard boolean? - Credit card. The hotel accepts payment by a card issued by a bank or credit card company. Also known as charge card, debit card, bank card, or charge plate.
- creditCardException string? - Credit card exception.
- debitCard boolean? - Debit card. The hotel accepts a bank-issued card that immediately deducts the charged funds from the guest's bank account upon processing.
- debitCardException string? - Debit card exception.
- mobileNfc boolean? - Mobile nfc. The hotel has the compatible computer hardware terminal that reads and charges a payment app on the guest's smartphone without requiring the two devices to make physical contact. Also known as Apple Pay, Google Pay, Samsung Pay.
- mobileNfcException string? - Mobile nfc exception.
googleapis.mybusiness: PersonalProtection
Personal protection measures implemented by the hotel during COVID-19.
Fields
- commonAreasOfferSanitizingItems boolean? - Hand-sanitizer and/or sanitizing wipes are offered in common areas.
- commonAreasOfferSanitizingItemsException string? - Common areas offer sanitizing items exception.
- faceMaskRequired boolean? - Masks required on the property.
- faceMaskRequiredException string? - Face mask required exception.
- guestRoomHygieneKitsAvailable boolean? - In-room hygiene kits with masks, hand sanitizer, and/or antibacterial wipes.
- guestRoomHygieneKitsAvailableException string? - Guest room hygiene kits available exception.
- protectiveEquipmentAvailable boolean? - Masks and/or gloves available for guests.
- protectiveEquipmentAvailableException string? - Protective equipment available exception.
googleapis.mybusiness: Pets
Policies regarding guest-owned animals.
Fields
- catsAllowed boolean? - Cats allowed. Domesticated felines are permitted at the property and allowed to stay in the guest room of their owner. May or may not require a fee.
- catsAllowedException string? - Cats allowed exception.
- dogsAllowed boolean? - Dogs allowed. Domesticated canines are permitted at the property and allowed to stay in the guest room of their owner. May or may not require a fee.
- dogsAllowedException string? - Dogs allowed exception.
- petsAllowed boolean? - Pets allowed. Household animals are allowed at the property and in the specific guest room of their owner. May or may not include dogs, cats, reptiles and/or fish. May or may not require a fee. Service animals are not considered to be pets, so not governed by this policy.
- petsAllowedException string? - Pets allowed exception.
- petsAllowedFree boolean? - Pets allowed free. Household animals are allowed at the property and in the specific guest room of their owner for free. May or may not include dogs, cats, reptiles, and/or fish.
- petsAllowedFreeException string? - Pets allowed free exception.
googleapis.mybusiness: PhoneInput
Input for PHONE_CALL/SMS verification.
Fields
- phoneNumber string? - The phone number that should be called or be sent SMS to. It must be one of the phone numbers in the eligible options.
googleapis.mybusiness: PhoneVerificationData
Display Data for verifications through phone, e.g. phone call, sms.
Fields
- phoneNumber string? - Phone number that the PIN will be sent to.
googleapis.mybusiness: PhysicalDistancing
Physical distancing measures implemented by the hotel during COVID-19.
Fields
- commonAreasPhysicalDistancingArranged boolean? - Common areas arranged to maintain physical distancing.
- commonAreasPhysicalDistancingArrangedException string? - Common areas physical distancing arranged exception.
- physicalDistancingRequired boolean? - Physical distancing required.
- physicalDistancingRequiredException string? - Physical distancing required exception.
- safetyDividers boolean? - Safety dividers at front desk and other locations.
- safetyDividersException string? - Safety dividers exception.
- sharedAreasLimitedOccupancy boolean? - Guest occupancy limited within shared facilities.
- sharedAreasLimitedOccupancyException string? - Shared areas limited occupancy exception.
- wellnessAreasHavePrivateSpaces boolean? - Private spaces designated in spa and wellness areas.
- wellnessAreasHavePrivateSpacesException string? - Wellness areas have private spaces exception.
googleapis.mybusiness: PlaceInfo
Defines an area that's represented by a place ID.
Fields
- name string? - The localized name of the place. For example,
Scottsdale, AZ
.
- placeId string? - The ID of the place. Must correspond to a [region.] (https://developers.google.com/places/web-service/supported_types#table3)
googleapis.mybusiness: Places
Defines the union of areas represented by a set of places.
Fields
- placeInfos PlaceInfo[]? - The areas represented by place IDs. Limited to a maximum of 20 places.
googleapis.mybusiness: PointRadius
A radius around a particular point (latitude/longitude).
Fields
- latlng LatLng? - An object that represents a latitude/longitude pair. This is expressed as a pair of doubles to represent degrees latitude and degrees longitude. Unless specified otherwise, this must conform to the WGS84 standard. Values must be within normalized ranges.
- radiusKm float? - The distance in kilometers of the area around the point.
googleapis.mybusiness: Policies
Property rules that impact guests.
Fields
- allInclusiveAvailable boolean? - All inclusive available. The hotel offers a rate option that includes the cost of the room, meals, activities, and other amenities that might otherwise be charged separately.
- allInclusiveAvailableException string? - All inclusive available exception.
- allInclusiveOnly boolean? - All inclusive only. The only rate option offered by the hotel is a rate that includes the cost of the room, meals, activities and other amenities that might otherwise be charged separately.
- allInclusiveOnlyException string? - All inclusive only exception.
