googleapis.manufacturercenter
Module googleapis.manufacturercenter
API
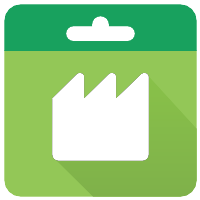
ballerinax/googleapis.manufacturercenter Ballerina library
Overview
This is a generated connector for Google Manufacturer Center API v1 OpenAPI specification. Public API for managing Manufacturer Center related data.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Google account
- Obtain tokens - Follow this link
Quickstart
To use the Google Manufacturer Center connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/googleapis.manufacturercenter
module into the Ballerina project.
import ballerinax/googleapis.manufacturercenter;
Step 2: Create a new connector instance
Create a manufacturercenter:ClientConfig
with the OAuth2 tokens obtained, and initialize the connector with it.
manufacturercenter:ClientConfig clientConfig = { auth: { clientId: <CLIENT_ID>, clientSecret: <CLIENT_SECRET>, refreshUrl: <REFRESH_URL>, refreshToken: <REFRESH_TOKEN> } }; manufacturercenter:Client baseClient = check new Client(clientConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to list all the products in a Manufacturer Center account using the connector.
Lists all the products in a Manufacturer Center account
public function main() { manufacturercenter:ListProductsResponse|error response = baseClient->listAccountsProducts("accounts/<account_id>"); if (response is manufacturercenter:ListProductsResponse) { log:printInfo(response.toString()); } else { log:printError(response.message()); } }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
googleapis.manufacturercenter: Client
This is a generated connector for Google Manufacturer Center API v1 OpenAPI specification. Public API for managing Manufacturer Center related data.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Google account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://manufacturers.googleapis.com/" - URL of the target service
listAccountsProducts
function listAccountsProducts(string parent, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, string[]? include, int? pageSize, string? pageToken) returns ListProductsResponse|error
Lists all the products in a Manufacturer Center account.
Parameters
- parent string - Parent ID in the format
accounts/{account_id}
.account_id
- The ID of the Manufacturer Center account.
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- include string[]? (default ()) - The information to be included in the response. Only sections listed here will be returned.
- pageSize int? (default ()) - Maximum number of product statuses to return in the response, used for paging.
- pageToken string? (default ()) - The token returned by the previous request.
Return Type
- ListProductsResponse|error - Successful response
getAccountsProducts
function getAccountsProducts(string parent, string name, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, string[]? include) returns Product|error
Gets the product from a Manufacturer Center account, including product issues. A recently updated product takes around 15 minutes to process. Changes are only visible after it has been processed. While some issues may be available once the product has been processed, other issues may take days to appear.
Parameters
- parent string - Parent ID in the format
accounts/{account_id}
.account_id
- The ID of the Manufacturer Center account.
- name string - Name in the format
{target_country}:{content_language}:{product_id}
.target_country
- The target country of the product as a CLDR territory code (for example, US).content_language
- The content language of the product as a two-letter ISO 639-1 language code (for example, en).product_id
- The ID of the product. For more information, see https://support.google.com/manufacturers/answer/6124116#id.
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- include string[]? (default ()) - The information to be included in the response. Only sections listed here will be returned.
updateAccountsProducts
function updateAccountsProducts(string parent, string name, Attributes payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns Response|error
Inserts or updates the attributes of the product in a Manufacturer Center account. Creates a product with the provided attributes. If the product already exists, then all attributes are replaced with the new ones. The checks at upload time are minimal. All required attributes need to be present for a product to be valid. Issues may show up later after the API has accepted a new upload for a product and it is possible to overwrite an existing valid product with an invalid product. To detect this, you should retrieve the product and check it for issues once the new version is available. Uploaded attributes first need to be processed before they can be retrieved. Until then, new products will be unavailable, and retrieval of previously uploaded products will return the original state of the product.
Parameters
- parent string - Parent ID in the format
accounts/{account_id}
.account_id
- The ID of the Manufacturer Center account.
- name string - Name in the format
{target_country}:{content_language}:{product_id}
.target_country
- The target country of the product as a CLDR territory code (for example, US).content_language
- The content language of the product as a two-letter ISO 639-1 language code (for example, en).product_id
- The ID of the product. For more information, see https://support.google.com/manufacturers/answer/6124116#id.
- payload Attributes - Attributes request
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
deleteAccountsProducts
function deleteAccountsProducts(string parent, string name, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns Response|error
Deletes the product from a Manufacturer Center account.
Parameters
- parent string - Parent ID in the format
accounts/{account_id}
.account_id
- The ID of the Manufacturer Center account.