- checkinTime TimeOfDay? - Represents a time of day. The date and time zone are either not significant or are specified elsewhere. An API may choose to allow leap seconds. Related types are google.type.Date and
google.protobuf.Timestamp
.
- checkinTimeException string? - Check-in time exception.
- checkoutTime TimeOfDay? - Represents a time of day. The date and time zone are either not significant or are specified elsewhere. An API may choose to allow leap seconds. Related types are google.type.Date and
google.protobuf.Timestamp
.
- checkoutTimeException string? - Check-out time exception.
- kidsStayFree boolean? - Kids stay free. The children of guests are allowed to stay in the room/suite of a parent or adult without an additional fee. The policy may or may not stipulate a limit of the child's age or the overall number of children allowed.
- kidsStayFreeException string? - Kids stay free exception.
- maxChildAge int? - Max child age. The hotel allows children up to a certain age to stay in the room/suite of a parent or adult without an additional fee.
- maxChildAgeException string? - Max child age exception.
- maxKidsStayFreeCount int? - Max kids stay free count. The hotel allows a specific, defined number of children to stay in the room/suite of a parent or adult without an additional fee.
- maxKidsStayFreeCountException string? - Max kids stay free count exception.
- paymentOptions PaymentOptions? - Forms of payment accepted at the property.
- smokeFreeProperty boolean? - Smoke free property. Smoking is not allowed inside the building, on balconies, or in outside spaces. Hotels that offer a designated area for guests to smoke are not considered smoke-free properties.
- smokeFreePropertyException string? - Smoke free property exception.
googleapis.mybusiness: Pools
Swimming pool or recreational water facilities available at the hotel.
Fields
- adultPool boolean? - Adult pool. A pool restricted for use by adults only. Can be indoors or outdoors.
- adultPoolException string? - Adult pool exception.
- hotTub boolean? - Hot tub. A man-made pool containing bubbling water maintained at a higher temperature and circulated by aerating jets for the purpose of soaking, relaxation and hydrotherapy. Can be indoors or outdoors. Not used for active swimming. Also known as Jacuzzi. Hot tub must be in a common area where all guests can access it. Does not apply to room-specific hot tubs that are only accessible to guest occupying that room.
- hotTubException string? - Hot tub exception.
- indoorPool boolean? - Indoor pool. A pool located inside the hotel and available for guests to use for swimming and/or soaking. Use may or may not be restricted to adults and/or children.
- indoorPoolException string? - Indoor pool exception.
- indoorPoolsCount int? - Indoor pools count. The sum of all indoor pools at the hotel.
- indoorPoolsCountException string? - Indoor pools count exception.
- lazyRiver boolean? - Lazy river. A man-made pool or several interconnected recreational pools built to mimic the shape and current of a winding river where guests float in the water on inflated rubber tubes. Can be indoors or outdoors.
- lazyRiverException string? - Lazy river exception.
- lifeguard boolean? - Lifeguard. A trained member of the hotel staff stationed by the hotel's indoor or outdoor swimming area and responsible for the safety of swimming guests.
- lifeguardException string? - Lifeguard exception.
- outdoorPool boolean? - Outdoor pool. A pool located outside on the grounds of the hotel and available for guests to use for swimming, soaking or recreation. Use may or may not be restricted to adults and/or children.
- outdoorPoolException string? - Outdoor pool exception.
- outdoorPoolsCount int? - Outdoor pools count. The sum of all outdoor pools at the hotel.
- outdoorPoolsCountException string? - Outdoor pools count exception.
- pool boolean? - Pool. The presence of a pool, either indoors or outdoors, for guests to use for swimming and/or soaking. Use may or may not be restricted to adults and/or children.
- poolException string? - Pool exception.
- poolsCount int? - Pools count. The sum of all pools at the hotel.
- poolsCountException string? - Pools count exception.
- wadingPool boolean? - Wading pool. A shallow pool designed for small children to play in. Can be indoors or outdoors. Also known as kiddie pool.
- wadingPoolException string? - Wading pool exception.
- waterPark boolean? - Water park. An aquatic recreation area with a large pool or series of pools that has features such as a water slide or tube, wavepool, fountains, rope swings, and/or obstacle course. Can be indoors or outdoors. Also known as adventure pool.
- waterParkException string? - Water park exception.
- waterslide boolean? - Waterslide. A continuously wetted chute positioned by an indoor or outdoor pool which people slide down into the water.
- waterslideException string? - Waterslide exception.
- wavePool boolean? - Wave pool. A large indoor or outdoor pool with a machine that produces water currents to mimic the ocean's crests.
- wavePoolException string? - Wave pool exception.
googleapis.mybusiness: PortionSize
Serving portion size of a food dish.
Fields
- quantity int? - Required. Number of the portion.
- unit MenuLabel[]? - Required. The repeated name_info field is for the unit in multiple languages.
googleapis.mybusiness: PostalAddress
Represents a postal address, e.g. for postal delivery or payments addresses. Given a postal address, a postal service can deliver items to a premise, P.O. Box or similar. It is not intended to model geographical locations (roads, towns, mountains). In typical usage an address would be created via user input or from importing existing data, depending on the type of process. Advice on address input / editing: - Use an i18n-ready address widget such as https://github.com/google/libaddressinput) - Users should not be presented with UI elements for input or editing of fields outside countries where that field is used. For more guidance on how to use this schema, please see: https://support.google.com/business/answer/6397478
Fields
- addressLines string[]? - Unstructured address lines describing the lower levels of an address. Because values in address_lines do not have type information and may sometimes contain multiple values in a single field (e.g. "Austin, TX"), it is important that the line order is clear. The order of address lines should be "envelope order" for the country/region of the address. In places where this can vary (e.g. Japan), address_language is used to make it explicit (e.g. "ja" for large-to-small ordering and "ja-Latn" or "en" for small-to-large). This way, the most specific line of an address can be selected based on the language. The minimum permitted structural representation of an address consists of a region_code with all remaining information placed in the address_lines. It would be possible to format such an address very approximately without geocoding, but no semantic reasoning could be made about any of the address components until it was at least partially resolved. Creating an address only containing a region_code and address_lines, and then geocoding is the recommended way to handle completely unstructured addresses (as opposed to guessing which parts of the address should be localities or administrative areas).
- administrativeArea string? - Optional. Highest administrative subdivision which is used for postal addresses of a country or region. For example, this can be a state, a province, an oblast, or a prefecture. Specifically, for Spain this is the province and not the autonomous community (e.g. "Barcelona" and not "Catalonia"). Many countries don't use an administrative area in postal addresses. E.g. in Switzerland this should be left unpopulated.
- languageCode string? - Optional. BCP-47 language code of the contents of this address (if known). This is often the UI language of the input form or is expected to match one of the languages used in the address' country/region, or their transliterated equivalents. This can affect formatting in certain countries, but is not critical to the correctness of the data and will never affect any validation or other non-formatting related operations. If this value is not known, it should be omitted (rather than specifying a possibly incorrect default). Examples: "zh-Hant", "ja", "ja-Latn", "en".
- locality string? - Optional. Generally refers to the city/town portion of the address. Examples: US city, IT comune, UK post town. In regions of the world where localities are not well defined or do not fit into this structure well, leave locality empty and use address_lines.
- organization string? - Optional. The name of the organization at the address.
- postalCode string? - Optional. Postal code of the address. Not all countries use or require postal codes to be present, but where they are used, they may trigger additional validation with other parts of the address (e.g. state/zip validation in the U.S.A.).
- recipients string[]? - Optional. The recipient at the address. This field may, under certain circumstances, contain multiline information. For example, it might contain "care of" information.
- regionCode string? - Required. CLDR region code of the country/region of the address. This is never inferred and it is up to the user to ensure the value is correct. See http://cldr.unicode.org/ and http://www.unicode.org/cldr/charts/30/supplemental/territory_information.html for details. Example: "CH" for Switzerland.
- revision int? - The schema revision of the
PostalAddress
. This must be set to 0, which is the latest revision. All new revisions must be backward compatible with old revisions.
- sortingCode string? - Optional. Additional, country-specific, sorting code. This is not used in most regions. Where it is used, the value is either a string like "CEDEX", optionally followed by a number (e.g. "CEDEX 7"), or just a number alone, representing the "sector code" (Jamaica), "delivery area indicator" (Malawi) or "post office indicator" (e.g. Côte d'Ivoire).
- sublocality string? - Optional. Sublocality of the address. For example, this can be neighborhoods, boroughs, districts.
googleapis.mybusiness: PriceList
A list of item price information. Price lists are structured as one or more price lists, each containing one or more sections with one or more items. For example, food price lists may represent breakfast/lunch/dinner menus, with sections for burgers/steak/seafood.
Fields
- labels Label[]? - Required. Language-tagged labels for the price list.
- priceListId string? - Required. ID for the price list. Price list, section, and item IDs cannot be duplicated within this Location.
- sections Section[]? - Required. Sections for this price list. Each price list must contain at least one section.
- sourceUrl string? - Optional source URL of where the price list was retrieved from. For example, this could be the URL of the page that was automatically scraped to populate the menu information.
googleapis.mybusiness: Profile
All information pertaining to the location's profile.
Fields
- description string? - Description of the location in your own voice, not editable by anyone else.
googleapis.mybusiness: Property
General factual information about the property's physical structure and important dates.
Fields
- builtYear int? - Built year. The year that construction of the property was completed.
- builtYearException string? - Built year exception.
- floorsCount int? - Floors count. The number of stories the building has from the ground floor to the top floor that are accessible to guests.
- floorsCountException string? - Floors count exception.
- lastRenovatedYear int? - Last renovated year. The year when the most recent renovation of the property was completed. Renovation may include all or any combination of the following: the units, the public spaces, the exterior, or the interior.
- lastRenovatedYearException string? - Last renovated year exception.
- roomsCount int? - Rooms count. The total number of rooms and suites bookable by guests for an overnight stay. Does not include event space, public spaces, conference rooms, fitness rooms, business centers, spa, salon, restaurants/bars, or shops.
- roomsCountException string? - Rooms count exception.
googleapis.mybusiness: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
googleapis.mybusiness: Question
Represents a single question and some of its answers.
Fields
- author Author? - Represents the author of a question or answer
- createTime string? - Output only. The timestamp for when the question was written.
- name string? - Output only. The unique name for the question. accounts//locations//questions/*
- text string? - The text of the question. It should contain at least three words and the total length should be greater than or equal to 10 characters. The maximum length is 4096 characters.
- topAnswers Answer[]? - Output only. A list of answers to the question, sorted by upvotes. This may not be a complete list of answers depending on the request parameters (answers_per_question)
- totalAnswerCount int? - Output only. The total number of answers posted for this question.
- updateTime string? - Output only. The timestamp for when the question was last modified.