- name string - Name in the format
{target_country}:{content_language}:{product_id}
.target_country
- The target country of the product as a CLDR territory code (for example, US).content_language
- The content language of the product as a two-letter ISO 639-1 language code (for example, en).product_id
- The ID of the product. For more information, see https://support.google.com/manufacturers/answer/6124116#id.
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Records
googleapis.manufacturercenter: Attributes
Attributes of the product. For more information, see https://support.google.com/manufacturers/answer/6124116.
Fields
- additionalImageLink Image[]? - The additional images of the product. For more information, see https://support.google.com/manufacturers/answer/6124116#addlimage.
- ageGroup string? - The target age group of the product. For more information, see https://support.google.com/manufacturers/answer/6124116#agegroup.
- brand string? - The brand name of the product. For more information, see https://support.google.com/manufacturers/answer/6124116#brand.
- capacity Capacity? - The capacity of a product. For more information, see https://support.google.com/manufacturers/answer/6124116#capacity.
- color string? - The color of the product. For more information, see https://support.google.com/manufacturers/answer/6124116#color.
- count Count? - The number of products in a single package. For more information, see https://support.google.com/manufacturers/answer/6124116#count.
- description string? - The description of the product. For more information, see https://support.google.com/manufacturers/answer/6124116#description.
- disclosureDate string? - The disclosure date of the product. For more information, see https://support.google.com/manufacturers/answer/6124116#disclosure.
- excludedDestination string[]? - A list of excluded destinations.
- featureDescription FeatureDescription[]? - The rich format description of the product. For more information, see https://support.google.com/manufacturers/answer/6124116#featuredesc.
- flavor string? - The flavor of the product. For more information, see https://support.google.com/manufacturers/answer/6124116#flavor.
- format string? - The format of the product. For more information, see https://support.google.com/manufacturers/answer/6124116#format.
- gender string? - The target gender of the product. For more information, see https://support.google.com/manufacturers/answer/6124116#gender.
- gtin string[]? - The Global Trade Item Number (GTIN) of the product. For more information, see https://support.google.com/manufacturers/answer/6124116#gtin.
- imageLink Image? - An image.
- includedDestination string[]? - A list of included destinations.
- itemGroupId string? - The item group id of the product. For more information, see https://support.google.com/manufacturers/answer/6124116#itemgroupid.
- material string? - The material of the product. For more information, see https://support.google.com/manufacturers/answer/6124116#material.
- mpn string? - The Manufacturer Part Number (MPN) of the product. For more information, see https://support.google.com/manufacturers/answer/6124116#mpn.
- pattern string? - The pattern of the product. For more information, see https://support.google.com/manufacturers/answer/6124116#pattern.
- productDetail ProductDetail[]? - The details of the product. For more information, see https://support.google.com/manufacturers/answer/6124116#productdetail.
- productHighlight string[]? - The product highlights. For more information, see https://support.google.com/manufacturers/answer/10066942
- productLine string? - The name of the group of products related to the product. For more information, see https://support.google.com/manufacturers/answer/6124116#productline.
- productName string? - The canonical name of the product. For more information, see https://support.google.com/manufacturers/answer/6124116#productname.
- productPageUrl string? - The URL of the detail page of the product. For more information, see https://support.google.com/manufacturers/answer/6124116#productpage.
- productType string[]? - The type or category of the product. For more information, see https://support.google.com/manufacturers/answer/6124116#producttype.
- releaseDate string? - The release date of the product. For more information, see https://support.google.com/manufacturers/answer/6124116#release.
- richProductContent string[]? - Rich product content. For more information, see https://support.google.com/manufacturers/answer/9389865
- scent string? - The scent of the product. For more information, see https://support.google.com/manufacturers/answer/6124116#scent.
- size string? - The size of the product. For more information, see https://support.google.com/manufacturers/answer/6124116#size.
- sizeSystem string? - The size system of the product. For more information, see https://support.google.com/manufacturers/answer/6124116#sizesystem.
- sizeType string[]? - The size type of the product. For more information, see https://support.google.com/manufacturers/answer/6124116#sizetype.
- suggestedRetailPrice Price? - A price.
- targetClientId string? - The target client id. Should only be used in the accounts of the data partners.
- theme string? - The theme of the product. For more information, see https://support.google.com/manufacturers/answer/6124116#theme.
- title string? - The title of the product. For more information, see https://support.google.com/manufacturers/answer/6124116#title.
- videoLink string[]? - The videos of the product. For more information, see https://support.google.com/manufacturers/answer/6124116#video.
googleapis.manufacturercenter: Capacity
The capacity of a product. For more information, see https://support.google.com/manufacturers/answer/6124116#capacity.