- upvoteCount int? - Output only. The number of upvotes for the question.
googleapis.mybusiness: RegionCount
A region with its associated request count.
Fields
- count string? - Number of driving-direction requests from this region.
- label string? - Human-readable label for the region.
- latlng LatLng? - An object that represents a latitude/longitude pair. This is expressed as a pair of doubles to represent degrees latitude and degrees longitude. Unless specified otherwise, this must conform to the WGS84 standard. Values must be within normalized ranges.
googleapis.mybusiness: RelationshipData
Information of all parent and children locations related to this one.
Fields
- parentChain string? - The resource name of the Chain that this location is member of. How to find Chain ID
googleapis.mybusiness: RepeatedEnumAttributeValue
Values for an attribute with a value_type
of REPEATED_ENUM. This consists of two lists of value IDs: those that are set (true) and those that are unset (false). Values absent are considered unknown. At least one value must be specified.
Fields
- setValues string[]? - Enum values that are set.
- unsetValues string[]? - Enum values that are unset.
googleapis.mybusiness: ReportGoogleLocationRequest
Request message for reporting a GoogleLocation.
Fields
- locationGroupName string? - Optional. The resource name of the location group that this Google Location is being reported for, in the format
accounts/{account_id}
.
- reportReasonBadLocation string? - The reason for which the user is reporting this location when the issue is with the location itself.
- reportReasonBadRecommendation string? - The reason for which the user is reporting this location when the issue is with the recommendation. This report is useful if the location has been recommended to the GMB account.
- reportReasonElaboration string? - Optional. A text entry for elaborating on the reason for which the user is reporting this location. The maximum length is 512 characters.
- reportReasonLanguageCode string? - Optional. The BCP 47 code of language used in
report_reason_elaboration
.
googleapis.mybusiness: ReportLocalPostInsightsRequest
Request message for Insights.ReportLocalPostInsights
Fields
- basicRequest BasicMetricsRequest? - A request for basic metric insights.
- localPostNames string[]? - Required. The list of posts for which to fetch insights data. All posts have to belong to the location whose name is specified in the
name
field.
googleapis.mybusiness: ReportLocalPostInsightsResponse
Response message for Insights.ReportLocalPostInsights
Fields
- localPostMetrics LocalPostMetrics[]? - One entry per requested post corresponding to this location.
- name string? - Name
- timeZone string? - Time zone (IANA timezone IDs, eg, 'Europe/London') of the location.
googleapis.mybusiness: ReportLocationInsightsRequest
Request message for Insights.ReportLocationInsights.
Fields
- basicRequest BasicMetricsRequest? - A request for basic metric insights.
- drivingDirectionsRequest DrivingDirectionMetricsRequest? - A request for driving direction insights.
- locationNames string[]? - A collection of locations to fetch insights for, specified by their names.
googleapis.mybusiness: ReportLocationInsightsResponse
Response message for Insights.ReportLocationInsights
.
Fields
- locationDrivingDirectionMetrics LocationDrivingDirectionMetrics[]? - A collection of values for driving direction-related metrics.
- locationMetrics LocationMetrics[]? - A collection of metric values by location.
googleapis.mybusiness: Review
Output only. Represents a review for a location.
Fields
- comment string? - The body of the review as plain text with markups.
- createTime string? - The timestamp for when the review was written.
- name string? - The resource name. For Review it is of the form
accounts/{account_id}/locations/{location_id}/reviews/{review_id}
- reviewId string? - The encrypted unique identifier.
- reviewReply ReviewReply? - Represents the location owner/manager's reply to a review.
- reviewer Reviewer? - Represents the author of the review.
- starRating string? - The star rating of the review.
- updateTime string? - The timestamp for when the review was last modified.
googleapis.mybusiness: Reviewer
Represents the author of the review.
Fields
- displayName string? - The name of the reviewer. Only populated with the reviewer's real name if
is_anonymous
is false.
- isAnonymous boolean? - Indicates whether the reviewer has opted to remain anonymous.
- profilePhotoUrl string? - The profile photo link of the reviewer. Only populated if
is_anonymous
is false.
googleapis.mybusiness: ReviewReply
Represents the location owner/manager's reply to a review.
Fields
- comment string? - The body of the reply as plain text with markups. The maximum length is 4096 bytes.
- updateTime string? - Output only. The timestamp for when the reply was last modified.
googleapis.mybusiness: SearchChainsResponse
Response message for Locations.SearchChains.
Fields
- chains Chain[]? - Chains that match the queried chain_display_name in SearchChainsRequest. If there are no matches, this field will be empty. Results are listed in order of relevance.
googleapis.mybusiness: SearchGoogleLocationsRequest
Request message for GoogleLocations.SearchGoogleLocations.
Fields
- location Location? - A location. See the [help center article] (https://support.google.com/business/answer/3038177) for a detailed description of these fields, or the category endpoint for a list of valid business categories.
- query string? - Text query to search for. The search results from a query string will be less accurate than if providing an exact location, but can provide more inexact matches.
- resultCount int? - The number of matches to return. The default value is 3, with a maximum of 10. Note that latency may increase if more are requested. There is no pagination.
googleapis.mybusiness: SearchGoogleLocationsResponse
Response message for GoogleLocations.SearchGoogleLocations.
Fields
- googleLocations GoogleLocation[]? - A collection of GoogleLocations that are potential matches to the specified request, listed in order from most to least accuracy.
googleapis.mybusiness: Section
A section of the price list containing one or more items.
Fields
- items Item[]? - Items that are contained within this section of the price list.
- labels Label[]? - Required. Language-tagged labels for the section. We recommend that section names and descriptions be 140 characters or less. At least one set of labels is required.
- sectionId string? - Required. ID for the section. Price list, section, and item IDs cannot be duplicated within this Location.
- sectionType string? - Optional. Type of the current price list section. Default value is FOOD.
googleapis.mybusiness: ServiceAreaBusiness
Service area businesses provide their service at the customer's location (for example, a locksmith or plumber).
Fields
- businessType string? - Indicates the type of the service area business.
- places Places? - Defines the union of areas represented by a set of places.
- radius PointRadius? - A radius around a particular point (latitude/longitude).
googleapis.mybusiness: ServiceBusinessContext
Additional data for service business verification.
Fields
- address PostalAddress? - Represents a postal address, e.g. for postal delivery or payments addresses. Given a postal address, a postal service can deliver items to a premise, P.O. Box or similar. It is not intended to model geographical locations (roads, towns, mountains). In typical usage an address would be created via user input or from importing existing data, depending on the type of process. Advice on address input / editing: - Use an i18n-ready address widget such as https://github.com/google/libaddressinput) - Users should not be presented with UI elements for input or editing of fields outside countries where that field is used. For more guidance on how to use this schema, please see: https://support.google.com/business/answer/6397478
googleapis.mybusiness: ServiceItem
A message that describes a single service item. It is used to describe the type of service that the merchant provides. For example, haircut can be a service.
Fields
- freeFormServiceItem FreeFormServiceItem? - Represents a free-form service offered by the merchant. These are services that are not exposed as part of our structure service data. The merchant manually enters the names for of such services via a geomerchant surface.
- isOffered boolean? - Optional. This field decides whether or not the input service is offered by the merchant.
- price Money? - Represents an amount of money with its currency type.
- structuredServiceItem StructuredServiceItem? - Represents a structured service offered by the merchant. For eg: toilet_installation.
googleapis.mybusiness: ServiceList
A service list containing one or more service items.
Fields
- name string? - Required. Google identifier for this location in the form:
accounts/{account_id}/locations/{location_id}/serviceList
- serviceItems ServiceItem[]? - Service items that are contained within this service list. Duplicated service items will be removed automatically.
googleapis.mybusiness: Services
Conveniences or help provided by the property to facilitate an easier, more comfortable stay.
Fields
- baggageStorage boolean? - Baggage storage. A provision for guests to leave their bags at the hotel when they arrive for their stay before the official check-in time. May or may not apply for guests who wish to leave their bags after check-out and before departing the locale. Also known as bag dropoff.
- baggageStorageException string? - Baggage storage exception.
- concierge boolean? - Concierge. Hotel staff member(s) responsible for facilitating an easy, comfortable stay through making reservations for meals, sourcing theater tickets, arranging tours, finding a doctor, making recommendations, and answering questions.
- conciergeException string? - Concierge exception.
- convenienceStore boolean? - Convenience store. A shop at the hotel primarily selling snacks, drinks, non-prescription medicines, health and beauty aids, magazines and newspapers.
- convenienceStoreException string? - Convenience store exception.
- currencyExchange boolean? - Currency exchange. A staff member or automated machine tasked with the transaction of providing the native currency of the hotel's locale in exchange for the foreign currency provided by a guest.
- currencyExchangeException string? - Currency exchange exception.
- elevator boolean? - Elevator. A passenger elevator that transports guests from one story to another. Also known as lift.
- elevatorException string? - Elevator exception.
- frontDesk boolean? - Front desk. A counter or desk in the lobby or the immediate interior of the hotel where a member of the staff greets guests and processes the information related to their stay (including check-in and check-out). May or may not be manned and open 24/7.
- frontDeskException string? - Front desk exception.
- fullServiceLaundry boolean? - Full service laundry. Laundry and dry cleaning facilitated and handled by the hotel on behalf of the guest. Does not include the provision for guests to do their own laundry in on-site machines.
- fullServiceLaundryException string? - Full service laundry exception.
- giftShop boolean? - Gift shop. An on-site store primarily selling souvenirs, mementos and other gift items. May or may not also sell sundries, magazines and newspapers, clothing, or snacks.
- giftShopException string? - Gift shop exception.
- languagesSpoken LanguagesSpoken? - Languages spoken by at least one staff member.
- selfServiceLaundry boolean? - Self service laundry. On-site clothes washers and dryers accessible to guests for the purpose of washing and drying their own clothes. May or may not require payment to use the machines.
- selfServiceLaundryException string? - Self service laundry exception.
- socialHour boolean? - Social hour. A reception with complimentary soft drinks, tea, coffee, wine and/or cocktails in the afternoon or evening. Can be hosted by hotel staff or guests may serve themselves. Also known as wine hour. The availability of coffee/tea in the lobby throughout the day does not constitute a social or wine hour.
- socialHourException string? - Social hour exception.
- twentyFourHourFrontDesk boolean? - 24hr front desk. Front desk is staffed 24 hours a day.
- twentyFourHourFrontDeskException string? - 24hr front desk exception.
- wakeUpCalls boolean? - Wake up calls. By direction of the guest, a hotel staff member will phone the guest unit at the requested hour. Also known as morning call.
- wakeUpCallsException string? - Wake up calls exception.
googleapis.mybusiness: ServiceType
A message describing a service type that the business offers.