Fields
- unit string? - The unit of the capacity, i.e., MB, GB, or TB.
- value string? - The numeric value of the capacity.
googleapis.manufacturercenter: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
googleapis.manufacturercenter: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
googleapis.manufacturercenter: Count
The number of products in a single package. For more information, see https://support.google.com/manufacturers/answer/6124116#count.
Fields
- unit string? - The unit in which these products are counted.
- value string? - The numeric value of the number of products in a package.
googleapis.manufacturercenter: DestinationStatus
The destination status.
Fields
- destination string? - The name of the destination.
- status string? - The status of the destination.
googleapis.manufacturercenter: Empty
A generic empty message that you can re-use to avoid defining duplicated empty messages in your APIs. A typical example is to use it as the request or the response type of an API method. For instance: service Foo { rpc Bar(google.protobuf.Empty) returns (google.protobuf.Empty); } The JSON representation for Empty
is empty JSON object {}
.
googleapis.manufacturercenter: FeatureDescription
A feature description of the product. For more information, see https://support.google.com/manufacturers/answer/6124116#featuredesc.
Fields
- headline string? - A short description of the feature.
- image Image? - An image.
- text string? - A detailed description of the feature.
googleapis.manufacturercenter: Image
An image.
Fields
- imageUrl string? - The URL of the image. For crawled images, this is the provided URL. For uploaded images, this is a serving URL from Google if the image has been processed successfully.
- status string? - The status of the image. @OutputOnly
- 'type string? - The type of the image, i.e., crawled or uploaded. @OutputOnly
googleapis.manufacturercenter: Issue
Product issue.
Fields
- attribute string? - If present, the attribute that triggered the issue. For more information about attributes, see https://support.google.com/manufacturers/answer/6124116.
- description string? - Longer description of the issue focused on how to resolve it.
- destination string? - The destination this issue applies to.
- resolution string? - What needs to happen to resolve the issue.
- severity string? - The severity of the issue.
- timestamp string? - The timestamp when this issue appeared.
- title string? - Short title describing the nature of the issue.
- 'type string? - The server-generated type of the issue, for example, “INCORRECT_TEXT_FORMATTING”, “IMAGE_NOT_SERVEABLE”, etc.
googleapis.manufacturercenter: ListProductsResponse
Fields
- nextPageToken string? - The token for the retrieval of the next page of product statuses.
- products Product[]? - List of the products.
googleapis.manufacturercenter: OAuth2RefreshTokenGrantConfig
OAuth2 Refresh Token Grant Configs
Fields
- Fields Included from *OAuth2RefreshTokenGrantConfig
- refreshUrl string(default "https://accounts.google.com/o/oauth2/token") - Refresh URL
googleapis.manufacturercenter: Price
A price.
Fields
- amount string? - The numeric value of the price.
- currency string? - The currency in which the price is denoted.
googleapis.manufacturercenter: Product
Product data.
Fields
- attributes Attributes? - Attributes of the product. For more information, see https://support.google.com/manufacturers/answer/6124116.
- contentLanguage string? - The content language of the product as a two-letter ISO 639-1 language code (for example, en).
- destinationStatuses DestinationStatus[]? - The status of the destinations.
- issues Issue[]? - A server-generated list of issues associated with the product.
- name string? - Name in the format
{target_country}:{content_language}:{product_id}
.target_country
- The target country of the product as a CLDR territory code (for example, US).content_language
- The content language of the product as a two-letter ISO 639-1 language code (for example, en).product_id
- The ID of the product. For more information, see https://support.google.com/manufacturers/answer/6124116#id.
- parent string? - Parent ID in the format
accounts/{account_id}
.account_id
- The ID of the Manufacturer Center account.
- productId string? - The ID of the product. For more information, see https://support.google.com/manufacturers/answer/6124116#id.
- targetCountry string? - The target country of the product as a CLDR territory code (for example, US).
googleapis.manufacturercenter: ProductDetail
A product detail of the product. For more information, see https://support.google.com/manufacturers/answer/6124116#productdetail.
Fields
- attributeName string? - The name of the attribute.
- attributeValue string? - The value of the attribute.
- sectionName string? - A short section name that can be reused between multiple product details.
googleapis.manufacturercenter: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
Import
import ballerinax/googleapis.manufacturercenter;
Metadata
Released date: about 2 years ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 6
Current verison: 6
Weekly downloads
Keywords
Commerce/eCommerce
Cost/Free
Vendor/Google
Contributors
Dependencies