Fields
- displayName string? - Output only. The human-readable display name for the service type.
- serviceTypeId string? - Output only. A stable ID (provided by Google) for this service type.
googleapis.mybusiness: SpecialHourPeriod
Represents a single time period when a location's operational hours differ from its normal business hours. A special hour period must represent a range of less than 24 hours. The open_time
and start_date
must predate the close_time
and end_date
. The close_time
and end_date
can extend to 11:59 a.m. on the day after the specified start_date
. For example, the following inputs are valid: start_date=2015-11-23, open_time=08:00, close_time=18:00 start_date=2015-11-23, end_date=2015-11-23, open_time=08:00, close_time=18:00 start_date=2015-11-23, end_date=2015-11-24, open_time=13:00, close_time=11:59 The following inputs are not valid: start_date=2015-11-23, open_time=13:00, close_time=11:59 start_date=2015-11-23, end_date=2015-11-24, open_time=13:00, close_time=12:00 start_date=2015-11-23, end_date=2015-11-25, open_time=08:00, close_time=18:00
Fields
- closeTime string? - The wall time on
end_date
when a location closes, expressed in 24hr ISO 8601 extended format. (hh:mm) Valid values are 00:00-24:00, where 24:00 represents midnight at the end of the specified day field. Must be specified ifis_closed
is false.
- endDate Date? - Represents a whole or partial calendar date, such as a birthday. The time of day and time zone are either specified elsewhere or are insignificant. The date is relative to the Gregorian Calendar. This can represent one of the following: * A full date, with non-zero year, month, and day values * A month and day value, with a zero year, such as an anniversary * A year on its own, with zero month and day values * A year and month value, with a zero day, such as a credit card expiration date Related types are google.type.TimeOfDay and
google.protobuf.Timestamp
.
- isClosed boolean? - If true,
end_date
,open_time
, andclose_time
are ignored, and the date specified instart_date
is treated as the location being closed for the entire day.
- openTime string? - The wall time on
start_date
when a location opens, expressed in 24hr ISO 8601 extended format. (hh:mm) Valid values are 00:00-24:00, where 24:00 represents midnight at the end of the specified day field. Must be specified ifis_closed
is false.
- startDate Date? - Represents a whole or partial calendar date, such as a birthday. The time of day and time zone are either specified elsewhere or are insignificant. The date is relative to the Gregorian Calendar. This can represent one of the following: * A full date, with non-zero year, month, and day values * A month and day value, with a zero year, such as an anniversary * A year on its own, with zero month and day values * A year and month value, with a zero day, such as a credit card expiration date Related types are google.type.TimeOfDay and
google.protobuf.Timestamp
.
googleapis.mybusiness: SpecialHours
Represents a set of time periods when a location's operational hours differ from its normal business hours.
Fields
- specialHourPeriods SpecialHourPeriod[]? - A list of exceptions to the business's regular hours.
googleapis.mybusiness: StartUploadMediaItemDataRequest
Request message for Media.StartUploadMediaItemData.
googleapis.mybusiness: StructuredServiceItem
Represents a structured service offered by the merchant. For eg: toilet_installation.
Fields
- description string? - Optional. Description of structured service item. The character limit is 300.
- serviceTypeId string? - Required. The
service_type_id
field is a Google provided unique ID that can be found inServiceTypeMetadata
. This information is provided by BatchGetBusinessCategories rpc service.
googleapis.mybusiness: TargetLocation
Represents a target location for a pending invitation.
Fields
- locationAddress string? - The address of the location to which the user is invited.
- locationName string? - The name of the location to which the user is invited.
googleapis.mybusiness: TimeDimension
The dimension for which data is divided over.
Fields
- dayOfWeek string? - The day of the week ("MONDAY" to "SUNDAY") this value corresponds to. Set for BREAKDOWN_DAY_OF_WEEK option.
- timeOfDay TimeOfDay? - Represents a time of day. The date and time zone are either not significant or are specified elsewhere. An API may choose to allow leap seconds. Related types are google.type.Date and
google.protobuf.Timestamp
.
- timeRange TimeRange? - A range of time. Data will be pulled over the range as a half-open inverval (that is, [start_time, end_time)).
googleapis.mybusiness: TimeInterval
An interval of time, inclusive. It must contain all fields to be valid.
Fields
- endDate Date? - Represents a whole or partial calendar date, such as a birthday. The time of day and time zone are either specified elsewhere or are insignificant. The date is relative to the Gregorian Calendar. This can represent one of the following: * A full date, with non-zero year, month, and day values * A month and day value, with a zero year, such as an anniversary * A year on its own, with zero month and day values * A year and month value, with a zero day, such as a credit card expiration date Related types are google.type.TimeOfDay and
google.protobuf.Timestamp
.
- endTime TimeOfDay? - Represents a time of day. The date and time zone are either not significant or are specified elsewhere. An API may choose to allow leap seconds. Related types are google.type.Date and
google.protobuf.Timestamp
.
- startDate Date? - Represents a whole or partial calendar date, such as a birthday. The time of day and time zone are either specified elsewhere or are insignificant. The date is relative to the Gregorian Calendar. This can represent one of the following: * A full date, with non-zero year, month, and day values * A month and day value, with a zero year, such as an anniversary * A year on its own, with zero month and day values * A year and month value, with a zero day, such as a credit card expiration date Related types are google.type.TimeOfDay and
google.protobuf.Timestamp
.
- startTime TimeOfDay? - Represents a time of day. The date and time zone are either not significant or are specified elsewhere. An API may choose to allow leap seconds. Related types are google.type.Date and
google.protobuf.Timestamp
.
googleapis.mybusiness: TimeOfDay
Represents a time of day. The date and time zone are either not significant or are specified elsewhere. An API may choose to allow leap seconds. Related types are google.type.Date and google.protobuf.Timestamp
.
Fields
- hours int? - Hours of day in 24 hour format. Should be from 0 to 23. An API may choose to allow the value "24:00:00" for scenarios like business closing time.
- minutes int? - Minutes of hour of day. Must be from 0 to 59.
- nanos int? - Fractions of seconds in nanoseconds. Must be from 0 to 999,999,999.
- seconds int? - Seconds of minutes of the time. Must normally be from 0 to 59. An API may allow the value 60 if it allows leap-seconds.
googleapis.mybusiness: TimePeriod
Represents a span of time that the business is open, starting on the specified open day/time and closing on the specified close day/time. The closing time must occur after the opening time, for example later in the same day, or on a subsequent day.
Fields
- closeDay string? - Indicates the day of the week this period ends on.
- closeTime string? - Time in 24hr ISO 8601 extended format (hh:mm). Valid values are 00:00-24:00, where 24:00 represents midnight at the end of the specified day field.
- openDay string? - Indicates the day of the week this period starts on.
- openTime string? - Time in 24hr ISO 8601 extended format (hh:mm). Valid values are 00:00-24:00, where 24:00 represents midnight at the end of the specified day field.
googleapis.mybusiness: TimeRange
A range of time. Data will be pulled over the range as a half-open inverval (that is, [start_time, end_time)).
Fields
- endTime string? - Epoch timestamp for the end of the range (exclusive).
- startTime string? - Epoch timestamp for the start of the range (inclusive).
googleapis.mybusiness: TopDirectionSources
Top regions where driving-direction requests originated from.
Fields
- dayCount int? - The number of days data is aggregated over.
- regionCounts RegionCount[]? - Regions sorted in descending order by count.
googleapis.mybusiness: TransferLocationRequest
Request message for Locations.TransferLocation.
Fields
- toAccount string? - Name of the account resource to transfer the location to (for example, "accounts/8675309").
googleapis.mybusiness: Transportation
Vehicles or vehicular services facilitated or owned by the property.
Fields
- airportShuttle boolean? - Airport shuttle. The hotel provides guests with a chauffeured van or bus to and from the airport. Can be free or for a fee. Guests may share the vehicle with other guests unknown to them. Applies if the hotel has a third-party shuttle service (office/desk etc.) within the hotel. As long as hotel provides this service, it doesn't matter if it's directly with them or a third party they work with. Does not apply if guest has to coordinate with an entity outside/other than the hotel.
- airportShuttleException string? - Airport shuttle exception.
- carRentalOnProperty boolean? - Car rental on property. A branch of a rental car company with a processing desk in the hotel. Available cars for rent may be awaiting at the hotel or in a nearby lot.
- carRentalOnPropertyException string? - Car rental on property exception.
- freeAirportShuttle boolean? - Free airport shuttle. Airport shuttle is free to guests. Must be free to all guests without any conditions.
- freeAirportShuttleException string? - Free airport shuttle exception.
- freePrivateCarService boolean? - Free private car service. Private chauffeured car service is free to guests.
- freePrivateCarServiceException string? - Free private car service exception.
- localShuttle boolean? - Local shuttle. A car, van or bus provided by the hotel to transport guests to destinations within a specified range of distance around the hotel. Usually shopping and/or convention centers, downtown districts, or beaches. Can be free or for a fee.
- localShuttleException string? - Local shuttle exception.
- privateCarService boolean? - Private car service. Hotel provides a private chauffeured car to transport guests to destinations. Passengers in the car are either alone or are known to one another and have requested the car together. Service can be free or for a fee and travel distance is usually limited to a specific range. Not a taxi.
- privateCarServiceException string? - Private car service exception.
- transfer boolean? - Transfer. Hotel provides a shuttle service or car service to take guests to and from the nearest airport or train station. Can be free or for a fee. Guests may share the vehicle with other guests unknown to them.
- transferException string? - Transfer exception.
googleapis.mybusiness: UpsertAnswerRequest
Request message for QuestionsAndAnswers.UpsertAnswer
Fields
- answer Answer? - Represents an answer to a question
googleapis.mybusiness: UrlAttributeValue
Values for an attribute with a value_type
of URL.
Fields
- url string? - The URL.
googleapis.mybusiness: Verification
A verification represents a verification attempt on a location.
Fields
- createTime string? - The timestamp when the verification is requested.
- method string? - The method of the verification.
- name string? - Resource name of the verification.
- state string? - The state of the verification.
googleapis.mybusiness: VerificationOption
The verification option represents how to verify the location (indicated by verification method) and where the verification will be sent to (indicated by display data).
Fields
- addressData AddressVerificationData? - Display data for verifications through postcard.
- emailData EmailVerificationData? - Display data for verifications through email.
- phoneData PhoneVerificationData? - Display Data for verifications through phone, e.g. phone call, sms.
- verificationMethod string? - Method to verify the location.
googleapis.mybusiness: VerifyLocationRequest
Request message for Verifications.VerifyLocation.
Fields
- addressInput AddressInput? - Input for ADDRESS verification.
- context ServiceBusinessContext? - Additional data for service business verification.
- emailInput EmailInput? - Input for EMAIL verification.
- languageCode string? - The BCP 47 language code representing the language that is to be used for the verification process.
- method string? - Verification method.
- phoneInput PhoneInput? - Input for PHONE_CALL/SMS verification.
googleapis.mybusiness: VerifyLocationResponse
Response message for Verifications.VerifyLocation.
Fields
- verification Verification? - A verification represents a verification attempt on a location.
googleapis.mybusiness: ViewsFromUnit
Views available from the guest unit itself.
Fields
- beachView boolean? - Beach view. A guestroom that features a window through which guests can see the beach.
- beachViewException string? - Beach view exception.
- cityView boolean? - City view. A guestroom that features a window through which guests can see the buildings, parks and/or streets of the city.
- cityViewException string? - City view exception.
- gardenView boolean? - Garden view. A guestroom that features a window through which guests can see a garden.
- gardenViewException string? - Garden view exception.
- lakeView boolean? - Lake view.
- lakeViewException string? - Lake view exception.
- landmarkView boolean? - Landmark view. A guestroom that features a window through which guests can see a landmark such as the countryside, a golf course, the forest, a park, a rain forst, a mountain or a slope.
- landmarkViewException string? - Landmark view exception.
- oceanView boolean? - Ocean view. A guestroom that features a window through which guests can see the ocean.
- oceanViewException string? - Ocean view exception.
- poolView boolean? - Pool view. A guestroom that features a window through which guests can see the hotel's swimming pool.
- poolViewException string? - Pool view exception.
- valleyView boolean? - Valley view. A guestroom that features a window through which guests can see over a valley.
- valleyViewException string? - Valley view exception.
googleapis.mybusiness: Wellness
Guest facilities at the property to promote or maintain health, beauty, and fitness.
Fields
- doctorOnCall boolean? - Doctor on call. The hotel has a contract with a medical professional who provides services to hotel guests should they fall ill during their stay. The doctor may or may not have an on-site office or be at the hotel at all times.
- doctorOnCallException string? - Doctor on call exception.
- ellipticalMachine boolean? - Elliptical machine. An electric, stationary fitness machine with pedals that simulates climbing, walking or running and provides a user-controlled range of speeds and tensions. May not have arm-controlled levers to work out the upper body as well. Commonly found in a gym, fitness room, health center, or health club.
- ellipticalMachineException string? - Elliptical machine exception.
- fitnessCenter boolean? - Fitness center. A room or building at the hotel containing equipment to promote physical activity, such as treadmills, elliptical machines, stationary bikes, weight machines, free weights, and/or stretching mats. Use of the fitness center can be free or for a fee. May or may not be staffed. May or may not offer instructor-led classes in various styles of physical conditioning. May or may not be open 24/7. May or may not include locker rooms and showers. Also known as health club, gym, fitness room, health center.
- fitnessCenterException string? - Fitness center exception.
- freeFitnessCenter boolean? - Free fitness center. Guests may use the fitness center for free.
- freeFitnessCenterException string? - Free fitness center exception.
- freeWeights boolean? - Free weights. Individual handheld fitness equipment of varied weights used for upper body strength training or bodybuilding. Also known as barbells, dumbbells, or kettlebells. Often stored on a rack with the weights arranged from light to heavy. Commonly found in a gym, fitness room, health center, or health club.
- freeWeightsException string? - Free weights exception.
- massage boolean? - Massage. A service provided by a trained massage therapist involving the physical manipulation of a guest's muscles in order to achieve relaxation or pain relief.
- massageException string? - Massage exception.
- salon boolean? - Salon. A room at the hotel where professionals provide hair styling services such as shampooing, blow drying, hair dos, hair cutting and hair coloring. Also known as hairdresser or beauty salon.
- salonException string? - Salon exception.
- sauna boolean? - Sauna. A wood-paneled room heated to a high temperature where guests sit on built-in wood benches for the purpose of perspiring and relaxing their muscles. Can be dry or slightly wet heat. Not a steam room.
- saunaException string? - Sauna exception.
- spa boolean? - Spa. A designated area, room or building at the hotel offering health and beauty treatment through such means as steam baths, exercise equipment, and massage. May also offer facials, nail care, and hair care. Services are usually available by appointment and for an additional fee. Does not apply if hotel only offers a steam room; must offer other beauty and/or health treatments as well.
- spaException string? - Spa exception.
- treadmill boolean? - Treadmill. An electric stationary fitness machine that simulates a moving path to promote walking or running within a range of user-controlled speeds and inclines. Also known as running machine. Commonly found in a gym, fitness room, health center, or health club.
- treadmillException string? - Treadmill exception.
- weightMachine boolean? - Weight machine. Non-electronic fitness equipment designed for the user to target the exertion of different muscles. Usually incorporates a padded seat, a stack of flat weights and various bars and pulleys. May be designed for toning a specific part of the body or may involve different user-controlled settings, hardware and pulleys so as to provide an overall workout in one machine. Commonly found in a gym, fitness center, fitness room, or health club.
- weightMachineException string? - Weight machine exception.
Import
import ballerinax/googleapis.mybusiness;
Metadata
Released date: about 1 year ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 0
Current verison: 0
Weekly downloads
Keywords
Commerce/eCommerce
Cost/Free
Vendor/Google
Contributors
Dependencies